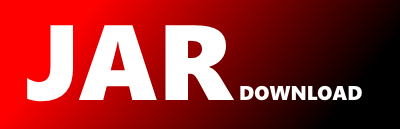
com.wavefront.agent.queueing.TaskQueue Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proxy Show documentation
Show all versions of proxy Show documentation
Service for batching and relaying metric traffic to Wavefront
package com.wavefront.agent.queueing;
import com.wavefront.agent.data.DataSubmissionTask;
import java.io.IOException;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
/**
* Proxy-specific queue interface, which is basically a wrapper for a Tape queue. This allows us to
* potentially support more than one backing storage in the future.
*
* @param type of objects stored.
* @author [email protected].
*/
public interface TaskQueue> extends Iterable {
/**
* Retrieve a task that is currently at the head of the queue.
*
* @return task object
*/
@Nullable
T peek();
/**
* Add a task to the end of the queue
*
* @param entry task
* @throws IOException IO exceptions caught by the storage engine
*/
void add(@Nonnull T entry) throws IOException;
/**
* Remove a task from the head of the queue. Requires peek() to be called first, otherwise an
* {@code IllegalStateException} is thrown.
*
* @throws IOException IO exceptions caught by the storage engine
*/
void remove() throws IOException;
/** Empty and re-initialize the queue. */
void clear() throws IOException;
/**
* Returns a number of tasks currently in the queue.
*
* @return number of tasks
*/
int size();
/** Close the queue. Should be invoked before a graceful shutdown. */
void close() throws IOException;
/**
* Returns the total weight of the queue (sum of weights of all tasks).
*
* @return weight of the queue (null if unknown)
*/
@Nullable
Long weight();
/**
* Returns the total number of pre-allocated but unused bytes in the backing file. May return null
* if not applicable.
*
* @return total number of available bytes in the file or null
*/
@Nullable
Long getAvailableBytes();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy