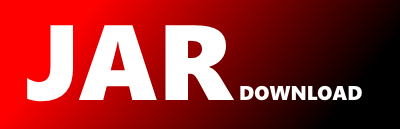
com.volcengine.fwcenter.model.DataForAssetListOutput Maven / Gradle / Ivy
/*
* fwcenter
* No description provided (generated by Swagger Codegen https://github.com/swagger-api/swagger-codegen)
*
* OpenAPI spec version: common-version
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.volcengine.fwcenter.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.v3.oas.annotations.media.Schema;
import java.io.IOException;
import javax.validation.constraints.*;
import javax.validation.Valid;
/**
* DataForAssetListOutput
*/
public class DataForAssetListOutput {
@SerializedName("AccountID")
private String accountID = null;
@SerializedName("InstanceID")
private String instanceID = null;
@SerializedName("eip_id")
private String eipId = null;
@SerializedName("enable")
private Boolean enable = null;
@SerializedName("ip")
private String ip = null;
@SerializedName("latest_7_days_peak_traffic")
private Integer latest7DaysPeakTraffic = null;
@SerializedName("name")
private String name = null;
@SerializedName("region")
private String region = null;
@SerializedName("regionN")
private String regionN = null;
@SerializedName("type")
private String type = null;
public DataForAssetListOutput accountID(String accountID) {
this.accountID = accountID;
return this;
}
/**
* Get accountID
* @return accountID
**/
@Schema(description = "")
public String getAccountID() {
return accountID;
}
public void setAccountID(String accountID) {
this.accountID = accountID;
}
public DataForAssetListOutput instanceID(String instanceID) {
this.instanceID = instanceID;
return this;
}
/**
* Get instanceID
* @return instanceID
**/
@Schema(description = "")
public String getInstanceID() {
return instanceID;
}
public void setInstanceID(String instanceID) {
this.instanceID = instanceID;
}
public DataForAssetListOutput eipId(String eipId) {
this.eipId = eipId;
return this;
}
/**
* Get eipId
* @return eipId
**/
@Schema(description = "")
public String getEipId() {
return eipId;
}
public void setEipId(String eipId) {
this.eipId = eipId;
}
public DataForAssetListOutput enable(Boolean enable) {
this.enable = enable;
return this;
}
/**
* Get enable
* @return enable
**/
@Schema(description = "")
public Boolean isEnable() {
return enable;
}
public void setEnable(Boolean enable) {
this.enable = enable;
}
public DataForAssetListOutput ip(String ip) {
this.ip = ip;
return this;
}
/**
* Get ip
* @return ip
**/
@Schema(description = "")
public String getIp() {
return ip;
}
public void setIp(String ip) {
this.ip = ip;
}
public DataForAssetListOutput latest7DaysPeakTraffic(Integer latest7DaysPeakTraffic) {
this.latest7DaysPeakTraffic = latest7DaysPeakTraffic;
return this;
}
/**
* Get latest7DaysPeakTraffic
* @return latest7DaysPeakTraffic
**/
@Schema(description = "")
public Integer getLatest7DaysPeakTraffic() {
return latest7DaysPeakTraffic;
}
public void setLatest7DaysPeakTraffic(Integer latest7DaysPeakTraffic) {
this.latest7DaysPeakTraffic = latest7DaysPeakTraffic;
}
public DataForAssetListOutput name(String name) {
this.name = name;
return this;
}
/**
* Get name
* @return name
**/
@Schema(description = "")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public DataForAssetListOutput region(String region) {
this.region = region;
return this;
}
/**
* Get region
* @return region
**/
@Schema(description = "")
public String getRegion() {
return region;
}
public void setRegion(String region) {
this.region = region;
}
public DataForAssetListOutput regionN(String regionN) {
this.regionN = regionN;
return this;
}
/**
* Get regionN
* @return regionN
**/
@Schema(description = "")
public String getRegionN() {
return regionN;
}
public void setRegionN(String regionN) {
this.regionN = regionN;
}
public DataForAssetListOutput type(String type) {
this.type = type;
return this;
}
/**
* Get type
* @return type
**/
@Schema(description = "")
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
DataForAssetListOutput dataForAssetListOutput = (DataForAssetListOutput) o;
return Objects.equals(this.accountID, dataForAssetListOutput.accountID) &&
Objects.equals(this.instanceID, dataForAssetListOutput.instanceID) &&
Objects.equals(this.eipId, dataForAssetListOutput.eipId) &&
Objects.equals(this.enable, dataForAssetListOutput.enable) &&
Objects.equals(this.ip, dataForAssetListOutput.ip) &&
Objects.equals(this.latest7DaysPeakTraffic, dataForAssetListOutput.latest7DaysPeakTraffic) &&
Objects.equals(this.name, dataForAssetListOutput.name) &&
Objects.equals(this.region, dataForAssetListOutput.region) &&
Objects.equals(this.regionN, dataForAssetListOutput.regionN) &&
Objects.equals(this.type, dataForAssetListOutput.type);
}
@Override
public int hashCode() {
return Objects.hash(accountID, instanceID, eipId, enable, ip, latest7DaysPeakTraffic, name, region, regionN, type);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class DataForAssetListOutput {\n");
sb.append(" accountID: ").append(toIndentedString(accountID)).append("\n");
sb.append(" instanceID: ").append(toIndentedString(instanceID)).append("\n");
sb.append(" eipId: ").append(toIndentedString(eipId)).append("\n");
sb.append(" enable: ").append(toIndentedString(enable)).append("\n");
sb.append(" ip: ").append(toIndentedString(ip)).append("\n");
sb.append(" latest7DaysPeakTraffic: ").append(toIndentedString(latest7DaysPeakTraffic)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" region: ").append(toIndentedString(region)).append("\n");
sb.append(" regionN: ").append(toIndentedString(regionN)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy