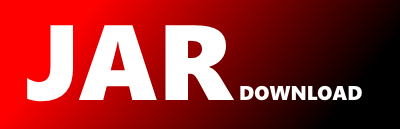
com.wadpam.open.dao.GeneratedDAppDomainDao Maven / Gradle / Ivy
package com.wadpam.open.dao;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import net.sf.mardao.core.CursorPage;
import net.sf.mardao.core.dao.Dao;
import com.wadpam.open.domain.DAppDomain;
import net.sf.mardao.core.geo.DLocation;
/**
* DAO interface with finder methods for DAppDomain entities.
*
* Generated on 2013-04-09T17:21:48.335+0700.
* @author mardao DAO generator (net.sf.mardao.plugin.ProcessDomainMojo)
*/
public interface GeneratedDAppDomainDao extends Dao {
/** Column name for primary key attribute is "id" */
static final String COLUMN_NAME_ID = "id";
/** Column name for field analyticsTrackingCode is "analyticsTrackingCode" */
static final String COLUMN_NAME_ANALYTICSTRACKINGCODE = "analyticsTrackingCode";
/** Column name for field appArg1 is "appArg1" */
static final String COLUMN_NAME_APPARG1 = "appArg1";
/** Column name for field appArg2 is "appArg2" */
static final String COLUMN_NAME_APPARG2 = "appArg2";
/** Column name for field createdBy is "createdBy" */
static final String COLUMN_NAME_CREATEDBY = "createdBy";
/** Column name for field createdDate is "createdDate" */
static final String COLUMN_NAME_CREATEDDATE = "createdDate";
/** Column name for field description is "description" */
static final String COLUMN_NAME_DESCRIPTION = "description";
/** Column name for field email is "email" */
static final String COLUMN_NAME_EMAIL = "email";
/** Column name for field password is "password" */
static final String COLUMN_NAME_PASSWORD = "password";
/** Column name for field updatedBy is "updatedBy" */
static final String COLUMN_NAME_UPDATEDBY = "updatedBy";
/** Column name for field updatedDate is "updatedDate" */
static final String COLUMN_NAME_UPDATEDDATE = "updatedDate";
/** Column name for field username is "username" */
static final String COLUMN_NAME_USERNAME = "username";
/** The list of attribute names */
static final List COLUMN_NAMES = Arrays.asList( COLUMN_NAME_ANALYTICSTRACKINGCODE,
COLUMN_NAME_APPARG1,
COLUMN_NAME_APPARG2,
COLUMN_NAME_CREATEDBY,
COLUMN_NAME_CREATEDDATE,
COLUMN_NAME_DESCRIPTION,
COLUMN_NAME_EMAIL,
COLUMN_NAME_PASSWORD,
COLUMN_NAME_UPDATEDBY,
COLUMN_NAME_UPDATEDDATE,
COLUMN_NAME_USERNAME);
/** The list of Basic attribute names */
static final List BASIC_NAMES = Arrays.asList( COLUMN_NAME_ANALYTICSTRACKINGCODE,
COLUMN_NAME_APPARG1,
COLUMN_NAME_APPARG2,
COLUMN_NAME_CREATEDBY,
COLUMN_NAME_CREATEDDATE,
COLUMN_NAME_DESCRIPTION,
COLUMN_NAME_EMAIL,
COLUMN_NAME_PASSWORD,
COLUMN_NAME_UPDATEDBY,
COLUMN_NAME_UPDATEDDATE,
COLUMN_NAME_USERNAME);
/** The list of attribute names */
static final List MANY_TO_ONE_NAMES = Arrays.asList();
// ----------------------- field finders -------------------------------
/**
* query-by method for field analyticsTrackingCode
* @param analyticsTrackingCode the specified attribute
* @return an Iterable of DAppDomains for the specified analyticsTrackingCode
*/
Iterable queryByAnalyticsTrackingCode(java.lang.String analyticsTrackingCode);
/**
* query-keys-by method for field analyticsTrackingCode
* @param analyticsTrackingCode the specified attribute
* @return an Iterable of DAppDomains for the specified analyticsTrackingCode
*/
Iterable queryKeysByAnalyticsTrackingCode(java.lang.String analyticsTrackingCode);
/**
* query-page-by method for field analyticsTrackingCode
* @param analyticsTrackingCode the specified attribute
* @param pageSize the number of domain entities in the page
* @param cursorString non-null if get next page
* @return a Page of DAppDomains for the specified analyticsTrackingCode
*/
CursorPage queryPageByAnalyticsTrackingCode(java.lang.String analyticsTrackingCode,
int pageSize, String cursorString);
/**
* query-by method for field appArg1
* @param appArg1 the specified attribute
* @return an Iterable of DAppDomains for the specified appArg1
*/
Iterable queryByAppArg1(java.lang.String appArg1);
/**
* query-keys-by method for field appArg1
* @param appArg1 the specified attribute
* @return an Iterable of DAppDomains for the specified appArg1
*/
Iterable queryKeysByAppArg1(java.lang.String appArg1);
/**
* query-page-by method for field appArg1
* @param appArg1 the specified attribute
* @param pageSize the number of domain entities in the page
* @param cursorString non-null if get next page
* @return a Page of DAppDomains for the specified appArg1
*/
CursorPage queryPageByAppArg1(java.lang.String appArg1,
int pageSize, String cursorString);
/**
* query-by method for field appArg2
* @param appArg2 the specified attribute
* @return an Iterable of DAppDomains for the specified appArg2
*/
Iterable queryByAppArg2(java.lang.String appArg2);
/**
* query-keys-by method for field appArg2
* @param appArg2 the specified attribute
* @return an Iterable of DAppDomains for the specified appArg2
*/
Iterable queryKeysByAppArg2(java.lang.String appArg2);
/**
* query-page-by method for field appArg2
* @param appArg2 the specified attribute
* @param pageSize the number of domain entities in the page
* @param cursorString non-null if get next page
* @return a Page of DAppDomains for the specified appArg2
*/
CursorPage queryPageByAppArg2(java.lang.String appArg2,
int pageSize, String cursorString);
/**
* query-by method for field createdBy
* @param createdBy the specified attribute
* @return an Iterable of DAppDomains for the specified createdBy
*/
Iterable queryByCreatedBy(java.lang.String createdBy);
/**
* query-keys-by method for field createdBy
* @param createdBy the specified attribute
* @return an Iterable of DAppDomains for the specified createdBy
*/
Iterable queryKeysByCreatedBy(java.lang.String createdBy);
/**
* query-page-by method for field createdBy
* @param createdBy the specified attribute
* @param pageSize the number of domain entities in the page
* @param cursorString non-null if get next page
* @return a Page of DAppDomains for the specified createdBy
*/
CursorPage queryPageByCreatedBy(java.lang.String createdBy,
int pageSize, String cursorString);
/**
* query-by method for field createdDate
* @param createdDate the specified attribute
* @return an Iterable of DAppDomains for the specified createdDate
*/
Iterable queryByCreatedDate(java.util.Date createdDate);
/**
* query-keys-by method for field createdDate
* @param createdDate the specified attribute
* @return an Iterable of DAppDomains for the specified createdDate
*/
Iterable queryKeysByCreatedDate(java.util.Date createdDate);
/**
* query-page-by method for field createdDate
* @param createdDate the specified attribute
* @param pageSize the number of domain entities in the page
* @param cursorString non-null if get next page
* @return a Page of DAppDomains for the specified createdDate
*/
CursorPage queryPageByCreatedDate(java.util.Date createdDate,
int pageSize, String cursorString);
/**
* query-by method for field description
* @param description the specified attribute
* @return an Iterable of DAppDomains for the specified description
*/
Iterable queryByDescription(java.lang.String description);
/**
* query-keys-by method for field description
* @param description the specified attribute
* @return an Iterable of DAppDomains for the specified description
*/
Iterable queryKeysByDescription(java.lang.String description);
/**
* query-page-by method for field description
* @param description the specified attribute
* @param pageSize the number of domain entities in the page
* @param cursorString non-null if get next page
* @return a Page of DAppDomains for the specified description
*/
CursorPage queryPageByDescription(java.lang.String description,
int pageSize, String cursorString);
/**
* query-by method for field email
* @param email the specified attribute
* @return an Iterable of DAppDomains for the specified email
*/
Iterable queryByEmail(com.google.appengine.api.datastore.Email email);
/**
* query-keys-by method for field email
* @param email the specified attribute
* @return an Iterable of DAppDomains for the specified email
*/
Iterable queryKeysByEmail(com.google.appengine.api.datastore.Email email);
/**
* query-page-by method for field email
* @param email the specified attribute
* @param pageSize the number of domain entities in the page
* @param cursorString non-null if get next page
* @return a Page of DAppDomains for the specified email
*/
CursorPage queryPageByEmail(com.google.appengine.api.datastore.Email email,
int pageSize, String cursorString);
/**
* query-by method for field password
* @param password the specified attribute
* @return an Iterable of DAppDomains for the specified password
*/
Iterable queryByPassword(java.lang.String password);
/**
* query-keys-by method for field password
* @param password the specified attribute
* @return an Iterable of DAppDomains for the specified password
*/
Iterable queryKeysByPassword(java.lang.String password);
/**
* query-page-by method for field password
* @param password the specified attribute
* @param pageSize the number of domain entities in the page
* @param cursorString non-null if get next page
* @return a Page of DAppDomains for the specified password
*/
CursorPage queryPageByPassword(java.lang.String password,
int pageSize, String cursorString);
/**
* query-by method for field updatedBy
* @param updatedBy the specified attribute
* @return an Iterable of DAppDomains for the specified updatedBy
*/
Iterable queryByUpdatedBy(java.lang.String updatedBy);
/**
* query-keys-by method for field updatedBy
* @param updatedBy the specified attribute
* @return an Iterable of DAppDomains for the specified updatedBy
*/
Iterable queryKeysByUpdatedBy(java.lang.String updatedBy);
/**
* query-page-by method for field updatedBy
* @param updatedBy the specified attribute
* @param pageSize the number of domain entities in the page
* @param cursorString non-null if get next page
* @return a Page of DAppDomains for the specified updatedBy
*/
CursorPage queryPageByUpdatedBy(java.lang.String updatedBy,
int pageSize, String cursorString);
/**
* query-by method for field updatedDate
* @param updatedDate the specified attribute
* @return an Iterable of DAppDomains for the specified updatedDate
*/
Iterable queryByUpdatedDate(java.util.Date updatedDate);
/**
* query-keys-by method for field updatedDate
* @param updatedDate the specified attribute
* @return an Iterable of DAppDomains for the specified updatedDate
*/
Iterable queryKeysByUpdatedDate(java.util.Date updatedDate);
/**
* query-page-by method for field updatedDate
* @param updatedDate the specified attribute
* @param pageSize the number of domain entities in the page
* @param cursorString non-null if get next page
* @return a Page of DAppDomains for the specified updatedDate
*/
CursorPage queryPageByUpdatedDate(java.util.Date updatedDate,
int pageSize, String cursorString);
/**
* query-by method for field username
* @param username the specified attribute
* @return an Iterable of DAppDomains for the specified username
*/
Iterable queryByUsername(java.lang.String username);
/**
* query-keys-by method for field username
* @param username the specified attribute
* @return an Iterable of DAppDomains for the specified username
*/
Iterable queryKeysByUsername(java.lang.String username);
/**
* query-page-by method for field username
* @param username the specified attribute
* @param pageSize the number of domain entities in the page
* @param cursorString non-null if get next page
* @return a Page of DAppDomains for the specified username
*/
CursorPage queryPageByUsername(java.lang.String username,
int pageSize, String cursorString);
// ----------------------- one-to-one finders -------------------------
// ----------------------- many-to-one finders -------------------------
// ----------------------- many-to-many finders -------------------------
// ----------------------- uniqueFields finders -------------------------
// ----------------------- populate / persist method -------------------------
/**
* Persist an entity given all attributes
*/
DAppDomain persist( java.lang.String id,
java.lang.String analyticsTrackingCode,
java.lang.String appArg1,
java.lang.String appArg2,
java.lang.String description,
com.google.appengine.api.datastore.Email email,
java.lang.String password,
java.lang.String username);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy