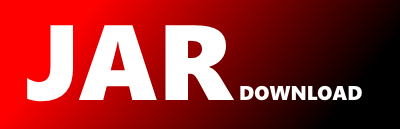
com.volcengine.vefaas.model.GetFunctionResponse Maven / Gradle / Ivy
/*
* vefaas
* No description provided (generated by Swagger Codegen https://github.com/swagger-api/swagger-codegen)
*
* OpenAPI spec version: common-version
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.volcengine.vefaas.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.volcengine.vefaas.model.EnvForGetFunctionOutput;
import com.volcengine.vefaas.model.NasStorageForGetFunctionOutput;
import com.volcengine.vefaas.model.TlsConfigForGetFunctionOutput;
import com.volcengine.vefaas.model.TosMountConfigForGetFunctionOutput;
import com.volcengine.vefaas.model.VpcConfigForGetFunctionOutput;
import io.swagger.v3.oas.annotations.media.Schema;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import javax.validation.constraints.*;
import javax.validation.Valid;
/**
* GetFunctionResponse
*/
public class GetFunctionResponse extends com.volcengine.model.AbstractResponse {
@SerializedName("CodeSize")
private Integer codeSize = null;
@SerializedName("CodeSizeLimit")
private Integer codeSizeLimit = null;
@SerializedName("Command")
private String command = null;
@SerializedName("CreationTime")
private String creationTime = null;
@SerializedName("Description")
private String description = null;
@SerializedName("Envs")
private List envs = null;
@SerializedName("ExclusiveMode")
private Boolean exclusiveMode = null;
@SerializedName("Id")
private String id = null;
@SerializedName("InitializerSec")
private Integer initializerSec = null;
@SerializedName("InstanceType")
private String instanceType = null;
@SerializedName("LastUpdateTime")
private String lastUpdateTime = null;
@SerializedName("MaxConcurrency")
private Integer maxConcurrency = null;
@SerializedName("MemoryMB")
private Integer memoryMB = null;
@SerializedName("Name")
private String name = null;
@SerializedName("NasStorage")
private NasStorageForGetFunctionOutput nasStorage = null;
@SerializedName("Owner")
private String owner = null;
@SerializedName("ProjectName")
private String projectName = null;
@SerializedName("RequestTimeout")
private Integer requestTimeout = null;
@SerializedName("Runtime")
private String runtime = null;
@SerializedName("SourceLocation")
private String sourceLocation = null;
@SerializedName("SourceType")
private String sourceType = null;
@SerializedName("TlsConfig")
private TlsConfigForGetFunctionOutput tlsConfig = null;
@SerializedName("TosMountConfig")
private TosMountConfigForGetFunctionOutput tosMountConfig = null;
@SerializedName("TriggersCount")
private Integer triggersCount = null;
@SerializedName("VpcConfig")
private VpcConfigForGetFunctionOutput vpcConfig = null;
public GetFunctionResponse codeSize(Integer codeSize) {
this.codeSize = codeSize;
return this;
}
/**
* Get codeSize
* @return codeSize
**/
@Schema(description = "")
public Integer getCodeSize() {
return codeSize;
}
public void setCodeSize(Integer codeSize) {
this.codeSize = codeSize;
}
public GetFunctionResponse codeSizeLimit(Integer codeSizeLimit) {
this.codeSizeLimit = codeSizeLimit;
return this;
}
/**
* Get codeSizeLimit
* @return codeSizeLimit
**/
@Schema(description = "")
public Integer getCodeSizeLimit() {
return codeSizeLimit;
}
public void setCodeSizeLimit(Integer codeSizeLimit) {
this.codeSizeLimit = codeSizeLimit;
}
public GetFunctionResponse command(String command) {
this.command = command;
return this;
}
/**
* Get command
* @return command
**/
@Schema(description = "")
public String getCommand() {
return command;
}
public void setCommand(String command) {
this.command = command;
}
public GetFunctionResponse creationTime(String creationTime) {
this.creationTime = creationTime;
return this;
}
/**
* Get creationTime
* @return creationTime
**/
@Schema(description = "")
public String getCreationTime() {
return creationTime;
}
public void setCreationTime(String creationTime) {
this.creationTime = creationTime;
}
public GetFunctionResponse description(String description) {
this.description = description;
return this;
}
/**
* Get description
* @return description
**/
@Schema(description = "")
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public GetFunctionResponse envs(List envs) {
this.envs = envs;
return this;
}
public GetFunctionResponse addEnvsItem(EnvForGetFunctionOutput envsItem) {
if (this.envs == null) {
this.envs = new ArrayList();
}
this.envs.add(envsItem);
return this;
}
/**
* Get envs
* @return envs
**/
@Valid
@Schema(description = "")
public List getEnvs() {
return envs;
}
public void setEnvs(List envs) {
this.envs = envs;
}
public GetFunctionResponse exclusiveMode(Boolean exclusiveMode) {
this.exclusiveMode = exclusiveMode;
return this;
}
/**
* Get exclusiveMode
* @return exclusiveMode
**/
@Schema(description = "")
public Boolean isExclusiveMode() {
return exclusiveMode;
}
public void setExclusiveMode(Boolean exclusiveMode) {
this.exclusiveMode = exclusiveMode;
}
public GetFunctionResponse id(String id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
**/
@Schema(description = "")
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public GetFunctionResponse initializerSec(Integer initializerSec) {
this.initializerSec = initializerSec;
return this;
}
/**
* Get initializerSec
* @return initializerSec
**/
@Schema(description = "")
public Integer getInitializerSec() {
return initializerSec;
}
public void setInitializerSec(Integer initializerSec) {
this.initializerSec = initializerSec;
}
public GetFunctionResponse instanceType(String instanceType) {
this.instanceType = instanceType;
return this;
}
/**
* Get instanceType
* @return instanceType
**/
@Schema(description = "")
public String getInstanceType() {
return instanceType;
}
public void setInstanceType(String instanceType) {
this.instanceType = instanceType;
}
public GetFunctionResponse lastUpdateTime(String lastUpdateTime) {
this.lastUpdateTime = lastUpdateTime;
return this;
}
/**
* Get lastUpdateTime
* @return lastUpdateTime
**/
@Schema(description = "")
public String getLastUpdateTime() {
return lastUpdateTime;
}
public void setLastUpdateTime(String lastUpdateTime) {
this.lastUpdateTime = lastUpdateTime;
}
public GetFunctionResponse maxConcurrency(Integer maxConcurrency) {
this.maxConcurrency = maxConcurrency;
return this;
}
/**
* Get maxConcurrency
* @return maxConcurrency
**/
@Schema(description = "")
public Integer getMaxConcurrency() {
return maxConcurrency;
}
public void setMaxConcurrency(Integer maxConcurrency) {
this.maxConcurrency = maxConcurrency;
}
public GetFunctionResponse memoryMB(Integer memoryMB) {
this.memoryMB = memoryMB;
return this;
}
/**
* Get memoryMB
* @return memoryMB
**/
@Schema(description = "")
public Integer getMemoryMB() {
return memoryMB;
}
public void setMemoryMB(Integer memoryMB) {
this.memoryMB = memoryMB;
}
public GetFunctionResponse name(String name) {
this.name = name;
return this;
}
/**
* Get name
* @return name
**/
@Schema(description = "")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public GetFunctionResponse nasStorage(NasStorageForGetFunctionOutput nasStorage) {
this.nasStorage = nasStorage;
return this;
}
/**
* Get nasStorage
* @return nasStorage
**/
@Valid
@Schema(description = "")
public NasStorageForGetFunctionOutput getNasStorage() {
return nasStorage;
}
public void setNasStorage(NasStorageForGetFunctionOutput nasStorage) {
this.nasStorage = nasStorage;
}
public GetFunctionResponse owner(String owner) {
this.owner = owner;
return this;
}
/**
* Get owner
* @return owner
**/
@Schema(description = "")
public String getOwner() {
return owner;
}
public void setOwner(String owner) {
this.owner = owner;
}
public GetFunctionResponse projectName(String projectName) {
this.projectName = projectName;
return this;
}
/**
* Get projectName
* @return projectName
**/
@Schema(description = "")
public String getProjectName() {
return projectName;
}
public void setProjectName(String projectName) {
this.projectName = projectName;
}
public GetFunctionResponse requestTimeout(Integer requestTimeout) {
this.requestTimeout = requestTimeout;
return this;
}
/**
* Get requestTimeout
* @return requestTimeout
**/
@Schema(description = "")
public Integer getRequestTimeout() {
return requestTimeout;
}
public void setRequestTimeout(Integer requestTimeout) {
this.requestTimeout = requestTimeout;
}
public GetFunctionResponse runtime(String runtime) {
this.runtime = runtime;
return this;
}
/**
* Get runtime
* @return runtime
**/
@Schema(description = "")
public String getRuntime() {
return runtime;
}
public void setRuntime(String runtime) {
this.runtime = runtime;
}
public GetFunctionResponse sourceLocation(String sourceLocation) {
this.sourceLocation = sourceLocation;
return this;
}
/**
* Get sourceLocation
* @return sourceLocation
**/
@Schema(description = "")
public String getSourceLocation() {
return sourceLocation;
}
public void setSourceLocation(String sourceLocation) {
this.sourceLocation = sourceLocation;
}
public GetFunctionResponse sourceType(String sourceType) {
this.sourceType = sourceType;
return this;
}
/**
* Get sourceType
* @return sourceType
**/
@Schema(description = "")
public String getSourceType() {
return sourceType;
}
public void setSourceType(String sourceType) {
this.sourceType = sourceType;
}
public GetFunctionResponse tlsConfig(TlsConfigForGetFunctionOutput tlsConfig) {
this.tlsConfig = tlsConfig;
return this;
}
/**
* Get tlsConfig
* @return tlsConfig
**/
@Valid
@Schema(description = "")
public TlsConfigForGetFunctionOutput getTlsConfig() {
return tlsConfig;
}
public void setTlsConfig(TlsConfigForGetFunctionOutput tlsConfig) {
this.tlsConfig = tlsConfig;
}
public GetFunctionResponse tosMountConfig(TosMountConfigForGetFunctionOutput tosMountConfig) {
this.tosMountConfig = tosMountConfig;
return this;
}
/**
* Get tosMountConfig
* @return tosMountConfig
**/
@Valid
@Schema(description = "")
public TosMountConfigForGetFunctionOutput getTosMountConfig() {
return tosMountConfig;
}
public void setTosMountConfig(TosMountConfigForGetFunctionOutput tosMountConfig) {
this.tosMountConfig = tosMountConfig;
}
public GetFunctionResponse triggersCount(Integer triggersCount) {
this.triggersCount = triggersCount;
return this;
}
/**
* Get triggersCount
* @return triggersCount
**/
@Schema(description = "")
public Integer getTriggersCount() {
return triggersCount;
}
public void setTriggersCount(Integer triggersCount) {
this.triggersCount = triggersCount;
}
public GetFunctionResponse vpcConfig(VpcConfigForGetFunctionOutput vpcConfig) {
this.vpcConfig = vpcConfig;
return this;
}
/**
* Get vpcConfig
* @return vpcConfig
**/
@Valid
@Schema(description = "")
public VpcConfigForGetFunctionOutput getVpcConfig() {
return vpcConfig;
}
public void setVpcConfig(VpcConfigForGetFunctionOutput vpcConfig) {
this.vpcConfig = vpcConfig;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
GetFunctionResponse getFunctionResponse = (GetFunctionResponse) o;
return Objects.equals(this.codeSize, getFunctionResponse.codeSize) &&
Objects.equals(this.codeSizeLimit, getFunctionResponse.codeSizeLimit) &&
Objects.equals(this.command, getFunctionResponse.command) &&
Objects.equals(this.creationTime, getFunctionResponse.creationTime) &&
Objects.equals(this.description, getFunctionResponse.description) &&
Objects.equals(this.envs, getFunctionResponse.envs) &&
Objects.equals(this.exclusiveMode, getFunctionResponse.exclusiveMode) &&
Objects.equals(this.id, getFunctionResponse.id) &&
Objects.equals(this.initializerSec, getFunctionResponse.initializerSec) &&
Objects.equals(this.instanceType, getFunctionResponse.instanceType) &&
Objects.equals(this.lastUpdateTime, getFunctionResponse.lastUpdateTime) &&
Objects.equals(this.maxConcurrency, getFunctionResponse.maxConcurrency) &&
Objects.equals(this.memoryMB, getFunctionResponse.memoryMB) &&
Objects.equals(this.name, getFunctionResponse.name) &&
Objects.equals(this.nasStorage, getFunctionResponse.nasStorage) &&
Objects.equals(this.owner, getFunctionResponse.owner) &&
Objects.equals(this.projectName, getFunctionResponse.projectName) &&
Objects.equals(this.requestTimeout, getFunctionResponse.requestTimeout) &&
Objects.equals(this.runtime, getFunctionResponse.runtime) &&
Objects.equals(this.sourceLocation, getFunctionResponse.sourceLocation) &&
Objects.equals(this.sourceType, getFunctionResponse.sourceType) &&
Objects.equals(this.tlsConfig, getFunctionResponse.tlsConfig) &&
Objects.equals(this.tosMountConfig, getFunctionResponse.tosMountConfig) &&
Objects.equals(this.triggersCount, getFunctionResponse.triggersCount) &&
Objects.equals(this.vpcConfig, getFunctionResponse.vpcConfig);
}
@Override
public int hashCode() {
return Objects.hash(codeSize, codeSizeLimit, command, creationTime, description, envs, exclusiveMode, id, initializerSec, instanceType, lastUpdateTime, maxConcurrency, memoryMB, name, nasStorage, owner, projectName, requestTimeout, runtime, sourceLocation, sourceType, tlsConfig, tosMountConfig, triggersCount, vpcConfig);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class GetFunctionResponse {\n");
sb.append(" codeSize: ").append(toIndentedString(codeSize)).append("\n");
sb.append(" codeSizeLimit: ").append(toIndentedString(codeSizeLimit)).append("\n");
sb.append(" command: ").append(toIndentedString(command)).append("\n");
sb.append(" creationTime: ").append(toIndentedString(creationTime)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" envs: ").append(toIndentedString(envs)).append("\n");
sb.append(" exclusiveMode: ").append(toIndentedString(exclusiveMode)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" initializerSec: ").append(toIndentedString(initializerSec)).append("\n");
sb.append(" instanceType: ").append(toIndentedString(instanceType)).append("\n");
sb.append(" lastUpdateTime: ").append(toIndentedString(lastUpdateTime)).append("\n");
sb.append(" maxConcurrency: ").append(toIndentedString(maxConcurrency)).append("\n");
sb.append(" memoryMB: ").append(toIndentedString(memoryMB)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" nasStorage: ").append(toIndentedString(nasStorage)).append("\n");
sb.append(" owner: ").append(toIndentedString(owner)).append("\n");
sb.append(" projectName: ").append(toIndentedString(projectName)).append("\n");
sb.append(" requestTimeout: ").append(toIndentedString(requestTimeout)).append("\n");
sb.append(" runtime: ").append(toIndentedString(runtime)).append("\n");
sb.append(" sourceLocation: ").append(toIndentedString(sourceLocation)).append("\n");
sb.append(" sourceType: ").append(toIndentedString(sourceType)).append("\n");
sb.append(" tlsConfig: ").append(toIndentedString(tlsConfig)).append("\n");
sb.append(" tosMountConfig: ").append(toIndentedString(tosMountConfig)).append("\n");
sb.append(" triggersCount: ").append(toIndentedString(triggersCount)).append("\n");
sb.append(" vpcConfig: ").append(toIndentedString(vpcConfig)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy