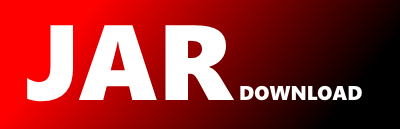
com.wavefront.agent.handlers.LogSenderTask Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proxy Show documentation
Show all versions of proxy Show documentation
Service for batching and relaying metric traffic to Wavefront
package com.wavefront.agent.handlers;
import com.wavefront.agent.data.EntityProperties;
import com.wavefront.agent.data.LogDataSubmissionTask;
import com.wavefront.agent.data.QueueingReason;
import com.wavefront.agent.data.TaskResult;
import com.wavefront.agent.queueing.TaskQueue;
import com.wavefront.api.LogAPI;
import com.wavefront.dto.Log;
import java.util.List;
import java.util.UUID;
import java.util.concurrent.ScheduledExecutorService;
import javax.annotation.Nullable;
/**
* This class is responsible for accumulating logs and uploading them in batches.
*
* @author [email protected]
*/
public class LogSenderTask extends AbstractSenderTask {
private final LogAPI logAPI;
private final UUID proxyId;
private final TaskQueue backlog;
/**
* @param handlerKey handler key, that serves as an identifier of the log pipeline.
* @param logAPI handles interaction with log systems as well as queueing.
* @param proxyId id of the proxy.
* @param threadId thread number.
* @param properties container for mutable proxy settings.
* @param scheduler executor service for running this task
* @param backlog backing queue
*/
LogSenderTask(
HandlerKey handlerKey,
LogAPI logAPI,
UUID proxyId,
int threadId,
EntityProperties properties,
ScheduledExecutorService scheduler,
TaskQueue backlog) {
super(handlerKey, threadId, properties, scheduler);
this.logAPI = logAPI;
this.proxyId = proxyId;
this.backlog = backlog;
}
@Override
TaskResult processSingleBatch(List batch) {
LogDataSubmissionTask task =
new LogDataSubmissionTask(
logAPI, proxyId, properties, backlog, handlerKey.getHandle(), batch, null);
return task.execute();
}
@Override
public void flushSingleBatch(List batch, @Nullable QueueingReason reason) {
LogDataSubmissionTask task =
new LogDataSubmissionTask(
logAPI, proxyId, properties, backlog, handlerKey.getHandle(), batch, null);
task.enqueue(reason);
}
@Override
protected int getDataSize(List batch) {
int size = 0;
for (Log l : batch) {
size += l.getDataSize();
}
return size;
}
@Override
protected int getBlockSize(List datum, int rateLimit, int batchSize) {
int maxDataSize = Math.min(rateLimit, batchSize);
int size = 0;
for (int i = 0; i < datum.size(); i++) {
size += datum.get(i).getDataSize();
if (size > maxDataSize) {
return i;
}
}
return datum.size();
}
@Override
protected int getObjectSize(Log object) {
return object.getDataSize();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy