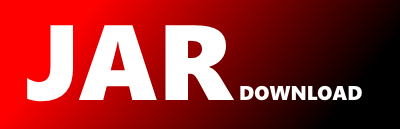
com.vwo.services.http.HttpRequestBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vwo-java-sdk Show documentation
Show all versions of vwo-java-sdk Show documentation
Java library to initiate VWo instance for server side a/b testing
/**
* Copyright 2019-2021 Wingify Software Pvt. Ltd.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.vwo.services.http;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.PropertyAccessor;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.vwo.enums.HTTPEnums;
import com.vwo.enums.LoggerMessagesEnums;
import com.vwo.enums.UriEnums;
import com.vwo.logger.Logger;
import com.vwo.models.Campaign;
import com.vwo.models.Goal;
import com.vwo.models.Settings;
import com.vwo.models.Variation;
import com.vwo.services.batch.FlushInterface;
import com.vwo.services.settings.SettingFile;
import com.vwo.utils.UUIDUtils;
import org.apache.http.Header;
import org.apache.http.message.BasicHeader;
import java.io.UnsupportedEncodingException;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
import java.time.Instant;
import java.util.HashMap;
import java.util.Map;
import java.util.Queue;
import java.util.UUID;
public class HttpRequestBuilder {
public static final String VWO_HOST = UriEnums.BASE_URL.toString();
public static final String IMPRESSION_PATH = UriEnums.TRACK_USER.toString();
public static final String GOAL_PATH = UriEnums.TRACK_GOAL.toString();
public static final String SETTINGS_URL = UriEnums.SETTINGS_URL.toString();
public static final String WEBHOOK_SETTINGS_URL = UriEnums.WEBHOOK_SETTINGS_URL.toString();
public static final String PUSH = UriEnums.PUSH.toString();
public static final String BATCH_EVENTS = UriEnums.BATCH_EVENTS.toString();
private static final ObjectMapper objectMapper = new ObjectMapper();
private static final Logger LOGGER = Logger.getLogger(HttpRequestBuilder.class);
public static HttpParams getSettingParams(String accountID, String sdkKey, boolean isViaWebhook) {
BuildQueryParams requestParams =
BuildQueryParams.Builder.getInstance()
.withSettingsAccountId(accountID)
.withR(Math.random())
.withSdkKey(sdkKey)
.withsdk()
.withsdkVersion()
.withPlatform()
.build();
LOGGER.debug(LoggerMessagesEnums.DEBUG_MESSAGES.GET_SETTINGS_IMPRESSION_CREATED.value());
Map map = requestParams.convertToMap();
objectMapper.setVisibility(PropertyAccessor.FIELD, JsonAutoDetect.Visibility.ANY);
if (isViaWebhook) {
return new HttpParams(VWO_HOST, WEBHOOK_SETTINGS_URL, map, HTTPEnums.Verbs.GET);
} else {
return new HttpParams(VWO_HOST, SETTINGS_URL, map, HTTPEnums.Verbs.GET);
}
}
public static HttpParams getUserParams(SettingFile settingFile, Campaign campaign, String userId, Variation variation, Map usageStats) {
Settings settings = settingFile.getSettings();
BuildQueryParams requestParams = BuildQueryParams.Builder.getInstance()
.withAccountId(settings.getAccountId())
.withCampaignId(campaign.getId())
.withRandom(Math.random())
.withAp()
.withEd()
.withUuid(settings.getAccountId(), userId)
.withSid(Instant.now().getEpochSecond())
.withVariation(variation.getId())
.withsdk()
.withsdkVersion()
.withUsageStats(usageStats)
.withEnvironment(settings.getSdkKey())
.build();
LOGGER.debug(LoggerMessagesEnums.DEBUG_MESSAGES.TRACK_USER_IMPRESSION_CREATED.value(new HashMap() {
{
put("userId", userId);
}
}));
Map map = requestParams.convertToMap();
objectMapper.setVisibility(PropertyAccessor.FIELD, JsonAutoDetect.Visibility.ANY);
return new HttpParams(VWO_HOST, IMPRESSION_PATH, map, HTTPEnums.Verbs.GET);
}
public static Map getBatchEventForTrackingUser(SettingFile settingFile, Campaign campaign, String userId, Variation variation) {
Settings settings = settingFile.getSettings();
BuildQueryParams requestParams =
BuildQueryParams.Builder.getInstance()
.withMinifiedCampaignId(campaign.getId())
.withMinifiedVariationId(variation.getId())
.withMinifiedEventType(1)
.withSid(Instant.now().getEpochSecond())
.withUuid(settings.getAccountId(), userId)
.build();
LOGGER.debug(LoggerMessagesEnums.DEBUG_MESSAGES.TRACK_USER_IMPRESSION_CREATED.value(new HashMap() {
{
put("userId", userId);
}
}));
Map map = requestParams.convertToMap();
return requestParams.removeNullValues(map);
}
public static HttpParams getGoalParams(SettingFile settingFile, Campaign campaign, String userId, Goal goal, Variation variation, Object revenueValue) {
Settings settings = settingFile.getSettings();
BuildQueryParams requestParams =
BuildQueryParams.Builder.getInstance()
.withAccountId(settings.getAccountId())
.withCampaignId(campaign.getId())
.withRandom(Math.random())
.withAp()
.withUuid(settings.getAccountId(), userId)
.withGoalId(goal.getId())
.withSid(Instant.now().getEpochSecond())
.withRevenue(revenueValue)
.withVariation(variation.getId())
.withsdk()
.withsdkVersion()
.withEnvironment(settings.getSdkKey())
.build();
LOGGER.debug(LoggerMessagesEnums.DEBUG_MESSAGES.TRACK_GOAL_IMPRESSION_CREATED.value(new HashMap() {
{
put("userId", userId);
}
}));
Map map = requestParams.convertToMap();
objectMapper.setVisibility(PropertyAccessor.FIELD, JsonAutoDetect.Visibility.ANY);
return new HttpParams(VWO_HOST, GOAL_PATH, map, HTTPEnums.Verbs.GET);
}
public static Map getBatchEventForTrackingGoal(SettingFile settingFile, Campaign campaign, String userId, Goal goal, Variation variation, Object revenueValue) {
Settings settings = settingFile.getSettings();
BuildQueryParams requestParams =
BuildQueryParams.Builder.getInstance()
.withMinifiedCampaignId(campaign.getId())
.withMinifiedVariationId(variation.getId())
.withMinifiedEventType(2)
.withMinifiedGoalId(goal.getId())
.withRevenue(revenueValue)
.withSid(Instant.now().getEpochSecond())
.withUuid(settings.getAccountId(), userId)
.build();
LOGGER.debug(LoggerMessagesEnums.DEBUG_MESSAGES.TRACK_GOAL_IMPRESSION_CREATED.value(new HashMap() {
{
put("userId", userId);
}
}));
Map map = requestParams.convertToMap();
return requestParams.removeNullValues(map);
}
public static HttpParams getCustomDimensionParams(SettingFile settingFile, String tagKey, String tagValue, String userId) {
Settings settings = settingFile.getSettings();
BuildQueryParams requestParams =
BuildQueryParams.Builder.getInstance()
.withAccountId(settings.getAccountId())
.withUuid(settings.getAccountId(), userId)
.withTags(tagKey, tagValue)
.withSid(Instant.now().getEpochSecond())
.withRandom(Math.random())
.withAp()
.withsdk()
.withsdkVersion()
.withEnvironment(settings.getSdkKey())
.build();
LOGGER.debug(LoggerMessagesEnums.DEBUG_MESSAGES.POST_SEGMENTATION_REQUEST_CREATED.value(new HashMap() {
{
put("userId", userId);
}
}));
Map map = requestParams.convertToMap();
objectMapper.setVisibility(PropertyAccessor.FIELD, JsonAutoDetect.Visibility.ANY);
return new HttpParams(VWO_HOST, PUSH, map, HTTPEnums.Verbs.GET);
}
public static Map getBatchEventForCustomDimension(SettingFile settingFile, String tagKey, String tagValue, String userId) {
Settings settings = settingFile.getSettings();
BuildQueryParams requestParams =
BuildQueryParams.Builder.getInstance()
.withMinifiedEventType(3)
.withMinifiedTags(tagKey, tagValue)
.withSid(Instant.now().getEpochSecond())
.withUuid(settings.getAccountId(), userId)
.build();
LOGGER.debug(LoggerMessagesEnums.DEBUG_MESSAGES.POST_SEGMENTATION_REQUEST_CREATED.value(new HashMap() {
{
put("userId", userId);
}
}));
Map map = requestParams.convertToMap();
return requestParams.removeNullValues(map);
}
public static HttpParams getBatchEventPostCallParams(String accountId, String apiKey, Queue
© 2015 - 2025 Weber Informatics LLC | Privacy Policy