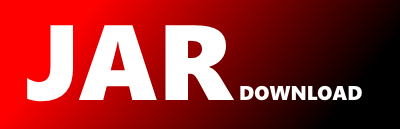
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.scala Maven / Gradle / Ivy
The newest version!
// Generated by the Scala Plugin for the Protocol Buffer Compiler.
// Do not edit!
//
// Protofile syntax: PROTO3
package com.wavesplatform.protobuf.snapshot
@SerialVersionUID(0L)
final case class TransactionStateSnapshot(
balances: _root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Balance] = _root_.scala.Seq.empty,
leaseBalances: _root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.LeaseBalance] = _root_.scala.Seq.empty,
newLeases: _root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewLease] = _root_.scala.Seq.empty,
cancelledLeases: _root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.CancelledLease] = _root_.scala.Seq.empty,
assetStatics: _root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewAsset] = _root_.scala.Seq.empty,
assetVolumes: _root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetVolume] = _root_.scala.Seq.empty,
assetNamesAndDescriptions: _root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetNameAndDescription] = _root_.scala.Seq.empty,
assetScripts: _root_.scala.Option[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetScript] = _root_.scala.None,
aliases: _root_.scala.Option[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Alias] = _root_.scala.None,
orderFills: _root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.OrderFill] = _root_.scala.Seq.empty,
accountScripts: _root_.scala.Option[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountScript] = _root_.scala.None,
accountData: _root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountData] = _root_.scala.Seq.empty,
sponsorships: _root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Sponsorship] = _root_.scala.Seq.empty,
transactionStatus: com.wavesplatform.protobuf.snapshot.TransactionStatus = com.wavesplatform.protobuf.snapshot.TransactionStatus.SUCCEEDED,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[TransactionStateSnapshot] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
balances.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
leaseBalances.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
newLeases.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
cancelledLeases.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
assetStatics.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
assetVolumes.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
assetNamesAndDescriptions.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
if (assetScripts.isDefined) {
val __value = assetScripts.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
if (aliases.isDefined) {
val __value = aliases.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
orderFills.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
if (accountScripts.isDefined) {
val __value = accountScripts.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
accountData.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
sponsorships.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
{
val __value = transactionStatus.value
if (__value != 0) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeEnumSize(14, __value)
}
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
balances.foreach { __v =>
val __m = __v
_output__.writeTag(1, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
leaseBalances.foreach { __v =>
val __m = __v
_output__.writeTag(2, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
newLeases.foreach { __v =>
val __m = __v
_output__.writeTag(3, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
cancelledLeases.foreach { __v =>
val __m = __v
_output__.writeTag(4, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
assetStatics.foreach { __v =>
val __m = __v
_output__.writeTag(5, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
assetVolumes.foreach { __v =>
val __m = __v
_output__.writeTag(6, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
assetNamesAndDescriptions.foreach { __v =>
val __m = __v
_output__.writeTag(7, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
assetScripts.foreach { __v =>
val __m = __v
_output__.writeTag(8, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
aliases.foreach { __v =>
val __m = __v
_output__.writeTag(9, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
orderFills.foreach { __v =>
val __m = __v
_output__.writeTag(10, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
accountScripts.foreach { __v =>
val __m = __v
_output__.writeTag(11, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
accountData.foreach { __v =>
val __m = __v
_output__.writeTag(12, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
sponsorships.foreach { __v =>
val __m = __v
_output__.writeTag(13, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
{
val __v = transactionStatus.value
if (__v != 0) {
_output__.writeEnum(14, __v)
}
};
unknownFields.writeTo(_output__)
}
def clearBalances = copy(balances = _root_.scala.Seq.empty)
def addBalances(__vs: com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Balance *): TransactionStateSnapshot = addAllBalances(__vs)
def addAllBalances(__vs: Iterable[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Balance]): TransactionStateSnapshot = copy(balances = balances ++ __vs)
def withBalances(__v: _root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Balance]): TransactionStateSnapshot = copy(balances = __v)
def clearLeaseBalances = copy(leaseBalances = _root_.scala.Seq.empty)
def addLeaseBalances(__vs: com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.LeaseBalance *): TransactionStateSnapshot = addAllLeaseBalances(__vs)
def addAllLeaseBalances(__vs: Iterable[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.LeaseBalance]): TransactionStateSnapshot = copy(leaseBalances = leaseBalances ++ __vs)
def withLeaseBalances(__v: _root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.LeaseBalance]): TransactionStateSnapshot = copy(leaseBalances = __v)
def clearNewLeases = copy(newLeases = _root_.scala.Seq.empty)
def addNewLeases(__vs: com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewLease *): TransactionStateSnapshot = addAllNewLeases(__vs)
def addAllNewLeases(__vs: Iterable[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewLease]): TransactionStateSnapshot = copy(newLeases = newLeases ++ __vs)
def withNewLeases(__v: _root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewLease]): TransactionStateSnapshot = copy(newLeases = __v)
def clearCancelledLeases = copy(cancelledLeases = _root_.scala.Seq.empty)
def addCancelledLeases(__vs: com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.CancelledLease *): TransactionStateSnapshot = addAllCancelledLeases(__vs)
def addAllCancelledLeases(__vs: Iterable[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.CancelledLease]): TransactionStateSnapshot = copy(cancelledLeases = cancelledLeases ++ __vs)
def withCancelledLeases(__v: _root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.CancelledLease]): TransactionStateSnapshot = copy(cancelledLeases = __v)
def clearAssetStatics = copy(assetStatics = _root_.scala.Seq.empty)
def addAssetStatics(__vs: com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewAsset *): TransactionStateSnapshot = addAllAssetStatics(__vs)
def addAllAssetStatics(__vs: Iterable[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewAsset]): TransactionStateSnapshot = copy(assetStatics = assetStatics ++ __vs)
def withAssetStatics(__v: _root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewAsset]): TransactionStateSnapshot = copy(assetStatics = __v)
def clearAssetVolumes = copy(assetVolumes = _root_.scala.Seq.empty)
def addAssetVolumes(__vs: com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetVolume *): TransactionStateSnapshot = addAllAssetVolumes(__vs)
def addAllAssetVolumes(__vs: Iterable[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetVolume]): TransactionStateSnapshot = copy(assetVolumes = assetVolumes ++ __vs)
def withAssetVolumes(__v: _root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetVolume]): TransactionStateSnapshot = copy(assetVolumes = __v)
def clearAssetNamesAndDescriptions = copy(assetNamesAndDescriptions = _root_.scala.Seq.empty)
def addAssetNamesAndDescriptions(__vs: com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetNameAndDescription *): TransactionStateSnapshot = addAllAssetNamesAndDescriptions(__vs)
def addAllAssetNamesAndDescriptions(__vs: Iterable[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetNameAndDescription]): TransactionStateSnapshot = copy(assetNamesAndDescriptions = assetNamesAndDescriptions ++ __vs)
def withAssetNamesAndDescriptions(__v: _root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetNameAndDescription]): TransactionStateSnapshot = copy(assetNamesAndDescriptions = __v)
def getAssetScripts: com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetScript = assetScripts.getOrElse(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetScript.defaultInstance)
def clearAssetScripts: TransactionStateSnapshot = copy(assetScripts = _root_.scala.None)
def withAssetScripts(__v: com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetScript): TransactionStateSnapshot = copy(assetScripts = Option(__v))
def getAliases: com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Alias = aliases.getOrElse(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Alias.defaultInstance)
def clearAliases: TransactionStateSnapshot = copy(aliases = _root_.scala.None)
def withAliases(__v: com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Alias): TransactionStateSnapshot = copy(aliases = Option(__v))
def clearOrderFills = copy(orderFills = _root_.scala.Seq.empty)
def addOrderFills(__vs: com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.OrderFill *): TransactionStateSnapshot = addAllOrderFills(__vs)
def addAllOrderFills(__vs: Iterable[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.OrderFill]): TransactionStateSnapshot = copy(orderFills = orderFills ++ __vs)
def withOrderFills(__v: _root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.OrderFill]): TransactionStateSnapshot = copy(orderFills = __v)
def getAccountScripts: com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountScript = accountScripts.getOrElse(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountScript.defaultInstance)
def clearAccountScripts: TransactionStateSnapshot = copy(accountScripts = _root_.scala.None)
def withAccountScripts(__v: com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountScript): TransactionStateSnapshot = copy(accountScripts = Option(__v))
def clearAccountData = copy(accountData = _root_.scala.Seq.empty)
def addAccountData(__vs: com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountData *): TransactionStateSnapshot = addAllAccountData(__vs)
def addAllAccountData(__vs: Iterable[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountData]): TransactionStateSnapshot = copy(accountData = accountData ++ __vs)
def withAccountData(__v: _root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountData]): TransactionStateSnapshot = copy(accountData = __v)
def clearSponsorships = copy(sponsorships = _root_.scala.Seq.empty)
def addSponsorships(__vs: com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Sponsorship *): TransactionStateSnapshot = addAllSponsorships(__vs)
def addAllSponsorships(__vs: Iterable[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Sponsorship]): TransactionStateSnapshot = copy(sponsorships = sponsorships ++ __vs)
def withSponsorships(__v: _root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Sponsorship]): TransactionStateSnapshot = copy(sponsorships = __v)
def withTransactionStatus(__v: com.wavesplatform.protobuf.snapshot.TransactionStatus): TransactionStateSnapshot = copy(transactionStatus = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => balances
case 2 => leaseBalances
case 3 => newLeases
case 4 => cancelledLeases
case 5 => assetStatics
case 6 => assetVolumes
case 7 => assetNamesAndDescriptions
case 8 => assetScripts.orNull
case 9 => aliases.orNull
case 10 => orderFills
case 11 => accountScripts.orNull
case 12 => accountData
case 13 => sponsorships
case 14 => {
val __t = transactionStatus.javaValueDescriptor
if (__t.getNumber() != 0) __t else null
}
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PRepeated(balances.iterator.map(_.toPMessage).toVector)
case 2 => _root_.scalapb.descriptors.PRepeated(leaseBalances.iterator.map(_.toPMessage).toVector)
case 3 => _root_.scalapb.descriptors.PRepeated(newLeases.iterator.map(_.toPMessage).toVector)
case 4 => _root_.scalapb.descriptors.PRepeated(cancelledLeases.iterator.map(_.toPMessage).toVector)
case 5 => _root_.scalapb.descriptors.PRepeated(assetStatics.iterator.map(_.toPMessage).toVector)
case 6 => _root_.scalapb.descriptors.PRepeated(assetVolumes.iterator.map(_.toPMessage).toVector)
case 7 => _root_.scalapb.descriptors.PRepeated(assetNamesAndDescriptions.iterator.map(_.toPMessage).toVector)
case 8 => assetScripts.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 9 => aliases.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 10 => _root_.scalapb.descriptors.PRepeated(orderFills.iterator.map(_.toPMessage).toVector)
case 11 => accountScripts.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 12 => _root_.scalapb.descriptors.PRepeated(accountData.iterator.map(_.toPMessage).toVector)
case 13 => _root_.scalapb.descriptors.PRepeated(sponsorships.iterator.map(_.toPMessage).toVector)
case 14 => _root_.scalapb.descriptors.PEnum(transactionStatus.scalaValueDescriptor)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.type = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot
// @@protoc_insertion_point(GeneratedMessage[waves.TransactionStateSnapshot])
}
object TransactionStateSnapshot extends scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot = {
val __balances: _root_.scala.collection.immutable.VectorBuilder[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Balance] = new _root_.scala.collection.immutable.VectorBuilder[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Balance]
val __leaseBalances: _root_.scala.collection.immutable.VectorBuilder[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.LeaseBalance] = new _root_.scala.collection.immutable.VectorBuilder[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.LeaseBalance]
val __newLeases: _root_.scala.collection.immutable.VectorBuilder[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewLease] = new _root_.scala.collection.immutable.VectorBuilder[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewLease]
val __cancelledLeases: _root_.scala.collection.immutable.VectorBuilder[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.CancelledLease] = new _root_.scala.collection.immutable.VectorBuilder[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.CancelledLease]
val __assetStatics: _root_.scala.collection.immutable.VectorBuilder[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewAsset] = new _root_.scala.collection.immutable.VectorBuilder[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewAsset]
val __assetVolumes: _root_.scala.collection.immutable.VectorBuilder[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetVolume] = new _root_.scala.collection.immutable.VectorBuilder[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetVolume]
val __assetNamesAndDescriptions: _root_.scala.collection.immutable.VectorBuilder[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetNameAndDescription] = new _root_.scala.collection.immutable.VectorBuilder[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetNameAndDescription]
var __assetScripts: _root_.scala.Option[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetScript] = _root_.scala.None
var __aliases: _root_.scala.Option[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Alias] = _root_.scala.None
val __orderFills: _root_.scala.collection.immutable.VectorBuilder[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.OrderFill] = new _root_.scala.collection.immutable.VectorBuilder[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.OrderFill]
var __accountScripts: _root_.scala.Option[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountScript] = _root_.scala.None
val __accountData: _root_.scala.collection.immutable.VectorBuilder[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountData] = new _root_.scala.collection.immutable.VectorBuilder[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountData]
val __sponsorships: _root_.scala.collection.immutable.VectorBuilder[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Sponsorship] = new _root_.scala.collection.immutable.VectorBuilder[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Sponsorship]
var __transactionStatus: com.wavesplatform.protobuf.snapshot.TransactionStatus = com.wavesplatform.protobuf.snapshot.TransactionStatus.SUCCEEDED
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__balances += _root_.scalapb.LiteParser.readMessage[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Balance](_input__)
case 18 =>
__leaseBalances += _root_.scalapb.LiteParser.readMessage[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.LeaseBalance](_input__)
case 26 =>
__newLeases += _root_.scalapb.LiteParser.readMessage[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewLease](_input__)
case 34 =>
__cancelledLeases += _root_.scalapb.LiteParser.readMessage[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.CancelledLease](_input__)
case 42 =>
__assetStatics += _root_.scalapb.LiteParser.readMessage[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewAsset](_input__)
case 50 =>
__assetVolumes += _root_.scalapb.LiteParser.readMessage[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetVolume](_input__)
case 58 =>
__assetNamesAndDescriptions += _root_.scalapb.LiteParser.readMessage[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetNameAndDescription](_input__)
case 66 =>
__assetScripts = _root_.scala.Option(__assetScripts.fold(_root_.scalapb.LiteParser.readMessage[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetScript](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case 74 =>
__aliases = _root_.scala.Option(__aliases.fold(_root_.scalapb.LiteParser.readMessage[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Alias](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case 82 =>
__orderFills += _root_.scalapb.LiteParser.readMessage[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.OrderFill](_input__)
case 90 =>
__accountScripts = _root_.scala.Option(__accountScripts.fold(_root_.scalapb.LiteParser.readMessage[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountScript](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case 98 =>
__accountData += _root_.scalapb.LiteParser.readMessage[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountData](_input__)
case 106 =>
__sponsorships += _root_.scalapb.LiteParser.readMessage[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Sponsorship](_input__)
case 112 =>
__transactionStatus = com.wavesplatform.protobuf.snapshot.TransactionStatus.fromValue(_input__.readEnum())
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot(
balances = __balances.result(),
leaseBalances = __leaseBalances.result(),
newLeases = __newLeases.result(),
cancelledLeases = __cancelledLeases.result(),
assetStatics = __assetStatics.result(),
assetVolumes = __assetVolumes.result(),
assetNamesAndDescriptions = __assetNamesAndDescriptions.result(),
assetScripts = __assetScripts,
aliases = __aliases,
orderFills = __orderFills.result(),
accountScripts = __accountScripts,
accountData = __accountData.result(),
sponsorships = __sponsorships.result(),
transactionStatus = __transactionStatus,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot(
balances = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Balance]]).getOrElse(_root_.scala.Seq.empty),
leaseBalances = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).map(_.as[_root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.LeaseBalance]]).getOrElse(_root_.scala.Seq.empty),
newLeases = __fieldsMap.get(scalaDescriptor.findFieldByNumber(3).get).map(_.as[_root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewLease]]).getOrElse(_root_.scala.Seq.empty),
cancelledLeases = __fieldsMap.get(scalaDescriptor.findFieldByNumber(4).get).map(_.as[_root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.CancelledLease]]).getOrElse(_root_.scala.Seq.empty),
assetStatics = __fieldsMap.get(scalaDescriptor.findFieldByNumber(5).get).map(_.as[_root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewAsset]]).getOrElse(_root_.scala.Seq.empty),
assetVolumes = __fieldsMap.get(scalaDescriptor.findFieldByNumber(6).get).map(_.as[_root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetVolume]]).getOrElse(_root_.scala.Seq.empty),
assetNamesAndDescriptions = __fieldsMap.get(scalaDescriptor.findFieldByNumber(7).get).map(_.as[_root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetNameAndDescription]]).getOrElse(_root_.scala.Seq.empty),
assetScripts = __fieldsMap.get(scalaDescriptor.findFieldByNumber(8).get).flatMap(_.as[_root_.scala.Option[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetScript]]),
aliases = __fieldsMap.get(scalaDescriptor.findFieldByNumber(9).get).flatMap(_.as[_root_.scala.Option[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Alias]]),
orderFills = __fieldsMap.get(scalaDescriptor.findFieldByNumber(10).get).map(_.as[_root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.OrderFill]]).getOrElse(_root_.scala.Seq.empty),
accountScripts = __fieldsMap.get(scalaDescriptor.findFieldByNumber(11).get).flatMap(_.as[_root_.scala.Option[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountScript]]),
accountData = __fieldsMap.get(scalaDescriptor.findFieldByNumber(12).get).map(_.as[_root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountData]]).getOrElse(_root_.scala.Seq.empty),
sponsorships = __fieldsMap.get(scalaDescriptor.findFieldByNumber(13).get).map(_.as[_root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Sponsorship]]).getOrElse(_root_.scala.Seq.empty),
transactionStatus = com.wavesplatform.protobuf.snapshot.TransactionStatus.fromValue(__fieldsMap.get(scalaDescriptor.findFieldByNumber(14).get).map(_.as[_root_.scalapb.descriptors.EnumValueDescriptor]).getOrElse(com.wavesplatform.protobuf.snapshot.TransactionStatus.SUCCEEDED.scalaValueDescriptor).number)
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = TransactionStateSnapshotProto.javaDescriptor.getMessageTypes().get(0)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = TransactionStateSnapshotProto.scalaDescriptor.messages(0)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = {
var __out: _root_.scalapb.GeneratedMessageCompanion[_] = null
(__number: @_root_.scala.unchecked) match {
case 1 => __out = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Balance
case 2 => __out = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.LeaseBalance
case 3 => __out = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewLease
case 4 => __out = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.CancelledLease
case 5 => __out = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewAsset
case 6 => __out = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetVolume
case 7 => __out = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetNameAndDescription
case 8 => __out = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetScript
case 9 => __out = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Alias
case 10 => __out = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.OrderFill
case 11 => __out = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountScript
case 12 => __out = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountData
case 13 => __out = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Sponsorship
}
__out
}
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] =
Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]](
_root_.com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Balance,
_root_.com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.LeaseBalance,
_root_.com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewLease,
_root_.com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.CancelledLease,
_root_.com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewAsset,
_root_.com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetVolume,
_root_.com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetNameAndDescription,
_root_.com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetScript,
_root_.com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Alias,
_root_.com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.OrderFill,
_root_.com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountScript,
_root_.com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountData,
_root_.com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Sponsorship
)
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 14 => com.wavesplatform.protobuf.snapshot.TransactionStatus
}
}
lazy val defaultInstance = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot(
balances = _root_.scala.Seq.empty,
leaseBalances = _root_.scala.Seq.empty,
newLeases = _root_.scala.Seq.empty,
cancelledLeases = _root_.scala.Seq.empty,
assetStatics = _root_.scala.Seq.empty,
assetVolumes = _root_.scala.Seq.empty,
assetNamesAndDescriptions = _root_.scala.Seq.empty,
assetScripts = _root_.scala.None,
aliases = _root_.scala.None,
orderFills = _root_.scala.Seq.empty,
accountScripts = _root_.scala.None,
accountData = _root_.scala.Seq.empty,
sponsorships = _root_.scala.Seq.empty,
transactionStatus = com.wavesplatform.protobuf.snapshot.TransactionStatus.SUCCEEDED
)
@SerialVersionUID(0L)
final case class Balance(
address: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
amount: _root_.scala.Option[com.wavesplatform.protobuf.Amount] = _root_.scala.None,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[Balance] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = address
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(1, __value)
}
};
if (amount.isDefined) {
val __value = amount.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = address
if (!__v.isEmpty) {
_output__.writeBytes(1, __v)
}
};
amount.foreach { __v =>
val __m = __v
_output__.writeTag(2, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
unknownFields.writeTo(_output__)
}
def withAddress(__v: _root_.com.google.protobuf.ByteString): Balance = copy(address = __v)
def getAmount: com.wavesplatform.protobuf.Amount = amount.getOrElse(com.wavesplatform.protobuf.Amount.defaultInstance)
def clearAmount: Balance = copy(amount = _root_.scala.None)
def withAmount(__v: com.wavesplatform.protobuf.Amount): Balance = copy(amount = Option(__v))
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = address
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
case 2 => amount.orNull
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PByteString(address)
case 2 => amount.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Balance.type = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Balance
// @@protoc_insertion_point(GeneratedMessage[waves.TransactionStateSnapshot.Balance])
}
object Balance extends scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Balance] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Balance] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Balance = {
var __address: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var __amount: _root_.scala.Option[com.wavesplatform.protobuf.Amount] = _root_.scala.None
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__address = _input__.readBytes()
case 18 =>
__amount = _root_.scala.Option(__amount.fold(_root_.scalapb.LiteParser.readMessage[com.wavesplatform.protobuf.Amount](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Balance(
address = __address,
amount = __amount,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Balance] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Balance(
address = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY),
amount = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).flatMap(_.as[_root_.scala.Option[com.wavesplatform.protobuf.Amount]])
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.javaDescriptor.getNestedTypes().get(0)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.scalaDescriptor.nestedMessages(0)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = {
var __out: _root_.scalapb.GeneratedMessageCompanion[_] = null
(__number: @_root_.scala.unchecked) match {
case 2 => __out = com.wavesplatform.protobuf.Amount
}
__out
}
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] = Seq.empty
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Balance(
address = _root_.com.google.protobuf.ByteString.EMPTY,
amount = _root_.scala.None
)
implicit class BalanceLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Balance]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Balance](_l) {
def address: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.address)((c_, f_) => c_.copy(address = f_))
def amount: _root_.scalapb.lenses.Lens[UpperPB, com.wavesplatform.protobuf.Amount] = field(_.getAmount)((c_, f_) => c_.copy(amount = _root_.scala.Option(f_)))
def optionalAmount: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[com.wavesplatform.protobuf.Amount]] = field(_.amount)((c_, f_) => c_.copy(amount = f_))
}
final val ADDRESS_FIELD_NUMBER = 1
final val AMOUNT_FIELD_NUMBER = 2
def of(
address: _root_.com.google.protobuf.ByteString,
amount: _root_.scala.Option[com.wavesplatform.protobuf.Amount]
): _root_.com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Balance = _root_.com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Balance(
address,
amount
)
// @@protoc_insertion_point(GeneratedMessageCompanion[waves.TransactionStateSnapshot.Balance])
}
@SerialVersionUID(0L)
final case class LeaseBalance(
address: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
in: _root_.scala.Long = 0L,
out: _root_.scala.Long = 0L,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[LeaseBalance] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = address
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(1, __value)
}
};
{
val __value = in
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt64Size(2, __value)
}
};
{
val __value = out
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt64Size(3, __value)
}
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = address
if (!__v.isEmpty) {
_output__.writeBytes(1, __v)
}
};
{
val __v = in
if (__v != 0L) {
_output__.writeInt64(2, __v)
}
};
{
val __v = out
if (__v != 0L) {
_output__.writeInt64(3, __v)
}
};
unknownFields.writeTo(_output__)
}
def withAddress(__v: _root_.com.google.protobuf.ByteString): LeaseBalance = copy(address = __v)
def withIn(__v: _root_.scala.Long): LeaseBalance = copy(in = __v)
def withOut(__v: _root_.scala.Long): LeaseBalance = copy(out = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = address
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
case 2 => {
val __t = in
if (__t != 0L) __t else null
}
case 3 => {
val __t = out
if (__t != 0L) __t else null
}
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PByteString(address)
case 2 => _root_.scalapb.descriptors.PLong(in)
case 3 => _root_.scalapb.descriptors.PLong(out)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.LeaseBalance.type = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.LeaseBalance
// @@protoc_insertion_point(GeneratedMessage[waves.TransactionStateSnapshot.LeaseBalance])
}
object LeaseBalance extends scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.LeaseBalance] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.LeaseBalance] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.LeaseBalance = {
var __address: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var __in: _root_.scala.Long = 0L
var __out: _root_.scala.Long = 0L
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__address = _input__.readBytes()
case 16 =>
__in = _input__.readInt64()
case 24 =>
__out = _input__.readInt64()
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.LeaseBalance(
address = __address,
in = __in,
out = __out,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.LeaseBalance] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.LeaseBalance(
address = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY),
in = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).map(_.as[_root_.scala.Long]).getOrElse(0L),
out = __fieldsMap.get(scalaDescriptor.findFieldByNumber(3).get).map(_.as[_root_.scala.Long]).getOrElse(0L)
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.javaDescriptor.getNestedTypes().get(1)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.scalaDescriptor.nestedMessages(1)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = throw new MatchError(__number)
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] = Seq.empty
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.LeaseBalance(
address = _root_.com.google.protobuf.ByteString.EMPTY,
in = 0L,
out = 0L
)
implicit class LeaseBalanceLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.LeaseBalance]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.LeaseBalance](_l) {
def address: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.address)((c_, f_) => c_.copy(address = f_))
def in: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.in)((c_, f_) => c_.copy(in = f_))
def out: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.out)((c_, f_) => c_.copy(out = f_))
}
final val ADDRESS_FIELD_NUMBER = 1
final val IN_FIELD_NUMBER = 2
final val OUT_FIELD_NUMBER = 3
def of(
address: _root_.com.google.protobuf.ByteString,
in: _root_.scala.Long,
out: _root_.scala.Long
): _root_.com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.LeaseBalance = _root_.com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.LeaseBalance(
address,
in,
out
)
// @@protoc_insertion_point(GeneratedMessageCompanion[waves.TransactionStateSnapshot.LeaseBalance])
}
@SerialVersionUID(0L)
final case class NewLease(
leaseId: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
senderPublicKey: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
recipientAddress: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
amount: _root_.scala.Long = 0L,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[NewLease] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = leaseId
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(1, __value)
}
};
{
val __value = senderPublicKey
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(2, __value)
}
};
{
val __value = recipientAddress
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(3, __value)
}
};
{
val __value = amount
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt64Size(4, __value)
}
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = leaseId
if (!__v.isEmpty) {
_output__.writeBytes(1, __v)
}
};
{
val __v = senderPublicKey
if (!__v.isEmpty) {
_output__.writeBytes(2, __v)
}
};
{
val __v = recipientAddress
if (!__v.isEmpty) {
_output__.writeBytes(3, __v)
}
};
{
val __v = amount
if (__v != 0L) {
_output__.writeInt64(4, __v)
}
};
unknownFields.writeTo(_output__)
}
def withLeaseId(__v: _root_.com.google.protobuf.ByteString): NewLease = copy(leaseId = __v)
def withSenderPublicKey(__v: _root_.com.google.protobuf.ByteString): NewLease = copy(senderPublicKey = __v)
def withRecipientAddress(__v: _root_.com.google.protobuf.ByteString): NewLease = copy(recipientAddress = __v)
def withAmount(__v: _root_.scala.Long): NewLease = copy(amount = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = leaseId
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
case 2 => {
val __t = senderPublicKey
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
case 3 => {
val __t = recipientAddress
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
case 4 => {
val __t = amount
if (__t != 0L) __t else null
}
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PByteString(leaseId)
case 2 => _root_.scalapb.descriptors.PByteString(senderPublicKey)
case 3 => _root_.scalapb.descriptors.PByteString(recipientAddress)
case 4 => _root_.scalapb.descriptors.PLong(amount)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewLease.type = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewLease
// @@protoc_insertion_point(GeneratedMessage[waves.TransactionStateSnapshot.NewLease])
}
object NewLease extends scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewLease] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewLease] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewLease = {
var __leaseId: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var __senderPublicKey: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var __recipientAddress: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var __amount: _root_.scala.Long = 0L
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__leaseId = _input__.readBytes()
case 18 =>
__senderPublicKey = _input__.readBytes()
case 26 =>
__recipientAddress = _input__.readBytes()
case 32 =>
__amount = _input__.readInt64()
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewLease(
leaseId = __leaseId,
senderPublicKey = __senderPublicKey,
recipientAddress = __recipientAddress,
amount = __amount,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewLease] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewLease(
leaseId = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY),
senderPublicKey = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY),
recipientAddress = __fieldsMap.get(scalaDescriptor.findFieldByNumber(3).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY),
amount = __fieldsMap.get(scalaDescriptor.findFieldByNumber(4).get).map(_.as[_root_.scala.Long]).getOrElse(0L)
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.javaDescriptor.getNestedTypes().get(2)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.scalaDescriptor.nestedMessages(2)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = throw new MatchError(__number)
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] = Seq.empty
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewLease(
leaseId = _root_.com.google.protobuf.ByteString.EMPTY,
senderPublicKey = _root_.com.google.protobuf.ByteString.EMPTY,
recipientAddress = _root_.com.google.protobuf.ByteString.EMPTY,
amount = 0L
)
implicit class NewLeaseLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewLease]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewLease](_l) {
def leaseId: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.leaseId)((c_, f_) => c_.copy(leaseId = f_))
def senderPublicKey: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.senderPublicKey)((c_, f_) => c_.copy(senderPublicKey = f_))
def recipientAddress: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.recipientAddress)((c_, f_) => c_.copy(recipientAddress = f_))
def amount: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.amount)((c_, f_) => c_.copy(amount = f_))
}
final val LEASE_ID_FIELD_NUMBER = 1
final val SENDER_PUBLIC_KEY_FIELD_NUMBER = 2
final val RECIPIENT_ADDRESS_FIELD_NUMBER = 3
final val AMOUNT_FIELD_NUMBER = 4
def of(
leaseId: _root_.com.google.protobuf.ByteString,
senderPublicKey: _root_.com.google.protobuf.ByteString,
recipientAddress: _root_.com.google.protobuf.ByteString,
amount: _root_.scala.Long
): _root_.com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewLease = _root_.com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewLease(
leaseId,
senderPublicKey,
recipientAddress,
amount
)
// @@protoc_insertion_point(GeneratedMessageCompanion[waves.TransactionStateSnapshot.NewLease])
}
@SerialVersionUID(0L)
final case class CancelledLease(
leaseId: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[CancelledLease] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = leaseId
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(1, __value)
}
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = leaseId
if (!__v.isEmpty) {
_output__.writeBytes(1, __v)
}
};
unknownFields.writeTo(_output__)
}
def withLeaseId(__v: _root_.com.google.protobuf.ByteString): CancelledLease = copy(leaseId = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = leaseId
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PByteString(leaseId)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.CancelledLease.type = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.CancelledLease
// @@protoc_insertion_point(GeneratedMessage[waves.TransactionStateSnapshot.CancelledLease])
}
object CancelledLease extends scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.CancelledLease] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.CancelledLease] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.CancelledLease = {
var __leaseId: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__leaseId = _input__.readBytes()
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.CancelledLease(
leaseId = __leaseId,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.CancelledLease] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.CancelledLease(
leaseId = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY)
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.javaDescriptor.getNestedTypes().get(3)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.scalaDescriptor.nestedMessages(3)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = throw new MatchError(__number)
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] = Seq.empty
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.CancelledLease(
leaseId = _root_.com.google.protobuf.ByteString.EMPTY
)
implicit class CancelledLeaseLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.CancelledLease]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.CancelledLease](_l) {
def leaseId: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.leaseId)((c_, f_) => c_.copy(leaseId = f_))
}
final val LEASE_ID_FIELD_NUMBER = 1
def of(
leaseId: _root_.com.google.protobuf.ByteString
): _root_.com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.CancelledLease = _root_.com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.CancelledLease(
leaseId
)
// @@protoc_insertion_point(GeneratedMessageCompanion[waves.TransactionStateSnapshot.CancelledLease])
}
@SerialVersionUID(0L)
final case class NewAsset(
assetId: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
issuerPublicKey: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
decimals: _root_.scala.Int = 0,
nft: _root_.scala.Boolean = false,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[NewAsset] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = assetId
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(1, __value)
}
};
{
val __value = issuerPublicKey
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(2, __value)
}
};
{
val __value = decimals
if (__value != 0) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt32Size(3, __value)
}
};
{
val __value = nft
if (__value != false) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBoolSize(4, __value)
}
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = assetId
if (!__v.isEmpty) {
_output__.writeBytes(1, __v)
}
};
{
val __v = issuerPublicKey
if (!__v.isEmpty) {
_output__.writeBytes(2, __v)
}
};
{
val __v = decimals
if (__v != 0) {
_output__.writeInt32(3, __v)
}
};
{
val __v = nft
if (__v != false) {
_output__.writeBool(4, __v)
}
};
unknownFields.writeTo(_output__)
}
def withAssetId(__v: _root_.com.google.protobuf.ByteString): NewAsset = copy(assetId = __v)
def withIssuerPublicKey(__v: _root_.com.google.protobuf.ByteString): NewAsset = copy(issuerPublicKey = __v)
def withDecimals(__v: _root_.scala.Int): NewAsset = copy(decimals = __v)
def withNft(__v: _root_.scala.Boolean): NewAsset = copy(nft = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = assetId
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
case 2 => {
val __t = issuerPublicKey
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
case 3 => {
val __t = decimals
if (__t != 0) __t else null
}
case 4 => {
val __t = nft
if (__t != false) __t else null
}
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PByteString(assetId)
case 2 => _root_.scalapb.descriptors.PByteString(issuerPublicKey)
case 3 => _root_.scalapb.descriptors.PInt(decimals)
case 4 => _root_.scalapb.descriptors.PBoolean(nft)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewAsset.type = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewAsset
// @@protoc_insertion_point(GeneratedMessage[waves.TransactionStateSnapshot.NewAsset])
}
object NewAsset extends scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewAsset] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewAsset] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewAsset = {
var __assetId: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var __issuerPublicKey: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var __decimals: _root_.scala.Int = 0
var __nft: _root_.scala.Boolean = false
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__assetId = _input__.readBytes()
case 18 =>
__issuerPublicKey = _input__.readBytes()
case 24 =>
__decimals = _input__.readInt32()
case 32 =>
__nft = _input__.readBool()
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewAsset(
assetId = __assetId,
issuerPublicKey = __issuerPublicKey,
decimals = __decimals,
nft = __nft,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewAsset] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewAsset(
assetId = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY),
issuerPublicKey = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY),
decimals = __fieldsMap.get(scalaDescriptor.findFieldByNumber(3).get).map(_.as[_root_.scala.Int]).getOrElse(0),
nft = __fieldsMap.get(scalaDescriptor.findFieldByNumber(4).get).map(_.as[_root_.scala.Boolean]).getOrElse(false)
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.javaDescriptor.getNestedTypes().get(4)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.scalaDescriptor.nestedMessages(4)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = throw new MatchError(__number)
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] = Seq.empty
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewAsset(
assetId = _root_.com.google.protobuf.ByteString.EMPTY,
issuerPublicKey = _root_.com.google.protobuf.ByteString.EMPTY,
decimals = 0,
nft = false
)
implicit class NewAssetLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewAsset]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewAsset](_l) {
def assetId: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.assetId)((c_, f_) => c_.copy(assetId = f_))
def issuerPublicKey: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.issuerPublicKey)((c_, f_) => c_.copy(issuerPublicKey = f_))
def decimals: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Int] = field(_.decimals)((c_, f_) => c_.copy(decimals = f_))
def nft: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Boolean] = field(_.nft)((c_, f_) => c_.copy(nft = f_))
}
final val ASSET_ID_FIELD_NUMBER = 1
final val ISSUER_PUBLIC_KEY_FIELD_NUMBER = 2
final val DECIMALS_FIELD_NUMBER = 3
final val NFT_FIELD_NUMBER = 4
def of(
assetId: _root_.com.google.protobuf.ByteString,
issuerPublicKey: _root_.com.google.protobuf.ByteString,
decimals: _root_.scala.Int,
nft: _root_.scala.Boolean
): _root_.com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewAsset = _root_.com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewAsset(
assetId,
issuerPublicKey,
decimals,
nft
)
// @@protoc_insertion_point(GeneratedMessageCompanion[waves.TransactionStateSnapshot.NewAsset])
}
@SerialVersionUID(0L)
final case class AssetVolume(
assetId: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
reissuable: _root_.scala.Boolean = false,
volume: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[AssetVolume] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = assetId
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(1, __value)
}
};
{
val __value = reissuable
if (__value != false) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBoolSize(2, __value)
}
};
{
val __value = volume
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(3, __value)
}
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = assetId
if (!__v.isEmpty) {
_output__.writeBytes(1, __v)
}
};
{
val __v = reissuable
if (__v != false) {
_output__.writeBool(2, __v)
}
};
{
val __v = volume
if (!__v.isEmpty) {
_output__.writeBytes(3, __v)
}
};
unknownFields.writeTo(_output__)
}
def withAssetId(__v: _root_.com.google.protobuf.ByteString): AssetVolume = copy(assetId = __v)
def withReissuable(__v: _root_.scala.Boolean): AssetVolume = copy(reissuable = __v)
def withVolume(__v: _root_.com.google.protobuf.ByteString): AssetVolume = copy(volume = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = assetId
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
case 2 => {
val __t = reissuable
if (__t != false) __t else null
}
case 3 => {
val __t = volume
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PByteString(assetId)
case 2 => _root_.scalapb.descriptors.PBoolean(reissuable)
case 3 => _root_.scalapb.descriptors.PByteString(volume)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetVolume.type = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetVolume
// @@protoc_insertion_point(GeneratedMessage[waves.TransactionStateSnapshot.AssetVolume])
}
object AssetVolume extends scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetVolume] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetVolume] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetVolume = {
var __assetId: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var __reissuable: _root_.scala.Boolean = false
var __volume: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__assetId = _input__.readBytes()
case 16 =>
__reissuable = _input__.readBool()
case 26 =>
__volume = _input__.readBytes()
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetVolume(
assetId = __assetId,
reissuable = __reissuable,
volume = __volume,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetVolume] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetVolume(
assetId = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY),
reissuable = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).map(_.as[_root_.scala.Boolean]).getOrElse(false),
volume = __fieldsMap.get(scalaDescriptor.findFieldByNumber(3).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY)
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.javaDescriptor.getNestedTypes().get(5)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.scalaDescriptor.nestedMessages(5)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = throw new MatchError(__number)
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] = Seq.empty
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetVolume(
assetId = _root_.com.google.protobuf.ByteString.EMPTY,
reissuable = false,
volume = _root_.com.google.protobuf.ByteString.EMPTY
)
implicit class AssetVolumeLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetVolume]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetVolume](_l) {
def assetId: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.assetId)((c_, f_) => c_.copy(assetId = f_))
def reissuable: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Boolean] = field(_.reissuable)((c_, f_) => c_.copy(reissuable = f_))
def volume: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.volume)((c_, f_) => c_.copy(volume = f_))
}
final val ASSET_ID_FIELD_NUMBER = 1
final val REISSUABLE_FIELD_NUMBER = 2
final val VOLUME_FIELD_NUMBER = 3
def of(
assetId: _root_.com.google.protobuf.ByteString,
reissuable: _root_.scala.Boolean,
volume: _root_.com.google.protobuf.ByteString
): _root_.com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetVolume = _root_.com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetVolume(
assetId,
reissuable,
volume
)
// @@protoc_insertion_point(GeneratedMessageCompanion[waves.TransactionStateSnapshot.AssetVolume])
}
@SerialVersionUID(0L)
final case class AssetNameAndDescription(
assetId: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
name: _root_.scala.Predef.String = "",
description: _root_.scala.Predef.String = "",
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[AssetNameAndDescription] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = assetId
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(1, __value)
}
};
{
val __value = name
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(2, __value)
}
};
{
val __value = description
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(3, __value)
}
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = assetId
if (!__v.isEmpty) {
_output__.writeBytes(1, __v)
}
};
{
val __v = name
if (!__v.isEmpty) {
_output__.writeString(2, __v)
}
};
{
val __v = description
if (!__v.isEmpty) {
_output__.writeString(3, __v)
}
};
unknownFields.writeTo(_output__)
}
def withAssetId(__v: _root_.com.google.protobuf.ByteString): AssetNameAndDescription = copy(assetId = __v)
def withName(__v: _root_.scala.Predef.String): AssetNameAndDescription = copy(name = __v)
def withDescription(__v: _root_.scala.Predef.String): AssetNameAndDescription = copy(description = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = assetId
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
case 2 => {
val __t = name
if (__t != "") __t else null
}
case 3 => {
val __t = description
if (__t != "") __t else null
}
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PByteString(assetId)
case 2 => _root_.scalapb.descriptors.PString(name)
case 3 => _root_.scalapb.descriptors.PString(description)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetNameAndDescription.type = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetNameAndDescription
// @@protoc_insertion_point(GeneratedMessage[waves.TransactionStateSnapshot.AssetNameAndDescription])
}
object AssetNameAndDescription extends scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetNameAndDescription] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetNameAndDescription] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetNameAndDescription = {
var __assetId: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var __name: _root_.scala.Predef.String = ""
var __description: _root_.scala.Predef.String = ""
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__assetId = _input__.readBytes()
case 18 =>
__name = _input__.readStringRequireUtf8()
case 26 =>
__description = _input__.readStringRequireUtf8()
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetNameAndDescription(
assetId = __assetId,
name = __name,
description = __description,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetNameAndDescription] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetNameAndDescription(
assetId = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY),
name = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).map(_.as[_root_.scala.Predef.String]).getOrElse(""),
description = __fieldsMap.get(scalaDescriptor.findFieldByNumber(3).get).map(_.as[_root_.scala.Predef.String]).getOrElse("")
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.javaDescriptor.getNestedTypes().get(6)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.scalaDescriptor.nestedMessages(6)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = throw new MatchError(__number)
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] = Seq.empty
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetNameAndDescription(
assetId = _root_.com.google.protobuf.ByteString.EMPTY,
name = "",
description = ""
)
implicit class AssetNameAndDescriptionLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetNameAndDescription]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetNameAndDescription](_l) {
def assetId: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.assetId)((c_, f_) => c_.copy(assetId = f_))
def name: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.name)((c_, f_) => c_.copy(name = f_))
def description: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.description)((c_, f_) => c_.copy(description = f_))
}
final val ASSET_ID_FIELD_NUMBER = 1
final val NAME_FIELD_NUMBER = 2
final val DESCRIPTION_FIELD_NUMBER = 3
def of(
assetId: _root_.com.google.protobuf.ByteString,
name: _root_.scala.Predef.String,
description: _root_.scala.Predef.String
): _root_.com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetNameAndDescription = _root_.com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetNameAndDescription(
assetId,
name,
description
)
// @@protoc_insertion_point(GeneratedMessageCompanion[waves.TransactionStateSnapshot.AssetNameAndDescription])
}
@SerialVersionUID(0L)
final case class AssetScript(
assetId: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
script: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[AssetScript] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = assetId
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(1, __value)
}
};
{
val __value = script
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(2, __value)
}
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = assetId
if (!__v.isEmpty) {
_output__.writeBytes(1, __v)
}
};
{
val __v = script
if (!__v.isEmpty) {
_output__.writeBytes(2, __v)
}
};
unknownFields.writeTo(_output__)
}
def withAssetId(__v: _root_.com.google.protobuf.ByteString): AssetScript = copy(assetId = __v)
def withScript(__v: _root_.com.google.protobuf.ByteString): AssetScript = copy(script = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = assetId
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
case 2 => {
val __t = script
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PByteString(assetId)
case 2 => _root_.scalapb.descriptors.PByteString(script)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetScript.type = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetScript
// @@protoc_insertion_point(GeneratedMessage[waves.TransactionStateSnapshot.AssetScript])
}
object AssetScript extends scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetScript] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetScript] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetScript = {
var __assetId: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var __script: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__assetId = _input__.readBytes()
case 18 =>
__script = _input__.readBytes()
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetScript(
assetId = __assetId,
script = __script,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetScript] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetScript(
assetId = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY),
script = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY)
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.javaDescriptor.getNestedTypes().get(7)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.scalaDescriptor.nestedMessages(7)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = throw new MatchError(__number)
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] = Seq.empty
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetScript(
assetId = _root_.com.google.protobuf.ByteString.EMPTY,
script = _root_.com.google.protobuf.ByteString.EMPTY
)
implicit class AssetScriptLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetScript]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetScript](_l) {
def assetId: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.assetId)((c_, f_) => c_.copy(assetId = f_))
def script: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.script)((c_, f_) => c_.copy(script = f_))
}
final val ASSET_ID_FIELD_NUMBER = 1
final val SCRIPT_FIELD_NUMBER = 2
def of(
assetId: _root_.com.google.protobuf.ByteString,
script: _root_.com.google.protobuf.ByteString
): _root_.com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetScript = _root_.com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetScript(
assetId,
script
)
// @@protoc_insertion_point(GeneratedMessageCompanion[waves.TransactionStateSnapshot.AssetScript])
}
@SerialVersionUID(0L)
final case class Alias(
address: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
alias: _root_.scala.Predef.String = "",
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[Alias] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = address
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(1, __value)
}
};
{
val __value = alias
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(2, __value)
}
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = address
if (!__v.isEmpty) {
_output__.writeBytes(1, __v)
}
};
{
val __v = alias
if (!__v.isEmpty) {
_output__.writeString(2, __v)
}
};
unknownFields.writeTo(_output__)
}
def withAddress(__v: _root_.com.google.protobuf.ByteString): Alias = copy(address = __v)
def withAlias(__v: _root_.scala.Predef.String): Alias = copy(alias = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = address
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
case 2 => {
val __t = alias
if (__t != "") __t else null
}
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PByteString(address)
case 2 => _root_.scalapb.descriptors.PString(alias)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Alias.type = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Alias
// @@protoc_insertion_point(GeneratedMessage[waves.TransactionStateSnapshot.Alias])
}
object Alias extends scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Alias] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Alias] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Alias = {
var __address: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var __alias: _root_.scala.Predef.String = ""
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__address = _input__.readBytes()
case 18 =>
__alias = _input__.readStringRequireUtf8()
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Alias(
address = __address,
alias = __alias,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Alias] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Alias(
address = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY),
alias = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).map(_.as[_root_.scala.Predef.String]).getOrElse("")
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.javaDescriptor.getNestedTypes().get(8)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.scalaDescriptor.nestedMessages(8)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = throw new MatchError(__number)
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] = Seq.empty
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Alias(
address = _root_.com.google.protobuf.ByteString.EMPTY,
alias = ""
)
implicit class AliasLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Alias]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Alias](_l) {
def address: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.address)((c_, f_) => c_.copy(address = f_))
def alias: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.alias)((c_, f_) => c_.copy(alias = f_))
}
final val ADDRESS_FIELD_NUMBER = 1
final val ALIAS_FIELD_NUMBER = 2
def of(
address: _root_.com.google.protobuf.ByteString,
alias: _root_.scala.Predef.String
): _root_.com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Alias = _root_.com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Alias(
address,
alias
)
// @@protoc_insertion_point(GeneratedMessageCompanion[waves.TransactionStateSnapshot.Alias])
}
@SerialVersionUID(0L)
final case class OrderFill(
orderId: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
volume: _root_.scala.Long = 0L,
fee: _root_.scala.Long = 0L,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[OrderFill] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = orderId
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(1, __value)
}
};
{
val __value = volume
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt64Size(2, __value)
}
};
{
val __value = fee
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt64Size(3, __value)
}
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = orderId
if (!__v.isEmpty) {
_output__.writeBytes(1, __v)
}
};
{
val __v = volume
if (__v != 0L) {
_output__.writeInt64(2, __v)
}
};
{
val __v = fee
if (__v != 0L) {
_output__.writeInt64(3, __v)
}
};
unknownFields.writeTo(_output__)
}
def withOrderId(__v: _root_.com.google.protobuf.ByteString): OrderFill = copy(orderId = __v)
def withVolume(__v: _root_.scala.Long): OrderFill = copy(volume = __v)
def withFee(__v: _root_.scala.Long): OrderFill = copy(fee = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = orderId
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
case 2 => {
val __t = volume
if (__t != 0L) __t else null
}
case 3 => {
val __t = fee
if (__t != 0L) __t else null
}
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PByteString(orderId)
case 2 => _root_.scalapb.descriptors.PLong(volume)
case 3 => _root_.scalapb.descriptors.PLong(fee)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.OrderFill.type = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.OrderFill
// @@protoc_insertion_point(GeneratedMessage[waves.TransactionStateSnapshot.OrderFill])
}
object OrderFill extends scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.OrderFill] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.OrderFill] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.OrderFill = {
var __orderId: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var __volume: _root_.scala.Long = 0L
var __fee: _root_.scala.Long = 0L
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__orderId = _input__.readBytes()
case 16 =>
__volume = _input__.readInt64()
case 24 =>
__fee = _input__.readInt64()
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.OrderFill(
orderId = __orderId,
volume = __volume,
fee = __fee,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.OrderFill] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.OrderFill(
orderId = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY),
volume = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).map(_.as[_root_.scala.Long]).getOrElse(0L),
fee = __fieldsMap.get(scalaDescriptor.findFieldByNumber(3).get).map(_.as[_root_.scala.Long]).getOrElse(0L)
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.javaDescriptor.getNestedTypes().get(9)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.scalaDescriptor.nestedMessages(9)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = throw new MatchError(__number)
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] = Seq.empty
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.OrderFill(
orderId = _root_.com.google.protobuf.ByteString.EMPTY,
volume = 0L,
fee = 0L
)
implicit class OrderFillLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.OrderFill]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.OrderFill](_l) {
def orderId: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.orderId)((c_, f_) => c_.copy(orderId = f_))
def volume: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.volume)((c_, f_) => c_.copy(volume = f_))
def fee: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.fee)((c_, f_) => c_.copy(fee = f_))
}
final val ORDER_ID_FIELD_NUMBER = 1
final val VOLUME_FIELD_NUMBER = 2
final val FEE_FIELD_NUMBER = 3
def of(
orderId: _root_.com.google.protobuf.ByteString,
volume: _root_.scala.Long,
fee: _root_.scala.Long
): _root_.com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.OrderFill = _root_.com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.OrderFill(
orderId,
volume,
fee
)
// @@protoc_insertion_point(GeneratedMessageCompanion[waves.TransactionStateSnapshot.OrderFill])
}
@SerialVersionUID(0L)
final case class AccountScript(
senderPublicKey: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
script: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
verifierComplexity: _root_.scala.Long = 0L,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[AccountScript] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = senderPublicKey
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(1, __value)
}
};
{
val __value = script
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(2, __value)
}
};
{
val __value = verifierComplexity
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt64Size(3, __value)
}
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = senderPublicKey
if (!__v.isEmpty) {
_output__.writeBytes(1, __v)
}
};
{
val __v = script
if (!__v.isEmpty) {
_output__.writeBytes(2, __v)
}
};
{
val __v = verifierComplexity
if (__v != 0L) {
_output__.writeInt64(3, __v)
}
};
unknownFields.writeTo(_output__)
}
def withSenderPublicKey(__v: _root_.com.google.protobuf.ByteString): AccountScript = copy(senderPublicKey = __v)
def withScript(__v: _root_.com.google.protobuf.ByteString): AccountScript = copy(script = __v)
def withVerifierComplexity(__v: _root_.scala.Long): AccountScript = copy(verifierComplexity = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = senderPublicKey
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
case 2 => {
val __t = script
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
case 3 => {
val __t = verifierComplexity
if (__t != 0L) __t else null
}
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PByteString(senderPublicKey)
case 2 => _root_.scalapb.descriptors.PByteString(script)
case 3 => _root_.scalapb.descriptors.PLong(verifierComplexity)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountScript.type = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountScript
// @@protoc_insertion_point(GeneratedMessage[waves.TransactionStateSnapshot.AccountScript])
}
object AccountScript extends scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountScript] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountScript] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountScript = {
var __senderPublicKey: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var __script: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var __verifierComplexity: _root_.scala.Long = 0L
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__senderPublicKey = _input__.readBytes()
case 18 =>
__script = _input__.readBytes()
case 24 =>
__verifierComplexity = _input__.readInt64()
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountScript(
senderPublicKey = __senderPublicKey,
script = __script,
verifierComplexity = __verifierComplexity,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountScript] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountScript(
senderPublicKey = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY),
script = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY),
verifierComplexity = __fieldsMap.get(scalaDescriptor.findFieldByNumber(3).get).map(_.as[_root_.scala.Long]).getOrElse(0L)
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.javaDescriptor.getNestedTypes().get(10)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.scalaDescriptor.nestedMessages(10)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = throw new MatchError(__number)
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] = Seq.empty
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountScript(
senderPublicKey = _root_.com.google.protobuf.ByteString.EMPTY,
script = _root_.com.google.protobuf.ByteString.EMPTY,
verifierComplexity = 0L
)
implicit class AccountScriptLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountScript]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountScript](_l) {
def senderPublicKey: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.senderPublicKey)((c_, f_) => c_.copy(senderPublicKey = f_))
def script: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.script)((c_, f_) => c_.copy(script = f_))
def verifierComplexity: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.verifierComplexity)((c_, f_) => c_.copy(verifierComplexity = f_))
}
final val SENDER_PUBLIC_KEY_FIELD_NUMBER = 1
final val SCRIPT_FIELD_NUMBER = 2
final val VERIFIER_COMPLEXITY_FIELD_NUMBER = 3
def of(
senderPublicKey: _root_.com.google.protobuf.ByteString,
script: _root_.com.google.protobuf.ByteString,
verifierComplexity: _root_.scala.Long
): _root_.com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountScript = _root_.com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountScript(
senderPublicKey,
script,
verifierComplexity
)
// @@protoc_insertion_point(GeneratedMessageCompanion[waves.TransactionStateSnapshot.AccountScript])
}
@SerialVersionUID(0L)
final case class AccountData(
address: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
entries: _root_.scala.Seq[com.wavesplatform.protobuf.transaction.DataEntry] = _root_.scala.Seq.empty,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[AccountData] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = address
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(1, __value)
}
};
entries.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = address
if (!__v.isEmpty) {
_output__.writeBytes(1, __v)
}
};
entries.foreach { __v =>
val __m = __v
_output__.writeTag(2, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
unknownFields.writeTo(_output__)
}
def withAddress(__v: _root_.com.google.protobuf.ByteString): AccountData = copy(address = __v)
def clearEntries = copy(entries = _root_.scala.Seq.empty)
def addEntries(__vs: com.wavesplatform.protobuf.transaction.DataEntry *): AccountData = addAllEntries(__vs)
def addAllEntries(__vs: Iterable[com.wavesplatform.protobuf.transaction.DataEntry]): AccountData = copy(entries = entries ++ __vs)
def withEntries(__v: _root_.scala.Seq[com.wavesplatform.protobuf.transaction.DataEntry]): AccountData = copy(entries = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = address
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
case 2 => entries
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PByteString(address)
case 2 => _root_.scalapb.descriptors.PRepeated(entries.iterator.map(_.toPMessage).toVector)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountData.type = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountData
// @@protoc_insertion_point(GeneratedMessage[waves.TransactionStateSnapshot.AccountData])
}
object AccountData extends scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountData] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountData] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountData = {
var __address: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
val __entries: _root_.scala.collection.immutable.VectorBuilder[com.wavesplatform.protobuf.transaction.DataEntry] = new _root_.scala.collection.immutable.VectorBuilder[com.wavesplatform.protobuf.transaction.DataEntry]
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__address = _input__.readBytes()
case 18 =>
__entries += _root_.scalapb.LiteParser.readMessage[com.wavesplatform.protobuf.transaction.DataEntry](_input__)
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountData(
address = __address,
entries = __entries.result(),
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountData] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountData(
address = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY),
entries = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).map(_.as[_root_.scala.Seq[com.wavesplatform.protobuf.transaction.DataEntry]]).getOrElse(_root_.scala.Seq.empty)
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.javaDescriptor.getNestedTypes().get(11)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.scalaDescriptor.nestedMessages(11)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = {
var __out: _root_.scalapb.GeneratedMessageCompanion[_] = null
(__number: @_root_.scala.unchecked) match {
case 2 => __out = com.wavesplatform.protobuf.transaction.DataEntry
}
__out
}
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] = Seq.empty
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountData(
address = _root_.com.google.protobuf.ByteString.EMPTY,
entries = _root_.scala.Seq.empty
)
implicit class AccountDataLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountData]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountData](_l) {
def address: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.address)((c_, f_) => c_.copy(address = f_))
def entries: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[com.wavesplatform.protobuf.transaction.DataEntry]] = field(_.entries)((c_, f_) => c_.copy(entries = f_))
}
final val ADDRESS_FIELD_NUMBER = 1
final val ENTRIES_FIELD_NUMBER = 2
def of(
address: _root_.com.google.protobuf.ByteString,
entries: _root_.scala.Seq[com.wavesplatform.protobuf.transaction.DataEntry]
): _root_.com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountData = _root_.com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountData(
address,
entries
)
// @@protoc_insertion_point(GeneratedMessageCompanion[waves.TransactionStateSnapshot.AccountData])
}
@SerialVersionUID(0L)
final case class Sponsorship(
assetId: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
minFee: _root_.scala.Long = 0L,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[Sponsorship] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = assetId
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(1, __value)
}
};
{
val __value = minFee
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt64Size(2, __value)
}
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = assetId
if (!__v.isEmpty) {
_output__.writeBytes(1, __v)
}
};
{
val __v = minFee
if (__v != 0L) {
_output__.writeInt64(2, __v)
}
};
unknownFields.writeTo(_output__)
}
def withAssetId(__v: _root_.com.google.protobuf.ByteString): Sponsorship = copy(assetId = __v)
def withMinFee(__v: _root_.scala.Long): Sponsorship = copy(minFee = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = assetId
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
case 2 => {
val __t = minFee
if (__t != 0L) __t else null
}
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PByteString(assetId)
case 2 => _root_.scalapb.descriptors.PLong(minFee)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Sponsorship.type = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Sponsorship
// @@protoc_insertion_point(GeneratedMessage[waves.TransactionStateSnapshot.Sponsorship])
}
object Sponsorship extends scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Sponsorship] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Sponsorship] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Sponsorship = {
var __assetId: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var __minFee: _root_.scala.Long = 0L
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__assetId = _input__.readBytes()
case 16 =>
__minFee = _input__.readInt64()
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Sponsorship(
assetId = __assetId,
minFee = __minFee,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Sponsorship] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Sponsorship(
assetId = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY),
minFee = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).map(_.as[_root_.scala.Long]).getOrElse(0L)
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.javaDescriptor.getNestedTypes().get(12)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.scalaDescriptor.nestedMessages(12)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = throw new MatchError(__number)
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] = Seq.empty
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Sponsorship(
assetId = _root_.com.google.protobuf.ByteString.EMPTY,
minFee = 0L
)
implicit class SponsorshipLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Sponsorship]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Sponsorship](_l) {
def assetId: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.assetId)((c_, f_) => c_.copy(assetId = f_))
def minFee: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.minFee)((c_, f_) => c_.copy(minFee = f_))
}
final val ASSET_ID_FIELD_NUMBER = 1
final val MIN_FEE_FIELD_NUMBER = 2
def of(
assetId: _root_.com.google.protobuf.ByteString,
minFee: _root_.scala.Long
): _root_.com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Sponsorship = _root_.com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Sponsorship(
assetId,
minFee
)
// @@protoc_insertion_point(GeneratedMessageCompanion[waves.TransactionStateSnapshot.Sponsorship])
}
implicit class TransactionStateSnapshotLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot](_l) {
def balances: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Balance]] = field(_.balances)((c_, f_) => c_.copy(balances = f_))
def leaseBalances: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.LeaseBalance]] = field(_.leaseBalances)((c_, f_) => c_.copy(leaseBalances = f_))
def newLeases: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewLease]] = field(_.newLeases)((c_, f_) => c_.copy(newLeases = f_))
def cancelledLeases: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.CancelledLease]] = field(_.cancelledLeases)((c_, f_) => c_.copy(cancelledLeases = f_))
def assetStatics: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewAsset]] = field(_.assetStatics)((c_, f_) => c_.copy(assetStatics = f_))
def assetVolumes: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetVolume]] = field(_.assetVolumes)((c_, f_) => c_.copy(assetVolumes = f_))
def assetNamesAndDescriptions: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetNameAndDescription]] = field(_.assetNamesAndDescriptions)((c_, f_) => c_.copy(assetNamesAndDescriptions = f_))
def assetScripts: _root_.scalapb.lenses.Lens[UpperPB, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetScript] = field(_.getAssetScripts)((c_, f_) => c_.copy(assetScripts = _root_.scala.Option(f_)))
def optionalAssetScripts: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetScript]] = field(_.assetScripts)((c_, f_) => c_.copy(assetScripts = f_))
def aliases: _root_.scalapb.lenses.Lens[UpperPB, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Alias] = field(_.getAliases)((c_, f_) => c_.copy(aliases = _root_.scala.Option(f_)))
def optionalAliases: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Alias]] = field(_.aliases)((c_, f_) => c_.copy(aliases = f_))
def orderFills: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.OrderFill]] = field(_.orderFills)((c_, f_) => c_.copy(orderFills = f_))
def accountScripts: _root_.scalapb.lenses.Lens[UpperPB, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountScript] = field(_.getAccountScripts)((c_, f_) => c_.copy(accountScripts = _root_.scala.Option(f_)))
def optionalAccountScripts: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountScript]] = field(_.accountScripts)((c_, f_) => c_.copy(accountScripts = f_))
def accountData: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountData]] = field(_.accountData)((c_, f_) => c_.copy(accountData = f_))
def sponsorships: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Sponsorship]] = field(_.sponsorships)((c_, f_) => c_.copy(sponsorships = f_))
def transactionStatus: _root_.scalapb.lenses.Lens[UpperPB, com.wavesplatform.protobuf.snapshot.TransactionStatus] = field(_.transactionStatus)((c_, f_) => c_.copy(transactionStatus = f_))
}
final val BALANCES_FIELD_NUMBER = 1
final val LEASE_BALANCES_FIELD_NUMBER = 2
final val NEW_LEASES_FIELD_NUMBER = 3
final val CANCELLED_LEASES_FIELD_NUMBER = 4
final val ASSET_STATICS_FIELD_NUMBER = 5
final val ASSET_VOLUMES_FIELD_NUMBER = 6
final val ASSET_NAMES_AND_DESCRIPTIONS_FIELD_NUMBER = 7
final val ASSET_SCRIPTS_FIELD_NUMBER = 8
final val ALIASES_FIELD_NUMBER = 9
final val ORDER_FILLS_FIELD_NUMBER = 10
final val ACCOUNT_SCRIPTS_FIELD_NUMBER = 11
final val ACCOUNT_DATA_FIELD_NUMBER = 12
final val SPONSORSHIPS_FIELD_NUMBER = 13
final val TRANSACTION_STATUS_FIELD_NUMBER = 14
def of(
balances: _root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Balance],
leaseBalances: _root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.LeaseBalance],
newLeases: _root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewLease],
cancelledLeases: _root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.CancelledLease],
assetStatics: _root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.NewAsset],
assetVolumes: _root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetVolume],
assetNamesAndDescriptions: _root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetNameAndDescription],
assetScripts: _root_.scala.Option[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AssetScript],
aliases: _root_.scala.Option[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Alias],
orderFills: _root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.OrderFill],
accountScripts: _root_.scala.Option[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountScript],
accountData: _root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.AccountData],
sponsorships: _root_.scala.Seq[com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot.Sponsorship],
transactionStatus: com.wavesplatform.protobuf.snapshot.TransactionStatus
): _root_.com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot = _root_.com.wavesplatform.protobuf.snapshot.TransactionStateSnapshot(
balances,
leaseBalances,
newLeases,
cancelledLeases,
assetStatics,
assetVolumes,
assetNamesAndDescriptions,
assetScripts,
aliases,
orderFills,
accountScripts,
accountData,
sponsorships,
transactionStatus
)
// @@protoc_insertion_point(GeneratedMessageCompanion[waves.TransactionStateSnapshot])
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy