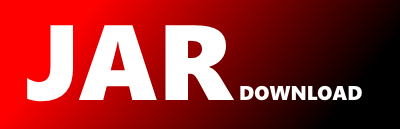
com.volcengine.vepfs.model.FileSystemForDescribeFileSystemsOutput Maven / Gradle / Ivy
/*
* vepfs
* No description provided (generated by Swagger Codegen https://github.com/swagger-api/swagger-codegen)
*
* OpenAPI spec version: common-version
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.volcengine.vepfs.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.volcengine.vepfs.model.CapacityInfoForDescribeFileSystemsOutput;
import com.volcengine.vepfs.model.MountPointForDescribeFileSystemsOutput;
import com.volcengine.vepfs.model.StorageForDescribeFileSystemsOutput;
import com.volcengine.vepfs.model.TagForDescribeFileSystemsOutput;
import com.volcengine.vepfs.model.TradeInfoForDescribeFileSystemsOutput;
import io.swagger.v3.oas.annotations.media.Schema;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import javax.validation.constraints.*;
import javax.validation.Valid;
/**
* FileSystemForDescribeFileSystemsOutput
*/
public class FileSystemForDescribeFileSystemsOutput {
@SerializedName("AccountId")
private String accountId = null;
@SerializedName("AttachStatus")
private String attachStatus = null;
@SerializedName("AutoRenew")
private Boolean autoRenew = null;
@SerializedName("Bandwidth")
private Integer bandwidth = null;
@SerializedName("CapacityInfo")
private CapacityInfoForDescribeFileSystemsOutput capacityInfo = null;
@SerializedName("ChargeStatus")
private String chargeStatus = null;
@SerializedName("ChargeType")
private String chargeType = null;
@SerializedName("CreateTime")
private String createTime = null;
@SerializedName("Description")
private String description = null;
@SerializedName("EceCode")
private String eceCode = null;
@SerializedName("ExpireTime")
private String expireTime = null;
@SerializedName("FileSystemId")
private String fileSystemId = null;
@SerializedName("FileSystemName")
private String fileSystemName = null;
@SerializedName("FileSystemType")
private String fileSystemType = null;
@SerializedName("FreeTime")
private String freeTime = null;
@SerializedName("LastModifyTime")
private String lastModifyTime = null;
@SerializedName("Month")
private Integer month = null;
@SerializedName("MountPoints")
private List mountPoints = null;
@SerializedName("Project")
private String project = null;
@SerializedName("ProtocolType")
private String protocolType = null;
@SerializedName("RegionId")
private String regionId = null;
@SerializedName("ReplicasNum")
private Integer replicasNum = null;
@SerializedName("SecurityGroupId")
private String securityGroupId = null;
@SerializedName("Status")
private String status = null;
@SerializedName("StopServiceTime")
private String stopServiceTime = null;
@SerializedName("Storage")
private StorageForDescribeFileSystemsOutput storage = null;
@SerializedName("StoreType")
private String storeType = null;
@SerializedName("SubnetId")
private String subnetId = null;
@SerializedName("SubnetName")
private String subnetName = null;
@SerializedName("Tags")
private List tags = null;
@SerializedName("TradeInfo")
private TradeInfoForDescribeFileSystemsOutput tradeInfo = null;
@SerializedName("UpgradeEndTime")
private String upgradeEndTime = null;
@SerializedName("UpgradeError")
private String upgradeError = null;
@SerializedName("UpgradeStartTime")
private String upgradeStartTime = null;
@SerializedName("UserId")
private String userId = null;
@SerializedName("Version")
private String version = null;
@SerializedName("VpcId")
private String vpcId = null;
@SerializedName("VpcName")
private String vpcName = null;
@SerializedName("ZoneId")
private String zoneId = null;
@SerializedName("ZoneName")
private String zoneName = null;
public FileSystemForDescribeFileSystemsOutput accountId(String accountId) {
this.accountId = accountId;
return this;
}
/**
* Get accountId
* @return accountId
**/
@Schema(description = "")
public String getAccountId() {
return accountId;
}
public void setAccountId(String accountId) {
this.accountId = accountId;
}
public FileSystemForDescribeFileSystemsOutput attachStatus(String attachStatus) {
this.attachStatus = attachStatus;
return this;
}
/**
* Get attachStatus
* @return attachStatus
**/
@Schema(description = "")
public String getAttachStatus() {
return attachStatus;
}
public void setAttachStatus(String attachStatus) {
this.attachStatus = attachStatus;
}
public FileSystemForDescribeFileSystemsOutput autoRenew(Boolean autoRenew) {
this.autoRenew = autoRenew;
return this;
}
/**
* Get autoRenew
* @return autoRenew
**/
@Schema(description = "")
public Boolean isAutoRenew() {
return autoRenew;
}
public void setAutoRenew(Boolean autoRenew) {
this.autoRenew = autoRenew;
}
public FileSystemForDescribeFileSystemsOutput bandwidth(Integer bandwidth) {
this.bandwidth = bandwidth;
return this;
}
/**
* Get bandwidth
* @return bandwidth
**/
@Schema(description = "")
public Integer getBandwidth() {
return bandwidth;
}
public void setBandwidth(Integer bandwidth) {
this.bandwidth = bandwidth;
}
public FileSystemForDescribeFileSystemsOutput capacityInfo(CapacityInfoForDescribeFileSystemsOutput capacityInfo) {
this.capacityInfo = capacityInfo;
return this;
}
/**
* Get capacityInfo
* @return capacityInfo
**/
@Valid
@Schema(description = "")
public CapacityInfoForDescribeFileSystemsOutput getCapacityInfo() {
return capacityInfo;
}
public void setCapacityInfo(CapacityInfoForDescribeFileSystemsOutput capacityInfo) {
this.capacityInfo = capacityInfo;
}
public FileSystemForDescribeFileSystemsOutput chargeStatus(String chargeStatus) {
this.chargeStatus = chargeStatus;
return this;
}
/**
* Get chargeStatus
* @return chargeStatus
**/
@Schema(description = "")
public String getChargeStatus() {
return chargeStatus;
}
public void setChargeStatus(String chargeStatus) {
this.chargeStatus = chargeStatus;
}
public FileSystemForDescribeFileSystemsOutput chargeType(String chargeType) {
this.chargeType = chargeType;
return this;
}
/**
* Get chargeType
* @return chargeType
**/
@Schema(description = "")
public String getChargeType() {
return chargeType;
}
public void setChargeType(String chargeType) {
this.chargeType = chargeType;
}
public FileSystemForDescribeFileSystemsOutput createTime(String createTime) {
this.createTime = createTime;
return this;
}
/**
* Get createTime
* @return createTime
**/
@Schema(description = "")
public String getCreateTime() {
return createTime;
}
public void setCreateTime(String createTime) {
this.createTime = createTime;
}
public FileSystemForDescribeFileSystemsOutput description(String description) {
this.description = description;
return this;
}
/**
* Get description
* @return description
**/
@Schema(description = "")
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public FileSystemForDescribeFileSystemsOutput eceCode(String eceCode) {
this.eceCode = eceCode;
return this;
}
/**
* Get eceCode
* @return eceCode
**/
@Schema(description = "")
public String getEceCode() {
return eceCode;
}
public void setEceCode(String eceCode) {
this.eceCode = eceCode;
}
public FileSystemForDescribeFileSystemsOutput expireTime(String expireTime) {
this.expireTime = expireTime;
return this;
}
/**
* Get expireTime
* @return expireTime
**/
@Schema(description = "")
public String getExpireTime() {
return expireTime;
}
public void setExpireTime(String expireTime) {
this.expireTime = expireTime;
}
public FileSystemForDescribeFileSystemsOutput fileSystemId(String fileSystemId) {
this.fileSystemId = fileSystemId;
return this;
}
/**
* Get fileSystemId
* @return fileSystemId
**/
@Schema(description = "")
public String getFileSystemId() {
return fileSystemId;
}
public void setFileSystemId(String fileSystemId) {
this.fileSystemId = fileSystemId;
}
public FileSystemForDescribeFileSystemsOutput fileSystemName(String fileSystemName) {
this.fileSystemName = fileSystemName;
return this;
}
/**
* Get fileSystemName
* @return fileSystemName
**/
@Schema(description = "")
public String getFileSystemName() {
return fileSystemName;
}
public void setFileSystemName(String fileSystemName) {
this.fileSystemName = fileSystemName;
}
public FileSystemForDescribeFileSystemsOutput fileSystemType(String fileSystemType) {
this.fileSystemType = fileSystemType;
return this;
}
/**
* Get fileSystemType
* @return fileSystemType
**/
@Schema(description = "")
public String getFileSystemType() {
return fileSystemType;
}
public void setFileSystemType(String fileSystemType) {
this.fileSystemType = fileSystemType;
}
public FileSystemForDescribeFileSystemsOutput freeTime(String freeTime) {
this.freeTime = freeTime;
return this;
}
/**
* Get freeTime
* @return freeTime
**/
@Schema(description = "")
public String getFreeTime() {
return freeTime;
}
public void setFreeTime(String freeTime) {
this.freeTime = freeTime;
}
public FileSystemForDescribeFileSystemsOutput lastModifyTime(String lastModifyTime) {
this.lastModifyTime = lastModifyTime;
return this;
}
/**
* Get lastModifyTime
* @return lastModifyTime
**/
@Schema(description = "")
public String getLastModifyTime() {
return lastModifyTime;
}
public void setLastModifyTime(String lastModifyTime) {
this.lastModifyTime = lastModifyTime;
}
public FileSystemForDescribeFileSystemsOutput month(Integer month) {
this.month = month;
return this;
}
/**
* Get month
* @return month
**/
@Schema(description = "")
public Integer getMonth() {
return month;
}
public void setMonth(Integer month) {
this.month = month;
}
public FileSystemForDescribeFileSystemsOutput mountPoints(List mountPoints) {
this.mountPoints = mountPoints;
return this;
}
public FileSystemForDescribeFileSystemsOutput addMountPointsItem(MountPointForDescribeFileSystemsOutput mountPointsItem) {
if (this.mountPoints == null) {
this.mountPoints = new ArrayList();
}
this.mountPoints.add(mountPointsItem);
return this;
}
/**
* Get mountPoints
* @return mountPoints
**/
@Valid
@Schema(description = "")
public List getMountPoints() {
return mountPoints;
}
public void setMountPoints(List mountPoints) {
this.mountPoints = mountPoints;
}
public FileSystemForDescribeFileSystemsOutput project(String project) {
this.project = project;
return this;
}
/**
* Get project
* @return project
**/
@Schema(description = "")
public String getProject() {
return project;
}
public void setProject(String project) {
this.project = project;
}
public FileSystemForDescribeFileSystemsOutput protocolType(String protocolType) {
this.protocolType = protocolType;
return this;
}
/**
* Get protocolType
* @return protocolType
**/
@Schema(description = "")
public String getProtocolType() {
return protocolType;
}
public void setProtocolType(String protocolType) {
this.protocolType = protocolType;
}
public FileSystemForDescribeFileSystemsOutput regionId(String regionId) {
this.regionId = regionId;
return this;
}
/**
* Get regionId
* @return regionId
**/
@Schema(description = "")
public String getRegionId() {
return regionId;
}
public void setRegionId(String regionId) {
this.regionId = regionId;
}
public FileSystemForDescribeFileSystemsOutput replicasNum(Integer replicasNum) {
this.replicasNum = replicasNum;
return this;
}
/**
* Get replicasNum
* @return replicasNum
**/
@Schema(description = "")
public Integer getReplicasNum() {
return replicasNum;
}
public void setReplicasNum(Integer replicasNum) {
this.replicasNum = replicasNum;
}
public FileSystemForDescribeFileSystemsOutput securityGroupId(String securityGroupId) {
this.securityGroupId = securityGroupId;
return this;
}
/**
* Get securityGroupId
* @return securityGroupId
**/
@Schema(description = "")
public String getSecurityGroupId() {
return securityGroupId;
}
public void setSecurityGroupId(String securityGroupId) {
this.securityGroupId = securityGroupId;
}
public FileSystemForDescribeFileSystemsOutput status(String status) {
this.status = status;
return this;
}
/**
* Get status
* @return status
**/
@Schema(description = "")
public String getStatus() {
return status;
}
public void setStatus(String status) {
this.status = status;
}
public FileSystemForDescribeFileSystemsOutput stopServiceTime(String stopServiceTime) {
this.stopServiceTime = stopServiceTime;
return this;
}
/**
* Get stopServiceTime
* @return stopServiceTime
**/
@Schema(description = "")
public String getStopServiceTime() {
return stopServiceTime;
}
public void setStopServiceTime(String stopServiceTime) {
this.stopServiceTime = stopServiceTime;
}
public FileSystemForDescribeFileSystemsOutput storage(StorageForDescribeFileSystemsOutput storage) {
this.storage = storage;
return this;
}
/**
* Get storage
* @return storage
**/
@Valid
@Schema(description = "")
public StorageForDescribeFileSystemsOutput getStorage() {
return storage;
}
public void setStorage(StorageForDescribeFileSystemsOutput storage) {
this.storage = storage;
}
public FileSystemForDescribeFileSystemsOutput storeType(String storeType) {
this.storeType = storeType;
return this;
}
/**
* Get storeType
* @return storeType
**/
@Schema(description = "")
public String getStoreType() {
return storeType;
}
public void setStoreType(String storeType) {
this.storeType = storeType;
}
public FileSystemForDescribeFileSystemsOutput subnetId(String subnetId) {
this.subnetId = subnetId;
return this;
}
/**
* Get subnetId
* @return subnetId
**/
@Schema(description = "")
public String getSubnetId() {
return subnetId;
}
public void setSubnetId(String subnetId) {
this.subnetId = subnetId;
}
public FileSystemForDescribeFileSystemsOutput subnetName(String subnetName) {
this.subnetName = subnetName;
return this;
}
/**
* Get subnetName
* @return subnetName
**/
@Schema(description = "")
public String getSubnetName() {
return subnetName;
}
public void setSubnetName(String subnetName) {
this.subnetName = subnetName;
}
public FileSystemForDescribeFileSystemsOutput tags(List tags) {
this.tags = tags;
return this;
}
public FileSystemForDescribeFileSystemsOutput addTagsItem(TagForDescribeFileSystemsOutput tagsItem) {
if (this.tags == null) {
this.tags = new ArrayList();
}
this.tags.add(tagsItem);
return this;
}
/**
* Get tags
* @return tags
**/
@Valid
@Schema(description = "")
public List getTags() {
return tags;
}
public void setTags(List tags) {
this.tags = tags;
}
public FileSystemForDescribeFileSystemsOutput tradeInfo(TradeInfoForDescribeFileSystemsOutput tradeInfo) {
this.tradeInfo = tradeInfo;
return this;
}
/**
* Get tradeInfo
* @return tradeInfo
**/
@Valid
@Schema(description = "")
public TradeInfoForDescribeFileSystemsOutput getTradeInfo() {
return tradeInfo;
}
public void setTradeInfo(TradeInfoForDescribeFileSystemsOutput tradeInfo) {
this.tradeInfo = tradeInfo;
}
public FileSystemForDescribeFileSystemsOutput upgradeEndTime(String upgradeEndTime) {
this.upgradeEndTime = upgradeEndTime;
return this;
}
/**
* Get upgradeEndTime
* @return upgradeEndTime
**/
@Schema(description = "")
public String getUpgradeEndTime() {
return upgradeEndTime;
}
public void setUpgradeEndTime(String upgradeEndTime) {
this.upgradeEndTime = upgradeEndTime;
}
public FileSystemForDescribeFileSystemsOutput upgradeError(String upgradeError) {
this.upgradeError = upgradeError;
return this;
}
/**
* Get upgradeError
* @return upgradeError
**/
@Schema(description = "")
public String getUpgradeError() {
return upgradeError;
}
public void setUpgradeError(String upgradeError) {
this.upgradeError = upgradeError;
}
public FileSystemForDescribeFileSystemsOutput upgradeStartTime(String upgradeStartTime) {
this.upgradeStartTime = upgradeStartTime;
return this;
}
/**
* Get upgradeStartTime
* @return upgradeStartTime
**/
@Schema(description = "")
public String getUpgradeStartTime() {
return upgradeStartTime;
}
public void setUpgradeStartTime(String upgradeStartTime) {
this.upgradeStartTime = upgradeStartTime;
}
public FileSystemForDescribeFileSystemsOutput userId(String userId) {
this.userId = userId;
return this;
}
/**
* Get userId
* @return userId
**/
@Schema(description = "")
public String getUserId() {
return userId;
}
public void setUserId(String userId) {
this.userId = userId;
}
public FileSystemForDescribeFileSystemsOutput version(String version) {
this.version = version;
return this;
}
/**
* Get version
* @return version
**/
@Schema(description = "")
public String getVersion() {
return version;
}
public void setVersion(String version) {
this.version = version;
}
public FileSystemForDescribeFileSystemsOutput vpcId(String vpcId) {
this.vpcId = vpcId;
return this;
}
/**
* Get vpcId
* @return vpcId
**/
@Schema(description = "")
public String getVpcId() {
return vpcId;
}
public void setVpcId(String vpcId) {
this.vpcId = vpcId;
}
public FileSystemForDescribeFileSystemsOutput vpcName(String vpcName) {
this.vpcName = vpcName;
return this;
}
/**
* Get vpcName
* @return vpcName
**/
@Schema(description = "")
public String getVpcName() {
return vpcName;
}
public void setVpcName(String vpcName) {
this.vpcName = vpcName;
}
public FileSystemForDescribeFileSystemsOutput zoneId(String zoneId) {
this.zoneId = zoneId;
return this;
}
/**
* Get zoneId
* @return zoneId
**/
@Schema(description = "")
public String getZoneId() {
return zoneId;
}
public void setZoneId(String zoneId) {
this.zoneId = zoneId;
}
public FileSystemForDescribeFileSystemsOutput zoneName(String zoneName) {
this.zoneName = zoneName;
return this;
}
/**
* Get zoneName
* @return zoneName
**/
@Schema(description = "")
public String getZoneName() {
return zoneName;
}
public void setZoneName(String zoneName) {
this.zoneName = zoneName;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
FileSystemForDescribeFileSystemsOutput fileSystemForDescribeFileSystemsOutput = (FileSystemForDescribeFileSystemsOutput) o;
return Objects.equals(this.accountId, fileSystemForDescribeFileSystemsOutput.accountId) &&
Objects.equals(this.attachStatus, fileSystemForDescribeFileSystemsOutput.attachStatus) &&
Objects.equals(this.autoRenew, fileSystemForDescribeFileSystemsOutput.autoRenew) &&
Objects.equals(this.bandwidth, fileSystemForDescribeFileSystemsOutput.bandwidth) &&
Objects.equals(this.capacityInfo, fileSystemForDescribeFileSystemsOutput.capacityInfo) &&
Objects.equals(this.chargeStatus, fileSystemForDescribeFileSystemsOutput.chargeStatus) &&
Objects.equals(this.chargeType, fileSystemForDescribeFileSystemsOutput.chargeType) &&
Objects.equals(this.createTime, fileSystemForDescribeFileSystemsOutput.createTime) &&
Objects.equals(this.description, fileSystemForDescribeFileSystemsOutput.description) &&
Objects.equals(this.eceCode, fileSystemForDescribeFileSystemsOutput.eceCode) &&
Objects.equals(this.expireTime, fileSystemForDescribeFileSystemsOutput.expireTime) &&
Objects.equals(this.fileSystemId, fileSystemForDescribeFileSystemsOutput.fileSystemId) &&
Objects.equals(this.fileSystemName, fileSystemForDescribeFileSystemsOutput.fileSystemName) &&
Objects.equals(this.fileSystemType, fileSystemForDescribeFileSystemsOutput.fileSystemType) &&
Objects.equals(this.freeTime, fileSystemForDescribeFileSystemsOutput.freeTime) &&
Objects.equals(this.lastModifyTime, fileSystemForDescribeFileSystemsOutput.lastModifyTime) &&
Objects.equals(this.month, fileSystemForDescribeFileSystemsOutput.month) &&
Objects.equals(this.mountPoints, fileSystemForDescribeFileSystemsOutput.mountPoints) &&
Objects.equals(this.project, fileSystemForDescribeFileSystemsOutput.project) &&
Objects.equals(this.protocolType, fileSystemForDescribeFileSystemsOutput.protocolType) &&
Objects.equals(this.regionId, fileSystemForDescribeFileSystemsOutput.regionId) &&
Objects.equals(this.replicasNum, fileSystemForDescribeFileSystemsOutput.replicasNum) &&
Objects.equals(this.securityGroupId, fileSystemForDescribeFileSystemsOutput.securityGroupId) &&
Objects.equals(this.status, fileSystemForDescribeFileSystemsOutput.status) &&
Objects.equals(this.stopServiceTime, fileSystemForDescribeFileSystemsOutput.stopServiceTime) &&
Objects.equals(this.storage, fileSystemForDescribeFileSystemsOutput.storage) &&
Objects.equals(this.storeType, fileSystemForDescribeFileSystemsOutput.storeType) &&
Objects.equals(this.subnetId, fileSystemForDescribeFileSystemsOutput.subnetId) &&
Objects.equals(this.subnetName, fileSystemForDescribeFileSystemsOutput.subnetName) &&
Objects.equals(this.tags, fileSystemForDescribeFileSystemsOutput.tags) &&
Objects.equals(this.tradeInfo, fileSystemForDescribeFileSystemsOutput.tradeInfo) &&
Objects.equals(this.upgradeEndTime, fileSystemForDescribeFileSystemsOutput.upgradeEndTime) &&
Objects.equals(this.upgradeError, fileSystemForDescribeFileSystemsOutput.upgradeError) &&
Objects.equals(this.upgradeStartTime, fileSystemForDescribeFileSystemsOutput.upgradeStartTime) &&
Objects.equals(this.userId, fileSystemForDescribeFileSystemsOutput.userId) &&
Objects.equals(this.version, fileSystemForDescribeFileSystemsOutput.version) &&
Objects.equals(this.vpcId, fileSystemForDescribeFileSystemsOutput.vpcId) &&
Objects.equals(this.vpcName, fileSystemForDescribeFileSystemsOutput.vpcName) &&
Objects.equals(this.zoneId, fileSystemForDescribeFileSystemsOutput.zoneId) &&
Objects.equals(this.zoneName, fileSystemForDescribeFileSystemsOutput.zoneName);
}
@Override
public int hashCode() {
return Objects.hash(accountId, attachStatus, autoRenew, bandwidth, capacityInfo, chargeStatus, chargeType, createTime, description, eceCode, expireTime, fileSystemId, fileSystemName, fileSystemType, freeTime, lastModifyTime, month, mountPoints, project, protocolType, regionId, replicasNum, securityGroupId, status, stopServiceTime, storage, storeType, subnetId, subnetName, tags, tradeInfo, upgradeEndTime, upgradeError, upgradeStartTime, userId, version, vpcId, vpcName, zoneId, zoneName);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class FileSystemForDescribeFileSystemsOutput {\n");
sb.append(" accountId: ").append(toIndentedString(accountId)).append("\n");
sb.append(" attachStatus: ").append(toIndentedString(attachStatus)).append("\n");
sb.append(" autoRenew: ").append(toIndentedString(autoRenew)).append("\n");
sb.append(" bandwidth: ").append(toIndentedString(bandwidth)).append("\n");
sb.append(" capacityInfo: ").append(toIndentedString(capacityInfo)).append("\n");
sb.append(" chargeStatus: ").append(toIndentedString(chargeStatus)).append("\n");
sb.append(" chargeType: ").append(toIndentedString(chargeType)).append("\n");
sb.append(" createTime: ").append(toIndentedString(createTime)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" eceCode: ").append(toIndentedString(eceCode)).append("\n");
sb.append(" expireTime: ").append(toIndentedString(expireTime)).append("\n");
sb.append(" fileSystemId: ").append(toIndentedString(fileSystemId)).append("\n");
sb.append(" fileSystemName: ").append(toIndentedString(fileSystemName)).append("\n");
sb.append(" fileSystemType: ").append(toIndentedString(fileSystemType)).append("\n");
sb.append(" freeTime: ").append(toIndentedString(freeTime)).append("\n");
sb.append(" lastModifyTime: ").append(toIndentedString(lastModifyTime)).append("\n");
sb.append(" month: ").append(toIndentedString(month)).append("\n");
sb.append(" mountPoints: ").append(toIndentedString(mountPoints)).append("\n");
sb.append(" project: ").append(toIndentedString(project)).append("\n");
sb.append(" protocolType: ").append(toIndentedString(protocolType)).append("\n");
sb.append(" regionId: ").append(toIndentedString(regionId)).append("\n");
sb.append(" replicasNum: ").append(toIndentedString(replicasNum)).append("\n");
sb.append(" securityGroupId: ").append(toIndentedString(securityGroupId)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" stopServiceTime: ").append(toIndentedString(stopServiceTime)).append("\n");
sb.append(" storage: ").append(toIndentedString(storage)).append("\n");
sb.append(" storeType: ").append(toIndentedString(storeType)).append("\n");
sb.append(" subnetId: ").append(toIndentedString(subnetId)).append("\n");
sb.append(" subnetName: ").append(toIndentedString(subnetName)).append("\n");
sb.append(" tags: ").append(toIndentedString(tags)).append("\n");
sb.append(" tradeInfo: ").append(toIndentedString(tradeInfo)).append("\n");
sb.append(" upgradeEndTime: ").append(toIndentedString(upgradeEndTime)).append("\n");
sb.append(" upgradeError: ").append(toIndentedString(upgradeError)).append("\n");
sb.append(" upgradeStartTime: ").append(toIndentedString(upgradeStartTime)).append("\n");
sb.append(" userId: ").append(toIndentedString(userId)).append("\n");
sb.append(" version: ").append(toIndentedString(version)).append("\n");
sb.append(" vpcId: ").append(toIndentedString(vpcId)).append("\n");
sb.append(" vpcName: ").append(toIndentedString(vpcName)).append("\n");
sb.append(" zoneId: ").append(toIndentedString(zoneId)).append("\n");
sb.append(" zoneName: ").append(toIndentedString(zoneName)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy