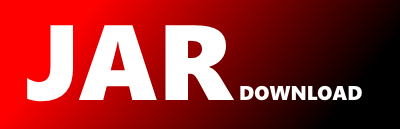
com.wacai.file.gateway.FileManager Maven / Gradle / Ivy
/*
package com.wacai.file.gateway;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.wacai.file.gateway.entity.LocalFile;
import com.wacai.file.gateway.entity.RemoteFile;
import com.wacai.file.gateway.entity.Response;
import com.wacai.file.gateway.entity.StreamFile;
import com.wacai.file.token.ApplyToken;
import com.wacai.file.token.response.AccessToken;
import lombok.extern.slf4j.Slf4j;
import org.springframework.core.io.FileSystemResource;
import org.springframework.core.io.InputStreamResource;
import org.springframework.http.*;
import org.springframework.http.client.SimpleClientHttpRequestFactory;
import org.springframework.util.LinkedMultiValueMap;
import org.springframework.util.MultiValueMap;
import org.springframework.web.client.RestTemplate;
import java.io.IOException;
import java.util.List;
*/
/**
* Created by fulushou on 2018/5/10.
*//*
@Slf4j
public class FileManager {
private String appKey = "3y3nmtkx3ykc";
private String appSecret = "8cnukuk9tu7annnr";
private String gatewayAuthUrl = "http://open-token-boot.loan.k2.test.wacai.info/token/auth";
private volatile String xAccessToken = null;
private ApplyToken applyToken;
private RestTemplate client = new RestTemplate();
private String url;
private String namespace;
private int execTimes = 3;
public FileManager(String url, String namespace,String appKey,String appSecret,String gatewayAuthUrl) {
this.url = url;
this.namespace = namespace;
this.applyToken = new ApplyToken(appKey,appSecret,gatewayAuthUrl);
this.setTimeout(10000L);
}
public Response> uploadFilesRetry(List localFiles) throws IOException {
for (int i = 0; i < execTimes; i++) {
try{
return uploadFiles(localFiles);
}catch (Exception e){
xAccessToken = null;
log.error("upload files:{} exception:",localFiles,e);
}
}
Response response = new Response();
response.code = 1;
response.error = "upload error try three times";
return response;
}
public Response> uploadStreamsRetry(List streamFiles) throws IOException {
for (int i = 0; i < execTimes; i++) {
try{
return uploadStreams(streamFiles);
}catch (Exception e){
xAccessToken = null;
log.error("upload streams:{} exception:",streamFiles,e);
}
}
Response response = new Response();
response.code = 1;
response.error = "upload streams try three times";
return response;
}
public Response uploadFileRetry(LocalFile localFile) throws IOException {
for (int i = 0; i < execTimes; i++) {
try{
return uploadFile(localFile);
}catch (Exception e){
xAccessToken = null;
log.error("upload file:{} exception:",localFile,e);
}
}
Response response = new Response();
response.code = 1;
response.error = "upload error try three times";
return response;
}
public Response uploadStreamRetry(StreamFile streamFile) throws IOException {
for (int i = 0; i < execTimes; i++) {
try{
return uploadStream(streamFile);
}catch (Exception e){
xAccessToken = null;
log.error("upload stream:{} exception:",streamFile,e);
}
}
Response response = new Response();
response.code = 1;
response.error = "upload stream try three times";
return response;
}
private Response> uploadFiles(List localFiles) throws IOException {
MultiValueMap body = this.addLocalFiles(localFiles);
HttpHeaders headers = this.generateHeaders();
final HttpEntity> request = new HttpEntity>(body, headers);
String tempUrl = url.endsWith("/") ? (url + "upload/normal/" + namespace) : (url + "/upload/normal/" + namespace);
final ResponseEntity result = client.postForEntity(tempUrl,request,String.class);
ObjectMapper mapper = new ObjectMapper();
Response> res = mapper.readValue(result.getBody(),new TypeReference>>(){});
return res;
}
private Response> uploadStreams(List streamFiles) throws IOException {
MultiValueMap body = this.addStreamFiles(streamFiles);
HttpHeaders headers = this.generateHeaders();
final HttpEntity> request = new HttpEntity>(body, headers);
String tempUrl = url.endsWith("/") ? (url + "upload/normal/" + namespace) : (url + "/upload/normal/" + namespace);
final ResponseEntity result = client.postForEntity(tempUrl,request,String.class);
ObjectMapper mapper = new ObjectMapper();
Response> res = mapper.readValue(result.getBody(),new TypeReference>>(){});
return res;
}
private Response uploadFile(LocalFile localFile) throws IOException {
MultiValueMap body = this.addLocalFile(localFile);
HttpHeaders headers = this.generateHeaders();
final HttpEntity> request = new HttpEntity>(body, headers);
String tempUrl = url.endsWith("/") ? (url + "upload/online/" + namespace) : (url + "/upload/online/" + namespace);
final ResponseEntity result = client.postForEntity(tempUrl,request,String.class);
ObjectMapper mapper = new ObjectMapper();
Response res = mapper.readValue(result.getBody(),new TypeReference>(){});
return res;
}
public Response uploadStream(StreamFile streamFile) throws IOException {
MultiValueMap body = this.addStreamFile(streamFile);
HttpHeaders headers = this.generateHeaders();
final HttpEntity> request = new HttpEntity>(body, headers);
String tempUrl = url.endsWith("/") ? (url + "upload/online/" + namespace) : (url + "/upload/online/" + namespace);
final ResponseEntity result = client.postForEntity(tempUrl,request,String.class);
ObjectMapper mapper = new ObjectMapper();
Response res = mapper.readValue(result.getBody(),new TypeReference>(){});
return res;
}
private LinkedMultiValueMap addStreamFile(final StreamFile streamFile){
LinkedMultiValueMap body = new LinkedMultiValueMap();
body.add("file",new InputStreamResource(streamFile.getInputStream()));
body.add("filename",streamFile.getFilename());
body.add("expireSeconds",streamFile.getExpireSeconds());
return body;
}
private LinkedMultiValueMap addLocalFile(final LocalFile localFile){
LinkedMultiValueMap body = new LinkedMultiValueMap();
body.add("file",new FileSystemResource(localFile.getFile()));
body.add("filename",localFile.getFilename());
body.add("expireSeconds",localFile.getExpireSeconds());
return body;
}
private LinkedMultiValueMap addStreamFiles(final List streamFiles){
LinkedMultiValueMap body = new LinkedMultiValueMap();
for (StreamFile streamFile : streamFiles) {
final String filename = streamFile.getFilename();
body.add("files",new InputStreamResource(streamFile.getInputStream()){
@Override
public String getFilename() {
return filename == null?super.getFilename():filename;
}
});
}
return body;
}
private LinkedMultiValueMap addLocalFiles(final List localFiles){
LinkedMultiValueMap body = new LinkedMultiValueMap();
for (LocalFile localFile : localFiles) {
final String filename = localFile.getFilename();
body.add("files",new FileSystemResource(localFile.getFile()){
@Override
public String getFilename() {
return filename == null?super.getFilename():filename;
}
});
}
return body;
}
public byte[] downloadRetry(RemoteFile remoteFile) {
for (int i = 0; i < execTimes; i++) {
try{
return download(remoteFile);
}catch (Exception e){
xAccessToken = null;
log.error("upload remoteFile:{} exception:",remoteFile,e);
}
}
return null;
}
public byte[] downloadSecretKeyRetry(RemoteFile remoteFile) {
for (int i = 0; i < execTimes; i++) {
try{
return downloadSecretKey(remoteFile);
}catch (Exception e){
xAccessToken = null;
log.error("upload downloadSecretKey:{} exception:",remoteFile,e);
}
}
return null;
}
private byte[] download(RemoteFile remoteFile) {
HttpHeaders headers = this.generateHeaders();
final HttpEntity requestEntity = new HttpEntity(null, headers);
String tempUrl = url.endsWith("/") ? (url + "download/" + remoteFile.getNamespace() + "/" + remoteFile.getFilename() + "/") : (url + "/download/" + remoteFile.getNamespace() + "/" + remoteFile.getFilename() + "/");
ResponseEntity result = client.exchange(tempUrl, HttpMethod.GET, requestEntity, byte[].class);
return result.getBody();
}
private byte[] downloadSecretKey(RemoteFile remoteFile) {
if(remoteFile.getSecretKey() == null ) {
throw new RuntimeException("secretKey can not be null");
}
String tempUrl = url.endsWith("/") ? (url + "download/" + remoteFile.getNamespace() + "/" + remoteFile.getFilename() + "/" + remoteFile.getSecretKey()) : (url + "/download/" + remoteFile.getNamespace() + "/" + remoteFile.getFilename() + "/" + remoteFile.getSecretKey());
ResponseEntity result = client.exchange(tempUrl, HttpMethod.GET,null, byte[].class);
return result.getBody();
}
private HttpHeaders generateHeaders() {
if(xAccessToken == null){
synchronized (lock) {
if(xAccessToken == null) {
AccessToken accessToken = applyToken.applyAccessToken();
xAccessToken = accessToken.getAccessToken();
}
}
}
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.MULTIPART_FORM_DATA);
headers.add("X-access-token",xAccessToken);
headers.add("appKey",appKey);
return headers;
}
public void setTimeout(Long timeout) {
if (timeout != null && client.getRequestFactory() instanceof SimpleClientHttpRequestFactory) {
((SimpleClientHttpRequestFactory) client.getRequestFactory()).setReadTimeout(timeout.intValue());
}
}
public void setExecTimes(int execTimes) {
if(execTimes >=1 && execTimes <= 10)
this.execTimes = execTimes;
}
private Object lock = new Object();
}
*/
© 2015 - 2025 Weber Informatics LLC | Privacy Policy