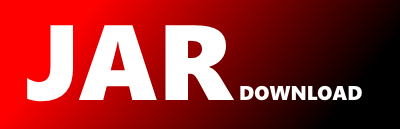
com.volcengine.filenas.model.CreateFileSystemRequest Maven / Gradle / Ivy
/*
* filenas
* No description provided (generated by Swagger Codegen https://github.com/swagger-api/swagger-codegen)
*
* OpenAPI spec version: common-version
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.volcengine.filenas.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.volcengine.filenas.model.TagForCreateFileSystemInput;
import io.swagger.v3.oas.annotations.media.Schema;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import javax.validation.constraints.*;
import javax.validation.Valid;
/**
* CreateFileSystemRequest
*/
public class CreateFileSystemRequest {
@SerializedName("Capacity")
private Integer capacity = null;
/**
* Gets or Sets chargeType
*/
@JsonAdapter(ChargeTypeEnum.Adapter.class)
public enum ChargeTypeEnum {
@SerializedName("PayAsYouGo")
PAYASYOUGO("PayAsYouGo");
private String value;
ChargeTypeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static ChargeTypeEnum fromValue(String input) {
for (ChargeTypeEnum b : ChargeTypeEnum.values()) {
if (b.value.equals(input)) {
return b;
}
}
return null;
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final ChargeTypeEnum enumeration) throws IOException {
jsonWriter.value(String.valueOf(enumeration.getValue()));
}
@Override
public ChargeTypeEnum read(final JsonReader jsonReader) throws IOException {
Object value = jsonReader.nextString();
return ChargeTypeEnum.fromValue((String)(value));
}
}
} @SerializedName("ChargeType")
private ChargeTypeEnum chargeType = null;
@SerializedName("ClientToken")
private String clientToken = null;
@SerializedName("Description")
private String description = null;
@SerializedName("FileSystemName")
private String fileSystemName = null;
/**
* Gets or Sets fileSystemType
*/
@JsonAdapter(FileSystemTypeEnum.Adapter.class)
public enum FileSystemTypeEnum {
@SerializedName("Extreme")
EXTREME("Extreme"),
@SerializedName("Capacity")
CAPACITY("Capacity"),
@SerializedName("Cache")
CACHE("Cache");
private String value;
FileSystemTypeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static FileSystemTypeEnum fromValue(String input) {
for (FileSystemTypeEnum b : FileSystemTypeEnum.values()) {
if (b.value.equals(input)) {
return b;
}
}
return null;
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final FileSystemTypeEnum enumeration) throws IOException {
jsonWriter.value(String.valueOf(enumeration.getValue()));
}
@Override
public FileSystemTypeEnum read(final JsonReader jsonReader) throws IOException {
Object value = jsonReader.nextString();
return FileSystemTypeEnum.fromValue((String)(value));
}
}
} @SerializedName("FileSystemType")
private FileSystemTypeEnum fileSystemType = null;
@SerializedName("ProjectName")
private String projectName = null;
/**
* Gets or Sets protocolType
*/
@JsonAdapter(ProtocolTypeEnum.Adapter.class)
public enum ProtocolTypeEnum {
@SerializedName("NFS")
NFS("NFS");
private String value;
ProtocolTypeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static ProtocolTypeEnum fromValue(String input) {
for (ProtocolTypeEnum b : ProtocolTypeEnum.values()) {
if (b.value.equals(input)) {
return b;
}
}
return null;
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final ProtocolTypeEnum enumeration) throws IOException {
jsonWriter.value(String.valueOf(enumeration.getValue()));
}
@Override
public ProtocolTypeEnum read(final JsonReader jsonReader) throws IOException {
Object value = jsonReader.nextString();
return ProtocolTypeEnum.fromValue((String)(value));
}
}
} @SerializedName("ProtocolType")
private ProtocolTypeEnum protocolType = null;
@SerializedName("SnapshotId")
private String snapshotId = null;
/**
* Gets or Sets storageType
*/
@JsonAdapter(StorageTypeEnum.Adapter.class)
public enum StorageTypeEnum {
@SerializedName("Standard")
STANDARD("Standard");
private String value;
StorageTypeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static StorageTypeEnum fromValue(String input) {
for (StorageTypeEnum b : StorageTypeEnum.values()) {
if (b.value.equals(input)) {
return b;
}
}
return null;
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final StorageTypeEnum enumeration) throws IOException {
jsonWriter.value(String.valueOf(enumeration.getValue()));
}
@Override
public StorageTypeEnum read(final JsonReader jsonReader) throws IOException {
Object value = jsonReader.nextString();
return StorageTypeEnum.fromValue((String)(value));
}
}
} @SerializedName("StorageType")
private StorageTypeEnum storageType = null;
@SerializedName("Tags")
private List tags = null;
@SerializedName("ZoneId")
private String zoneId = null;
public CreateFileSystemRequest capacity(Integer capacity) {
this.capacity = capacity;
return this;
}
/**
* Get capacity
* @return capacity
**/
@NotNull
@Schema(required = true, description = "")
public Integer getCapacity() {
return capacity;
}
public void setCapacity(Integer capacity) {
this.capacity = capacity;
}
public CreateFileSystemRequest chargeType(ChargeTypeEnum chargeType) {
this.chargeType = chargeType;
return this;
}
/**
* Get chargeType
* @return chargeType
**/
@NotNull
@Schema(required = true, description = "")
public ChargeTypeEnum getChargeType() {
return chargeType;
}
public void setChargeType(ChargeTypeEnum chargeType) {
this.chargeType = chargeType;
}
public CreateFileSystemRequest clientToken(String clientToken) {
this.clientToken = clientToken;
return this;
}
/**
* Get clientToken
* @return clientToken
**/
@Schema(description = "")
public String getClientToken() {
return clientToken;
}
public void setClientToken(String clientToken) {
this.clientToken = clientToken;
}
public CreateFileSystemRequest description(String description) {
this.description = description;
return this;
}
/**
* Get description
* @return description
**/
@Size(max=120) @Schema(description = "")
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public CreateFileSystemRequest fileSystemName(String fileSystemName) {
this.fileSystemName = fileSystemName;
return this;
}
/**
* Get fileSystemName
* @return fileSystemName
**/
@NotNull
@Size(min=1,max=128) @Schema(required = true, description = "")
public String getFileSystemName() {
return fileSystemName;
}
public void setFileSystemName(String fileSystemName) {
this.fileSystemName = fileSystemName;
}
public CreateFileSystemRequest fileSystemType(FileSystemTypeEnum fileSystemType) {
this.fileSystemType = fileSystemType;
return this;
}
/**
* Get fileSystemType
* @return fileSystemType
**/
@NotNull
@Schema(required = true, description = "")
public FileSystemTypeEnum getFileSystemType() {
return fileSystemType;
}
public void setFileSystemType(FileSystemTypeEnum fileSystemType) {
this.fileSystemType = fileSystemType;
}
public CreateFileSystemRequest projectName(String projectName) {
this.projectName = projectName;
return this;
}
/**
* Get projectName
* @return projectName
**/
@Schema(description = "")
public String getProjectName() {
return projectName;
}
public void setProjectName(String projectName) {
this.projectName = projectName;
}
public CreateFileSystemRequest protocolType(ProtocolTypeEnum protocolType) {
this.protocolType = protocolType;
return this;
}
/**
* Get protocolType
* @return protocolType
**/
@NotNull
@Schema(required = true, description = "")
public ProtocolTypeEnum getProtocolType() {
return protocolType;
}
public void setProtocolType(ProtocolTypeEnum protocolType) {
this.protocolType = protocolType;
}
public CreateFileSystemRequest snapshotId(String snapshotId) {
this.snapshotId = snapshotId;
return this;
}
/**
* Get snapshotId
* @return snapshotId
**/
@Schema(description = "")
public String getSnapshotId() {
return snapshotId;
}
public void setSnapshotId(String snapshotId) {
this.snapshotId = snapshotId;
}
public CreateFileSystemRequest storageType(StorageTypeEnum storageType) {
this.storageType = storageType;
return this;
}
/**
* Get storageType
* @return storageType
**/
@Schema(description = "")
public StorageTypeEnum getStorageType() {
return storageType;
}
public void setStorageType(StorageTypeEnum storageType) {
this.storageType = storageType;
}
public CreateFileSystemRequest tags(List tags) {
this.tags = tags;
return this;
}
public CreateFileSystemRequest addTagsItem(TagForCreateFileSystemInput tagsItem) {
if (this.tags == null) {
this.tags = new ArrayList();
}
this.tags.add(tagsItem);
return this;
}
/**
* Get tags
* @return tags
**/
@Valid
@Schema(description = "")
public List getTags() {
return tags;
}
public void setTags(List tags) {
this.tags = tags;
}
public CreateFileSystemRequest zoneId(String zoneId) {
this.zoneId = zoneId;
return this;
}
/**
* Get zoneId
* @return zoneId
**/
@NotNull
@Schema(required = true, description = "")
public String getZoneId() {
return zoneId;
}
public void setZoneId(String zoneId) {
this.zoneId = zoneId;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CreateFileSystemRequest createFileSystemRequest = (CreateFileSystemRequest) o;
return Objects.equals(this.capacity, createFileSystemRequest.capacity) &&
Objects.equals(this.chargeType, createFileSystemRequest.chargeType) &&
Objects.equals(this.clientToken, createFileSystemRequest.clientToken) &&
Objects.equals(this.description, createFileSystemRequest.description) &&
Objects.equals(this.fileSystemName, createFileSystemRequest.fileSystemName) &&
Objects.equals(this.fileSystemType, createFileSystemRequest.fileSystemType) &&
Objects.equals(this.projectName, createFileSystemRequest.projectName) &&
Objects.equals(this.protocolType, createFileSystemRequest.protocolType) &&
Objects.equals(this.snapshotId, createFileSystemRequest.snapshotId) &&
Objects.equals(this.storageType, createFileSystemRequest.storageType) &&
Objects.equals(this.tags, createFileSystemRequest.tags) &&
Objects.equals(this.zoneId, createFileSystemRequest.zoneId);
}
@Override
public int hashCode() {
return Objects.hash(capacity, chargeType, clientToken, description, fileSystemName, fileSystemType, projectName, protocolType, snapshotId, storageType, tags, zoneId);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CreateFileSystemRequest {\n");
sb.append(" capacity: ").append(toIndentedString(capacity)).append("\n");
sb.append(" chargeType: ").append(toIndentedString(chargeType)).append("\n");
sb.append(" clientToken: ").append(toIndentedString(clientToken)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" fileSystemName: ").append(toIndentedString(fileSystemName)).append("\n");
sb.append(" fileSystemType: ").append(toIndentedString(fileSystemType)).append("\n");
sb.append(" projectName: ").append(toIndentedString(projectName)).append("\n");
sb.append(" protocolType: ").append(toIndentedString(protocolType)).append("\n");
sb.append(" snapshotId: ").append(toIndentedString(snapshotId)).append("\n");
sb.append(" storageType: ").append(toIndentedString(storageType)).append("\n");
sb.append(" tags: ").append(toIndentedString(tags)).append("\n");
sb.append(" zoneId: ").append(toIndentedString(zoneId)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy