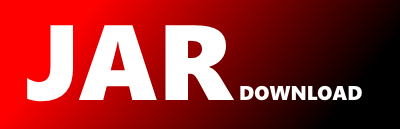
com.voodoodyne.jackson.jsog.JSOGGenerator Maven / Gradle / Ivy
package com.voodoodyne.jackson.jsog;
import com.fasterxml.jackson.annotation.ObjectIdGenerator;
/**
* Use this as an object id generator and your class will serialize as jsog.
*
* @author Jeff Schnitzer
*/
public class JSOGGenerator extends ObjectIdGenerator {
private static final long serialVersionUID = 1L;
protected transient int _nextValue;
protected final Class> _scope;
public JSOGGenerator() { this(null, -1); }
public JSOGGenerator(Class> scope, int nextValue) {
_scope = scope;
_nextValue = nextValue;
}
@Override
public Class> getScope() {
return _scope;
}
@Override
public boolean canUseFor(ObjectIdGenerator> gen) {
return (gen.getClass() == getClass()) && (gen.getScope() == _scope);
}
@Override
public ObjectIdGenerator forScope(Class> scope) {
return (_scope == scope) ? this : new JSOGGenerator(scope, _nextValue);
}
@Override
public ObjectIdGenerator newForSerialization(Object context) {
return new JSOGGenerator(_scope, 1);
}
@Override
public com.fasterxml.jackson.annotation.ObjectIdGenerator.IdKey key(Object key) {
return new IdKey(getClass(), _scope, key);
}
@Override
public JSOGRef generateId(Object forPojo) {
int id = _nextValue;
++_nextValue;
return new JSOGRef(id);
}
@Override
public boolean maySerializeAsObject() {
return true;
}
@Override
public boolean isValidReferencePropertyName(String name, Object parser) {
return JSOGRef.REF_KEY.equals(name);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy