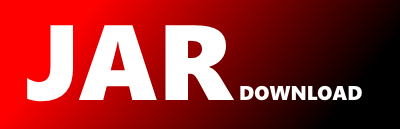
com.volcengine.filenas.model.SaleForDescribeZonesOutput Maven / Gradle / Ivy
/*
* filenas
* No description provided (generated by Swagger Codegen https://github.com/swagger-api/swagger-codegen)
*
* OpenAPI spec version: common-version
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.volcengine.filenas.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.v3.oas.annotations.media.Schema;
import java.io.IOException;
import javax.validation.constraints.*;
import javax.validation.Valid;
/**
* SaleForDescribeZonesOutput
*/
public class SaleForDescribeZonesOutput {
/**
* Gets or Sets fileSystemType
*/
@JsonAdapter(FileSystemTypeEnum.Adapter.class)
public enum FileSystemTypeEnum {
@SerializedName("Extreme")
EXTREME("Extreme"),
@SerializedName("Common")
COMMON("Common"),
@SerializedName("Cache")
CACHE("Cache");
private String value;
FileSystemTypeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static FileSystemTypeEnum fromValue(String input) {
for (FileSystemTypeEnum b : FileSystemTypeEnum.values()) {
if (b.value.equals(input)) {
return b;
}
}
return null;
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final FileSystemTypeEnum enumeration) throws IOException {
jsonWriter.value(String.valueOf(enumeration.getValue()));
}
@Override
public FileSystemTypeEnum read(final JsonReader jsonReader) throws IOException {
Object value = jsonReader.nextString();
return FileSystemTypeEnum.fromValue((String)(value));
}
}
} @SerializedName("FileSystemType")
private FileSystemTypeEnum fileSystemType = null;
/**
* Gets or Sets protocolType
*/
@JsonAdapter(ProtocolTypeEnum.Adapter.class)
public enum ProtocolTypeEnum {
@SerializedName("NFS")
NFS("NFS"),
@SerializedName("SMB")
SMB("SMB");
private String value;
ProtocolTypeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static ProtocolTypeEnum fromValue(String input) {
for (ProtocolTypeEnum b : ProtocolTypeEnum.values()) {
if (b.value.equals(input)) {
return b;
}
}
return null;
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final ProtocolTypeEnum enumeration) throws IOException {
jsonWriter.value(String.valueOf(enumeration.getValue()));
}
@Override
public ProtocolTypeEnum read(final JsonReader jsonReader) throws IOException {
Object value = jsonReader.nextString();
return ProtocolTypeEnum.fromValue((String)(value));
}
}
} @SerializedName("ProtocolType")
private ProtocolTypeEnum protocolType = null;
/**
* Gets or Sets status
*/
@JsonAdapter(StatusEnum.Adapter.class)
public enum StatusEnum {
@SerializedName("OnSale")
ONSALE("OnSale"),
@SerializedName("SoldOut")
SOLDOUT("SoldOut"),
@SerializedName("UnSold")
UNSOLD("UnSold");
private String value;
StatusEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static StatusEnum fromValue(String input) {
for (StatusEnum b : StatusEnum.values()) {
if (b.value.equals(input)) {
return b;
}
}
return null;
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final StatusEnum enumeration) throws IOException {
jsonWriter.value(String.valueOf(enumeration.getValue()));
}
@Override
public StatusEnum read(final JsonReader jsonReader) throws IOException {
Object value = jsonReader.nextString();
return StatusEnum.fromValue((String)(value));
}
}
} @SerializedName("Status")
private StatusEnum status = null;
@SerializedName("StorageType")
private String storageType = null;
public SaleForDescribeZonesOutput fileSystemType(FileSystemTypeEnum fileSystemType) {
this.fileSystemType = fileSystemType;
return this;
}
/**
* Get fileSystemType
* @return fileSystemType
**/
@Schema(description = "")
public FileSystemTypeEnum getFileSystemType() {
return fileSystemType;
}
public void setFileSystemType(FileSystemTypeEnum fileSystemType) {
this.fileSystemType = fileSystemType;
}
public SaleForDescribeZonesOutput protocolType(ProtocolTypeEnum protocolType) {
this.protocolType = protocolType;
return this;
}
/**
* Get protocolType
* @return protocolType
**/
@Schema(description = "")
public ProtocolTypeEnum getProtocolType() {
return protocolType;
}
public void setProtocolType(ProtocolTypeEnum protocolType) {
this.protocolType = protocolType;
}
public SaleForDescribeZonesOutput status(StatusEnum status) {
this.status = status;
return this;
}
/**
* Get status
* @return status
**/
@Schema(description = "")
public StatusEnum getStatus() {
return status;
}
public void setStatus(StatusEnum status) {
this.status = status;
}
public SaleForDescribeZonesOutput storageType(String storageType) {
this.storageType = storageType;
return this;
}
/**
* Get storageType
* @return storageType
**/
@Schema(description = "")
public String getStorageType() {
return storageType;
}
public void setStorageType(String storageType) {
this.storageType = storageType;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SaleForDescribeZonesOutput saleForDescribeZonesOutput = (SaleForDescribeZonesOutput) o;
return Objects.equals(this.fileSystemType, saleForDescribeZonesOutput.fileSystemType) &&
Objects.equals(this.protocolType, saleForDescribeZonesOutput.protocolType) &&
Objects.equals(this.status, saleForDescribeZonesOutput.status) &&
Objects.equals(this.storageType, saleForDescribeZonesOutput.storageType);
}
@Override
public int hashCode() {
return Objects.hash(fileSystemType, protocolType, status, storageType);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class SaleForDescribeZonesOutput {\n");
sb.append(" fileSystemType: ").append(toIndentedString(fileSystemType)).append("\n");
sb.append(" protocolType: ").append(toIndentedString(protocolType)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" storageType: ").append(toIndentedString(storageType)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy