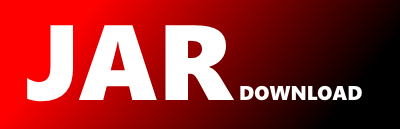
com.wadpam.oauth2.dao.GeneratedDConnectionDao Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of oauth2-client Show documentation
Show all versions of oauth2-client Show documentation
Implements OAuth2 authentication on top of Open-Server security
The newest version!
package com.wadpam.oauth2.dao;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import net.sf.mardao.core.CursorPage;
import net.sf.mardao.core.dao.Dao;
import com.wadpam.oauth2.domain.DConnection;
import net.sf.mardao.core.geo.DLocation;
/**
* DAO interface with finder methods for DConnection entities.
*
* Generated on 2013-07-25T10:05:08.359+0700.
* @author mardao DAO generator (net.sf.mardao.plugin.ProcessDomainMojo)
*/
public interface GeneratedDConnectionDao extends Dao {
/** Column name for primary key attribute is "id" */
static final String COLUMN_NAME_ID = "id";
/** Column name for field appArg0 is "appArg0" */
static final String COLUMN_NAME_APPARG0 = "appArg0";
/** Column name for field createdBy is "createdBy" */
static final String COLUMN_NAME_CREATEDBY = "createdBy";
/** Column name for field createdDate is "createdDate" */
static final String COLUMN_NAME_CREATEDDATE = "createdDate";
/** Column name for field displayName is "displayName" */
static final String COLUMN_NAME_DISPLAYNAME = "displayName";
/** Column name for field expireTime is "expireTime" */
static final String COLUMN_NAME_EXPIRETIME = "expireTime";
/** Column name for field imageUrl is "imageUrl" */
static final String COLUMN_NAME_IMAGEURL = "imageUrl";
/** Column name for field profileUrl is "profileUrl" */
static final String COLUMN_NAME_PROFILEURL = "profileUrl";
/** Column name for field providerId is "providerId" */
static final String COLUMN_NAME_PROVIDERID = "providerId";
/** Column name for field providerUserId is "providerUserId" */
static final String COLUMN_NAME_PROVIDERUSERID = "providerUserId";
/** Column name for field refreshToken is "refreshToken" */
static final String COLUMN_NAME_REFRESHTOKEN = "refreshToken";
/** Column name for field secret is "secret" */
static final String COLUMN_NAME_SECRET = "secret";
/** Column name for field updatedBy is "updatedBy" */
static final String COLUMN_NAME_UPDATEDBY = "updatedBy";
/** Column name for field updatedDate is "updatedDate" */
static final String COLUMN_NAME_UPDATEDDATE = "updatedDate";
/** Column name for field userId is "userId" */
static final String COLUMN_NAME_USERID = "userId";
/** Column name for field userRoles is "userRoles" */
static final String COLUMN_NAME_USERROLES = "userRoles";
/** The list of attribute names */
static final List COLUMN_NAMES = Arrays.asList( COLUMN_NAME_APPARG0,
COLUMN_NAME_CREATEDBY,
COLUMN_NAME_CREATEDDATE,
COLUMN_NAME_DISPLAYNAME,
COLUMN_NAME_EXPIRETIME,
COLUMN_NAME_IMAGEURL,
COLUMN_NAME_PROFILEURL,
COLUMN_NAME_PROVIDERID,
COLUMN_NAME_PROVIDERUSERID,
COLUMN_NAME_REFRESHTOKEN,
COLUMN_NAME_SECRET,
COLUMN_NAME_UPDATEDBY,
COLUMN_NAME_UPDATEDDATE,
COLUMN_NAME_USERID,
COLUMN_NAME_USERROLES);
/** The list of Basic attribute names */
static final List BASIC_NAMES = Arrays.asList( COLUMN_NAME_APPARG0,
COLUMN_NAME_CREATEDBY,
COLUMN_NAME_CREATEDDATE,
COLUMN_NAME_DISPLAYNAME,
COLUMN_NAME_EXPIRETIME,
COLUMN_NAME_IMAGEURL,
COLUMN_NAME_PROFILEURL,
COLUMN_NAME_PROVIDERID,
COLUMN_NAME_PROVIDERUSERID,
COLUMN_NAME_REFRESHTOKEN,
COLUMN_NAME_SECRET,
COLUMN_NAME_UPDATEDBY,
COLUMN_NAME_UPDATEDDATE,
COLUMN_NAME_USERID,
COLUMN_NAME_USERROLES);
/** The list of attribute names */
static final List MANY_TO_ONE_NAMES = Arrays.asList();
// ----------------------- field finders -------------------------------
/**
* query-by method for field appArg0
* @param appArg0 the specified attribute
* @return an Iterable of DConnections for the specified appArg0
*/
Iterable queryByAppArg0(java.lang.String appArg0);
/**
* query-keys-by method for field appArg0
* @param appArg0 the specified attribute
* @return an Iterable of DConnections for the specified appArg0
*/
Iterable queryKeysByAppArg0(java.lang.String appArg0);
/**
* query-page-by method for field appArg0
* @param appArg0 the specified attribute
* @param pageSize the number of domain entities in the page
* @param cursorString non-null if get next page
* @return a Page of DConnections for the specified appArg0
*/
CursorPage queryPageByAppArg0(java.lang.String appArg0,
int pageSize, String cursorString);
/**
* query-by method for field createdBy
* @param createdBy the specified attribute
* @return an Iterable of DConnections for the specified createdBy
*/
Iterable queryByCreatedBy(java.lang.String createdBy);
/**
* query-keys-by method for field createdBy
* @param createdBy the specified attribute
* @return an Iterable of DConnections for the specified createdBy
*/
Iterable queryKeysByCreatedBy(java.lang.String createdBy);
/**
* query-page-by method for field createdBy
* @param createdBy the specified attribute
* @param pageSize the number of domain entities in the page
* @param cursorString non-null if get next page
* @return a Page of DConnections for the specified createdBy
*/
CursorPage queryPageByCreatedBy(java.lang.String createdBy,
int pageSize, String cursorString);
/**
* query-by method for field createdDate
* @param createdDate the specified attribute
* @return an Iterable of DConnections for the specified createdDate
*/
Iterable queryByCreatedDate(java.util.Date createdDate);
/**
* query-keys-by method for field createdDate
* @param createdDate the specified attribute
* @return an Iterable of DConnections for the specified createdDate
*/
Iterable queryKeysByCreatedDate(java.util.Date createdDate);
/**
* query-page-by method for field createdDate
* @param createdDate the specified attribute
* @param pageSize the number of domain entities in the page
* @param cursorString non-null if get next page
* @return a Page of DConnections for the specified createdDate
*/
CursorPage queryPageByCreatedDate(java.util.Date createdDate,
int pageSize, String cursorString);
/**
* query-by method for field displayName
* @param displayName the specified attribute
* @return an Iterable of DConnections for the specified displayName
*/
Iterable queryByDisplayName(java.lang.String displayName);
/**
* query-keys-by method for field displayName
* @param displayName the specified attribute
* @return an Iterable of DConnections for the specified displayName
*/
Iterable queryKeysByDisplayName(java.lang.String displayName);
/**
* query-page-by method for field displayName
* @param displayName the specified attribute
* @param pageSize the number of domain entities in the page
* @param cursorString non-null if get next page
* @return a Page of DConnections for the specified displayName
*/
CursorPage queryPageByDisplayName(java.lang.String displayName,
int pageSize, String cursorString);
/**
* query-by method for field expireTime
* @param expireTime the specified attribute
* @return an Iterable of DConnections for the specified expireTime
*/
Iterable queryByExpireTime(java.util.Date expireTime);
/**
* query-keys-by method for field expireTime
* @param expireTime the specified attribute
* @return an Iterable of DConnections for the specified expireTime
*/
Iterable queryKeysByExpireTime(java.util.Date expireTime);
/**
* query-page-by method for field expireTime
* @param expireTime the specified attribute
* @param pageSize the number of domain entities in the page
* @param cursorString non-null if get next page
* @return a Page of DConnections for the specified expireTime
*/
CursorPage queryPageByExpireTime(java.util.Date expireTime,
int pageSize, String cursorString);
/**
* query-by method for field imageUrl
* @param imageUrl the specified attribute
* @return an Iterable of DConnections for the specified imageUrl
*/
Iterable queryByImageUrl(java.lang.String imageUrl);
/**
* query-keys-by method for field imageUrl
* @param imageUrl the specified attribute
* @return an Iterable of DConnections for the specified imageUrl
*/
Iterable queryKeysByImageUrl(java.lang.String imageUrl);
/**
* query-page-by method for field imageUrl
* @param imageUrl the specified attribute
* @param pageSize the number of domain entities in the page
* @param cursorString non-null if get next page
* @return a Page of DConnections for the specified imageUrl
*/
CursorPage queryPageByImageUrl(java.lang.String imageUrl,
int pageSize, String cursorString);
/**
* query-by method for field profileUrl
* @param profileUrl the specified attribute
* @return an Iterable of DConnections for the specified profileUrl
*/
Iterable queryByProfileUrl(java.lang.String profileUrl);
/**
* query-keys-by method for field profileUrl
* @param profileUrl the specified attribute
* @return an Iterable of DConnections for the specified profileUrl
*/
Iterable queryKeysByProfileUrl(java.lang.String profileUrl);
/**
* query-page-by method for field profileUrl
* @param profileUrl the specified attribute
* @param pageSize the number of domain entities in the page
* @param cursorString non-null if get next page
* @return a Page of DConnections for the specified profileUrl
*/
CursorPage queryPageByProfileUrl(java.lang.String profileUrl,
int pageSize, String cursorString);
/**
* query-by method for field providerId
* @param providerId the specified attribute
* @return an Iterable of DConnections for the specified providerId
*/
Iterable queryByProviderId(java.lang.String providerId);
/**
* query-keys-by method for field providerId
* @param providerId the specified attribute
* @return an Iterable of DConnections for the specified providerId
*/
Iterable queryKeysByProviderId(java.lang.String providerId);
/**
* query-page-by method for field providerId
* @param providerId the specified attribute
* @param pageSize the number of domain entities in the page
* @param cursorString non-null if get next page
* @return a Page of DConnections for the specified providerId
*/
CursorPage queryPageByProviderId(java.lang.String providerId,
int pageSize, String cursorString);
/**
* query-by method for field providerUserId
* @param providerUserId the specified attribute
* @return an Iterable of DConnections for the specified providerUserId
*/
Iterable queryByProviderUserId(java.lang.String providerUserId);
/**
* query-keys-by method for field providerUserId
* @param providerUserId the specified attribute
* @return an Iterable of DConnections for the specified providerUserId
*/
Iterable queryKeysByProviderUserId(java.lang.String providerUserId);
/**
* query-page-by method for field providerUserId
* @param providerUserId the specified attribute
* @param pageSize the number of domain entities in the page
* @param cursorString non-null if get next page
* @return a Page of DConnections for the specified providerUserId
*/
CursorPage queryPageByProviderUserId(java.lang.String providerUserId,
int pageSize, String cursorString);
/**
* find-by method for unique field refreshToken
* @param refreshToken the unique attribute
* @return the unique DConnection for the specified refreshToken
*/
DConnection findByRefreshToken(java.lang.String refreshToken);
/**
* find-key-by method for unique attribute field refreshToken
* @param refreshToken the unique attribute
* @return the unique DConnection for the specified attribute
*/
java.lang.String findKeyByRefreshToken(java.lang.String refreshToken);
/**
* query-by method for field secret
* @param secret the specified attribute
* @return an Iterable of DConnections for the specified secret
*/
Iterable queryBySecret(java.lang.String secret);
/**
* query-keys-by method for field secret
* @param secret the specified attribute
* @return an Iterable of DConnections for the specified secret
*/
Iterable queryKeysBySecret(java.lang.String secret);
/**
* query-page-by method for field secret
* @param secret the specified attribute
* @param pageSize the number of domain entities in the page
* @param cursorString non-null if get next page
* @return a Page of DConnections for the specified secret
*/
CursorPage queryPageBySecret(java.lang.String secret,
int pageSize, String cursorString);
/**
* query-by method for field updatedBy
* @param updatedBy the specified attribute
* @return an Iterable of DConnections for the specified updatedBy
*/
Iterable queryByUpdatedBy(java.lang.String updatedBy);
/**
* query-keys-by method for field updatedBy
* @param updatedBy the specified attribute
* @return an Iterable of DConnections for the specified updatedBy
*/
Iterable queryKeysByUpdatedBy(java.lang.String updatedBy);
/**
* query-page-by method for field updatedBy
* @param updatedBy the specified attribute
* @param pageSize the number of domain entities in the page
* @param cursorString non-null if get next page
* @return a Page of DConnections for the specified updatedBy
*/
CursorPage queryPageByUpdatedBy(java.lang.String updatedBy,
int pageSize, String cursorString);
/**
* query-by method for field updatedDate
* @param updatedDate the specified attribute
* @return an Iterable of DConnections for the specified updatedDate
*/
Iterable queryByUpdatedDate(java.util.Date updatedDate);
/**
* query-keys-by method for field updatedDate
* @param updatedDate the specified attribute
* @return an Iterable of DConnections for the specified updatedDate
*/
Iterable queryKeysByUpdatedDate(java.util.Date updatedDate);
/**
* query-page-by method for field updatedDate
* @param updatedDate the specified attribute
* @param pageSize the number of domain entities in the page
* @param cursorString non-null if get next page
* @return a Page of DConnections for the specified updatedDate
*/
CursorPage queryPageByUpdatedDate(java.util.Date updatedDate,
int pageSize, String cursorString);
/**
* query-by method for field userId
* @param userId the specified attribute
* @return an Iterable of DConnections for the specified userId
*/
Iterable queryByUserId(java.lang.String userId);
/**
* query-keys-by method for field userId
* @param userId the specified attribute
* @return an Iterable of DConnections for the specified userId
*/
Iterable queryKeysByUserId(java.lang.String userId);
/**
* query-page-by method for field userId
* @param userId the specified attribute
* @param pageSize the number of domain entities in the page
* @param cursorString non-null if get next page
* @return a Page of DConnections for the specified userId
*/
CursorPage queryPageByUserId(java.lang.String userId,
int pageSize, String cursorString);
/**
* query-by method for field userRoles
* @param userRoles the specified attribute
* @return an Iterable of DConnections for the specified userRoles
*/
Iterable queryByUserRoles(java.lang.String userRoles);
/**
* query-keys-by method for field userRoles
* @param userRoles the specified attribute
* @return an Iterable of DConnections for the specified userRoles
*/
Iterable queryKeysByUserRoles(java.lang.String userRoles);
/**
* query-page-by method for field userRoles
* @param userRoles the specified attribute
* @param pageSize the number of domain entities in the page
* @param cursorString non-null if get next page
* @return a Page of DConnections for the specified userRoles
*/
CursorPage queryPageByUserRoles(java.lang.String userRoles,
int pageSize, String cursorString);
// ----------------------- one-to-one finders -------------------------
// ----------------------- many-to-one finders -------------------------
// ----------------------- many-to-many finders -------------------------
// ----------------------- uniqueFields finders -------------------------
// ----------------------- populate / persist method -------------------------
/**
* Persist an entity given all attributes
*/
DConnection persist( java.lang.String id,
java.lang.String appArg0,
java.lang.String displayName,
java.util.Date expireTime,
java.lang.String imageUrl,
java.lang.String profileUrl,
java.lang.String providerId,
java.lang.String providerUserId,
java.lang.String refreshToken,
java.lang.String secret,
java.lang.String userId,
java.lang.String userRoles);
/**
* Persists an entity unless it already exists
*/
DConnection persist(java.lang.String refreshToken,
java.lang.String appArg0,
java.lang.String displayName,
java.util.Date expireTime,
java.lang.String imageUrl,
java.lang.String profileUrl,
java.lang.String providerId,
java.lang.String providerUserId,
java.lang.String secret,
java.lang.String userId,
java.lang.String userRoles);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy