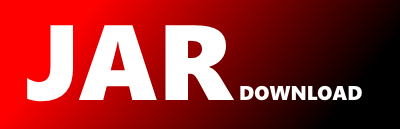
com.wandrell.tabletop.interval.table.IntervalsTable Maven / Gradle / Ivy
Show all versions of intervals Show documentation
/**
* Copyright 2015 the original author or authors
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package com.wandrell.tabletop.interval.table;
import java.util.Map;
import com.wandrell.tabletop.interval.Interval;
/**
* Interface for representing an intervals table. This is a table composed of
* consecutive intervals, each of them having a value assigned to it. Another
* way to see it is as an interval divided into several smaller intervals, each
* with a value assigned to it.
*
* Each of the contained intervals is mapped to a value, which can be anything.
* Some common values are score points or items such as weapons or armor, but
* may include more abstract concepts like deployment zones or in-game events.
*
* For example, this is Bloodbowl's weather table:
*
*
* Interval
* Result
*
*
* 2
* Sweltering Heat
*
*
* 3
* Very Sunny
*
*
* 4-10
* Nice
*
*
* 11
* Pouring Rain
*
*
* 12
* Blizzard
*
*
*
* Here weather events are mapped to the different intervals, some of them being
* not proper intervals.
*
* To use this table two dice of six sides are rolled, and the result is
* compared to the first column. Then the weather event on the second column is
* applied to the game.
*
* @author Bernardo Martínez Garrido
* @param
* the type of the values assigned to the intervals
*/
public interface IntervalsTable extends Interval {
/**
* Returns all the intervals and their assigned values.
*
* @return all the values and their value
*/
public Map getIntervals();
/**
* Gets the value assigned to the specified number.
*
* This will search for the interval containing the number, and then return
* the value assigned to it.
*
* If the value is not contained on the table, it is expected to indicate
* this through an {@code IndexOutOfBoundsException} exception.
*
* @param number
* the number whose value to find
* @return the value assigned to the number
*/
public V getValue(final Integer number);
}