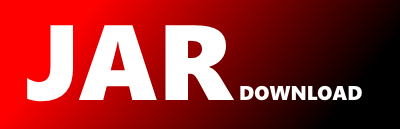
com.volcengine.waf.model.UpdateAclRuleRequest Maven / Gradle / Ivy
/*
* waf
* No description provided (generated by Swagger Codegen https://github.com/swagger-api/swagger-codegen)
*
* OpenAPI spec version: common-version
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.volcengine.waf.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.volcengine.waf.model.AccurateGroupForUpdateAclRuleInput;
import io.swagger.v3.oas.annotations.media.Schema;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import javax.validation.constraints.*;
import javax.validation.Valid;
/**
* UpdateAclRuleRequest
*/
public class UpdateAclRuleRequest {
@SerializedName("AccurateGroup")
private AccurateGroupForUpdateAclRuleInput accurateGroup = null;
@SerializedName("AclType")
private String aclType = null;
@SerializedName("Action")
private String action = null;
@SerializedName("Advanced")
private Integer advanced = null;
@SerializedName("Description")
private String description = null;
@SerializedName("Enable")
private Integer enable = null;
@SerializedName("HostAddType")
private Integer hostAddType = null;
@SerializedName("HostGroupId")
private List hostGroupId = null;
@SerializedName("HostList")
private List hostList = null;
@SerializedName("ID")
private Integer ID = null;
@SerializedName("IpAddType")
private Integer ipAddType = null;
@SerializedName("IpGroupId")
private List ipGroupId = null;
@SerializedName("IpList")
private List ipList = null;
@SerializedName("IpLocationCountry")
private List ipLocationCountry = null;
@SerializedName("IpLocationSubregion")
private List ipLocationSubregion = null;
@SerializedName("Name")
private String name = null;
@SerializedName("PrefixSwitch")
private Integer prefixSwitch = null;
@SerializedName("Url")
private String url = null;
public UpdateAclRuleRequest accurateGroup(AccurateGroupForUpdateAclRuleInput accurateGroup) {
this.accurateGroup = accurateGroup;
return this;
}
/**
* Get accurateGroup
* @return accurateGroup
**/
@Valid
@Schema(description = "")
public AccurateGroupForUpdateAclRuleInput getAccurateGroup() {
return accurateGroup;
}
public void setAccurateGroup(AccurateGroupForUpdateAclRuleInput accurateGroup) {
this.accurateGroup = accurateGroup;
}
public UpdateAclRuleRequest aclType(String aclType) {
this.aclType = aclType;
return this;
}
/**
* Get aclType
* @return aclType
**/
@NotNull
@Schema(required = true, description = "")
public String getAclType() {
return aclType;
}
public void setAclType(String aclType) {
this.aclType = aclType;
}
public UpdateAclRuleRequest action(String action) {
this.action = action;
return this;
}
/**
* Get action
* @return action
**/
@Schema(description = "")
public String getAction() {
return action;
}
public void setAction(String action) {
this.action = action;
}
public UpdateAclRuleRequest advanced(Integer advanced) {
this.advanced = advanced;
return this;
}
/**
* Get advanced
* @return advanced
**/
@Schema(description = "")
public Integer getAdvanced() {
return advanced;
}
public void setAdvanced(Integer advanced) {
this.advanced = advanced;
}
public UpdateAclRuleRequest description(String description) {
this.description = description;
return this;
}
/**
* Get description
* @return description
**/
@Schema(description = "")
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public UpdateAclRuleRequest enable(Integer enable) {
this.enable = enable;
return this;
}
/**
* Get enable
* @return enable
**/
@NotNull
@Schema(required = true, description = "")
public Integer getEnable() {
return enable;
}
public void setEnable(Integer enable) {
this.enable = enable;
}
public UpdateAclRuleRequest hostAddType(Integer hostAddType) {
this.hostAddType = hostAddType;
return this;
}
/**
* Get hostAddType
* @return hostAddType
**/
@NotNull
@Schema(required = true, description = "")
public Integer getHostAddType() {
return hostAddType;
}
public void setHostAddType(Integer hostAddType) {
this.hostAddType = hostAddType;
}
public UpdateAclRuleRequest hostGroupId(List hostGroupId) {
this.hostGroupId = hostGroupId;
return this;
}
public UpdateAclRuleRequest addHostGroupIdItem(Integer hostGroupIdItem) {
if (this.hostGroupId == null) {
this.hostGroupId = new ArrayList();
}
this.hostGroupId.add(hostGroupIdItem);
return this;
}
/**
* Get hostGroupId
* @return hostGroupId
**/
@Schema(description = "")
public List getHostGroupId() {
return hostGroupId;
}
public void setHostGroupId(List hostGroupId) {
this.hostGroupId = hostGroupId;
}
public UpdateAclRuleRequest hostList(List hostList) {
this.hostList = hostList;
return this;
}
public UpdateAclRuleRequest addHostListItem(String hostListItem) {
if (this.hostList == null) {
this.hostList = new ArrayList();
}
this.hostList.add(hostListItem);
return this;
}
/**
* Get hostList
* @return hostList
**/
@Schema(description = "")
public List getHostList() {
return hostList;
}
public void setHostList(List hostList) {
this.hostList = hostList;
}
public UpdateAclRuleRequest ID(Integer ID) {
this.ID = ID;
return this;
}
/**
* Get ID
* @return ID
**/
@NotNull
@Schema(required = true, description = "")
public Integer getID() {
return ID;
}
public void setID(Integer ID) {
this.ID = ID;
}
public UpdateAclRuleRequest ipAddType(Integer ipAddType) {
this.ipAddType = ipAddType;
return this;
}
/**
* Get ipAddType
* @return ipAddType
**/
@NotNull
@Schema(required = true, description = "")
public Integer getIpAddType() {
return ipAddType;
}
public void setIpAddType(Integer ipAddType) {
this.ipAddType = ipAddType;
}
public UpdateAclRuleRequest ipGroupId(List ipGroupId) {
this.ipGroupId = ipGroupId;
return this;
}
public UpdateAclRuleRequest addIpGroupIdItem(Integer ipGroupIdItem) {
if (this.ipGroupId == null) {
this.ipGroupId = new ArrayList();
}
this.ipGroupId.add(ipGroupIdItem);
return this;
}
/**
* Get ipGroupId
* @return ipGroupId
**/
@Schema(description = "")
public List getIpGroupId() {
return ipGroupId;
}
public void setIpGroupId(List ipGroupId) {
this.ipGroupId = ipGroupId;
}
public UpdateAclRuleRequest ipList(List ipList) {
this.ipList = ipList;
return this;
}
public UpdateAclRuleRequest addIpListItem(String ipListItem) {
if (this.ipList == null) {
this.ipList = new ArrayList();
}
this.ipList.add(ipListItem);
return this;
}
/**
* Get ipList
* @return ipList
**/
@Schema(description = "")
public List getIpList() {
return ipList;
}
public void setIpList(List ipList) {
this.ipList = ipList;
}
public UpdateAclRuleRequest ipLocationCountry(List ipLocationCountry) {
this.ipLocationCountry = ipLocationCountry;
return this;
}
public UpdateAclRuleRequest addIpLocationCountryItem(String ipLocationCountryItem) {
if (this.ipLocationCountry == null) {
this.ipLocationCountry = new ArrayList();
}
this.ipLocationCountry.add(ipLocationCountryItem);
return this;
}
/**
* Get ipLocationCountry
* @return ipLocationCountry
**/
@Schema(description = "")
public List getIpLocationCountry() {
return ipLocationCountry;
}
public void setIpLocationCountry(List ipLocationCountry) {
this.ipLocationCountry = ipLocationCountry;
}
public UpdateAclRuleRequest ipLocationSubregion(List ipLocationSubregion) {
this.ipLocationSubregion = ipLocationSubregion;
return this;
}
public UpdateAclRuleRequest addIpLocationSubregionItem(String ipLocationSubregionItem) {
if (this.ipLocationSubregion == null) {
this.ipLocationSubregion = new ArrayList();
}
this.ipLocationSubregion.add(ipLocationSubregionItem);
return this;
}
/**
* Get ipLocationSubregion
* @return ipLocationSubregion
**/
@Schema(description = "")
public List getIpLocationSubregion() {
return ipLocationSubregion;
}
public void setIpLocationSubregion(List ipLocationSubregion) {
this.ipLocationSubregion = ipLocationSubregion;
}
public UpdateAclRuleRequest name(String name) {
this.name = name;
return this;
}
/**
* Get name
* @return name
**/
@NotNull
@Schema(required = true, description = "")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public UpdateAclRuleRequest prefixSwitch(Integer prefixSwitch) {
this.prefixSwitch = prefixSwitch;
return this;
}
/**
* Get prefixSwitch
* @return prefixSwitch
**/
@Schema(description = "")
public Integer getPrefixSwitch() {
return prefixSwitch;
}
public void setPrefixSwitch(Integer prefixSwitch) {
this.prefixSwitch = prefixSwitch;
}
public UpdateAclRuleRequest url(String url) {
this.url = url;
return this;
}
/**
* Get url
* @return url
**/
@NotNull
@Schema(required = true, description = "")
public String getUrl() {
return url;
}
public void setUrl(String url) {
this.url = url;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
UpdateAclRuleRequest updateAclRuleRequest = (UpdateAclRuleRequest) o;
return Objects.equals(this.accurateGroup, updateAclRuleRequest.accurateGroup) &&
Objects.equals(this.aclType, updateAclRuleRequest.aclType) &&
Objects.equals(this.action, updateAclRuleRequest.action) &&
Objects.equals(this.advanced, updateAclRuleRequest.advanced) &&
Objects.equals(this.description, updateAclRuleRequest.description) &&
Objects.equals(this.enable, updateAclRuleRequest.enable) &&
Objects.equals(this.hostAddType, updateAclRuleRequest.hostAddType) &&
Objects.equals(this.hostGroupId, updateAclRuleRequest.hostGroupId) &&
Objects.equals(this.hostList, updateAclRuleRequest.hostList) &&
Objects.equals(this.ID, updateAclRuleRequest.ID) &&
Objects.equals(this.ipAddType, updateAclRuleRequest.ipAddType) &&
Objects.equals(this.ipGroupId, updateAclRuleRequest.ipGroupId) &&
Objects.equals(this.ipList, updateAclRuleRequest.ipList) &&
Objects.equals(this.ipLocationCountry, updateAclRuleRequest.ipLocationCountry) &&
Objects.equals(this.ipLocationSubregion, updateAclRuleRequest.ipLocationSubregion) &&
Objects.equals(this.name, updateAclRuleRequest.name) &&
Objects.equals(this.prefixSwitch, updateAclRuleRequest.prefixSwitch) &&
Objects.equals(this.url, updateAclRuleRequest.url);
}
@Override
public int hashCode() {
return Objects.hash(accurateGroup, aclType, action, advanced, description, enable, hostAddType, hostGroupId, hostList, ID, ipAddType, ipGroupId, ipList, ipLocationCountry, ipLocationSubregion, name, prefixSwitch, url);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class UpdateAclRuleRequest {\n");
sb.append(" accurateGroup: ").append(toIndentedString(accurateGroup)).append("\n");
sb.append(" aclType: ").append(toIndentedString(aclType)).append("\n");
sb.append(" action: ").append(toIndentedString(action)).append("\n");
sb.append(" advanced: ").append(toIndentedString(advanced)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" enable: ").append(toIndentedString(enable)).append("\n");
sb.append(" hostAddType: ").append(toIndentedString(hostAddType)).append("\n");
sb.append(" hostGroupId: ").append(toIndentedString(hostGroupId)).append("\n");
sb.append(" hostList: ").append(toIndentedString(hostList)).append("\n");
sb.append(" ID: ").append(toIndentedString(ID)).append("\n");
sb.append(" ipAddType: ").append(toIndentedString(ipAddType)).append("\n");
sb.append(" ipGroupId: ").append(toIndentedString(ipGroupId)).append("\n");
sb.append(" ipList: ").append(toIndentedString(ipList)).append("\n");
sb.append(" ipLocationCountry: ").append(toIndentedString(ipLocationCountry)).append("\n");
sb.append(" ipLocationSubregion: ").append(toIndentedString(ipLocationSubregion)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" prefixSwitch: ").append(toIndentedString(prefixSwitch)).append("\n");
sb.append(" url: ").append(toIndentedString(url)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy