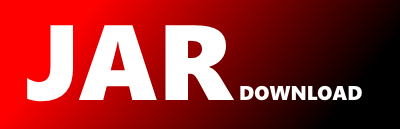
com.volcengine.cdn.model.ModuleLockConfigForDescribeCdnConfigOutput Maven / Gradle / Ivy
/*
* cdn
* No description provided (generated by Swagger Codegen https://github.com/swagger-api/swagger-codegen)
*
* OpenAPI spec version: common-version
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.volcengine.cdn.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.v3.oas.annotations.media.Schema;
import java.io.IOException;
import javax.validation.constraints.*;
import javax.validation.Valid;
/**
* ModuleLockConfigForDescribeCdnConfigOutput
*/
public class ModuleLockConfigForDescribeCdnConfigOutput {
@SerializedName("BrowserCacheLocked")
private Boolean browserCacheLocked = null;
@SerializedName("CacheKeyLocked")
private Boolean cacheKeyLocked = null;
@SerializedName("CacheLocked")
private Boolean cacheLocked = null;
@SerializedName("CompressionLocked")
private Boolean compressionLocked = null;
@SerializedName("CustomizeAccessRuleLocked")
private Boolean customizeAccessRuleLocked = null;
@SerializedName("DownloadSpeedLimitLocked")
private Boolean downloadSpeedLimitLocked = null;
@SerializedName("ErrorPageLocked")
private Boolean errorPageLocked = null;
@SerializedName("IpAccessRuleLocked")
private Boolean ipAccessRuleLocked = null;
@SerializedName("NegativeCacheLocked")
private Boolean negativeCacheLocked = null;
@SerializedName("OriginAccessRuleLocked")
private Boolean originAccessRuleLocked = null;
@SerializedName("OriginLocked")
private Boolean originLocked = null;
@SerializedName("OriginRewriteLocked")
private Boolean originRewriteLocked = null;
@SerializedName("QuicLocked")
private Boolean quicLocked = null;
@SerializedName("RedirectionRewriteLocked")
private Boolean redirectionRewriteLocked = null;
@SerializedName("RefererAccessRuleLocked")
private Boolean refererAccessRuleLocked = null;
@SerializedName("RemoteAuthLocked")
private Boolean remoteAuthLocked = null;
@SerializedName("RequestBlockRuleLocked")
private Boolean requestBlockRuleLocked = null;
@SerializedName("RequestHeaderLocked")
private Boolean requestHeaderLocked = null;
@SerializedName("ResponseHeaderLocked")
private Boolean responseHeaderLocked = null;
@SerializedName("ShareCacheLocked")
private Boolean shareCacheLocked = null;
@SerializedName("SignUrlAuthLocked")
private Boolean signUrlAuthLocked = null;
@SerializedName("UAAccessRuleLocked")
private Boolean uaAccessRuleLocked = null;
public ModuleLockConfigForDescribeCdnConfigOutput browserCacheLocked(Boolean browserCacheLocked) {
this.browserCacheLocked = browserCacheLocked;
return this;
}
/**
* Get browserCacheLocked
* @return browserCacheLocked
**/
@Schema(description = "")
public Boolean isBrowserCacheLocked() {
return browserCacheLocked;
}
public void setBrowserCacheLocked(Boolean browserCacheLocked) {
this.browserCacheLocked = browserCacheLocked;
}
public ModuleLockConfigForDescribeCdnConfigOutput cacheKeyLocked(Boolean cacheKeyLocked) {
this.cacheKeyLocked = cacheKeyLocked;
return this;
}
/**
* Get cacheKeyLocked
* @return cacheKeyLocked
**/
@Schema(description = "")
public Boolean isCacheKeyLocked() {
return cacheKeyLocked;
}
public void setCacheKeyLocked(Boolean cacheKeyLocked) {
this.cacheKeyLocked = cacheKeyLocked;
}
public ModuleLockConfigForDescribeCdnConfigOutput cacheLocked(Boolean cacheLocked) {
this.cacheLocked = cacheLocked;
return this;
}
/**
* Get cacheLocked
* @return cacheLocked
**/
@Schema(description = "")
public Boolean isCacheLocked() {
return cacheLocked;
}
public void setCacheLocked(Boolean cacheLocked) {
this.cacheLocked = cacheLocked;
}
public ModuleLockConfigForDescribeCdnConfigOutput compressionLocked(Boolean compressionLocked) {
this.compressionLocked = compressionLocked;
return this;
}
/**
* Get compressionLocked
* @return compressionLocked
**/
@Schema(description = "")
public Boolean isCompressionLocked() {
return compressionLocked;
}
public void setCompressionLocked(Boolean compressionLocked) {
this.compressionLocked = compressionLocked;
}
public ModuleLockConfigForDescribeCdnConfigOutput customizeAccessRuleLocked(Boolean customizeAccessRuleLocked) {
this.customizeAccessRuleLocked = customizeAccessRuleLocked;
return this;
}
/**
* Get customizeAccessRuleLocked
* @return customizeAccessRuleLocked
**/
@Schema(description = "")
public Boolean isCustomizeAccessRuleLocked() {
return customizeAccessRuleLocked;
}
public void setCustomizeAccessRuleLocked(Boolean customizeAccessRuleLocked) {
this.customizeAccessRuleLocked = customizeAccessRuleLocked;
}
public ModuleLockConfigForDescribeCdnConfigOutput downloadSpeedLimitLocked(Boolean downloadSpeedLimitLocked) {
this.downloadSpeedLimitLocked = downloadSpeedLimitLocked;
return this;
}
/**
* Get downloadSpeedLimitLocked
* @return downloadSpeedLimitLocked
**/
@Schema(description = "")
public Boolean isDownloadSpeedLimitLocked() {
return downloadSpeedLimitLocked;
}
public void setDownloadSpeedLimitLocked(Boolean downloadSpeedLimitLocked) {
this.downloadSpeedLimitLocked = downloadSpeedLimitLocked;
}
public ModuleLockConfigForDescribeCdnConfigOutput errorPageLocked(Boolean errorPageLocked) {
this.errorPageLocked = errorPageLocked;
return this;
}
/**
* Get errorPageLocked
* @return errorPageLocked
**/
@Schema(description = "")
public Boolean isErrorPageLocked() {
return errorPageLocked;
}
public void setErrorPageLocked(Boolean errorPageLocked) {
this.errorPageLocked = errorPageLocked;
}
public ModuleLockConfigForDescribeCdnConfigOutput ipAccessRuleLocked(Boolean ipAccessRuleLocked) {
this.ipAccessRuleLocked = ipAccessRuleLocked;
return this;
}
/**
* Get ipAccessRuleLocked
* @return ipAccessRuleLocked
**/
@Schema(description = "")
public Boolean isIpAccessRuleLocked() {
return ipAccessRuleLocked;
}
public void setIpAccessRuleLocked(Boolean ipAccessRuleLocked) {
this.ipAccessRuleLocked = ipAccessRuleLocked;
}
public ModuleLockConfigForDescribeCdnConfigOutput negativeCacheLocked(Boolean negativeCacheLocked) {
this.negativeCacheLocked = negativeCacheLocked;
return this;
}
/**
* Get negativeCacheLocked
* @return negativeCacheLocked
**/
@Schema(description = "")
public Boolean isNegativeCacheLocked() {
return negativeCacheLocked;
}
public void setNegativeCacheLocked(Boolean negativeCacheLocked) {
this.negativeCacheLocked = negativeCacheLocked;
}
public ModuleLockConfigForDescribeCdnConfigOutput originAccessRuleLocked(Boolean originAccessRuleLocked) {
this.originAccessRuleLocked = originAccessRuleLocked;
return this;
}
/**
* Get originAccessRuleLocked
* @return originAccessRuleLocked
**/
@Schema(description = "")
public Boolean isOriginAccessRuleLocked() {
return originAccessRuleLocked;
}
public void setOriginAccessRuleLocked(Boolean originAccessRuleLocked) {
this.originAccessRuleLocked = originAccessRuleLocked;
}
public ModuleLockConfigForDescribeCdnConfigOutput originLocked(Boolean originLocked) {
this.originLocked = originLocked;
return this;
}
/**
* Get originLocked
* @return originLocked
**/
@Schema(description = "")
public Boolean isOriginLocked() {
return originLocked;
}
public void setOriginLocked(Boolean originLocked) {
this.originLocked = originLocked;
}
public ModuleLockConfigForDescribeCdnConfigOutput originRewriteLocked(Boolean originRewriteLocked) {
this.originRewriteLocked = originRewriteLocked;
return this;
}
/**
* Get originRewriteLocked
* @return originRewriteLocked
**/
@Schema(description = "")
public Boolean isOriginRewriteLocked() {
return originRewriteLocked;
}
public void setOriginRewriteLocked(Boolean originRewriteLocked) {
this.originRewriteLocked = originRewriteLocked;
}
public ModuleLockConfigForDescribeCdnConfigOutput quicLocked(Boolean quicLocked) {
this.quicLocked = quicLocked;
return this;
}
/**
* Get quicLocked
* @return quicLocked
**/
@Schema(description = "")
public Boolean isQuicLocked() {
return quicLocked;
}
public void setQuicLocked(Boolean quicLocked) {
this.quicLocked = quicLocked;
}
public ModuleLockConfigForDescribeCdnConfigOutput redirectionRewriteLocked(Boolean redirectionRewriteLocked) {
this.redirectionRewriteLocked = redirectionRewriteLocked;
return this;
}
/**
* Get redirectionRewriteLocked
* @return redirectionRewriteLocked
**/
@Schema(description = "")
public Boolean isRedirectionRewriteLocked() {
return redirectionRewriteLocked;
}
public void setRedirectionRewriteLocked(Boolean redirectionRewriteLocked) {
this.redirectionRewriteLocked = redirectionRewriteLocked;
}
public ModuleLockConfigForDescribeCdnConfigOutput refererAccessRuleLocked(Boolean refererAccessRuleLocked) {
this.refererAccessRuleLocked = refererAccessRuleLocked;
return this;
}
/**
* Get refererAccessRuleLocked
* @return refererAccessRuleLocked
**/
@Schema(description = "")
public Boolean isRefererAccessRuleLocked() {
return refererAccessRuleLocked;
}
public void setRefererAccessRuleLocked(Boolean refererAccessRuleLocked) {
this.refererAccessRuleLocked = refererAccessRuleLocked;
}
public ModuleLockConfigForDescribeCdnConfigOutput remoteAuthLocked(Boolean remoteAuthLocked) {
this.remoteAuthLocked = remoteAuthLocked;
return this;
}
/**
* Get remoteAuthLocked
* @return remoteAuthLocked
**/
@Schema(description = "")
public Boolean isRemoteAuthLocked() {
return remoteAuthLocked;
}
public void setRemoteAuthLocked(Boolean remoteAuthLocked) {
this.remoteAuthLocked = remoteAuthLocked;
}
public ModuleLockConfigForDescribeCdnConfigOutput requestBlockRuleLocked(Boolean requestBlockRuleLocked) {
this.requestBlockRuleLocked = requestBlockRuleLocked;
return this;
}
/**
* Get requestBlockRuleLocked
* @return requestBlockRuleLocked
**/
@Schema(description = "")
public Boolean isRequestBlockRuleLocked() {
return requestBlockRuleLocked;
}
public void setRequestBlockRuleLocked(Boolean requestBlockRuleLocked) {
this.requestBlockRuleLocked = requestBlockRuleLocked;
}
public ModuleLockConfigForDescribeCdnConfigOutput requestHeaderLocked(Boolean requestHeaderLocked) {
this.requestHeaderLocked = requestHeaderLocked;
return this;
}
/**
* Get requestHeaderLocked
* @return requestHeaderLocked
**/
@Schema(description = "")
public Boolean isRequestHeaderLocked() {
return requestHeaderLocked;
}
public void setRequestHeaderLocked(Boolean requestHeaderLocked) {
this.requestHeaderLocked = requestHeaderLocked;
}
public ModuleLockConfigForDescribeCdnConfigOutput responseHeaderLocked(Boolean responseHeaderLocked) {
this.responseHeaderLocked = responseHeaderLocked;
return this;
}
/**
* Get responseHeaderLocked
* @return responseHeaderLocked
**/
@Schema(description = "")
public Boolean isResponseHeaderLocked() {
return responseHeaderLocked;
}
public void setResponseHeaderLocked(Boolean responseHeaderLocked) {
this.responseHeaderLocked = responseHeaderLocked;
}
public ModuleLockConfigForDescribeCdnConfigOutput shareCacheLocked(Boolean shareCacheLocked) {
this.shareCacheLocked = shareCacheLocked;
return this;
}
/**
* Get shareCacheLocked
* @return shareCacheLocked
**/
@Schema(description = "")
public Boolean isShareCacheLocked() {
return shareCacheLocked;
}
public void setShareCacheLocked(Boolean shareCacheLocked) {
this.shareCacheLocked = shareCacheLocked;
}
public ModuleLockConfigForDescribeCdnConfigOutput signUrlAuthLocked(Boolean signUrlAuthLocked) {
this.signUrlAuthLocked = signUrlAuthLocked;
return this;
}
/**
* Get signUrlAuthLocked
* @return signUrlAuthLocked
**/
@Schema(description = "")
public Boolean isSignUrlAuthLocked() {
return signUrlAuthLocked;
}
public void setSignUrlAuthLocked(Boolean signUrlAuthLocked) {
this.signUrlAuthLocked = signUrlAuthLocked;
}
public ModuleLockConfigForDescribeCdnConfigOutput uaAccessRuleLocked(Boolean uaAccessRuleLocked) {
this.uaAccessRuleLocked = uaAccessRuleLocked;
return this;
}
/**
* Get uaAccessRuleLocked
* @return uaAccessRuleLocked
**/
@Schema(description = "")
public Boolean isUaAccessRuleLocked() {
return uaAccessRuleLocked;
}
public void setUaAccessRuleLocked(Boolean uaAccessRuleLocked) {
this.uaAccessRuleLocked = uaAccessRuleLocked;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ModuleLockConfigForDescribeCdnConfigOutput moduleLockConfigForDescribeCdnConfigOutput = (ModuleLockConfigForDescribeCdnConfigOutput) o;
return Objects.equals(this.browserCacheLocked, moduleLockConfigForDescribeCdnConfigOutput.browserCacheLocked) &&
Objects.equals(this.cacheKeyLocked, moduleLockConfigForDescribeCdnConfigOutput.cacheKeyLocked) &&
Objects.equals(this.cacheLocked, moduleLockConfigForDescribeCdnConfigOutput.cacheLocked) &&
Objects.equals(this.compressionLocked, moduleLockConfigForDescribeCdnConfigOutput.compressionLocked) &&
Objects.equals(this.customizeAccessRuleLocked, moduleLockConfigForDescribeCdnConfigOutput.customizeAccessRuleLocked) &&
Objects.equals(this.downloadSpeedLimitLocked, moduleLockConfigForDescribeCdnConfigOutput.downloadSpeedLimitLocked) &&
Objects.equals(this.errorPageLocked, moduleLockConfigForDescribeCdnConfigOutput.errorPageLocked) &&
Objects.equals(this.ipAccessRuleLocked, moduleLockConfigForDescribeCdnConfigOutput.ipAccessRuleLocked) &&
Objects.equals(this.negativeCacheLocked, moduleLockConfigForDescribeCdnConfigOutput.negativeCacheLocked) &&
Objects.equals(this.originAccessRuleLocked, moduleLockConfigForDescribeCdnConfigOutput.originAccessRuleLocked) &&
Objects.equals(this.originLocked, moduleLockConfigForDescribeCdnConfigOutput.originLocked) &&
Objects.equals(this.originRewriteLocked, moduleLockConfigForDescribeCdnConfigOutput.originRewriteLocked) &&
Objects.equals(this.quicLocked, moduleLockConfigForDescribeCdnConfigOutput.quicLocked) &&
Objects.equals(this.redirectionRewriteLocked, moduleLockConfigForDescribeCdnConfigOutput.redirectionRewriteLocked) &&
Objects.equals(this.refererAccessRuleLocked, moduleLockConfigForDescribeCdnConfigOutput.refererAccessRuleLocked) &&
Objects.equals(this.remoteAuthLocked, moduleLockConfigForDescribeCdnConfigOutput.remoteAuthLocked) &&
Objects.equals(this.requestBlockRuleLocked, moduleLockConfigForDescribeCdnConfigOutput.requestBlockRuleLocked) &&
Objects.equals(this.requestHeaderLocked, moduleLockConfigForDescribeCdnConfigOutput.requestHeaderLocked) &&
Objects.equals(this.responseHeaderLocked, moduleLockConfigForDescribeCdnConfigOutput.responseHeaderLocked) &&
Objects.equals(this.shareCacheLocked, moduleLockConfigForDescribeCdnConfigOutput.shareCacheLocked) &&
Objects.equals(this.signUrlAuthLocked, moduleLockConfigForDescribeCdnConfigOutput.signUrlAuthLocked) &&
Objects.equals(this.uaAccessRuleLocked, moduleLockConfigForDescribeCdnConfigOutput.uaAccessRuleLocked);
}
@Override
public int hashCode() {
return Objects.hash(browserCacheLocked, cacheKeyLocked, cacheLocked, compressionLocked, customizeAccessRuleLocked, downloadSpeedLimitLocked, errorPageLocked, ipAccessRuleLocked, negativeCacheLocked, originAccessRuleLocked, originLocked, originRewriteLocked, quicLocked, redirectionRewriteLocked, refererAccessRuleLocked, remoteAuthLocked, requestBlockRuleLocked, requestHeaderLocked, responseHeaderLocked, shareCacheLocked, signUrlAuthLocked, uaAccessRuleLocked);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ModuleLockConfigForDescribeCdnConfigOutput {\n");
sb.append(" browserCacheLocked: ").append(toIndentedString(browserCacheLocked)).append("\n");
sb.append(" cacheKeyLocked: ").append(toIndentedString(cacheKeyLocked)).append("\n");
sb.append(" cacheLocked: ").append(toIndentedString(cacheLocked)).append("\n");
sb.append(" compressionLocked: ").append(toIndentedString(compressionLocked)).append("\n");
sb.append(" customizeAccessRuleLocked: ").append(toIndentedString(customizeAccessRuleLocked)).append("\n");
sb.append(" downloadSpeedLimitLocked: ").append(toIndentedString(downloadSpeedLimitLocked)).append("\n");
sb.append(" errorPageLocked: ").append(toIndentedString(errorPageLocked)).append("\n");
sb.append(" ipAccessRuleLocked: ").append(toIndentedString(ipAccessRuleLocked)).append("\n");
sb.append(" negativeCacheLocked: ").append(toIndentedString(negativeCacheLocked)).append("\n");
sb.append(" originAccessRuleLocked: ").append(toIndentedString(originAccessRuleLocked)).append("\n");
sb.append(" originLocked: ").append(toIndentedString(originLocked)).append("\n");
sb.append(" originRewriteLocked: ").append(toIndentedString(originRewriteLocked)).append("\n");
sb.append(" quicLocked: ").append(toIndentedString(quicLocked)).append("\n");
sb.append(" redirectionRewriteLocked: ").append(toIndentedString(redirectionRewriteLocked)).append("\n");
sb.append(" refererAccessRuleLocked: ").append(toIndentedString(refererAccessRuleLocked)).append("\n");
sb.append(" remoteAuthLocked: ").append(toIndentedString(remoteAuthLocked)).append("\n");
sb.append(" requestBlockRuleLocked: ").append(toIndentedString(requestBlockRuleLocked)).append("\n");
sb.append(" requestHeaderLocked: ").append(toIndentedString(requestHeaderLocked)).append("\n");
sb.append(" responseHeaderLocked: ").append(toIndentedString(responseHeaderLocked)).append("\n");
sb.append(" shareCacheLocked: ").append(toIndentedString(shareCacheLocked)).append("\n");
sb.append(" signUrlAuthLocked: ").append(toIndentedString(signUrlAuthLocked)).append("\n");
sb.append(" uaAccessRuleLocked: ").append(toIndentedString(uaAccessRuleLocked)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy