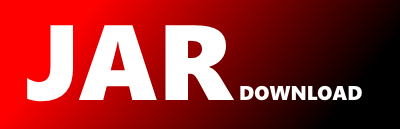
com.wavesplatform.DocSource.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lang Show documentation
Show all versions of lang Show documentation
The RIDE smart contract language compiler
The newest version!
package com.wavesplatform
object DocSource {
private val regex = "\\[(.+?)\\]\\(.+?\\)".r
lazy val funcsV3 = Map((("getBinaryValue", List("List[DataEntry]", "String"), 3), ("Find and extract data by key (fail on error)", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), (("getBoolean", List("List[DataEntry]", "Int"), 3), ("Extract data by index", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 30)), (("log", List("Int", "Int", "Int", "Int", "Int", "Ceiling|Down|Floor|HalfDown|HalfEven|HalfUp|Up"), 3), ("Returns the logarithm of a number.", List("The base.", "The number of decimals of the base.", "The exponent.", "The number of decimals of the exponent.", "The number of decimals of the resulting value.", "The rounding function.\n"), "math-functions", 100)), (("toBase64String", List("ByteVector"), 3), ("Encodes array of bytes to [Base64](https://en.wikipedia.org/wiki/Base64) string.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 10)), (("*", List("Int", "Int"), 3), ("Multiply integers.", List("Multiplier", "Multiplier"), "operators", 1)), (("toString", List("Int"), 3), ("Converts an integer to a string.", List("The integer to convert."), "converting-functions", 1)), (("getIntegerValue", List("List[DataEntry]", "Int"), 3), ("Extract data by index (fail on error)", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 30)), (("toInt", List("ByteVector"), 3), ("Converts an array of bytes to an integer.", List("The array of bytes to convert."), "converting-functions", 10)), (("toBase16String", List("ByteVector"), 3), ("Encodes array of bytes to [Base16](https://en.wikipedia.org/wiki/Hexadecimal) string.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 10)), (("addressFromRecipient", List("Address|Alias"), 3), ("Extract address or lookup alias", List("Address or alias of the account."), "converting-functions", 100)), (("parseIntValue", List("String"), 3), ("Converts the string representation of a number to its integer equivalent.\nRaises an exception if the string cannot be parsed.", List("The string to parse."), "converting-functions", 20)), (("addressFromPublicKey", List("ByteVector"), 3), ("Converts account public key to [address](blockhain/address.md).", List("The public key to convert."), "converting-functions", 82)), (("takeRight", List("ByteVector", "Int"), 3), ("Takes the last n bytes from an array of bytes.", List("The array of bytes.", "The number n."), "byte-array-functions", 19)), (("parseInt", List("String"), 3), ("Converts the string representation of a number to its integer equivalent.", List("The string to parse."), "converting-functions", 20)), (("split", List("String", "String"), 3), ("Splits a string delimited by a separator into a list of substrings", List("The string.", "The separator."), "string-functions", 100)), (("getStringValue", List("List[DataEntry]", "String"), 3), ("Find and extract data by key (fail on error)", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), (("==", List("T", "T"), 3), ("Check equality.", List("First value", "Second value"), "operators", 1)), (("getBooleanValue", List("List[DataEntry]", "String"), 3), ("Find and extract data by key (fail on error)", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), (("rsaVerify", List("Md5|NoAlg|Sha1|Sha224|Sha256|Sha3224|Sha3256|Sha3384|Sha3512|Sha384|Sha512", "ByteVector", "ByteVector", "ByteVector"), 3), ("Verifies an [RSA](https://en.wikipedia.org/wiki/RSA_%28cryptosystem%29) signature.", List("The RSA algorithm.", "The message.", "The signature.", "The public key."), "verification-functions", 300)), (("blockInfoByHeight", List("Int"), 3), ("Gets the information about a [block](/blockchain/block.md) by the [block height](/blockchain/block-height.md)", List("Block height."), "blockchain-functions", 100)), (("toString", List("Address"), 3), ("Convert address bytes to string", List("The address to convert"), "converting-functions", 10)), (("toBytes", List("Boolean"), 3), ("Converts a boolean to an array of bytes.", List("The boolean to convert."), "converting-functions", 1)), (("lastIndexOf", List("String", "String", "Int"), 3), ("Returns the index of the last occurrence of a substring after a certain index", List("The string.", "The substring.", "The index."), "string-functions", 20)), (("take", List("String", "Int"), 3), ("Takes the first n characters from a string", List("The string.", "The number n."), "string-functions", 1)), (("getBooleanValue", List("List[DataEntry]", "Int"), 3), ("Extract data by index (fail on error)", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 30)), (("getBooleanValue", List("Address|Alias", "String"), 3), ("Gets a boolean value by key. Throws an exception if there is no data.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 100)), (("pow", List("Int", "Int", "Int", "Int", "Int", "Ceiling|Down|Floor|HalfDown|HalfEven|HalfUp|Up"), 3), ("Returns a number raised to a power", List("The base.", "The number of decimals of the base.", "The exponent.", "The number of decimals of the exponent.", "The number of decimals of the resulting value.", "One of the rounding functions."), "math-functions", 100)), (("checkMerkleProof", List("ByteVector", "ByteVector", "ByteVector"), 3), ("Verifies if a tree of hashes is part of the [Merkle tree](https://en.wikipedia.org/wiki/Merkle_tree).", List("The root hash of the Merkle tree.", "The array of bytes of the Merkle tree proof.", "The tree of hashes."), "verification-functions", 30)), (("fromBase64String", List("String"), 3), ("Decodes [Base64](https://en.wikipedia.org/wiki/Base64) string to an array of bytes.", List("The string to decode."), "encoding-and-decoding-functions", 10)), (("+", List("ByteVector", "ByteVector"), 3), ("Concat limited byte vectors.", List("First value", "Second value"), "operators", 10)), ((">", List("Int", "Int"), 3), ("Check if integer greater comparison.", List("First value", "Second value"), "operators", 1)), (("getBinaryValue", List("List[DataEntry]", "Int"), 3), ("Extract data by index (fail on error)", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 30)), (("sha256", List("ByteVector"), 3), ("[SHA-256](https://en.wikipedia.org/wiki/SHA-2) hash function.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 10)), (("indexOf", List("String", "String", "Int"), 3), ("Returns the index of the first occurrence of a substring after a certain index", List("The string.", "The substring.", "The index."), "string-functions", 20)), (("isDefined", List("T|Unit"), 3), ("Checks if a value is not `Unit`", List("Optional value"), "optional-value-functions", 1)), (("indexOf", List("String", "String"), 3), ("Returns the index of the first occurrence of a substring", List("The string.", "The substring."), "string-functions", 20)), (("%", List("Int", "Int"), 3), ("Calculate modulo.", List("Divisible", "Divisor"), "operators", 1)), (("getBinary", List("Address|Alias", "String"), 3), ("Gets an array of bytes by key.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 100)), (("-", List("Int"), 3), ("Change integer sign.", List("Value"), "operators", 1)), (("/", List("Int", "Int"), 3), ("Divide integers.", List("Divisible", "Divisor"), "operators", 1)), (("fromBase16String", List("String"), 3), ("Decodes [Base16](https://en.wikipedia.org/wiki/Hexadecimal) string to an array of bytes.", List("The string to decode."), "encoding-and-decoding-functions", 10)), (("getInteger", List("List[DataEntry]", "String"), 3), ("Find and extract data by key", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), (("getIntegerValue", List("List[DataEntry]", "String"), 3), ("Find and extract data by key (fail on error)", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), (("toString", List("Boolean"), 3), ("Converts a boolean to a string.", List("The boolean to convert."), "converting-functions", 1)), (("getStringValue", List("Address|Alias", "String"), 3), ("Gets a string by key. Throws an exception if there is no data.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 100)), (("getElement", List("List[T]", "Int"), 3), ("Returns the size of a list.", List("The list.", "The index of the element."), "list-functions", 2)), (("drop", List("ByteVector", "Int"), 3), ("Drops the first n bytes of an array of bytes.", List("The array of bytes.", "The number n."), "byte-array-functions", 1)), (("getInteger", List("Address|Alias", "String"), 3), ("Gets an integer by key.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 100)), (("getBinary", List("List[DataEntry]", "Int"), 3), ("Extract data by index", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 30)), (("dropRight", List("ByteVector", "Int"), 3), ("Drops the first n bytes of an array of bytes.", List("The array of bytes.", "The number n."), "byte-array-functions", 19)), (("size", List("String"), 3), ("Returns the size of a string", List("The string."), "string-functions", 1)), (("blake2b256", List("ByteVector"), 3), ("[BLAKE-256](https://en.wikipedia.org/wiki/BLAKE_%28hash_function%29) hash function.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 10)), (("-", List("Int", "Int"), 3), ("Substitute integers.", List("First value", "Second value"), "operators", 1)), (("transactionHeightById", List("ByteVector"), 3), ("Gets the [block height](/blockchain/block-height.md) of a transaction.", List("ID of the transaction."), "blockchain-functions", 100)), (("getBinaryValue", List("Address|Alias", "String"), 3), ("Gets an array of bytes by key. Throws an exception if there is no data.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 100)), (("toInt", List("ByteVector", "Int"), 3), ("Converts an array of bytes to an integer starting from a certain index.", List("The array of bytes to convert.", "The index to start from."), "converting-functions", 10)), (("toBytes", List("String"), 3), ("Converts a string to an array of bytes.", List("The string to convert."), "converting-functions", 1)), (("assetInfo", List("ByteVector"), 3), ("Gets the information about a [token](/blockchain/token.md).", List("ID of the [token](/blockchain/token.md)."), "blockchain-functions", 100)), (("getString", List("Address|Alias", "String"), 3), ("Gets a string by key.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 100)), (("fromBase58String", List("String"), 3), ("Decodes [Base58](https://en.wikipedia.org/wiki/Base58) string to an array of bytes.", List("The string to decode."), "encoding-and-decoding-functions", 10)), (("toBytes", List("Int"), 3), ("Converts an integer to an array of bytes.", List("The integer to convert."), "converting-functions", 1)), (("getString", List("List[DataEntry]", "Int"), 3), ("Extract data by index", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 30)), (("+", List("String", "String"), 3), ("Concat limited strings.", List("First value", "Second value"), "operators", 10)), (("takeRight", List("String", "Int"), 3), ("Takes the last n characters from a string", List("The string.", "The number n."), "string-functions", 19)), (("sigVerify", List("ByteVector", "ByteVector", "ByteVector"), 3), ("Verifies a [Curve25519](https://en.wikipedia.org/wiki/Curve25519) signature.", List("The message.", "The signature.", "The account public key."), "verification-functions", 100)), (("value", List("T|Unit"), 3), ("Extract value from option or fail", List("Optional value"), "optional-value-functions", 13)), (("!=", List("T", "T"), 3), ("Check inequality.", List("First value", "Second value"), "operators", 1)), (("transferTransactionById", List("ByteVector"), 3), ("Gets the data of a transfer transaction.", List("ID of the transfer transaction."), "blockchain-functions", 100)), (("size", List("ByteVector"), 3), ("Returns the size of an array of bytes.", List("The array of bytes."), "byte-array-functions", 1)), (("dropRight", List("String", "Int"), 3), ("Drops the last n characters of a string", List("The string.", "The number n."), "string-functions", 19)), (("getBoolean", List("List[DataEntry]", "String"), 3), ("Find and extract data by key", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), (("extract", List("T|Unit"), 3), ("Extract value from option or fail", List("Optional value"), "optional-value-functions", 13)), (("cons", List("A", "List[B]"), 3), ("Gets an element from a list by index.", List("The element.", "The list."), "list-functions", 2)), (("toUtf8String", List("ByteVector"), 3), ("Converts an array of bytes to a UTF-8 string.", List("The array of bytes to convert."), "converting-functions", 20)), (("getIntegerValue", List("Address|Alias", "String"), 3), ("Gets an integer by key. Throws an exception if there is no data.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 100)), (("size", List("List[T]"), 3), ("Prepends a new element to the list.", List("The list."), "list-functions", 2)), (("drop", List("String", "Int"), 3), ("Drops the first n characters of a string", List("The string.", "The number n."), "string-functions", 1)), (("take", List("ByteVector", "Int"), 3), ("Takes the first n bytes from an array of bytes.", List("The array of bytes.", "The number n."), "byte-array-functions", 1)), (("addressFromStringValue", List("String"), 3), ("Decodes address from [Base58](https://en.wikipedia.org/wiki/Base58) string.\nRaises an exception if the address cannot be decoded.", List("The string to decode."), "encoding-and-decoding-functions", 124)), (("throw", List(), 3), ("Raises an exception.", List(), "exception-functions", 1)), (("getInteger", List("List[DataEntry]", "Int"), 3), ("Extract data by index", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 30)), ((">=", List("Int", "Int"), 3), ("Check if integer greater or equal comparison.", List("First value", "Second value"), "operators", 1)), (("addressFromString", List("String"), 3), ("Decodes address from [Base58](https://en.wikipedia.org/wiki/Base58) string.", List("The string to decode."), "encoding-and-decoding-functions", 124)), (("lastIndexOf", List("String", "String"), 3), ("Returns the index of the last occurrence of a substring", List("The string.", "The substring."), "string-functions", 20)), (("getStringValue", List("List[DataEntry]", "Int"), 3), ("Extract data by index (fail on error)", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 30)), (("!", List("Boolean"), 3), ("Unary negation.", List("Value"), "operators", 1)), (("throw", List("String"), 3), ("Raises an exception with a message.", List("The exception message."), "exception-functions", 1)), (("keccak256", List("ByteVector"), 3), ("[Keccak-256](https://keccak.team/files/Keccak-submission-3.pdf) hash function.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 10)), (("getBoolean", List("Address|Alias", "String"), 3), ("Gets a boolean value by key.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 100)), (("assetBalance", List("Address|Alias", "ByteVector|Unit"), 3), ("Gets account balance by token ID.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "Token ID."), "account-data-storage-functions", 100)), (("toBase58String", List("ByteVector"), 3), ("Encodes array of bytes to [Base58](https://en.wikipedia.org/wiki/Base58) string.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 10)), (("getString", List("List[DataEntry]", "String"), 3), ("Find and extract data by key", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), (("valueOrErrorMessage", List("T|Unit", "String"), 3), ("Extract value from option or fail with message", List("Optional value", "Error message"), "optional-value-functions", 13)), (("+", List("Int", "Int"), 3), ("Sum integers.", List("First value", "Second value"), "operators", 1)), (("getBinary", List("List[DataEntry]", "String"), 3), ("Find and extract data by key", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), (("fraction", List("Int", "Int", "Int"), 3), ("Multiply and division with unlimited intermediate representation", List("Multiplier", "Multiplier", "Divisor"), "math-functions", 1)), (("wavesBalance", List("Address|Alias"), 3), ("Gets account balance in [WAVES](/blockchain/token/waves.md).", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account."), "account-data-storage-functions", 109)))
lazy val funcsV4 = Map((("createMerkleRoot", List("List[ByteVector]", "ByteVector", "Int"), 4), ("Calculates the Merkle root hash for transactions of block.", List("Array of sibling hashes of the Merkle tree.", "Hash of transaction.", "Index of the transaction in the block."), "verification-functions", 30)), (("keccak256", List("ByteVector"), 4), ("[Keccak-256](https://keccak.team/files/Keccak-submission-3.pdf) hash function.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 200)), (("addressFromStringValue", List("String"), 4), ("Decodes address from [Base58](https://en.wikipedia.org/wiki/Base58) string.\nRaises an exception if the address cannot be decoded.", List("The string to decode."), "encoding-and-decoding-functions", 1)), (("keccak256_64Kb", List("ByteVector"), 4), ("[Keccak-256](https://keccak.team/files/Keccak-submission-3.pdf) hash function. For array with size <= 64Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 50)), (("drop", List("String", "Int"), 4), ("Drops the first n characters of a string", List("The string.", "The number n."), "string-functions", 20)), (("sigVerify_64Kb", List("ByteVector", "ByteVector", "ByteVector"), 4), ("Verifies a [Curve25519](https://en.wikipedia.org/wiki/Curve25519) signature. For message size <= 64Kb.", List("The message.", "The signature.", "The account public key."), "verification-functions", 102)), (("getElement", List("List[T]", "Int"), 4), ("Gets an element from a list by index.", List("The list.", "The index of the element."), "list-functions", 2)), (("-", List("Int"), 4), ("Change integer sign.", List("Value"), "operators", 1)), (("blake2b256_128Kb", List("ByteVector"), 4), ("[BLAKE-256](https://en.wikipedia.org/wiki/BLAKE_%28hash_function%29) hash function. For array with size <= 128Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 100)), (("rsaVerify_16Kb", List("RsaDigestAlgs", "ByteVector", "ByteVector", "ByteVector"), 4), ("Verifies an [RSA](https://en.wikipedia.org/wiki/RSA_%28cryptosystem%29) signature. For message size <= 16Kb.", List("The RSA algorithm.", "The message.", "The signature.", "The public key."), "verification-functions", 500)), (("rsaVerify_32Kb", List("RsaDigestAlgs", "ByteVector", "ByteVector", "ByteVector"), 4), ("Verifies an [RSA](https://en.wikipedia.org/wiki/RSA_%28cryptosystem%29) signature. For message size <= 32Kb.", List("The RSA algorithm.", "The message.", "The signature.", "The public key."), "verification-functions", 550)), (("++", List("List[A]", "List[B]"), 4), ("List Concatenation.", List("First list", "Second list"), "operators", 4)), (("lastIndexOf", List("String", "String", "Int"), 4), ("Returns the index of the last occurrence of a substring after a certain index", List("The string.", "The substring.", "The index."), "string-functions", 3)), (("blake2b256_16Kb", List("ByteVector"), 4), ("[BLAKE-256](https://en.wikipedia.org/wiki/BLAKE_%28hash_function%29) hash function. For array with size <= 16Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 10)), (("getBinaryValue", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "Int"), 4), ("Extract data by index (fail on error)", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 4)), ((">=", List("Int", "Int"), 4), ("Check if integer greater or equal comparison.", List("First value", "Second value"), "operators", 1)), (("median", List("List[Int]"), 4), ("Returns the median of the list.", List("The list of integers."), "list-functions", 20)), (("bn256Groth16Verify_7inputs", List("ByteVector", "ByteVector", "ByteVector"), 4), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 7 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1150)), (("max", List("List[Int]"), 4), ("Returns the maximum of the list.", List("The list of integers."), "list-functions", 3)), (("groth16Verify_7inputs", List("ByteVector", "ByteVector", "ByteVector"), 4), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 7 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1800)), (("transferTransactionFromProto", List("ByteVector"), 4), ("Deserializes transfer transaction: converts protobuf-encoded binary format to a TransferTransaction structure.", List("Transfer transaction in protobuf-encoded binary format."), "blockchain-functions", 5)), (("value", List("T|Unit"), 4), ("Extract value from option or fail", List("Optional value"), "optional-value-functions", 2)), (("takeRight", List("ByteVector", "Int"), 4), ("Takes the last n bytes from an array of bytes.", List("The array of bytes.", "The number n."), "byte-array-functions", 6)), (("toBase16String", List("ByteVector"), 4), ("Encodes array of bytes to [Base16](https://en.wikipedia.org/wiki/Hexadecimal) string.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 10)), (("indexOf", List("String", "String"), 4), ("Returns the index of the first occurrence of a substring", List("The string.", "The substring."), "string-functions", 3)), (("groth16Verify_14inputs", List("ByteVector", "ByteVector", "ByteVector"), 4), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 14 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 2500)), (("containsElement", List("List[T]", "T"), 4), ("Tests whether the list contains a given value as an element", List("The list.", "The element."), "list-functions", 5)), (("bn256Groth16Verify_15inputs", List("ByteVector", "ByteVector", "ByteVector"), 4), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 15 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1600)), (("bn256Groth16Verify_11inputs", List("ByteVector", "ByteVector", "ByteVector"), 4), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 11 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1350)), (("+", List("ByteVector", "ByteVector"), 4), ("Concat limited byte vectors.", List("First value", "Second value"), "operators", 2)), (("rsaVerify_64Kb", List("RsaDigestAlgs", "ByteVector", "ByteVector", "ByteVector"), 4), ("Verifies an [RSA](https://en.wikipedia.org/wiki/RSA_%28cryptosystem%29) signature. For message size <= 64Kb.", List("The RSA algorithm.", "The message.", "The signature.", "The public key."), "verification-functions", 625)), (("keccak256_32Kb", List("ByteVector"), 4), ("[Keccak-256](https://keccak.team/files/Keccak-submission-3.pdf) hash function. For array with size <= 32Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 25)), (("rsaVerify", List("RsaDigestAlgs", "ByteVector", "ByteVector", "ByteVector"), 4), ("Verifies an [RSA](https://en.wikipedia.org/wiki/RSA_%28cryptosystem%29) signature.", List("The RSA algorithm.", "The message.", "The signature.", "The public key."), "verification-functions", 1000)), (("getBinaryValue", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "String"), 4), ("Find and extract data by key (fail on error)", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), (("bn256Groth16Verify_3inputs", List("ByteVector", "ByteVector", "ByteVector"), 4), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 3 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 950)), (("groth16Verify_13inputs", List("ByteVector", "ByteVector", "ByteVector"), 4), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 13 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 2400)), (("+", List("String", "String"), 4), ("Concat limited strings.", List("First value", "Second value"), "operators", 20)), (("Issue", List("String", "String", "Int", "Int", "Boolean", "Script|Unit", "Int"), 4), ("Issue action detailed constructor", List("Token name", "Token description", "Amount of the token", "Number of digits in decimal part", "Reissue ability flag", "Smart asset script (optional)", "Sequential number"), "internal-functions", 1)), (("drop", List("ByteVector", "Int"), 4), ("Drops the first n bytes of an array of bytes.", List("The array of bytes.", "The number n."), "byte-array-functions", 6)), (("take", List("String", "Int"), 4), ("Takes the first n characters from a string", List("The string.", "The number n."), "string-functions", 20)), (("sigVerify_128Kb", List("ByteVector", "ByteVector", "ByteVector"), 4), ("Verifies a [Curve25519](https://en.wikipedia.org/wiki/Curve25519) signature. For message size <= 128Kb.", List("The message.", "The signature.", "The account public key."), "verification-functions", 172)), (("assetBalance", List("Address|Alias", "ByteVector"), 4), ("Gets account balance by token ID.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "Token ID."), "account-data-storage-functions", 10)), (("bn256Groth16Verify_5inputs", List("ByteVector", "ByteVector", "ByteVector"), 4), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 5 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1050)), (("Issue", List("String", "String", "Int", "Int", "Boolean"), 4), ("Issue action simplified constructor", List("Token name", "Token description", "Amount of the token", "Number of digits in decimal part", "Reissue ability flag"), "internal-functions", 1)), (("getInteger", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "Int"), 4), ("Gets integer from a list of data entries by index", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 4)), (("-", List("Int", "Int"), 4), ("Substitute integers.", List("First value", "Second value"), "operators", 1)), (("size", List("List[T]"), 4), ("Returns the size of a list.", List("The list."), "list-functions", 2)), (("wavesBalance", List("Address|Alias"), 4), ("Gets account waves balance details.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account."), "account-data-storage-functions", 10)), (("getBoolean", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "Int"), 4), ("Gets a boolean value from a list of data entries by index", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 4)), (("parseIntValue", List("String"), 4), ("Converts the string representation of a number to its integer equivalent.\nRaises an exception if the string cannot be parsed.", List("The string to parse."), "converting-functions", 2)), (("getBinary", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "String"), 4), ("Gets a binary value from a list of data entries by key", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), (("getBooleanValue", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "String"), 4), ("Find and extract data by key (fail on error)", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), (("getInteger", List("Address|Alias", "String"), 4), ("Gets an integer by key.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 10)), (("ecrecover", List("ByteVector", "ByteVector"), 4), ("Recovers the public key from hash of ECDSA-encoded message and signature.", List("The hash of encoded message.", "The signature."), "encoding-and-decoding-functions", 70)), (("groth16Verify", List("ByteVector", "ByteVector", "ByteVector"), 4), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 2700)), ((">", List("Int", "Int"), 4), ("Check if integer greater comparison.", List("First value", "Second value"), "operators", 1)), (("dropRight", List("ByteVector", "Int"), 4), ("Drops the first n bytes of an array of bytes.", List("The array of bytes.", "The number n."), "byte-array-functions", 6)), (("sha256_16Kb", List("ByteVector"), 4), ("[SHA-256](https://en.wikipedia.org/wiki/SHA-2) hash function. For array with size <= 16Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 10)), (("sigVerify_8Kb", List("ByteVector", "ByteVector", "ByteVector"), 4), ("Verifies a [Curve25519](https://en.wikipedia.org/wiki/Curve25519) signature. For message size <= 16Kb.", List("The message.", "The signature.", "The account public key."), "verification-functions", 47)), (("getInteger", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "String"), 4), ("Gets integer from a list of data entries by key", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), (("bn256Groth16Verify_8inputs", List("ByteVector", "ByteVector", "ByteVector"), 4), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 8 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1200)), (("cons", List("A", "List[B]"), 4), ("Prepends a new element to the list.", List("The element.", "The list."), "list-functions", 1)), (("+", List("Int", "Int"), 4), ("Sum integers.", List("First value", "Second value"), "operators", 1)), (("bn256Groth16Verify_2inputs", List("ByteVector", "ByteVector", "ByteVector"), 4), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 2 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 850)), (("toBytes", List("Boolean"), 4), ("Converts a boolean to an array of bytes.", List("The boolean to convert."), "converting-functions", 1)), (("size", List("String"), 4), ("Returns the size of a string", List("The string."), "string-functions", 1)), (("throw", List("String"), 4), ("Raises an exception with a message.", List("The exception message."), "exception-functions", 1)), (("fromBase64String", List("String"), 4), ("Decodes [Base64](https://en.wikipedia.org/wiki/Base64) string to an array of bytes.", List("The string to decode."), "encoding-and-decoding-functions", 40)), (("getBoolean", List("Address|Alias", "String"), 4), ("Gets a boolean value by key.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 10)), (("groth16Verify_10inputs", List("ByteVector", "ByteVector", "ByteVector"), 4), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 10 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 2100)), (("getStringValue", List("Address|Alias", "String"), 4), ("Gets a string by key. Throws an exception if there is no data.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 10)), (("bn256Groth16Verify_6inputs", List("ByteVector", "ByteVector", "ByteVector"), 4), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 6 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1100)), (("groth16Verify_1inputs", List("ByteVector", "ByteVector", "ByteVector"), 4), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 1 input.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1200)), (("sigVerify", List("ByteVector", "ByteVector", "ByteVector"), 4), ("Verifies a [Curve25519](https://en.wikipedia.org/wiki/Curve25519) signature.", List("The message.", "The signature.", "The account public key."), "verification-functions", 200)), (("sigVerify_16Kb", List("ByteVector", "ByteVector", "ByteVector"), 4), ("Verifies a [Curve25519](https://en.wikipedia.org/wiki/Curve25519) signature. For message size <= 16Kb.", List("The message.", "The signature.", "The account public key."), "verification-functions", 57)), (("removeByIndex", List("List[T]", "Int"), 4), ("Removes the element at given index from the list and returns new list.", List("The list.", "The element."), "list-functions", 7)), (("toString", List("Boolean"), 4), ("Converts a boolean to a string.", List("The boolean to convert."), "converting-functions", 1)), (("groth16Verify_8inputs", List("ByteVector", "ByteVector", "ByteVector"), 4), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 8 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1900)), (("bn256Groth16Verify_4inputs", List("ByteVector", "ByteVector", "ByteVector"), 4), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 4 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1000)), (("blockInfoByHeight", List("Int"), 4), ("Gets the information about a [block](/blockchain/block.md) by the [block height](/blockchain/block-height.md)", List("Block height."), "blockchain-functions", 5)), (("keccak256_16Kb", List("ByteVector"), 4), ("[Keccak-256](https://keccak.team/files/Keccak-submission-3.pdf) hash function. For array with size <= 16Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 10)), (("bn256Groth16Verify_12inputs", List("ByteVector", "ByteVector", "ByteVector"), 4), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 12 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1400)), (("toUtf8String", List("ByteVector"), 4), ("Converts an array of bytes to a UTF-8 string.", List("The array of bytes to convert."), "converting-functions", 7)), (("groth16Verify_4inputs", List("ByteVector", "ByteVector", "ByteVector"), 4), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 4 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1500)), (("blake2b256", List("ByteVector"), 4), ("[BLAKE-256](https://en.wikipedia.org/wiki/BLAKE_%28hash_function%29) hash function.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 200)), (("sha256_32Kb", List("ByteVector"), 4), ("[SHA-256](https://en.wikipedia.org/wiki/SHA-2) hash function. For array with size <= 32Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 25)), (("fraction", List("Int", "Int", "Int"), 4), ("Multiply and division with unlimited intermediate representation", List("Multiplier", "Multiplier", "Divisor"), "math-functions", 1)), (("groth16Verify_15inputs", List("ByteVector", "ByteVector", "ByteVector"), 4), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 15 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 2600)), (("getBinary", List("Address|Alias", "String"), 4), ("Gets an array of bytes by key.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 10)), (("groth16Verify_9inputs", List("ByteVector", "ByteVector", "ByteVector"), 4), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 9 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 2000)), (("groth16Verify_5inputs", List("ByteVector", "ByteVector", "ByteVector"), 4), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 5 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1600)), (("groth16Verify_11inputs", List("ByteVector", "ByteVector", "ByteVector"), 4), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 11 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 2200)), (("log", List("Int", "Int", "Int", "Int", "Int", "Ceiling|Down|Floor|HalfDown|HalfEven|HalfUp|Up"), 4), ("Returns the logarithm of a number.", List("The base.", "The number of decimals of the base.", "The exponent.", "The number of decimals of the exponent.", "The number of decimals of the resulting value.", "The rounding function.\n"), "math-functions", 100)), (("/", List("Int", "Int"), 4), ("Divide integers.", List("Divisible", "Divisor"), "operators", 1)), (("getString", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "String"), 4), ("Gets a string value from a list of data entries by key", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), (("bn256Groth16Verify_9inputs", List("ByteVector", "ByteVector", "ByteVector"), 4), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 9 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1250)), (("fromBase16String", List("String"), 4), ("Decodes [Base16](https://en.wikipedia.org/wiki/Hexadecimal) string to an array of bytes.", List("The string to decode."), "encoding-and-decoding-functions", 10)), (("getBoolean", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "String"), 4), ("Gets a boolean value from a list of data entries by key", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), (("isDefined", List("T|Unit"), 4), ("Checks if a value is not `Unit`", List("Optional value"), "optional-value-functions", 1)), (("==", List("T", "T"), 4), ("Check equality.", List("First value", "Second value"), "operators", 1)), (("getIntegerValue", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "Int"), 4), ("Extract data by index (fail on error)", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 4)), (("!", List("Boolean"), 4), ("Unary negation.", List("Value"), "operators", 1)), (("bn256Groth16Verify_14inputs", List("ByteVector", "ByteVector", "ByteVector"), 4), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 14 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1550)), (("getStringValue", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "String"), 4), ("Find and extract data by key (fail on error)", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), (("sha256", List("ByteVector"), 4), ("[SHA-256](https://en.wikipedia.org/wiki/SHA-2) hash function.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 200)), (("sha256_128Kb", List("ByteVector"), 4), ("[SHA-256](https://en.wikipedia.org/wiki/SHA-2) hash function. For array with size <= 128Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 100)), (("contains", List("String", "String"), 4), ("Checks whether the string contains substring", List("String to search in.", "String to search for."), "string-functions", 3)), (("%", List("Int", "Int"), 4), ("Calculate modulo.", List("Divisible", "Divisor"), "operators", 1)), (("getIntegerValue", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "String"), 4), ("Find and extract data by key (fail on error)", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), (("throw", List(), 4), ("Raises an exception.", List(), "exception-functions", 1)), (("makeString", List("List[String]", "String"), 4), ("Returns all the elements of the list in a string using the separator string.", List("The list of strings.", "The separator"), "list-functions", 30)), (("transactionHeightById", List("ByteVector"), 4), ("Gets the [block height](/blockchain/block-height.md) of a transaction.", List("ID of the transaction."), "blockchain-functions", 20)), (("addressFromPublicKey", List("ByteVector"), 4), ("Converts account public key to [address](blockhain/address.md).", List("The public key to convert."), "converting-functions", 63)), (("getBooleanValue", List("Address|Alias", "String"), 4), ("Gets a boolean value by key. Throws an exception if there is no data.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 10)), (("getStringValue", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "Int"), 4), ("Extract data by index (fail on error)", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 4)), (("getString", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "Int"), 4), ("Gets a string value from a list of data entries by index", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 4)), (("sha256_64Kb", List("ByteVector"), 4), ("[SHA-256](https://en.wikipedia.org/wiki/SHA-2) hash function. For array with size <= 64Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 50)), (("groth16Verify_12inputs", List("ByteVector", "ByteVector", "ByteVector"), 4), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 12 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 2300)), (("fromBase58String", List("String"), 4), ("Decodes [Base58](https://en.wikipedia.org/wiki/Base58) string to an array of bytes.", List("The string to decode."), "encoding-and-decoding-functions", 1)), (("groth16Verify_6inputs", List("ByteVector", "ByteVector", "ByteVector"), 4), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 6 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1700)), (("toInt", List("ByteVector"), 4), ("Converts an array of bytes to an integer.", List("The array of bytes to convert."), "converting-functions", 1)), (("keccak256_128Kb", List("ByteVector"), 4), ("[Keccak-256](https://keccak.team/files/Keccak-submission-3.pdf) hash function. For array with size <= 128Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 100)), (("getBinary", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "Int"), 4), ("Gets a binary value from a list of data entries by index", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 4)), (("valueOrElse", List("T|Unit", "T"), 4), ("Returns value from union type argument if it's not unit. Otherwise, returns the second argument.", List("The argument to return value from.", "Returned if the value of t is unit."), "optional-value-functions", 2)), (("valueOrErrorMessage", List("T|Unit", "String"), 4), ("Extract value from option or fail with message", List("Optional value", "Error message"), "optional-value-functions", 2)), (("getIntegerValue", List("Address|Alias", "String"), 4), ("Gets an integer by key. Throws an exception if there is no data.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 10)), (("toBytes", List("Int"), 4), ("Converts an integer to an array of bytes.", List("The integer to convert."), "converting-functions", 1)), (("addressFromString", List("String"), 4), ("Decodes address from [Base58](https://en.wikipedia.org/wiki/Base58) string.", List("The string to decode."), "encoding-and-decoding-functions", 1)), (("transferTransactionById", List("ByteVector"), 4), ("Gets the data of a transfer transaction.", List("ID of the transfer transaction."), "blockchain-functions", 60)), (("blake2b256_32Kb", List("ByteVector"), 4), ("[BLAKE-256](https://en.wikipedia.org/wiki/BLAKE_%28hash_function%29) hash function. For array with size <= 32Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 25)), (("addressFromRecipient", List("Address|Alias"), 4), ("Extract address or lookup alias", List("Address or alias of the account."), "converting-functions", 5)), (("split", List("String", "String"), 4), ("Splits a string delimited by a separator into a list of substrings", List("The string.", "The separator."), "string-functions", 75)), (("size", List("ByteVector"), 4), ("Returns the size of an array of bytes.", List("The array of bytes."), "byte-array-functions", 1)), (("toString", List("Int"), 4), ("Converts an integer to a string.", List("The integer to convert."), "converting-functions", 1)), (("bn256Groth16Verify_10inputs", List("ByteVector", "ByteVector", "ByteVector"), 4), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 10 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1300)), (("getBooleanValue", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "Int"), 4), ("Extract data by index (fail on error)", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 4)), (("getBinaryValue", List("Address|Alias", "String"), 4), ("Gets an array of bytes by key. Throws an exception if there is no data.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 10)), (("groth16Verify_2inputs", List("ByteVector", "ByteVector", "ByteVector"), 4), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 2 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1300)), (("bn256Groth16Verify", List("ByteVector", "ByteVector", "ByteVector"), 4), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1650)), ((":+", List("List[A]", "B"), 4), ("Adding the element to the end of the list.", List("The list", "The element"), "operators", 1)), (("takeRight", List("String", "Int"), 4), ("Takes the last n characters from a string", List("The string.", "The number n."), "string-functions", 20)), (("sigVerify_32Kb", List("ByteVector", "ByteVector", "ByteVector"), 4), ("Verifies a [Curve25519](https://en.wikipedia.org/wiki/Curve25519) signature. For message size <= 32Kb.", List("The message.", "The signature.", "The account public key."), "verification-functions", 70)), (("blake2b256_64Kb", List("ByteVector"), 4), ("[BLAKE-256](https://en.wikipedia.org/wiki/BLAKE_%28hash_function%29) hash function. For array with size <= 64Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 50)), (("rsaVerify_128Kb", List("RsaDigestAlgs", "ByteVector", "ByteVector", "ByteVector"), 4), ("Verifies an [RSA](https://en.wikipedia.org/wiki/RSA_%28cryptosystem%29) signature. For message size <= 128Kb.", List("The RSA algorithm.", "The message.", "The signature.", "The public key."), "verification-functions", 750)), (("dropRight", List("String", "Int"), 4), ("Drops the last n characters of a string", List("The string.", "The number n."), "string-functions", 20)), (("toBase58String", List("ByteVector"), 4), ("Encodes array of bytes to [Base58](https://en.wikipedia.org/wiki/Base58) string.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 3)), (("groth16Verify_3inputs", List("ByteVector", "ByteVector", "ByteVector"), 4), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 3 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1400)), (("take", List("ByteVector", "Int"), 4), ("Takes the first n bytes from an array of bytes.", List("The array of bytes.", "The number n."), "byte-array-functions", 6)), (("bn256Groth16Verify_1inputs", List("ByteVector", "ByteVector", "ByteVector"), 4), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 1 input.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 800)), (("calculateAssetId", List("Issue"), 4), ("Calculates ID of asset obtained by invoke script transaction's call of the Issue structure.", List("Structure of a token issue."), "blockchain-functions", 10)), (("min", List("List[Int]"), 4), ("Returns the minimum of the list.", List("The list of integers."), "list-functions", 3)), (("!=", List("T", "T"), 4), ("Check inequality.", List("First value", "Second value"), "operators", 1)), (("getString", List("Address|Alias", "String"), 4), ("Gets a string by key.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 10)), (("assetInfo", List("ByteVector"), 4), ("Gets the information about a [token](/blockchain/token.md).", List("ID of the [token](/blockchain/token.md)."), "blockchain-functions", 15)), (("*", List("Int", "Int"), 4), ("Multiply integers.", List("Multiplier", "Multiplier"), "operators", 1)), (("bn256Groth16Verify_13inputs", List("ByteVector", "ByteVector", "ByteVector"), 4), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 13 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1450)), (("toBase64String", List("ByteVector"), 4), ("Encodes array of bytes to [Base64](https://en.wikipedia.org/wiki/Base64) string.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 35)), (("lastIndexOf", List("String", "String"), 4), ("Returns the index of the last occurrence of a substring", List("The string.", "The substring."), "string-functions", 3)), (("indexOf", List("List[T]", "T"), 4), ("Returns the index of the first occurrence of given value", List("The list.", "The element."), "list-functions", 5)), (("toString", List("Address"), 4), ("Convert address bytes to string", List("The address to convert"), "converting-functions", 10)), (("toInt", List("ByteVector", "Int"), 4), ("Converts an array of bytes to an integer starting from a certain index.", List("The array of bytes to convert.", "The index to start from."), "converting-functions", 1)), (("indexOf", List("String", "String", "Int"), 4), ("Returns the index of the first occurrence of a substring after a certain index", List("The string.", "The substring.", "The index."), "string-functions", 3)), (("toBytes", List("String"), 4), ("Converts a string to an array of bytes.", List("The string to convert."), "converting-functions", 8)), (("lastIndexOf", List("List[T]", "T"), 4), ("Returns the index of the last occurrence of given value", List("The list.", "The element."), "list-functions", 5)), (("parseInt", List("String"), 4), ("Converts the string representation of a number to its integer equivalent.", List("The string to parse."), "converting-functions", 2)), (("pow", List("Int", "Int", "Int", "Int", "Int", "Ceiling|Down|Floor|HalfDown|HalfEven|HalfUp|Up"), 4), ("Returns a number raised to a power", List("The base.", "The number of decimals of the base.", "The exponent.", "The number of decimals of the exponent.", "The number of decimals of the resulting value.", "One of the rounding functions."), "math-functions", 100)))
lazy val funcsV5 = Map((("getBinary", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "Int"), 5), ("Gets a binary value from a list of data entries by index", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 4)), (("sigVerify", List("ByteVector", "ByteVector", "ByteVector"), 5), ("Verifies a [Curve25519](https://en.wikipedia.org/wiki/Curve25519) signature.", List("The message.", "The signature.", "The account public key."), "verification-functions", 200)), (("reentrantInvoke", List("Address|Alias", "String|Unit", "List[Any]", "List[AttachedPayment]"), 5), ("Invoke function from other dApp allowing reentrancy to the current one.", List("dApp", "function name", "arguments", "Attached payments"), "blockchain-functions", 75)), (("getElement", List("List[T]", "Int"), 5), ("Gets an element from a list by index.", List("The list.", "The index of the element."), "list-functions", 2)), (("++", List("List[A]", "List[B]"), 5), ("List Concatenation.", List("First list", "Second list"), "operators", 4)), (("size", List("ByteVector"), 5), ("Returns the size of an array of bytes.", List("The array of bytes."), "byte-array-functions", 1)), (("calculateLeaseId", List("Lease"), 5), ("Calculates ID of lease obtained by invoke script transaction's call of the Lease structure.", List("Structure of a lease action."), "blockchain-functions", 1)), (("getBoolean", List("String"), 5), ("Gets a boolean value by key from state of current account.", List("The key."), "account-self-data-storage-functions", 10)), (("takeRight", List("String", "Int"), 5), ("Takes the last n characters from a string", List("The string.", "The number n."), "string-functions", 20)), (("bn256Groth16Verify_14inputs", List("ByteVector", "ByteVector", "ByteVector"), 5), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 14 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1550)), (("median", List("List[Int]"), 5), ("Returns the median of the list.", List("The list of integers."), "list-functions", 20)), (("toBase16String", List("ByteVector"), 5), ("Encodes array of bytes to [Base16](https://en.wikipedia.org/wiki/Hexadecimal) string.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 10)), (("getBinary", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "String"), 5), ("Gets a binary value from a list of data entries by key", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), (("keccak256", List("ByteVector"), 5), ("[Keccak-256](https://keccak.team/files/Keccak-submission-3.pdf) hash function.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 200)), (("parseBigInt", List("String"), 5), ("Converts an string to an integer.", List("The string to convert."), "converting-functions", 65)), (("createMerkleRoot", List("List[ByteVector]", "ByteVector", "Int"), 5), ("Calculates the Merkle root hash for transactions of block.", List("Array of sibling hashes of the Merkle tree.", "Hash of transaction.", "Index of the transaction in the block."), "verification-functions", 30)), (("blake2b256_64Kb", List("ByteVector"), 5), ("[BLAKE-256](https://en.wikipedia.org/wiki/BLAKE_%28hash_function%29) hash function. For array with size <= 64Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 50)), (("min", List("List[Int]"), 5), ("Returns the minimum of the list.", List("The list of integers."), "list-functions", 3)), (("rsaVerify_128Kb", List("RsaDigestAlgs", "ByteVector", "ByteVector", "ByteVector"), 5), ("Verifies an [RSA](https://en.wikipedia.org/wiki/RSA_%28cryptosystem%29) signature. For message size <= 128Kb.", List("The RSA algorithm.", "The message.", "The signature.", "The public key."), "verification-functions", 750)), (("Issue", List("String", "String", "Int", "Int", "Boolean"), 5), ("Issue action simplified constructor", List("Token name", "Token description", "Amount of the token", "Number of digits in decimal part", "Reissue ability flag"), "internal-functions", 1)), (("Lease", List("Address|Alias", "Int"), 5), ("Lease action simplified constructor", List("Recipient", "Amount of Waves"), "internal-functions", 1)), (("rsaVerify_16Kb", List("RsaDigestAlgs", "ByteVector", "ByteVector", "ByteVector"), 5), ("Verifies an [RSA](https://en.wikipedia.org/wiki/RSA_%28cryptosystem%29) signature. For message size <= 16Kb.", List("The RSA algorithm.", "The message.", "The signature.", "The public key."), "verification-functions", 500)), (("sha256_32Kb", List("ByteVector"), 5), ("[SHA-256](https://en.wikipedia.org/wiki/SHA-2) hash function. For array with size <= 32Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 25)), (("take", List("String", "Int"), 5), ("Takes the first n characters from a string", List("The string.", "The number n."), "string-functions", 20)), (("getInteger", List("Address|Alias", "String"), 5), ("Gets an integer by key.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 10)), (("invoke", List("Address|Alias", "String|Unit", "List[Any]", "List[AttachedPayment]"), 5), ("Invoke function from other dApp.", List("dApp", "function name", "arguments", "Attached payments"), "blockchain-functions", 75)), (("groth16Verify_12inputs", List("ByteVector", "ByteVector", "ByteVector"), 5), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 12 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 2300)), (("pow", List("BigInt", "Int", "BigInt", "Int", "Int", "Ceiling|Down|Floor|HalfEven|HalfUp"), 5), ("Returns a number raised to a power", List("The base.", "The number of decimals of the base.", "The exponent.", "The number of decimals of the exponent.", "The number of decimals of the resulting value.", "One of the rounding functions."), "math-functions", 200)), (("/", List("Int", "Int"), 5), ("Divide integers.", List("Divisible", "Divisor"), "operators", 1)), (("getBinaryValue", List("Address|Alias", "String"), 5), ("Gets an array of bytes by key. Throws an exception if there is no data.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 10)), (("indexOf", List("String", "String", "Int"), 5), ("Returns the index of the first occurrence of a substring after a certain index", List("The string.", "The substring.", "The index."), "string-functions", 3)), (("scriptHash", List("Address|Alias"), 5), ("blake2b hash of account's script", List("account"), "blockchain-functions", 200)), (("!", List("Boolean"), 5), ("Unary negation.", List("Value"), "operators", 1)), (("bn256Groth16Verify_6inputs", List("ByteVector", "ByteVector", "ByteVector"), 5), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 6 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1100)), (("sigVerify_64Kb", List("ByteVector", "ByteVector", "ByteVector"), 5), ("Verifies a [Curve25519](https://en.wikipedia.org/wiki/Curve25519) signature. For message size <= 64Kb.", List("The message.", "The signature.", "The account public key."), "verification-functions", 102)), (("rsaVerify", List("RsaDigestAlgs", "ByteVector", "ByteVector", "ByteVector"), 5), ("Verifies an [RSA](https://en.wikipedia.org/wiki/RSA_%28cryptosystem%29) signature.", List("The RSA algorithm.", "The message.", "The signature.", "The public key."), "verification-functions", 1000)), (("drop", List("ByteVector", "Int"), 5), ("Drops the first n bytes of an array of bytes.", List("The array of bytes.", "The number n."), "byte-array-functions", 6)), (("blake2b256_32Kb", List("ByteVector"), 5), ("[BLAKE-256](https://en.wikipedia.org/wiki/BLAKE_%28hash_function%29) hash function. For array with size <= 32Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 25)), (("wavesBalance", List("Address|Alias"), 5), ("Gets account waves balance details.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account."), "account-data-storage-functions", 10)), (("toInt", List("ByteVector"), 5), ("Converts an array of bytes to an integer.", List("The array of bytes to convert."), "converting-functions", 1)), (("size", List("List[T]"), 5), ("Returns the size of a list.", List("The list."), "list-functions", 2)), (("Lease", List("Address|Alias", "Int", "Int"), 5), ("Lease action detailed constructor", List("Recipient", "Amount of Waves", "Nonce to distinguish"), "internal-functions", 1)), (("sigVerify_8Kb", List("ByteVector", "ByteVector", "ByteVector"), 5), ("Verifies a [Curve25519](https://en.wikipedia.org/wiki/Curve25519) signature. For message size <= 16Kb.", List("The message.", "The signature.", "The account public key."), "verification-functions", 47)), (("assetInfo", List("ByteVector"), 5), ("Gets the information about a [token](/blockchain/token.md).", List("ID of the [token](/blockchain/token.md)."), "blockchain-functions", 15)), (("calculateAssetId", List("Issue"), 5), ("Calculates ID of asset obtained by invoke script transaction's call of the Issue structure.", List("Structure of a token issue."), "blockchain-functions", 10)), (("getBooleanValue", List("Address|Alias", "String"), 5), ("Gets a boolean value by key. Throws an exception if there is no data.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 10)), (("assetBalance", List("Address|Alias", "ByteVector"), 5), ("Gets account balance by token ID.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "Token ID."), "account-data-storage-functions", 10)), ((">=", List("BigInt", "BigInt"), 5), ("Check if integer greater or equal comparison.", List("First value", "Second value"), "operators", 8)), (("pow", List("Int", "Int", "Int", "Int", "Int", "Ceiling|Down|Floor|HalfEven|HalfUp"), 5), ("Returns a number raised to a power", List("The base.", "The number of decimals of the base.", "The exponent.", "The number of decimals of the exponent.", "The number of decimals of the resulting value.", "One of the rounding functions."), "math-functions", 100)), (("groth16Verify", List("ByteVector", "ByteVector", "ByteVector"), 5), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 2700)), (("%", List("BigInt", "BigInt"), 5), ("Calculate big modulo.", List("Divisible", "Divisor"), "operators", 64)), (("min", List("List[BigInt]"), 5), ("Returns the minimum of the list.", List("The list of big integers."), "list-functions", 192)), (("sha256_128Kb", List("ByteVector"), 5), ("[SHA-256](https://en.wikipedia.org/wiki/SHA-2) hash function. For array with size <= 128Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 100)), (("median", List("List[BigInt]"), 5), ("Returns the median of the list.", List("The list of BigInt."), "list-functions", 160)), (("groth16Verify_3inputs", List("ByteVector", "ByteVector", "ByteVector"), 5), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 3 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1400)), (("fraction", List("BigInt", "BigInt", "BigInt", "Ceiling|Down|Floor|HalfEven|HalfUp"), 5), ("Multiply and division with unlimited intermediate representation", List("Multiplier", "Multiplier", "Divisor", "Rounding mode"), "math-functions", 128)), (("value", List("T|Unit"), 5), ("Extract value from option or fail", List("Optional value"), "optional-value-functions", 2)), (("bn256Groth16Verify_13inputs", List("ByteVector", "ByteVector", "ByteVector"), 5), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 13 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1450)), (("groth16Verify_13inputs", List("ByteVector", "ByteVector", "ByteVector"), 5), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 13 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 2400)), (("contains", List("String", "String"), 5), ("Checks whether the string contains substring", List("String to search in.", "String to search for."), "string-functions", 3)), (("indexOf", List("List[T]", "T"), 5), ("Returns the index of the first occurrence of given value", List("The list.", "The element."), "list-functions", 5)), (("==", List("T", "T"), 5), ("Check equality.", List("First value", "Second value"), "operators", 1)), (("getString", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "String"), 5), ("Gets a string value from a list of data entries by key", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), (("bn256Groth16Verify_7inputs", List("ByteVector", "ByteVector", "ByteVector"), 5), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 7 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1150)), (("transactionHeightById", List("ByteVector"), 5), ("Gets the [block height](/blockchain/block-height.md) of a transaction.", List("ID of the transaction."), "blockchain-functions", 20)), (("toBase58String", List("ByteVector"), 5), ("Encodes array of bytes to [Base58](https://en.wikipedia.org/wiki/Base58) string.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 3)), (("groth16Verify_2inputs", List("ByteVector", "ByteVector", "ByteVector"), 5), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 2 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1300)), (("bn256Groth16Verify_15inputs", List("ByteVector", "ByteVector", "ByteVector"), 5), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 15 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1600)), (("blockInfoByHeight", List("Int"), 5), ("Gets the information about a [block](/blockchain/block.md) by the [block height](/blockchain/block-height.md)", List("Block height."), "blockchain-functions", 5)), (("getBooleanValue", List("String"), 5), ("Gets a boolean value by key from state of current account. Throws an exception if there is no data.", List("The key."), "account-self-data-storage-functions", 10)), (("addressFromString", List("String"), 5), ("Decodes address from [Base58](https://en.wikipedia.org/wiki/Base58) string.", List("The string to decode."), "encoding-and-decoding-functions", 1)), (("max", List("List[Int]"), 5), ("Returns the maximum of the list.", List("The list of integers."), "list-functions", 3)), (("getIntegerValue", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "Int"), 5), ("Extract data by index (fail on error)", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 4)), (("sigVerify_128Kb", List("ByteVector", "ByteVector", "ByteVector"), 5), ("Verifies a [Curve25519](https://en.wikipedia.org/wiki/Curve25519) signature. For message size <= 128Kb.", List("The message.", "The signature.", "The account public key."), "verification-functions", 172)), (("sha256", List("ByteVector"), 5), ("[SHA-256](https://en.wikipedia.org/wiki/SHA-2) hash function.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 200)), (("+", List("ByteVector", "ByteVector"), 5), ("Concat limited byte vectors.", List("First value", "Second value"), "operators", 2)), (("addressFromStringValue", List("String"), 5), ("Decodes address from [Base58](https://en.wikipedia.org/wiki/Base58) string.\nRaises an exception if the address cannot be decoded.", List("The string to decode."), "encoding-and-decoding-functions", 1)), (("bn256Groth16Verify_9inputs", List("ByteVector", "ByteVector", "ByteVector"), 5), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 9 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1250)), ((">=", List("Int", "Int"), 5), ("Check if integer greater or equal comparison.", List("First value", "Second value"), "operators", 1)), (("isDefined", List("T|Unit"), 5), ("Checks if a value is not `Unit`", List("Optional value"), "optional-value-functions", 1)), (("toString", List("BigInt"), 5), ("Convert BigInt to string", List("The BigInt to convert"), "converting-functions", 65)), (("rsaVerify_64Kb", List("RsaDigestAlgs", "ByteVector", "ByteVector", "ByteVector"), 5), ("Verifies an [RSA](https://en.wikipedia.org/wiki/RSA_%28cryptosystem%29) signature. For message size <= 64Kb.", List("The RSA algorithm.", "The message.", "The signature.", "The public key."), "verification-functions", 625)), ((">", List("BigInt", "BigInt"), 5), ("Check if integer greater comparison.", List("First value", "Second value"), "operators", 8)), (("toBigInt", List("ByteVector"), 5), ("Converts an array of bytes to an big integer.", List("The array of bytes to convert."), "converting-functions", 65)), (("toInt", List("BigInt"), 5), ("Converts a BigInt to an integer.", List("The BigInt to convert."), "converting-functions", 1)), (("take", List("ByteVector", "Int"), 5), ("Takes the first n bytes from an array of bytes.", List("The array of bytes.", "The number n."), "byte-array-functions", 6)), (("max", List("List[BigInt]"), 5), ("Returns the maximum of the list.", List("The list of big integers."), "list-functions", 192)), (("dropRight", List("ByteVector", "Int"), 5), ("Drops the first n bytes of an array of bytes.", List("The array of bytes.", "The number n."), "byte-array-functions", 6)), (("getBinaryValue", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "String"), 5), ("Find and extract data by key (fail on error)", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), (("size", List("String"), 5), ("Returns the size of a string", List("The string."), "string-functions", 1)), (("getString", List("Address|Alias", "String"), 5), ("Gets a string by key.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 10)), (("!=", List("T", "T"), 5), ("Check inequality.", List("First value", "Second value"), "operators", 1)), (("getInteger", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "String"), 5), ("Gets integer from a list of data entries by key", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), (("groth16Verify_7inputs", List("ByteVector", "ByteVector", "ByteVector"), 5), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 7 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1800)), (("-", List("BigInt"), 5), ("Change integer sign.", List("Value"), "operators", 8)), (("groth16Verify_8inputs", List("ByteVector", "ByteVector", "ByteVector"), 5), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 8 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1900)), (("bn256Groth16Verify_12inputs", List("ByteVector", "ByteVector", "ByteVector"), 5), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 12 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1400)), (("getIntegerValue", List("String"), 5), ("Gets an integer by key from state of current account. Throws an exception if there is no data.", List("The key."), "account-self-data-storage-functions", 10)), (("sha256_16Kb", List("ByteVector"), 5), ("[SHA-256](https://en.wikipedia.org/wiki/SHA-2) hash function. For array with size <= 16Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 10)), (("groth16Verify_15inputs", List("ByteVector", "ByteVector", "ByteVector"), 5), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 15 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 2600)), (("indexOf", List("String", "String"), 5), ("Returns the index of the first occurrence of a substring", List("The string.", "The substring."), "string-functions", 3)), (("toUtf8String", List("ByteVector"), 5), ("Converts an array of bytes to a UTF-8 string.", List("The array of bytes to convert."), "converting-functions", 7)), (("bn256Groth16Verify_4inputs", List("ByteVector", "ByteVector", "ByteVector"), 5), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 4 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1000)), (("%", List("Int", "Int"), 5), ("Calculate modulo.", List("Divisible", "Divisor"), "operators", 1)), (("toString", List("Boolean"), 5), ("Converts a boolean to a string.", List("The boolean to convert."), "converting-functions", 1)), (("keccak256_16Kb", List("ByteVector"), 5), ("[Keccak-256](https://keccak.team/files/Keccak-submission-3.pdf) hash function. For array with size <= 16Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 10)), (("ecrecover", List("ByteVector", "ByteVector"), 5), ("Recovers the public key from hash of ECDSA-encoded message and signature.", List("The hash of encoded message.", "The signature."), "encoding-and-decoding-functions", 70)), (("bn256Groth16Verify_2inputs", List("ByteVector", "ByteVector", "ByteVector"), 5), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 2 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 850)), (("toBigInt", List("ByteVector", "Int", "Int"), 5), ("Converts an array of bytes to an big integer.", List("The array of bytes to convert.", "Offset", "Size"), "converting-functions", 65)), (("bn256Groth16Verify_5inputs", List("ByteVector", "ByteVector", "ByteVector"), 5), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 5 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1050)), (("groth16Verify_14inputs", List("ByteVector", "ByteVector", "ByteVector"), 5), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 14 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 2500)), (("toBigInt", List("Int"), 5), ("Converts an integer to an integer.", List("The integer to convert."), "converting-functions", 1)), (("+", List("BigInt", "BigInt"), 5), ("Sum big integers.", List("First value", "Second value"), "operators", 8)), (("toBytes", List("BigInt"), 5), ("Converts an BigInt to an array of bytes.", List("The BigInt to convert."), "converting-functions", 65)), (("keccak256_128Kb", List("ByteVector"), 5), ("[Keccak-256](https://keccak.team/files/Keccak-submission-3.pdf) hash function. For array with size <= 128Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 100)), (("dropRight", List("String", "Int"), 5), ("Drops the last n characters of a string", List("The string.", "The number n."), "string-functions", 20)), (("blake2b256_16Kb", List("ByteVector"), 5), ("[BLAKE-256](https://en.wikipedia.org/wiki/BLAKE_%28hash_function%29) hash function. For array with size <= 16Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 10)), (("bn256Groth16Verify_11inputs", List("ByteVector", "ByteVector", "ByteVector"), 5), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 11 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1350)), (("toBase64String", List("ByteVector"), 5), ("Encodes array of bytes to [Base64](https://en.wikipedia.org/wiki/Base64) string.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 35)), (("sha256_64Kb", List("ByteVector"), 5), ("[SHA-256](https://en.wikipedia.org/wiki/SHA-2) hash function. For array with size <= 64Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 50)), ((":+", List("List[A]", "B"), 5), ("Adding the element to the end of the list.", List("The list", "The element"), "operators", 1)), (("-", List("Int"), 5), ("Change integer sign.", List("Value"), "operators", 1)), (("getBooleanValue", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "Int"), 5), ("Extract data by index (fail on error)", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 4)), (("-", List("BigInt", "BigInt"), 5), ("Subtract big integers.", List("First value", "Second value"), "operators", 8)), (("addressFromRecipient", List("Address|Alias"), 5), ("Extract address or lookup alias", List("Address or alias of the account."), "converting-functions", 5)), (("lastIndexOf", List("List[T]", "T"), 5), ("Returns the index of the last occurrence of given value", List("The list.", "The element."), "list-functions", 5)), (("/", List("BigInt", "BigInt"), 5), ("Divide big integers.", List("Divisible", "Divisor"), "operators", 64)), (("fraction", List("Int", "Int", "Int", "Ceiling|Down|Floor|HalfEven|HalfUp"), 5), ("Multiply and division with big integer intermediate representation", List("Multiplier", "Multiplier", "Divisor", "Rounding mode"), "math-functions", 17)), (("toString", List("Address"), 5), ("Convert address bytes to string", List("The address to convert"), "converting-functions", 10)), (("keccak256_32Kb", List("ByteVector"), 5), ("[Keccak-256](https://keccak.team/files/Keccak-submission-3.pdf) hash function. For array with size <= 32Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 25)), (("groth16Verify_11inputs", List("ByteVector", "ByteVector", "ByteVector"), 5), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 11 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 2200)), (("+", List("String", "String"), 5), ("Concat limited strings.", List("First value", "Second value"), "operators", 20)), (("parseIntValue", List("String"), 5), ("Converts the string representation of a number to its integer equivalent.\nRaises an exception if the string cannot be parsed.", List("The string to parse."), "converting-functions", 2)), (("transferTransactionById", List("ByteVector"), 5), ("Gets the data of a transfer transaction.", List("ID of the transfer transaction."), "blockchain-functions", 60)), (("fromBase58String", List("String"), 5), ("Decodes [Base58](https://en.wikipedia.org/wiki/Base58) string to an array of bytes.", List("The string to decode."), "encoding-and-decoding-functions", 1)), (("getStringValue", List("Address|Alias", "String"), 5), ("Gets a string by key. Throws an exception if there is no data.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 10)), (("toBytes", List("Int"), 5), ("Converts an integer to an array of bytes.", List("The integer to convert."), "converting-functions", 1)), (("getBinaryValue", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "Int"), 5), ("Extract data by index (fail on error)", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 4)), (("getString", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "Int"), 5), ("Gets a string value from a list of data entries by index", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 4)), (("keccak256_64Kb", List("ByteVector"), 5), ("[Keccak-256](https://keccak.team/files/Keccak-submission-3.pdf) hash function. For array with size <= 64Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 50)), (("getBooleanValue", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "String"), 5), ("Find and extract data by key (fail on error)", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), (("*", List("Int", "Int"), 5), ("Multiply integers.", List("Multiplier", "Multiplier"), "operators", 1)), (("addressFromPublicKey", List("ByteVector"), 5), ("Converts account public key to [address](blockhain/address.md).", List("The public key to convert."), "converting-functions", 63)), (("groth16Verify_9inputs", List("ByteVector", "ByteVector", "ByteVector"), 5), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 9 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 2000)), (("+", List("Int", "Int"), 5), ("Sum integers.", List("First value", "Second value"), "operators", 1)), (("bn256Groth16Verify_10inputs", List("ByteVector", "ByteVector", "ByteVector"), 5), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 10 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1300)), (("getBinary", List("String"), 5), ("Gets an array of bytes by key from state of current account.", List("The key."), "account-self-data-storage-functions", 10)), (("groth16Verify_1inputs", List("ByteVector", "ByteVector", "ByteVector"), 5), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 1 input.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1200)), (("parseBigIntValue", List("String"), 5), ("Converts an string to an integer.", List("The string to convert."), "converting-functions", 65)), (("groth16Verify_4inputs", List("ByteVector", "ByteVector", "ByteVector"), 5), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 4 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1500)), (("throw", List(), 5), ("Raises an exception.", List(), "exception-functions", 1)), (("getStringValue", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "String"), 5), ("Find and extract data by key (fail on error)", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), (("Issue", List("String", "String", "Int", "Int", "Boolean", "Script|Unit", "Int"), 5), ("Issue action detailed constructor", List("Token name", "Token description", "Amount of the token", "Number of digits in decimal part", "Reissue ability flag", "Smart asset script (optional)", "Sequential number"), "internal-functions", 1)), (("takeRight", List("ByteVector", "Int"), 5), ("Takes the last n bytes from an array of bytes.", List("The array of bytes.", "The number n."), "byte-array-functions", 6)), (("lastIndexOf", List("String", "String"), 5), ("Returns the index of the last occurrence of a substring", List("The string.", "The substring."), "string-functions", 3)), (("throw", List("String"), 5), ("Raises an exception with a message.", List("The exception message."), "exception-functions", 1)), (("cons", List("A", "List[B]"), 5), ("Prepends a new element to the list.", List("The element.", "The list."), "list-functions", 1)), (("fromBase16String", List("String"), 5), ("Decodes [Base16](https://en.wikipedia.org/wiki/Hexadecimal) string to an array of bytes.", List("The string to decode."), "encoding-and-decoding-functions", 10)), (("removeByIndex", List("List[T]", "Int"), 5), ("Removes the element at given index from the list and returns new list.", List("The list.", "The element."), "list-functions", 7)), (("rsaVerify_32Kb", List("RsaDigestAlgs", "ByteVector", "ByteVector", "ByteVector"), 5), ("Verifies an [RSA](https://en.wikipedia.org/wiki/RSA_%28cryptosystem%29) signature. For message size <= 32Kb.", List("The RSA algorithm.", "The message.", "The signature.", "The public key."), "verification-functions", 550)), (("log", List("BigInt", "Int", "BigInt", "Int", "Int", "Ceiling|Down|Floor|HalfEven|HalfUp"), 5), ("Returns the logarithm of a number.", List("The base.", "The number of decimals of the base.", "The exponent.", "The number of decimals of the exponent.", "The number of decimals of the resulting value.", "The rounding function.\n"), "math-functions", 200)), (("toInt", List("ByteVector", "Int"), 5), ("Converts an array of bytes to an integer starting from a certain index.", List("The array of bytes to convert.", "The index to start from."), "converting-functions", 1)), (("valueOrElse", List("T|Unit", "T"), 5), ("Returns value from union type argument if it's not unit. Otherwise, returns the second argument.", List("The argument to return value from.", "Returned if the value of t is unit."), "optional-value-functions", 2)), (("getStringValue", List("String"), 5), ("Gets a string by key from state of current account. Throws an exception if there is no data.", List("The key."), "account-self-data-storage-functions", 10)), (("getBinary", List("Address|Alias", "String"), 5), ("Gets an array of bytes by key.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 10)), (("groth16Verify_10inputs", List("ByteVector", "ByteVector", "ByteVector"), 5), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 10 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 2100)), (("containsElement", List("List[T]", "T"), 5), ("Tests whether the list contains a given value as an element", List("The list.", "The element."), "list-functions", 5)), (("getBoolean", List("Address|Alias", "String"), 5), ("Gets a boolean value by key.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 10)), (("sigVerify_32Kb", List("ByteVector", "ByteVector", "ByteVector"), 5), ("Verifies a [Curve25519](https://en.wikipedia.org/wiki/Curve25519) signature. For message size <= 32Kb.", List("The message.", "The signature.", "The account public key."), "verification-functions", 70)), (("isDataStorageUntouched", List("Address|Alias"), 5), ("Check that no data was stored on account.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account."), "account-data-storage-functions", 10)), (("-", List("Int", "Int"), 5), ("Subtract integers.", List("First value", "Second value"), "operators", 1)), (("getBoolean", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "String"), 5), ("Gets a boolean value from a list of data entries by key", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), (("bn256Groth16Verify_8inputs", List("ByteVector", "ByteVector", "ByteVector"), 5), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 8 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1200)), (("getInteger", List("String"), 5), ("Gets an integer by key from state of current account.", List("The key."), "account-self-data-storage-functions", 10)), (("split", List("String", "String"), 5), ("Splits a string delimited by a separator into a list of substrings", List("The string.", "The separator."), "string-functions", 75)), (("getIntegerValue", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "String"), 5), ("Find and extract data by key (fail on error)", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), (("bn256Groth16Verify", List("ByteVector", "ByteVector", "ByteVector"), 5), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1650)), (("valueOrErrorMessage", List("T|Unit", "String"), 5), ("Extract value from option or fail with message", List("Optional value", "Error message"), "optional-value-functions", 2)), (("lastIndexOf", List("String", "String", "Int"), 5), ("Returns the index of the last occurrence of a substring after a certain index", List("The string.", "The substring.", "The index."), "string-functions", 3)), (("sigVerify_16Kb", List("ByteVector", "ByteVector", "ByteVector"), 5), ("Verifies a [Curve25519](https://en.wikipedia.org/wiki/Curve25519) signature. For message size <= 16Kb.", List("The message.", "The signature.", "The account public key."), "verification-functions", 57)), (("getBoolean", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "Int"), 5), ("Gets a boolean value from a list of data entries by index", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 4)), (("getIntegerValue", List("Address|Alias", "String"), 5), ("Gets an integer by key. Throws an exception if there is no data.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 10)), (("drop", List("String", "Int"), 5), ("Drops the first n characters of a string", List("The string.", "The number n."), "string-functions", 20)), (("fromBase64String", List("String"), 5), ("Decodes [Base64](https://en.wikipedia.org/wiki/Base64) string to an array of bytes.", List("The string to decode."), "encoding-and-decoding-functions", 40)), (("blake2b256", List("ByteVector"), 5), ("[BLAKE-256](https://en.wikipedia.org/wiki/BLAKE_%28hash_function%29) hash function.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 200)), (("getInteger", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "Int"), 5), ("Gets integer from a list of data entries by index", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 4)), (("blake2b256_128Kb", List("ByteVector"), 5), ("[BLAKE-256](https://en.wikipedia.org/wiki/BLAKE_%28hash_function%29) hash function. For array with size <= 128Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 100)), (("bn256Groth16Verify_3inputs", List("ByteVector", "ByteVector", "ByteVector"), 5), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 3 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 950)), (("parseInt", List("String"), 5), ("Converts the string representation of a number to its integer equivalent.", List("The string to parse."), "converting-functions", 2)), (("groth16Verify_6inputs", List("ByteVector", "ByteVector", "ByteVector"), 5), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 6 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1700)), (("*", List("BigInt", "BigInt"), 5), ("Multiply big integers.", List("Multiplier", "Multiplier"), "operators", 64)), (("toString", List("Int"), 5), ("Converts an integer to a string.", List("The integer to convert."), "converting-functions", 1)), (("getBinaryValue", List("String"), 5), ("Gets an array of bytes by key from state of current account. Throws an exception if there is no data.", List("The key."), "account-self-data-storage-functions", 10)), (("fraction", List("Int", "Int", "Int"), 5), ("Multiply and division with unlimited intermediate representation", List("Multiplier", "Multiplier", "Divisor"), "math-functions", 14)), (("getStringValue", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "Int"), 5), ("Extract data by index (fail on error)", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 4)), ((">", List("Int", "Int"), 5), ("Check if integer greater comparison.", List("First value", "Second value"), "operators", 1)), (("toBytes", List("String"), 5), ("Converts a string to an array of bytes.", List("The string to convert."), "converting-functions", 8)), (("makeString", List("List[String]", "String"), 5), ("Returns all the elements of the list in a string using the separator string.", List("The list of strings.", "The separator"), "list-functions", 30)), (("getString", List("String"), 5), ("Gets a string by key from state of current account.", List("The key."), "account-self-data-storage-functions", 10)), (("log", List("Int", "Int", "Int", "Int", "Int", "Ceiling|Down|Floor|HalfEven|HalfUp"), 5), ("Returns the logarithm of a number.", List("The base.", "The number of decimals of the base.", "The exponent.", "The number of decimals of the exponent.", "The number of decimals of the resulting value.", "The rounding function.\n"), "math-functions", 100)), (("toBytes", List("Boolean"), 5), ("Converts a boolean to an array of bytes.", List("The boolean to convert."), "converting-functions", 1)), (("bn256Groth16Verify_1inputs", List("ByteVector", "ByteVector", "ByteVector"), 5), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 1 input.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 800)), (("transferTransactionFromProto", List("ByteVector"), 5), ("Deserializes transfer transaction: converts protobuf-encoded binary format to a TransferTransaction structure.", List("Transfer transaction in protobuf-encoded binary format."), "blockchain-functions", 5)), (("groth16Verify_5inputs", List("ByteVector", "ByteVector", "ByteVector"), 5), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 5 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1600)), (("fraction", List("BigInt", "BigInt", "BigInt"), 5), ("Multiply and division with unlimited intermediate representation", List("Multiplier", "Multiplier", "Divisor"), "math-functions", 128)))
lazy val funcsV6 = Map((("contains", List("String", "String"), 6), ("Checks whether the string contains substring", List("String to search in.", "String to search for."), "string-functions", 3)), (("sigVerify_128Kb", List("ByteVector", "ByteVector", "ByteVector"), 6), ("Verifies a [Curve25519](https://en.wikipedia.org/wiki/Curve25519) signature. For message size <= 128Kb.", List("The message.", "The signature.", "The account public key."), "verification-functions", 150)), ((">", List("BigInt", "BigInt"), 6), ("Check if integer greater comparison.", List("First value", "Second value"), "operators", 8)), (("getElement", List("List[T]", "Int"), 6), ("Gets an element from a list by index.", List("The list.", "The index of the element."), "list-functions", 2)), (("blake2b256_16Kb", List("ByteVector"), 6), ("[BLAKE-256](https://en.wikipedia.org/wiki/BLAKE_%28hash_function%29) hash function. For array with size <= 16Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 13)), (("Lease", List("Address|Alias", "Int"), 6), ("Lease action simplified constructor", List("Recipient", "Amount of Waves"), "internal-functions", 1)), (("!=", List("T", "T"), 6), ("Check inequality.", List("First value", "Second value"), "operators", 1)), (("getStringValue", List("Address|Alias", "String"), 6), ("Gets a string by key. Throws an exception if there is no data.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 10)), (("getIntegerValue", List("String"), 6), ("Gets an integer by key from state of current account. Throws an exception if there is no data.", List("The key."), "account-self-data-storage-functions", 10)), (("toUtf8String", List("ByteVector"), 6), ("Converts an array of bytes to a UTF-8 string.", List("The array of bytes to convert."), "converting-functions", 7)), (("bn256Groth16Verify_15inputs", List("ByteVector", "ByteVector", "ByteVector"), 6), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 15 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1600)), (("getBinaryValue", List("String"), 6), ("Gets an array of bytes by key from state of current account. Throws an exception if there is no data.", List("The key."), "account-self-data-storage-functions", 10)), (("take", List("String", "Int"), 6), ("Takes the first n characters from a string", List("The string.", "The number n."), "string-functions", 20)), (("bn256Groth16Verify_5inputs", List("ByteVector", "ByteVector", "ByteVector"), 6), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 5 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1050)), (("bn256Groth16Verify_14inputs", List("ByteVector", "ByteVector", "ByteVector"), 6), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 14 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1550)), (("%", List("Int", "Int"), 6), ("Calculate modulo.", List("Divisible", "Divisor"), "operators", 1)), (("fraction", List("BigInt", "BigInt", "BigInt", "Ceiling|Down|Floor|HalfEven|HalfUp"), 6), ("Multiply and division with unlimited intermediate representation", List("Multiplier", "Multiplier", "Divisor", "Rounding mode"), "math-functions", 1)), (("wavesBalance", List("Address|Alias"), 6), ("Gets account waves balance details.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account."), "account-data-storage-functions", 10)), (("size", List("List[T]"), 6), ("Returns the size of a list.", List("The list."), "list-functions", 2)), (("toBytes", List("Boolean"), 6), ("Converts a boolean to an array of bytes.", List("The boolean to convert."), "converting-functions", 1)), (("split_51C", List("String", "String"), 6), ("Splits a string delimited by a separator into a list of substrings. Input string size limit = 32767 bytes. Output list size limit = 1000", List("The string.", "The separator."), "string-functions", 51)), (("toBytes", List("Int"), 6), ("Converts an integer to an array of bytes.", List("The integer to convert."), "converting-functions", 1)), (("max", List("List[BigInt]"), 6), ("Returns the maximum of the list.", List("The list of big integers."), "list-functions", 192)), (("groth16Verify", List("ByteVector", "ByteVector", "ByteVector"), 6), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 2700)), (("/", List("BigInt", "BigInt"), 6), ("Divide big integers.", List("Divisible", "Divisor"), "operators", 64)), (("getBooleanValue", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "String"), 6), ("Find and extract data by key (fail on error)", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), (("isDefined", List("T|Unit"), 6), ("Checks if a value is not `Unit`", List("Optional value"), "optional-value-functions", 1)), (("groth16Verify_15inputs", List("ByteVector", "ByteVector", "ByteVector"), 6), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 15 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 2600)), (("parseInt", List("String"), 6), ("Converts the string representation of a number to its integer equivalent.", List("The string to parse."), "converting-functions", 2)), (("log", List("BigInt", "Int", "BigInt", "Int", "Int", "Ceiling|Down|Floor|HalfEven|HalfUp"), 6), ("Returns the logarithm of a number.", List("The base.", "The number of decimals of the base.", "The exponent.", "The number of decimals of the exponent.", "The number of decimals of the resulting value.", "The rounding function.\n"), "math-functions", 200)), (("toString", List("Int"), 6), ("Converts an integer to a string.", List("The integer to convert."), "converting-functions", 1)), (("blake2b256_32Kb", List("ByteVector"), 6), ("[BLAKE-256](https://en.wikipedia.org/wiki/BLAKE_%28hash_function%29) hash function. For array with size <= 32Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 29)), (("sigVerify_32Kb", List("ByteVector", "ByteVector", "ByteVector"), 6), ("Verifies a [Curve25519](https://en.wikipedia.org/wiki/Curve25519) signature. For message size <= 32Kb.", List("The message.", "The signature.", "The account public key."), "verification-functions", 64)), (("sha256", List("ByteVector"), 6), ("[SHA-256](https://en.wikipedia.org/wiki/SHA-2) hash function.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 118)), (("transferTransactionById", List("ByteVector"), 6), ("Gets the data of a transfer transaction.", List("ID of the transfer transaction."), "blockchain-functions", 60)), (("!", List("Boolean"), 6), ("Unary negation.", List("Value"), "operators", 1)), (("calculateAssetId", List("Issue"), 6), ("Calculates ID of asset obtained by invoke script transaction's call of the Issue structure.", List("Structure of a token issue."), "blockchain-functions", 10)), (("==", List("T", "T"), 6), ("Check equality.", List("First value", "Second value"), "operators", 1)), (("getInteger", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "String"), 6), ("Gets integer from a list of data entries by key", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), (("reentrantInvoke", List("Address|Alias", "String|Unit", "List[Any]", "List[AttachedPayment]"), 6), ("Invoke function from other dApp allowing reentrancy to the current one.", List("dApp", "function name", "arguments", "Attached payments"), "blockchain-functions", 75)), (("keccak256_16Kb", List("ByteVector"), 6), ("[Keccak-256](https://keccak.team/files/Keccak-submission-3.pdf) hash function. For array with size <= 16Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 20)), (("assetInfo", List("ByteVector"), 6), ("Gets the information about a [token](/blockchain/token.md).", List("ID of the [token](/blockchain/token.md)."), "blockchain-functions", 15)), (("parseBigIntValue", List("String"), 6), ("Converts an string to an integer.", List("The string to convert."), "converting-functions", 65)), (("median", List("List[Int]"), 6), ("Returns the median of the list.", List("The list of integers."), "list-functions", 20)), (("groth16Verify_13inputs", List("ByteVector", "ByteVector", "ByteVector"), 6), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 13 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 2400)), (("toInt", List("BigInt"), 6), ("Converts a BigInt to an integer.", List("The BigInt to convert."), "converting-functions", 1)), (("getBooleanValue", List("String"), 6), ("Gets a boolean value by key from state of current account. Throws an exception if there is no data.", List("The key."), "account-self-data-storage-functions", 10)), (("getBooleanValue", List("Address|Alias", "String"), 6), ("Gets a boolean value by key. Throws an exception if there is no data.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 10)), (("indexOf", List("List[T]", "T"), 6), ("Returns the index of the first occurrence of given value", List("The list.", "The element."), "list-functions", 5)), (("%", List("BigInt", "BigInt"), 6), ("Calculate big modulo.", List("Divisible", "Divisor"), "operators", 64)), (("toBytes", List("BigInt"), 6), ("Converts an BigInt to an array of bytes.", List("The BigInt to convert."), "converting-functions", 65)), (("throw", List("String"), 6), ("Raises an exception with a message.", List("The exception message."), "exception-functions", 1)), (("getString", List("Address|Alias", "String"), 6), ("Gets a string by key.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 10)), (("transferTransactionFromProto", List("ByteVector"), 6), ("Deserializes transfer transaction: converts protobuf-encoded binary format to a TransferTransaction structure.", List("Transfer transaction in protobuf-encoded binary format."), "blockchain-functions", 5)), (("getStringValue", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "String"), 6), ("Find and extract data by key (fail on error)", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), (("++", List("List[A]", "List[B]"), 6), ("List Concatenation.", List("First list", "Second list"), "operators", 4)), (("removeByIndex", List("List[T]", "Int"), 6), ("Removes the element at given index from the list and returns new list.", List("The list.", "The element."), "list-functions", 7)), (("toString", List("Boolean"), 6), ("Converts a boolean to a string.", List("The boolean to convert."), "converting-functions", 1)), (("+", List("ByteVector", "ByteVector"), 6), ("Concat limited byte vectors.", List("First value", "Second value"), "operators", 2)), (("getInteger", List("String"), 6), ("Gets an integer by key from state of current account.", List("The key."), "account-self-data-storage-functions", 10)), (("+", List("Int", "Int"), 6), ("Sum integers.", List("First value", "Second value"), "operators", 1)), (("keccak256_32Kb", List("ByteVector"), 6), ("[Keccak-256](https://keccak.team/files/Keccak-submission-3.pdf) hash function. For array with size <= 32Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 39)), (("pow", List("BigInt", "Int", "BigInt", "Int", "Int", "Ceiling|Down|Floor|HalfEven|HalfUp"), 6), ("Returns a number raised to a power", List("The base.", "The number of decimals of the base.", "The exponent.", "The number of decimals of the exponent.", "The number of decimals of the resulting value.", "One of the rounding functions."), "math-functions", 270)), (("min", List("List[Int]"), 6), ("Returns the minimum of the list.", List("The list of integers."), "list-functions", 3)), (("getBinary", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "String"), 6), ("Gets a binary value from a list of data entries by key", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), (("getBinary", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "Int"), 6), ("Gets a binary value from a list of data entries by index", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 4)), (("fraction", List("Int", "Int", "Int"), 6), ("Multiply and division with unlimited intermediate representation", List("Multiplier", "Multiplier", "Divisor"), "math-functions", 1)), (("fraction", List("BigInt", "BigInt", "BigInt"), 6), ("Multiply and division with unlimited intermediate representation", List("Multiplier", "Multiplier", "Divisor"), "math-functions", 1)), (("toBytes", List("String"), 6), ("Converts a string to an array of bytes.", List("The string to convert."), "converting-functions", 8)), (("takeRight", List("String", "Int"), 6), ("Takes the last n characters from a string", List("The string.", "The number n."), "string-functions", 20)), (("toString", List("BigInt"), 6), ("Convert BigInt to string", List("The BigInt to convert"), "converting-functions", 1)), (("getString", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "String"), 6), ("Gets a string value from a list of data entries by key", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), (("getStringValue", List("String"), 6), ("Gets a string by key from state of current account. Throws an exception if there is no data.", List("The key."), "account-self-data-storage-functions", 10)), (("toInt", List("ByteVector"), 6), ("Converts an array of bytes to an integer.", List("The array of bytes to convert."), "converting-functions", 1)), (("*", List("BigInt", "BigInt"), 6), ("Multiply big integers.", List("Multiplier", "Multiplier"), "operators", 64)), ((">", List("Int", "Int"), 6), ("Check if integer greater comparison.", List("First value", "Second value"), "operators", 1)), (("groth16Verify_7inputs", List("ByteVector", "ByteVector", "ByteVector"), 6), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 7 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1800)), (("bn256Groth16Verify_9inputs", List("ByteVector", "ByteVector", "ByteVector"), 6), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 9 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1250)), (("sigVerify_16Kb", List("ByteVector", "ByteVector", "ByteVector"), 6), ("Verifies a [Curve25519](https://en.wikipedia.org/wiki/Curve25519) signature. For message size <= 16Kb.", List("The message.", "The signature.", "The account public key."), "verification-functions", 50)), (("ecrecover", List("ByteVector", "ByteVector"), 6), ("Recovers the public key from hash of ECDSA-encoded message and signature.", List("The hash of encoded message.", "The signature."), "encoding-and-decoding-functions", 70)), (("log", List("Int", "Int", "Int", "Int", "Int", "Ceiling|Down|Floor|HalfEven|HalfUp"), 6), ("Returns the logarithm of a number.", List("The base.", "The number of decimals of the base.", "The exponent.", "The number of decimals of the exponent.", "The number of decimals of the resulting value.", "The rounding function.\n"), "math-functions", 100)), (("toBigInt", List("Int"), 6), ("Converts an integer to an integer.", List("The integer to convert."), "converting-functions", 1)), (("valueOrElse", List("T|Unit", "T"), 6), ("Returns value from union type argument if it's not unit. Otherwise, returns the second argument.", List("The argument to return value from.", "Returned if the value of t is unit."), "optional-value-functions", 2)), (("makeString_11C", List("List[String]", "String"), 6), ("Returns all the elements of the list in a string using the separator string. Input list size limit = 1000. Output string size limit = 32767 bytes", List("The list of strings.", "The separator"), "list-functions", 11)), (("fromBase58String", List("String"), 6), ("Decodes [Base58](https://en.wikipedia.org/wiki/Base58) string to an array of bytes.", List("The string to decode."), "encoding-and-decoding-functions", 1)), (("sigVerify_8Kb", List("ByteVector", "ByteVector", "ByteVector"), 6), ("Verifies a [Curve25519](https://en.wikipedia.org/wiki/Curve25519) signature. For message size <= 16Kb.", List("The message.", "The signature.", "The account public key."), "verification-functions", 43)), (("sha256_64Kb", List("ByteVector"), 6), ("[SHA-256](https://en.wikipedia.org/wiki/SHA-2) hash function. For array with size <= 64Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 47)), (("dropRight", List("ByteVector", "Int"), 6), ("Drops the first n bytes of an array of bytes.", List("The array of bytes.", "The number n."), "byte-array-functions", 6)), (("keccak256", List("ByteVector"), 6), ("[Keccak-256](https://keccak.team/files/Keccak-submission-3.pdf) hash function.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 195)), (("getBinary", List("Address|Alias", "String"), 6), ("Gets an array of bytes by key.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 10)), (("groth16Verify_3inputs", List("ByteVector", "ByteVector", "ByteVector"), 6), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 3 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1400)), (("getBoolean", List("String"), 6), ("Gets a boolean value by key from state of current account.", List("The key."), "account-self-data-storage-functions", 10)), (("size", List("String"), 6), ("Returns the size of a string", List("The string."), "string-functions", 1)), (("lastIndexOf", List("String", "String", "Int"), 6), ("Returns the index of the last occurrence of a substring after a certain index", List("The string.", "The substring.", "The index."), "string-functions", 3)), (("Issue", List("String", "String", "Int", "Int", "Boolean", "Script|Unit", "Int"), 6), ("Issue action detailed constructor", List("Token name", "Token description", "Amount of the token", "Number of digits in decimal part", "Reissue ability flag", "Smart asset script (optional)", "Sequential number"), "internal-functions", 1)), (("min", List("List[BigInt]"), 6), ("Returns the minimum of the list.", List("The list of big integers."), "list-functions", 192)), (("bn256Groth16Verify_1inputs", List("ByteVector", "ByteVector", "ByteVector"), 6), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 1 input.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 800)), (("rsaVerify", List("Md5|NoAlg|Sha1|Sha224|Sha256|Sha3224|Sha3256|Sha3384|Sha3512|Sha384|Sha512", "ByteVector", "ByteVector", "ByteVector"), 6), ("Verifies an [RSA](https://en.wikipedia.org/wiki/RSA_%28cryptosystem%29) signature.", List("The RSA algorithm.", "The message.", "The signature.", "The public key."), "verification-functions", 1000)), (("dropRight", List("String", "Int"), 6), ("Drops the last n characters of a string", List("The string.", "The number n."), "string-functions", 20)), (("getBoolean", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "Int"), 6), ("Gets a boolean value from a list of data entries by index", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 4)), (("sigVerify_64Kb", List("ByteVector", "ByteVector", "ByteVector"), 6), ("Verifies a [Curve25519](https://en.wikipedia.org/wiki/Curve25519) signature. For message size <= 64Kb.", List("The message.", "The signature.", "The account public key."), "verification-functions", 93)), (("getString", List("String"), 6), ("Gets a string by key from state of current account.", List("The key."), "account-self-data-storage-functions", 10)), (("scriptHash", List("Address|Alias"), 6), ("blake2b hash of account's script", List("account"), "blockchain-functions", 200)), (("addressFromStringValue", List("String"), 6), ("Decodes address from [Base58](https://en.wikipedia.org/wiki/Base58) string.\nRaises an exception if the address cannot be decoded.", List("The string to decode."), "encoding-and-decoding-functions", 1)), (("groth16Verify_4inputs", List("ByteVector", "ByteVector", "ByteVector"), 6), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 4 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1500)), (("sha256_16Kb", List("ByteVector"), 6), ("[SHA-256](https://en.wikipedia.org/wiki/SHA-2) hash function. For array with size <= 16Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 12)), (("bn256Groth16Verify_6inputs", List("ByteVector", "ByteVector", "ByteVector"), 6), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 6 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1100)), (("blockInfoByHeight", List("Int"), 6), ("Gets the information about a [block](/blockchain/block.md) by the [block height](/blockchain/block-height.md)", List("Block height."), "blockchain-functions", 5)), (("invoke", List("Address|Alias", "String|Unit", "List[Any]", "List[AttachedPayment]"), 6), ("Invoke function from other dApp.", List("dApp", "function name", "arguments", "Attached payments"), "blockchain-functions", 75)), (("getBinaryValue", List("Address|Alias", "String"), 6), ("Gets an array of bytes by key. Throws an exception if there is no data.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 10)), ((">=", List("BigInt", "BigInt"), 6), ("Check if integer greater or equal comparison.", List("First value", "Second value"), "operators", 8)), (("+", List("BigInt", "BigInt"), 6), ("Sum big integers.", List("First value", "Second value"), "operators", 8)), (("value", List("T|Unit"), 6), ("Extract value from option or fail", List("Optional value"), "optional-value-functions", 2)), (("toBigInt", List("ByteVector", "Int", "Int"), 6), ("Converts an array of bytes to an big integer.", List("The array of bytes to convert.", "Offset", "Size"), "converting-functions", 65)), (("fromBase64String", List("String"), 6), ("Decodes [Base64](https://en.wikipedia.org/wiki/Base64) string to an array of bytes.", List("The string to decode."), "encoding-and-decoding-functions", 40)), (("-", List("BigInt", "BigInt"), 6), ("Subtract big integers.", List("First value", "Second value"), "operators", 8)), (("Lease", List("Address|Alias", "Int", "Int"), 6), ("Lease action detailed constructor", List("Recipient", "Amount of Waves", "Nonce to distinguish"), "internal-functions", 1)), (("take", List("ByteVector", "Int"), 6), ("Takes the first n bytes from an array of bytes.", List("The array of bytes.", "The number n."), "byte-array-functions", 6)), (("bn256Groth16Verify_11inputs", List("ByteVector", "ByteVector", "ByteVector"), 6), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 11 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1350)), ((":+", List("List[A]", "B"), 6), ("Adding the element to the end of the list.", List("The list", "The element"), "operators", 1)), (("blake2b256", List("ByteVector"), 6), ("[BLAKE-256](https://en.wikipedia.org/wiki/BLAKE_%28hash_function%29) hash function.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 136)), (("-", List("Int"), 6), ("Change integer sign.", List("Value"), "operators", 1)), (("*", List("Int", "Int"), 6), ("Multiply integers.", List("Multiplier", "Multiplier"), "operators", 1)), (("getInteger", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "Int"), 6), ("Gets integer from a list of data entries by index", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 4)), (("rsaVerify_32Kb", List("Md5|NoAlg|Sha1|Sha224|Sha256|Sha3224|Sha3256|Sha3384|Sha3512|Sha384|Sha512", "ByteVector", "ByteVector", "ByteVector"), 6), ("Verifies an [RSA](https://en.wikipedia.org/wiki/RSA_%28cryptosystem%29) signature. For message size <= 32Kb.", List("The RSA algorithm.", "The message.", "The signature.", "The public key."), "verification-functions", 550)), (("sha256_128Kb", List("ByteVector"), 6), ("[SHA-256](https://en.wikipedia.org/wiki/SHA-2) hash function. For array with size <= 128Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 93)), (("bn256Groth16Verify_8inputs", List("ByteVector", "ByteVector", "ByteVector"), 6), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 8 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1200)), (("lastIndexOf", List("String", "String"), 6), ("Returns the index of the last occurrence of a substring", List("The string.", "The substring."), "string-functions", 3)), (("groth16Verify_8inputs", List("ByteVector", "ByteVector", "ByteVector"), 6), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 8 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1900)), (("keccak256_64Kb", List("ByteVector"), 6), ("[Keccak-256](https://keccak.team/files/Keccak-submission-3.pdf) hash function. For array with size <= 64Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 74)), (("groth16Verify_1inputs", List("ByteVector", "ByteVector", "ByteVector"), 6), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 1 input.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1200)), (("toBase16String", List("ByteVector"), 6), ("Encodes array of bytes to [Base16](https://en.wikipedia.org/wiki/Hexadecimal) string.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 10)), (("fromBase16String", List("String"), 6), ("Decodes [Base16](https://en.wikipedia.org/wiki/Hexadecimal) string to an array of bytes.", List("The string to decode."), "encoding-and-decoding-functions", 10)), (("blake2b256_64Kb", List("ByteVector"), 6), ("[BLAKE-256](https://en.wikipedia.org/wiki/BLAKE_%28hash_function%29) hash function. For array with size <= 64Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 58)), (("bn256Groth16Verify_10inputs", List("ByteVector", "ByteVector", "ByteVector"), 6), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 10 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1300)), (("bn256Groth16Verify_7inputs", List("ByteVector", "ByteVector", "ByteVector"), 6), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 7 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1150)), (("getBinaryValue", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "String"), 6), ("Find and extract data by key (fail on error)", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), (("isDataStorageUntouched", List("Address|Alias"), 6), ("Check that no data was stored on account.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account."), "account-data-storage-functions", 10)), (("bn256Groth16Verify_3inputs", List("ByteVector", "ByteVector", "ByteVector"), 6), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 3 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 950)), (("getBinaryValue", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "Int"), 6), ("Extract data by index (fail on error)", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 4)), (("takeRight", List("ByteVector", "Int"), 6), ("Takes the last n bytes from an array of bytes.", List("The array of bytes.", "The number n."), "byte-array-functions", 6)), (("createMerkleRoot", List("List[ByteVector]", "ByteVector", "Int"), 6), ("Calculates the Merkle root hash for transactions of block.", List("Array of sibling hashes of the Merkle tree.", "Hash of transaction.", "Index of the transaction in the block."), "verification-functions", 30)), (("toBigInt", List("ByteVector"), 6), ("Converts an array of bytes to an big integer.", List("The array of bytes to convert."), "converting-functions", 65)), (("makeString", List("List[String]", "String"), 6), ("Returns all the elements of the list in a string using the separator string. Input list size limit = 70. Output string size limit = 500 bytes", List("The list of strings.", "The separator"), "list-functions", 1)), (("-", List("Int", "Int"), 6), ("Subtract integers.", List("First value", "Second value"), "operators", 1)), (("groth16Verify_14inputs", List("ByteVector", "ByteVector", "ByteVector"), 6), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 14 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 2500)), (("getBinary", List("String"), 6), ("Gets an array of bytes by key from state of current account.", List("The key."), "account-self-data-storage-functions", 10)), ((">=", List("Int", "Int"), 6), ("Check if integer greater or equal comparison.", List("First value", "Second value"), "operators", 1)), (("bn256Groth16Verify_2inputs", List("ByteVector", "ByteVector", "ByteVector"), 6), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 2 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 850)), (("getString", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "Int"), 6), ("Gets a string value from a list of data entries by index", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 4)), (("addressFromRecipient", List("Address|Alias"), 6), ("Extract address or lookup alias", List("Address or alias of the account."), "converting-functions", 5)), (("getIntegerValue", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "String"), 6), ("Find and extract data by key (fail on error)", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), (("bn256Groth16Verify_12inputs", List("ByteVector", "ByteVector", "ByteVector"), 6), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 12 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1400)), (("indexOf", List("String", "String"), 6), ("Returns the index of the first occurrence of a substring", List("The string.", "The substring."), "string-functions", 3)), (("cons", List("A", "List[B]"), 6), ("Prepends a new element to the list.", List("The element.", "The list."), "list-functions", 1)), (("getBoolean", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "String"), 6), ("Gets a boolean value from a list of data entries by key", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), (("keccak256_128Kb", List("ByteVector"), 6), ("[Keccak-256](https://keccak.team/files/Keccak-submission-3.pdf) hash function. For array with size <= 128Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 147)), (("split_4C", List("String", "String"), 6), ("Splits a string delimited by a separator into a list of substrings. Input string size limit = 6000 bytes. Output list size limit = 100", List("The string.", "The separator."), "string-functions", 4)), (("sigVerify", List("ByteVector", "ByteVector", "ByteVector"), 6), ("Verifies a [Curve25519](https://en.wikipedia.org/wiki/Curve25519) signature.", List("The message.", "The signature.", "The account public key."), "verification-functions", 180)), (("median", List("List[BigInt]"), 6), ("Returns the median of the list.", List("The list of BigInt."), "list-functions", 160)), (("lastIndexOf", List("List[T]", "T"), 6), ("Returns the index of the last occurrence of given value", List("The list.", "The element."), "list-functions", 5)), (("indexOf", List("String", "String", "Int"), 6), ("Returns the index of the first occurrence of a substring after a certain index", List("The string.", "The substring.", "The index."), "string-functions", 3)), (("drop", List("ByteVector", "Int"), 6), ("Drops the first n bytes of an array of bytes.", List("The array of bytes.", "The number n."), "byte-array-functions", 6)), (("groth16Verify_10inputs", List("ByteVector", "ByteVector", "ByteVector"), 6), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 10 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 2100)), (("assetBalance", List("Address|Alias", "ByteVector"), 6), ("Gets account balance by token ID.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "Token ID."), "account-data-storage-functions", 10)), (("makeString_2C", List("List[String]", "String"), 6), ("Returns all the elements of the list in a string using the separator string. Input list size limit = 100. Output string size limit = 6000 bytes", List("The list of strings.", "The separator"), "list-functions", 2)), (("pow", List("Int", "Int", "Int", "Int", "Int", "Ceiling|Down|Floor|HalfEven|HalfUp"), 6), ("Returns a number raised to a power", List("The base.", "The number of decimals of the base.", "The exponent.", "The number of decimals of the exponent.", "The number of decimals of the resulting value.", "One of the rounding functions."), "math-functions", 28)), (("getIntegerValue", List("Address|Alias", "String"), 6), ("Gets an integer by key. Throws an exception if there is no data.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 10)), (("rsaVerify_16Kb", List("Md5|NoAlg|Sha1|Sha224|Sha256|Sha3224|Sha3256|Sha3384|Sha3512|Sha384|Sha512", "ByteVector", "ByteVector", "ByteVector"), 6), ("Verifies an [RSA](https://en.wikipedia.org/wiki/RSA_%28cryptosystem%29) signature. For message size <= 16Kb.", List("The RSA algorithm.", "The message.", "The signature.", "The public key."), "verification-functions", 500)), (("addressFromPublicKey", List("ByteVector"), 6), ("Converts account public key to [address](blockhain/address.md).", List("The public key to convert."), "converting-functions", 1)), (("sqrt", List("BigInt", "Int", "Int", "Ceiling|Down|Floor|HalfEven|HalfUp"), 6), ("Returns a square root.", List("The base.", "The number of decimals of the base.", "The number of decimals of the resulting value.", "One of the rounding functions."), "math-functions", 5)), (("split", List("String", "String"), 6), ("Splits a string delimited by a separator into a list of substrings. Input string size limit = 500 bytes. Output list size limit = 20", List("The string.", "The separator."), "string-functions", 1)), (("toString", List("Address"), 6), ("Convert address bytes to string", List("The address to convert"), "converting-functions", 1)), (("parseBigInt", List("String"), 6), ("Converts an string to an integer.", List("The string to convert."), "converting-functions", 65)), (("blake2b256_128Kb", List("ByteVector"), 6), ("[BLAKE-256](https://en.wikipedia.org/wiki/BLAKE_%28hash_function%29) hash function. For array with size <= 128Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 115)), (("fraction", List("Int", "Int", "Int", "Ceiling|Down|Floor|HalfEven|HalfUp"), 6), ("Multiply and division with big integer intermediate representation", List("Multiplier", "Multiplier", "Divisor", "Rounding mode"), "math-functions", 1)), (("toBase64String", List("ByteVector"), 6), ("Encodes array of bytes to [Base64](https://en.wikipedia.org/wiki/Base64) string.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 35)), (("groth16Verify_9inputs", List("ByteVector", "ByteVector", "ByteVector"), 6), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 9 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 2000)), (("getBooleanValue", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "Int"), 6), ("Extract data by index (fail on error)", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 4)), (("addressFromString", List("String"), 6), ("Decodes address from [Base58](https://en.wikipedia.org/wiki/Base58) string.", List("The string to decode."), "encoding-and-decoding-functions", 1)), (("getBoolean", List("Address|Alias", "String"), 6), ("Gets a boolean value by key.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 10)), (("groth16Verify_11inputs", List("ByteVector", "ByteVector", "ByteVector"), 6), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 11 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 2200)), (("toInt", List("ByteVector", "Int"), 6), ("Converts an array of bytes to an integer starting from a certain index.", List("The array of bytes to convert.", "The index to start from."), "converting-functions", 1)), (("-", List("BigInt"), 6), ("Change integer sign.", List("Value"), "operators", 8)), (("throw", List(), 6), ("Raises an exception.", List(), "exception-functions", 1)), (("/", List("Int", "Int"), 6), ("Divide integers.", List("Divisible", "Divisor"), "operators", 1)), (("calculateLeaseId", List("Lease"), 6), ("Calculates ID of lease obtained by invoke script transaction's call of the Lease structure.", List("Structure of a lease action."), "blockchain-functions", 1)), (("toBase58String", List("ByteVector"), 6), ("Encodes array of bytes to [Base58](https://en.wikipedia.org/wiki/Base58) string.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 3)), (("rsaVerify_128Kb", List("Md5|NoAlg|Sha1|Sha224|Sha256|Sha3224|Sha3256|Sha3384|Sha3512|Sha384|Sha512", "ByteVector", "ByteVector", "ByteVector"), 6), ("Verifies an [RSA](https://en.wikipedia.org/wiki/RSA_%28cryptosystem%29) signature. For message size <= 128Kb.", List("The RSA algorithm.", "The message.", "The signature.", "The public key."), "verification-functions", 750)), (("groth16Verify_2inputs", List("ByteVector", "ByteVector", "ByteVector"), 6), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 2 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1300)), (("getInteger", List("Address|Alias", "String"), 6), ("Gets an integer by key.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 10)), (("bn256Groth16Verify", List("ByteVector", "ByteVector", "ByteVector"), 6), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1650)), (("groth16Verify_6inputs", List("ByteVector", "ByteVector", "ByteVector"), 6), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 6 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1700)), (("sha256_32Kb", List("ByteVector"), 6), ("[SHA-256](https://en.wikipedia.org/wiki/SHA-2) hash function. For array with size <= 32Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 23)), (("bn256Groth16Verify_4inputs", List("ByteVector", "ByteVector", "ByteVector"), 6), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 4 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1000)), (("getIntegerValue", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "Int"), 6), ("Extract data by index (fail on error)", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 4)), (("bn256Groth16Verify_13inputs", List("ByteVector", "ByteVector", "ByteVector"), 6), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 13 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1450)), (("rsaVerify_64Kb", List("Md5|NoAlg|Sha1|Sha224|Sha256|Sha3224|Sha3256|Sha3384|Sha3512|Sha384|Sha512", "ByteVector", "ByteVector", "ByteVector"), 6), ("Verifies an [RSA](https://en.wikipedia.org/wiki/RSA_%28cryptosystem%29) signature. For message size <= 64Kb.", List("The RSA algorithm.", "The message.", "The signature.", "The public key."), "verification-functions", 625)), (("containsElement", List("List[T]", "T"), 6), ("Tests whether the list contains a given value as an element", List("The list.", "The element."), "list-functions", 5)), (("getStringValue", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "Int"), 6), ("Extract data by index (fail on error)", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 4)), (("sqrt", List("Int", "Int", "Int", "Ceiling|Down|Floor|HalfEven|HalfUp"), 6), ("Returns a square root.", List("The number", "The number of decimals of the number.", "The number of decimals of the resulting value.", "One of the rounding functions."), "math-functions", 2)), (("groth16Verify_5inputs", List("ByteVector", "ByteVector", "ByteVector"), 6), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 5 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1600)), (("transactionHeightById", List("ByteVector"), 6), ("Gets the [block height](/blockchain/block-height.md) of a transaction.", List("ID of the transaction."), "blockchain-functions", 20)), (("+", List("String", "String"), 6), ("Concat limited strings.", List("First value", "Second value"), "operators", 1)), (("Issue", List("String", "String", "Int", "Int", "Boolean"), 6), ("Issue action simplified constructor", List("Token name", "Token description", "Amount of the token", "Number of digits in decimal part", "Reissue ability flag"), "internal-functions", 1)), (("valueOrErrorMessage", List("T|Unit", "String"), 6), ("Extract value from option or fail with message", List("Optional value", "Error message"), "optional-value-functions", 2)), (("max", List("List[Int]"), 6), ("Returns the maximum of the list.", List("The list of integers."), "list-functions", 3)), (("drop", List("String", "Int"), 6), ("Drops the first n characters of a string", List("The string.", "The number n."), "string-functions", 20)), (("groth16Verify_12inputs", List("ByteVector", "ByteVector", "ByteVector"), 6), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 12 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 2300)), (("parseIntValue", List("String"), 6), ("Converts the string representation of a number to its integer equivalent.\nRaises an exception if the string cannot be parsed.", List("The string to parse."), "converting-functions", 2)), (("size", List("(A, B)|(A, B, C)|(A, B, C, D)|(A, B, C, D, E)|(A, B, C, D, E, F)|(A, B, C, D, E, F, G)|(A, B, C, D, E, F, G, H)|(A, B, C, D, E, F, G, H, I)|(A, B, C, D, E, F, G, H, I, J)|(A, B, C, D, E, F, G, H, I, J, K)|(A, B, C, D, E, F, G, H, I, J, K, L)|(A, B, C, D, E, F, G, H, I, J, K, L, M)|(A, B, C, D, E, F, G, H, I, J, K, L, M, N)|(A, B, C, D, E, F, G, H, I, J, K, L, M, N, O)|(A, B, C, D, E, F, G, H, I, J, K, L, M, N, O, P)|(A, B, C, D, E, F, G, H, I, J, K, L, M, N, O, P, Q)|(A, B, C, D, E, F, G, H, I, J, K, L, M, N, O, P, Q, R)|(A, B, C, D, E, F, G, H, I, J, K, L, M, N, O, P, Q, R, S)|(A, B, C, D, E, F, G, H, I, J, K, L, M, N, O, P, Q, R, S, T)|(A, B, C, D, E, F, G, H, I, J, K, L, M, N, O, P, Q, R, S, T, U)|(A, B, C, D, E, F, G, H, I, J, K, L, M, N, O, P, Q, R, S, T, U, V)"), 6), ("Number of elements of the tuple", List("The tuple"), "internal-functions", 1)), (("size", List("ByteVector"), 6), ("Returns the size of an array of bytes.", List("The array of bytes."), "byte-array-functions", 1)))
lazy val funcsV7 = Map((("bn256Groth16Verify", List("ByteVector", "ByteVector", "ByteVector"), 7), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1650)), (("throw", List("String"), 7), ("Raises an exception with a message.", List("The exception message."), "exception-functions", 1)), (("sqrt", List("BigInt", "Int", "Int", "Ceiling|Down|Floor|HalfEven|HalfUp"), 7), ("Returns a square root.", List("The base.", "The number of decimals of the base.", "The number of decimals of the resulting value.", "One of the rounding functions."), "math-functions", 5)), (("rsaVerify_32Kb", List("Md5|NoAlg|Sha1|Sha224|Sha256|Sha3224|Sha3256|Sha3384|Sha3512|Sha384|Sha512", "ByteVector", "ByteVector", "ByteVector"), 7), ("Verifies an [RSA](https://en.wikipedia.org/wiki/RSA_%28cryptosystem%29) signature. For message size <= 32Kb.", List("The RSA algorithm.", "The message.", "The signature.", "The public key."), "verification-functions", 550)), (("groth16Verify_4inputs", List("ByteVector", "ByteVector", "ByteVector"), 7), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 4 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1500)), (("sha256_16Kb", List("ByteVector"), 7), ("[SHA-256](https://en.wikipedia.org/wiki/SHA-2) hash function. For array with size <= 16Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 12)), (("getBooleanValue", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "String"), 7), ("Find and extract data by key (fail on error)", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), ((">", List("BigInt", "BigInt"), 7), ("Check if integer greater comparison.", List("First value", "Second value"), "operators", 8)), (("rsaVerify", List("Md5|NoAlg|Sha1|Sha224|Sha256|Sha3224|Sha3256|Sha3384|Sha3512|Sha384|Sha512", "ByteVector", "ByteVector", "ByteVector"), 7), ("Verifies an [RSA](https://en.wikipedia.org/wiki/RSA_%28cryptosystem%29) signature.", List("The RSA algorithm.", "The message.", "The signature.", "The public key."), "verification-functions", 1000)), (("!", List("Boolean"), 7), ("Unary negation.", List("Value"), "operators", 1)), (("getBooleanValue", List("Address|Alias", "String"), 7), ("Gets a boolean value by key. Throws an exception if there is no data.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 10)), (("groth16Verify_12inputs", List("ByteVector", "ByteVector", "ByteVector"), 7), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 12 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 2300)), (("rsaVerify_128Kb", List("Md5|NoAlg|Sha1|Sha224|Sha256|Sha3224|Sha3256|Sha3384|Sha3512|Sha384|Sha512", "ByteVector", "ByteVector", "ByteVector"), 7), ("Verifies an [RSA](https://en.wikipedia.org/wiki/RSA_%28cryptosystem%29) signature. For message size <= 128Kb.", List("The RSA algorithm.", "The message.", "The signature.", "The public key."), "verification-functions", 750)), (("+", List("String", "String"), 7), ("Concat limited strings.", List("First value", "Second value"), "operators", 1)), (("makeString_11C", List("List[String]", "String"), 7), ("Returns all the elements of the list in a string using the separator string. Input list size limit = 1000. Output string size limit = 32767 bytes", List("The list of strings.", "The separator"), "list-functions", 11)), ((">=", List("BigInt", "BigInt"), 7), ("Check if integer greater or equal comparison.", List("First value", "Second value"), "operators", 8)), (("assetInfo", List("ByteVector"), 7), ("Gets the information about a [token](/blockchain/token.md).", List("ID of the [token](/blockchain/token.md)."), "blockchain-functions", 15)), (("toString", List("Boolean"), 7), ("Converts a boolean to a string.", List("The boolean to convert."), "converting-functions", 1)), (("*", List("Int", "Int"), 7), ("Multiply integers.", List("Multiplier", "Multiplier"), "operators", 1)), (("wavesBalance", List("Address|Alias"), 7), ("Gets account waves balance details.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account."), "account-data-storage-functions", 10)), (("bn256Groth16Verify_5inputs", List("ByteVector", "ByteVector", "ByteVector"), 7), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 5 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1050)), (("rsaVerify_16Kb", List("Md5|NoAlg|Sha1|Sha224|Sha256|Sha3224|Sha3256|Sha3384|Sha3512|Sha384|Sha512", "ByteVector", "ByteVector", "ByteVector"), 7), ("Verifies an [RSA](https://en.wikipedia.org/wiki/RSA_%28cryptosystem%29) signature. For message size <= 16Kb.", List("The RSA algorithm.", "The message.", "The signature.", "The public key."), "verification-functions", 500)), (("groth16Verify_14inputs", List("ByteVector", "ByteVector", "ByteVector"), 7), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 14 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 2500)), (("getIntegerValue", List("String"), 7), ("Gets an integer by key from state of current account. Throws an exception if there is no data.", List("The key."), "account-self-data-storage-functions", 10)), (("sigVerify_128Kb", List("ByteVector", "ByteVector", "ByteVector"), 7), ("Verifies a [Curve25519](https://en.wikipedia.org/wiki/Curve25519) signature. For message size <= 128Kb.", List("The message.", "The signature.", "The account public key."), "verification-functions", 150)), (("blake2b256_32Kb", List("ByteVector"), 7), ("[BLAKE-256](https://en.wikipedia.org/wiki/BLAKE_%28hash_function%29) hash function. For array with size <= 32Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 29)), (("sigVerify_8Kb", List("ByteVector", "ByteVector", "ByteVector"), 7), ("Verifies a [Curve25519](https://en.wikipedia.org/wiki/Curve25519) signature. For message size <= 16Kb.", List("The message.", "The signature.", "The account public key."), "verification-functions", 43)), (("takeRight", List("ByteVector", "Int"), 7), ("Takes the last n bytes from an array of bytes.", List("The array of bytes.", "The number n."), "byte-array-functions", 6)), (("bn256Groth16Verify_2inputs", List("ByteVector", "ByteVector", "ByteVector"), 7), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 2 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 850)), (("-", List("Int"), 7), ("Change integer sign.", List("Value"), "operators", 1)), (("calculateAssetId", List("Issue"), 7), ("Calculates ID of asset obtained by invoke script transaction's call of the Issue structure.", List("Structure of a token issue."), "blockchain-functions", 10)), (("size", List("ByteVector"), 7), ("Returns the size of an array of bytes.", List("The array of bytes."), "byte-array-functions", 1)), (("groth16Verify_8inputs", List("ByteVector", "ByteVector", "ByteVector"), 7), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 8 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1900)), (("containsElement", List("List[T]", "T"), 7), ("Tests whether the list contains a given value as an element", List("The list.", "The element."), "list-functions", 5)), (("getBinaryValue", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "String"), 7), ("Find and extract data by key (fail on error)", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), (("lastIndexOf", List("String", "String"), 7), ("Returns the index of the last occurrence of a substring", List("The string.", "The substring."), "string-functions", 3)), (("toBigInt", List("Int"), 7), ("Converts an integer to an integer.", List("The integer to convert."), "converting-functions", 1)), (("bn256Groth16Verify_1inputs", List("ByteVector", "ByteVector", "ByteVector"), 7), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 1 input.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 800)), (("groth16Verify_7inputs", List("ByteVector", "ByteVector", "ByteVector"), 7), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 7 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1800)), (("bn256Groth16Verify_12inputs", List("ByteVector", "ByteVector", "ByteVector"), 7), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 12 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1400)), (("throw", List(), 7), ("Raises an exception.", List(), "exception-functions", 1)), (("fromBase58String", List("String"), 7), ("Decodes [Base58](https://en.wikipedia.org/wiki/Base58) string to an array of bytes.", List("The string to decode."), "encoding-and-decoding-functions", 1)), (("isDataStorageUntouched", List("Address|Alias"), 7), ("Check that no data was stored on account.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account."), "account-data-storage-functions", 10)), (("addressFromString", List("String"), 7), ("Decodes address from [Base58](https://en.wikipedia.org/wiki/Base58) string.", List("The string to decode."), "encoding-and-decoding-functions", 1)), (("toBase58String", List("ByteVector"), 7), ("Encodes array of bytes to [Base58](https://en.wikipedia.org/wiki/Base58) string.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 3)), (("sqrt", List("Int", "Int", "Int", "Ceiling|Down|Floor|HalfEven|HalfUp"), 7), ("Returns a square root.", List("The number", "The number of decimals of the number.", "The number of decimals of the resulting value.", "One of the rounding functions."), "math-functions", 2)), (("getBinaryValue", List("String"), 7), ("Gets an array of bytes by key from state of current account. Throws an exception if there is no data.", List("The key."), "account-self-data-storage-functions", 10)), (("bn256Groth16Verify_13inputs", List("ByteVector", "ByteVector", "ByteVector"), 7), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 13 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1450)), (("contains", List("String", "String"), 7), ("Checks whether the string contains substring", List("String to search in.", "String to search for."), "string-functions", 3)), (("toString", List("BigInt"), 7), ("Convert BigInt to string", List("The BigInt to convert"), "converting-functions", 1)), (("sigVerify_16Kb", List("ByteVector", "ByteVector", "ByteVector"), 7), ("Verifies a [Curve25519](https://en.wikipedia.org/wiki/Curve25519) signature. For message size <= 16Kb.", List("The message.", "The signature.", "The account public key."), "verification-functions", 50)), (("fraction", List("Int", "Int", "Int", "Ceiling|Down|Floor|HalfEven|HalfUp"), 7), ("Multiply and division with big integer intermediate representation", List("Multiplier", "Multiplier", "Divisor", "Rounding mode"), "math-functions", 1)), (("bn256Groth16Verify_8inputs", List("ByteVector", "ByteVector", "ByteVector"), 7), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 8 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1200)), (("getElement", List("List[T]", "Int"), 7), ("Gets an element from a list by index.", List("The list.", "The index of the element."), "list-functions", 2)), (("indexOf", List("String", "String"), 7), ("Returns the index of the first occurrence of a substring", List("The string.", "The substring."), "string-functions", 3)), (("Issue", List("String", "String", "Int", "Int", "Boolean"), 7), ("Issue action simplified constructor", List("Token name", "Token description", "Amount of the token", "Number of digits in decimal part", "Reissue ability flag"), "internal-functions", 1)), (("getBoolean", List("String"), 7), ("Gets a boolean value by key from state of current account.", List("The key."), "account-self-data-storage-functions", 10)), (("reentrantInvoke", List("Address|Alias", "String|Unit", "List[Any]", "List[AttachedPayment]"), 7), ("Invoke function from other dApp allowing reentrancy to the current one.", List("dApp", "function name", "arguments", "Attached payments"), "blockchain-functions", 75)), (("groth16Verify_1inputs", List("ByteVector", "ByteVector", "ByteVector"), 7), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 1 input.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1200)), (("bn256Groth16Verify_6inputs", List("ByteVector", "ByteVector", "ByteVector"), 7), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 6 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1100)), (("getBoolean", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "String"), 7), ("Gets a boolean value from a list of data entries by key", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), (("/", List("BigInt", "BigInt"), 7), ("Divide big integers.", List("Divisible", "Divisor"), "operators", 64)), (("getIntegerValue", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "Int"), 7), ("Extract data by index (fail on error)", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 4)), (("getIntegerValue", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "String"), 7), ("Find and extract data by key (fail on error)", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), ((":+", List("List[A]", "B"), 7), ("Adding the element to the end of the list.", List("The list", "The element"), "operators", 1)), (("groth16Verify_13inputs", List("ByteVector", "ByteVector", "ByteVector"), 7), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 13 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 2400)), (("getBinary", List("Address|Alias", "String"), 7), ("Gets an array of bytes by key.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 10)), (("groth16Verify", List("ByteVector", "ByteVector", "ByteVector"), 7), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 2700)), (("bn256Groth16Verify_14inputs", List("ByteVector", "ByteVector", "ByteVector"), 7), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 14 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1550)), (("toBytes", List("Boolean"), 7), ("Converts a boolean to an array of bytes.", List("The boolean to convert."), "converting-functions", 1)), (("bn256Groth16Verify_9inputs", List("ByteVector", "ByteVector", "ByteVector"), 7), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 9 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1250)), (("toInt", List("ByteVector", "Int"), 7), ("Converts an array of bytes to an integer starting from a certain index.", List("The array of bytes to convert.", "The index to start from."), "converting-functions", 1)), (("indexOf", List("List[T]", "T"), 7), ("Returns the index of the first occurrence of given value", List("The list.", "The element."), "list-functions", 5)), (("drop", List("ByteVector", "Int"), 7), ("Drops the first n bytes of an array of bytes.", List("The array of bytes.", "The number n."), "byte-array-functions", 6)), (("fraction", List("Int", "Int", "Int"), 7), ("Multiply and division with unlimited intermediate representation", List("Multiplier", "Multiplier", "Divisor"), "math-functions", 1)), (("-", List("BigInt", "BigInt"), 7), ("Subtract big integers.", List("First value", "Second value"), "operators", 8)), (("bn256Groth16Verify_3inputs", List("ByteVector", "ByteVector", "ByteVector"), 7), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 3 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 950)), (("blake2b256_128Kb", List("ByteVector"), 7), ("[BLAKE-256](https://en.wikipedia.org/wiki/BLAKE_%28hash_function%29) hash function. For array with size <= 128Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 115)), (("getIntegerValue", List("Address|Alias", "String"), 7), ("Gets an integer by key. Throws an exception if there is no data.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 10)), (("getInteger", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "Int"), 7), ("Gets integer from a list of data entries by index", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 4)), (("calculateLeaseId", List("Lease"), 7), ("Calculates ID of lease obtained by invoke script transaction's call of the Lease structure.", List("Structure of a lease action."), "blockchain-functions", 1)), (("sigVerify_32Kb", List("ByteVector", "ByteVector", "ByteVector"), 7), ("Verifies a [Curve25519](https://en.wikipedia.org/wiki/Curve25519) signature. For message size <= 32Kb.", List("The message.", "The signature.", "The account public key."), "verification-functions", 64)), (("takeRight", List("String", "Int"), 7), ("Takes the last n characters from a string", List("The string.", "The number n."), "string-functions", 20)), (("getBooleanValue", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "Int"), 7), ("Extract data by index (fail on error)", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 4)), (("split", List("String", "String"), 7), ("Splits a string delimited by a separator into a list of substrings. Input string size limit = 500 bytes. Output list size limit = 20", List("The string.", "The separator."), "string-functions", 1)), (("sigVerify", List("ByteVector", "ByteVector", "ByteVector"), 7), ("Verifies a [Curve25519](https://en.wikipedia.org/wiki/Curve25519) signature.", List("The message.", "The signature.", "The account public key."), "verification-functions", 180)), (("toBytes", List("String"), 7), ("Converts a string to an array of bytes.", List("The string to convert."), "converting-functions", 8)), (("addressFromStringValue", List("String"), 7), ("Decodes address from [Base58](https://en.wikipedia.org/wiki/Base58) string.\nRaises an exception if the address cannot be decoded.", List("The string to decode."), "encoding-and-decoding-functions", 1)), (("groth16Verify_6inputs", List("ByteVector", "ByteVector", "ByteVector"), 7), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 6 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1700)), (("getBooleanValue", List("String"), 7), ("Gets a boolean value by key from state of current account. Throws an exception if there is no data.", List("The key."), "account-self-data-storage-functions", 10)), (("makeString", List("List[String]", "String"), 7), ("Returns all the elements of the list in a string using the separator string. Input list size limit = 70. Output string size limit = 500 bytes", List("The list of strings.", "The separator"), "list-functions", 1)), (("fromBase16String", List("String"), 7), ("Decodes [Base16](https://en.wikipedia.org/wiki/Hexadecimal) string to an array of bytes.", List("The string to decode."), "encoding-and-decoding-functions", 10)), (("groth16Verify_11inputs", List("ByteVector", "ByteVector", "ByteVector"), 7), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 11 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 2200)), (("getBoolean", List("Address|Alias", "String"), 7), ("Gets a boolean value by key.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 10)), (("take", List("String", "Int"), 7), ("Takes the first n characters from a string", List("The string.", "The number n."), "string-functions", 20)), (("toBigInt", List("ByteVector"), 7), ("Converts an array of bytes to an big integer.", List("The array of bytes to convert."), "converting-functions", 65)), (("getString", List("Address|Alias", "String"), 7), ("Gets a string by key.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 10)), (("keccak256_128Kb", List("ByteVector"), 7), ("[Keccak-256](https://keccak.team/files/Keccak-submission-3.pdf) hash function. For array with size <= 128Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 147)), (("parseBigInt", List("String"), 7), ("Converts an string to an integer.", List("The string to convert."), "converting-functions", 65)), (("pow", List("BigInt", "Int", "BigInt", "Int", "Int", "Ceiling|Down|Floor|HalfEven|HalfUp"), 7), ("Returns a number raised to a power", List("The base.", "The number of decimals of the base.", "The exponent.", "The number of decimals of the exponent.", "The number of decimals of the resulting value.", "One of the rounding functions."), "math-functions", 270)), (("size", List("List[T]"), 7), ("Returns the size of a list.", List("The list."), "list-functions", 2)), (("getBinaryValue", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "Int"), 7), ("Extract data by index (fail on error)", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 4)), (("toBase64String", List("ByteVector"), 7), ("Encodes array of bytes to [Base64](https://en.wikipedia.org/wiki/Base64) string.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 35)), (("blake2b256_64Kb", List("ByteVector"), 7), ("[BLAKE-256](https://en.wikipedia.org/wiki/BLAKE_%28hash_function%29) hash function. For array with size <= 64Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 58)), (("toString", List("Int"), 7), ("Converts an integer to a string.", List("The integer to convert."), "converting-functions", 1)), (("drop", List("String", "Int"), 7), ("Drops the first n characters of a string", List("The string.", "The number n."), "string-functions", 20)), (("bn256Groth16Verify_4inputs", List("ByteVector", "ByteVector", "ByteVector"), 7), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 4 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1000)), (("toInt", List("ByteVector"), 7), ("Converts an array of bytes to an integer.", List("The array of bytes to convert."), "converting-functions", 1)), (("sigVerify_64Kb", List("ByteVector", "ByteVector", "ByteVector"), 7), ("Verifies a [Curve25519](https://en.wikipedia.org/wiki/Curve25519) signature. For message size <= 64Kb.", List("The message.", "The signature.", "The account public key."), "verification-functions", 93)), (("getString", List("String"), 7), ("Gets a string by key from state of current account.", List("The key."), "account-self-data-storage-functions", 10)), (("groth16Verify_10inputs", List("ByteVector", "ByteVector", "ByteVector"), 7), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 10 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 2100)), (("getBinary", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "Int"), 7), ("Gets a binary value from a list of data entries by index", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 4)), (("toUtf8String", List("ByteVector"), 7), ("Converts an array of bytes to a UTF-8 string.", List("The array of bytes to convert."), "converting-functions", 7)), (("log", List("Int", "Int", "Int", "Int", "Int", "Ceiling|Down|Floor|HalfEven|HalfUp"), 7), ("Returns the logarithm of a number.", List("The base.", "The number of decimals of the base.", "The exponent.", "The number of decimals of the exponent.", "The number of decimals of the resulting value.", "The rounding function.\n"), "math-functions", 100)), (("median", List("List[Int]"), 7), ("Returns the median of the list.", List("The list of integers."), "list-functions", 20)), (("toBigInt", List("ByteVector", "Int", "Int"), 7), ("Converts an array of bytes to an big integer.", List("The array of bytes to convert.", "Offset", "Size"), "converting-functions", 65)), (("dropRight", List("ByteVector", "Int"), 7), ("Drops the first n bytes of an array of bytes.", List("The array of bytes.", "The number n."), "byte-array-functions", 6)), (("getBinary", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "String"), 7), ("Gets a binary value from a list of data entries by key", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), (("transactionHeightById", List("ByteVector"), 7), ("Gets the [block height](/blockchain/block-height.md) of a transaction.", List("ID of the transaction."), "blockchain-functions", 20)), (("-", List("BigInt"), 7), ("Change integer sign.", List("Value"), "operators", 8)), (("blake2b256_16Kb", List("ByteVector"), 7), ("[BLAKE-256](https://en.wikipedia.org/wiki/BLAKE_%28hash_function%29) hash function. For array with size <= 16Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 13)), (("bn256Groth16Verify_10inputs", List("ByteVector", "ByteVector", "ByteVector"), 7), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 10 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1300)), (("makeString_2C", List("List[String]", "String"), 7), ("Returns all the elements of the list in a string using the separator string. Input list size limit = 100. Output string size limit = 6000 bytes", List("The list of strings.", "The separator"), "list-functions", 2)), (("cons", List("A", "List[B]"), 7), ("Prepends a new element to the list.", List("The element.", "The list."), "list-functions", 1)), (("groth16Verify_9inputs", List("ByteVector", "ByteVector", "ByteVector"), 7), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 9 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 2000)), (("Lease", List("Address|Alias", "Int"), 7), ("Lease action simplified constructor", List("Recipient", "Amount of Waves"), "internal-functions", 1)), (("toBytes", List("Int"), 7), ("Converts an integer to an array of bytes.", List("The integer to convert."), "converting-functions", 1)), (("getInteger", List("String"), 7), ("Gets an integer by key from state of current account.", List("The key."), "account-self-data-storage-functions", 10)), (("assetBalance", List("Address|Alias", "ByteVector"), 7), ("Gets account balance by token ID.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "Token ID."), "account-data-storage-functions", 10)), (("toString", List("Address"), 7), ("Convert address bytes to string", List("The address to convert"), "converting-functions", 1)), (("invoke", List("Address|Alias", "String|Unit", "List[Any]", "List[AttachedPayment]"), 7), ("Invoke function from other dApp.", List("dApp", "function name", "arguments", "Attached payments"), "blockchain-functions", 75)), (("!=", List("T", "T"), 7), ("Check inequality.", List("First value", "Second value"), "operators", 1)), (("getStringValue", List("String"), 7), ("Gets a string by key from state of current account. Throws an exception if there is no data.", List("The key."), "account-self-data-storage-functions", 10)), (("-", List("Int", "Int"), 7), ("Subtract integers.", List("First value", "Second value"), "operators", 1)), (("parseIntValue", List("String"), 7), ("Converts the string representation of a number to its integer equivalent.\nRaises an exception if the string cannot be parsed.", List("The string to parse."), "converting-functions", 2)), (("++", List("List[A]", "List[B]"), 7), ("List Concatenation.", List("First list", "Second list"), "operators", 4)), (("+", List("BigInt", "BigInt"), 7), ("Sum big integers.", List("First value", "Second value"), "operators", 8)), (("toBytes", List("BigInt"), 7), ("Converts an BigInt to an array of bytes.", List("The BigInt to convert."), "converting-functions", 65)), (("Issue", List("String", "String", "Int", "Int", "Boolean", "Script|Unit", "Int"), 7), ("Issue action detailed constructor", List("Token name", "Token description", "Amount of the token", "Number of digits in decimal part", "Reissue ability flag", "Smart asset script (optional)", "Sequential number"), "internal-functions", 1)), (("toBase16String", List("ByteVector"), 7), ("Encodes array of bytes to [Base16](https://en.wikipedia.org/wiki/Hexadecimal) string.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 10)), (("getInteger", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "String"), 7), ("Gets integer from a list of data entries by key", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), (("isDefined", List("T|Unit"), 7), ("Checks if a value is not `Unit`", List("Optional value"), "optional-value-functions", 1)), (("groth16Verify_5inputs", List("ByteVector", "ByteVector", "ByteVector"), 7), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 5 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1600)), (("bn256Groth16Verify_11inputs", List("ByteVector", "ByteVector", "ByteVector"), 7), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 11 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1350)), (("size", List("String"), 7), ("Returns the size of a string", List("The string."), "string-functions", 1)), (("valueOrErrorMessage", List("T|Unit", "String"), 7), ("Extract value from option or fail with message", List("Optional value", "Error message"), "optional-value-functions", 2)), (("pow", List("Int", "Int", "Int", "Int", "Int", "Ceiling|Down|Floor|HalfEven|HalfUp"), 7), ("Returns a number raised to a power", List("The base.", "The number of decimals of the base.", "The exponent.", "The number of decimals of the exponent.", "The number of decimals of the resulting value.", "One of the rounding functions."), "math-functions", 28)), (("+", List("Int", "Int"), 7), ("Sum integers.", List("First value", "Second value"), "operators", 1)), (("transferTransactionFromProto", List("ByteVector"), 7), ("Deserializes transfer transaction: converts protobuf-encoded binary format to a TransferTransaction structure.", List("Transfer transaction in protobuf-encoded binary format."), "blockchain-functions", 5)), (("groth16Verify_2inputs", List("ByteVector", "ByteVector", "ByteVector"), 7), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 2 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1300)), (("groth16Verify_3inputs", List("ByteVector", "ByteVector", "ByteVector"), 7), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 3 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1400)), (("take", List("ByteVector", "Int"), 7), ("Takes the first n bytes from an array of bytes.", List("The array of bytes.", "The number n."), "byte-array-functions", 6)), (("dropRight", List("String", "Int"), 7), ("Drops the last n characters of a string", List("The string.", "The number n."), "string-functions", 20)), (("median", List("List[BigInt]"), 7), ("Returns the median of the list.", List("The list of BigInt."), "list-functions", 160)), (("%", List("Int", "Int"), 7), ("Calculate modulo.", List("Divisible", "Divisor"), "operators", 1)), (("Lease", List("Address|Alias", "Int", "Int"), 7), ("Lease action detailed constructor", List("Recipient", "Amount of Waves", "Nonce to distinguish"), "internal-functions", 1)), (("log", List("BigInt", "Int", "BigInt", "Int", "Int", "Ceiling|Down|Floor|HalfEven|HalfUp"), 7), ("Returns the logarithm of a number.", List("The base.", "The number of decimals of the base.", "The exponent.", "The number of decimals of the exponent.", "The number of decimals of the resulting value.", "The rounding function.\n"), "math-functions", 200)), (("keccak256_32Kb", List("ByteVector"), 7), ("[Keccak-256](https://keccak.team/files/Keccak-submission-3.pdf) hash function. For array with size <= 32Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 39)), (("getString", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "String"), 7), ("Gets a string value from a list of data entries by key", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), (("blake2b256", List("ByteVector"), 7), ("[BLAKE-256](https://en.wikipedia.org/wiki/BLAKE_%28hash_function%29) hash function.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 136)), (("ecrecover", List("ByteVector", "ByteVector"), 7), ("Recovers the public key from hash of ECDSA-encoded message and signature.", List("The hash of encoded message.", "The signature."), "encoding-and-decoding-functions", 70)), (("blockInfoByHeight", List("Int"), 7), ("Gets the information about a [block](/blockchain/block.md) by the [block height](/blockchain/block-height.md)", List("Block height."), "blockchain-functions", 5)), (("min", List("List[BigInt]"), 7), ("Returns the minimum of the list.", List("The list of big integers."), "list-functions", 192)), (("min", List("List[Int]"), 7), ("Returns the minimum of the list.", List("The list of integers."), "list-functions", 3)), ((">", List("Int", "Int"), 7), ("Check if integer greater comparison.", List("First value", "Second value"), "operators", 1)), (("sha256_128Kb", List("ByteVector"), 7), ("[SHA-256](https://en.wikipedia.org/wiki/SHA-2) hash function. For array with size <= 128Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 93)), (("split_4C", List("String", "String"), 7), ("Splits a string delimited by a separator into a list of substrings. Input string size limit = 6000 bytes. Output list size limit = 100", List("The string.", "The separator."), "string-functions", 4)), (("addressFromRecipient", List("Address|Alias"), 7), ("Extract address or lookup alias", List("Address or alias of the account."), "converting-functions", 5)), (("valueOrElse", List("T|Unit", "T"), 7), ("Returns value from union type argument if it's not unit. Otherwise, returns the second argument.", List("The argument to return value from.", "Returned if the value of t is unit."), "optional-value-functions", 2)), (("keccak256", List("ByteVector"), 7), ("[Keccak-256](https://keccak.team/files/Keccak-submission-3.pdf) hash function.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 195)), (("getBinaryValue", List("Address|Alias", "String"), 7), ("Gets an array of bytes by key. Throws an exception if there is no data.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 10)), (("==", List("T", "T"), 7), ("Check equality.", List("First value", "Second value"), "operators", 1)), (("*", List("BigInt", "BigInt"), 7), ("Multiply big integers.", List("Multiplier", "Multiplier"), "operators", 64)), (("removeByIndex", List("List[T]", "Int"), 7), ("Removes the element at given index from the list and returns new list.", List("The list.", "The element."), "list-functions", 7)), (("max", List("List[BigInt]"), 7), ("Returns the maximum of the list.", List("The list of big integers."), "list-functions", 192)), (("parseInt", List("String"), 7), ("Converts the string representation of a number to its integer equivalent.", List("The string to parse."), "converting-functions", 2)), (("getBinary", List("String"), 7), ("Gets an array of bytes by key from state of current account.", List("The key."), "account-self-data-storage-functions", 10)), (("lastIndexOf", List("List[T]", "T"), 7), ("Returns the index of the last occurrence of given value", List("The list.", "The element."), "list-functions", 5)), (("groth16Verify_15inputs", List("ByteVector", "ByteVector", "ByteVector"), 7), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 15 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 2600)), (("scriptHash", List("Address|Alias"), 7), ("blake2b hash of account's script", List("account"), "blockchain-functions", 200)), (("split_51C", List("String", "String"), 7), ("Splits a string delimited by a separator into a list of substrings. Input string size limit = 32767 bytes. Output list size limit = 1000", List("The string.", "The separator."), "string-functions", 51)), (("getStringValue", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "String"), 7), ("Find and extract data by key (fail on error)", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), (("/", List("Int", "Int"), 7), ("Divide integers.", List("Divisible", "Divisor"), "operators", 1)), (("size", List("(A, B)|(A, B, C)|(A, B, C, D)|(A, B, C, D, E)|(A, B, C, D, E, F)|(A, B, C, D, E, F, G)|(A, B, C, D, E, F, G, H)|(A, B, C, D, E, F, G, H, I)|(A, B, C, D, E, F, G, H, I, J)|(A, B, C, D, E, F, G, H, I, J, K)|(A, B, C, D, E, F, G, H, I, J, K, L)|(A, B, C, D, E, F, G, H, I, J, K, L, M)|(A, B, C, D, E, F, G, H, I, J, K, L, M, N)|(A, B, C, D, E, F, G, H, I, J, K, L, M, N, O)|(A, B, C, D, E, F, G, H, I, J, K, L, M, N, O, P)|(A, B, C, D, E, F, G, H, I, J, K, L, M, N, O, P, Q)|(A, B, C, D, E, F, G, H, I, J, K, L, M, N, O, P, Q, R)|(A, B, C, D, E, F, G, H, I, J, K, L, M, N, O, P, Q, R, S)|(A, B, C, D, E, F, G, H, I, J, K, L, M, N, O, P, Q, R, S, T)|(A, B, C, D, E, F, G, H, I, J, K, L, M, N, O, P, Q, R, S, T, U)|(A, B, C, D, E, F, G, H, I, J, K, L, M, N, O, P, Q, R, S, T, U, V)"), 7), ("Number of elements of the tuple", List("The tuple"), "internal-functions", 1)), (("sha256", List("ByteVector"), 7), ("[SHA-256](https://en.wikipedia.org/wiki/SHA-2) hash function.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 118)), (("toInt", List("BigInt"), 7), ("Converts a BigInt to an integer.", List("The BigInt to convert."), "converting-functions", 1)), (("createMerkleRoot", List("List[ByteVector]", "ByteVector", "Int"), 7), ("Calculates the Merkle root hash for transactions of block.", List("Array of sibling hashes of the Merkle tree.", "Hash of transaction.", "Index of the transaction in the block."), "verification-functions", 30)), (("fromBase64String", List("String"), 7), ("Decodes [Base64](https://en.wikipedia.org/wiki/Base64) string to an array of bytes.", List("The string to decode."), "encoding-and-decoding-functions", 40)), (("keccak256_16Kb", List("ByteVector"), 7), ("[Keccak-256](https://keccak.team/files/Keccak-submission-3.pdf) hash function. For array with size <= 16Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 20)), (("indexOf", List("String", "String", "Int"), 7), ("Returns the index of the first occurrence of a substring after a certain index", List("The string.", "The substring.", "The index."), "string-functions", 3)), (("bn256Groth16Verify_15inputs", List("ByteVector", "ByteVector", "ByteVector"), 7), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 15 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1600)), (("getInteger", List("Address|Alias", "String"), 7), ("Gets an integer by key.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 10)), (("parseBigIntValue", List("String"), 7), ("Converts an string to an integer.", List("The string to convert."), "converting-functions", 65)), (("fraction", List("BigInt", "BigInt", "BigInt"), 7), ("Multiply and division with unlimited intermediate representation", List("Multiplier", "Multiplier", "Divisor"), "math-functions", 1)), (("transferTransactionById", List("ByteVector"), 7), ("Gets the data of a transfer transaction.", List("ID of the transfer transaction."), "blockchain-functions", 60)), (("%", List("BigInt", "BigInt"), 7), ("Calculate big modulo.", List("Divisible", "Divisor"), "operators", 64)), ((">=", List("Int", "Int"), 7), ("Check if integer greater or equal comparison.", List("First value", "Second value"), "operators", 1)), (("+", List("ByteVector", "ByteVector"), 7), ("Concat limited byte vectors.", List("First value", "Second value"), "operators", 2)), (("lastIndexOf", List("String", "String", "Int"), 7), ("Returns the index of the last occurrence of a substring after a certain index", List("The string.", "The substring.", "The index."), "string-functions", 3)), (("addressFromPublicKey", List("ByteVector"), 7), ("Converts account public key to [address](blockhain/address.md).", List("The public key to convert."), "converting-functions", 1)), (("rsaVerify_64Kb", List("Md5|NoAlg|Sha1|Sha224|Sha256|Sha3224|Sha3256|Sha3384|Sha3512|Sha384|Sha512", "ByteVector", "ByteVector", "ByteVector"), 7), ("Verifies an [RSA](https://en.wikipedia.org/wiki/RSA_%28cryptosystem%29) signature. For message size <= 64Kb.", List("The RSA algorithm.", "The message.", "The signature.", "The public key."), "verification-functions", 625)), (("sha256_64Kb", List("ByteVector"), 7), ("[SHA-256](https://en.wikipedia.org/wiki/SHA-2) hash function. For array with size <= 64Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 47)), (("value", List("T|Unit"), 7), ("Extract value from option or fail", List("Optional value"), "optional-value-functions", 2)), (("getStringValue", List("Address|Alias", "String"), 7), ("Gets a string by key. Throws an exception if there is no data.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 10)), (("fraction", List("BigInt", "BigInt", "BigInt", "Ceiling|Down|Floor|HalfEven|HalfUp"), 7), ("Multiply and division with unlimited intermediate representation", List("Multiplier", "Multiplier", "Divisor", "Rounding mode"), "math-functions", 1)), (("bn256Groth16Verify_7inputs", List("ByteVector", "ByteVector", "ByteVector"), 7), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 7 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1150)), (("sha256_32Kb", List("ByteVector"), 7), ("[SHA-256](https://en.wikipedia.org/wiki/SHA-2) hash function. For array with size <= 32Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 23)), (("getString", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "Int"), 7), ("Gets a string value from a list of data entries by index", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 4)), (("getStringValue", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "Int"), 7), ("Extract data by index (fail on error)", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 4)), (("max", List("List[Int]"), 7), ("Returns the maximum of the list.", List("The list of integers."), "list-functions", 3)), (("keccak256_64Kb", List("ByteVector"), 7), ("[Keccak-256](https://keccak.team/files/Keccak-submission-3.pdf) hash function. For array with size <= 64Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 74)), (("getBoolean", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "Int"), 7), ("Gets a boolean value from a list of data entries by index", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 4)))
lazy val funcsV8 = Map(((":+", List("List[A]", "B"), 8), ("Adding the element to the end of the list.", List("The list", "The element"), "operators", 1)), (("blake2b256", List("ByteVector"), 8), ("[BLAKE-256](https://en.wikipedia.org/wiki/BLAKE_%28hash_function%29) hash function.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 136)), (("getInteger", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "Int"), 8), ("Gets integer from a list of data entries by index", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 4)), (("groth16Verify_5inputs", List("ByteVector", "ByteVector", "ByteVector"), 8), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 5 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1600)), (("makeString", List("List[String]", "String"), 8), ("Returns all the elements of the list in a string using the separator string. Input list size limit = 70. Output string size limit = 500 bytes", List("The list of strings.", "The separator"), "list-functions", 1)), (("-", List("BigInt"), 8), ("Change integer sign.", List("Value"), "operators", 1)), (("removeByIndex", List("List[T]", "Int"), 8), ("Removes the element at given index from the list and returns new list.", List("The list.", "The index."), "list-functions", 4)), (("getInteger", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "String"), 8), ("Gets integer from a list of data entries by key", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), (("getBinary", List("String"), 8), ("Gets an array of bytes by key from state of current account.", List("The key."), "account-self-data-storage-functions", 10)), (("keccak256_16Kb", List("ByteVector"), 8), ("[Keccak-256](https://keccak.team/files/Keccak-submission-3.pdf) hash function. For array with size <= 16Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 20)), (("toBigInt", List("Int"), 8), ("Converts an integer to an integer.", List("The integer to convert."), "converting-functions", 1)), (("throw", List("String"), 8), ("Raises an exception with a message.", List("The exception message."), "exception-functions", 1)), (("!", List("Boolean"), 8), ("Unary negation.", List("Value"), "operators", 1)), (("toBytes", List("Boolean"), 8), ("Converts a boolean to an array of bytes.", List("The boolean to convert."), "converting-functions", 1)), (("toString", List("Boolean"), 8), ("Converts a boolean to a string.", List("The boolean to convert."), "converting-functions", 1)), (("getBinaryValue", List("Address|Alias", "String"), 8), ("Gets an array of bytes by key. Throws an exception if there is no data.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 10)), (("sigVerify_64Kb", List("ByteVector", "ByteVector", "ByteVector"), 8), ("Verifies a [Curve25519](https://en.wikipedia.org/wiki/Curve25519) signature. For message size <= 64Kb.", List("The message.", "The signature.", "The account public key."), "verification-functions", 93)), (("getString", List("String"), 8), ("Gets a string by key from state of current account.", List("The key."), "account-self-data-storage-functions", 10)), (("reentrantInvoke", List("Address|Alias", "String|Unit", "List[Any]", "List[AttachedPayment]"), 8), ("Invoke function from other dApp allowing reentrancy to the current one.", List("dApp", "function name", "arguments", "Attached payments"), "blockchain-functions", 75)), (("groth16Verify_8inputs", List("ByteVector", "ByteVector", "ByteVector"), 8), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 8 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1900)), (("min", List("List[BigInt]"), 8), ("Returns the minimum of the list.", List("The list of big integers."), "list-functions", 6)), (("keccak256", List("ByteVector"), 8), ("[Keccak-256](https://keccak.team/files/Keccak-submission-3.pdf) hash function.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 195)), (("getBooleanValue", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "String"), 8), ("Find and extract data by key (fail on error)", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), (("max", List("List[Int]"), 8), ("Returns the maximum of the list.", List("The list of integers."), "list-functions", 3)), (("value", List("T|Unit"), 8), ("Extract value from option or fail", List("Optional value"), "optional-value-functions", 2)), (("bn256Groth16Verify", List("ByteVector", "ByteVector", "ByteVector"), 8), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1650)), (("getBinary", List("Address|Alias", "String"), 8), ("Gets an array of bytes by key.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 10)), (("groth16Verify", List("ByteVector", "ByteVector", "ByteVector"), 8), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 2700)), (("bn256Groth16Verify_7inputs", List("ByteVector", "ByteVector", "ByteVector"), 8), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 7 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1150)), (("size", List("String"), 8), ("Returns the size of a string", List("The string."), "string-functions", 1)), (("getBinaryValue", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "String"), 8), ("Find and extract data by key (fail on error)", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), (("toInt", List("BigInt"), 8), ("Converts a BigInt to an integer.", List("The BigInt to convert."), "converting-functions", 1)), (("bn256Groth16Verify_1inputs", List("ByteVector", "ByteVector", "ByteVector"), 8), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 1 input.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 800)), (("getIntegerValue", List("String"), 8), ("Gets an integer by key from state of current account. Throws an exception if there is no data.", List("The key."), "account-self-data-storage-functions", 10)), (("median", List("List[BigInt]"), 8), ("Returns the median of the list.", List("The list of BigInt."), "list-functions", 35)), (("sigVerify_32Kb", List("ByteVector", "ByteVector", "ByteVector"), 8), ("Verifies a [Curve25519](https://en.wikipedia.org/wiki/Curve25519) signature. For message size <= 32Kb.", List("The message.", "The signature.", "The account public key."), "verification-functions", 64)), (("-", List("Int", "Int"), 8), ("Subtract integers.", List("First value", "Second value"), "operators", 1)), (("getBoolean", List("String"), 8), ("Gets a boolean value by key from state of current account.", List("The key."), "account-self-data-storage-functions", 10)), (("take", List("String", "Int"), 8), ("Takes the first n characters from a string", List("The string.", "The number n."), "string-functions", 20)), (("log", List("Int", "Int", "Int", "Int", "Int", "Ceiling|Down|Floor|HalfEven|HalfUp"), 8), ("Returns the logarithm of a number.", List("The base.", "The number of decimals of the base.", "The exponent.", "The number of decimals of the exponent.", "The number of decimals of the resulting value.", "The rounding function.\n"), "math-functions", 100)), (("takeRight", List("String", "Int"), 8), ("Takes the last n characters from a string", List("The string.", "The number n."), "string-functions", 20)), (("pow", List("Int", "Int", "Int", "Int", "Int", "Ceiling|Down|Floor|HalfEven|HalfUp"), 8), ("Returns a number raised to a power", List("The base.", "The number of decimals of the base.", "The exponent.", "The number of decimals of the exponent.", "The number of decimals of the resulting value.", "One of the rounding functions."), "math-functions", 28)), (("makeString_11C", List("List[String]", "String"), 8), ("Returns all the elements of the list in a string using the separator string. Input list size limit = 1000. Output string size limit = 32767 bytes", List("The list of strings.", "The separator"), "list-functions", 11)), (("toBigInt", List("ByteVector"), 8), ("Converts an array of bytes to an big integer.", List("The array of bytes to convert."), "converting-functions", 1)), (("blockInfoByHeight", List("Int"), 8), ("Gets the information about a [block](/blockchain/block.md) by the [block height](/blockchain/block-height.md)", List("Block height."), "blockchain-functions", 5)), (("sigVerify_16Kb", List("ByteVector", "ByteVector", "ByteVector"), 8), ("Verifies a [Curve25519](https://en.wikipedia.org/wiki/Curve25519) signature. For message size <= 16Kb.", List("The message.", "The signature.", "The account public key."), "verification-functions", 50)), (("rsaVerify_128Kb", List("Md5|NoAlg|Sha1|Sha224|Sha256|Sha3224|Sha3256|Sha3384|Sha3512|Sha384|Sha512", "ByteVector", "ByteVector", "ByteVector"), 8), ("Verifies an [RSA](https://en.wikipedia.org/wiki/RSA_%28cryptosystem%29) signature. For message size <= 128Kb.", List("The RSA algorithm.", "The message.", "The signature.", "The public key."), "verification-functions", 750)), (("fromBase58String", List("String"), 8), ("Decodes [Base58](https://en.wikipedia.org/wiki/Base58) string to an array of bytes.", List("The string to decode."), "encoding-and-decoding-functions", 1)), (("makeString_2C", List("List[String]", "String"), 8), ("Returns all the elements of the list in a string using the separator string. Input list size limit = 100. Output string size limit = 6000 bytes", List("The list of strings.", "The separator"), "list-functions", 2)), (("isDefined", List("T|Unit"), 8), ("Checks if a value is not `Unit`", List("Optional value"), "optional-value-functions", 1)), (("fraction", List("Int", "Int", "Int"), 8), ("Multiply and division with unlimited intermediate representation", List("Multiplier", "Multiplier", "Divisor"), "math-functions", 1)), (("sha256", List("ByteVector"), 8), ("[SHA-256](https://en.wikipedia.org/wiki/SHA-2) hash function.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 118)), (("dropRight", List("ByteVector", "Int"), 8), ("Drops the first n bytes of an array of bytes.", List("The array of bytes.", "The number n."), "byte-array-functions", 6)), (("bn256Groth16Verify_14inputs", List("ByteVector", "ByteVector", "ByteVector"), 8), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 14 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1550)), (("getStringValue", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "Int"), 8), ("Extract data by index (fail on error)", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 4)), (("takeRight", List("ByteVector", "Int"), 8), ("Takes the last n bytes from an array of bytes.", List("The array of bytes.", "The number n."), "byte-array-functions", 6)), (("+", List("String", "String"), 8), ("Concat limited strings.", List("First value", "Second value"), "operators", 1)), (("drop", List("ByteVector", "Int"), 8), ("Drops the first n bytes of an array of bytes.", List("The array of bytes.", "The number n."), "byte-array-functions", 6)), (("bn256Groth16Verify_2inputs", List("ByteVector", "ByteVector", "ByteVector"), 8), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 2 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 850)), (("Issue", List("String", "String", "Int", "Int", "Boolean", "Script|Unit", "Int"), 8), ("Issue action detailed constructor", List("Token name", "Token description", "Amount of the token", "Number of digits in decimal part", "Reissue ability flag", "Smart asset script (optional)", "Sequential number"), "internal-functions", 1)), (("parseBigInt", List("String"), 8), ("Converts an string to an integer.", List("The string to convert."), "converting-functions", 1)), (("sqrt", List("Int", "Int", "Int", "Ceiling|Down|Floor|HalfEven|HalfUp"), 8), ("Returns a square root.", List("The number", "The number of decimals of the number.", "The number of decimals of the resulting value.", "One of the rounding functions."), "math-functions", 2)), (("drop", List("String", "Int"), 8), ("Drops the first n characters of a string", List("The string.", "The number n."), "string-functions", 20)), (("dropRight", List("String", "Int"), 8), ("Drops the last n characters of a string", List("The string.", "The number n."), "string-functions", 20)), (("bn256Groth16Verify_3inputs", List("ByteVector", "ByteVector", "ByteVector"), 8), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 3 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 950)), (("groth16Verify_7inputs", List("ByteVector", "ByteVector", "ByteVector"), 8), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 7 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1800)), (("getStringValue", List("Address|Alias", "String"), 8), ("Gets a string by key. Throws an exception if there is no data.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 10)), (("toBase64String", List("ByteVector"), 8), ("Encodes array of bytes to [Base64](https://en.wikipedia.org/wiki/Base64) string.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 35)), (("groth16Verify_14inputs", List("ByteVector", "ByteVector", "ByteVector"), 8), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 14 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 2500)), (("fraction", List("BigInt", "BigInt", "BigInt"), 8), ("Multiply and division with unlimited intermediate representation", List("Multiplier", "Multiplier", "Divisor"), "math-functions", 1)), (("bn256Groth16Verify_8inputs", List("ByteVector", "ByteVector", "ByteVector"), 8), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 8 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1200)), (("Issue", List("String", "String", "Int", "Int", "Boolean"), 8), ("Issue action simplified constructor", List("Token name", "Token description", "Amount of the token", "Number of digits in decimal part", "Reissue ability flag"), "internal-functions", 1)), (("size", List("List[T]"), 8), ("Returns the size of a list.", List("The list."), "list-functions", 2)), (("getInteger", List("String"), 8), ("Gets an integer by key from state of current account.", List("The key."), "account-self-data-storage-functions", 10)), (("transactionHeightById", List("ByteVector"), 8), ("Gets the [block height](/blockchain/block-height.md) of a transaction.", List("ID of the transaction."), "blockchain-functions", 20)), (("parseBigIntValue", List("String"), 8), ("Converts an string to an integer.", List("The string to convert."), "converting-functions", 1)), (("/", List("BigInt", "BigInt"), 8), ("Divide big integers.", List("Divisible", "Divisor"), "operators", 1)), (("getBinaryValue", List("String"), 8), ("Gets an array of bytes by key from state of current account. Throws an exception if there is no data.", List("The key."), "account-self-data-storage-functions", 10)), (("split_51C", List("String", "String"), 8), ("Splits a string delimited by a separator into a list of substrings. Input string size limit = 32767 bytes. Output list size limit = 1000", List("The string.", "The separator."), "string-functions", 51)), (("/", List("Int", "Int"), 8), ("Divide integers.", List("Divisible", "Divisor"), "operators", 1)), (("getBoolean", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "String"), 8), ("Gets a boolean value from a list of data entries by key", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), (("getString", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "Int"), 8), ("Gets a string value from a list of data entries by index", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 4)), (("wavesBalance", List("Address|Alias"), 8), ("Gets account waves balance details.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account."), "account-data-storage-functions", 10)), (("getBooleanValue", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "Int"), 8), ("Extract data by index (fail on error)", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 4)), (("toBytes", List("BigInt"), 8), ("Converts an BigInt to an array of bytes.", List("The BigInt to convert."), "converting-functions", 1)), (("getBoolean", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "Int"), 8), ("Gets a boolean value from a list of data entries by index", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 4)), ((">=", List("BigInt", "BigInt"), 8), ("Check if integer greater or equal comparison.", List("First value", "Second value"), "operators", 1)), (("+", List("Int", "Int"), 8), ("Sum integers.", List("First value", "Second value"), "operators", 1)), (("blake2b256_64Kb", List("ByteVector"), 8), ("[BLAKE-256](https://en.wikipedia.org/wiki/BLAKE_%28hash_function%29) hash function. For array with size <= 64Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 58)), (("calculateLeaseId", List("Lease"), 8), ("Calculates ID of lease obtained by invoke script transaction's call of the Lease structure.", List("Structure of a lease action."), "blockchain-functions", 1)), (("addressFromPublicKey", List("ByteVector"), 8), ("Converts account public key to [address](blockhain/address.md).", List("The public key to convert."), "converting-functions", 1)), (("lastIndexOf", List("String", "String"), 8), ("Returns the index of the last occurrence of a substring", List("The string.", "The substring."), "string-functions", 3)), (("rsaVerify_32Kb", List("Md5|NoAlg|Sha1|Sha224|Sha256|Sha3224|Sha3256|Sha3384|Sha3512|Sha384|Sha512", "ByteVector", "ByteVector", "ByteVector"), 8), ("Verifies an [RSA](https://en.wikipedia.org/wiki/RSA_%28cryptosystem%29) signature. For message size <= 32Kb.", List("The RSA algorithm.", "The message.", "The signature.", "The public key."), "verification-functions", 550)), (("rsaVerify", List("Md5|NoAlg|Sha1|Sha224|Sha256|Sha3224|Sha3256|Sha3384|Sha3512|Sha384|Sha512", "ByteVector", "ByteVector", "ByteVector"), 8), ("Verifies an [RSA](https://en.wikipedia.org/wiki/RSA_%28cryptosystem%29) signature.", List("The RSA algorithm.", "The message.", "The signature.", "The public key."), "verification-functions", 1000)), (("min", List("List[Int]"), 8), ("Returns the minimum of the list.", List("The list of integers."), "list-functions", 3)), (("sigVerify_8Kb", List("ByteVector", "ByteVector", "ByteVector"), 8), ("Verifies a [Curve25519](https://en.wikipedia.org/wiki/Curve25519) signature. For message size <= 16Kb.", List("The message.", "The signature.", "The account public key."), "verification-functions", 43)), (("split", List("String", "String"), 8), ("Splits a string delimited by a separator into a list of substrings. Input string size limit = 500 bytes. Output list size limit = 20", List("The string.", "The separator."), "string-functions", 1)), (("fromBase64String", List("String"), 8), ("Decodes [Base64](https://en.wikipedia.org/wiki/Base64) string to an array of bytes.", List("The string to decode."), "encoding-and-decoding-functions", 40)), (("*", List("Int", "Int"), 8), ("Multiply integers.", List("Multiplier", "Multiplier"), "operators", 1)), (("ecrecover", List("ByteVector", "ByteVector"), 8), ("Recovers the public key from hash of ECDSA-encoded message and signature.", List("The hash of encoded message.", "The signature."), "encoding-and-decoding-functions", 70)), (("lastIndexOf", List("List[T]", "T"), 8), ("Returns the index of the last occurrence of given value", List("The list.", "The element."), "list-functions", 5)), (("rsaVerify_16Kb", List("Md5|NoAlg|Sha1|Sha224|Sha256|Sha3224|Sha3256|Sha3384|Sha3512|Sha384|Sha512", "ByteVector", "ByteVector", "ByteVector"), 8), ("Verifies an [RSA](https://en.wikipedia.org/wiki/RSA_%28cryptosystem%29) signature. For message size <= 16Kb.", List("The RSA algorithm.", "The message.", "The signature.", "The public key."), "verification-functions", 500)), (("valueOrErrorMessage", List("T|Unit", "String"), 8), ("Extract value from option or fail with message", List("Optional value", "Error message"), "optional-value-functions", 2)), (("indexOf", List("String", "String", "Int"), 8), ("Returns the index of the first occurrence of a substring after a certain index", List("The string.", "The substring.", "The index."), "string-functions", 3)), (("groth16Verify_4inputs", List("ByteVector", "ByteVector", "ByteVector"), 8), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 4 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1500)), (("sha256_32Kb", List("ByteVector"), 8), ("[SHA-256](https://en.wikipedia.org/wiki/SHA-2) hash function. For array with size <= 32Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 23)), (("toString", List("Address"), 8), ("Convert address bytes to string", List("The address to convert"), "converting-functions", 1)), (("bn256Groth16Verify_12inputs", List("ByteVector", "ByteVector", "ByteVector"), 8), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 12 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1400)), (("bn256Groth16Verify_13inputs", List("ByteVector", "ByteVector", "ByteVector"), 8), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 13 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1450)), (("getBoolean", List("Address|Alias", "String"), 8), ("Gets a boolean value by key.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 10)), (("sqrt", List("BigInt", "Int", "Int", "Ceiling|Down|Floor|HalfEven|HalfUp"), 8), ("Returns a square root.", List("The base.", "The number of decimals of the base.", "The number of decimals of the resulting value.", "One of the rounding functions."), "math-functions", 5)), (("size", List("(A, B)|(A, B, C)|(A, B, C, D)|(A, B, C, D, E)|(A, B, C, D, E, F)|(A, B, C, D, E, F, G)|(A, B, C, D, E, F, G, H)|(A, B, C, D, E, F, G, H, I)|(A, B, C, D, E, F, G, H, I, J)|(A, B, C, D, E, F, G, H, I, J, K)|(A, B, C, D, E, F, G, H, I, J, K, L)|(A, B, C, D, E, F, G, H, I, J, K, L, M)|(A, B, C, D, E, F, G, H, I, J, K, L, M, N)|(A, B, C, D, E, F, G, H, I, J, K, L, M, N, O)|(A, B, C, D, E, F, G, H, I, J, K, L, M, N, O, P)|(A, B, C, D, E, F, G, H, I, J, K, L, M, N, O, P, Q)|(A, B, C, D, E, F, G, H, I, J, K, L, M, N, O, P, Q, R)|(A, B, C, D, E, F, G, H, I, J, K, L, M, N, O, P, Q, R, S)|(A, B, C, D, E, F, G, H, I, J, K, L, M, N, O, P, Q, R, S, T)|(A, B, C, D, E, F, G, H, I, J, K, L, M, N, O, P, Q, R, S, T, U)|(A, B, C, D, E, F, G, H, I, J, K, L, M, N, O, P, Q, R, S, T, U, V)"), 8), ("Number of elements of the tuple", List("The tuple"), "internal-functions", 1)), (("groth16Verify_13inputs", List("ByteVector", "ByteVector", "ByteVector"), 8), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 13 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 2400)), (("lastIndexOf", List("String", "String", "Int"), 8), ("Returns the index of the last occurrence of a substring after a certain index", List("The string.", "The substring.", "The index."), "string-functions", 3)), (("blake2b256_128Kb", List("ByteVector"), 8), ("[BLAKE-256](https://en.wikipedia.org/wiki/BLAKE_%28hash_function%29) hash function. For array with size <= 128Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 115)), (("addressFromRecipient", List("Address|Alias"), 8), ("Extract address or lookup alias", List("Address or alias of the account."), "converting-functions", 5)), (("fromBase16String", List("String"), 8), ("Decodes [Base16](https://en.wikipedia.org/wiki/Hexadecimal) string to an array of bytes.", List("The string to decode."), "encoding-and-decoding-functions", 10)), (("groth16Verify_6inputs", List("ByteVector", "ByteVector", "ByteVector"), 8), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 6 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1700)), (("max", List("List[BigInt]"), 8), ("Returns the maximum of the list.", List("The list of big integers."), "list-functions", 6)), (("bn256Groth16Verify_6inputs", List("ByteVector", "ByteVector", "ByteVector"), 8), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 6 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1100)), (("toBase16String", List("ByteVector"), 8), ("Encodes array of bytes to [Base16](https://en.wikipedia.org/wiki/Hexadecimal) string.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 10)), (("parseInt", List("String"), 8), ("Converts the string representation of a number to its integer equivalent.", List("The string to parse."), "converting-functions", 2)), (("bn256Groth16Verify_9inputs", List("ByteVector", "ByteVector", "ByteVector"), 8), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 9 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1250)), (("assetBalance", List("Address|Alias", "ByteVector"), 8), ("Gets account balance by token ID.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "Token ID."), "account-data-storage-functions", 10)), (("sha256_64Kb", List("ByteVector"), 8), ("[SHA-256](https://en.wikipedia.org/wiki/SHA-2) hash function. For array with size <= 64Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 47)), (("getBooleanValue", List("Address|Alias", "String"), 8), ("Gets a boolean value by key. Throws an exception if there is no data.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 10)), (("calculateAssetId", List("Issue"), 8), ("Calculates ID of asset obtained by invoke script transaction's call of the Issue structure.", List("Structure of a token issue."), "blockchain-functions", 10)), (("invoke", List("Address|Alias", "String|Unit", "List[Any]", "List[AttachedPayment]"), 8), ("Invoke function from other dApp.", List("dApp", "function name", "arguments", "Attached payments"), "blockchain-functions", 75)), (("==", List("T", "T"), 8), ("Check equality.", List("First value", "Second value"), "operators", 1)), (("blake2b256_16Kb", List("ByteVector"), 8), ("[BLAKE-256](https://en.wikipedia.org/wiki/BLAKE_%28hash_function%29) hash function. For array with size <= 16Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 13)), ((">", List("Int", "Int"), 8), ("Check if integer greater comparison.", List("First value", "Second value"), "operators", 1)), (("getString", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "String"), 8), ("Gets a string value from a list of data entries by key", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), (("getIntegerValue", List("Address|Alias", "String"), 8), ("Gets an integer by key. Throws an exception if there is no data.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 10)), (("createMerkleRoot", List("List[ByteVector]", "ByteVector", "Int"), 8), ("Calculates the Merkle root hash for transactions of block.", List("Array of sibling hashes of the Merkle tree.", "Hash of transaction.", "Index of the transaction in the block."), "verification-functions", 30)), (("toBytes", List("Int"), 8), ("Converts an integer to an array of bytes.", List("The integer to convert."), "converting-functions", 1)), ((">", List("BigInt", "BigInt"), 8), ("Check if integer greater comparison.", List("First value", "Second value"), "operators", 1)), (("toInt", List("ByteVector", "Int"), 8), ("Converts an array of bytes to an integer starting from a certain index.", List("The array of bytes to convert.", "The index to start from."), "converting-functions", 1)), (("keccak256_128Kb", List("ByteVector"), 8), ("[Keccak-256](https://keccak.team/files/Keccak-submission-3.pdf) hash function. For array with size <= 128Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 147)), (("groth16Verify_10inputs", List("ByteVector", "ByteVector", "ByteVector"), 8), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 10 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 2100)), (("toUtf8String", List("ByteVector"), 8), ("Converts an array of bytes to a UTF-8 string.", List("The array of bytes to convert."), "converting-functions", 7)), (("*", List("BigInt", "BigInt"), 8), ("Multiply big integers.", List("Multiplier", "Multiplier"), "operators", 1)), (("Lease", List("Address|Alias", "Int", "Int"), 8), ("Lease action detailed constructor", List("Recipient", "Amount of Waves", "Nonce to distinguish"), "internal-functions", 1)), (("bn256Groth16Verify_5inputs", List("ByteVector", "ByteVector", "ByteVector"), 8), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 5 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1050)), (("isDataStorageUntouched", List("Address|Alias"), 8), ("Check that no data was stored on account.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account."), "account-data-storage-functions", 10)), (("groth16Verify_1inputs", List("ByteVector", "ByteVector", "ByteVector"), 8), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 1 input.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1200)), (("-", List("Int"), 8), ("Change integer sign.", List("Value"), "operators", 1)), (("bn256Groth16Verify_4inputs", List("ByteVector", "ByteVector", "ByteVector"), 8), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 4 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1000)), (("keccak256_32Kb", List("ByteVector"), 8), ("[Keccak-256](https://keccak.team/files/Keccak-submission-3.pdf) hash function. For array with size <= 32Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 39)), (("toBytes", List("String"), 8), ("Converts a string to an array of bytes.", List("The string to convert."), "converting-functions", 8)), (("%", List("BigInt", "BigInt"), 8), ("Calculate big modulo.", List("Divisible", "Divisor"), "operators", 1)), (("getBooleanValue", List("String"), 8), ("Gets a boolean value by key from state of current account. Throws an exception if there is no data.", List("The key."), "account-self-data-storage-functions", 10)), (("getInteger", List("Address|Alias", "String"), 8), ("Gets an integer by key.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 10)), (("replaceByIndex", List("List[T]", "Int", "T"), 8), ("Replaces the element at given index in the list and returns new list.", List("The list.", "The index.", "New element."), "list-functions", 4)), (("getElement", List("List[T]", "Int"), 8), ("Gets an element from a list by index.", List("The list.", "The index of the element."), "list-functions", 2)), (("+", List("BigInt", "BigInt"), 8), ("Sum big integers.", List("First value", "Second value"), "operators", 1)), (("fraction", List("BigInt", "BigInt", "BigInt", "Ceiling|Down|Floor|HalfEven|HalfUp"), 8), ("Multiply and division with unlimited intermediate representation", List("Multiplier", "Multiplier", "Divisor", "Rounding mode"), "math-functions", 1)), (("getStringValue", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "String"), 8), ("Find and extract data by key (fail on error)", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), (("groth16Verify_9inputs", List("ByteVector", "ByteVector", "ByteVector"), 8), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 9 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 2000)), (("rsaVerify_64Kb", List("Md5|NoAlg|Sha1|Sha224|Sha256|Sha3224|Sha3256|Sha3384|Sha3512|Sha384|Sha512", "ByteVector", "ByteVector", "ByteVector"), 8), ("Verifies an [RSA](https://en.wikipedia.org/wiki/RSA_%28cryptosystem%29) signature. For message size <= 64Kb.", List("The RSA algorithm.", "The message.", "The signature.", "The public key."), "verification-functions", 625)), (("parseIntValue", List("String"), 8), ("Converts the string representation of a number to its integer equivalent.\nRaises an exception if the string cannot be parsed.", List("The string to parse."), "converting-functions", 2)), (("toString", List("Int"), 8), ("Converts an integer to a string.", List("The integer to convert."), "converting-functions", 1)), ((">=", List("Int", "Int"), 8), ("Check if integer greater or equal comparison.", List("First value", "Second value"), "operators", 1)), (("split_4C", List("String", "String"), 8), ("Splits a string delimited by a separator into a list of substrings. Input string size limit = 6000 bytes. Output list size limit = 100", List("The string.", "The separator."), "string-functions", 4)), (("bn256Groth16Verify_15inputs", List("ByteVector", "ByteVector", "ByteVector"), 8), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 15 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1600)), (("scriptHash", List("Address|Alias"), 8), ("blake2b hash of account's script", List("account"), "blockchain-functions", 200)), (("keccak256_64Kb", List("ByteVector"), 8), ("[Keccak-256](https://keccak.team/files/Keccak-submission-3.pdf) hash function. For array with size <= 64Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 74)), (("containsElement", List("List[T]", "T"), 8), ("Tests whether the list contains a given value as an element", List("The list.", "The element."), "list-functions", 5)), (("addressFromStringValue", List("String"), 8), ("Decodes address from [Base58](https://en.wikipedia.org/wiki/Base58) string.\nRaises an exception if the address cannot be decoded.", List("The string to decode."), "encoding-and-decoding-functions", 1)), (("getIntegerValue", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "Int"), 8), ("Extract data by index (fail on error)", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 4)), (("pow", List("BigInt", "Int", "BigInt", "Int", "Int", "Ceiling|Down|Floor|HalfEven|HalfUp"), 8), ("Returns a number raised to a power", List("The base.", "The number of decimals of the base.", "The exponent.", "The number of decimals of the exponent.", "The number of decimals of the resulting value.", "One of the rounding functions."), "math-functions", 270)), (("throw", List(), 8), ("Raises an exception.", List(), "exception-functions", 1)), (("getBinary", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "Int"), 8), ("Gets a binary value from a list of data entries by index", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 4)), (("median", List("List[Int]"), 8), ("Returns the median of the list.", List("The list of integers."), "list-functions", 20)), (("-", List("BigInt", "BigInt"), 8), ("Subtract big integers.", List("First value", "Second value"), "operators", 1)), (("indexOf", List("List[T]", "T"), 8), ("Returns the index of the first occurrence of given value", List("The list.", "The element."), "list-functions", 5)), (("take", List("ByteVector", "Int"), 8), ("Takes the first n bytes from an array of bytes.", List("The array of bytes.", "The number n."), "byte-array-functions", 6)), (("toBigInt", List("ByteVector", "Int", "Int"), 8), ("Converts an array of bytes to an big integer.", List("The array of bytes to convert.", "Offset", "Size"), "converting-functions", 1)), (("groth16Verify_2inputs", List("ByteVector", "ByteVector", "ByteVector"), 8), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 2 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1300)), (("getStringValue", List("String"), 8), ("Gets a string by key from state of current account. Throws an exception if there is no data.", List("The key."), "account-self-data-storage-functions", 10)), (("sigVerify_128Kb", List("ByteVector", "ByteVector", "ByteVector"), 8), ("Verifies a [Curve25519](https://en.wikipedia.org/wiki/Curve25519) signature. For message size <= 128Kb.", List("The message.", "The signature.", "The account public key."), "verification-functions", 150)), (("getIntegerValue", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "String"), 8), ("Find and extract data by key (fail on error)", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), (("calculateDelay", List("Address", "Int"), 8), ("Calculates mining delay using Fair PoS calculator.", List("generator address", "generator balance"), "blockchain-functions", 1)), (("+", List("ByteVector", "ByteVector"), 8), ("Concat limited byte vectors.", List("First value", "Second value"), "operators", 2)), (("indexOf", List("String", "String"), 8), ("Returns the index of the first occurrence of a substring", List("The string.", "The substring."), "string-functions", 3)), (("Lease", List("Address|Alias", "Int"), 8), ("Lease action simplified constructor", List("Recipient", "Amount of Waves"), "internal-functions", 1)), (("groth16Verify_15inputs", List("ByteVector", "ByteVector", "ByteVector"), 8), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 15 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 2600)), (("toBase58String", List("ByteVector"), 8), ("Encodes array of bytes to [Base58](https://en.wikipedia.org/wiki/Base58) string.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 3)), (("groth16Verify_11inputs", List("ByteVector", "ByteVector", "ByteVector"), 8), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 11 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 2200)), (("contains", List("String", "String"), 8), ("Checks whether the string contains substring", List("String to search in.", "String to search for."), "string-functions", 3)), (("!=", List("T", "T"), 8), ("Check inequality.", List("First value", "Second value"), "operators", 1)), (("%", List("Int", "Int"), 8), ("Calculate modulo.", List("Divisible", "Divisor"), "operators", 1)), (("bn256Groth16Verify_11inputs", List("ByteVector", "ByteVector", "ByteVector"), 8), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 11 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1350)), (("groth16Verify_12inputs", List("ByteVector", "ByteVector", "ByteVector"), 8), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 12 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 2300)), (("fraction", List("Int", "Int", "Int", "Ceiling|Down|Floor|HalfEven|HalfUp"), 8), ("Multiply and division with big integer intermediate representation", List("Multiplier", "Multiplier", "Divisor", "Rounding mode"), "math-functions", 1)), (("log", List("BigInt", "Int", "BigInt", "Int", "Int", "Ceiling|Down|Floor|HalfEven|HalfUp"), 8), ("Returns the logarithm of a number.", List("The base.", "The number of decimals of the base.", "The exponent.", "The number of decimals of the exponent.", "The number of decimals of the resulting value.", "The rounding function.\n"), "math-functions", 200)), (("addressFromString", List("String"), 8), ("Decodes address from [Base58](https://en.wikipedia.org/wiki/Base58) string.", List("The string to decode."), "encoding-and-decoding-functions", 1)), (("toString", List("BigInt"), 8), ("Convert BigInt to string", List("The BigInt to convert"), "converting-functions", 1)), (("sha256_16Kb", List("ByteVector"), 8), ("[SHA-256](https://en.wikipedia.org/wiki/SHA-2) hash function. For array with size <= 16Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 12)), (("getBinaryValue", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "Int"), 8), ("Extract data by index (fail on error)", List("DataEntry list, usually tx.data", "index"), "extracting-data-functions", 4)), (("valueOrElse", List("T|Unit", "T"), 8), ("Returns value from union type argument if it's not unit. Otherwise, returns the second argument.", List("The argument to return value from.", "Returned if the value of t is unit."), "optional-value-functions", 2)), (("sha256_128Kb", List("ByteVector"), 8), ("[SHA-256](https://en.wikipedia.org/wiki/SHA-2) hash function. For array with size <= 128Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 93)), (("bn256Groth16Verify_10inputs", List("ByteVector", "ByteVector", "ByteVector"), 8), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 10 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1300)), (("toInt", List("ByteVector"), 8), ("Converts an array of bytes to an integer.", List("The array of bytes to convert."), "converting-functions", 1)), (("cons", List("A", "List[B]"), 8), ("Prepends a new element to the list.", List("The element.", "The list."), "list-functions", 1)), (("groth16Verify_3inputs", List("ByteVector", "ByteVector", "ByteVector"), 8), ("[Zero-knowledge proof](https://en.wikipedia.org/wiki/Zero-knowledge_proof) verifier function. With 3 inputs.", List("Verifying key.", "Proofs.", "Inputs."), "verification-functions", 1400)), (("transferTransactionById", List("ByteVector"), 8), ("Gets the data of a transfer transaction.", List("ID of the transfer transaction."), "blockchain-functions", 60)), (("getBinary", List("List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]", "String"), 8), ("Gets a binary value from a list of data entries by key", List("DataEntry list, usually tx.data", "key"), "extracting-data-functions", 10)), (("size", List("ByteVector"), 8), ("Returns the size of an array of bytes.", List("The array of bytes."), "byte-array-functions", 1)), (("transferTransactionFromProto", List("ByteVector"), 8), ("Deserializes transfer transaction: converts protobuf-encoded binary format to a TransferTransaction structure.", List("Transfer transaction in protobuf-encoded binary format."), "blockchain-functions", 5)), (("blake2b256_32Kb", List("ByteVector"), 8), ("[BLAKE-256](https://en.wikipedia.org/wiki/BLAKE_%28hash_function%29) hash function. For array with size <= 32Kb.", List("The array of bytes to encode."), "encoding-and-decoding-functions", 29)), (("++", List("List[A]", "List[B]"), 8), ("List Concatenation.", List("First list", "Second list"), "operators", 4)), (("assetInfo", List("ByteVector"), 8), ("Gets the information about a [token](/blockchain/token.md).", List("ID of the [token](/blockchain/token.md)."), "blockchain-functions", 15)), (("getString", List("Address|Alias", "String"), 8), ("Gets a string by key.", List("[Address](/blockchain/address.md) or [alias](/blockchain/alias.md) of the account.", "The key."), "account-data-storage-functions", 10)), (("sigVerify", List("ByteVector", "ByteVector", "ByteVector"), 8), ("Verifies a [Curve25519](https://en.wikipedia.org/wiki/Curve25519) signature.", List("The message.", "The signature.", "The account public key."), "verification-functions", 180)))
lazy val varsV3 = Map((("this", 3), ("Script address")), (("HALFDOWN", 3), ("'HALF_DOWN' rounding mode")), (("SHA224", 3), ("SHA224 digest algorithm")), (("SHA3256", 3), ("SHA3-256 digest algorithm")), (("Sell", 3), ("Sell OrderType")), (("SHA3224", 3), ("SHA3-224 digest algorithm")), (("MD5", 3), ("MD5 digest algorithm")), (("NOALG", 3), ("NONE digest algorithm")), (("SHA3512", 3), ("SHA3-512 digest algorithm")), (("SHA1", 3), ("SHA1 digest algorithm")), (("Buy", 3), ("Buy OrderType")), (("height", 3), ("Current blockchain height")), (("SHA256", 3), ("SHA256 digest algorithm")), (("DOWN", 3), ("'DOWN' rounding mode")), (("FLOOR", 3), ("'FLOOR' rounding mode")), (("SHA3384", 3), ("SHA3-384 digest algorithm")), (("CEILING", 3), ("'CEILING' rounding mode")), (("tx", 3), ("Processing transaction")), (("HALFUP", 3), ("'HALF_UP' rounding mode")), (("SHA384", 3), ("SHA384 digest algorithm")), (("UP", 3), ("'UP' rounding mode")), (("lastBlock", 3), ("Last block info")), (("nil", 3), ("empty list of any type")), (("SHA512", 3), ("SHA512 digest algorithm")), (("HALFEVEN", 3), ("'HALF_EVEN' rounding mode")), (("unit", 3), ("Single instance value")))
lazy val varsV4 = Map((("SHA512", 4), ("SHA512 digest algorithm")), (("this", 4), ("Script address")), (("SHA256", 4), ("SHA256 digest algorithm")), (("height", 4), ("Current blockchain height")), (("tx", 4), ("Processing transaction")), (("SHA3256", 4), ("SHA3-256 digest algorithm")), (("FLOOR", 4), ("'FLOOR' rounding mode")), (("CEILING", 4), ("'CEILING' rounding mode")), (("nil", 4), ("empty list of any type")), (("HALFUP", 4), ("'HALF_UP' rounding mode")), (("NOALG", 4), ("NONE digest algorithm")), (("SHA1", 4), ("SHA1 digest algorithm")), (("UP", 4), ("'UP' rounding mode")), (("HALFDOWN", 4), ("'HALF_DOWN' rounding mode")), (("HALFEVEN", 4), ("'HALF_EVEN' rounding mode")), (("Buy", 4), ("Buy OrderType")), (("SHA3512", 4), ("SHA3-512 digest algorithm")), (("SHA384", 4), ("SHA384 digest algorithm")), (("unit", 4), ("Single instance value")), (("SHA224", 4), ("SHA224 digest algorithm")), (("SHA3224", 4), ("SHA3-224 digest algorithm")), (("SHA3384", 4), ("SHA3-384 digest algorithm")), (("MD5", 4), ("MD5 digest algorithm")), (("Sell", 4), ("Sell OrderType")), (("DOWN", 4), ("'DOWN' rounding mode")), (("lastBlock", 4), ("Last block info")))
lazy val varsV5 = Map((("MD5", 5), ("MD5 digest algorithm")), (("SHA1", 5), ("SHA1 digest algorithm")), (("nil", 5), ("empty list of any type")), (("HALFEVEN", 5), ("'HALF_EVEN' rounding mode")), (("SHA3256", 5), ("SHA3-256 digest algorithm")), (("HALFUP", 5), ("'HALF_UP' rounding mode")), (("SHA384", 5), ("SHA384 digest algorithm")), (("DOWN", 5), ("'DOWN' rounding mode")), (("SHA3512", 5), ("SHA3-512 digest algorithm")), (("unit", 5), ("Single instance value")), (("FLOOR", 5), ("'FLOOR' rounding mode")), (("this", 5), ("Script address")), (("SHA3224", 5), ("SHA3-224 digest algorithm")), (("tx", 5), ("Processing transaction")), (("Sell", 5), ("Sell OrderType")), (("height", 5), ("Current blockchain height")), (("lastBlock", 5), ("Last block info")), (("NOALG", 5), ("NONE digest algorithm")), (("SHA224", 5), ("SHA224 digest algorithm")), (("SHA3384", 5), ("SHA3-384 digest algorithm")), (("CEILING", 5), ("'CEILING' rounding mode")), (("SHA512", 5), ("SHA512 digest algorithm")), (("SHA256", 5), ("SHA256 digest algorithm")), (("Buy", 5), ("Buy OrderType")))
lazy val varsV6 = Map((("DOWN", 6), ("'DOWN' rounding mode")), (("HALFUP", 6), ("'HALF_UP' rounding mode")), (("tx", 6), ("Processing transaction")), (("SHA224", 6), ("SHA224 digest algorithm")), (("SHA3224", 6), ("SHA3-224 digest algorithm")), (("SHA3512", 6), ("SHA3-512 digest algorithm")), (("this", 6), ("Script address")), (("unit", 6), ("Single instance value")), (("nil", 6), ("empty list of any type")), (("SHA1", 6), ("SHA1 digest algorithm")), (("HALFEVEN", 6), ("'HALF_EVEN' rounding mode")), (("lastBlock", 6), ("Last block info")), (("SHA3256", 6), ("SHA3-256 digest algorithm")), (("MD5", 6), ("MD5 digest algorithm")), (("SHA512", 6), ("SHA512 digest algorithm")), (("NOALG", 6), ("NONE digest algorithm")), (("Sell", 6), ("Sell OrderType")), (("SHA3384", 6), ("SHA3-384 digest algorithm")), (("SHA256", 6), ("SHA256 digest algorithm")), (("SHA384", 6), ("SHA384 digest algorithm")), (("FLOOR", 6), ("'FLOOR' rounding mode")), (("Buy", 6), ("Buy OrderType")), (("CEILING", 6), ("'CEILING' rounding mode")), (("height", 6), ("Current blockchain height")))
lazy val varsV7 = Map((("SHA3384", 7), ("SHA3-384 digest algorithm")), (("MD5", 7), ("MD5 digest algorithm")), (("SHA512", 7), ("SHA512 digest algorithm")), (("FLOOR", 7), ("'FLOOR' rounding mode")), (("SHA256", 7), ("SHA256 digest algorithm")), (("Buy", 7), ("Buy OrderType")), (("tx", 7), ("Processing transaction")), (("SHA3224", 7), ("SHA3-224 digest algorithm")), (("SHA3256", 7), ("SHA3-256 digest algorithm")), (("nil", 7), ("empty list of any type")), (("Sell", 7), ("Sell OrderType")), (("HALFEVEN", 7), ("'HALF_EVEN' rounding mode")), (("SHA384", 7), ("SHA384 digest algorithm")), (("SHA1", 7), ("SHA1 digest algorithm")), (("unit", 7), ("Single instance value")), (("height", 7), ("Current blockchain height")), (("SHA3512", 7), ("SHA3-512 digest algorithm")), (("this", 7), ("Script address")), (("HALFUP", 7), ("'HALF_UP' rounding mode")), (("NOALG", 7), ("NONE digest algorithm")), (("DOWN", 7), ("'DOWN' rounding mode")), (("CEILING", 7), ("'CEILING' rounding mode")), (("lastBlock", 7), ("Last block info")), (("SHA224", 7), ("SHA224 digest algorithm")))
lazy val varsV8 = Map((("FLOOR", 8), ("'FLOOR' rounding mode")), (("unit", 8), ("Single instance value")), (("CEILING", 8), ("'CEILING' rounding mode")), (("HALFEVEN", 8), ("'HALF_EVEN' rounding mode")), (("nil", 8), ("empty list of any type")), (("lastBlock", 8), ("Last block info")), (("SHA3384", 8), ("SHA3-384 digest algorithm")), (("SHA3224", 8), ("SHA3-224 digest algorithm")), (("SHA512", 8), ("SHA512 digest algorithm")), (("NOALG", 8), ("NONE digest algorithm")), (("SHA1", 8), ("SHA1 digest algorithm")), (("SHA224", 8), ("SHA224 digest algorithm")), (("Sell", 8), ("Sell OrderType")), (("MD5", 8), ("MD5 digest algorithm")), (("tx", 8), ("Processing transaction")), (("Buy", 8), ("Buy OrderType")), (("SHA384", 8), ("SHA384 digest algorithm")), (("SHA3256", 8), ("SHA3-256 digest algorithm")), (("height", 8), ("Current blockchain height")), (("SHA256", 8), ("SHA256 digest algorithm")), (("this", 8), ("Script address")), (("SHA3512", 8), ("SHA3-512 digest algorithm")), (("DOWN", 8), ("'DOWN' rounding mode")), (("HALFUP", 8), ("'HALF_UP' rounding mode")))
lazy val typeData = Map((("Address", 1), (List(("bytes", "ByteVector")))), (("MassTransferTransaction", 1), (List(("feeAssetId", "ByteVector|Unit"), ("assetId", "ByteVector|Unit"), ("totalAmount", "Int"), ("transfers", "List[Transfer]"), ("transferCount", "Int"), ("attachment", "ByteVector"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("BurnTransaction", 1), (List(("quantity", "Int"), ("assetId", "ByteVector"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("SponsorFeeTransaction", 1), (List(("assetId", "ByteVector"), ("minSponsoredAssetFee", "Int|Unit"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("ExchangeTransaction", 1), (List(("buyOrder", "Order"), ("sellOrder", "Order"), ("price", "Int"), ("amount", "Int"), ("buyMatcherFee", "Int"), ("sellMatcherFee", "Int"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Order", 1), (List(("id", "ByteVector"), ("matcherPublicKey", "ByteVector"), ("assetPair", "AssetPair"), ("orderType", "Buy|Sell"), ("price", "Int"), ("amount", "Int"), ("timestamp", "Int"), ("expiration", "Int"), ("matcherFee", "Int"), ("matcherFeeAssetId", "ByteVector|Unit"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("SetAssetScriptTransaction", 1), (List(("script", "ByteVector|Unit"), ("assetId", "ByteVector"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("IssueTransaction", 1), (List(("quantity", "Int"), ("name", "ByteVector"), ("description", "ByteVector"), ("reissuable", "Boolean"), ("decimals", "Int"), ("script", "ByteVector|Unit"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("AssetPair", 1), (List(("amountAsset", "ByteVector|Unit"), ("priceAsset", "ByteVector|Unit")))), (("Alias", 1), (List(("alias", "String")))), (("DataEntry", 1), (List(("key", "String"), ("value", "Boolean|ByteVector|Int|String")))), (("HalfDown", 1), (List())), (("Floor", 1), (List())), (("CreateAliasTransaction", 1), (List(("alias", "String"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("GenesisTransaction", 1), (List(("amount", "Int"), ("recipient", "Address|Alias"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int")))), (("PaymentTransaction", 1), (List(("amount", "Int"), ("recipient", "Address|Alias"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Transfer", 1), (List(("recipient", "Address|Alias"), ("amount", "Int")))), (("HalfUp", 1), (List())), (("Down", 1), (List())), (("Ceiling", 1), (List())), (("Up", 1), (List())), (("TransferTransaction", 1), (List(("feeAssetId", "ByteVector|Unit"), ("amount", "Int"), ("assetId", "ByteVector|Unit"), ("recipient", "Address|Alias"), ("attachment", "ByteVector"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("SetScriptTransaction", 1), (List(("script", "ByteVector|Unit"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Unit", 1), (List())), (("ReissueTransaction", 1), (List(("quantity", "Int"), ("assetId", "ByteVector"), ("reissuable", "Boolean"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("LeaseTransaction", 1), (List(("amount", "Int"), ("recipient", "Address|Alias"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("LeaseCancelTransaction", 1), (List(("leaseId", "ByteVector"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("HalfEven", 1), (List())), (("DataTransaction", 1), (List(("data", "List[DataEntry]"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]"))))) ++ Map((("HalfUp", 2), (List())), (("AssetPair", 2), (List(("amountAsset", "ByteVector|Unit"), ("priceAsset", "ByteVector|Unit")))), (("ExchangeTransaction", 2), (List(("buyOrder", "Order"), ("sellOrder", "Order"), ("price", "Int"), ("amount", "Int"), ("buyMatcherFee", "Int"), ("sellMatcherFee", "Int"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Order", 2), (List(("id", "ByteVector"), ("matcherPublicKey", "ByteVector"), ("assetPair", "AssetPair"), ("orderType", "Buy|Sell"), ("price", "Int"), ("amount", "Int"), ("timestamp", "Int"), ("expiration", "Int"), ("matcherFee", "Int"), ("matcherFeeAssetId", "ByteVector|Unit"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("MassTransferTransaction", 2), (List(("feeAssetId", "ByteVector|Unit"), ("assetId", "ByteVector|Unit"), ("totalAmount", "Int"), ("transfers", "List[Transfer]"), ("transferCount", "Int"), ("attachment", "ByteVector"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("SponsorFeeTransaction", 2), (List(("assetId", "ByteVector"), ("minSponsoredAssetFee", "Int|Unit"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("DataEntry", 2), (List(("key", "String"), ("value", "Boolean|ByteVector|Int|String")))), (("BurnTransaction", 2), (List(("quantity", "Int"), ("assetId", "ByteVector"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("TransferTransaction", 2), (List(("feeAssetId", "ByteVector|Unit"), ("amount", "Int"), ("assetId", "ByteVector|Unit"), ("recipient", "Address|Alias"), ("attachment", "ByteVector"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Unit", 2), (List())), (("HalfDown", 2), (List())), (("HalfEven", 2), (List())), (("LeaseTransaction", 2), (List(("amount", "Int"), ("recipient", "Address|Alias"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("SetAssetScriptTransaction", 2), (List(("script", "ByteVector|Unit"), ("assetId", "ByteVector"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("PaymentTransaction", 2), (List(("amount", "Int"), ("recipient", "Address|Alias"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("CreateAliasTransaction", 2), (List(("alias", "String"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("SetScriptTransaction", 2), (List(("script", "ByteVector|Unit"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Address", 2), (List(("bytes", "ByteVector")))), (("Transfer", 2), (List(("recipient", "Address|Alias"), ("amount", "Int")))), (("LeaseCancelTransaction", 2), (List(("leaseId", "ByteVector"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Ceiling", 2), (List())), (("GenesisTransaction", 2), (List(("amount", "Int"), ("recipient", "Address|Alias"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int")))), (("ReissueTransaction", 2), (List(("quantity", "Int"), ("assetId", "ByteVector"), ("reissuable", "Boolean"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Up", 2), (List())), (("Floor", 2), (List())), (("Down", 2), (List())), (("DataTransaction", 2), (List(("data", "List[DataEntry]"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Alias", 2), (List(("alias", "String")))), (("IssueTransaction", 2), (List(("quantity", "Int"), ("name", "ByteVector"), ("description", "ByteVector"), ("reissuable", "Boolean"), ("decimals", "Int"), ("script", "ByteVector|Unit"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]"))))) ++ Map((("Unit", 3), (List())), (("Floor", 3), (List())), (("Sha224", 3), (List())), (("BurnTransaction", 3), (List(("quantity", "Int"), ("assetId", "ByteVector"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("TransferSet", 3), (List(("transfers", "List[ScriptTransfer]")))), (("Sha1", 3), (List())), (("NoAlg", 3), (List())), (("ExchangeTransaction", 3), (List(("buyOrder", "Order"), ("sellOrder", "Order"), ("price", "Int"), ("amount", "Int"), ("buyMatcherFee", "Int"), ("sellMatcherFee", "Int"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("HalfUp", 3), (List())), (("ScriptResult", 3), (List(("writeSet", "WriteSet"), ("transferSet", "TransferSet")))), (("Address", 3), (List(("bytes", "ByteVector")))), (("GenesisTransaction", 3), (List(("amount", "Int"), ("recipient", "Address|Alias"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int")))), (("LeaseTransaction", 3), (List(("amount", "Int"), ("recipient", "Address|Alias"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Invocation", 3), (List(("payment", "AttachedPayment|Unit"), ("caller", "Address"), ("callerPublicKey", "ByteVector"), ("transactionId", "ByteVector"), ("fee", "Int"), ("feeAssetId", "ByteVector|Unit")))), (("SponsorFeeTransaction", 3), (List(("assetId", "ByteVector"), ("minSponsoredAssetFee", "Int|Unit"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("ScriptTransfer", 3), (List(("recipient", "Address|Alias"), ("amount", "Int"), ("asset", "ByteVector|Unit")))), (("MassTransferTransaction", 3), (List(("feeAssetId", "ByteVector|Unit"), ("assetId", "ByteVector|Unit"), ("totalAmount", "Int"), ("transfers", "List[Transfer]"), ("transferCount", "Int"), ("attachment", "ByteVector"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("AttachedPayment", 3), (List(("assetId", "ByteVector|Unit"), ("amount", "Int")))), (("Sha512", 3), (List())), (("Sha256", 3), (List())), (("Transfer", 3), (List(("recipient", "Address|Alias"), ("amount", "Int")))), (("Alias", 3), (List(("alias", "String")))), (("HalfEven", 3), (List())), (("BlockInfo", 3), (List(("timestamp", "Int"), ("height", "Int"), ("baseTarget", "Int"), ("generationSignature", "ByteVector"), ("generator", "Address"), ("generatorPublicKey", "ByteVector")))), (("IssueTransaction", 3), (List(("quantity", "Int"), ("name", "ByteVector"), ("description", "ByteVector"), ("reissuable", "Boolean"), ("decimals", "Int"), ("script", "ByteVector|Unit"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Ceiling", 3), (List())), (("Asset", 3), (List(("id", "ByteVector"), ("quantity", "Int"), ("decimals", "Int"), ("issuer", "Address"), ("issuerPublicKey", "ByteVector"), ("reissuable", "Boolean"), ("scripted", "Boolean"), ("sponsored", "Boolean")))), (("CreateAliasTransaction", 3), (List(("alias", "String"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Sha3384", 3), (List())), (("Md5", 3), (List())), (("Order", 3), (List(("id", "ByteVector"), ("matcherPublicKey", "ByteVector"), ("assetPair", "AssetPair"), ("orderType", "Buy|Sell"), ("price", "Int"), ("amount", "Int"), ("timestamp", "Int"), ("expiration", "Int"), ("matcherFee", "Int"), ("matcherFeeAssetId", "ByteVector|Unit"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("DataEntry", 3), (List(("key", "String"), ("value", "Boolean|ByteVector|Int|String")))), (("Sha3224", 3), (List())), (("InvokeScriptTransaction", 3), (List(("dApp", "Address|Alias"), ("feeAssetId", "ByteVector|Unit"), ("function", "String"), ("args", "List[Boolean|ByteVector|Int|List[Boolean|ByteVector|Int|String]|String]"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("payment", "AttachedPayment|Unit"), ("proofs", "List[ByteVector]")))), (("HalfDown", 3), (List())), (("ReissueTransaction", 3), (List(("quantity", "Int"), ("assetId", "ByteVector"), ("reissuable", "Boolean"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Up", 3), (List())), (("Down", 3), (List())), (("WriteSet", 3), (List(("data", "List[DataEntry]")))), (("SetScriptTransaction", 3), (List(("script", "ByteVector|Unit"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Sha3512", 3), (List())), (("LeaseCancelTransaction", 3), (List(("leaseId", "ByteVector"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("DataTransaction", 3), (List(("data", "List[DataEntry]"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("TransferTransaction", 3), (List(("feeAssetId", "ByteVector|Unit"), ("amount", "Int"), ("assetId", "ByteVector|Unit"), ("recipient", "Address|Alias"), ("attachment", "ByteVector"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Sha3256", 3), (List())), (("Sha384", 3), (List())), (("AssetPair", 3), (List(("amountAsset", "ByteVector|Unit"), ("priceAsset", "ByteVector|Unit")))), (("SetAssetScriptTransaction", 3), (List(("script", "ByteVector|Unit"), ("assetId", "ByteVector"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("PaymentTransaction", 3), (List(("amount", "Int"), ("recipient", "Address|Alias"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]"))))) ++ Map((("ScriptTransfer", 4), (List(("recipient", "Address|Alias"), ("amount", "Int"), ("asset", "ByteVector|Unit")))), (("HalfUp", 4), (List())), (("Sha256", 4), (List())), (("Ceiling", 4), (List())), (("Reissue", 4), (List(("assetId", "ByteVector"), ("quantity", "Int"), ("isReissuable", "Boolean")))), (("AssetPair", 4), (List(("amountAsset", "ByteVector|Unit"), ("priceAsset", "ByteVector|Unit")))), (("Floor", 4), (List())), (("TransferTransaction", 4), (List(("feeAssetId", "ByteVector|Unit"), ("amount", "Int"), ("assetId", "ByteVector|Unit"), ("recipient", "Address|Alias"), ("attachment", "ByteVector"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Invocation", 4), (List(("payments", "List[AttachedPayment]"), ("caller", "Address"), ("callerPublicKey", "ByteVector"), ("transactionId", "ByteVector"), ("fee", "Int"), ("feeAssetId", "ByteVector|Unit")))), (("CreateAliasTransaction", 4), (List(("alias", "String"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Transfer", 4), (List(("recipient", "Address|Alias"), ("amount", "Int")))), (("Order", 4), (List(("id", "ByteVector"), ("matcherPublicKey", "ByteVector"), ("assetPair", "AssetPair"), ("orderType", "Buy|Sell"), ("price", "Int"), ("amount", "Int"), ("timestamp", "Int"), ("expiration", "Int"), ("matcherFee", "Int"), ("matcherFeeAssetId", "ByteVector|Unit"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Alias", 4), (List(("alias", "String")))), (("Sha512", 4), (List())), (("Sha3224", 4), (List())), (("MassTransferTransaction", 4), (List(("feeAssetId", "ByteVector|Unit"), ("assetId", "ByteVector|Unit"), ("totalAmount", "Int"), ("transfers", "List[Transfer]"), ("transferCount", "Int"), ("attachment", "ByteVector"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Md5", 4), (List())), (("BlockInfo", 4), (List(("timestamp", "Int"), ("height", "Int"), ("baseTarget", "Int"), ("generationSignature", "ByteVector"), ("generator", "Address"), ("generatorPublicKey", "ByteVector"), ("vrf", "ByteVector|Unit")))), (("UpdateAssetInfoTransaction", 4), (List(("assetId", "ByteVector"), ("name", "String"), ("description", "String"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Down", 4), (List())), (("InvokeScriptTransaction", 4), (List(("dApp", "Address|Alias"), ("feeAssetId", "ByteVector|Unit"), ("function", "String"), ("args", "List[Boolean|ByteVector|Int|List[Boolean|ByteVector|Int|String]|String]"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("payments", "List[AttachedPayment]"), ("proofs", "List[ByteVector]")))), (("PaymentTransaction", 4), (List(("amount", "Int"), ("recipient", "Address|Alias"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("StringEntry", 4), (List(("key", "String"), ("value", "String")))), (("BalanceDetails", 4), (List(("available", "Int"), ("regular", "Int"), ("generating", "Int"), ("effective", "Int")))), (("NoAlg", 4), (List())), (("Unit", 4), (List())), (("DeleteEntry", 4), (List(("key", "String")))), (("Up", 4), (List())), (("Sha224", 4), (List())), (("IntegerEntry", 4), (List(("key", "String"), ("value", "Int")))), (("Address", 4), (List(("bytes", "ByteVector")))), (("BurnTransaction", 4), (List(("quantity", "Int"), ("assetId", "ByteVector"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("AttachedPayment", 4), (List(("assetId", "ByteVector|Unit"), ("amount", "Int")))), (("BinaryEntry", 4), (List(("key", "String"), ("value", "ByteVector")))), (("SetScriptTransaction", 4), (List(("script", "ByteVector|Unit"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Sha3512", 4), (List())), (("ReissueTransaction", 4), (List(("quantity", "Int"), ("assetId", "ByteVector"), ("reissuable", "Boolean"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("SponsorFeeTransaction", 4), (List(("assetId", "ByteVector"), ("minSponsoredAssetFee", "Int|Unit"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Sha384", 4), (List())), (("IssueTransaction", 4), (List(("quantity", "Int"), ("name", "String"), ("description", "String"), ("reissuable", "Boolean"), ("decimals", "Int"), ("script", "ByteVector|Unit"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Sha1", 4), (List())), (("GenesisTransaction", 4), (List(("amount", "Int"), ("recipient", "Address|Alias"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int")))), (("Burn", 4), (List(("assetId", "ByteVector"), ("quantity", "Int")))), (("Sha3384", 4), (List())), (("Issue", 4), (List(("name", "String"), ("description", "String"), ("quantity", "Int"), ("decimals", "Int"), ("isReissuable", "Boolean"), ("compiledScript", "Script|Unit"), ("nonce", "Int")))), (("DataTransaction", 4), (List(("data", "List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("BooleanEntry", 4), (List(("key", "String"), ("value", "Boolean")))), (("HalfEven", 4), (List())), (("HalfDown", 4), (List())), (("ExchangeTransaction", 4), (List(("buyOrder", "Order"), ("sellOrder", "Order"), ("price", "Int"), ("amount", "Int"), ("buyMatcherFee", "Int"), ("sellMatcherFee", "Int"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("LeaseTransaction", 4), (List(("amount", "Int"), ("recipient", "Address|Alias"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("SetAssetScriptTransaction", 4), (List(("script", "ByteVector|Unit"), ("assetId", "ByteVector"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("SponsorFee", 4), (List(("assetId", "ByteVector"), ("minSponsoredAssetFee", "Int|Unit")))), (("Asset", 4), (List(("id", "ByteVector"), ("quantity", "Int"), ("decimals", "Int"), ("issuer", "Address"), ("issuerPublicKey", "ByteVector"), ("reissuable", "Boolean"), ("scripted", "Boolean"), ("minSponsoredFee", "Int|Unit"), ("name", "String"), ("description", "String")))), (("LeaseCancelTransaction", 4), (List(("leaseId", "ByteVector"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Sha3256", 4), (List()))) ++ Map((("UpdateAssetInfoTransaction", 5), (List(("assetId", "ByteVector"), ("name", "String"), ("description", "String"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Sha512", 5), (List())), (("LeaseCancel", 5), (List(("leaseId", "ByteVector")))), (("ExchangeTransaction", 5), (List(("buyOrder", "Order"), ("sellOrder", "Order"), ("price", "Int"), ("amount", "Int"), ("buyMatcherFee", "Int"), ("sellMatcherFee", "Int"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Issue", 5), (List(("name", "String"), ("description", "String"), ("quantity", "Int"), ("decimals", "Int"), ("isReissuable", "Boolean"), ("compiledScript", "Script|Unit"), ("nonce", "Int")))), (("Md5", 5), (List())), (("DeleteEntry", 5), (List(("key", "String")))), (("Address", 5), (List(("bytes", "ByteVector")))), (("BlockInfo", 5), (List(("timestamp", "Int"), ("height", "Int"), ("baseTarget", "Int"), ("generationSignature", "ByteVector"), ("generator", "Address"), ("generatorPublicKey", "ByteVector"), ("vrf", "ByteVector|Unit")))), (("IssueTransaction", 5), (List(("quantity", "Int"), ("name", "String"), ("description", "String"), ("reissuable", "Boolean"), ("decimals", "Int"), ("script", "ByteVector|Unit"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("TransferTransaction", 5), (List(("feeAssetId", "ByteVector|Unit"), ("amount", "Int"), ("assetId", "ByteVector|Unit"), ("recipient", "Address|Alias"), ("attachment", "ByteVector"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("SponsorFee", 5), (List(("assetId", "ByteVector"), ("minSponsoredAssetFee", "Int|Unit")))), (("Invocation", 5), (List(("payments", "List[AttachedPayment]"), ("caller", "Address"), ("callerPublicKey", "ByteVector"), ("transactionId", "ByteVector"), ("fee", "Int"), ("feeAssetId", "ByteVector|Unit"), ("originCaller", "Address"), ("originCallerPublicKey", "ByteVector")))), (("Sha3224", 5), (List())), (("Sha3384", 5), (List())), (("SetAssetScriptTransaction", 5), (List(("script", "ByteVector|Unit"), ("assetId", "ByteVector"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("AttachedPayment", 5), (List(("assetId", "ByteVector|Unit"), ("amount", "Int")))), (("StringEntry", 5), (List(("key", "String"), ("value", "String")))), (("Ceiling", 5), (List())), (("LeaseTransaction", 5), (List(("amount", "Int"), ("recipient", "Address|Alias"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("LeaseCancelTransaction", 5), (List(("leaseId", "ByteVector"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Unit", 5), (List())), (("BalanceDetails", 5), (List(("available", "Int"), ("regular", "Int"), ("generating", "Int"), ("effective", "Int")))), (("Asset", 5), (List(("id", "ByteVector"), ("quantity", "Int"), ("decimals", "Int"), ("issuer", "Address"), ("issuerPublicKey", "ByteVector"), ("reissuable", "Boolean"), ("scripted", "Boolean"), ("minSponsoredFee", "Int|Unit"), ("name", "String"), ("description", "String")))), (("BooleanEntry", 5), (List(("key", "String"), ("value", "Boolean")))), (("InvokeScriptTransaction", 5), (List(("dApp", "Address|Alias"), ("feeAssetId", "ByteVector|Unit"), ("function", "String"), ("args", "List[Boolean|ByteVector|Int|List[Boolean|ByteVector|Int|String]|String]"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("payments", "List[AttachedPayment]"), ("proofs", "List[ByteVector]")))), (("Burn", 5), (List(("assetId", "ByteVector"), ("quantity", "Int")))), (("Floor", 5), (List())), (("Sha224", 5), (List())), (("IntegerEntry", 5), (List(("key", "String"), ("value", "Int")))), (("Down", 5), (List())), (("Transfer", 5), (List(("recipient", "Address|Alias"), ("amount", "Int")))), (("Sha1", 5), (List())), (("Sha3512", 5), (List())), (("MassTransferTransaction", 5), (List(("assetId", "ByteVector|Unit"), ("totalAmount", "Int"), ("transfers", "List[Transfer]"), ("transferCount", "Int"), ("attachment", "ByteVector"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("PaymentTransaction", 5), (List(("amount", "Int"), ("recipient", "Address|Alias"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Sha384", 5), (List())), (("ReissueTransaction", 5), (List(("quantity", "Int"), ("assetId", "ByteVector"), ("reissuable", "Boolean"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("HalfEven", 5), (List())), (("SetScriptTransaction", 5), (List(("script", "ByteVector|Unit"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("AssetPair", 5), (List(("amountAsset", "ByteVector|Unit"), ("priceAsset", "ByteVector|Unit")))), (("Sha3256", 5), (List())), (("BinaryEntry", 5), (List(("key", "String"), ("value", "ByteVector")))), (("HalfUp", 5), (List())), (("BurnTransaction", 5), (List(("quantity", "Int"), ("assetId", "ByteVector"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Lease", 5), (List(("recipient", "Address|Alias"), ("amount", "Int"), ("nonce", "Int")))), (("SponsorFeeTransaction", 5), (List(("assetId", "ByteVector"), ("minSponsoredAssetFee", "Int|Unit"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Order", 5), (List(("id", "ByteVector"), ("matcherPublicKey", "ByteVector"), ("assetPair", "AssetPair"), ("orderType", "Buy|Sell"), ("price", "Int"), ("amount", "Int"), ("timestamp", "Int"), ("expiration", "Int"), ("matcherFee", "Int"), ("matcherFeeAssetId", "ByteVector|Unit"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Alias", 5), (List(("alias", "String")))), (("Sha256", 5), (List())), (("Reissue", 5), (List(("assetId", "ByteVector"), ("quantity", "Int"), ("isReissuable", "Boolean")))), (("DataTransaction", 5), (List(("data", "List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("ScriptTransfer", 5), (List(("recipient", "Address|Alias"), ("amount", "Int"), ("asset", "ByteVector|Unit")))), (("GenesisTransaction", 5), (List(("amount", "Int"), ("recipient", "Address|Alias"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int")))), (("NoAlg", 5), (List())), (("CreateAliasTransaction", 5), (List(("alias", "String"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]"))))) ++ Map((("PaymentTransaction", 6), (List(("amount", "Int"), ("recipient", "Address|Alias"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("HalfUp", 6), (List())), (("HalfEven", 6), (List())), (("DataTransaction", 6), (List(("data", "List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Sha3512", 6), (List())), (("Sha1", 6), (List())), (("Unit", 6), (List())), (("ExchangeTransaction", 6), (List(("buyOrder", "Order"), ("sellOrder", "Order"), ("price", "Int"), ("amount", "Int"), ("buyMatcherFee", "Int"), ("sellMatcherFee", "Int"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("ReissueTransaction", 6), (List(("quantity", "Int"), ("assetId", "ByteVector"), ("reissuable", "Boolean"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("SponsorFeeTransaction", 6), (List(("assetId", "ByteVector"), ("minSponsoredAssetFee", "Int|Unit"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Sha256", 6), (List())), (("Floor", 6), (List())), (("Invocation", 6), (List(("payments", "List[AttachedPayment]"), ("caller", "Address"), ("callerPublicKey", "ByteVector"), ("transactionId", "ByteVector"), ("fee", "Int"), ("feeAssetId", "ByteVector|Unit"), ("originCaller", "Address"), ("originCallerPublicKey", "ByteVector")))), (("InvokeScriptTransaction", 6), (List(("dApp", "Address|Alias"), ("feeAssetId", "ByteVector|Unit"), ("function", "String"), ("args", "List[Boolean|ByteVector|Int|List[Boolean|ByteVector|Int|String]|String]"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("payments", "List[AttachedPayment]"), ("proofs", "List[ByteVector]")))), (("CreateAliasTransaction", 6), (List(("alias", "String"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("LeaseCancelTransaction", 6), (List(("leaseId", "ByteVector"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("LeaseCancel", 6), (List(("leaseId", "ByteVector")))), (("Issue", 6), (List(("name", "String"), ("description", "String"), ("quantity", "Int"), ("decimals", "Int"), ("isReissuable", "Boolean"), ("compiledScript", "Script|Unit"), ("nonce", "Int")))), (("IssueTransaction", 6), (List(("quantity", "Int"), ("name", "String"), ("description", "String"), ("reissuable", "Boolean"), ("decimals", "Int"), ("script", "ByteVector|Unit"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Lease", 6), (List(("recipient", "Address|Alias"), ("amount", "Int"), ("nonce", "Int")))), (("Sha3384", 6), (List())), (("Ceiling", 6), (List())), (("BooleanEntry", 6), (List(("key", "String"), ("value", "Boolean")))), (("BlockInfo", 6), (List(("timestamp", "Int"), ("height", "Int"), ("baseTarget", "Int"), ("generationSignature", "ByteVector"), ("generator", "Address"), ("generatorPublicKey", "ByteVector"), ("vrf", "ByteVector|Unit")))), (("Order", 6), (List(("id", "ByteVector"), ("matcherPublicKey", "ByteVector"), ("assetPair", "AssetPair"), ("orderType", "Buy|Sell"), ("price", "Int"), ("amount", "Int"), ("timestamp", "Int"), ("expiration", "Int"), ("matcherFee", "Int"), ("matcherFeeAssetId", "ByteVector|Unit"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Reissue", 6), (List(("assetId", "ByteVector"), ("quantity", "Int"), ("isReissuable", "Boolean")))), (("LeaseTransaction", 6), (List(("amount", "Int"), ("recipient", "Address|Alias"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Sha384", 6), (List())), (("IntegerEntry", 6), (List(("key", "String"), ("value", "Int")))), (("Alias", 6), (List(("alias", "String")))), (("Sha3256", 6), (List())), (("Transfer", 6), (List(("recipient", "Address|Alias"), ("amount", "Int")))), (("TransferTransaction", 6), (List(("feeAssetId", "ByteVector|Unit"), ("amount", "Int"), ("assetId", "ByteVector|Unit"), ("recipient", "Address|Alias"), ("attachment", "ByteVector"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Asset", 6), (List(("id", "ByteVector"), ("quantity", "Int"), ("decimals", "Int"), ("issuer", "Address"), ("issuerPublicKey", "ByteVector"), ("reissuable", "Boolean"), ("scripted", "Boolean"), ("minSponsoredFee", "Int|Unit"), ("name", "String"), ("description", "String")))), (("Md5", 6), (List())), (("ScriptTransfer", 6), (List(("recipient", "Address|Alias"), ("amount", "Int"), ("asset", "ByteVector|Unit")))), (("SetAssetScriptTransaction", 6), (List(("script", "ByteVector|Unit"), ("assetId", "ByteVector"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("SponsorFee", 6), (List(("assetId", "ByteVector"), ("minSponsoredAssetFee", "Int|Unit")))), (("Down", 6), (List())), (("AttachedPayment", 6), (List(("assetId", "ByteVector|Unit"), ("amount", "Int")))), (("Address", 6), (List(("bytes", "ByteVector")))), (("DeleteEntry", 6), (List(("key", "String")))), (("NoAlg", 6), (List())), (("MassTransferTransaction", 6), (List(("assetId", "ByteVector|Unit"), ("totalAmount", "Int"), ("transfers", "List[Transfer]"), ("transferCount", "Int"), ("attachment", "ByteVector"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Sha3224", 6), (List())), (("SetScriptTransaction", 6), (List(("script", "ByteVector|Unit"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("BinaryEntry", 6), (List(("key", "String"), ("value", "ByteVector")))), (("Burn", 6), (List(("assetId", "ByteVector"), ("quantity", "Int")))), (("Sha224", 6), (List())), (("InvokeExpressionTransaction", 6), (List(("expression", "ByteVector"), ("feeAssetId", "ByteVector|Unit"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Sha512", 6), (List())), (("GenesisTransaction", 6), (List(("amount", "Int"), ("recipient", "Address|Alias"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int")))), (("BalanceDetails", 6), (List(("available", "Int"), ("regular", "Int"), ("generating", "Int"), ("effective", "Int")))), (("BurnTransaction", 6), (List(("quantity", "Int"), ("assetId", "ByteVector"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("AssetPair", 6), (List(("amountAsset", "ByteVector|Unit"), ("priceAsset", "ByteVector|Unit")))), (("StringEntry", 6), (List(("key", "String"), ("value", "String")))), (("UpdateAssetInfoTransaction", 6), (List(("assetId", "ByteVector"), ("name", "String"), ("description", "String"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]"))))) ++ Map((("BinaryEntry", 7), (List(("key", "String"), ("value", "ByteVector")))), (("Invocation", 7), (List(("payments", "List[AttachedPayment]"), ("caller", "Address"), ("callerPublicKey", "ByteVector"), ("transactionId", "ByteVector"), ("fee", "Int"), ("feeAssetId", "ByteVector|Unit"), ("originCaller", "Address"), ("originCallerPublicKey", "ByteVector")))), (("Sha3256", 7), (List())), (("NoAlg", 7), (List())), (("Address", 7), (List(("bytes", "ByteVector")))), (("Sha3384", 7), (List())), (("MassTransferTransaction", 7), (List(("assetId", "ByteVector|Unit"), ("totalAmount", "Int"), ("transfers", "List[Transfer]"), ("transferCount", "Int"), ("attachment", "ByteVector"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("BooleanEntry", 7), (List(("key", "String"), ("value", "Boolean")))), (("CreateAliasTransaction", 7), (List(("alias", "String"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("DeleteEntry", 7), (List(("key", "String")))), (("Down", 7), (List())), (("Floor", 7), (List())), (("SetAssetScriptTransaction", 7), (List(("script", "ByteVector|Unit"), ("assetId", "ByteVector"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Sha224", 7), (List())), (("DataTransaction", 7), (List(("data", "List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Alias", 7), (List(("alias", "String")))), (("HalfUp", 7), (List())), (("Burn", 7), (List(("assetId", "ByteVector"), ("quantity", "Int")))), (("Sha3512", 7), (List())), (("StringEntry", 7), (List(("key", "String"), ("value", "String")))), (("ScriptTransfer", 7), (List(("recipient", "Address|Alias"), ("amount", "Int"), ("asset", "ByteVector|Unit")))), (("Order", 7), (List(("id", "ByteVector"), ("matcherPublicKey", "ByteVector"), ("assetPair", "AssetPair"), ("orderType", "Buy|Sell"), ("price", "Int"), ("amount", "Int"), ("timestamp", "Int"), ("expiration", "Int"), ("matcherFee", "Int"), ("matcherFeeAssetId", "ByteVector|Unit"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Sha3224", 7), (List())), (("Transfer", 7), (List(("recipient", "Address|Alias"), ("amount", "Int")))), (("IssueTransaction", 7), (List(("quantity", "Int"), ("name", "String"), ("description", "String"), ("reissuable", "Boolean"), ("decimals", "Int"), ("script", "ByteVector|Unit"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("InvokeExpressionTransaction", 7), (List(("expression", "ByteVector"), ("feeAssetId", "ByteVector|Unit"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("BurnTransaction", 7), (List(("quantity", "Int"), ("assetId", "ByteVector"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Sha1", 7), (List())), (("Unit", 7), (List())), (("Issue", 7), (List(("name", "String"), ("description", "String"), ("quantity", "Int"), ("decimals", "Int"), ("isReissuable", "Boolean"), ("compiledScript", "Script|Unit"), ("nonce", "Int")))), (("UpdateAssetInfoTransaction", 7), (List(("assetId", "ByteVector"), ("name", "String"), ("description", "String"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Lease", 7), (List(("recipient", "Address|Alias"), ("amount", "Int"), ("nonce", "Int")))), (("SponsorFeeTransaction", 7), (List(("assetId", "ByteVector"), ("minSponsoredAssetFee", "Int|Unit"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("InvokeScriptTransaction", 7), (List(("dApp", "Address|Alias"), ("feeAssetId", "ByteVector|Unit"), ("function", "String"), ("args", "List[Boolean|ByteVector|Int|List[Boolean|ByteVector|Int|String]|String]"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("payments", "List[AttachedPayment]"), ("proofs", "List[ByteVector]")))), (("TransferTransaction", 7), (List(("feeAssetId", "ByteVector|Unit"), ("amount", "Int"), ("assetId", "ByteVector|Unit"), ("recipient", "Address|Alias"), ("attachment", "ByteVector"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("AttachedPayment", 7), (List(("assetId", "ByteVector|Unit"), ("amount", "Int")))), (("AssetPair", 7), (List(("amountAsset", "ByteVector|Unit"), ("priceAsset", "ByteVector|Unit")))), (("Md5", 7), (List())), (("PaymentTransaction", 7), (List(("amount", "Int"), ("recipient", "Address|Alias"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Ceiling", 7), (List())), (("ExchangeTransaction", 7), (List(("buyOrder", "Order"), ("sellOrder", "Order"), ("price", "Int"), ("amount", "Int"), ("buyMatcherFee", "Int"), ("sellMatcherFee", "Int"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("LeaseCancel", 7), (List(("leaseId", "ByteVector")))), (("BlockInfo", 7), (List(("timestamp", "Int"), ("height", "Int"), ("baseTarget", "Int"), ("generationSignature", "ByteVector"), ("generator", "Address"), ("generatorPublicKey", "ByteVector"), ("vrf", "ByteVector|Unit"), ("rewards", "List[(Address, Int)]")))), (("BalanceDetails", 7), (List(("available", "Int"), ("regular", "Int"), ("generating", "Int"), ("effective", "Int")))), (("Sha256", 7), (List())), (("Reissue", 7), (List(("assetId", "ByteVector"), ("quantity", "Int"), ("isReissuable", "Boolean")))), (("Sha512", 7), (List())), (("SponsorFee", 7), (List(("assetId", "ByteVector"), ("minSponsoredAssetFee", "Int|Unit")))), (("SetScriptTransaction", 7), (List(("script", "ByteVector|Unit"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("ReissueTransaction", 7), (List(("quantity", "Int"), ("assetId", "ByteVector"), ("reissuable", "Boolean"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Sha384", 7), (List())), (("IntegerEntry", 7), (List(("key", "String"), ("value", "Int")))), (("LeaseTransaction", 7), (List(("amount", "Int"), ("recipient", "Address|Alias"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Asset", 7), (List(("id", "ByteVector"), ("quantity", "Int"), ("decimals", "Int"), ("issuer", "Address"), ("issuerPublicKey", "ByteVector"), ("reissuable", "Boolean"), ("scripted", "Boolean"), ("minSponsoredFee", "Int|Unit"), ("name", "String"), ("description", "String")))), (("GenesisTransaction", 7), (List(("amount", "Int"), ("recipient", "Address|Alias"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int")))), (("LeaseCancelTransaction", 7), (List(("leaseId", "ByteVector"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("HalfEven", 7), (List()))) ++ Map((("TransferTransaction", 8), (List(("feeAssetId", "ByteVector|Unit"), ("amount", "Int"), ("assetId", "ByteVector|Unit"), ("recipient", "Address|Alias"), ("attachment", "ByteVector"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("NoAlg", 8), (List())), (("Order", 8), (List(("id", "ByteVector"), ("matcherPublicKey", "ByteVector"), ("assetPair", "AssetPair"), ("orderType", "Buy|Sell"), ("price", "Int"), ("amount", "Int"), ("timestamp", "Int"), ("expiration", "Int"), ("matcherFee", "Int"), ("matcherFeeAssetId", "ByteVector|Unit"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("attachment", "ByteVector|Unit"), ("proofs", "List[ByteVector]")))), (("Unit", 8), (List())), (("DataTransaction", 8), (List(("data", "List[BinaryEntry|BooleanEntry|DeleteEntry|IntegerEntry|StringEntry]"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("BalanceDetails", 8), (List(("available", "Int"), ("regular", "Int"), ("generating", "Int"), ("effective", "Int")))), (("DeleteEntry", 8), (List(("key", "String")))), (("Sha3224", 8), (List())), (("GenesisTransaction", 8), (List(("amount", "Int"), ("recipient", "Address|Alias"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int")))), (("SetScriptTransaction", 8), (List(("script", "ByteVector|Unit"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("CreateAliasTransaction", 8), (List(("alias", "String"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("AssetPair", 8), (List(("amountAsset", "ByteVector|Unit"), ("priceAsset", "ByteVector|Unit")))), (("Alias", 8), (List(("alias", "String")))), (("Md5", 8), (List())), (("ExchangeTransaction", 8), (List(("buyOrder", "Order"), ("sellOrder", "Order"), ("price", "Int"), ("amount", "Int"), ("buyMatcherFee", "Int"), ("sellMatcherFee", "Int"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Address", 8), (List(("bytes", "ByteVector")))), (("AttachedPayment", 8), (List(("assetId", "ByteVector|Unit"), ("amount", "Int")))), (("Sha1", 8), (List())), (("Sha384", 8), (List())), (("BooleanEntry", 8), (List(("key", "String"), ("value", "Boolean")))), (("Floor", 8), (List())), (("InvokeScriptTransaction", 8), (List(("dApp", "Address|Alias"), ("feeAssetId", "ByteVector|Unit"), ("function", "String"), ("args", "List[Boolean|ByteVector|Int|List[Boolean|ByteVector|Int|String]|String]"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("payments", "List[AttachedPayment]"), ("proofs", "List[ByteVector]")))), (("InvokeExpressionTransaction", 8), (List(("expression", "ByteVector"), ("feeAssetId", "ByteVector|Unit"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Reissue", 8), (List(("assetId", "ByteVector"), ("quantity", "Int"), ("isReissuable", "Boolean")))), (("BlockInfo", 8), (List(("timestamp", "Int"), ("height", "Int"), ("baseTarget", "Int"), ("generationSignature", "ByteVector"), ("generator", "Address"), ("generatorPublicKey", "ByteVector"), ("vrf", "ByteVector|Unit"), ("rewards", "List[(Address, Int)]")))), (("IntegerEntry", 8), (List(("key", "String"), ("value", "Int")))), (("LeaseTransaction", 8), (List(("amount", "Int"), ("recipient", "Address|Alias"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Burn", 8), (List(("assetId", "ByteVector"), ("quantity", "Int")))), (("StringEntry", 8), (List(("key", "String"), ("value", "String")))), (("Transfer", 8), (List(("recipient", "Address|Alias"), ("amount", "Int")))), (("Invocation", 8), (List(("payments", "List[AttachedPayment]"), ("caller", "Address"), ("callerPublicKey", "ByteVector"), ("transactionId", "ByteVector"), ("fee", "Int"), ("feeAssetId", "ByteVector|Unit"), ("originCaller", "Address"), ("originCallerPublicKey", "ByteVector")))), (("ScriptTransfer", 8), (List(("recipient", "Address|Alias"), ("amount", "Int"), ("asset", "ByteVector|Unit")))), (("Sha3512", 8), (List())), (("Asset", 8), (List(("id", "ByteVector"), ("quantity", "Int"), ("decimals", "Int"), ("issuer", "Address"), ("issuerPublicKey", "ByteVector"), ("reissuable", "Boolean"), ("scripted", "Boolean"), ("minSponsoredFee", "Int|Unit"), ("name", "String"), ("description", "String")))), (("BinaryEntry", 8), (List(("key", "String"), ("value", "ByteVector")))), (("ReissueTransaction", 8), (List(("quantity", "Int"), ("assetId", "ByteVector"), ("reissuable", "Boolean"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("HalfEven", 8), (List())), (("MassTransferTransaction", 8), (List(("assetId", "ByteVector|Unit"), ("totalAmount", "Int"), ("transfers", "List[Transfer]"), ("transferCount", "Int"), ("attachment", "ByteVector"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("SetAssetScriptTransaction", 8), (List(("script", "ByteVector|Unit"), ("assetId", "ByteVector"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("BurnTransaction", 8), (List(("quantity", "Int"), ("assetId", "ByteVector"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Sha224", 8), (List())), (("HalfUp", 8), (List())), (("Sha512", 8), (List())), (("Lease", 8), (List(("recipient", "Address|Alias"), ("amount", "Int"), ("nonce", "Int")))), (("PaymentTransaction", 8), (List(("amount", "Int"), ("recipient", "Address|Alias"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("LeaseCancelTransaction", 8), (List(("leaseId", "ByteVector"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Sha3384", 8), (List())), (("SponsorFeeTransaction", 8), (List(("assetId", "ByteVector"), ("minSponsoredAssetFee", "Int|Unit"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("UpdateAssetInfoTransaction", 8), (List(("assetId", "ByteVector"), ("name", "String"), ("description", "String"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("LeaseCancel", 8), (List(("leaseId", "ByteVector")))), (("Issue", 8), (List(("name", "String"), ("description", "String"), ("quantity", "Int"), ("decimals", "Int"), ("isReissuable", "Boolean"), ("compiledScript", "Script|Unit"), ("nonce", "Int")))), (("Down", 8), (List())), (("Sha256", 8), (List())), (("Sha3256", 8), (List())), (("SponsorFee", 8), (List(("assetId", "ByteVector"), ("minSponsoredAssetFee", "Int|Unit")))), (("IssueTransaction", 8), (List(("quantity", "Int"), ("name", "String"), ("description", "String"), ("reissuable", "Boolean"), ("decimals", "Int"), ("script", "ByteVector|Unit"), ("id", "ByteVector"), ("fee", "Int"), ("timestamp", "Int"), ("version", "Int"), ("sender", "Address"), ("senderPublicKey", "ByteVector"), ("bodyBytes", "ByteVector"), ("proofs", "List[ByteVector]")))), (("Ceiling", 8), (List())))
lazy val varData = Map((("FLOOR", 1), ("'FLOOR' rounding mode")), (("tx", 1), ("Processing transaction")), (("HALFEVEN", 1), ("'HALF_EVEN' rounding mode")), (("UP", 1), ("'UP' rounding mode")), (("unit", 1), ("Single instance value")), (("CEILING", 1), ("'CEILING' rounding mode")), (("HALFUP", 1), ("'HALF_UP' rounding mode")), (("DOWN", 1), ("'DOWN' rounding mode")), (("HALFDOWN", 1), ("'HALF_DOWN' rounding mode")), (("height", 1), ("Current blockchain height"))) ++ Map((("HALFUP", 2), ("'HALF_UP' rounding mode")), (("FLOOR", 2), ("'FLOOR' rounding mode")), (("tx", 2), ("Processing transaction")), (("height", 2), ("Current blockchain height")), (("CEILING", 2), ("'CEILING' rounding mode")), (("DOWN", 2), ("'DOWN' rounding mode")), (("unit", 2), ("Single instance value")), (("Sell", 2), ("Sell OrderType")), (("Buy", 2), ("Buy OrderType")), (("HALFEVEN", 2), ("'HALF_EVEN' rounding mode")), (("UP", 2), ("'UP' rounding mode")), (("HALFDOWN", 2), ("'HALF_DOWN' rounding mode"))) ++ varsV3 ++ varsV4 ++ varsV5 ++ varsV6 ++ varsV7 ++ varsV8
lazy val funcData = Map((("-", List("Int"), 1), ("Change integer sign", List("value"), 9)), (("+", List("ByteVector", "ByteVector"), 1), ("Limited byte vectors concatenation", List("prefix", "suffix"), 10)), (("getString", List("Address|Alias", "String"), 1), ("get data from the account state", List("account", "key"), 100)), (("*", List("Int", "Int"), 1), ("Integer multiplication", List("multiplier", "multiplier"), 1)), (("addressFromPublicKey", List("ByteVector"), 1), ("Convert public key to account address", List("public key"), 82)), (("isDefined", List("T|Unit"), 1), ("Check the value is defined", List("Option value"), 35)), (("dropRight", List("String", "Int"), 1), ("Remove string suffix", List("string", "suffix size in characters"), 19)), (("transactionHeightById", List("ByteVector"), 1), ("get height when transaction was stored to blockchain", List("transaction Id"), 100)), (("assetBalance", List("Address|Alias", "ByteVector|Unit"), 1), ("get asset balance for account", List("account", "assetId (WAVES if none)"), 100)), (("+", List("Int", "Int"), 1), ("Integer sum", List("term", "term"), 1)), (("toBase58String", List("ByteVector"), 1), ("Base58 encode", List("value"), 10)), (("!=", List("T", "T"), 1), ("Inequality", List("value", "value"), 26)), (("!", List("Boolean"), 1), ("unary negation", List("boolean"), 11)), (("getInteger", List("Address|Alias", "String"), 1), ("get data from the account state", List("account", "key"), 100)), (("getString", List("List[DataEntry]", "Int"), 1), ("Extract data by index", List("DataEntry list, usually tx.data", "index"), 30)), (("fromBase58String", List("String"), 1), ("Base58 decode", List("base58 encoded string"), 10)), (("getInteger", List("List[DataEntry]", "String"), 1), ("Find and extract data by key", List("DataEntry list, usually tx.data", "key"), 10)), (("take", List("ByteVector", "Int"), 1), ("Take first bytes subvector", List("vector", "Bytes number"), 1)), (("sigVerify", List("ByteVector", "ByteVector", "ByteVector"), 1), ("check signature", List("value", "signature", "public key"), 100)), (("dropRight", List("ByteVector", "Int"), 1), ("Cut vectors tail", List("vector", "cutting size"), 19)), (("/", List("Int", "Int"), 1), ("Integer division", List("divisible", "divisor"), 1)), ((">", List("Int", "Int"), 1), ("Integer greater comparison", List("term", "term"), 1)), (("sha256", List("ByteVector"), 1), ("256 bit SHA-2", List("value"), 10)), (("toBytes", List("Boolean"), 1), ("Bytes array representation", List("value"), 1)), (("drop", List("String", "Int"), 1), ("Remove string prefix", List("string", "prefix size"), 1)), (("keccak256", List("ByteVector"), 1), ("256 bit Keccak/SHA-3/TIPS-202", List("value"), 10)), (("drop", List("ByteVector", "Int"), 1), ("Skip first bytes", List("vector", "Bytes number"), 1)), (("addressFromRecipient", List("Address|Alias"), 1), ("Extract address or lookup alias", List("address or alias, usually tx.recipient"), 100)), (("==", List("T", "T"), 1), ("Equality", List("value", "value"), 1)), (("getBoolean", List("List[DataEntry]", "String"), 1), ("Find and extract data by key", List("DataEntry list, usually tx.data", "key"), 10)), (("size", List("List[T]"), 1), ("Size of list", List("list"), 2)), (("addressFromString", List("String"), 1), ("Decode account address", List("string address representation"), 124)), (("wavesBalance", List("Address|Alias"), 1), ("get WAVES balance for account", List("account"), 109)), (("+", List("String", "String"), 1), ("Limited strings concatenation", List("prefix", "suffix"), 10)), (("getInteger", List("List[DataEntry]", "Int"), 1), ("Extract data by index", List("DataEntry list, usually tx.data", "index"), 30)), (("size", List("String"), 1), ("String size in characters", List("string"), 1)), (("-", List("Int", "Int"), 1), ("Integer substitution", List("term", "term"), 1)), (("extract", List("T|Unit"), 1), ("Extract value from option or fail", List("Optional value"), 13)), (("fromBase64String", List("String"), 1), ("Base64 decode", List("base64 encoded string"), 10)), (("getString", List("List[DataEntry]", "String"), 1), ("Find and extract data by key", List("DataEntry list, usually tx.data", "key"), 10)), (("getElement", List("List[T]", "Int"), 1), ("Get list element by position", List("list", "element position"), 2)), (("toBytes", List("String"), 1), ("Bytes array representation", List("value"), 1)), (("getBinary", List("List[DataEntry]", "Int"), 1), ("Extract data by index", List("DataEntry list, usually tx.data", "index"), 30)), (("getBinary", List("Address|Alias", "String"), 1), ("get data from the account state", List("account", "key"), 100)), (("getBoolean", List("Address|Alias", "String"), 1), ("get data from the account state", List("account", "key"), 100)), (("toBase64String", List("ByteVector"), 1), ("Base64 encode", List("value"), 10)), (("size", List("ByteVector"), 1), ("Size of bytes str", List("vector"), 1)), (("getBoolean", List("List[DataEntry]", "Int"), 1), ("Extract data by index", List("DataEntry list, usually tx.data", "index"), 30)), (("fraction", List("Int", "Int", "Int"), 1), ("Multiply and division with unlimited intermediate representation", List("multiplier", "multiplier", "divisor"), 1)), (("toString", List("Int"), 1), ("String representation", List("value"), 1)), (("takeRight", List("ByteVector", "Int"), 1), ("Take vector tail", List("vector", "taking size"), 19)), ((">=", List("Int", "Int"), 1), ("Integer greater or equal comparison", List("term", "term"), 1)), (("throw", List("String"), 1), ("Fail script", List("Error message"), 1)), (("getBinary", List("List[DataEntry]", "String"), 1), ("Find and extract data by key", List("DataEntry list, usually tx.data", "key"), 10)), (("transactionById", List("ByteVector"), 1), ("Lookup transaction", List("transaction Id"), 100)), (("takeRight", List("String", "Int"), 1), ("Take string suffix", List("String", "suffix size in characters"), 19)), (("%", List("Int", "Int"), 1), ("Modulo", List("divisible", "divisor"), 1)), (("take", List("String", "Int"), 1), ("Take string prefix", List("string", "prefix size in characters"), 1)), (("toBytes", List("Int"), 1), ("Bytes array representation", List("value"), 1)), (("throw", List(), 1), ("Fail script", List(), 2)), (("blake2b256", List("ByteVector"), 1), ("256 bit BLAKE", List("value"), 10)), (("toString", List("Boolean"), 1), ("String representation", List("value"), 1))) ++ Map((("getElement", List("List[T]", "Int"), 2), ("Get list element by position", List("list", "element position"), 2)), ((">=", List("Int", "Int"), 2), ("Integer greater or equal comparison", List("term", "term"), 1)), ((">", List("Int", "Int"), 2), ("Integer greater comparison", List("term", "term"), 1)), (("fraction", List("Int", "Int", "Int"), 2), ("Multiply and division with unlimited intermediate representation", List("multiplier", "multiplier", "divisor"), 1)), (("getInteger", List("Address|Alias", "String"), 2), ("get data from the account state", List("account", "key"), 100)), (("takeRight", List("String", "Int"), 2), ("Take string suffix", List("String", "suffix size in characters"), 19)), (("dropRight", List("String", "Int"), 2), ("Remove string suffix", List("string", "suffix size in characters"), 19)), (("%", List("Int", "Int"), 2), ("Modulo", List("divisible", "divisor"), 1)), (("size", List("String"), 2), ("String size in characters", List("string"), 1)), (("toBase58String", List("ByteVector"), 2), ("Base58 encode", List("value"), 10)), (("wavesBalance", List("Address|Alias"), 2), ("get WAVES balance for account", List("account"), 109)), (("addressFromRecipient", List("Address|Alias"), 2), ("Extract address or lookup alias", List("address or alias, usually tx.recipient"), 100)), (("transactionHeightById", List("ByteVector"), 2), ("get height when transaction was stored to blockchain", List("transaction Id"), 100)), (("size", List("List[T]"), 2), ("Size of list", List("list"), 2)), (("sigVerify", List("ByteVector", "ByteVector", "ByteVector"), 2), ("check signature", List("value", "signature", "public key"), 100)), (("keccak256", List("ByteVector"), 2), ("256 bit Keccak/SHA-3/TIPS-202", List("value"), 10)), (("getBinary", List("List[DataEntry]", "Int"), 2), ("Extract data by index", List("DataEntry list, usually tx.data", "index"), 30)), (("==", List("T", "T"), 2), ("Equality", List("value", "value"), 1)), (("getInteger", List("List[DataEntry]", "String"), 2), ("Find and extract data by key", List("DataEntry list, usually tx.data", "key"), 10)), (("toBytes", List("Boolean"), 2), ("Bytes array representation", List("value"), 1)), (("throw", List(), 2), ("Fail script", List(), 2)), (("takeRight", List("ByteVector", "Int"), 2), ("Take vector tail", List("vector", "taking size"), 19)), (("toBase64String", List("ByteVector"), 2), ("Base64 encode", List("value"), 10)), (("addressFromPublicKey", List("ByteVector"), 2), ("Convert public key to account address", List("public key"), 82)), (("-", List("Int"), 2), ("Change integer sign", List("value"), 9)), (("toString", List("Int"), 2), ("String representation", List("value"), 1)), (("!=", List("T", "T"), 2), ("Inequality", List("value", "value"), 26)), (("isDefined", List("T|Unit"), 2), ("Check the value is defined", List("Option value"), 35)), (("+", List("String", "String"), 2), ("Limited strings concatenation", List("prefix", "suffix"), 10)), (("take", List("ByteVector", "Int"), 2), ("Take first bytes subvector", List("vector", "Bytes number"), 1)), (("transactionById", List("ByteVector"), 2), ("Lookup transaction", List("transaction Id"), 100)), (("getInteger", List("List[DataEntry]", "Int"), 2), ("Extract data by index", List("DataEntry list, usually tx.data", "index"), 30)), (("+", List("Int", "Int"), 2), ("Integer sum", List("term", "term"), 1)), (("take", List("String", "Int"), 2), ("Take string prefix", List("string", "prefix size in characters"), 1)), (("throw", List("String"), 2), ("Fail script", List("Error message"), 1)), (("getBinary", List("List[DataEntry]", "String"), 2), ("Find and extract data by key", List("DataEntry list, usually tx.data", "key"), 10)), (("assetBalance", List("Address|Alias", "ByteVector|Unit"), 2), ("get asset balance for account", List("account", "assetId (WAVES if none)"), 100)), (("toString", List("Boolean"), 2), ("String representation", List("value"), 1)), (("getBinary", List("Address|Alias", "String"), 2), ("get data from the account state", List("account", "key"), 100)), (("-", List("Int", "Int"), 2), ("Integer substitution", List("term", "term"), 1)), (("+", List("ByteVector", "ByteVector"), 2), ("Limited byte vectors concatenation", List("prefix", "suffix"), 10)), (("extract", List("T|Unit"), 2), ("Extract value from option or fail", List("Optional value"), 13)), (("getString", List("List[DataEntry]", "Int"), 2), ("Extract data by index", List("DataEntry list, usually tx.data", "index"), 30)), (("getBoolean", List("List[DataEntry]", "Int"), 2), ("Extract data by index", List("DataEntry list, usually tx.data", "index"), 30)), (("!", List("Boolean"), 2), ("unary negation", List("boolean"), 11)), (("drop", List("ByteVector", "Int"), 2), ("Skip first bytes", List("vector", "Bytes number"), 1)), (("toBytes", List("Int"), 2), ("Bytes array representation", List("value"), 1)), (("drop", List("String", "Int"), 2), ("Remove string prefix", List("string", "prefix size"), 1)), (("getBoolean", List("List[DataEntry]", "String"), 2), ("Find and extract data by key", List("DataEntry list, usually tx.data", "key"), 10)), (("fromBase64String", List("String"), 2), ("Base64 decode", List("base64 encoded string"), 10)), (("toBytes", List("String"), 2), ("Bytes array representation", List("value"), 1)), (("size", List("ByteVector"), 2), ("Size of bytes str", List("vector"), 1)), (("getString", List("List[DataEntry]", "String"), 2), ("Find and extract data by key", List("DataEntry list, usually tx.data", "key"), 10)), (("/", List("Int", "Int"), 2), ("Integer division", List("divisible", "divisor"), 1)), (("getBoolean", List("Address|Alias", "String"), 2), ("get data from the account state", List("account", "key"), 100)), (("blake2b256", List("ByteVector"), 2), ("256 bit BLAKE", List("value"), 10)), (("addressFromString", List("String"), 2), ("Decode account address", List("string address representation"), 124)), (("dropRight", List("ByteVector", "Int"), 2), ("Cut vectors tail", List("vector", "cutting size"), 19)), (("fromBase58String", List("String"), 2), ("Base58 decode", List("base58 encoded string"), 10)), (("*", List("Int", "Int"), 2), ("Integer multiplication", List("multiplier", "multiplier"), 1)), (("getString", List("Address|Alias", "String"), 2), ("get data from the account state", List("account", "key"), 100)), (("sha256", List("ByteVector"), 2), ("256 bit SHA-2", List("value"), 10))) ++ (funcsV3 ++ funcsV4 ++ funcsV5 ++ funcsV6 ++ funcsV7 ++ funcsV8).view.mapValues(v => (regex.replaceAllIn(v._1, _.group(1)), v._2, v._4))
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy