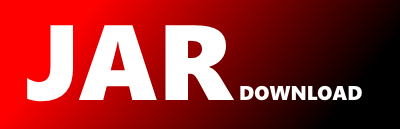
com.volcengine.vefaas.model.MountPointForCreateFunctionInput Maven / Gradle / Ivy
/*
* vefaas
* No description provided (generated by Swagger Codegen https://github.com/swagger-api/swagger-codegen)
*
* OpenAPI spec version: common-version
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.volcengine.vefaas.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.v3.oas.annotations.media.Schema;
import java.io.IOException;
import javax.validation.constraints.*;
import javax.validation.Valid;
/**
* MountPointForCreateFunctionInput
*/
public class MountPointForCreateFunctionInput {
@SerializedName("BucketName")
private String bucketName = null;
@SerializedName("BucketPath")
private String bucketPath = null;
@SerializedName("Endpoint")
private String endpoint = null;
@SerializedName("LocalMountPath")
private String localMountPath = null;
@SerializedName("ReadOnly")
private Boolean readOnly = null;
public MountPointForCreateFunctionInput bucketName(String bucketName) {
this.bucketName = bucketName;
return this;
}
/**
* Get bucketName
* @return bucketName
**/
@Schema(description = "")
public String getBucketName() {
return bucketName;
}
public void setBucketName(String bucketName) {
this.bucketName = bucketName;
}
public MountPointForCreateFunctionInput bucketPath(String bucketPath) {
this.bucketPath = bucketPath;
return this;
}
/**
* Get bucketPath
* @return bucketPath
**/
@Schema(description = "")
public String getBucketPath() {
return bucketPath;
}
public void setBucketPath(String bucketPath) {
this.bucketPath = bucketPath;
}
public MountPointForCreateFunctionInput endpoint(String endpoint) {
this.endpoint = endpoint;
return this;
}
/**
* Get endpoint
* @return endpoint
**/
@Schema(description = "")
public String getEndpoint() {
return endpoint;
}
public void setEndpoint(String endpoint) {
this.endpoint = endpoint;
}
public MountPointForCreateFunctionInput localMountPath(String localMountPath) {
this.localMountPath = localMountPath;
return this;
}
/**
* Get localMountPath
* @return localMountPath
**/
@Schema(description = "")
public String getLocalMountPath() {
return localMountPath;
}
public void setLocalMountPath(String localMountPath) {
this.localMountPath = localMountPath;
}
public MountPointForCreateFunctionInput readOnly(Boolean readOnly) {
this.readOnly = readOnly;
return this;
}
/**
* Get readOnly
* @return readOnly
**/
@Schema(description = "")
public Boolean isReadOnly() {
return readOnly;
}
public void setReadOnly(Boolean readOnly) {
this.readOnly = readOnly;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
MountPointForCreateFunctionInput mountPointForCreateFunctionInput = (MountPointForCreateFunctionInput) o;
return Objects.equals(this.bucketName, mountPointForCreateFunctionInput.bucketName) &&
Objects.equals(this.bucketPath, mountPointForCreateFunctionInput.bucketPath) &&
Objects.equals(this.endpoint, mountPointForCreateFunctionInput.endpoint) &&
Objects.equals(this.localMountPath, mountPointForCreateFunctionInput.localMountPath) &&
Objects.equals(this.readOnly, mountPointForCreateFunctionInput.readOnly);
}
@Override
public int hashCode() {
return Objects.hash(bucketName, bucketPath, endpoint, localMountPath, readOnly);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class MountPointForCreateFunctionInput {\n");
sb.append(" bucketName: ").append(toIndentedString(bucketName)).append("\n");
sb.append(" bucketPath: ").append(toIndentedString(bucketPath)).append("\n");
sb.append(" endpoint: ").append(toIndentedString(endpoint)).append("\n");
sb.append(" localMountPath: ").append(toIndentedString(localMountPath)).append("\n");
sb.append(" readOnly: ").append(toIndentedString(readOnly)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy