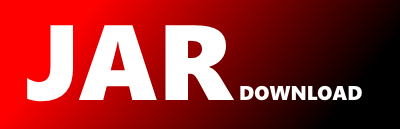
com.wadpam.open.io.JsonConverter Maven / Gradle / Ivy
package com.wadpam.open.io;
import com.wadpam.open.json.SkipNullObjectMapper;
import java.io.IOException;
import java.io.OutputStream;
import java.io.PrintWriter;
import java.util.Map;
import org.codehaus.jackson.map.ObjectMapper;
/**
*
* @author os
*/
public class JsonConverter implements Converter {
private final ThreadLocal pw = new ThreadLocal();
private final ThreadLocal perDao = new ThreadLocal();
private final ObjectMapper MAPPER = new SkipNullObjectMapper();
@Override
public Object preExport(OutputStream out, Object arg, Object preExport, D[] daos) {
pw.set(new PrintWriter(out));
final String s = "{[";
pw.get().println(s);
return s;
}
@Override
public Object postExport(OutputStream out, Object arg, Object preExport, Object postExport, D[] daos) {
final String s = String.format("]}");
pw.get().println(s);
pw.get().flush();
pw.remove();
return s;
}
@Override
public Object initPreDao(OutputStream out, Object arg) {
return null;
}
@Override
public Object preDao(OutputStream out, Object arg, Object preExport, Object preDao,
String tableName, Iterable columns, Map headers,
int daoIndex, D dao) {
perDao.set(null == pw.get());
if (perDao.get()) {
pw.set(new PrintWriter(out));
}
final String s = String.format("%s{\"tableName\":\"%s\", \"entities\":[",
0 < daoIndex ? "," : "",
tableName);
pw.get().println(s);
return s;
}
@Override
public Object postDao(OutputStream out, Object arg, Object preExport, Object preDao, Object postDao, D dao) {
final String s = String.format("]}");
pw.get().println(s);
pw.get().flush();
if (perDao.get()) {
pw.remove();
}
return s;
}
@Override
public Object writeValues(OutputStream out, Object arg, Object preExport, Object preDao,
Iterable columns, int daoIndex, D dao, int entityIndex, Object entity,
Map values) {
String s = null;
// skip this entity / row ?
if (null != values) {
StringBuffer sb = new StringBuffer();
try {
// prefix with comma?
if (0 < entityIndex) {
sb.append(',');
}
sb.append(MAPPER.writeValueAsString(values));
s = sb.toString();
} catch (IOException ex) {
}
pw.get().println(s);
}
return s;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy