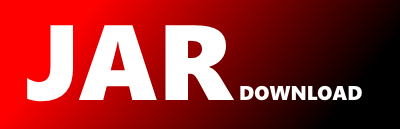
com.volcengine.dns.model.QueryZoneResponse Maven / Gradle / Ivy
/*
* dns
* No description provided (generated by Swagger Codegen https://github.com/swagger-api/swagger-codegen)
*
* OpenAPI spec version: common-version
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.volcengine.dns.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.v3.oas.annotations.media.Schema;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import javax.validation.constraints.*;
import javax.validation.Valid;
/**
* QueryZoneResponse
*/
public class QueryZoneResponse extends com.volcengine.model.AbstractResponse {
@SerializedName("AllocateDNSServerList")
private List allocateDNSServerList = null;
@SerializedName("AutoRenew")
private Boolean autoRenew = null;
@SerializedName("ConfigurationCode")
private String configurationCode = null;
@SerializedName("DnsSecurity")
private String dnsSecurity = null;
@SerializedName("ExpiredTime")
private Long expiredTime = null;
@SerializedName("InstanceNo")
private String instanceNo = null;
@SerializedName("IsNSCorrect")
private Boolean isNSCorrect = null;
@SerializedName("IsSubDomain")
private Boolean isSubDomain = null;
@SerializedName("RealDNSServerList")
private List realDNSServerList = null;
@SerializedName("RecordCount")
private Integer recordCount = null;
@SerializedName("Remark")
private String remark = null;
@SerializedName("Stage")
private Integer stage = null;
@SerializedName("Status")
private Integer status = null;
@SerializedName("SubDomainHost")
private String subDomainHost = null;
@SerializedName("TradeCode")
private String tradeCode = null;
@SerializedName("UpdatedAt")
private String updatedAt = null;
@SerializedName("ZoneName")
private String zoneName = null;
public QueryZoneResponse allocateDNSServerList(List allocateDNSServerList) {
this.allocateDNSServerList = allocateDNSServerList;
return this;
}
public QueryZoneResponse addAllocateDNSServerListItem(String allocateDNSServerListItem) {
if (this.allocateDNSServerList == null) {
this.allocateDNSServerList = new ArrayList();
}
this.allocateDNSServerList.add(allocateDNSServerListItem);
return this;
}
/**
* Get allocateDNSServerList
* @return allocateDNSServerList
**/
@Schema(description = "")
public List getAllocateDNSServerList() {
return allocateDNSServerList;
}
public void setAllocateDNSServerList(List allocateDNSServerList) {
this.allocateDNSServerList = allocateDNSServerList;
}
public QueryZoneResponse autoRenew(Boolean autoRenew) {
this.autoRenew = autoRenew;
return this;
}
/**
* Get autoRenew
* @return autoRenew
**/
@Schema(description = "")
public Boolean isAutoRenew() {
return autoRenew;
}
public void setAutoRenew(Boolean autoRenew) {
this.autoRenew = autoRenew;
}
public QueryZoneResponse configurationCode(String configurationCode) {
this.configurationCode = configurationCode;
return this;
}
/**
* Get configurationCode
* @return configurationCode
**/
@Schema(description = "")
public String getConfigurationCode() {
return configurationCode;
}
public void setConfigurationCode(String configurationCode) {
this.configurationCode = configurationCode;
}
public QueryZoneResponse dnsSecurity(String dnsSecurity) {
this.dnsSecurity = dnsSecurity;
return this;
}
/**
* Get dnsSecurity
* @return dnsSecurity
**/
@Schema(description = "")
public String getDnsSecurity() {
return dnsSecurity;
}
public void setDnsSecurity(String dnsSecurity) {
this.dnsSecurity = dnsSecurity;
}
public QueryZoneResponse expiredTime(Long expiredTime) {
this.expiredTime = expiredTime;
return this;
}
/**
* Get expiredTime
* @return expiredTime
**/
@Schema(description = "")
public Long getExpiredTime() {
return expiredTime;
}
public void setExpiredTime(Long expiredTime) {
this.expiredTime = expiredTime;
}
public QueryZoneResponse instanceNo(String instanceNo) {
this.instanceNo = instanceNo;
return this;
}
/**
* Get instanceNo
* @return instanceNo
**/
@Schema(description = "")
public String getInstanceNo() {
return instanceNo;
}
public void setInstanceNo(String instanceNo) {
this.instanceNo = instanceNo;
}
public QueryZoneResponse isNSCorrect(Boolean isNSCorrect) {
this.isNSCorrect = isNSCorrect;
return this;
}
/**
* Get isNSCorrect
* @return isNSCorrect
**/
@Schema(description = "")
public Boolean isIsNSCorrect() {
return isNSCorrect;
}
public void setIsNSCorrect(Boolean isNSCorrect) {
this.isNSCorrect = isNSCorrect;
}
public QueryZoneResponse isSubDomain(Boolean isSubDomain) {
this.isSubDomain = isSubDomain;
return this;
}
/**
* Get isSubDomain
* @return isSubDomain
**/
@Schema(description = "")
public Boolean isIsSubDomain() {
return isSubDomain;
}
public void setIsSubDomain(Boolean isSubDomain) {
this.isSubDomain = isSubDomain;
}
public QueryZoneResponse realDNSServerList(List realDNSServerList) {
this.realDNSServerList = realDNSServerList;
return this;
}
public QueryZoneResponse addRealDNSServerListItem(String realDNSServerListItem) {
if (this.realDNSServerList == null) {
this.realDNSServerList = new ArrayList();
}
this.realDNSServerList.add(realDNSServerListItem);
return this;
}
/**
* Get realDNSServerList
* @return realDNSServerList
**/
@Schema(description = "")
public List getRealDNSServerList() {
return realDNSServerList;
}
public void setRealDNSServerList(List realDNSServerList) {
this.realDNSServerList = realDNSServerList;
}
public QueryZoneResponse recordCount(Integer recordCount) {
this.recordCount = recordCount;
return this;
}
/**
* Get recordCount
* @return recordCount
**/
@Schema(description = "")
public Integer getRecordCount() {
return recordCount;
}
public void setRecordCount(Integer recordCount) {
this.recordCount = recordCount;
}
public QueryZoneResponse remark(String remark) {
this.remark = remark;
return this;
}
/**
* Get remark
* @return remark
**/
@Schema(description = "")
public String getRemark() {
return remark;
}
public void setRemark(String remark) {
this.remark = remark;
}
public QueryZoneResponse stage(Integer stage) {
this.stage = stage;
return this;
}
/**
* Get stage
* @return stage
**/
@Schema(description = "")
public Integer getStage() {
return stage;
}
public void setStage(Integer stage) {
this.stage = stage;
}
public QueryZoneResponse status(Integer status) {
this.status = status;
return this;
}
/**
* Get status
* @return status
**/
@Schema(description = "")
public Integer getStatus() {
return status;
}
public void setStatus(Integer status) {
this.status = status;
}
public QueryZoneResponse subDomainHost(String subDomainHost) {
this.subDomainHost = subDomainHost;
return this;
}
/**
* Get subDomainHost
* @return subDomainHost
**/
@Schema(description = "")
public String getSubDomainHost() {
return subDomainHost;
}
public void setSubDomainHost(String subDomainHost) {
this.subDomainHost = subDomainHost;
}
public QueryZoneResponse tradeCode(String tradeCode) {
this.tradeCode = tradeCode;
return this;
}
/**
* Get tradeCode
* @return tradeCode
**/
@Schema(description = "")
public String getTradeCode() {
return tradeCode;
}
public void setTradeCode(String tradeCode) {
this.tradeCode = tradeCode;
}
public QueryZoneResponse updatedAt(String updatedAt) {
this.updatedAt = updatedAt;
return this;
}
/**
* Get updatedAt
* @return updatedAt
**/
@Schema(description = "")
public String getUpdatedAt() {
return updatedAt;
}
public void setUpdatedAt(String updatedAt) {
this.updatedAt = updatedAt;
}
public QueryZoneResponse zoneName(String zoneName) {
this.zoneName = zoneName;
return this;
}
/**
* Get zoneName
* @return zoneName
**/
@Schema(description = "")
public String getZoneName() {
return zoneName;
}
public void setZoneName(String zoneName) {
this.zoneName = zoneName;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
QueryZoneResponse queryZoneResponse = (QueryZoneResponse) o;
return Objects.equals(this.allocateDNSServerList, queryZoneResponse.allocateDNSServerList) &&
Objects.equals(this.autoRenew, queryZoneResponse.autoRenew) &&
Objects.equals(this.configurationCode, queryZoneResponse.configurationCode) &&
Objects.equals(this.dnsSecurity, queryZoneResponse.dnsSecurity) &&
Objects.equals(this.expiredTime, queryZoneResponse.expiredTime) &&
Objects.equals(this.instanceNo, queryZoneResponse.instanceNo) &&
Objects.equals(this.isNSCorrect, queryZoneResponse.isNSCorrect) &&
Objects.equals(this.isSubDomain, queryZoneResponse.isSubDomain) &&
Objects.equals(this.realDNSServerList, queryZoneResponse.realDNSServerList) &&
Objects.equals(this.recordCount, queryZoneResponse.recordCount) &&
Objects.equals(this.remark, queryZoneResponse.remark) &&
Objects.equals(this.stage, queryZoneResponse.stage) &&
Objects.equals(this.status, queryZoneResponse.status) &&
Objects.equals(this.subDomainHost, queryZoneResponse.subDomainHost) &&
Objects.equals(this.tradeCode, queryZoneResponse.tradeCode) &&
Objects.equals(this.updatedAt, queryZoneResponse.updatedAt) &&
Objects.equals(this.zoneName, queryZoneResponse.zoneName);
}
@Override
public int hashCode() {
return Objects.hash(allocateDNSServerList, autoRenew, configurationCode, dnsSecurity, expiredTime, instanceNo, isNSCorrect, isSubDomain, realDNSServerList, recordCount, remark, stage, status, subDomainHost, tradeCode, updatedAt, zoneName);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class QueryZoneResponse {\n");
sb.append(" allocateDNSServerList: ").append(toIndentedString(allocateDNSServerList)).append("\n");
sb.append(" autoRenew: ").append(toIndentedString(autoRenew)).append("\n");
sb.append(" configurationCode: ").append(toIndentedString(configurationCode)).append("\n");
sb.append(" dnsSecurity: ").append(toIndentedString(dnsSecurity)).append("\n");
sb.append(" expiredTime: ").append(toIndentedString(expiredTime)).append("\n");
sb.append(" instanceNo: ").append(toIndentedString(instanceNo)).append("\n");
sb.append(" isNSCorrect: ").append(toIndentedString(isNSCorrect)).append("\n");
sb.append(" isSubDomain: ").append(toIndentedString(isSubDomain)).append("\n");
sb.append(" realDNSServerList: ").append(toIndentedString(realDNSServerList)).append("\n");
sb.append(" recordCount: ").append(toIndentedString(recordCount)).append("\n");
sb.append(" remark: ").append(toIndentedString(remark)).append("\n");
sb.append(" stage: ").append(toIndentedString(stage)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" subDomainHost: ").append(toIndentedString(subDomainHost)).append("\n");
sb.append(" tradeCode: ").append(toIndentedString(tradeCode)).append("\n");
sb.append(" updatedAt: ").append(toIndentedString(updatedAt)).append("\n");
sb.append(" zoneName: ").append(toIndentedString(zoneName)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy