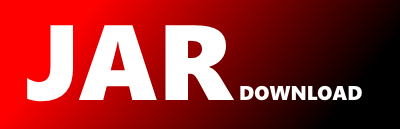
com.vwo.config.VWOConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vwo-java-sdk Show documentation
Show all versions of vwo-java-sdk Show documentation
Java library to initiate VWo instance for server side a/b testing
package com.vwo.config;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.vwo.JacksonParser;
import com.vwo.Parser;
import com.vwo.enums.LoggerMessagesEnum;
import com.vwo.logger.LoggerManager;
import com.vwo.models.Campaign;
import com.vwo.models.SettingFileConfig;
import com.vwo.models.Variation;
import java.util.List;
import javafx.util.Pair;
public class VWOConfig implements ProjectConfig {
private SettingFileConfig settingFileConfig;
private final double MAX_TRAFFIC_VALUE = 10000;
private static final LoggerManager LOGGER = LoggerManager.getLogger(VWOConfig.class);
public VWOConfig(SettingFileConfig settingFileConfig) {
this.settingFileConfig = settingFileConfig;
}
public SettingFileConfig processSettingsFile() {
List campaignList = settingFileConfig.getCampaigns();
for (Campaign singleCampaign : campaignList) {
setVariationBucketing(singleCampaign);
}
return this.settingFileConfig;
}
public Campaign setVariationBucketing(Campaign campaign) {
return setVariationAllocation(campaign);
}
public Campaign setVariationAllocation(Campaign campaign) {
List variationList = campaign.getVariations();
for (Variation singleVariation : variationList) {
Double stepFactor = getVariationBucketRange(singleVariation.getWeight());
if (stepFactor != null && stepFactor != -1) {
singleVariation.setStartRangeVariation((int) campaign.getCurrentAllocationVariation() + 1);
campaign.setCurrentAllocationVariation(Math.ceil(campaign.getCurrentAllocationVariation() + stepFactor));
singleVariation.setEndRangeVariation((int) campaign.getCurrentAllocationVariation());
} else {
singleVariation.setStartRangeVariation(-1);
singleVariation.setEndRangeVariation(-1);
}
LOGGER.info(LoggerMessagesEnum.INFO_MESSAGES.VARIATION_ALLOCATED_SUCCESSFULLY.value(
new Pair<>("campaignTestKey", campaign.getKey()),
new Pair<>("variation", singleVariation.getName()),
new Pair<>("weight", String.valueOf(singleVariation.getWeight())),
new Pair<>("startRange", String.valueOf(singleVariation.getStartRangeVariation())),
new Pair<>("endRange", String.valueOf(singleVariation.getEndRangeVariation()))
));
}
return campaign;
}
public Double getVariationBucketRange(double variationWeight) {
double startRange = variationWeight * 100;
if (startRange == 0) {
startRange = -1;
}
return Math.min(startRange, MAX_TRAFFIC_VALUE);
}
public Campaign getCampaignTestKey(String campaignTestKey) {
for (Campaign campaign : settingFileConfig.getCampaigns()) {
if (campaign.getKey().equalsIgnoreCase(campaignTestKey)) {
LOGGER.debug(LoggerMessagesEnum.DEBUG_MESSAGES.CAMPAIGN_KEY_FOUND.value(new Pair<>("campaignTestKey", campaignTestKey)));
return campaign;
}
}
return null;
}
public SettingFileConfig getSettingFileConfig() {
return settingFileConfig;
}
public static class Builder {
private String settingFile;
private final ObjectMapper objectMapper = new ObjectMapper();
private Parser parser = null;
private Builder(String settingFile) {
this.settingFile = settingFile;
}
public static Builder getInstance(String settingFile) {
Builder builder = new Builder(settingFile);
if (builder.parser == null) {
builder.parser = new JacksonParser(builder.objectMapper);
}
return builder;
}
public Builder withParser(Parser parser) {
this.parser = parser;
return this;
}
public VWOConfig build() throws ConfigParseException {
if (settingFile == null) {
throw new ConfigParseException("Parsing Failed due to null settings file.");
}
if (settingFile.isEmpty()) {
throw new ConfigParseException("Parsing Failed due to empty settings file.");
}
SettingFileConfig settingFileConfig = parser.isValid(settingFile);
return new VWOConfig(settingFileConfig);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy