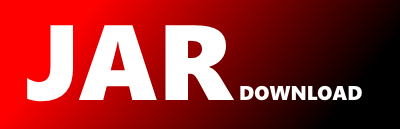
com.wallee.sdk.service.SubscriptionService Maven / Gradle / Ivy
Show all versions of wallee-java-sdk Show documentation
package com.wallee.sdk.service;
import static com.wallee.sdk.ErrorCode.*;
import com.wallee.sdk.ApiClient;
import com.wallee.sdk.ErrorCode;
import com.wallee.sdk.exception.WalleeSdkException;
import com.wallee.sdk.util.URIBuilderUtil;
import com.wallee.sdk.StringUtil;
import com.wallee.sdk.model.ClientError;
import com.wallee.sdk.model.EntityQuery;
import com.wallee.sdk.model.EntityQueryFilter;
import com.wallee.sdk.model.ServerError;
import com.wallee.sdk.model.Subscription;
import com.wallee.sdk.model.SubscriptionChangeRequest;
import com.wallee.sdk.model.SubscriptionCharge;
import com.wallee.sdk.model.SubscriptionCreateRequest;
import com.wallee.sdk.model.SubscriptionUpdateRequest;
import com.wallee.sdk.model.SubscriptionVersion;
import com.wallee.sdk.model.TransactionInvoice;
import com.fasterxml.jackson.core.type.TypeReference;
import com.google.api.client.http.*;
import com.google.api.client.json.Json;
import org.apache.http.client.utils.URIBuilder;
import java.io.IOException;
import java.util.Collection;
import java.util.HashMap;
import java.util.Map;
import java.util.List;
import java.util.ArrayList;
import java.util.Objects;
public class SubscriptionService {
private ApiClient apiClient;
public SubscriptionService(ApiClient apiClient) {
this.apiClient = Objects.requireNonNull(apiClient, "ApiClient must be non null");
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = Objects.requireNonNull(apiClient, "ApiClient must be non null");
}
/**
* apply changes
* This operation allows to apply changes on a subscription.
* 200 - This status code indicates that a client request was successfully received, understood, and accepted.
*
409 - This status code indicates that there was a conflict with the current version of the data in the database and the provided data in the request.
*
442 - This status code indicates that the server cannot or will not process the request due to something that is perceived to be a client error.
*
542 - This status code indicates that the server encountered an unexpected condition that prevented it from fulfilling the client request.
* @param spaceId
* @param request
* @return SubscriptionVersion
* @throws IOException if an error occurs while attempting to invoke the API
* For more information visit this link.
* @see apply changes Documentation
**/
public SubscriptionVersion applyChanges(Long spaceId, SubscriptionChangeRequest request) throws IOException {
HttpResponse response = applyChangesForHttpResponse(spaceId, request);
String returnType = "SubscriptionVersion";
if(returnType.equals("String")){
return (SubscriptionVersion) (Object) response.parseAsString();
}
TypeReference typeRef = new TypeReference() {};
if (isNoBodyResponse(response)) {
throw new WalleeSdkException(ErrorCode.ENTITY_NOT_FOUND, "Entity was not found for: " + typeRef.getType().getTypeName());
}
return (SubscriptionVersion)apiClient.getObjectMapper().readValue(response.getContent(), typeRef);
}
/**
* apply changes
* This operation allows to apply changes on a subscription.
* 200 - This status code indicates that a client request was successfully received, understood, and accepted.
*
409 - This status code indicates that there was a conflict with the current version of the data in the database and the provided data in the request.
*
442 - This status code indicates that the server cannot or will not process the request due to something that is perceived to be a client error.
*
542 - This status code indicates that the server encountered an unexpected condition that prevented it from fulfilling the client request.
* @param spaceId
* @param request
* @param params Map of query params. A collection will be interpreted as passing in multiple instances of the same query param.
* @return SubscriptionVersion
* @throws IOException if an error occurs while attempting to invoke the API
* For more information visit this link.
* @see apply changes Documentation
**/
public SubscriptionVersion applyChanges(Long spaceId, SubscriptionChangeRequest request, Map params) throws IOException {
HttpResponse response = applyChangesForHttpResponse(spaceId, request, params);
String returnType = "SubscriptionVersion";
if(returnType.equals("String")){
return (SubscriptionVersion) (Object) response.parseAsString();
}
TypeReference typeRef = new TypeReference() {};
if (isNoBodyResponse(response)) {
throw new WalleeSdkException(ErrorCode.ENTITY_NOT_FOUND, "Entity was not found for: " + typeRef.getType().getTypeName());
}
return (SubscriptionVersion)apiClient.getObjectMapper().readValue(response.getContent(), typeRef);
}
public HttpResponse applyChangesForHttpResponse(Long spaceId, SubscriptionChangeRequest request) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling applyChanges");
}
// verify the required parameter 'request' is set
if (request == null) {
throw new IllegalArgumentException("Missing the required parameter 'request' when calling applyChanges");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/subscription/applyChanges");
if (spaceId != null) {
String key = "spaceId";
Object value = spaceId;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = apiClient.new JacksonJsonHttpContent(request);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
public HttpResponse applyChangesForHttpResponse(Long spaceId, java.io.InputStream request, String mediaType) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling applyChanges");
}
// verify the required parameter 'request' is set
if (request == null) {
throw new IllegalArgumentException("Missing the required parameter 'request' when calling applyChanges");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/subscription/applyChanges");
if (spaceId != null) {
String key = "spaceId";
Object value = spaceId;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = request == null ?
apiClient.new JacksonJsonHttpContent(null) :
new InputStreamContent(mediaType == null ? Json.MEDIA_TYPE : mediaType, request);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
public HttpResponse applyChangesForHttpResponse(Long spaceId, SubscriptionChangeRequest request, Map params) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling applyChanges");
}
// verify the required parameter 'request' is set
if (request == null) {
throw new IllegalArgumentException("Missing the required parameter 'request' when calling applyChanges");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/subscription/applyChanges");
// Copy the params argument if present, to allow passing in immutable maps
Map allParams = params == null ? new HashMap() : new HashMap(params);
// Add the required query param 'spaceId' to the map of query params
allParams.put("spaceId", spaceId);
for (Map.Entry entryMap: allParams.entrySet()) {
String key = entryMap.getKey();
Object value = entryMap.getValue();
if (key != null && value != null) {
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = apiClient.new JacksonJsonHttpContent(request);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
/**
* Count
* Counts the number of items in the database as restricted by the given filter.
* 200 - This status code indicates that a client request was successfully received, understood, and accepted.
*
442 - This status code indicates that the server cannot or will not process the request due to something that is perceived to be a client error.
*
542 - This status code indicates that the server encountered an unexpected condition that prevented it from fulfilling the client request.
* @param spaceId
* @param filter The filter which restricts the entities which are used to calculate the count.
* @return Long
* @throws IOException if an error occurs while attempting to invoke the API
* For more information visit this link.
* @see Count Documentation
**/
public Long count(Long spaceId, EntityQueryFilter filter) throws IOException {
HttpResponse response = countForHttpResponse(spaceId, filter);
String returnType = "Long";
if(returnType.equals("String")){
return (Long) (Object) response.parseAsString();
}
TypeReference typeRef = new TypeReference() {};
if (isNoBodyResponse(response)) {
throw new WalleeSdkException(ErrorCode.ENTITY_NOT_FOUND, "Entity was not found for: " + typeRef.getType().getTypeName());
}
return (Long)apiClient.getObjectMapper().readValue(response.getContent(), typeRef);
}
/**
* Count
* Counts the number of items in the database as restricted by the given filter.
* 200 - This status code indicates that a client request was successfully received, understood, and accepted.
*
442 - This status code indicates that the server cannot or will not process the request due to something that is perceived to be a client error.
*
542 - This status code indicates that the server encountered an unexpected condition that prevented it from fulfilling the client request.
* @param spaceId
* @param params Map of query params. A collection will be interpreted as passing in multiple instances of the same query param.
* @return Long
* @throws IOException if an error occurs while attempting to invoke the API
* For more information visit this link.
* @see Count Documentation
**/
public Long count(EntityQueryFilter filter, Long spaceId, Map params) throws IOException {
HttpResponse response = countForHttpResponse(filter, spaceId, params);
String returnType = "Long";
if(returnType.equals("String")){
return (Long) (Object) response.parseAsString();
}
TypeReference typeRef = new TypeReference() {};
if (isNoBodyResponse(response)) {
throw new WalleeSdkException(ErrorCode.ENTITY_NOT_FOUND, "Entity was not found for: " + typeRef.getType().getTypeName());
}
return (Long)apiClient.getObjectMapper().readValue(response.getContent(), typeRef);
}
public HttpResponse countForHttpResponse(Long spaceId, EntityQueryFilter filter) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling count");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/subscription/count");
if (spaceId != null) {
String key = "spaceId";
Object value = spaceId;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = apiClient.new JacksonJsonHttpContent(filter);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
public HttpResponse countForHttpResponse(Long spaceId, java.io.InputStream filter, String mediaType) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling count");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/subscription/count");
if (spaceId != null) {
String key = "spaceId";
Object value = spaceId;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = filter == null ?
apiClient.new JacksonJsonHttpContent(null) :
new InputStreamContent(mediaType == null ? Json.MEDIA_TYPE : mediaType, filter);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
public HttpResponse countForHttpResponse(EntityQueryFilter filter, Long spaceId, Map params) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling count");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/subscription/count");
// Copy the params argument if present, to allow passing in immutable maps
Map allParams = params == null ? new HashMap() : new HashMap(params);
// Add the required query param 'spaceId' to the map of query params
allParams.put("spaceId", spaceId);
for (Map.Entry entryMap: allParams.entrySet()) {
String key = entryMap.getKey();
Object value = entryMap.getValue();
if (key != null && value != null) {
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = apiClient.new JacksonJsonHttpContent(filter);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
/**
* Create
* The create operation creates a new subscription and a corresponding subscription version.
* 200 - This status code indicates that a client request was successfully received, understood, and accepted.
*
442 - This status code indicates that the server cannot or will not process the request due to something that is perceived to be a client error.
*
542 - This status code indicates that the server encountered an unexpected condition that prevented it from fulfilling the client request.
* @param spaceId
* @param createRequest
* @return SubscriptionVersion
* @throws IOException if an error occurs while attempting to invoke the API
* For more information visit this link.
* @see Create Documentation
**/
public SubscriptionVersion create(Long spaceId, SubscriptionCreateRequest createRequest) throws IOException {
HttpResponse response = createForHttpResponse(spaceId, createRequest);
String returnType = "SubscriptionVersion";
if(returnType.equals("String")){
return (SubscriptionVersion) (Object) response.parseAsString();
}
TypeReference typeRef = new TypeReference() {};
if (isNoBodyResponse(response)) {
throw new WalleeSdkException(ErrorCode.ENTITY_NOT_FOUND, "Entity was not found for: " + typeRef.getType().getTypeName());
}
return (SubscriptionVersion)apiClient.getObjectMapper().readValue(response.getContent(), typeRef);
}
/**
* Create
* The create operation creates a new subscription and a corresponding subscription version.
* 200 - This status code indicates that a client request was successfully received, understood, and accepted.
*
442 - This status code indicates that the server cannot or will not process the request due to something that is perceived to be a client error.
*
542 - This status code indicates that the server encountered an unexpected condition that prevented it from fulfilling the client request.
* @param spaceId
* @param createRequest
* @param params Map of query params. A collection will be interpreted as passing in multiple instances of the same query param.
* @return SubscriptionVersion
* @throws IOException if an error occurs while attempting to invoke the API
* For more information visit this link.
* @see Create Documentation
**/
public SubscriptionVersion create(Long spaceId, SubscriptionCreateRequest createRequest, Map params) throws IOException {
HttpResponse response = createForHttpResponse(spaceId, createRequest, params);
String returnType = "SubscriptionVersion";
if(returnType.equals("String")){
return (SubscriptionVersion) (Object) response.parseAsString();
}
TypeReference typeRef = new TypeReference() {};
if (isNoBodyResponse(response)) {
throw new WalleeSdkException(ErrorCode.ENTITY_NOT_FOUND, "Entity was not found for: " + typeRef.getType().getTypeName());
}
return (SubscriptionVersion)apiClient.getObjectMapper().readValue(response.getContent(), typeRef);
}
public HttpResponse createForHttpResponse(Long spaceId, SubscriptionCreateRequest createRequest) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling create");
}
// verify the required parameter 'createRequest' is set
if (createRequest == null) {
throw new IllegalArgumentException("Missing the required parameter 'createRequest' when calling create");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/subscription/create");
if (spaceId != null) {
String key = "spaceId";
Object value = spaceId;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = apiClient.new JacksonJsonHttpContent(createRequest);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
public HttpResponse createForHttpResponse(Long spaceId, java.io.InputStream createRequest, String mediaType) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling create");
}
// verify the required parameter 'createRequest' is set
if (createRequest == null) {
throw new IllegalArgumentException("Missing the required parameter 'createRequest' when calling create");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/subscription/create");
if (spaceId != null) {
String key = "spaceId";
Object value = spaceId;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = createRequest == null ?
apiClient.new JacksonJsonHttpContent(null) :
new InputStreamContent(mediaType == null ? Json.MEDIA_TYPE : mediaType, createRequest);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
public HttpResponse createForHttpResponse(Long spaceId, SubscriptionCreateRequest createRequest, Map params) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling create");
}
// verify the required parameter 'createRequest' is set
if (createRequest == null) {
throw new IllegalArgumentException("Missing the required parameter 'createRequest' when calling create");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/subscription/create");
// Copy the params argument if present, to allow passing in immutable maps
Map allParams = params == null ? new HashMap() : new HashMap(params);
// Add the required query param 'spaceId' to the map of query params
allParams.put("spaceId", spaceId);
for (Map.Entry entryMap: allParams.entrySet()) {
String key = entryMap.getKey();
Object value = entryMap.getValue();
if (key != null && value != null) {
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = apiClient.new JacksonJsonHttpContent(createRequest);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
/**
* initialize
* The initialize operation initializes a subscription. This method uses charge flows to carry out the transaction.
* 200 - This status code indicates that a client request was successfully received, understood, and accepted.
*
442 - This status code indicates that the server cannot or will not process the request due to something that is perceived to be a client error.
*
542 - This status code indicates that the server encountered an unexpected condition that prevented it from fulfilling the client request.
* @param spaceId
* @param subscriptionId The provided subscription id will be used to lookup the subscription which should be initialized.
* @return SubscriptionCharge
* @throws IOException if an error occurs while attempting to invoke the API
* For more information visit this link.
* @see initialize Documentation
**/
public SubscriptionCharge initialize(Long spaceId, Long subscriptionId) throws IOException {
HttpResponse response = initializeForHttpResponse(spaceId, subscriptionId);
String returnType = "SubscriptionCharge";
if(returnType.equals("String")){
return (SubscriptionCharge) (Object) response.parseAsString();
}
TypeReference typeRef = new TypeReference() {};
if (isNoBodyResponse(response)) {
throw new WalleeSdkException(ErrorCode.ENTITY_NOT_FOUND, "Entity was not found for: " + typeRef.getType().getTypeName());
}
return (SubscriptionCharge)apiClient.getObjectMapper().readValue(response.getContent(), typeRef);
}
/**
* initialize
* The initialize operation initializes a subscription. This method uses charge flows to carry out the transaction.
* 200 - This status code indicates that a client request was successfully received, understood, and accepted.
*
442 - This status code indicates that the server cannot or will not process the request due to something that is perceived to be a client error.
*
542 - This status code indicates that the server encountered an unexpected condition that prevented it from fulfilling the client request.
* @param spaceId
* @param subscriptionId The provided subscription id will be used to lookup the subscription which should be initialized.
* @param params Map of query params. A collection will be interpreted as passing in multiple instances of the same query param.
* @return SubscriptionCharge
* @throws IOException if an error occurs while attempting to invoke the API
* For more information visit this link.
* @see initialize Documentation
**/
public SubscriptionCharge initialize(Long spaceId, Long subscriptionId, Map params) throws IOException {
HttpResponse response = initializeForHttpResponse(spaceId, subscriptionId, params);
String returnType = "SubscriptionCharge";
if(returnType.equals("String")){
return (SubscriptionCharge) (Object) response.parseAsString();
}
TypeReference typeRef = new TypeReference() {};
if (isNoBodyResponse(response)) {
throw new WalleeSdkException(ErrorCode.ENTITY_NOT_FOUND, "Entity was not found for: " + typeRef.getType().getTypeName());
}
return (SubscriptionCharge)apiClient.getObjectMapper().readValue(response.getContent(), typeRef);
}
public HttpResponse initializeForHttpResponse(Long spaceId, Long subscriptionId) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling initialize");
}
// verify the required parameter 'subscriptionId' is set
if (subscriptionId == null) {
throw new IllegalArgumentException("Missing the required parameter 'subscriptionId' when calling initialize");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/subscription/initialize");
if (spaceId != null) {
String key = "spaceId";
Object value = spaceId;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
if (subscriptionId != null) {
String key = "subscriptionId";
Object value = subscriptionId;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = apiClient.new JacksonJsonHttpContent(null);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
public HttpResponse initializeForHttpResponse(Long spaceId, Long subscriptionId, Map params) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling initialize");
}
// verify the required parameter 'subscriptionId' is set
if (subscriptionId == null) {
throw new IllegalArgumentException("Missing the required parameter 'subscriptionId' when calling initialize");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/subscription/initialize");
// Copy the params argument if present, to allow passing in immutable maps
Map allParams = params == null ? new HashMap() : new HashMap(params);
// Add the required query param 'spaceId' to the map of query params
allParams.put("spaceId", spaceId);
// Add the required query param 'subscriptionId' to the map of query params
allParams.put("subscriptionId", subscriptionId);
for (Map.Entry entryMap: allParams.entrySet()) {
String key = entryMap.getKey();
Object value = entryMap.getValue();
if (key != null && value != null) {
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = apiClient.new JacksonJsonHttpContent(null);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
/**
* initializeSubscriberPresent
* The initialize operation initializes a subscription when the subscriber is present. The method will initialize a transaction which has to be completed by using the transaction service.
* 200 - This status code indicates that a client request was successfully received, understood, and accepted.
*
409 - This status code indicates that there was a conflict with the current version of the data in the database and the provided data in the request.
*
442 - This status code indicates that the server cannot or will not process the request due to something that is perceived to be a client error.
*
542 - This status code indicates that the server encountered an unexpected condition that prevented it from fulfilling the client request.
* @param spaceId
* @param subscriptionId
* @param successUrl The subscriber will be redirected to the success URL when the transaction is successful.
* @param failedUrl The subscriber will be redirected to the fail URL when the transaction fails.
* @return SubscriptionCharge
* @throws IOException if an error occurs while attempting to invoke the API
* For more information visit this link.
* @see initializeSubscriberPresent Documentation
**/
public SubscriptionCharge initializeSubscriberPresent(Long spaceId, Long subscriptionId, String successUrl, String failedUrl) throws IOException {
HttpResponse response = initializeSubscriberPresentForHttpResponse(spaceId, subscriptionId, successUrl, failedUrl);
String returnType = "SubscriptionCharge";
if(returnType.equals("String")){
return (SubscriptionCharge) (Object) response.parseAsString();
}
TypeReference typeRef = new TypeReference() {};
if (isNoBodyResponse(response)) {
throw new WalleeSdkException(ErrorCode.ENTITY_NOT_FOUND, "Entity was not found for: " + typeRef.getType().getTypeName());
}
return (SubscriptionCharge)apiClient.getObjectMapper().readValue(response.getContent(), typeRef);
}
/**
* initializeSubscriberPresent
* The initialize operation initializes a subscription when the subscriber is present. The method will initialize a transaction which has to be completed by using the transaction service.
* 200 - This status code indicates that a client request was successfully received, understood, and accepted.
*
409 - This status code indicates that there was a conflict with the current version of the data in the database and the provided data in the request.
*
442 - This status code indicates that the server cannot or will not process the request due to something that is perceived to be a client error.
*
542 - This status code indicates that the server encountered an unexpected condition that prevented it from fulfilling the client request.
* @param spaceId
* @param subscriptionId
* @param params Map of query params. A collection will be interpreted as passing in multiple instances of the same query param.
* @return SubscriptionCharge
* @throws IOException if an error occurs while attempting to invoke the API
* For more information visit this link.
* @see initializeSubscriberPresent Documentation
**/
public SubscriptionCharge initializeSubscriberPresent(Long spaceId, Long subscriptionId, Map params) throws IOException {
HttpResponse response = initializeSubscriberPresentForHttpResponse(spaceId, subscriptionId, params);
String returnType = "SubscriptionCharge";
if(returnType.equals("String")){
return (SubscriptionCharge) (Object) response.parseAsString();
}
TypeReference typeRef = new TypeReference() {};
if (isNoBodyResponse(response)) {
throw new WalleeSdkException(ErrorCode.ENTITY_NOT_FOUND, "Entity was not found for: " + typeRef.getType().getTypeName());
}
return (SubscriptionCharge)apiClient.getObjectMapper().readValue(response.getContent(), typeRef);
}
public HttpResponse initializeSubscriberPresentForHttpResponse(Long spaceId, Long subscriptionId, String successUrl, String failedUrl) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling initializeSubscriberPresent");
}
// verify the required parameter 'subscriptionId' is set
if (subscriptionId == null) {
throw new IllegalArgumentException("Missing the required parameter 'subscriptionId' when calling initializeSubscriberPresent");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/subscription/initializeSubscriberPresent");
if (spaceId != null) {
String key = "spaceId";
Object value = spaceId;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
if (subscriptionId != null) {
String key = "subscriptionId";
Object value = subscriptionId;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
if (successUrl != null) {
String key = "successUrl";
Object value = successUrl;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
if (failedUrl != null) {
String key = "failedUrl";
Object value = failedUrl;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = apiClient.new JacksonJsonHttpContent(null);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
public HttpResponse initializeSubscriberPresentForHttpResponse(Long spaceId, Long subscriptionId, Map params) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling initializeSubscriberPresent");
}
// verify the required parameter 'subscriptionId' is set
if (subscriptionId == null) {
throw new IllegalArgumentException("Missing the required parameter 'subscriptionId' when calling initializeSubscriberPresent");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/subscription/initializeSubscriberPresent");
// Copy the params argument if present, to allow passing in immutable maps
Map allParams = params == null ? new HashMap() : new HashMap(params);
// Add the required query param 'spaceId' to the map of query params
allParams.put("spaceId", spaceId);
// Add the required query param 'subscriptionId' to the map of query params
allParams.put("subscriptionId", subscriptionId);
for (Map.Entry entryMap: allParams.entrySet()) {
String key = entryMap.getKey();
Object value = entryMap.getValue();
if (key != null && value != null) {
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = apiClient.new JacksonJsonHttpContent(null);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
/**
* Read
* Reads the entity with the given 'id' and returns it.
* 200 - This status code indicates that a client request was successfully received, understood, and accepted.
*
442 - This status code indicates that the server cannot or will not process the request due to something that is perceived to be a client error.
*
542 - This status code indicates that the server encountered an unexpected condition that prevented it from fulfilling the client request.
* @param spaceId
* @param id The id of the subscription which should be returned.
* @return Subscription
* @throws IOException if an error occurs while attempting to invoke the API
* For more information visit this link.
* @see Read Documentation
**/
public Subscription read(Long spaceId, Long id) throws IOException {
HttpResponse response = readForHttpResponse(spaceId, id);
String returnType = "Subscription";
if(returnType.equals("String")){
return (Subscription) (Object) response.parseAsString();
}
TypeReference typeRef = new TypeReference() {};
if (isNoBodyResponse(response)) {
throw new WalleeSdkException(ErrorCode.ENTITY_NOT_FOUND, "Entity was not found for: " + typeRef.getType().getTypeName());
}
return (Subscription)apiClient.getObjectMapper().readValue(response.getContent(), typeRef);
}
/**
* Read
* Reads the entity with the given 'id' and returns it.
* 200 - This status code indicates that a client request was successfully received, understood, and accepted.
*
442 - This status code indicates that the server cannot or will not process the request due to something that is perceived to be a client error.
*
542 - This status code indicates that the server encountered an unexpected condition that prevented it from fulfilling the client request.
* @param spaceId
* @param id The id of the subscription which should be returned.
* @param params Map of query params. A collection will be interpreted as passing in multiple instances of the same query param.
* @return Subscription
* @throws IOException if an error occurs while attempting to invoke the API
* For more information visit this link.
* @see Read Documentation
**/
public Subscription read(Long spaceId, Long id, Map params) throws IOException {
HttpResponse response = readForHttpResponse(spaceId, id, params);
String returnType = "Subscription";
if(returnType.equals("String")){
return (Subscription) (Object) response.parseAsString();
}
TypeReference typeRef = new TypeReference() {};
if (isNoBodyResponse(response)) {
throw new WalleeSdkException(ErrorCode.ENTITY_NOT_FOUND, "Entity was not found for: " + typeRef.getType().getTypeName());
}
return (Subscription)apiClient.getObjectMapper().readValue(response.getContent(), typeRef);
}
public HttpResponse readForHttpResponse(Long spaceId, Long id) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling read");
}
// verify the required parameter 'id' is set
if (id == null) {
throw new IllegalArgumentException("Missing the required parameter 'id' when calling read");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/subscription/read");
if (spaceId != null) {
String key = "spaceId";
Object value = spaceId;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
if (id != null) {
String key = "id";
Object value = id;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = null;
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.GET, genericUrl, content);
httpRequest.getHeaders().setContentType("*/*");
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
public HttpResponse readForHttpResponse(Long spaceId, Long id, Map params) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling read");
}
// verify the required parameter 'id' is set
if (id == null) {
throw new IllegalArgumentException("Missing the required parameter 'id' when calling read");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/subscription/read");
// Copy the params argument if present, to allow passing in immutable maps
Map allParams = params == null ? new HashMap() : new HashMap(params);
// Add the required query param 'spaceId' to the map of query params
allParams.put("spaceId", spaceId);
// Add the required query param 'id' to the map of query params
allParams.put("id", id);
for (Map.Entry entryMap: allParams.entrySet()) {
String key = entryMap.getKey();
Object value = entryMap.getValue();
if (key != null && value != null) {
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = null;
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.GET, genericUrl, content);
httpRequest.getHeaders().setContentType("*/*");
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
/**
* Search
* Searches for the entities as specified by the given query.
* 200 - This status code indicates that a client request was successfully received, understood, and accepted.
*
442 - This status code indicates that the server cannot or will not process the request due to something that is perceived to be a client error.
*
542 - This status code indicates that the server encountered an unexpected condition that prevented it from fulfilling the client request.
* @param spaceId
* @param query The query restricts the subscriptions which are returned by the search.
* @return List<Subscription>
* @throws IOException if an error occurs while attempting to invoke the API
* For more information visit this link.
* @see Search Documentation
**/
public List search(Long spaceId, EntityQuery query) throws IOException {
HttpResponse response = searchForHttpResponse(spaceId, query);
String returnType = "List<Subscription>";
if(returnType.equals("String")){
return (List) (Object) response.parseAsString();
}
TypeReference typeRef = new TypeReference>() {};
if (isNoBodyResponse(response)) {
throw new WalleeSdkException(ErrorCode.ENTITY_NOT_FOUND, "Entity was not found for: " + typeRef.getType().getTypeName());
}
return (List)apiClient.getObjectMapper().readValue(response.getContent(), typeRef);
}
/**
* Search
* Searches for the entities as specified by the given query.
* 200 - This status code indicates that a client request was successfully received, understood, and accepted.
*
442 - This status code indicates that the server cannot or will not process the request due to something that is perceived to be a client error.
*
542 - This status code indicates that the server encountered an unexpected condition that prevented it from fulfilling the client request.
* @param spaceId
* @param query The query restricts the subscriptions which are returned by the search.
* @param params Map of query params. A collection will be interpreted as passing in multiple instances of the same query param.
* @return List<Subscription>
* @throws IOException if an error occurs while attempting to invoke the API
* For more information visit this link.
* @see Search Documentation
**/
public List search(Long spaceId, EntityQuery query, Map params) throws IOException {
HttpResponse response = searchForHttpResponse(spaceId, query, params);
String returnType = "List<Subscription>";
if(returnType.equals("String")){
return (List) (Object) response.parseAsString();
}
TypeReference typeRef = new TypeReference>() {};
if (isNoBodyResponse(response)) {
throw new WalleeSdkException(ErrorCode.ENTITY_NOT_FOUND, "Entity was not found for: " + typeRef.getType().getTypeName());
}
return (List)apiClient.getObjectMapper().readValue(response.getContent(), typeRef);
}
public HttpResponse searchForHttpResponse(Long spaceId, EntityQuery query) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling search");
}
// verify the required parameter 'query' is set
if (query == null) {
throw new IllegalArgumentException("Missing the required parameter 'query' when calling search");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/subscription/search");
if (spaceId != null) {
String key = "spaceId";
Object value = spaceId;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = apiClient.new JacksonJsonHttpContent(query);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
public HttpResponse searchForHttpResponse(Long spaceId, java.io.InputStream query, String mediaType) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling search");
}
// verify the required parameter 'query' is set
if (query == null) {
throw new IllegalArgumentException("Missing the required parameter 'query' when calling search");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/subscription/search");
if (spaceId != null) {
String key = "spaceId";
Object value = spaceId;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = query == null ?
apiClient.new JacksonJsonHttpContent(null) :
new InputStreamContent(mediaType == null ? Json.MEDIA_TYPE : mediaType, query);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
public HttpResponse searchForHttpResponse(Long spaceId, EntityQuery query, Map params) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling search");
}
// verify the required parameter 'query' is set
if (query == null) {
throw new IllegalArgumentException("Missing the required parameter 'query' when calling search");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/subscription/search");
// Copy the params argument if present, to allow passing in immutable maps
Map allParams = params == null ? new HashMap() : new HashMap(params);
// Add the required query param 'spaceId' to the map of query params
allParams.put("spaceId", spaceId);
for (Map.Entry entryMap: allParams.entrySet()) {
String key = entryMap.getKey();
Object value = entryMap.getValue();
if (key != null && value != null) {
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = apiClient.new JacksonJsonHttpContent(query);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
/**
* Search Subscription Invoices
* This operation allows to search for subscription invoices.
* 200 - This status code indicates that a client request was successfully received, understood, and accepted.
*
442 - This status code indicates that the server cannot or will not process the request due to something that is perceived to be a client error.
*
542 - This status code indicates that the server encountered an unexpected condition that prevented it from fulfilling the client request.
* @param spaceId
* @param subscriptionId The id of the subscription for which the invoices should be searched for.
* @param query The query restricts the invoices which are returned by the search.
* @return List<TransactionInvoice>
* @throws IOException if an error occurs while attempting to invoke the API
* For more information visit this link.
* @see Search Subscription Invoices Documentation
**/
public List searchSubscriptionInvoices(Long spaceId, Long subscriptionId, EntityQuery query) throws IOException {
HttpResponse response = searchSubscriptionInvoicesForHttpResponse(spaceId, subscriptionId, query);
String returnType = "List<TransactionInvoice>";
if(returnType.equals("String")){
return (List) (Object) response.parseAsString();
}
TypeReference typeRef = new TypeReference>() {};
if (isNoBodyResponse(response)) {
throw new WalleeSdkException(ErrorCode.ENTITY_NOT_FOUND, "Entity was not found for: " + typeRef.getType().getTypeName());
}
return (List)apiClient.getObjectMapper().readValue(response.getContent(), typeRef);
}
/**
* Search Subscription Invoices
* This operation allows to search for subscription invoices.
* 200 - This status code indicates that a client request was successfully received, understood, and accepted.
*
442 - This status code indicates that the server cannot or will not process the request due to something that is perceived to be a client error.
*
542 - This status code indicates that the server encountered an unexpected condition that prevented it from fulfilling the client request.
* @param spaceId
* @param subscriptionId The id of the subscription for which the invoices should be searched for.
* @param query The query restricts the invoices which are returned by the search.
* @param params Map of query params. A collection will be interpreted as passing in multiple instances of the same query param.
* @return List<TransactionInvoice>
* @throws IOException if an error occurs while attempting to invoke the API
* For more information visit this link.
* @see Search Subscription Invoices Documentation
**/
public List searchSubscriptionInvoices(Long spaceId, Long subscriptionId, EntityQuery query, Map params) throws IOException {
HttpResponse response = searchSubscriptionInvoicesForHttpResponse(spaceId, subscriptionId, query, params);
String returnType = "List<TransactionInvoice>";
if(returnType.equals("String")){
return (List) (Object) response.parseAsString();
}
TypeReference typeRef = new TypeReference>() {};
if (isNoBodyResponse(response)) {
throw new WalleeSdkException(ErrorCode.ENTITY_NOT_FOUND, "Entity was not found for: " + typeRef.getType().getTypeName());
}
return (List)apiClient.getObjectMapper().readValue(response.getContent(), typeRef);
}
public HttpResponse searchSubscriptionInvoicesForHttpResponse(Long spaceId, Long subscriptionId, EntityQuery query) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling searchSubscriptionInvoices");
}
// verify the required parameter 'subscriptionId' is set
if (subscriptionId == null) {
throw new IllegalArgumentException("Missing the required parameter 'subscriptionId' when calling searchSubscriptionInvoices");
}
// verify the required parameter 'query' is set
if (query == null) {
throw new IllegalArgumentException("Missing the required parameter 'query' when calling searchSubscriptionInvoices");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/subscription/searchSubscriptionInvoices");
if (spaceId != null) {
String key = "spaceId";
Object value = spaceId;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
if (subscriptionId != null) {
String key = "subscriptionId";
Object value = subscriptionId;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = apiClient.new JacksonJsonHttpContent(query);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
public HttpResponse searchSubscriptionInvoicesForHttpResponse(Long spaceId, Long subscriptionId, java.io.InputStream query, String mediaType) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling searchSubscriptionInvoices");
}
// verify the required parameter 'subscriptionId' is set
if (subscriptionId == null) {
throw new IllegalArgumentException("Missing the required parameter 'subscriptionId' when calling searchSubscriptionInvoices");
}
// verify the required parameter 'query' is set
if (query == null) {
throw new IllegalArgumentException("Missing the required parameter 'query' when calling searchSubscriptionInvoices");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/subscription/searchSubscriptionInvoices");
if (spaceId != null) {
String key = "spaceId";
Object value = spaceId;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
if (subscriptionId != null) {
String key = "subscriptionId";
Object value = subscriptionId;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = query == null ?
apiClient.new JacksonJsonHttpContent(null) :
new InputStreamContent(mediaType == null ? Json.MEDIA_TYPE : mediaType, query);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
public HttpResponse searchSubscriptionInvoicesForHttpResponse(Long spaceId, Long subscriptionId, EntityQuery query, Map params) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling searchSubscriptionInvoices");
}
// verify the required parameter 'subscriptionId' is set
if (subscriptionId == null) {
throw new IllegalArgumentException("Missing the required parameter 'subscriptionId' when calling searchSubscriptionInvoices");
}
// verify the required parameter 'query' is set
if (query == null) {
throw new IllegalArgumentException("Missing the required parameter 'query' when calling searchSubscriptionInvoices");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/subscription/searchSubscriptionInvoices");
// Copy the params argument if present, to allow passing in immutable maps
Map allParams = params == null ? new HashMap() : new HashMap(params);
// Add the required query param 'spaceId' to the map of query params
allParams.put("spaceId", spaceId);
// Add the required query param 'subscriptionId' to the map of query params
allParams.put("subscriptionId", subscriptionId);
for (Map.Entry entryMap: allParams.entrySet()) {
String key = entryMap.getKey();
Object value = entryMap.getValue();
if (key != null && value != null) {
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = apiClient.new JacksonJsonHttpContent(query);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
/**
* terminate
* This operation allows to terminate a subscription.
* 442 - This status code indicates that the server cannot or will not process the request due to something that is perceived to be a client error.
*
542 - This status code indicates that the server encountered an unexpected condition that prevented it from fulfilling the client request.
* @param spaceId
* @param subscriptionId The subscription id identifies the subscription which should be terminated.
* @param respectTerminationPeriod The respect termination period controls whether the termination period configured on the product version should be respected or if the operation should take effect immediately.
* @throws IOException if an error occurs while attempting to invoke the API
* For more information visit this link.
* @see terminate Documentation
**/
public void terminate(Long spaceId, Long subscriptionId, Boolean respectTerminationPeriod) throws IOException {
terminateForHttpResponse(spaceId, subscriptionId, respectTerminationPeriod);
}
/**
* terminate
* This operation allows to terminate a subscription.
*
442 - This status code indicates that the server cannot or will not process the request due to something that is perceived to be a client error.
*
542 - This status code indicates that the server encountered an unexpected condition that prevented it from fulfilling the client request.
* @param spaceId
* @param subscriptionId The subscription id identifies the subscription which should be terminated.
* @param respectTerminationPeriod The respect termination period controls whether the termination period configured on the product version should be respected or if the operation should take effect immediately.
* @param params Map of query params. A collection will be interpreted as passing in multiple instances of the same query param.
* @throws IOException if an error occurs while attempting to invoke the API
* For more information visit this link.
* @see terminate Documentation
**/
public void terminate(Long spaceId, Long subscriptionId, Boolean respectTerminationPeriod, Map params) throws IOException {
terminateForHttpResponse(spaceId, subscriptionId, respectTerminationPeriod, params);
}
public HttpResponse terminateForHttpResponse(Long spaceId, Long subscriptionId, Boolean respectTerminationPeriod) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling terminate");
}
// verify the required parameter 'subscriptionId' is set
if (subscriptionId == null) {
throw new IllegalArgumentException("Missing the required parameter 'subscriptionId' when calling terminate");
}
// verify the required parameter 'respectTerminationPeriod' is set
if (respectTerminationPeriod == null) {
throw new IllegalArgumentException("Missing the required parameter 'respectTerminationPeriod' when calling terminate");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/subscription/terminate");
if (spaceId != null) {
String key = "spaceId";
Object value = spaceId;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
if (subscriptionId != null) {
String key = "subscriptionId";
Object value = subscriptionId;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
if (respectTerminationPeriod != null) {
String key = "respectTerminationPeriod";
Object value = respectTerminationPeriod;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = apiClient.new JacksonJsonHttpContent(null);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
public HttpResponse terminateForHttpResponse(Long spaceId, Long subscriptionId, Boolean respectTerminationPeriod, Map params) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling terminate");
}
// verify the required parameter 'subscriptionId' is set
if (subscriptionId == null) {
throw new IllegalArgumentException("Missing the required parameter 'subscriptionId' when calling terminate");
}
// verify the required parameter 'respectTerminationPeriod' is set
if (respectTerminationPeriod == null) {
throw new IllegalArgumentException("Missing the required parameter 'respectTerminationPeriod' when calling terminate");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/subscription/terminate");
// Copy the params argument if present, to allow passing in immutable maps
Map allParams = params == null ? new HashMap() : new HashMap(params);
// Add the required query param 'spaceId' to the map of query params
allParams.put("spaceId", spaceId);
// Add the required query param 'subscriptionId' to the map of query params
allParams.put("subscriptionId", subscriptionId);
// Add the required query param 'respectTerminationPeriod' to the map of query params
allParams.put("respectTerminationPeriod", respectTerminationPeriod);
for (Map.Entry entryMap: allParams.entrySet()) {
String key = entryMap.getKey();
Object value = entryMap.getValue();
if (key != null && value != null) {
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = apiClient.new JacksonJsonHttpContent(null);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
/**
* update
* This operation allows to update the subscription.
* 200 - This status code indicates that a client request was successfully received, understood, and accepted.
*
409 - This status code indicates that there was a conflict with the current version of the data in the database and the provided data in the request.
*
442 - This status code indicates that the server cannot or will not process the request due to something that is perceived to be a client error.
*
542 - This status code indicates that the server encountered an unexpected condition that prevented it from fulfilling the client request.
* @param spaceId
* @param subscriptionId
* @param request
* @return Subscription
* @throws IOException if an error occurs while attempting to invoke the API
* For more information visit this link.
* @see update Documentation
**/
public Subscription update(Long spaceId, Long subscriptionId, SubscriptionUpdateRequest request) throws IOException {
HttpResponse response = updateForHttpResponse(spaceId, subscriptionId, request);
String returnType = "Subscription";
if(returnType.equals("String")){
return (Subscription) (Object) response.parseAsString();
}
TypeReference typeRef = new TypeReference() {};
if (isNoBodyResponse(response)) {
throw new WalleeSdkException(ErrorCode.ENTITY_NOT_FOUND, "Entity was not found for: " + typeRef.getType().getTypeName());
}
return (Subscription)apiClient.getObjectMapper().readValue(response.getContent(), typeRef);
}
/**
* update
* This operation allows to update the subscription.
* 200 - This status code indicates that a client request was successfully received, understood, and accepted.
*
409 - This status code indicates that there was a conflict with the current version of the data in the database and the provided data in the request.
*
442 - This status code indicates that the server cannot or will not process the request due to something that is perceived to be a client error.
*
542 - This status code indicates that the server encountered an unexpected condition that prevented it from fulfilling the client request.
* @param spaceId
* @param subscriptionId
* @param request
* @param params Map of query params. A collection will be interpreted as passing in multiple instances of the same query param.
* @return Subscription
* @throws IOException if an error occurs while attempting to invoke the API
* For more information visit this link.
* @see update Documentation
**/
public Subscription update(Long spaceId, Long subscriptionId, SubscriptionUpdateRequest request, Map params) throws IOException {
HttpResponse response = updateForHttpResponse(spaceId, subscriptionId, request, params);
String returnType = "Subscription";
if(returnType.equals("String")){
return (Subscription) (Object) response.parseAsString();
}
TypeReference typeRef = new TypeReference() {};
if (isNoBodyResponse(response)) {
throw new WalleeSdkException(ErrorCode.ENTITY_NOT_FOUND, "Entity was not found for: " + typeRef.getType().getTypeName());
}
return (Subscription)apiClient.getObjectMapper().readValue(response.getContent(), typeRef);
}
public HttpResponse updateForHttpResponse(Long spaceId, Long subscriptionId, SubscriptionUpdateRequest request) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling update");
}
// verify the required parameter 'subscriptionId' is set
if (subscriptionId == null) {
throw new IllegalArgumentException("Missing the required parameter 'subscriptionId' when calling update");
}
// verify the required parameter 'request' is set
if (request == null) {
throw new IllegalArgumentException("Missing the required parameter 'request' when calling update");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/subscription/update");
if (spaceId != null) {
String key = "spaceId";
Object value = spaceId;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
if (subscriptionId != null) {
String key = "subscriptionId";
Object value = subscriptionId;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = apiClient.new JacksonJsonHttpContent(request);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
public HttpResponse updateForHttpResponse(Long spaceId, Long subscriptionId, java.io.InputStream request, String mediaType) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling update");
}
// verify the required parameter 'subscriptionId' is set
if (subscriptionId == null) {
throw new IllegalArgumentException("Missing the required parameter 'subscriptionId' when calling update");
}
// verify the required parameter 'request' is set
if (request == null) {
throw new IllegalArgumentException("Missing the required parameter 'request' when calling update");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/subscription/update");
if (spaceId != null) {
String key = "spaceId";
Object value = spaceId;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
if (subscriptionId != null) {
String key = "subscriptionId";
Object value = subscriptionId;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = request == null ?
apiClient.new JacksonJsonHttpContent(null) :
new InputStreamContent(mediaType == null ? Json.MEDIA_TYPE : mediaType, request);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
public HttpResponse updateForHttpResponse(Long spaceId, Long subscriptionId, SubscriptionUpdateRequest request, Map params) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling update");
}
// verify the required parameter 'subscriptionId' is set
if (subscriptionId == null) {
throw new IllegalArgumentException("Missing the required parameter 'subscriptionId' when calling update");
}
// verify the required parameter 'request' is set
if (request == null) {
throw new IllegalArgumentException("Missing the required parameter 'request' when calling update");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/subscription/update");
// Copy the params argument if present, to allow passing in immutable maps
Map allParams = params == null ? new HashMap() : new HashMap(params);
// Add the required query param 'spaceId' to the map of query params
allParams.put("spaceId", spaceId);
// Add the required query param 'subscriptionId' to the map of query params
allParams.put("subscriptionId", subscriptionId);
for (Map.Entry entryMap: allParams.entrySet()) {
String key = entryMap.getKey();
Object value = entryMap.getValue();
if (key != null && value != null) {
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = apiClient.new JacksonJsonHttpContent(request);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
/**
* update product version
* The update product version operation updates the product version of the subscription to the latest active product version.
* 200 - This status code indicates that a client request was successfully received, understood, and accepted.
*
409 - This status code indicates that there was a conflict with the current version of the data in the database and the provided data in the request.
*
442 - This status code indicates that the server cannot or will not process the request due to something that is perceived to be a client error.
*
542 - This status code indicates that the server encountered an unexpected condition that prevented it from fulfilling the client request.
* @param spaceId
* @param subscriptionId The subscription id identifies the subscription which should be updated to the latest version.
* @param respectTerminationPeriod The subscription version may be retired. The respect termination period controls whether the termination period configured on the product version should be respected or if the operation should take effect immediately.
* @return SubscriptionVersion
* @throws IOException if an error occurs while attempting to invoke the API
* For more information visit this link.
* @see update product version Documentation
**/
public SubscriptionVersion updateProductVersion(Long spaceId, Long subscriptionId, Boolean respectTerminationPeriod) throws IOException {
HttpResponse response = updateProductVersionForHttpResponse(spaceId, subscriptionId, respectTerminationPeriod);
String returnType = "SubscriptionVersion";
if(returnType.equals("String")){
return (SubscriptionVersion) (Object) response.parseAsString();
}
TypeReference typeRef = new TypeReference() {};
if (isNoBodyResponse(response)) {
throw new WalleeSdkException(ErrorCode.ENTITY_NOT_FOUND, "Entity was not found for: " + typeRef.getType().getTypeName());
}
return (SubscriptionVersion)apiClient.getObjectMapper().readValue(response.getContent(), typeRef);
}
/**
* update product version
* The update product version operation updates the product version of the subscription to the latest active product version.
* 200 - This status code indicates that a client request was successfully received, understood, and accepted.
*
409 - This status code indicates that there was a conflict with the current version of the data in the database and the provided data in the request.
*
442 - This status code indicates that the server cannot or will not process the request due to something that is perceived to be a client error.
*
542 - This status code indicates that the server encountered an unexpected condition that prevented it from fulfilling the client request.
* @param spaceId
* @param subscriptionId The subscription id identifies the subscription which should be updated to the latest version.
* @param respectTerminationPeriod The subscription version may be retired. The respect termination period controls whether the termination period configured on the product version should be respected or if the operation should take effect immediately.
* @param params Map of query params. A collection will be interpreted as passing in multiple instances of the same query param.
* @return SubscriptionVersion
* @throws IOException if an error occurs while attempting to invoke the API
* For more information visit this link.
* @see update product version Documentation
**/
public SubscriptionVersion updateProductVersion(Long spaceId, Long subscriptionId, Boolean respectTerminationPeriod, Map params) throws IOException {
HttpResponse response = updateProductVersionForHttpResponse(spaceId, subscriptionId, respectTerminationPeriod, params);
String returnType = "SubscriptionVersion";
if(returnType.equals("String")){
return (SubscriptionVersion) (Object) response.parseAsString();
}
TypeReference typeRef = new TypeReference() {};
if (isNoBodyResponse(response)) {
throw new WalleeSdkException(ErrorCode.ENTITY_NOT_FOUND, "Entity was not found for: " + typeRef.getType().getTypeName());
}
return (SubscriptionVersion)apiClient.getObjectMapper().readValue(response.getContent(), typeRef);
}
public HttpResponse updateProductVersionForHttpResponse(Long spaceId, Long subscriptionId, Boolean respectTerminationPeriod) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling updateProductVersion");
}
// verify the required parameter 'subscriptionId' is set
if (subscriptionId == null) {
throw new IllegalArgumentException("Missing the required parameter 'subscriptionId' when calling updateProductVersion");
}
// verify the required parameter 'respectTerminationPeriod' is set
if (respectTerminationPeriod == null) {
throw new IllegalArgumentException("Missing the required parameter 'respectTerminationPeriod' when calling updateProductVersion");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/subscription/updateProductVersion");
if (spaceId != null) {
String key = "spaceId";
Object value = spaceId;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
if (subscriptionId != null) {
String key = "subscriptionId";
Object value = subscriptionId;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
if (respectTerminationPeriod != null) {
String key = "respectTerminationPeriod";
Object value = respectTerminationPeriod;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = apiClient.new JacksonJsonHttpContent(null);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
public HttpResponse updateProductVersionForHttpResponse(Long spaceId, Long subscriptionId, Boolean respectTerminationPeriod, Map params) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling updateProductVersion");
}
// verify the required parameter 'subscriptionId' is set
if (subscriptionId == null) {
throw new IllegalArgumentException("Missing the required parameter 'subscriptionId' when calling updateProductVersion");
}
// verify the required parameter 'respectTerminationPeriod' is set
if (respectTerminationPeriod == null) {
throw new IllegalArgumentException("Missing the required parameter 'respectTerminationPeriod' when calling updateProductVersion");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/subscription/updateProductVersion");
// Copy the params argument if present, to allow passing in immutable maps
Map allParams = params == null ? new HashMap() : new HashMap(params);
// Add the required query param 'spaceId' to the map of query params
allParams.put("spaceId", spaceId);
// Add the required query param 'subscriptionId' to the map of query params
allParams.put("subscriptionId", subscriptionId);
// Add the required query param 'respectTerminationPeriod' to the map of query params
allParams.put("respectTerminationPeriod", respectTerminationPeriod);
for (Map.Entry entryMap: allParams.entrySet()) {
String key = entryMap.getKey();
Object value = entryMap.getValue();
if (key != null && value != null) {
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = apiClient.new JacksonJsonHttpContent(null);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
private boolean isNoBodyResponse(HttpResponse response) throws IOException {
java.io.InputStream content = response.getContent();
return content.available() == 0;
}
}