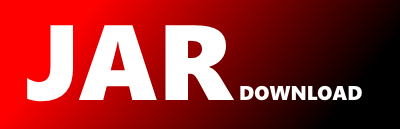
com.wallee.sdk.service.TokenService Maven / Gradle / Ivy
package com.wallee.sdk.service;
import static com.wallee.sdk.ErrorCode.*;
import com.wallee.sdk.ApiClient;
import com.wallee.sdk.ErrorCode;
import com.wallee.sdk.exception.WalleeSdkException;
import com.wallee.sdk.util.URIBuilderUtil;
import com.wallee.sdk.StringUtil;
import com.wallee.sdk.model.Charge;
import com.wallee.sdk.model.ClientError;
import com.wallee.sdk.model.EntityQuery;
import com.wallee.sdk.model.EntityQueryFilter;
import com.wallee.sdk.model.ServerError;
import com.wallee.sdk.model.Token;
import com.wallee.sdk.model.TokenCreate;
import com.wallee.sdk.model.TokenUpdate;
import com.wallee.sdk.model.TokenVersion;
import com.wallee.sdk.model.Transaction;
import com.fasterxml.jackson.core.type.TypeReference;
import com.google.api.client.http.*;
import com.google.api.client.json.Json;
import org.apache.http.client.utils.URIBuilder;
import java.io.IOException;
import java.util.Collection;
import java.util.HashMap;
import java.util.Map;
import java.util.List;
import java.util.ArrayList;
import java.util.Objects;
public class TokenService {
private ApiClient apiClient;
public TokenService(ApiClient apiClient) {
this.apiClient = Objects.requireNonNull(apiClient, "ApiClient must be non null");
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = Objects.requireNonNull(apiClient, "ApiClient must be non null");
}
/**
* Check If Token Creation Is Possible
* This operation checks if the given transaction can be used to create a token out of it.
* 200 - This status code indicates that a client request was successfully received, understood, and accepted.
*
442 - This status code indicates that the server cannot or will not process the request due to something that is perceived to be a client error.
*
542 - This status code indicates that the server encountered an unexpected condition that prevented it from fulfilling the client request.
* @param spaceId
* @param transactionId The id of the transaction for which we want to check if the token can be created or not.
* @return Boolean
* @throws IOException if an error occurs while attempting to invoke the API
* For more information visit this link.
* @see Check If Token Creation Is Possible Documentation
**/
public Boolean checkTokenCreationPossible(Long spaceId, Long transactionId) throws IOException {
HttpResponse response = checkTokenCreationPossibleForHttpResponse(spaceId, transactionId);
String returnType = "Boolean";
if(returnType.equals("String")){
return (Boolean) (Object) response.parseAsString();
}
TypeReference typeRef = new TypeReference() {};
if (isNoBodyResponse(response)) {
throw new WalleeSdkException(ErrorCode.ENTITY_NOT_FOUND, "Entity was not found for: " + typeRef.getType().getTypeName());
}
return (Boolean)apiClient.getObjectMapper().readValue(response.getContent(), typeRef);
}
/**
* Check If Token Creation Is Possible
* This operation checks if the given transaction can be used to create a token out of it.
* 200 - This status code indicates that a client request was successfully received, understood, and accepted.
*
442 - This status code indicates that the server cannot or will not process the request due to something that is perceived to be a client error.
*
542 - This status code indicates that the server encountered an unexpected condition that prevented it from fulfilling the client request.
* @param spaceId
* @param transactionId The id of the transaction for which we want to check if the token can be created or not.
* @param params Map of query params. A collection will be interpreted as passing in multiple instances of the same query param.
* @return Boolean
* @throws IOException if an error occurs while attempting to invoke the API
* For more information visit this link.
* @see Check If Token Creation Is Possible Documentation
**/
public Boolean checkTokenCreationPossible(Long spaceId, Long transactionId, Map params) throws IOException {
HttpResponse response = checkTokenCreationPossibleForHttpResponse(spaceId, transactionId, params);
String returnType = "Boolean";
if(returnType.equals("String")){
return (Boolean) (Object) response.parseAsString();
}
TypeReference typeRef = new TypeReference() {};
if (isNoBodyResponse(response)) {
throw new WalleeSdkException(ErrorCode.ENTITY_NOT_FOUND, "Entity was not found for: " + typeRef.getType().getTypeName());
}
return (Boolean)apiClient.getObjectMapper().readValue(response.getContent(), typeRef);
}
public HttpResponse checkTokenCreationPossibleForHttpResponse(Long spaceId, Long transactionId) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling checkTokenCreationPossible");
}
// verify the required parameter 'transactionId' is set
if (transactionId == null) {
throw new IllegalArgumentException("Missing the required parameter 'transactionId' when calling checkTokenCreationPossible");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/token/check-token-creation-possible");
if (spaceId != null) {
String key = "spaceId";
Object value = spaceId;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
if (transactionId != null) {
String key = "transactionId";
Object value = transactionId;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = apiClient.new JacksonJsonHttpContent(null);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
public HttpResponse checkTokenCreationPossibleForHttpResponse(Long spaceId, Long transactionId, Map params) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling checkTokenCreationPossible");
}
// verify the required parameter 'transactionId' is set
if (transactionId == null) {
throw new IllegalArgumentException("Missing the required parameter 'transactionId' when calling checkTokenCreationPossible");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/token/check-token-creation-possible");
// Copy the params argument if present, to allow passing in immutable maps
Map allParams = params == null ? new HashMap() : new HashMap(params);
// Add the required query param 'spaceId' to the map of query params
allParams.put("spaceId", spaceId);
// Add the required query param 'transactionId' to the map of query params
allParams.put("transactionId", transactionId);
for (Map.Entry entryMap: allParams.entrySet()) {
String key = entryMap.getKey();
Object value = entryMap.getValue();
if (key != null && value != null) {
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = apiClient.new JacksonJsonHttpContent(null);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
/**
* Count
* Counts the number of items in the database as restricted by the given filter.
* 200 - This status code indicates that a client request was successfully received, understood, and accepted.
*
442 - This status code indicates that the server cannot or will not process the request due to something that is perceived to be a client error.
*
542 - This status code indicates that the server encountered an unexpected condition that prevented it from fulfilling the client request.
* @param spaceId
* @param filter The filter which restricts the entities which are used to calculate the count.
* @return Long
* @throws IOException if an error occurs while attempting to invoke the API
* For more information visit this link.
* @see Count Documentation
**/
public Long count(Long spaceId, EntityQueryFilter filter) throws IOException {
HttpResponse response = countForHttpResponse(spaceId, filter);
String returnType = "Long";
if(returnType.equals("String")){
return (Long) (Object) response.parseAsString();
}
TypeReference typeRef = new TypeReference() {};
if (isNoBodyResponse(response)) {
throw new WalleeSdkException(ErrorCode.ENTITY_NOT_FOUND, "Entity was not found for: " + typeRef.getType().getTypeName());
}
return (Long)apiClient.getObjectMapper().readValue(response.getContent(), typeRef);
}
/**
* Count
* Counts the number of items in the database as restricted by the given filter.
* 200 - This status code indicates that a client request was successfully received, understood, and accepted.
*
442 - This status code indicates that the server cannot or will not process the request due to something that is perceived to be a client error.
*
542 - This status code indicates that the server encountered an unexpected condition that prevented it from fulfilling the client request.
* @param spaceId
* @param params Map of query params. A collection will be interpreted as passing in multiple instances of the same query param.
* @return Long
* @throws IOException if an error occurs while attempting to invoke the API
* For more information visit this link.
* @see Count Documentation
**/
public Long count(EntityQueryFilter filter, Long spaceId, Map params) throws IOException {
HttpResponse response = countForHttpResponse(filter, spaceId, params);
String returnType = "Long";
if(returnType.equals("String")){
return (Long) (Object) response.parseAsString();
}
TypeReference typeRef = new TypeReference() {};
if (isNoBodyResponse(response)) {
throw new WalleeSdkException(ErrorCode.ENTITY_NOT_FOUND, "Entity was not found for: " + typeRef.getType().getTypeName());
}
return (Long)apiClient.getObjectMapper().readValue(response.getContent(), typeRef);
}
public HttpResponse countForHttpResponse(Long spaceId, EntityQueryFilter filter) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling count");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/token/count");
if (spaceId != null) {
String key = "spaceId";
Object value = spaceId;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = apiClient.new JacksonJsonHttpContent(filter);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
public HttpResponse countForHttpResponse(Long spaceId, java.io.InputStream filter, String mediaType) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling count");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/token/count");
if (spaceId != null) {
String key = "spaceId";
Object value = spaceId;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = filter == null ?
apiClient.new JacksonJsonHttpContent(null) :
new InputStreamContent(mediaType == null ? Json.MEDIA_TYPE : mediaType, filter);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
public HttpResponse countForHttpResponse(EntityQueryFilter filter, Long spaceId, Map params) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling count");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/token/count");
// Copy the params argument if present, to allow passing in immutable maps
Map allParams = params == null ? new HashMap() : new HashMap(params);
// Add the required query param 'spaceId' to the map of query params
allParams.put("spaceId", spaceId);
for (Map.Entry entryMap: allParams.entrySet()) {
String key = entryMap.getKey();
Object value = entryMap.getValue();
if (key != null && value != null) {
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = apiClient.new JacksonJsonHttpContent(filter);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
/**
* Create
* Creates the entity with the given properties.
* 200 - This status code indicates that a client request was successfully received, understood, and accepted.
*
442 - This status code indicates that the server cannot or will not process the request due to something that is perceived to be a client error.
*
542 - This status code indicates that the server encountered an unexpected condition that prevented it from fulfilling the client request.
* @param spaceId
* @param entity The token object with the properties which should be created.
* @return Token
* @throws IOException if an error occurs while attempting to invoke the API
* For more information visit this link.
* @see Create Documentation
**/
public Token create(Long spaceId, TokenCreate entity) throws IOException {
HttpResponse response = createForHttpResponse(spaceId, entity);
String returnType = "Token";
if(returnType.equals("String")){
return (Token) (Object) response.parseAsString();
}
TypeReference typeRef = new TypeReference() {};
if (isNoBodyResponse(response)) {
throw new WalleeSdkException(ErrorCode.ENTITY_NOT_FOUND, "Entity was not found for: " + typeRef.getType().getTypeName());
}
return (Token)apiClient.getObjectMapper().readValue(response.getContent(), typeRef);
}
/**
* Create
* Creates the entity with the given properties.
* 200 - This status code indicates that a client request was successfully received, understood, and accepted.
*
442 - This status code indicates that the server cannot or will not process the request due to something that is perceived to be a client error.
*
542 - This status code indicates that the server encountered an unexpected condition that prevented it from fulfilling the client request.
* @param spaceId
* @param entity The token object with the properties which should be created.
* @param params Map of query params. A collection will be interpreted as passing in multiple instances of the same query param.
* @return Token
* @throws IOException if an error occurs while attempting to invoke the API
* For more information visit this link.
* @see Create Documentation
**/
public Token create(Long spaceId, TokenCreate entity, Map params) throws IOException {
HttpResponse response = createForHttpResponse(spaceId, entity, params);
String returnType = "Token";
if(returnType.equals("String")){
return (Token) (Object) response.parseAsString();
}
TypeReference typeRef = new TypeReference() {};
if (isNoBodyResponse(response)) {
throw new WalleeSdkException(ErrorCode.ENTITY_NOT_FOUND, "Entity was not found for: " + typeRef.getType().getTypeName());
}
return (Token)apiClient.getObjectMapper().readValue(response.getContent(), typeRef);
}
public HttpResponse createForHttpResponse(Long spaceId, TokenCreate entity) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling create");
}
// verify the required parameter 'entity' is set
if (entity == null) {
throw new IllegalArgumentException("Missing the required parameter 'entity' when calling create");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/token/create");
if (spaceId != null) {
String key = "spaceId";
Object value = spaceId;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = apiClient.new JacksonJsonHttpContent(entity);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
public HttpResponse createForHttpResponse(Long spaceId, java.io.InputStream entity, String mediaType) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling create");
}
// verify the required parameter 'entity' is set
if (entity == null) {
throw new IllegalArgumentException("Missing the required parameter 'entity' when calling create");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/token/create");
if (spaceId != null) {
String key = "spaceId";
Object value = spaceId;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = entity == null ?
apiClient.new JacksonJsonHttpContent(null) :
new InputStreamContent(mediaType == null ? Json.MEDIA_TYPE : mediaType, entity);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
public HttpResponse createForHttpResponse(Long spaceId, TokenCreate entity, Map params) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling create");
}
// verify the required parameter 'entity' is set
if (entity == null) {
throw new IllegalArgumentException("Missing the required parameter 'entity' when calling create");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/token/create");
// Copy the params argument if present, to allow passing in immutable maps
Map allParams = params == null ? new HashMap() : new HashMap(params);
// Add the required query param 'spaceId' to the map of query params
allParams.put("spaceId", spaceId);
for (Map.Entry entryMap: allParams.entrySet()) {
String key = entryMap.getKey();
Object value = entryMap.getValue();
if (key != null && value != null) {
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = apiClient.new JacksonJsonHttpContent(entity);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
/**
* Create Token
* This operation creates a token for the given transaction. The transaction payment information will be populated asynchronously as soon as all data becomes available.
* 200 - This status code indicates that a client request was successfully received, understood, and accepted.
*
442 - This status code indicates that the server cannot or will not process the request due to something that is perceived to be a client error.
*
542 - This status code indicates that the server encountered an unexpected condition that prevented it from fulfilling the client request.
* @param spaceId
* @param transactionId The id of the transaction for which we want to create the token.
* @return Token
* @throws IOException if an error occurs while attempting to invoke the API
* For more information visit this link.
* @see Create Token Documentation
**/
public Token createToken(Long spaceId, Long transactionId) throws IOException {
HttpResponse response = createTokenForHttpResponse(spaceId, transactionId);
String returnType = "Token";
if(returnType.equals("String")){
return (Token) (Object) response.parseAsString();
}
TypeReference typeRef = new TypeReference() {};
if (isNoBodyResponse(response)) {
throw new WalleeSdkException(ErrorCode.ENTITY_NOT_FOUND, "Entity was not found for: " + typeRef.getType().getTypeName());
}
return (Token)apiClient.getObjectMapper().readValue(response.getContent(), typeRef);
}
/**
* Create Token
* This operation creates a token for the given transaction. The transaction payment information will be populated asynchronously as soon as all data becomes available.
* 200 - This status code indicates that a client request was successfully received, understood, and accepted.
*
442 - This status code indicates that the server cannot or will not process the request due to something that is perceived to be a client error.
*
542 - This status code indicates that the server encountered an unexpected condition that prevented it from fulfilling the client request.
* @param spaceId
* @param transactionId The id of the transaction for which we want to create the token.
* @param params Map of query params. A collection will be interpreted as passing in multiple instances of the same query param.
* @return Token
* @throws IOException if an error occurs while attempting to invoke the API
* For more information visit this link.
* @see Create Token Documentation
**/
public Token createToken(Long spaceId, Long transactionId, Map params) throws IOException {
HttpResponse response = createTokenForHttpResponse(spaceId, transactionId, params);
String returnType = "Token";
if(returnType.equals("String")){
return (Token) (Object) response.parseAsString();
}
TypeReference typeRef = new TypeReference() {};
if (isNoBodyResponse(response)) {
throw new WalleeSdkException(ErrorCode.ENTITY_NOT_FOUND, "Entity was not found for: " + typeRef.getType().getTypeName());
}
return (Token)apiClient.getObjectMapper().readValue(response.getContent(), typeRef);
}
public HttpResponse createTokenForHttpResponse(Long spaceId, Long transactionId) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling createToken");
}
// verify the required parameter 'transactionId' is set
if (transactionId == null) {
throw new IllegalArgumentException("Missing the required parameter 'transactionId' when calling createToken");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/token/create-token");
if (spaceId != null) {
String key = "spaceId";
Object value = spaceId;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
if (transactionId != null) {
String key = "transactionId";
Object value = transactionId;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = apiClient.new JacksonJsonHttpContent(null);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
public HttpResponse createTokenForHttpResponse(Long spaceId, Long transactionId, Map params) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling createToken");
}
// verify the required parameter 'transactionId' is set
if (transactionId == null) {
throw new IllegalArgumentException("Missing the required parameter 'transactionId' when calling createToken");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/token/create-token");
// Copy the params argument if present, to allow passing in immutable maps
Map allParams = params == null ? new HashMap() : new HashMap(params);
// Add the required query param 'spaceId' to the map of query params
allParams.put("spaceId", spaceId);
// Add the required query param 'transactionId' to the map of query params
allParams.put("transactionId", transactionId);
for (Map.Entry entryMap: allParams.entrySet()) {
String key = entryMap.getKey();
Object value = entryMap.getValue();
if (key != null && value != null) {
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = apiClient.new JacksonJsonHttpContent(null);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
/**
* Create Token Based On Transaction And Fill It With Stored Payment Information
* This operation creates a token for the given transaction and fills it with the stored payment information of the transaction. The payment information for the transaction will be filled in immediately, if payment information is missing, an exception will be thrown.
* 200 - This status code indicates that a client request was successfully received, understood, and accepted.
*
442 - This status code indicates that the server cannot or will not process the request due to something that is perceived to be a client error.
*
542 - This status code indicates that the server encountered an unexpected condition that prevented it from fulfilling the client request.
* @param spaceId
* @param transactionId The id of the transaction for which we want to create the token.
* @return TokenVersion
* @throws IOException if an error occurs while attempting to invoke the API
* For more information visit this link.
* @see Create Token Based On Transaction And Fill It With Stored Payment Information Documentation
**/
public TokenVersion createTokenBasedOnTransaction(Long spaceId, Long transactionId) throws IOException {
HttpResponse response = createTokenBasedOnTransactionForHttpResponse(spaceId, transactionId);
String returnType = "TokenVersion";
if(returnType.equals("String")){
return (TokenVersion) (Object) response.parseAsString();
}
TypeReference typeRef = new TypeReference() {};
if (isNoBodyResponse(response)) {
throw new WalleeSdkException(ErrorCode.ENTITY_NOT_FOUND, "Entity was not found for: " + typeRef.getType().getTypeName());
}
return (TokenVersion)apiClient.getObjectMapper().readValue(response.getContent(), typeRef);
}
/**
* Create Token Based On Transaction And Fill It With Stored Payment Information
* This operation creates a token for the given transaction and fills it with the stored payment information of the transaction. The payment information for the transaction will be filled in immediately, if payment information is missing, an exception will be thrown.
* 200 - This status code indicates that a client request was successfully received, understood, and accepted.
*
442 - This status code indicates that the server cannot or will not process the request due to something that is perceived to be a client error.
*
542 - This status code indicates that the server encountered an unexpected condition that prevented it from fulfilling the client request.
* @param spaceId
* @param transactionId The id of the transaction for which we want to create the token.
* @param params Map of query params. A collection will be interpreted as passing in multiple instances of the same query param.
* @return TokenVersion
* @throws IOException if an error occurs while attempting to invoke the API
* For more information visit this link.
* @see Create Token Based On Transaction And Fill It With Stored Payment Information Documentation
**/
public TokenVersion createTokenBasedOnTransaction(Long spaceId, Long transactionId, Map params) throws IOException {
HttpResponse response = createTokenBasedOnTransactionForHttpResponse(spaceId, transactionId, params);
String returnType = "TokenVersion";
if(returnType.equals("String")){
return (TokenVersion) (Object) response.parseAsString();
}
TypeReference typeRef = new TypeReference() {};
if (isNoBodyResponse(response)) {
throw new WalleeSdkException(ErrorCode.ENTITY_NOT_FOUND, "Entity was not found for: " + typeRef.getType().getTypeName());
}
return (TokenVersion)apiClient.getObjectMapper().readValue(response.getContent(), typeRef);
}
public HttpResponse createTokenBasedOnTransactionForHttpResponse(Long spaceId, Long transactionId) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling createTokenBasedOnTransaction");
}
// verify the required parameter 'transactionId' is set
if (transactionId == null) {
throw new IllegalArgumentException("Missing the required parameter 'transactionId' when calling createTokenBasedOnTransaction");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/token/create-token-based-on-transaction");
if (spaceId != null) {
String key = "spaceId";
Object value = spaceId;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
if (transactionId != null) {
String key = "transactionId";
Object value = transactionId;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = apiClient.new JacksonJsonHttpContent(null);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
public HttpResponse createTokenBasedOnTransactionForHttpResponse(Long spaceId, Long transactionId, Map params) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling createTokenBasedOnTransaction");
}
// verify the required parameter 'transactionId' is set
if (transactionId == null) {
throw new IllegalArgumentException("Missing the required parameter 'transactionId' when calling createTokenBasedOnTransaction");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/token/create-token-based-on-transaction");
// Copy the params argument if present, to allow passing in immutable maps
Map allParams = params == null ? new HashMap() : new HashMap(params);
// Add the required query param 'spaceId' to the map of query params
allParams.put("spaceId", spaceId);
// Add the required query param 'transactionId' to the map of query params
allParams.put("transactionId", transactionId);
for (Map.Entry entryMap: allParams.entrySet()) {
String key = entryMap.getKey();
Object value = entryMap.getValue();
if (key != null && value != null) {
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = apiClient.new JacksonJsonHttpContent(null);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
/**
* Create Transaction for Token Update
* This operation creates a transaction which allows the updating of the provided token.
* 200 - This status code indicates that a client request was successfully received, understood, and accepted.
*
442 - This status code indicates that the server cannot or will not process the request due to something that is perceived to be a client error.
*
542 - This status code indicates that the server encountered an unexpected condition that prevented it from fulfilling the client request.
* @param spaceId
* @param tokenId The id of the token which should be updated.
* @return Transaction
* @throws IOException if an error occurs while attempting to invoke the API
* For more information visit this link.
* @see Create Transaction for Token Update Documentation
**/
public Transaction createTransactionForTokenUpdate(Long spaceId, Long tokenId) throws IOException {
HttpResponse response = createTransactionForTokenUpdateForHttpResponse(spaceId, tokenId);
String returnType = "Transaction";
if(returnType.equals("String")){
return (Transaction) (Object) response.parseAsString();
}
TypeReference typeRef = new TypeReference() {};
if (isNoBodyResponse(response)) {
throw new WalleeSdkException(ErrorCode.ENTITY_NOT_FOUND, "Entity was not found for: " + typeRef.getType().getTypeName());
}
return (Transaction)apiClient.getObjectMapper().readValue(response.getContent(), typeRef);
}
/**
* Create Transaction for Token Update
* This operation creates a transaction which allows the updating of the provided token.
* 200 - This status code indicates that a client request was successfully received, understood, and accepted.
*
442 - This status code indicates that the server cannot or will not process the request due to something that is perceived to be a client error.
*
542 - This status code indicates that the server encountered an unexpected condition that prevented it from fulfilling the client request.
* @param spaceId
* @param tokenId The id of the token which should be updated.
* @param params Map of query params. A collection will be interpreted as passing in multiple instances of the same query param.
* @return Transaction
* @throws IOException if an error occurs while attempting to invoke the API
* For more information visit this link.
* @see Create Transaction for Token Update Documentation
**/
public Transaction createTransactionForTokenUpdate(Long spaceId, Long tokenId, Map params) throws IOException {
HttpResponse response = createTransactionForTokenUpdateForHttpResponse(spaceId, tokenId, params);
String returnType = "Transaction";
if(returnType.equals("String")){
return (Transaction) (Object) response.parseAsString();
}
TypeReference typeRef = new TypeReference() {};
if (isNoBodyResponse(response)) {
throw new WalleeSdkException(ErrorCode.ENTITY_NOT_FOUND, "Entity was not found for: " + typeRef.getType().getTypeName());
}
return (Transaction)apiClient.getObjectMapper().readValue(response.getContent(), typeRef);
}
public HttpResponse createTransactionForTokenUpdateForHttpResponse(Long spaceId, Long tokenId) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling createTransactionForTokenUpdate");
}
// verify the required parameter 'tokenId' is set
if (tokenId == null) {
throw new IllegalArgumentException("Missing the required parameter 'tokenId' when calling createTransactionForTokenUpdate");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/token/createTransactionForTokenUpdate");
if (spaceId != null) {
String key = "spaceId";
Object value = spaceId;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
if (tokenId != null) {
String key = "tokenId";
Object value = tokenId;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = apiClient.new JacksonJsonHttpContent(null);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
public HttpResponse createTransactionForTokenUpdateForHttpResponse(Long spaceId, Long tokenId, Map params) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling createTransactionForTokenUpdate");
}
// verify the required parameter 'tokenId' is set
if (tokenId == null) {
throw new IllegalArgumentException("Missing the required parameter 'tokenId' when calling createTransactionForTokenUpdate");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/token/createTransactionForTokenUpdate");
// Copy the params argument if present, to allow passing in immutable maps
Map allParams = params == null ? new HashMap() : new HashMap(params);
// Add the required query param 'spaceId' to the map of query params
allParams.put("spaceId", spaceId);
// Add the required query param 'tokenId' to the map of query params
allParams.put("tokenId", tokenId);
for (Map.Entry entryMap: allParams.entrySet()) {
String key = entryMap.getKey();
Object value = entryMap.getValue();
if (key != null && value != null) {
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = apiClient.new JacksonJsonHttpContent(null);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
/**
* Delete
* Deletes the entity with the given id.
* 409 - This status code indicates that there was a conflict with the current version of the data in the database and the provided data in the request.
*
442 - This status code indicates that the server cannot or will not process the request due to something that is perceived to be a client error.
*
542 - This status code indicates that the server encountered an unexpected condition that prevented it from fulfilling the client request.
* @param spaceId
* @param id
* @throws IOException if an error occurs while attempting to invoke the API
* For more information visit this link.
* @see Delete Documentation
**/
public void delete(Long spaceId, Long id) throws IOException {
deleteForHttpResponse(spaceId, id);
}
/**
* Delete
* Deletes the entity with the given id.
*
409 - This status code indicates that there was a conflict with the current version of the data in the database and the provided data in the request.
*
442 - This status code indicates that the server cannot or will not process the request due to something that is perceived to be a client error.
*
542 - This status code indicates that the server encountered an unexpected condition that prevented it from fulfilling the client request.
* @param spaceId
* @param id
* @param params Map of query params. A collection will be interpreted as passing in multiple instances of the same query param.
* @throws IOException if an error occurs while attempting to invoke the API
* For more information visit this link.
* @see Delete Documentation
**/
public void delete(Long spaceId, Long id, Map params) throws IOException {
deleteForHttpResponse(spaceId, id, params);
}
public HttpResponse deleteForHttpResponse(Long spaceId, Long id) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling delete");
}
// verify the required parameter 'id' is set
if (id == null) {
throw new IllegalArgumentException("Missing the required parameter 'id' when calling delete");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/token/delete");
if (spaceId != null) {
String key = "spaceId";
Object value = spaceId;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = apiClient.new JacksonJsonHttpContent(id);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
public HttpResponse deleteForHttpResponse(Long spaceId, java.io.InputStream id, String mediaType) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling delete");
}
// verify the required parameter 'id' is set
if (id == null) {
throw new IllegalArgumentException("Missing the required parameter 'id' when calling delete");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/token/delete");
if (spaceId != null) {
String key = "spaceId";
Object value = spaceId;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = id == null ?
apiClient.new JacksonJsonHttpContent(null) :
new InputStreamContent(mediaType == null ? Json.MEDIA_TYPE : mediaType, id);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
public HttpResponse deleteForHttpResponse(Long spaceId, Long id, Map params) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling delete");
}
// verify the required parameter 'id' is set
if (id == null) {
throw new IllegalArgumentException("Missing the required parameter 'id' when calling delete");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/token/delete");
// Copy the params argument if present, to allow passing in immutable maps
Map allParams = params == null ? new HashMap() : new HashMap(params);
// Add the required query param 'spaceId' to the map of query params
allParams.put("spaceId", spaceId);
for (Map.Entry entryMap: allParams.entrySet()) {
String key = entryMap.getKey();
Object value = entryMap.getValue();
if (key != null && value != null) {
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = apiClient.new JacksonJsonHttpContent(id);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
/**
* Process Transaction
* This operation processes the given transaction by using the token associated with the transaction.
* 200 - This status code indicates that a client request was successfully received, understood, and accepted.
*
442 - This status code indicates that the server cannot or will not process the request due to something that is perceived to be a client error.
*
542 - This status code indicates that the server encountered an unexpected condition that prevented it from fulfilling the client request.
* @param spaceId
* @param transactionId The id of the transaction for which we want to check if the token can be created or not.
* @return Charge
* @throws IOException if an error occurs while attempting to invoke the API
* For more information visit this link.
* @see Process Transaction Documentation
**/
public Charge processTransaction(Long spaceId, Long transactionId) throws IOException {
HttpResponse response = processTransactionForHttpResponse(spaceId, transactionId);
String returnType = "Charge";
if(returnType.equals("String")){
return (Charge) (Object) response.parseAsString();
}
TypeReference typeRef = new TypeReference() {};
if (isNoBodyResponse(response)) {
throw new WalleeSdkException(ErrorCode.ENTITY_NOT_FOUND, "Entity was not found for: " + typeRef.getType().getTypeName());
}
return (Charge)apiClient.getObjectMapper().readValue(response.getContent(), typeRef);
}
/**
* Process Transaction
* This operation processes the given transaction by using the token associated with the transaction.
* 200 - This status code indicates that a client request was successfully received, understood, and accepted.
*
442 - This status code indicates that the server cannot or will not process the request due to something that is perceived to be a client error.
*
542 - This status code indicates that the server encountered an unexpected condition that prevented it from fulfilling the client request.
* @param spaceId
* @param transactionId The id of the transaction for which we want to check if the token can be created or not.
* @param params Map of query params. A collection will be interpreted as passing in multiple instances of the same query param.
* @return Charge
* @throws IOException if an error occurs while attempting to invoke the API
* For more information visit this link.
* @see Process Transaction Documentation
**/
public Charge processTransaction(Long spaceId, Long transactionId, Map params) throws IOException {
HttpResponse response = processTransactionForHttpResponse(spaceId, transactionId, params);
String returnType = "Charge";
if(returnType.equals("String")){
return (Charge) (Object) response.parseAsString();
}
TypeReference typeRef = new TypeReference() {};
if (isNoBodyResponse(response)) {
throw new WalleeSdkException(ErrorCode.ENTITY_NOT_FOUND, "Entity was not found for: " + typeRef.getType().getTypeName());
}
return (Charge)apiClient.getObjectMapper().readValue(response.getContent(), typeRef);
}
public HttpResponse processTransactionForHttpResponse(Long spaceId, Long transactionId) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling processTransaction");
}
// verify the required parameter 'transactionId' is set
if (transactionId == null) {
throw new IllegalArgumentException("Missing the required parameter 'transactionId' when calling processTransaction");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/token/process-transaction");
if (spaceId != null) {
String key = "spaceId";
Object value = spaceId;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
if (transactionId != null) {
String key = "transactionId";
Object value = transactionId;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = apiClient.new JacksonJsonHttpContent(null);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
public HttpResponse processTransactionForHttpResponse(Long spaceId, Long transactionId, Map params) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling processTransaction");
}
// verify the required parameter 'transactionId' is set
if (transactionId == null) {
throw new IllegalArgumentException("Missing the required parameter 'transactionId' when calling processTransaction");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/token/process-transaction");
// Copy the params argument if present, to allow passing in immutable maps
Map allParams = params == null ? new HashMap() : new HashMap(params);
// Add the required query param 'spaceId' to the map of query params
allParams.put("spaceId", spaceId);
// Add the required query param 'transactionId' to the map of query params
allParams.put("transactionId", transactionId);
for (Map.Entry entryMap: allParams.entrySet()) {
String key = entryMap.getKey();
Object value = entryMap.getValue();
if (key != null && value != null) {
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = apiClient.new JacksonJsonHttpContent(null);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
/**
* Read
* Reads the entity with the given 'id' and returns it.
* 200 - This status code indicates that a client request was successfully received, understood, and accepted.
*
442 - This status code indicates that the server cannot or will not process the request due to something that is perceived to be a client error.
*
542 - This status code indicates that the server encountered an unexpected condition that prevented it from fulfilling the client request.
* @param spaceId
* @param id The id of the token which should be returned.
* @return Token
* @throws IOException if an error occurs while attempting to invoke the API
* For more information visit this link.
* @see Read Documentation
**/
public Token read(Long spaceId, Long id) throws IOException {
HttpResponse response = readForHttpResponse(spaceId, id);
String returnType = "Token";
if(returnType.equals("String")){
return (Token) (Object) response.parseAsString();
}
TypeReference typeRef = new TypeReference() {};
if (isNoBodyResponse(response)) {
throw new WalleeSdkException(ErrorCode.ENTITY_NOT_FOUND, "Entity was not found for: " + typeRef.getType().getTypeName());
}
return (Token)apiClient.getObjectMapper().readValue(response.getContent(), typeRef);
}
/**
* Read
* Reads the entity with the given 'id' and returns it.
* 200 - This status code indicates that a client request was successfully received, understood, and accepted.
*
442 - This status code indicates that the server cannot or will not process the request due to something that is perceived to be a client error.
*
542 - This status code indicates that the server encountered an unexpected condition that prevented it from fulfilling the client request.
* @param spaceId
* @param id The id of the token which should be returned.
* @param params Map of query params. A collection will be interpreted as passing in multiple instances of the same query param.
* @return Token
* @throws IOException if an error occurs while attempting to invoke the API
* For more information visit this link.
* @see Read Documentation
**/
public Token read(Long spaceId, Long id, Map params) throws IOException {
HttpResponse response = readForHttpResponse(spaceId, id, params);
String returnType = "Token";
if(returnType.equals("String")){
return (Token) (Object) response.parseAsString();
}
TypeReference typeRef = new TypeReference() {};
if (isNoBodyResponse(response)) {
throw new WalleeSdkException(ErrorCode.ENTITY_NOT_FOUND, "Entity was not found for: " + typeRef.getType().getTypeName());
}
return (Token)apiClient.getObjectMapper().readValue(response.getContent(), typeRef);
}
public HttpResponse readForHttpResponse(Long spaceId, Long id) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling read");
}
// verify the required parameter 'id' is set
if (id == null) {
throw new IllegalArgumentException("Missing the required parameter 'id' when calling read");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/token/read");
if (spaceId != null) {
String key = "spaceId";
Object value = spaceId;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
if (id != null) {
String key = "id";
Object value = id;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = null;
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.GET, genericUrl, content);
httpRequest.getHeaders().setContentType("*/*");
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
public HttpResponse readForHttpResponse(Long spaceId, Long id, Map params) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling read");
}
// verify the required parameter 'id' is set
if (id == null) {
throw new IllegalArgumentException("Missing the required parameter 'id' when calling read");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/token/read");
// Copy the params argument if present, to allow passing in immutable maps
Map allParams = params == null ? new HashMap() : new HashMap(params);
// Add the required query param 'spaceId' to the map of query params
allParams.put("spaceId", spaceId);
// Add the required query param 'id' to the map of query params
allParams.put("id", id);
for (Map.Entry entryMap: allParams.entrySet()) {
String key = entryMap.getKey();
Object value = entryMap.getValue();
if (key != null && value != null) {
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = null;
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.GET, genericUrl, content);
httpRequest.getHeaders().setContentType("*/*");
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
/**
* Search
* Searches for the entities as specified by the given query.
* 200 - This status code indicates that a client request was successfully received, understood, and accepted.
*
442 - This status code indicates that the server cannot or will not process the request due to something that is perceived to be a client error.
*
542 - This status code indicates that the server encountered an unexpected condition that prevented it from fulfilling the client request.
* @param spaceId
* @param query The query restricts the tokens which are returned by the search.
* @return List<Token>
* @throws IOException if an error occurs while attempting to invoke the API
* For more information visit this link.
* @see Search Documentation
**/
public List search(Long spaceId, EntityQuery query) throws IOException {
HttpResponse response = searchForHttpResponse(spaceId, query);
String returnType = "List<Token>";
if(returnType.equals("String")){
return (List) (Object) response.parseAsString();
}
TypeReference typeRef = new TypeReference>() {};
if (isNoBodyResponse(response)) {
throw new WalleeSdkException(ErrorCode.ENTITY_NOT_FOUND, "Entity was not found for: " + typeRef.getType().getTypeName());
}
return (List)apiClient.getObjectMapper().readValue(response.getContent(), typeRef);
}
/**
* Search
* Searches for the entities as specified by the given query.
* 200 - This status code indicates that a client request was successfully received, understood, and accepted.
*
442 - This status code indicates that the server cannot or will not process the request due to something that is perceived to be a client error.
*
542 - This status code indicates that the server encountered an unexpected condition that prevented it from fulfilling the client request.
* @param spaceId
* @param query The query restricts the tokens which are returned by the search.
* @param params Map of query params. A collection will be interpreted as passing in multiple instances of the same query param.
* @return List<Token>
* @throws IOException if an error occurs while attempting to invoke the API
* For more information visit this link.
* @see Search Documentation
**/
public List search(Long spaceId, EntityQuery query, Map params) throws IOException {
HttpResponse response = searchForHttpResponse(spaceId, query, params);
String returnType = "List<Token>";
if(returnType.equals("String")){
return (List) (Object) response.parseAsString();
}
TypeReference typeRef = new TypeReference>() {};
if (isNoBodyResponse(response)) {
throw new WalleeSdkException(ErrorCode.ENTITY_NOT_FOUND, "Entity was not found for: " + typeRef.getType().getTypeName());
}
return (List)apiClient.getObjectMapper().readValue(response.getContent(), typeRef);
}
public HttpResponse searchForHttpResponse(Long spaceId, EntityQuery query) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling search");
}
// verify the required parameter 'query' is set
if (query == null) {
throw new IllegalArgumentException("Missing the required parameter 'query' when calling search");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/token/search");
if (spaceId != null) {
String key = "spaceId";
Object value = spaceId;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = apiClient.new JacksonJsonHttpContent(query);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
public HttpResponse searchForHttpResponse(Long spaceId, java.io.InputStream query, String mediaType) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling search");
}
// verify the required parameter 'query' is set
if (query == null) {
throw new IllegalArgumentException("Missing the required parameter 'query' when calling search");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/token/search");
if (spaceId != null) {
String key = "spaceId";
Object value = spaceId;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = query == null ?
apiClient.new JacksonJsonHttpContent(null) :
new InputStreamContent(mediaType == null ? Json.MEDIA_TYPE : mediaType, query);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
public HttpResponse searchForHttpResponse(Long spaceId, EntityQuery query, Map params) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling search");
}
// verify the required parameter 'query' is set
if (query == null) {
throw new IllegalArgumentException("Missing the required parameter 'query' when calling search");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/token/search");
// Copy the params argument if present, to allow passing in immutable maps
Map allParams = params == null ? new HashMap() : new HashMap(params);
// Add the required query param 'spaceId' to the map of query params
allParams.put("spaceId", spaceId);
for (Map.Entry entryMap: allParams.entrySet()) {
String key = entryMap.getKey();
Object value = entryMap.getValue();
if (key != null && value != null) {
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = apiClient.new JacksonJsonHttpContent(query);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
/**
* Update
* This updates the entity with the given properties. Only those properties which should be updated can be provided. The 'id' and 'version' are required to identify the entity.
* 200 - This status code indicates that a client request was successfully received, understood, and accepted.
*
409 - This status code indicates that there was a conflict with the current version of the data in the database and the provided data in the request.
*
442 - This status code indicates that the server cannot or will not process the request due to something that is perceived to be a client error.
*
542 - This status code indicates that the server encountered an unexpected condition that prevented it from fulfilling the client request.
* @param spaceId
* @param entity The object with all the properties which should be updated. The id and the version are required properties.
* @return Token
* @throws IOException if an error occurs while attempting to invoke the API
* For more information visit this link.
* @see Update Documentation
**/
public Token update(Long spaceId, TokenUpdate entity) throws IOException {
HttpResponse response = updateForHttpResponse(spaceId, entity);
String returnType = "Token";
if(returnType.equals("String")){
return (Token) (Object) response.parseAsString();
}
TypeReference typeRef = new TypeReference() {};
if (isNoBodyResponse(response)) {
throw new WalleeSdkException(ErrorCode.ENTITY_NOT_FOUND, "Entity was not found for: " + typeRef.getType().getTypeName());
}
return (Token)apiClient.getObjectMapper().readValue(response.getContent(), typeRef);
}
/**
* Update
* This updates the entity with the given properties. Only those properties which should be updated can be provided. The 'id' and 'version' are required to identify the entity.
* 200 - This status code indicates that a client request was successfully received, understood, and accepted.
*
409 - This status code indicates that there was a conflict with the current version of the data in the database and the provided data in the request.
*
442 - This status code indicates that the server cannot or will not process the request due to something that is perceived to be a client error.
*
542 - This status code indicates that the server encountered an unexpected condition that prevented it from fulfilling the client request.
* @param spaceId
* @param entity The object with all the properties which should be updated. The id and the version are required properties.
* @param params Map of query params. A collection will be interpreted as passing in multiple instances of the same query param.
* @return Token
* @throws IOException if an error occurs while attempting to invoke the API
* For more information visit this link.
* @see Update Documentation
**/
public Token update(Long spaceId, TokenUpdate entity, Map params) throws IOException {
HttpResponse response = updateForHttpResponse(spaceId, entity, params);
String returnType = "Token";
if(returnType.equals("String")){
return (Token) (Object) response.parseAsString();
}
TypeReference typeRef = new TypeReference() {};
if (isNoBodyResponse(response)) {
throw new WalleeSdkException(ErrorCode.ENTITY_NOT_FOUND, "Entity was not found for: " + typeRef.getType().getTypeName());
}
return (Token)apiClient.getObjectMapper().readValue(response.getContent(), typeRef);
}
public HttpResponse updateForHttpResponse(Long spaceId, TokenUpdate entity) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling update");
}
// verify the required parameter 'entity' is set
if (entity == null) {
throw new IllegalArgumentException("Missing the required parameter 'entity' when calling update");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/token/update");
if (spaceId != null) {
String key = "spaceId";
Object value = spaceId;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = apiClient.new JacksonJsonHttpContent(entity);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
public HttpResponse updateForHttpResponse(Long spaceId, java.io.InputStream entity, String mediaType) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling update");
}
// verify the required parameter 'entity' is set
if (entity == null) {
throw new IllegalArgumentException("Missing the required parameter 'entity' when calling update");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/token/update");
if (spaceId != null) {
String key = "spaceId";
Object value = spaceId;
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = entity == null ?
apiClient.new JacksonJsonHttpContent(null) :
new InputStreamContent(mediaType == null ? Json.MEDIA_TYPE : mediaType, entity);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
public HttpResponse updateForHttpResponse(Long spaceId, TokenUpdate entity, Map params) throws IOException {
// verify the required parameter 'spaceId' is set
if (spaceId == null) {
throw new IllegalArgumentException("Missing the required parameter 'spaceId' when calling update");
}
// verify the required parameter 'entity' is set
if (entity == null) {
throw new IllegalArgumentException("Missing the required parameter 'entity' when calling update");
}
URIBuilder uriBuilder = URIBuilderUtil.create(apiClient.getBasePath() + "/token/update");
// Copy the params argument if present, to allow passing in immutable maps
Map allParams = params == null ? new HashMap() : new HashMap(params);
// Add the required query param 'spaceId' to the map of query params
allParams.put("spaceId", spaceId);
for (Map.Entry entryMap: allParams.entrySet()) {
String key = entryMap.getKey();
Object value = entryMap.getValue();
if (key != null && value != null) {
uriBuilder = URIBuilderUtil.applyQueryParam(uriBuilder, key, value);
}
}
GenericUrl genericUrl = new GenericUrl(URIBuilderUtil.build(uriBuilder));
HttpContent content = apiClient.new JacksonJsonHttpContent(entity);
HttpRequest httpRequest = apiClient.getHttpRequestFactory().buildRequest(HttpMethods.POST, genericUrl, content);
int readTimeOut = apiClient.getReadTimeOut() * 1000;
httpRequest.setReadTimeout(readTimeOut);
return httpRequest.execute();
}
private boolean isNoBodyResponse(HttpResponse response) throws IOException {
java.io.InputStream content = response.getContent();
return content.available() == 0;
}
}