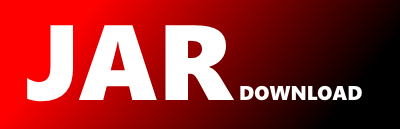
com.volcengine.dms.model.TaskProgressForListDataMigrateTaskOutput Maven / Gradle / Ivy
/*
* dms
* No description provided (generated by Swagger Codegen https://github.com/swagger-api/swagger-codegen)
*
* OpenAPI spec version: common-version
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.volcengine.dms.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.v3.oas.annotations.media.Schema;
import java.io.IOException;
import javax.validation.constraints.*;
import javax.validation.Valid;
/**
* TaskProgressForListDataMigrateTaskOutput
*/
public class TaskProgressForListDataMigrateTaskOutput {
@SerializedName("FailedBytes")
private Long failedBytes = null;
@SerializedName("FailedObjects")
private Long failedObjects = null;
@SerializedName("NotExistBytes")
private Long notExistBytes = null;
@SerializedName("NotExistObjectCount")
private Long notExistObjectCount = null;
@SerializedName("RemainingBytes")
private Long remainingBytes = null;
@SerializedName("RemainingObjects")
private Long remainingObjects = null;
@SerializedName("SkipBytes")
private Long skipBytes = null;
@SerializedName("SkipObjectCount")
private Long skipObjectCount = null;
@SerializedName("TotalBytes")
private Long totalBytes = null;
@SerializedName("TotalObjects")
private Long totalObjects = null;
@SerializedName("TransferBytesSpeed")
private Long transferBytesSpeed = null;
@SerializedName("TransferCountSpeed")
private Long transferCountSpeed = null;
@SerializedName("TransferredBytes")
private Long transferredBytes = null;
@SerializedName("TransferredObjects")
private Long transferredObjects = null;
public TaskProgressForListDataMigrateTaskOutput failedBytes(Long failedBytes) {
this.failedBytes = failedBytes;
return this;
}
/**
* Get failedBytes
* @return failedBytes
**/
@Schema(description = "")
public Long getFailedBytes() {
return failedBytes;
}
public void setFailedBytes(Long failedBytes) {
this.failedBytes = failedBytes;
}
public TaskProgressForListDataMigrateTaskOutput failedObjects(Long failedObjects) {
this.failedObjects = failedObjects;
return this;
}
/**
* Get failedObjects
* @return failedObjects
**/
@Schema(description = "")
public Long getFailedObjects() {
return failedObjects;
}
public void setFailedObjects(Long failedObjects) {
this.failedObjects = failedObjects;
}
public TaskProgressForListDataMigrateTaskOutput notExistBytes(Long notExistBytes) {
this.notExistBytes = notExistBytes;
return this;
}
/**
* Get notExistBytes
* @return notExistBytes
**/
@Schema(description = "")
public Long getNotExistBytes() {
return notExistBytes;
}
public void setNotExistBytes(Long notExistBytes) {
this.notExistBytes = notExistBytes;
}
public TaskProgressForListDataMigrateTaskOutput notExistObjectCount(Long notExistObjectCount) {
this.notExistObjectCount = notExistObjectCount;
return this;
}
/**
* Get notExistObjectCount
* @return notExistObjectCount
**/
@Schema(description = "")
public Long getNotExistObjectCount() {
return notExistObjectCount;
}
public void setNotExistObjectCount(Long notExistObjectCount) {
this.notExistObjectCount = notExistObjectCount;
}
public TaskProgressForListDataMigrateTaskOutput remainingBytes(Long remainingBytes) {
this.remainingBytes = remainingBytes;
return this;
}
/**
* Get remainingBytes
* @return remainingBytes
**/
@Schema(description = "")
public Long getRemainingBytes() {
return remainingBytes;
}
public void setRemainingBytes(Long remainingBytes) {
this.remainingBytes = remainingBytes;
}
public TaskProgressForListDataMigrateTaskOutput remainingObjects(Long remainingObjects) {
this.remainingObjects = remainingObjects;
return this;
}
/**
* Get remainingObjects
* @return remainingObjects
**/
@Schema(description = "")
public Long getRemainingObjects() {
return remainingObjects;
}
public void setRemainingObjects(Long remainingObjects) {
this.remainingObjects = remainingObjects;
}
public TaskProgressForListDataMigrateTaskOutput skipBytes(Long skipBytes) {
this.skipBytes = skipBytes;
return this;
}
/**
* Get skipBytes
* @return skipBytes
**/
@Schema(description = "")
public Long getSkipBytes() {
return skipBytes;
}
public void setSkipBytes(Long skipBytes) {
this.skipBytes = skipBytes;
}
public TaskProgressForListDataMigrateTaskOutput skipObjectCount(Long skipObjectCount) {
this.skipObjectCount = skipObjectCount;
return this;
}
/**
* Get skipObjectCount
* @return skipObjectCount
**/
@Schema(description = "")
public Long getSkipObjectCount() {
return skipObjectCount;
}
public void setSkipObjectCount(Long skipObjectCount) {
this.skipObjectCount = skipObjectCount;
}
public TaskProgressForListDataMigrateTaskOutput totalBytes(Long totalBytes) {
this.totalBytes = totalBytes;
return this;
}
/**
* Get totalBytes
* @return totalBytes
**/
@Schema(description = "")
public Long getTotalBytes() {
return totalBytes;
}
public void setTotalBytes(Long totalBytes) {
this.totalBytes = totalBytes;
}
public TaskProgressForListDataMigrateTaskOutput totalObjects(Long totalObjects) {
this.totalObjects = totalObjects;
return this;
}
/**
* Get totalObjects
* @return totalObjects
**/
@Schema(description = "")
public Long getTotalObjects() {
return totalObjects;
}
public void setTotalObjects(Long totalObjects) {
this.totalObjects = totalObjects;
}
public TaskProgressForListDataMigrateTaskOutput transferBytesSpeed(Long transferBytesSpeed) {
this.transferBytesSpeed = transferBytesSpeed;
return this;
}
/**
* Get transferBytesSpeed
* @return transferBytesSpeed
**/
@Schema(description = "")
public Long getTransferBytesSpeed() {
return transferBytesSpeed;
}
public void setTransferBytesSpeed(Long transferBytesSpeed) {
this.transferBytesSpeed = transferBytesSpeed;
}
public TaskProgressForListDataMigrateTaskOutput transferCountSpeed(Long transferCountSpeed) {
this.transferCountSpeed = transferCountSpeed;
return this;
}
/**
* Get transferCountSpeed
* @return transferCountSpeed
**/
@Schema(description = "")
public Long getTransferCountSpeed() {
return transferCountSpeed;
}
public void setTransferCountSpeed(Long transferCountSpeed) {
this.transferCountSpeed = transferCountSpeed;
}
public TaskProgressForListDataMigrateTaskOutput transferredBytes(Long transferredBytes) {
this.transferredBytes = transferredBytes;
return this;
}
/**
* Get transferredBytes
* @return transferredBytes
**/
@Schema(description = "")
public Long getTransferredBytes() {
return transferredBytes;
}
public void setTransferredBytes(Long transferredBytes) {
this.transferredBytes = transferredBytes;
}
public TaskProgressForListDataMigrateTaskOutput transferredObjects(Long transferredObjects) {
this.transferredObjects = transferredObjects;
return this;
}
/**
* Get transferredObjects
* @return transferredObjects
**/
@Schema(description = "")
public Long getTransferredObjects() {
return transferredObjects;
}
public void setTransferredObjects(Long transferredObjects) {
this.transferredObjects = transferredObjects;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
TaskProgressForListDataMigrateTaskOutput taskProgressForListDataMigrateTaskOutput = (TaskProgressForListDataMigrateTaskOutput) o;
return Objects.equals(this.failedBytes, taskProgressForListDataMigrateTaskOutput.failedBytes) &&
Objects.equals(this.failedObjects, taskProgressForListDataMigrateTaskOutput.failedObjects) &&
Objects.equals(this.notExistBytes, taskProgressForListDataMigrateTaskOutput.notExistBytes) &&
Objects.equals(this.notExistObjectCount, taskProgressForListDataMigrateTaskOutput.notExistObjectCount) &&
Objects.equals(this.remainingBytes, taskProgressForListDataMigrateTaskOutput.remainingBytes) &&
Objects.equals(this.remainingObjects, taskProgressForListDataMigrateTaskOutput.remainingObjects) &&
Objects.equals(this.skipBytes, taskProgressForListDataMigrateTaskOutput.skipBytes) &&
Objects.equals(this.skipObjectCount, taskProgressForListDataMigrateTaskOutput.skipObjectCount) &&
Objects.equals(this.totalBytes, taskProgressForListDataMigrateTaskOutput.totalBytes) &&
Objects.equals(this.totalObjects, taskProgressForListDataMigrateTaskOutput.totalObjects) &&
Objects.equals(this.transferBytesSpeed, taskProgressForListDataMigrateTaskOutput.transferBytesSpeed) &&
Objects.equals(this.transferCountSpeed, taskProgressForListDataMigrateTaskOutput.transferCountSpeed) &&
Objects.equals(this.transferredBytes, taskProgressForListDataMigrateTaskOutput.transferredBytes) &&
Objects.equals(this.transferredObjects, taskProgressForListDataMigrateTaskOutput.transferredObjects);
}
@Override
public int hashCode() {
return Objects.hash(failedBytes, failedObjects, notExistBytes, notExistObjectCount, remainingBytes, remainingObjects, skipBytes, skipObjectCount, totalBytes, totalObjects, transferBytesSpeed, transferCountSpeed, transferredBytes, transferredObjects);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class TaskProgressForListDataMigrateTaskOutput {\n");
sb.append(" failedBytes: ").append(toIndentedString(failedBytes)).append("\n");
sb.append(" failedObjects: ").append(toIndentedString(failedObjects)).append("\n");
sb.append(" notExistBytes: ").append(toIndentedString(notExistBytes)).append("\n");
sb.append(" notExistObjectCount: ").append(toIndentedString(notExistObjectCount)).append("\n");
sb.append(" remainingBytes: ").append(toIndentedString(remainingBytes)).append("\n");
sb.append(" remainingObjects: ").append(toIndentedString(remainingObjects)).append("\n");
sb.append(" skipBytes: ").append(toIndentedString(skipBytes)).append("\n");
sb.append(" skipObjectCount: ").append(toIndentedString(skipObjectCount)).append("\n");
sb.append(" totalBytes: ").append(toIndentedString(totalBytes)).append("\n");
sb.append(" totalObjects: ").append(toIndentedString(totalObjects)).append("\n");
sb.append(" transferBytesSpeed: ").append(toIndentedString(transferBytesSpeed)).append("\n");
sb.append(" transferCountSpeed: ").append(toIndentedString(transferCountSpeed)).append("\n");
sb.append(" transferredBytes: ").append(toIndentedString(transferredBytes)).append("\n");
sb.append(" transferredObjects: ").append(toIndentedString(transferredObjects)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy