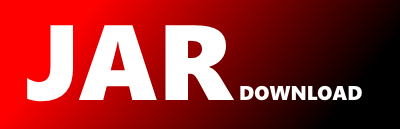
com.wavefront.agent.channel.PlainTextOrHttpFrameDecoder Maven / Gradle / Ivy
package com.wavefront.agent.channel;
import com.google.common.base.Charsets;
import io.netty.buffer.ByteBuf;
import io.netty.channel.ChannelHandler;
import io.netty.channel.ChannelHandlerContext;
import io.netty.channel.ChannelPipeline;
import io.netty.handler.codec.ByteToMessageDecoder;
import io.netty.handler.codec.compression.ZlibCodecFactory;
import io.netty.handler.codec.compression.ZlibWrapper;
import io.netty.handler.codec.http.HttpContentDecompressor;
import io.netty.handler.codec.http.HttpRequestDecoder;
import io.netty.handler.codec.http.HttpResponseEncoder;
import io.netty.handler.codec.http.cors.CorsConfig;
import io.netty.handler.codec.http.cors.CorsHandler;
import io.netty.handler.codec.string.StringDecoder;
import io.netty.handler.codec.string.StringEncoder;
import java.util.List;
import java.util.logging.Logger;
import javax.annotation.Nullable;
/**
* This class handles 2 different protocols on a single port. Supported protocols include HTTP and a
* plain text protocol. It dynamically adds the appropriate encoder/decoder based on the detected
* protocol. This class was largely adapted from the example provided with netty v4.0
*
* @see Netty
* Port Unification Example
* @author Mike McLaughlin ([email protected])
*/
public final class PlainTextOrHttpFrameDecoder extends ByteToMessageDecoder {
protected static final Logger logger =
Logger.getLogger(PlainTextOrHttpFrameDecoder.class.getName());
/** The object for handling requests of either protocol */
private final ChannelHandler handler;
private final boolean detectGzip;
@Nullable private final CorsConfig corsConfig;
private final int maxLengthPlaintext;
private final int maxLengthHttp;
private static final StringDecoder STRING_DECODER = new StringDecoder(Charsets.UTF_8);
private static final StringEncoder STRING_ENCODER = new StringEncoder(Charsets.UTF_8);
/**
* @param handler the object responsible for handling the incoming messages on either protocol.
* @param corsConfig enables CORS when {@link CorsConfig} is specified
* @param maxLengthPlaintext max allowed line length for line-delimiter protocol
* @param maxLengthHttp max allowed size for incoming HTTP requests
*/
public PlainTextOrHttpFrameDecoder(
final ChannelHandler handler,
@Nullable final CorsConfig corsConfig,
int maxLengthPlaintext,
int maxLengthHttp) {
this(handler, corsConfig, maxLengthPlaintext, maxLengthHttp, true);
}
private PlainTextOrHttpFrameDecoder(
final ChannelHandler handler,
@Nullable final CorsConfig corsConfig,
int maxLengthPlaintext,
int maxLengthHttp,
boolean detectGzip) {
this.handler = handler;
this.corsConfig = corsConfig;
this.maxLengthPlaintext = maxLengthPlaintext;
this.maxLengthHttp = maxLengthHttp;
this.detectGzip = detectGzip;
}
/**
* Dynamically adds the appropriate encoder/decoder(s) to the pipeline based on the detected
* protocol.
*/
@Override
protected void decode(final ChannelHandlerContext ctx, final ByteBuf buffer, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy