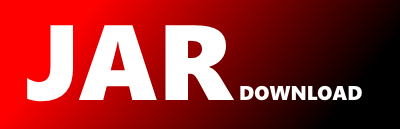
com.volcengine.waf.model.ListDomainRequest Maven / Gradle / Ivy
/*
* waf
* No description provided (generated by Swagger Codegen https://github.com/swagger-api/swagger-codegen)
*
* OpenAPI spec version: common-version
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.volcengine.waf.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.volcengine.waf.model.LBInfoForListDomainInput;
import io.swagger.v3.oas.annotations.media.Schema;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import javax.validation.constraints.*;
import javax.validation.Valid;
/**
* ListDomainRequest
*/
public class ListDomainRequest {
@SerializedName("AccessMode")
private List accessMode = null;
@SerializedName("AccurateQuery")
private Integer accurateQuery = null;
@SerializedName("AttackStatus")
private List attackStatus = null;
@SerializedName("ClientIp")
private String clientIp = null;
@SerializedName("DefenceMode")
private List defenceMode = null;
@SerializedName("Domain")
private String domain = null;
@SerializedName("DomainOrPath")
private String domainOrPath = null;
@SerializedName("LBInfo")
private List lbInfo = null;
@SerializedName("Page")
private Integer page = null;
@SerializedName("PageSize")
private Integer pageSize = null;
@SerializedName("PublicRealServer")
private List publicRealServer = null;
@SerializedName("Region")
private String region = null;
@SerializedName("SortByCreateTime")
private String sortByCreateTime = null;
@SerializedName("SortByDomainName")
private String sortByDomainName = null;
@SerializedName("SortByUpdateTime")
private String sortByUpdateTime = null;
@SerializedName("Status")
private List status = null;
@SerializedName("TLSEnable")
private List tlSEnable = null;
@SerializedName("VpcId")
private String vpcId = null;
@SerializedName("VpcName")
private String vpcName = null;
@SerializedName("VpcOwnerId")
private String vpcOwnerId = null;
@SerializedName("VpcOwnerName")
private String vpcOwnerName = null;
public ListDomainRequest accessMode(List accessMode) {
this.accessMode = accessMode;
return this;
}
public ListDomainRequest addAccessModeItem(Integer accessModeItem) {
if (this.accessMode == null) {
this.accessMode = new ArrayList();
}
this.accessMode.add(accessModeItem);
return this;
}
/**
* Get accessMode
* @return accessMode
**/
@Schema(description = "")
public List getAccessMode() {
return accessMode;
}
public void setAccessMode(List accessMode) {
this.accessMode = accessMode;
}
public ListDomainRequest accurateQuery(Integer accurateQuery) {
this.accurateQuery = accurateQuery;
return this;
}
/**
* Get accurateQuery
* @return accurateQuery
**/
@NotNull
@Schema(required = true, description = "")
public Integer getAccurateQuery() {
return accurateQuery;
}
public void setAccurateQuery(Integer accurateQuery) {
this.accurateQuery = accurateQuery;
}
public ListDomainRequest attackStatus(List attackStatus) {
this.attackStatus = attackStatus;
return this;
}
public ListDomainRequest addAttackStatusItem(Integer attackStatusItem) {
if (this.attackStatus == null) {
this.attackStatus = new ArrayList();
}
this.attackStatus.add(attackStatusItem);
return this;
}
/**
* Get attackStatus
* @return attackStatus
**/
@Schema(description = "")
public List getAttackStatus() {
return attackStatus;
}
public void setAttackStatus(List attackStatus) {
this.attackStatus = attackStatus;
}
public ListDomainRequest clientIp(String clientIp) {
this.clientIp = clientIp;
return this;
}
/**
* Get clientIp
* @return clientIp
**/
@Schema(description = "")
public String getClientIp() {
return clientIp;
}
public void setClientIp(String clientIp) {
this.clientIp = clientIp;
}
public ListDomainRequest defenceMode(List defenceMode) {
this.defenceMode = defenceMode;
return this;
}
public ListDomainRequest addDefenceModeItem(Integer defenceModeItem) {
if (this.defenceMode == null) {
this.defenceMode = new ArrayList();
}
this.defenceMode.add(defenceModeItem);
return this;
}
/**
* Get defenceMode
* @return defenceMode
**/
@Schema(description = "")
public List getDefenceMode() {
return defenceMode;
}
public void setDefenceMode(List defenceMode) {
this.defenceMode = defenceMode;
}
public ListDomainRequest domain(String domain) {
this.domain = domain;
return this;
}
/**
* Get domain
* @return domain
**/
@Schema(description = "")
public String getDomain() {
return domain;
}
public void setDomain(String domain) {
this.domain = domain;
}
public ListDomainRequest domainOrPath(String domainOrPath) {
this.domainOrPath = domainOrPath;
return this;
}
/**
* Get domainOrPath
* @return domainOrPath
**/
@Schema(description = "")
public String getDomainOrPath() {
return domainOrPath;
}
public void setDomainOrPath(String domainOrPath) {
this.domainOrPath = domainOrPath;
}
public ListDomainRequest lbInfo(List lbInfo) {
this.lbInfo = lbInfo;
return this;
}
public ListDomainRequest addLbInfoItem(LBInfoForListDomainInput lbInfoItem) {
if (this.lbInfo == null) {
this.lbInfo = new ArrayList();
}
this.lbInfo.add(lbInfoItem);
return this;
}
/**
* Get lbInfo
* @return lbInfo
**/
@Valid
@Schema(description = "")
public List getLbInfo() {
return lbInfo;
}
public void setLbInfo(List lbInfo) {
this.lbInfo = lbInfo;
}
public ListDomainRequest page(Integer page) {
this.page = page;
return this;
}
/**
* Get page
* @return page
**/
@NotNull
@Schema(required = true, description = "")
public Integer getPage() {
return page;
}
public void setPage(Integer page) {
this.page = page;
}
public ListDomainRequest pageSize(Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Get pageSize
* @return pageSize
**/
@NotNull
@Schema(required = true, description = "")
public Integer getPageSize() {
return pageSize;
}
public void setPageSize(Integer pageSize) {
this.pageSize = pageSize;
}
public ListDomainRequest publicRealServer(List publicRealServer) {
this.publicRealServer = publicRealServer;
return this;
}
public ListDomainRequest addPublicRealServerItem(Integer publicRealServerItem) {
if (this.publicRealServer == null) {
this.publicRealServer = new ArrayList();
}
this.publicRealServer.add(publicRealServerItem);
return this;
}
/**
* Get publicRealServer
* @return publicRealServer
**/
@Schema(description = "")
public List getPublicRealServer() {
return publicRealServer;
}
public void setPublicRealServer(List publicRealServer) {
this.publicRealServer = publicRealServer;
}
public ListDomainRequest region(String region) {
this.region = region;
return this;
}
/**
* Get region
* @return region
**/
@NotNull
@Schema(required = true, description = "")
public String getRegion() {
return region;
}
public void setRegion(String region) {
this.region = region;
}
public ListDomainRequest sortByCreateTime(String sortByCreateTime) {
this.sortByCreateTime = sortByCreateTime;
return this;
}
/**
* Get sortByCreateTime
* @return sortByCreateTime
**/
@Schema(description = "")
public String getSortByCreateTime() {
return sortByCreateTime;
}
public void setSortByCreateTime(String sortByCreateTime) {
this.sortByCreateTime = sortByCreateTime;
}
public ListDomainRequest sortByDomainName(String sortByDomainName) {
this.sortByDomainName = sortByDomainName;
return this;
}
/**
* Get sortByDomainName
* @return sortByDomainName
**/
@Schema(description = "")
public String getSortByDomainName() {
return sortByDomainName;
}
public void setSortByDomainName(String sortByDomainName) {
this.sortByDomainName = sortByDomainName;
}
public ListDomainRequest sortByUpdateTime(String sortByUpdateTime) {
this.sortByUpdateTime = sortByUpdateTime;
return this;
}
/**
* Get sortByUpdateTime
* @return sortByUpdateTime
**/
@Schema(description = "")
public String getSortByUpdateTime() {
return sortByUpdateTime;
}
public void setSortByUpdateTime(String sortByUpdateTime) {
this.sortByUpdateTime = sortByUpdateTime;
}
public ListDomainRequest status(List status) {
this.status = status;
return this;
}
public ListDomainRequest addStatusItem(Integer statusItem) {
if (this.status == null) {
this.status = new ArrayList();
}
this.status.add(statusItem);
return this;
}
/**
* Get status
* @return status
**/
@Schema(description = "")
public List getStatus() {
return status;
}
public void setStatus(List status) {
this.status = status;
}
public ListDomainRequest tlSEnable(List tlSEnable) {
this.tlSEnable = tlSEnable;
return this;
}
public ListDomainRequest addTlSEnableItem(Integer tlSEnableItem) {
if (this.tlSEnable == null) {
this.tlSEnable = new ArrayList();
}
this.tlSEnable.add(tlSEnableItem);
return this;
}
/**
* Get tlSEnable
* @return tlSEnable
**/
@Schema(description = "")
public List getTlSEnable() {
return tlSEnable;
}
public void setTlSEnable(List tlSEnable) {
this.tlSEnable = tlSEnable;
}
public ListDomainRequest vpcId(String vpcId) {
this.vpcId = vpcId;
return this;
}
/**
* Get vpcId
* @return vpcId
**/
@Schema(description = "")
public String getVpcId() {
return vpcId;
}
public void setVpcId(String vpcId) {
this.vpcId = vpcId;
}
public ListDomainRequest vpcName(String vpcName) {
this.vpcName = vpcName;
return this;
}
/**
* Get vpcName
* @return vpcName
**/
@Schema(description = "")
public String getVpcName() {
return vpcName;
}
public void setVpcName(String vpcName) {
this.vpcName = vpcName;
}
public ListDomainRequest vpcOwnerId(String vpcOwnerId) {
this.vpcOwnerId = vpcOwnerId;
return this;
}
/**
* Get vpcOwnerId
* @return vpcOwnerId
**/
@Schema(description = "")
public String getVpcOwnerId() {
return vpcOwnerId;
}
public void setVpcOwnerId(String vpcOwnerId) {
this.vpcOwnerId = vpcOwnerId;
}
public ListDomainRequest vpcOwnerName(String vpcOwnerName) {
this.vpcOwnerName = vpcOwnerName;
return this;
}
/**
* Get vpcOwnerName
* @return vpcOwnerName
**/
@Schema(description = "")
public String getVpcOwnerName() {
return vpcOwnerName;
}
public void setVpcOwnerName(String vpcOwnerName) {
this.vpcOwnerName = vpcOwnerName;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ListDomainRequest listDomainRequest = (ListDomainRequest) o;
return Objects.equals(this.accessMode, listDomainRequest.accessMode) &&
Objects.equals(this.accurateQuery, listDomainRequest.accurateQuery) &&
Objects.equals(this.attackStatus, listDomainRequest.attackStatus) &&
Objects.equals(this.clientIp, listDomainRequest.clientIp) &&
Objects.equals(this.defenceMode, listDomainRequest.defenceMode) &&
Objects.equals(this.domain, listDomainRequest.domain) &&
Objects.equals(this.domainOrPath, listDomainRequest.domainOrPath) &&
Objects.equals(this.lbInfo, listDomainRequest.lbInfo) &&
Objects.equals(this.page, listDomainRequest.page) &&
Objects.equals(this.pageSize, listDomainRequest.pageSize) &&
Objects.equals(this.publicRealServer, listDomainRequest.publicRealServer) &&
Objects.equals(this.region, listDomainRequest.region) &&
Objects.equals(this.sortByCreateTime, listDomainRequest.sortByCreateTime) &&
Objects.equals(this.sortByDomainName, listDomainRequest.sortByDomainName) &&
Objects.equals(this.sortByUpdateTime, listDomainRequest.sortByUpdateTime) &&
Objects.equals(this.status, listDomainRequest.status) &&
Objects.equals(this.tlSEnable, listDomainRequest.tlSEnable) &&
Objects.equals(this.vpcId, listDomainRequest.vpcId) &&
Objects.equals(this.vpcName, listDomainRequest.vpcName) &&
Objects.equals(this.vpcOwnerId, listDomainRequest.vpcOwnerId) &&
Objects.equals(this.vpcOwnerName, listDomainRequest.vpcOwnerName);
}
@Override
public int hashCode() {
return Objects.hash(accessMode, accurateQuery, attackStatus, clientIp, defenceMode, domain, domainOrPath, lbInfo, page, pageSize, publicRealServer, region, sortByCreateTime, sortByDomainName, sortByUpdateTime, status, tlSEnable, vpcId, vpcName, vpcOwnerId, vpcOwnerName);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ListDomainRequest {\n");
sb.append(" accessMode: ").append(toIndentedString(accessMode)).append("\n");
sb.append(" accurateQuery: ").append(toIndentedString(accurateQuery)).append("\n");
sb.append(" attackStatus: ").append(toIndentedString(attackStatus)).append("\n");
sb.append(" clientIp: ").append(toIndentedString(clientIp)).append("\n");
sb.append(" defenceMode: ").append(toIndentedString(defenceMode)).append("\n");
sb.append(" domain: ").append(toIndentedString(domain)).append("\n");
sb.append(" domainOrPath: ").append(toIndentedString(domainOrPath)).append("\n");
sb.append(" lbInfo: ").append(toIndentedString(lbInfo)).append("\n");
sb.append(" page: ").append(toIndentedString(page)).append("\n");
sb.append(" pageSize: ").append(toIndentedString(pageSize)).append("\n");
sb.append(" publicRealServer: ").append(toIndentedString(publicRealServer)).append("\n");
sb.append(" region: ").append(toIndentedString(region)).append("\n");
sb.append(" sortByCreateTime: ").append(toIndentedString(sortByCreateTime)).append("\n");
sb.append(" sortByDomainName: ").append(toIndentedString(sortByDomainName)).append("\n");
sb.append(" sortByUpdateTime: ").append(toIndentedString(sortByUpdateTime)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" tlSEnable: ").append(toIndentedString(tlSEnable)).append("\n");
sb.append(" vpcId: ").append(toIndentedString(vpcId)).append("\n");
sb.append(" vpcName: ").append(toIndentedString(vpcName)).append("\n");
sb.append(" vpcOwnerId: ").append(toIndentedString(vpcOwnerId)).append("\n");
sb.append(" vpcOwnerName: ").append(toIndentedString(vpcOwnerName)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy