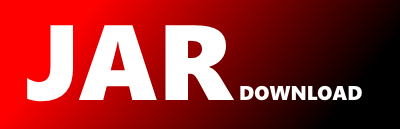
com.wandoulabs.nedis.protocol.SortedSetsCommands Maven / Gradle / Ivy
/**
* Copyright (c) 2015 Wandoujia Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.wandoulabs.nedis.protocol;
import io.netty.util.concurrent.Future;
import java.util.List;
import java.util.Map;
/**
* @author Apache9
* @see http://redis.io/commands#sorted_set
*/
public interface SortedSetsCommands {
Future zadd(byte[] key, double score, byte[] member);
Future zadd(byte[] key, Map member2Score);
Future zcard(byte[] key);
Future zcount(byte[] key, byte[] min, byte[] max);
Future zincrby(byte[] key, double delta, byte[] member);
Future zinterstore(byte[] dst, byte[]... keys);
Future zinterstore(byte[] dst, ZSetOpParams params);
Future zlexcount(byte[] key, byte[] min, byte[] max);
Future> zrange(byte[] key, long startInclusive, long stopInclusive);
Future> zrangeWithScores(byte[] key, long startInclusive,
long stopInclusive);
Future> zrangebylex(byte[] key, byte[] min, byte[] max);
Future> zrangebylex(byte[] key, byte[] min, byte[] max, long offset, long count);
Future> zrangebyscore(byte[] key, byte[] min, byte[] max);
Future> zrangebyscore(byte[] key, byte[] min, byte[] max, long offset, long count);
Future> zrangebyscoreWithScores(byte[] key, byte[] min, byte[] max);
Future> zrangebyscoreWithScores(byte[] key, byte[] min, byte[] max,
long offset, long count);
Future zrank(byte[] key, byte[] member);
Future zrem(byte[] key, byte[]... members);
Future zremrangebylex(byte[] key, byte[] min, byte[] max);
Future zremrangebyrank(byte[] key, long startInclusive, long stopInclusive);
Future zremrangebyscore(byte[] key, byte[] min, byte[] max);
Future> zrevrange(byte[] key, long startInclusive, long stopInclusive);
Future> zrevrangeWithScores(byte[] key, long startInclusive,
long stopInclusive);
Future> zrevrangebylex(byte[] key, byte[] max, byte[] min);
Future> zrevrangebylex(byte[] key, byte[] max, byte[] min, long offset, long count);
Future> zrevrangebyscore(byte[] key, byte[] max, byte[] min);
Future> zrevrangebyscore(byte[] key, byte[] max, byte[] min, long offset,
long count);
Future> zrevrangebyscoreWithScores(byte[] key, byte[] max, byte[] min);
Future> zrevrangebyscoreWithScores(byte[] key, byte[] max, byte[] min,
long offset, long count);
Future zrevrank(byte[] key, byte[] member);
Future> zscan(byte[] key, ScanParams params);
Future zscore(byte[] key, byte[] member);
Future zunionstore(byte[] dst, byte[]... keys);
Future zunionstore(byte[] dst, ZSetOpParams params);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy