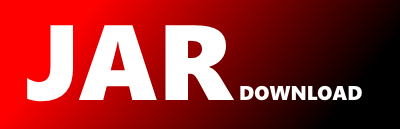
com.wavesplatform.protobuf.transaction.InvokeScriptResult.scala Maven / Gradle / Ivy
The newest version!
// Generated by the Scala Plugin for the Protocol Buffer Compiler.
// Do not edit!
//
// Protofile syntax: PROTO3
package com.wavesplatform.protobuf.transaction
@SerialVersionUID(0L)
final case class InvokeScriptResult(
data: _root_.scala.Seq[com.wavesplatform.protobuf.transaction.DataEntry] = _root_.scala.Seq.empty,
transfers: _root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Payment] = _root_.scala.Seq.empty,
issues: _root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Issue] = _root_.scala.Seq.empty,
reissues: _root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Reissue] = _root_.scala.Seq.empty,
burns: _root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Burn] = _root_.scala.Seq.empty,
errorMessage: _root_.scala.Option[com.wavesplatform.protobuf.transaction.InvokeScriptResult.ErrorMessage] = _root_.scala.None,
sponsorFees: _root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.SponsorFee] = _root_.scala.Seq.empty,
leases: _root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Lease] = _root_.scala.Seq.empty,
leaseCancels: _root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.LeaseCancel] = _root_.scala.Seq.empty,
invokes: _root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Invocation] = _root_.scala.Seq.empty,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[InvokeScriptResult] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
data.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
transfers.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
issues.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
reissues.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
burns.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
if (errorMessage.isDefined) {
val __value = errorMessage.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
sponsorFees.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
leases.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
leaseCancels.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
invokes.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
data.foreach { __v =>
val __m = __v
_output__.writeTag(1, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
transfers.foreach { __v =>
val __m = __v
_output__.writeTag(2, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
issues.foreach { __v =>
val __m = __v
_output__.writeTag(3, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
reissues.foreach { __v =>
val __m = __v
_output__.writeTag(4, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
burns.foreach { __v =>
val __m = __v
_output__.writeTag(5, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
errorMessage.foreach { __v =>
val __m = __v
_output__.writeTag(6, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
sponsorFees.foreach { __v =>
val __m = __v
_output__.writeTag(7, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
leases.foreach { __v =>
val __m = __v
_output__.writeTag(8, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
leaseCancels.foreach { __v =>
val __m = __v
_output__.writeTag(9, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
invokes.foreach { __v =>
val __m = __v
_output__.writeTag(10, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
unknownFields.writeTo(_output__)
}
def clearData = copy(data = _root_.scala.Seq.empty)
def addData(__vs: com.wavesplatform.protobuf.transaction.DataEntry *): InvokeScriptResult = addAllData(__vs)
def addAllData(__vs: Iterable[com.wavesplatform.protobuf.transaction.DataEntry]): InvokeScriptResult = copy(data = data ++ __vs)
def withData(__v: _root_.scala.Seq[com.wavesplatform.protobuf.transaction.DataEntry]): InvokeScriptResult = copy(data = __v)
def clearTransfers = copy(transfers = _root_.scala.Seq.empty)
def addTransfers(__vs: com.wavesplatform.protobuf.transaction.InvokeScriptResult.Payment *): InvokeScriptResult = addAllTransfers(__vs)
def addAllTransfers(__vs: Iterable[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Payment]): InvokeScriptResult = copy(transfers = transfers ++ __vs)
def withTransfers(__v: _root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Payment]): InvokeScriptResult = copy(transfers = __v)
def clearIssues = copy(issues = _root_.scala.Seq.empty)
def addIssues(__vs: com.wavesplatform.protobuf.transaction.InvokeScriptResult.Issue *): InvokeScriptResult = addAllIssues(__vs)
def addAllIssues(__vs: Iterable[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Issue]): InvokeScriptResult = copy(issues = issues ++ __vs)
def withIssues(__v: _root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Issue]): InvokeScriptResult = copy(issues = __v)
def clearReissues = copy(reissues = _root_.scala.Seq.empty)
def addReissues(__vs: com.wavesplatform.protobuf.transaction.InvokeScriptResult.Reissue *): InvokeScriptResult = addAllReissues(__vs)
def addAllReissues(__vs: Iterable[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Reissue]): InvokeScriptResult = copy(reissues = reissues ++ __vs)
def withReissues(__v: _root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Reissue]): InvokeScriptResult = copy(reissues = __v)
def clearBurns = copy(burns = _root_.scala.Seq.empty)
def addBurns(__vs: com.wavesplatform.protobuf.transaction.InvokeScriptResult.Burn *): InvokeScriptResult = addAllBurns(__vs)
def addAllBurns(__vs: Iterable[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Burn]): InvokeScriptResult = copy(burns = burns ++ __vs)
def withBurns(__v: _root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Burn]): InvokeScriptResult = copy(burns = __v)
def getErrorMessage: com.wavesplatform.protobuf.transaction.InvokeScriptResult.ErrorMessage = errorMessage.getOrElse(com.wavesplatform.protobuf.transaction.InvokeScriptResult.ErrorMessage.defaultInstance)
def clearErrorMessage: InvokeScriptResult = copy(errorMessage = _root_.scala.None)
def withErrorMessage(__v: com.wavesplatform.protobuf.transaction.InvokeScriptResult.ErrorMessage): InvokeScriptResult = copy(errorMessage = Option(__v))
def clearSponsorFees = copy(sponsorFees = _root_.scala.Seq.empty)
def addSponsorFees(__vs: com.wavesplatform.protobuf.transaction.InvokeScriptResult.SponsorFee *): InvokeScriptResult = addAllSponsorFees(__vs)
def addAllSponsorFees(__vs: Iterable[com.wavesplatform.protobuf.transaction.InvokeScriptResult.SponsorFee]): InvokeScriptResult = copy(sponsorFees = sponsorFees ++ __vs)
def withSponsorFees(__v: _root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.SponsorFee]): InvokeScriptResult = copy(sponsorFees = __v)
def clearLeases = copy(leases = _root_.scala.Seq.empty)
def addLeases(__vs: com.wavesplatform.protobuf.transaction.InvokeScriptResult.Lease *): InvokeScriptResult = addAllLeases(__vs)
def addAllLeases(__vs: Iterable[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Lease]): InvokeScriptResult = copy(leases = leases ++ __vs)
def withLeases(__v: _root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Lease]): InvokeScriptResult = copy(leases = __v)
def clearLeaseCancels = copy(leaseCancels = _root_.scala.Seq.empty)
def addLeaseCancels(__vs: com.wavesplatform.protobuf.transaction.InvokeScriptResult.LeaseCancel *): InvokeScriptResult = addAllLeaseCancels(__vs)
def addAllLeaseCancels(__vs: Iterable[com.wavesplatform.protobuf.transaction.InvokeScriptResult.LeaseCancel]): InvokeScriptResult = copy(leaseCancels = leaseCancels ++ __vs)
def withLeaseCancels(__v: _root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.LeaseCancel]): InvokeScriptResult = copy(leaseCancels = __v)
def clearInvokes = copy(invokes = _root_.scala.Seq.empty)
def addInvokes(__vs: com.wavesplatform.protobuf.transaction.InvokeScriptResult.Invocation *): InvokeScriptResult = addAllInvokes(__vs)
def addAllInvokes(__vs: Iterable[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Invocation]): InvokeScriptResult = copy(invokes = invokes ++ __vs)
def withInvokes(__v: _root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Invocation]): InvokeScriptResult = copy(invokes = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => data
case 2 => transfers
case 3 => issues
case 4 => reissues
case 5 => burns
case 6 => errorMessage.orNull
case 7 => sponsorFees
case 8 => leases
case 9 => leaseCancels
case 10 => invokes
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PRepeated(data.iterator.map(_.toPMessage).toVector)
case 2 => _root_.scalapb.descriptors.PRepeated(transfers.iterator.map(_.toPMessage).toVector)
case 3 => _root_.scalapb.descriptors.PRepeated(issues.iterator.map(_.toPMessage).toVector)
case 4 => _root_.scalapb.descriptors.PRepeated(reissues.iterator.map(_.toPMessage).toVector)
case 5 => _root_.scalapb.descriptors.PRepeated(burns.iterator.map(_.toPMessage).toVector)
case 6 => errorMessage.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 7 => _root_.scalapb.descriptors.PRepeated(sponsorFees.iterator.map(_.toPMessage).toVector)
case 8 => _root_.scalapb.descriptors.PRepeated(leases.iterator.map(_.toPMessage).toVector)
case 9 => _root_.scalapb.descriptors.PRepeated(leaseCancels.iterator.map(_.toPMessage).toVector)
case 10 => _root_.scalapb.descriptors.PRepeated(invokes.iterator.map(_.toPMessage).toVector)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: com.wavesplatform.protobuf.transaction.InvokeScriptResult.type = com.wavesplatform.protobuf.transaction.InvokeScriptResult
// @@protoc_insertion_point(GeneratedMessage[waves.InvokeScriptResult])
}
object InvokeScriptResult extends scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.transaction.InvokeScriptResult] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.transaction.InvokeScriptResult] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): com.wavesplatform.protobuf.transaction.InvokeScriptResult = {
val __data: _root_.scala.collection.immutable.VectorBuilder[com.wavesplatform.protobuf.transaction.DataEntry] = new _root_.scala.collection.immutable.VectorBuilder[com.wavesplatform.protobuf.transaction.DataEntry]
val __transfers: _root_.scala.collection.immutable.VectorBuilder[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Payment] = new _root_.scala.collection.immutable.VectorBuilder[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Payment]
val __issues: _root_.scala.collection.immutable.VectorBuilder[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Issue] = new _root_.scala.collection.immutable.VectorBuilder[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Issue]
val __reissues: _root_.scala.collection.immutable.VectorBuilder[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Reissue] = new _root_.scala.collection.immutable.VectorBuilder[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Reissue]
val __burns: _root_.scala.collection.immutable.VectorBuilder[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Burn] = new _root_.scala.collection.immutable.VectorBuilder[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Burn]
var __errorMessage: _root_.scala.Option[com.wavesplatform.protobuf.transaction.InvokeScriptResult.ErrorMessage] = _root_.scala.None
val __sponsorFees: _root_.scala.collection.immutable.VectorBuilder[com.wavesplatform.protobuf.transaction.InvokeScriptResult.SponsorFee] = new _root_.scala.collection.immutable.VectorBuilder[com.wavesplatform.protobuf.transaction.InvokeScriptResult.SponsorFee]
val __leases: _root_.scala.collection.immutable.VectorBuilder[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Lease] = new _root_.scala.collection.immutable.VectorBuilder[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Lease]
val __leaseCancels: _root_.scala.collection.immutable.VectorBuilder[com.wavesplatform.protobuf.transaction.InvokeScriptResult.LeaseCancel] = new _root_.scala.collection.immutable.VectorBuilder[com.wavesplatform.protobuf.transaction.InvokeScriptResult.LeaseCancel]
val __invokes: _root_.scala.collection.immutable.VectorBuilder[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Invocation] = new _root_.scala.collection.immutable.VectorBuilder[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Invocation]
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__data += _root_.scalapb.LiteParser.readMessage[com.wavesplatform.protobuf.transaction.DataEntry](_input__)
case 18 =>
__transfers += _root_.scalapb.LiteParser.readMessage[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Payment](_input__)
case 26 =>
__issues += _root_.scalapb.LiteParser.readMessage[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Issue](_input__)
case 34 =>
__reissues += _root_.scalapb.LiteParser.readMessage[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Reissue](_input__)
case 42 =>
__burns += _root_.scalapb.LiteParser.readMessage[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Burn](_input__)
case 50 =>
__errorMessage = _root_.scala.Option(__errorMessage.fold(_root_.scalapb.LiteParser.readMessage[com.wavesplatform.protobuf.transaction.InvokeScriptResult.ErrorMessage](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case 58 =>
__sponsorFees += _root_.scalapb.LiteParser.readMessage[com.wavesplatform.protobuf.transaction.InvokeScriptResult.SponsorFee](_input__)
case 66 =>
__leases += _root_.scalapb.LiteParser.readMessage[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Lease](_input__)
case 74 =>
__leaseCancels += _root_.scalapb.LiteParser.readMessage[com.wavesplatform.protobuf.transaction.InvokeScriptResult.LeaseCancel](_input__)
case 82 =>
__invokes += _root_.scalapb.LiteParser.readMessage[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Invocation](_input__)
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
com.wavesplatform.protobuf.transaction.InvokeScriptResult(
data = __data.result(),
transfers = __transfers.result(),
issues = __issues.result(),
reissues = __reissues.result(),
burns = __burns.result(),
errorMessage = __errorMessage,
sponsorFees = __sponsorFees.result(),
leases = __leases.result(),
leaseCancels = __leaseCancels.result(),
invokes = __invokes.result(),
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[com.wavesplatform.protobuf.transaction.InvokeScriptResult] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
com.wavesplatform.protobuf.transaction.InvokeScriptResult(
data = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.scala.Seq[com.wavesplatform.protobuf.transaction.DataEntry]]).getOrElse(_root_.scala.Seq.empty),
transfers = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).map(_.as[_root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Payment]]).getOrElse(_root_.scala.Seq.empty),
issues = __fieldsMap.get(scalaDescriptor.findFieldByNumber(3).get).map(_.as[_root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Issue]]).getOrElse(_root_.scala.Seq.empty),
reissues = __fieldsMap.get(scalaDescriptor.findFieldByNumber(4).get).map(_.as[_root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Reissue]]).getOrElse(_root_.scala.Seq.empty),
burns = __fieldsMap.get(scalaDescriptor.findFieldByNumber(5).get).map(_.as[_root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Burn]]).getOrElse(_root_.scala.Seq.empty),
errorMessage = __fieldsMap.get(scalaDescriptor.findFieldByNumber(6).get).flatMap(_.as[_root_.scala.Option[com.wavesplatform.protobuf.transaction.InvokeScriptResult.ErrorMessage]]),
sponsorFees = __fieldsMap.get(scalaDescriptor.findFieldByNumber(7).get).map(_.as[_root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.SponsorFee]]).getOrElse(_root_.scala.Seq.empty),
leases = __fieldsMap.get(scalaDescriptor.findFieldByNumber(8).get).map(_.as[_root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Lease]]).getOrElse(_root_.scala.Seq.empty),
leaseCancels = __fieldsMap.get(scalaDescriptor.findFieldByNumber(9).get).map(_.as[_root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.LeaseCancel]]).getOrElse(_root_.scala.Seq.empty),
invokes = __fieldsMap.get(scalaDescriptor.findFieldByNumber(10).get).map(_.as[_root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Invocation]]).getOrElse(_root_.scala.Seq.empty)
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = InvokeScriptResultProto.javaDescriptor.getMessageTypes().get(0)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = InvokeScriptResultProto.scalaDescriptor.messages(0)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = {
var __out: _root_.scalapb.GeneratedMessageCompanion[_] = null
(__number: @_root_.scala.unchecked) match {
case 1 => __out = com.wavesplatform.protobuf.transaction.DataEntry
case 2 => __out = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Payment
case 3 => __out = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Issue
case 4 => __out = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Reissue
case 5 => __out = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Burn
case 6 => __out = com.wavesplatform.protobuf.transaction.InvokeScriptResult.ErrorMessage
case 7 => __out = com.wavesplatform.protobuf.transaction.InvokeScriptResult.SponsorFee
case 8 => __out = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Lease
case 9 => __out = com.wavesplatform.protobuf.transaction.InvokeScriptResult.LeaseCancel
case 10 => __out = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Invocation
}
__out
}
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] =
Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]](
_root_.com.wavesplatform.protobuf.transaction.InvokeScriptResult.Payment,
_root_.com.wavesplatform.protobuf.transaction.InvokeScriptResult.Issue,
_root_.com.wavesplatform.protobuf.transaction.InvokeScriptResult.Reissue,
_root_.com.wavesplatform.protobuf.transaction.InvokeScriptResult.Burn,
_root_.com.wavesplatform.protobuf.transaction.InvokeScriptResult.SponsorFee,
_root_.com.wavesplatform.protobuf.transaction.InvokeScriptResult.Lease,
_root_.com.wavesplatform.protobuf.transaction.InvokeScriptResult.LeaseCancel,
_root_.com.wavesplatform.protobuf.transaction.InvokeScriptResult.ErrorMessage,
_root_.com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call,
_root_.com.wavesplatform.protobuf.transaction.InvokeScriptResult.Invocation
)
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = com.wavesplatform.protobuf.transaction.InvokeScriptResult(
data = _root_.scala.Seq.empty,
transfers = _root_.scala.Seq.empty,
issues = _root_.scala.Seq.empty,
reissues = _root_.scala.Seq.empty,
burns = _root_.scala.Seq.empty,
errorMessage = _root_.scala.None,
sponsorFees = _root_.scala.Seq.empty,
leases = _root_.scala.Seq.empty,
leaseCancels = _root_.scala.Seq.empty,
invokes = _root_.scala.Seq.empty
)
@SerialVersionUID(0L)
final case class Payment(
address: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
amount: _root_.scala.Option[com.wavesplatform.protobuf.Amount] = _root_.scala.None,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[Payment] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = address
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(1, __value)
}
};
if (amount.isDefined) {
val __value = amount.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = address
if (!__v.isEmpty) {
_output__.writeBytes(1, __v)
}
};
amount.foreach { __v =>
val __m = __v
_output__.writeTag(2, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
unknownFields.writeTo(_output__)
}
def withAddress(__v: _root_.com.google.protobuf.ByteString): Payment = copy(address = __v)
def getAmount: com.wavesplatform.protobuf.Amount = amount.getOrElse(com.wavesplatform.protobuf.Amount.defaultInstance)
def clearAmount: Payment = copy(amount = _root_.scala.None)
def withAmount(__v: com.wavesplatform.protobuf.Amount): Payment = copy(amount = Option(__v))
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = address
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
case 2 => amount.orNull
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PByteString(address)
case 2 => amount.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: com.wavesplatform.protobuf.transaction.InvokeScriptResult.Payment.type = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Payment
// @@protoc_insertion_point(GeneratedMessage[waves.InvokeScriptResult.Payment])
}
object Payment extends scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Payment] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Payment] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): com.wavesplatform.protobuf.transaction.InvokeScriptResult.Payment = {
var __address: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var __amount: _root_.scala.Option[com.wavesplatform.protobuf.Amount] = _root_.scala.None
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__address = _input__.readBytes()
case 18 =>
__amount = _root_.scala.Option(__amount.fold(_root_.scalapb.LiteParser.readMessage[com.wavesplatform.protobuf.Amount](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
com.wavesplatform.protobuf.transaction.InvokeScriptResult.Payment(
address = __address,
amount = __amount,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Payment] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
com.wavesplatform.protobuf.transaction.InvokeScriptResult.Payment(
address = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY),
amount = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).flatMap(_.as[_root_.scala.Option[com.wavesplatform.protobuf.Amount]])
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = com.wavesplatform.protobuf.transaction.InvokeScriptResult.javaDescriptor.getNestedTypes().get(0)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = com.wavesplatform.protobuf.transaction.InvokeScriptResult.scalaDescriptor.nestedMessages(0)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = {
var __out: _root_.scalapb.GeneratedMessageCompanion[_] = null
(__number: @_root_.scala.unchecked) match {
case 2 => __out = com.wavesplatform.protobuf.Amount
}
__out
}
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] = Seq.empty
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Payment(
address = _root_.com.google.protobuf.ByteString.EMPTY,
amount = _root_.scala.None
)
implicit class PaymentLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, com.wavesplatform.protobuf.transaction.InvokeScriptResult.Payment]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, com.wavesplatform.protobuf.transaction.InvokeScriptResult.Payment](_l) {
def address: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.address)((c_, f_) => c_.copy(address = f_))
def amount: _root_.scalapb.lenses.Lens[UpperPB, com.wavesplatform.protobuf.Amount] = field(_.getAmount)((c_, f_) => c_.copy(amount = _root_.scala.Option(f_)))
def optionalAmount: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[com.wavesplatform.protobuf.Amount]] = field(_.amount)((c_, f_) => c_.copy(amount = f_))
}
final val ADDRESS_FIELD_NUMBER = 1
final val AMOUNT_FIELD_NUMBER = 2
def of(
address: _root_.com.google.protobuf.ByteString,
amount: _root_.scala.Option[com.wavesplatform.protobuf.Amount]
): _root_.com.wavesplatform.protobuf.transaction.InvokeScriptResult.Payment = _root_.com.wavesplatform.protobuf.transaction.InvokeScriptResult.Payment(
address,
amount
)
// @@protoc_insertion_point(GeneratedMessageCompanion[waves.InvokeScriptResult.Payment])
}
@SerialVersionUID(0L)
final case class Issue(
assetId: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
name: _root_.scala.Predef.String = "",
description: _root_.scala.Predef.String = "",
amount: _root_.scala.Long = 0L,
decimals: _root_.scala.Int = 0,
reissuable: _root_.scala.Boolean = false,
script: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
nonce: _root_.scala.Long = 0L,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[Issue] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = assetId
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(1, __value)
}
};
{
val __value = name
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(2, __value)
}
};
{
val __value = description
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(3, __value)
}
};
{
val __value = amount
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt64Size(4, __value)
}
};
{
val __value = decimals
if (__value != 0) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt32Size(5, __value)
}
};
{
val __value = reissuable
if (__value != false) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBoolSize(6, __value)
}
};
{
val __value = script
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(7, __value)
}
};
{
val __value = nonce
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt64Size(8, __value)
}
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = assetId
if (!__v.isEmpty) {
_output__.writeBytes(1, __v)
}
};
{
val __v = name
if (!__v.isEmpty) {
_output__.writeString(2, __v)
}
};
{
val __v = description
if (!__v.isEmpty) {
_output__.writeString(3, __v)
}
};
{
val __v = amount
if (__v != 0L) {
_output__.writeInt64(4, __v)
}
};
{
val __v = decimals
if (__v != 0) {
_output__.writeInt32(5, __v)
}
};
{
val __v = reissuable
if (__v != false) {
_output__.writeBool(6, __v)
}
};
{
val __v = script
if (!__v.isEmpty) {
_output__.writeBytes(7, __v)
}
};
{
val __v = nonce
if (__v != 0L) {
_output__.writeInt64(8, __v)
}
};
unknownFields.writeTo(_output__)
}
def withAssetId(__v: _root_.com.google.protobuf.ByteString): Issue = copy(assetId = __v)
def withName(__v: _root_.scala.Predef.String): Issue = copy(name = __v)
def withDescription(__v: _root_.scala.Predef.String): Issue = copy(description = __v)
def withAmount(__v: _root_.scala.Long): Issue = copy(amount = __v)
def withDecimals(__v: _root_.scala.Int): Issue = copy(decimals = __v)
def withReissuable(__v: _root_.scala.Boolean): Issue = copy(reissuable = __v)
def withScript(__v: _root_.com.google.protobuf.ByteString): Issue = copy(script = __v)
def withNonce(__v: _root_.scala.Long): Issue = copy(nonce = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = assetId
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
case 2 => {
val __t = name
if (__t != "") __t else null
}
case 3 => {
val __t = description
if (__t != "") __t else null
}
case 4 => {
val __t = amount
if (__t != 0L) __t else null
}
case 5 => {
val __t = decimals
if (__t != 0) __t else null
}
case 6 => {
val __t = reissuable
if (__t != false) __t else null
}
case 7 => {
val __t = script
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
case 8 => {
val __t = nonce
if (__t != 0L) __t else null
}
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PByteString(assetId)
case 2 => _root_.scalapb.descriptors.PString(name)
case 3 => _root_.scalapb.descriptors.PString(description)
case 4 => _root_.scalapb.descriptors.PLong(amount)
case 5 => _root_.scalapb.descriptors.PInt(decimals)
case 6 => _root_.scalapb.descriptors.PBoolean(reissuable)
case 7 => _root_.scalapb.descriptors.PByteString(script)
case 8 => _root_.scalapb.descriptors.PLong(nonce)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: com.wavesplatform.protobuf.transaction.InvokeScriptResult.Issue.type = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Issue
// @@protoc_insertion_point(GeneratedMessage[waves.InvokeScriptResult.Issue])
}
object Issue extends scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Issue] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Issue] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): com.wavesplatform.protobuf.transaction.InvokeScriptResult.Issue = {
var __assetId: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var __name: _root_.scala.Predef.String = ""
var __description: _root_.scala.Predef.String = ""
var __amount: _root_.scala.Long = 0L
var __decimals: _root_.scala.Int = 0
var __reissuable: _root_.scala.Boolean = false
var __script: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var __nonce: _root_.scala.Long = 0L
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__assetId = _input__.readBytes()
case 18 =>
__name = _input__.readStringRequireUtf8()
case 26 =>
__description = _input__.readStringRequireUtf8()
case 32 =>
__amount = _input__.readInt64()
case 40 =>
__decimals = _input__.readInt32()
case 48 =>
__reissuable = _input__.readBool()
case 58 =>
__script = _input__.readBytes()
case 64 =>
__nonce = _input__.readInt64()
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
com.wavesplatform.protobuf.transaction.InvokeScriptResult.Issue(
assetId = __assetId,
name = __name,
description = __description,
amount = __amount,
decimals = __decimals,
reissuable = __reissuable,
script = __script,
nonce = __nonce,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Issue] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
com.wavesplatform.protobuf.transaction.InvokeScriptResult.Issue(
assetId = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY),
name = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).map(_.as[_root_.scala.Predef.String]).getOrElse(""),
description = __fieldsMap.get(scalaDescriptor.findFieldByNumber(3).get).map(_.as[_root_.scala.Predef.String]).getOrElse(""),
amount = __fieldsMap.get(scalaDescriptor.findFieldByNumber(4).get).map(_.as[_root_.scala.Long]).getOrElse(0L),
decimals = __fieldsMap.get(scalaDescriptor.findFieldByNumber(5).get).map(_.as[_root_.scala.Int]).getOrElse(0),
reissuable = __fieldsMap.get(scalaDescriptor.findFieldByNumber(6).get).map(_.as[_root_.scala.Boolean]).getOrElse(false),
script = __fieldsMap.get(scalaDescriptor.findFieldByNumber(7).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY),
nonce = __fieldsMap.get(scalaDescriptor.findFieldByNumber(8).get).map(_.as[_root_.scala.Long]).getOrElse(0L)
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = com.wavesplatform.protobuf.transaction.InvokeScriptResult.javaDescriptor.getNestedTypes().get(1)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = com.wavesplatform.protobuf.transaction.InvokeScriptResult.scalaDescriptor.nestedMessages(1)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = throw new MatchError(__number)
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] = Seq.empty
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Issue(
assetId = _root_.com.google.protobuf.ByteString.EMPTY,
name = "",
description = "",
amount = 0L,
decimals = 0,
reissuable = false,
script = _root_.com.google.protobuf.ByteString.EMPTY,
nonce = 0L
)
implicit class IssueLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, com.wavesplatform.protobuf.transaction.InvokeScriptResult.Issue]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, com.wavesplatform.protobuf.transaction.InvokeScriptResult.Issue](_l) {
def assetId: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.assetId)((c_, f_) => c_.copy(assetId = f_))
def name: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.name)((c_, f_) => c_.copy(name = f_))
def description: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.description)((c_, f_) => c_.copy(description = f_))
def amount: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.amount)((c_, f_) => c_.copy(amount = f_))
def decimals: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Int] = field(_.decimals)((c_, f_) => c_.copy(decimals = f_))
def reissuable: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Boolean] = field(_.reissuable)((c_, f_) => c_.copy(reissuable = f_))
def script: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.script)((c_, f_) => c_.copy(script = f_))
def nonce: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.nonce)((c_, f_) => c_.copy(nonce = f_))
}
final val ASSET_ID_FIELD_NUMBER = 1
final val NAME_FIELD_NUMBER = 2
final val DESCRIPTION_FIELD_NUMBER = 3
final val AMOUNT_FIELD_NUMBER = 4
final val DECIMALS_FIELD_NUMBER = 5
final val REISSUABLE_FIELD_NUMBER = 6
final val SCRIPT_FIELD_NUMBER = 7
final val NONCE_FIELD_NUMBER = 8
def of(
assetId: _root_.com.google.protobuf.ByteString,
name: _root_.scala.Predef.String,
description: _root_.scala.Predef.String,
amount: _root_.scala.Long,
decimals: _root_.scala.Int,
reissuable: _root_.scala.Boolean,
script: _root_.com.google.protobuf.ByteString,
nonce: _root_.scala.Long
): _root_.com.wavesplatform.protobuf.transaction.InvokeScriptResult.Issue = _root_.com.wavesplatform.protobuf.transaction.InvokeScriptResult.Issue(
assetId,
name,
description,
amount,
decimals,
reissuable,
script,
nonce
)
// @@protoc_insertion_point(GeneratedMessageCompanion[waves.InvokeScriptResult.Issue])
}
@SerialVersionUID(0L)
final case class Reissue(
assetId: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
amount: _root_.scala.Long = 0L,
isReissuable: _root_.scala.Boolean = false,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[Reissue] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = assetId
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(1, __value)
}
};
{
val __value = amount
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt64Size(2, __value)
}
};
{
val __value = isReissuable
if (__value != false) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBoolSize(3, __value)
}
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = assetId
if (!__v.isEmpty) {
_output__.writeBytes(1, __v)
}
};
{
val __v = amount
if (__v != 0L) {
_output__.writeInt64(2, __v)
}
};
{
val __v = isReissuable
if (__v != false) {
_output__.writeBool(3, __v)
}
};
unknownFields.writeTo(_output__)
}
def withAssetId(__v: _root_.com.google.protobuf.ByteString): Reissue = copy(assetId = __v)
def withAmount(__v: _root_.scala.Long): Reissue = copy(amount = __v)
def withIsReissuable(__v: _root_.scala.Boolean): Reissue = copy(isReissuable = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = assetId
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
case 2 => {
val __t = amount
if (__t != 0L) __t else null
}
case 3 => {
val __t = isReissuable
if (__t != false) __t else null
}
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PByteString(assetId)
case 2 => _root_.scalapb.descriptors.PLong(amount)
case 3 => _root_.scalapb.descriptors.PBoolean(isReissuable)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: com.wavesplatform.protobuf.transaction.InvokeScriptResult.Reissue.type = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Reissue
// @@protoc_insertion_point(GeneratedMessage[waves.InvokeScriptResult.Reissue])
}
object Reissue extends scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Reissue] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Reissue] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): com.wavesplatform.protobuf.transaction.InvokeScriptResult.Reissue = {
var __assetId: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var __amount: _root_.scala.Long = 0L
var __isReissuable: _root_.scala.Boolean = false
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__assetId = _input__.readBytes()
case 16 =>
__amount = _input__.readInt64()
case 24 =>
__isReissuable = _input__.readBool()
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
com.wavesplatform.protobuf.transaction.InvokeScriptResult.Reissue(
assetId = __assetId,
amount = __amount,
isReissuable = __isReissuable,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Reissue] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
com.wavesplatform.protobuf.transaction.InvokeScriptResult.Reissue(
assetId = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY),
amount = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).map(_.as[_root_.scala.Long]).getOrElse(0L),
isReissuable = __fieldsMap.get(scalaDescriptor.findFieldByNumber(3).get).map(_.as[_root_.scala.Boolean]).getOrElse(false)
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = com.wavesplatform.protobuf.transaction.InvokeScriptResult.javaDescriptor.getNestedTypes().get(2)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = com.wavesplatform.protobuf.transaction.InvokeScriptResult.scalaDescriptor.nestedMessages(2)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = throw new MatchError(__number)
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] = Seq.empty
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Reissue(
assetId = _root_.com.google.protobuf.ByteString.EMPTY,
amount = 0L,
isReissuable = false
)
implicit class ReissueLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, com.wavesplatform.protobuf.transaction.InvokeScriptResult.Reissue]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, com.wavesplatform.protobuf.transaction.InvokeScriptResult.Reissue](_l) {
def assetId: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.assetId)((c_, f_) => c_.copy(assetId = f_))
def amount: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.amount)((c_, f_) => c_.copy(amount = f_))
def isReissuable: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Boolean] = field(_.isReissuable)((c_, f_) => c_.copy(isReissuable = f_))
}
final val ASSET_ID_FIELD_NUMBER = 1
final val AMOUNT_FIELD_NUMBER = 2
final val IS_REISSUABLE_FIELD_NUMBER = 3
def of(
assetId: _root_.com.google.protobuf.ByteString,
amount: _root_.scala.Long,
isReissuable: _root_.scala.Boolean
): _root_.com.wavesplatform.protobuf.transaction.InvokeScriptResult.Reissue = _root_.com.wavesplatform.protobuf.transaction.InvokeScriptResult.Reissue(
assetId,
amount,
isReissuable
)
// @@protoc_insertion_point(GeneratedMessageCompanion[waves.InvokeScriptResult.Reissue])
}
@SerialVersionUID(0L)
final case class Burn(
assetId: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
amount: _root_.scala.Long = 0L,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[Burn] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = assetId
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(1, __value)
}
};
{
val __value = amount
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt64Size(2, __value)
}
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = assetId
if (!__v.isEmpty) {
_output__.writeBytes(1, __v)
}
};
{
val __v = amount
if (__v != 0L) {
_output__.writeInt64(2, __v)
}
};
unknownFields.writeTo(_output__)
}
def withAssetId(__v: _root_.com.google.protobuf.ByteString): Burn = copy(assetId = __v)
def withAmount(__v: _root_.scala.Long): Burn = copy(amount = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = assetId
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
case 2 => {
val __t = amount
if (__t != 0L) __t else null
}
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PByteString(assetId)
case 2 => _root_.scalapb.descriptors.PLong(amount)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: com.wavesplatform.protobuf.transaction.InvokeScriptResult.Burn.type = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Burn
// @@protoc_insertion_point(GeneratedMessage[waves.InvokeScriptResult.Burn])
}
object Burn extends scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Burn] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Burn] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): com.wavesplatform.protobuf.transaction.InvokeScriptResult.Burn = {
var __assetId: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var __amount: _root_.scala.Long = 0L
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__assetId = _input__.readBytes()
case 16 =>
__amount = _input__.readInt64()
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
com.wavesplatform.protobuf.transaction.InvokeScriptResult.Burn(
assetId = __assetId,
amount = __amount,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Burn] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
com.wavesplatform.protobuf.transaction.InvokeScriptResult.Burn(
assetId = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY),
amount = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).map(_.as[_root_.scala.Long]).getOrElse(0L)
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = com.wavesplatform.protobuf.transaction.InvokeScriptResult.javaDescriptor.getNestedTypes().get(3)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = com.wavesplatform.protobuf.transaction.InvokeScriptResult.scalaDescriptor.nestedMessages(3)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = throw new MatchError(__number)
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] = Seq.empty
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Burn(
assetId = _root_.com.google.protobuf.ByteString.EMPTY,
amount = 0L
)
implicit class BurnLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, com.wavesplatform.protobuf.transaction.InvokeScriptResult.Burn]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, com.wavesplatform.protobuf.transaction.InvokeScriptResult.Burn](_l) {
def assetId: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.assetId)((c_, f_) => c_.copy(assetId = f_))
def amount: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.amount)((c_, f_) => c_.copy(amount = f_))
}
final val ASSET_ID_FIELD_NUMBER = 1
final val AMOUNT_FIELD_NUMBER = 2
def of(
assetId: _root_.com.google.protobuf.ByteString,
amount: _root_.scala.Long
): _root_.com.wavesplatform.protobuf.transaction.InvokeScriptResult.Burn = _root_.com.wavesplatform.protobuf.transaction.InvokeScriptResult.Burn(
assetId,
amount
)
// @@protoc_insertion_point(GeneratedMessageCompanion[waves.InvokeScriptResult.Burn])
}
@SerialVersionUID(0L)
final case class SponsorFee(
minFee: _root_.scala.Option[com.wavesplatform.protobuf.Amount] = _root_.scala.None,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[SponsorFee] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
if (minFee.isDefined) {
val __value = minFee.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
minFee.foreach { __v =>
val __m = __v
_output__.writeTag(1, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
unknownFields.writeTo(_output__)
}
def getMinFee: com.wavesplatform.protobuf.Amount = minFee.getOrElse(com.wavesplatform.protobuf.Amount.defaultInstance)
def clearMinFee: SponsorFee = copy(minFee = _root_.scala.None)
def withMinFee(__v: com.wavesplatform.protobuf.Amount): SponsorFee = copy(minFee = Option(__v))
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => minFee.orNull
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => minFee.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: com.wavesplatform.protobuf.transaction.InvokeScriptResult.SponsorFee.type = com.wavesplatform.protobuf.transaction.InvokeScriptResult.SponsorFee
// @@protoc_insertion_point(GeneratedMessage[waves.InvokeScriptResult.SponsorFee])
}
object SponsorFee extends scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.transaction.InvokeScriptResult.SponsorFee] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.transaction.InvokeScriptResult.SponsorFee] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): com.wavesplatform.protobuf.transaction.InvokeScriptResult.SponsorFee = {
var __minFee: _root_.scala.Option[com.wavesplatform.protobuf.Amount] = _root_.scala.None
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__minFee = _root_.scala.Option(__minFee.fold(_root_.scalapb.LiteParser.readMessage[com.wavesplatform.protobuf.Amount](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
com.wavesplatform.protobuf.transaction.InvokeScriptResult.SponsorFee(
minFee = __minFee,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[com.wavesplatform.protobuf.transaction.InvokeScriptResult.SponsorFee] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
com.wavesplatform.protobuf.transaction.InvokeScriptResult.SponsorFee(
minFee = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).flatMap(_.as[_root_.scala.Option[com.wavesplatform.protobuf.Amount]])
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = com.wavesplatform.protobuf.transaction.InvokeScriptResult.javaDescriptor.getNestedTypes().get(4)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = com.wavesplatform.protobuf.transaction.InvokeScriptResult.scalaDescriptor.nestedMessages(4)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = {
var __out: _root_.scalapb.GeneratedMessageCompanion[_] = null
(__number: @_root_.scala.unchecked) match {
case 1 => __out = com.wavesplatform.protobuf.Amount
}
__out
}
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] = Seq.empty
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = com.wavesplatform.protobuf.transaction.InvokeScriptResult.SponsorFee(
minFee = _root_.scala.None
)
implicit class SponsorFeeLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, com.wavesplatform.protobuf.transaction.InvokeScriptResult.SponsorFee]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, com.wavesplatform.protobuf.transaction.InvokeScriptResult.SponsorFee](_l) {
def minFee: _root_.scalapb.lenses.Lens[UpperPB, com.wavesplatform.protobuf.Amount] = field(_.getMinFee)((c_, f_) => c_.copy(minFee = _root_.scala.Option(f_)))
def optionalMinFee: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[com.wavesplatform.protobuf.Amount]] = field(_.minFee)((c_, f_) => c_.copy(minFee = f_))
}
final val MIN_FEE_FIELD_NUMBER = 1
def of(
minFee: _root_.scala.Option[com.wavesplatform.protobuf.Amount]
): _root_.com.wavesplatform.protobuf.transaction.InvokeScriptResult.SponsorFee = _root_.com.wavesplatform.protobuf.transaction.InvokeScriptResult.SponsorFee(
minFee
)
// @@protoc_insertion_point(GeneratedMessageCompanion[waves.InvokeScriptResult.SponsorFee])
}
@SerialVersionUID(0L)
final case class Lease(
recipient: _root_.scala.Option[com.wavesplatform.protobuf.transaction.Recipient] = _root_.scala.None,
amount: _root_.scala.Long = 0L,
nonce: _root_.scala.Long = 0L,
leaseId: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[Lease] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
if (recipient.isDefined) {
val __value = recipient.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
{
val __value = amount
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt64Size(2, __value)
}
};
{
val __value = nonce
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt64Size(3, __value)
}
};
{
val __value = leaseId
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(4, __value)
}
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
recipient.foreach { __v =>
val __m = __v
_output__.writeTag(1, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
{
val __v = amount
if (__v != 0L) {
_output__.writeInt64(2, __v)
}
};
{
val __v = nonce
if (__v != 0L) {
_output__.writeInt64(3, __v)
}
};
{
val __v = leaseId
if (!__v.isEmpty) {
_output__.writeBytes(4, __v)
}
};
unknownFields.writeTo(_output__)
}
def getRecipient: com.wavesplatform.protobuf.transaction.Recipient = recipient.getOrElse(com.wavesplatform.protobuf.transaction.Recipient.defaultInstance)
def clearRecipient: Lease = copy(recipient = _root_.scala.None)
def withRecipient(__v: com.wavesplatform.protobuf.transaction.Recipient): Lease = copy(recipient = Option(__v))
def withAmount(__v: _root_.scala.Long): Lease = copy(amount = __v)
def withNonce(__v: _root_.scala.Long): Lease = copy(nonce = __v)
def withLeaseId(__v: _root_.com.google.protobuf.ByteString): Lease = copy(leaseId = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => recipient.orNull
case 2 => {
val __t = amount
if (__t != 0L) __t else null
}
case 3 => {
val __t = nonce
if (__t != 0L) __t else null
}
case 4 => {
val __t = leaseId
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => recipient.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 2 => _root_.scalapb.descriptors.PLong(amount)
case 3 => _root_.scalapb.descriptors.PLong(nonce)
case 4 => _root_.scalapb.descriptors.PByteString(leaseId)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: com.wavesplatform.protobuf.transaction.InvokeScriptResult.Lease.type = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Lease
// @@protoc_insertion_point(GeneratedMessage[waves.InvokeScriptResult.Lease])
}
object Lease extends scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Lease] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Lease] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): com.wavesplatform.protobuf.transaction.InvokeScriptResult.Lease = {
var __recipient: _root_.scala.Option[com.wavesplatform.protobuf.transaction.Recipient] = _root_.scala.None
var __amount: _root_.scala.Long = 0L
var __nonce: _root_.scala.Long = 0L
var __leaseId: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__recipient = _root_.scala.Option(__recipient.fold(_root_.scalapb.LiteParser.readMessage[com.wavesplatform.protobuf.transaction.Recipient](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case 16 =>
__amount = _input__.readInt64()
case 24 =>
__nonce = _input__.readInt64()
case 34 =>
__leaseId = _input__.readBytes()
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
com.wavesplatform.protobuf.transaction.InvokeScriptResult.Lease(
recipient = __recipient,
amount = __amount,
nonce = __nonce,
leaseId = __leaseId,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Lease] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
com.wavesplatform.protobuf.transaction.InvokeScriptResult.Lease(
recipient = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).flatMap(_.as[_root_.scala.Option[com.wavesplatform.protobuf.transaction.Recipient]]),
amount = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).map(_.as[_root_.scala.Long]).getOrElse(0L),
nonce = __fieldsMap.get(scalaDescriptor.findFieldByNumber(3).get).map(_.as[_root_.scala.Long]).getOrElse(0L),
leaseId = __fieldsMap.get(scalaDescriptor.findFieldByNumber(4).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY)
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = com.wavesplatform.protobuf.transaction.InvokeScriptResult.javaDescriptor.getNestedTypes().get(5)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = com.wavesplatform.protobuf.transaction.InvokeScriptResult.scalaDescriptor.nestedMessages(5)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = {
var __out: _root_.scalapb.GeneratedMessageCompanion[_] = null
(__number: @_root_.scala.unchecked) match {
case 1 => __out = com.wavesplatform.protobuf.transaction.Recipient
}
__out
}
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] = Seq.empty
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Lease(
recipient = _root_.scala.None,
amount = 0L,
nonce = 0L,
leaseId = _root_.com.google.protobuf.ByteString.EMPTY
)
implicit class LeaseLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, com.wavesplatform.protobuf.transaction.InvokeScriptResult.Lease]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, com.wavesplatform.protobuf.transaction.InvokeScriptResult.Lease](_l) {
def recipient: _root_.scalapb.lenses.Lens[UpperPB, com.wavesplatform.protobuf.transaction.Recipient] = field(_.getRecipient)((c_, f_) => c_.copy(recipient = _root_.scala.Option(f_)))
def optionalRecipient: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[com.wavesplatform.protobuf.transaction.Recipient]] = field(_.recipient)((c_, f_) => c_.copy(recipient = f_))
def amount: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.amount)((c_, f_) => c_.copy(amount = f_))
def nonce: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.nonce)((c_, f_) => c_.copy(nonce = f_))
def leaseId: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.leaseId)((c_, f_) => c_.copy(leaseId = f_))
}
final val RECIPIENT_FIELD_NUMBER = 1
final val AMOUNT_FIELD_NUMBER = 2
final val NONCE_FIELD_NUMBER = 3
final val LEASE_ID_FIELD_NUMBER = 4
def of(
recipient: _root_.scala.Option[com.wavesplatform.protobuf.transaction.Recipient],
amount: _root_.scala.Long,
nonce: _root_.scala.Long,
leaseId: _root_.com.google.protobuf.ByteString
): _root_.com.wavesplatform.protobuf.transaction.InvokeScriptResult.Lease = _root_.com.wavesplatform.protobuf.transaction.InvokeScriptResult.Lease(
recipient,
amount,
nonce,
leaseId
)
// @@protoc_insertion_point(GeneratedMessageCompanion[waves.InvokeScriptResult.Lease])
}
@SerialVersionUID(0L)
final case class LeaseCancel(
leaseId: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[LeaseCancel] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = leaseId
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(1, __value)
}
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = leaseId
if (!__v.isEmpty) {
_output__.writeBytes(1, __v)
}
};
unknownFields.writeTo(_output__)
}
def withLeaseId(__v: _root_.com.google.protobuf.ByteString): LeaseCancel = copy(leaseId = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = leaseId
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PByteString(leaseId)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: com.wavesplatform.protobuf.transaction.InvokeScriptResult.LeaseCancel.type = com.wavesplatform.protobuf.transaction.InvokeScriptResult.LeaseCancel
// @@protoc_insertion_point(GeneratedMessage[waves.InvokeScriptResult.LeaseCancel])
}
object LeaseCancel extends scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.transaction.InvokeScriptResult.LeaseCancel] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.transaction.InvokeScriptResult.LeaseCancel] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): com.wavesplatform.protobuf.transaction.InvokeScriptResult.LeaseCancel = {
var __leaseId: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__leaseId = _input__.readBytes()
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
com.wavesplatform.protobuf.transaction.InvokeScriptResult.LeaseCancel(
leaseId = __leaseId,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[com.wavesplatform.protobuf.transaction.InvokeScriptResult.LeaseCancel] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
com.wavesplatform.protobuf.transaction.InvokeScriptResult.LeaseCancel(
leaseId = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY)
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = com.wavesplatform.protobuf.transaction.InvokeScriptResult.javaDescriptor.getNestedTypes().get(6)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = com.wavesplatform.protobuf.transaction.InvokeScriptResult.scalaDescriptor.nestedMessages(6)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = throw new MatchError(__number)
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] = Seq.empty
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = com.wavesplatform.protobuf.transaction.InvokeScriptResult.LeaseCancel(
leaseId = _root_.com.google.protobuf.ByteString.EMPTY
)
implicit class LeaseCancelLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, com.wavesplatform.protobuf.transaction.InvokeScriptResult.LeaseCancel]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, com.wavesplatform.protobuf.transaction.InvokeScriptResult.LeaseCancel](_l) {
def leaseId: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.leaseId)((c_, f_) => c_.copy(leaseId = f_))
}
final val LEASE_ID_FIELD_NUMBER = 1
def of(
leaseId: _root_.com.google.protobuf.ByteString
): _root_.com.wavesplatform.protobuf.transaction.InvokeScriptResult.LeaseCancel = _root_.com.wavesplatform.protobuf.transaction.InvokeScriptResult.LeaseCancel(
leaseId
)
// @@protoc_insertion_point(GeneratedMessageCompanion[waves.InvokeScriptResult.LeaseCancel])
}
@SerialVersionUID(0L)
final case class ErrorMessage(
code: _root_.scala.Int = 0,
text: _root_.scala.Predef.String = "",
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[ErrorMessage] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = code
if (__value != 0) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt32Size(1, __value)
}
};
{
val __value = text
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(2, __value)
}
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = code
if (__v != 0) {
_output__.writeInt32(1, __v)
}
};
{
val __v = text
if (!__v.isEmpty) {
_output__.writeString(2, __v)
}
};
unknownFields.writeTo(_output__)
}
def withCode(__v: _root_.scala.Int): ErrorMessage = copy(code = __v)
def withText(__v: _root_.scala.Predef.String): ErrorMessage = copy(text = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = code
if (__t != 0) __t else null
}
case 2 => {
val __t = text
if (__t != "") __t else null
}
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PInt(code)
case 2 => _root_.scalapb.descriptors.PString(text)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: com.wavesplatform.protobuf.transaction.InvokeScriptResult.ErrorMessage.type = com.wavesplatform.protobuf.transaction.InvokeScriptResult.ErrorMessage
// @@protoc_insertion_point(GeneratedMessage[waves.InvokeScriptResult.ErrorMessage])
}
object ErrorMessage extends scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.transaction.InvokeScriptResult.ErrorMessage] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.transaction.InvokeScriptResult.ErrorMessage] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): com.wavesplatform.protobuf.transaction.InvokeScriptResult.ErrorMessage = {
var __code: _root_.scala.Int = 0
var __text: _root_.scala.Predef.String = ""
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 8 =>
__code = _input__.readInt32()
case 18 =>
__text = _input__.readStringRequireUtf8()
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
com.wavesplatform.protobuf.transaction.InvokeScriptResult.ErrorMessage(
code = __code,
text = __text,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[com.wavesplatform.protobuf.transaction.InvokeScriptResult.ErrorMessage] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
com.wavesplatform.protobuf.transaction.InvokeScriptResult.ErrorMessage(
code = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.scala.Int]).getOrElse(0),
text = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).map(_.as[_root_.scala.Predef.String]).getOrElse("")
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = com.wavesplatform.protobuf.transaction.InvokeScriptResult.javaDescriptor.getNestedTypes().get(7)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = com.wavesplatform.protobuf.transaction.InvokeScriptResult.scalaDescriptor.nestedMessages(7)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = throw new MatchError(__number)
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] = Seq.empty
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = com.wavesplatform.protobuf.transaction.InvokeScriptResult.ErrorMessage(
code = 0,
text = ""
)
implicit class ErrorMessageLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, com.wavesplatform.protobuf.transaction.InvokeScriptResult.ErrorMessage]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, com.wavesplatform.protobuf.transaction.InvokeScriptResult.ErrorMessage](_l) {
def code: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Int] = field(_.code)((c_, f_) => c_.copy(code = f_))
def text: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.text)((c_, f_) => c_.copy(text = f_))
}
final val CODE_FIELD_NUMBER = 1
final val TEXT_FIELD_NUMBER = 2
def of(
code: _root_.scala.Int,
text: _root_.scala.Predef.String
): _root_.com.wavesplatform.protobuf.transaction.InvokeScriptResult.ErrorMessage = _root_.com.wavesplatform.protobuf.transaction.InvokeScriptResult.ErrorMessage(
code,
text
)
// @@protoc_insertion_point(GeneratedMessageCompanion[waves.InvokeScriptResult.ErrorMessage])
}
@SerialVersionUID(0L)
final case class Call(
function: _root_.scala.Predef.String = "",
@scala.deprecated(message="Marked as deprecated in proto file", "") argsBytes: _root_.scala.Seq[_root_.com.google.protobuf.ByteString] = _root_.scala.Seq.empty,
args: _root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument] = _root_.scala.Seq.empty,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[Call] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = function
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(1, __value)
}
};
argsBytes.foreach { __item =>
val __value = __item
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(2, __value)
}
args.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = function
if (!__v.isEmpty) {
_output__.writeString(1, __v)
}
};
argsBytes.foreach { __v =>
val __m = __v
_output__.writeBytes(2, __m)
};
args.foreach { __v =>
val __m = __v
_output__.writeTag(3, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
unknownFields.writeTo(_output__)
}
def withFunction(__v: _root_.scala.Predef.String): Call = copy(function = __v)
def clearArgsBytes = copy(argsBytes = _root_.scala.Seq.empty)
def addArgsBytes(__vs: _root_.com.google.protobuf.ByteString *): Call = addAllArgsBytes(__vs)
def addAllArgsBytes(__vs: Iterable[_root_.com.google.protobuf.ByteString]): Call = copy(argsBytes = argsBytes ++ __vs)
def withArgsBytes(__v: _root_.scala.Seq[_root_.com.google.protobuf.ByteString]): Call = copy(argsBytes = __v)
def clearArgs = copy(args = _root_.scala.Seq.empty)
def addArgs(__vs: com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument *): Call = addAllArgs(__vs)
def addAllArgs(__vs: Iterable[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument]): Call = copy(args = args ++ __vs)
def withArgs(__v: _root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument]): Call = copy(args = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = function
if (__t != "") __t else null
}
case 2 => argsBytes
case 3 => args
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PString(function)
case 2 => _root_.scalapb.descriptors.PRepeated(argsBytes.iterator.map(_root_.scalapb.descriptors.PByteString(_)).toVector)
case 3 => _root_.scalapb.descriptors.PRepeated(args.iterator.map(_.toPMessage).toVector)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.type = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call
// @@protoc_insertion_point(GeneratedMessage[waves.InvokeScriptResult.Call])
}
object Call extends scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call = {
var __function: _root_.scala.Predef.String = ""
val __argsBytes: _root_.scala.collection.immutable.VectorBuilder[_root_.com.google.protobuf.ByteString] = new _root_.scala.collection.immutable.VectorBuilder[_root_.com.google.protobuf.ByteString]
val __args: _root_.scala.collection.immutable.VectorBuilder[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument] = new _root_.scala.collection.immutable.VectorBuilder[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument]
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__function = _input__.readStringRequireUtf8()
case 18 =>
__argsBytes += _input__.readBytes()
case 26 =>
__args += _root_.scalapb.LiteParser.readMessage[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument](_input__)
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call(
function = __function,
argsBytes = __argsBytes.result(),
args = __args.result(),
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call(
function = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.scala.Predef.String]).getOrElse(""),
argsBytes = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).map(_.as[_root_.scala.Seq[_root_.com.google.protobuf.ByteString]]).getOrElse(_root_.scala.Seq.empty),
args = __fieldsMap.get(scalaDescriptor.findFieldByNumber(3).get).map(_.as[_root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument]]).getOrElse(_root_.scala.Seq.empty)
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = com.wavesplatform.protobuf.transaction.InvokeScriptResult.javaDescriptor.getNestedTypes().get(8)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = com.wavesplatform.protobuf.transaction.InvokeScriptResult.scalaDescriptor.nestedMessages(8)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = {
var __out: _root_.scalapb.GeneratedMessageCompanion[_] = null
(__number: @_root_.scala.unchecked) match {
case 3 => __out = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument
}
__out
}
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] =
Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]](
_root_.com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument
)
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call(
function = "",
argsBytes = _root_.scala.Seq.empty,
args = _root_.scala.Seq.empty
)
@SerialVersionUID(0L)
final case class Argument(
value: com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.Value = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.Value.Empty,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[Argument] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
if (value.integerValue.isDefined) {
val __value = value.integerValue.get
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt64Size(1, __value)
};
if (value.binaryValue.isDefined) {
val __value = value.binaryValue.get
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(2, __value)
};
if (value.stringValue.isDefined) {
val __value = value.stringValue.get
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(3, __value)
};
if (value.booleanValue.isDefined) {
val __value = value.booleanValue.get
__size += _root_.com.google.protobuf.CodedOutputStream.computeBoolSize(4, __value)
};
if (value.caseObj.isDefined) {
val __value = value.caseObj.get
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(5, __value)
};
if (value.list.isDefined) {
val __value = value.list.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
value.integerValue.foreach { __v =>
val __m = __v
_output__.writeInt64(1, __m)
};
value.binaryValue.foreach { __v =>
val __m = __v
_output__.writeBytes(2, __m)
};
value.stringValue.foreach { __v =>
val __m = __v
_output__.writeString(3, __m)
};
value.booleanValue.foreach { __v =>
val __m = __v
_output__.writeBool(4, __m)
};
value.caseObj.foreach { __v =>
val __m = __v
_output__.writeBytes(5, __m)
};
value.list.foreach { __v =>
val __m = __v
_output__.writeTag(10, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
unknownFields.writeTo(_output__)
}
def getIntegerValue: _root_.scala.Long = value.integerValue.getOrElse(0L)
def withIntegerValue(__v: _root_.scala.Long): Argument = copy(value = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.Value.IntegerValue(__v))
def getBinaryValue: _root_.com.google.protobuf.ByteString = value.binaryValue.getOrElse(_root_.com.google.protobuf.ByteString.EMPTY)
def withBinaryValue(__v: _root_.com.google.protobuf.ByteString): Argument = copy(value = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.Value.BinaryValue(__v))
def getStringValue: _root_.scala.Predef.String = value.stringValue.getOrElse("")
def withStringValue(__v: _root_.scala.Predef.String): Argument = copy(value = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.Value.StringValue(__v))
def getBooleanValue: _root_.scala.Boolean = value.booleanValue.getOrElse(false)
def withBooleanValue(__v: _root_.scala.Boolean): Argument = copy(value = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.Value.BooleanValue(__v))
def getCaseObj: _root_.com.google.protobuf.ByteString = value.caseObj.getOrElse(_root_.com.google.protobuf.ByteString.EMPTY)
def withCaseObj(__v: _root_.com.google.protobuf.ByteString): Argument = copy(value = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.Value.CaseObj(__v))
def getList: com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.List = value.list.getOrElse(com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.List.defaultInstance)
def withList(__v: com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.List): Argument = copy(value = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.Value.List(__v))
def clearValue: Argument = copy(value = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.Value.Empty)
def withValue(__v: com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.Value): Argument = copy(value = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => value.integerValue.orNull
case 2 => value.binaryValue.orNull
case 3 => value.stringValue.orNull
case 4 => value.booleanValue.orNull
case 5 => value.caseObj.orNull
case 10 => value.list.orNull
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => value.integerValue.map(_root_.scalapb.descriptors.PLong(_)).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 2 => value.binaryValue.map(_root_.scalapb.descriptors.PByteString(_)).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 3 => value.stringValue.map(_root_.scalapb.descriptors.PString(_)).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 4 => value.booleanValue.map(_root_.scalapb.descriptors.PBoolean(_)).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 5 => value.caseObj.map(_root_.scalapb.descriptors.PByteString(_)).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 10 => value.list.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.type = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument
// @@protoc_insertion_point(GeneratedMessage[waves.InvokeScriptResult.Call.Argument])
}
object Argument extends scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument = {
var __value: com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.Value = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.Value.Empty
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 8 =>
__value = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.Value.IntegerValue(_input__.readInt64())
case 18 =>
__value = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.Value.BinaryValue(_input__.readBytes())
case 26 =>
__value = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.Value.StringValue(_input__.readStringRequireUtf8())
case 32 =>
__value = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.Value.BooleanValue(_input__.readBool())
case 42 =>
__value = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.Value.CaseObj(_input__.readBytes())
case 82 =>
__value = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.Value.List(__value.list.fold(_root_.scalapb.LiteParser.readMessage[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.List](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument(
value = __value,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument(
value = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).flatMap(_.as[_root_.scala.Option[_root_.scala.Long]]).map(com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.Value.IntegerValue(_))
.orElse[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.Value](__fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).flatMap(_.as[_root_.scala.Option[_root_.com.google.protobuf.ByteString]]).map(com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.Value.BinaryValue(_)))
.orElse[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.Value](__fieldsMap.get(scalaDescriptor.findFieldByNumber(3).get).flatMap(_.as[_root_.scala.Option[_root_.scala.Predef.String]]).map(com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.Value.StringValue(_)))
.orElse[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.Value](__fieldsMap.get(scalaDescriptor.findFieldByNumber(4).get).flatMap(_.as[_root_.scala.Option[_root_.scala.Boolean]]).map(com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.Value.BooleanValue(_)))
.orElse[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.Value](__fieldsMap.get(scalaDescriptor.findFieldByNumber(5).get).flatMap(_.as[_root_.scala.Option[_root_.com.google.protobuf.ByteString]]).map(com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.Value.CaseObj(_)))
.orElse[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.Value](__fieldsMap.get(scalaDescriptor.findFieldByNumber(10).get).flatMap(_.as[_root_.scala.Option[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.List]]).map(com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.Value.List(_)))
.getOrElse(com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.Value.Empty)
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.javaDescriptor.getNestedTypes().get(0)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.scalaDescriptor.nestedMessages(0)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = {
var __out: _root_.scalapb.GeneratedMessageCompanion[_] = null
(__number: @_root_.scala.unchecked) match {
case 10 => __out = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.List
}
__out
}
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] =
Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]](
_root_.com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.List
)
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument(
value = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.Value.Empty
)
sealed trait Value extends _root_.scalapb.GeneratedOneof {
def isEmpty: _root_.scala.Boolean = false
def isDefined: _root_.scala.Boolean = true
def isIntegerValue: _root_.scala.Boolean = false
def isBinaryValue: _root_.scala.Boolean = false
def isStringValue: _root_.scala.Boolean = false
def isBooleanValue: _root_.scala.Boolean = false
def isCaseObj: _root_.scala.Boolean = false
def isList: _root_.scala.Boolean = false
def integerValue: _root_.scala.Option[_root_.scala.Long] = _root_.scala.None
def binaryValue: _root_.scala.Option[_root_.com.google.protobuf.ByteString] = _root_.scala.None
def stringValue: _root_.scala.Option[_root_.scala.Predef.String] = _root_.scala.None
def booleanValue: _root_.scala.Option[_root_.scala.Boolean] = _root_.scala.None
def caseObj: _root_.scala.Option[_root_.com.google.protobuf.ByteString] = _root_.scala.None
def list: _root_.scala.Option[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.List] = _root_.scala.None
}
object Value {
@SerialVersionUID(0L)
case object Empty extends com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.Value {
type ValueType = _root_.scala.Nothing
override def isEmpty: _root_.scala.Boolean = true
override def isDefined: _root_.scala.Boolean = false
override def number: _root_.scala.Int = 0
override def value: _root_.scala.Nothing = throw new java.util.NoSuchElementException("Empty.value")
}
@SerialVersionUID(0L)
final case class IntegerValue(value: _root_.scala.Long) extends com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.Value {
type ValueType = _root_.scala.Long
override def isIntegerValue: _root_.scala.Boolean = true
override def integerValue: _root_.scala.Option[_root_.scala.Long] = Some(value)
override def number: _root_.scala.Int = 1
}
@SerialVersionUID(0L)
final case class BinaryValue(value: _root_.com.google.protobuf.ByteString) extends com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.Value {
type ValueType = _root_.com.google.protobuf.ByteString
override def isBinaryValue: _root_.scala.Boolean = true
override def binaryValue: _root_.scala.Option[_root_.com.google.protobuf.ByteString] = Some(value)
override def number: _root_.scala.Int = 2
}
@SerialVersionUID(0L)
final case class StringValue(value: _root_.scala.Predef.String) extends com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.Value {
type ValueType = _root_.scala.Predef.String
override def isStringValue: _root_.scala.Boolean = true
override def stringValue: _root_.scala.Option[_root_.scala.Predef.String] = Some(value)
override def number: _root_.scala.Int = 3
}
@SerialVersionUID(0L)
final case class BooleanValue(value: _root_.scala.Boolean) extends com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.Value {
type ValueType = _root_.scala.Boolean
override def isBooleanValue: _root_.scala.Boolean = true
override def booleanValue: _root_.scala.Option[_root_.scala.Boolean] = Some(value)
override def number: _root_.scala.Int = 4
}
@SerialVersionUID(0L)
final case class CaseObj(value: _root_.com.google.protobuf.ByteString) extends com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.Value {
type ValueType = _root_.com.google.protobuf.ByteString
override def isCaseObj: _root_.scala.Boolean = true
override def caseObj: _root_.scala.Option[_root_.com.google.protobuf.ByteString] = Some(value)
override def number: _root_.scala.Int = 5
}
@SerialVersionUID(0L)
final case class List(value: com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.List) extends com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.Value {
type ValueType = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.List
override def isList: _root_.scala.Boolean = true
override def list: _root_.scala.Option[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.List] = Some(value)
override def number: _root_.scala.Int = 10
}
}
@SerialVersionUID(0L)
final case class List(
items: _root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument] = _root_.scala.Seq.empty,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[List] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
items.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
items.foreach { __v =>
val __m = __v
_output__.writeTag(1, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
unknownFields.writeTo(_output__)
}
def clearItems = copy(items = _root_.scala.Seq.empty)
def addItems(__vs: com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument *): List = addAllItems(__vs)
def addAllItems(__vs: Iterable[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument]): List = copy(items = items ++ __vs)
def withItems(__v: _root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument]): List = copy(items = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => items
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PRepeated(items.iterator.map(_.toPMessage).toVector)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.List.type = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.List
// @@protoc_insertion_point(GeneratedMessage[waves.InvokeScriptResult.Call.Argument.List])
}
object List extends scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.List] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.List] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.List = {
val __items: _root_.scala.collection.immutable.VectorBuilder[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument] = new _root_.scala.collection.immutable.VectorBuilder[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument]
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__items += _root_.scalapb.LiteParser.readMessage[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument](_input__)
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.List(
items = __items.result(),
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.List] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.List(
items = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument]]).getOrElse(_root_.scala.Seq.empty)
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.javaDescriptor.getNestedTypes().get(0)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.scalaDescriptor.nestedMessages(0)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = {
var __out: _root_.scalapb.GeneratedMessageCompanion[_] = null
(__number: @_root_.scala.unchecked) match {
case 1 => __out = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument
}
__out
}
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] = Seq.empty
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.List(
items = _root_.scala.Seq.empty
)
implicit class ListLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.List]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.List](_l) {
def items: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument]] = field(_.items)((c_, f_) => c_.copy(items = f_))
}
final val ITEMS_FIELD_NUMBER = 1
def of(
items: _root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument]
): _root_.com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.List = _root_.com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.List(
items
)
// @@protoc_insertion_point(GeneratedMessageCompanion[waves.InvokeScriptResult.Call.Argument.List])
}
implicit class ArgumentLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument](_l) {
def integerValue: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.getIntegerValue)((c_, f_) => c_.copy(value = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.Value.IntegerValue(f_)))
def binaryValue: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.getBinaryValue)((c_, f_) => c_.copy(value = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.Value.BinaryValue(f_)))
def stringValue: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.getStringValue)((c_, f_) => c_.copy(value = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.Value.StringValue(f_)))
def booleanValue: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Boolean] = field(_.getBooleanValue)((c_, f_) => c_.copy(value = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.Value.BooleanValue(f_)))
def caseObj: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.getCaseObj)((c_, f_) => c_.copy(value = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.Value.CaseObj(f_)))
def list: _root_.scalapb.lenses.Lens[UpperPB, com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.List] = field(_.getList)((c_, f_) => c_.copy(value = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.Value.List(f_)))
def value: _root_.scalapb.lenses.Lens[UpperPB, com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.Value] = field(_.value)((c_, f_) => c_.copy(value = f_))
}
final val INTEGER_VALUE_FIELD_NUMBER = 1
final val BINARY_VALUE_FIELD_NUMBER = 2
final val STRING_VALUE_FIELD_NUMBER = 3
final val BOOLEAN_VALUE_FIELD_NUMBER = 4
final val CASE_OBJ_FIELD_NUMBER = 5
final val LIST_FIELD_NUMBER = 10
def of(
value: com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument.Value
): _root_.com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument = _root_.com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument(
value
)
// @@protoc_insertion_point(GeneratedMessageCompanion[waves.InvokeScriptResult.Call.Argument])
}
implicit class CallLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call](_l) {
def function: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.function)((c_, f_) => c_.copy(function = f_))
def argsBytes: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[_root_.com.google.protobuf.ByteString]] = field(_.argsBytes)((c_, f_) => c_.copy(argsBytes = f_))
def args: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument]] = field(_.args)((c_, f_) => c_.copy(args = f_))
}
final val FUNCTION_FIELD_NUMBER = 1
final val ARGS_BYTES_FIELD_NUMBER = 2
final val ARGS_FIELD_NUMBER = 3
def of(
function: _root_.scala.Predef.String,
argsBytes: _root_.scala.Seq[_root_.com.google.protobuf.ByteString],
args: _root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.Argument]
): _root_.com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call = _root_.com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call(
function,
argsBytes,
args
)
// @@protoc_insertion_point(GeneratedMessageCompanion[waves.InvokeScriptResult.Call])
}
@SerialVersionUID(0L)
final case class Invocation(
dApp: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
call: _root_.scala.Option[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call] = _root_.scala.None,
payments: _root_.scala.Seq[com.wavesplatform.protobuf.Amount] = _root_.scala.Seq.empty,
stateChanges: _root_.scala.Option[com.wavesplatform.protobuf.transaction.InvokeScriptResult] = _root_.scala.None,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[Invocation] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = dApp
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(1, __value)
}
};
if (call.isDefined) {
val __value = call.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
payments.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
if (stateChanges.isDefined) {
val __value = stateChanges.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = dApp
if (!__v.isEmpty) {
_output__.writeBytes(1, __v)
}
};
call.foreach { __v =>
val __m = __v
_output__.writeTag(2, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
payments.foreach { __v =>
val __m = __v
_output__.writeTag(3, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
stateChanges.foreach { __v =>
val __m = __v
_output__.writeTag(4, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
unknownFields.writeTo(_output__)
}
def withDApp(__v: _root_.com.google.protobuf.ByteString): Invocation = copy(dApp = __v)
def getCall: com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call = call.getOrElse(com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call.defaultInstance)
def clearCall: Invocation = copy(call = _root_.scala.None)
def withCall(__v: com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call): Invocation = copy(call = Option(__v))
def clearPayments = copy(payments = _root_.scala.Seq.empty)
def addPayments(__vs: com.wavesplatform.protobuf.Amount *): Invocation = addAllPayments(__vs)
def addAllPayments(__vs: Iterable[com.wavesplatform.protobuf.Amount]): Invocation = copy(payments = payments ++ __vs)
def withPayments(__v: _root_.scala.Seq[com.wavesplatform.protobuf.Amount]): Invocation = copy(payments = __v)
def getStateChanges: com.wavesplatform.protobuf.transaction.InvokeScriptResult = stateChanges.getOrElse(com.wavesplatform.protobuf.transaction.InvokeScriptResult.defaultInstance)
def clearStateChanges: Invocation = copy(stateChanges = _root_.scala.None)
def withStateChanges(__v: com.wavesplatform.protobuf.transaction.InvokeScriptResult): Invocation = copy(stateChanges = Option(__v))
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = dApp
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
case 2 => call.orNull
case 3 => payments
case 4 => stateChanges.orNull
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PByteString(dApp)
case 2 => call.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 3 => _root_.scalapb.descriptors.PRepeated(payments.iterator.map(_.toPMessage).toVector)
case 4 => stateChanges.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: com.wavesplatform.protobuf.transaction.InvokeScriptResult.Invocation.type = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Invocation
// @@protoc_insertion_point(GeneratedMessage[waves.InvokeScriptResult.Invocation])
}
object Invocation extends scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Invocation] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Invocation] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): com.wavesplatform.protobuf.transaction.InvokeScriptResult.Invocation = {
var __dApp: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var __call: _root_.scala.Option[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call] = _root_.scala.None
val __payments: _root_.scala.collection.immutable.VectorBuilder[com.wavesplatform.protobuf.Amount] = new _root_.scala.collection.immutable.VectorBuilder[com.wavesplatform.protobuf.Amount]
var __stateChanges: _root_.scala.Option[com.wavesplatform.protobuf.transaction.InvokeScriptResult] = _root_.scala.None
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__dApp = _input__.readBytes()
case 18 =>
__call = _root_.scala.Option(__call.fold(_root_.scalapb.LiteParser.readMessage[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case 26 =>
__payments += _root_.scalapb.LiteParser.readMessage[com.wavesplatform.protobuf.Amount](_input__)
case 34 =>
__stateChanges = _root_.scala.Option(__stateChanges.fold(_root_.scalapb.LiteParser.readMessage[com.wavesplatform.protobuf.transaction.InvokeScriptResult](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
com.wavesplatform.protobuf.transaction.InvokeScriptResult.Invocation(
dApp = __dApp,
call = __call,
payments = __payments.result(),
stateChanges = __stateChanges,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Invocation] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
com.wavesplatform.protobuf.transaction.InvokeScriptResult.Invocation(
dApp = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY),
call = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).flatMap(_.as[_root_.scala.Option[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call]]),
payments = __fieldsMap.get(scalaDescriptor.findFieldByNumber(3).get).map(_.as[_root_.scala.Seq[com.wavesplatform.protobuf.Amount]]).getOrElse(_root_.scala.Seq.empty),
stateChanges = __fieldsMap.get(scalaDescriptor.findFieldByNumber(4).get).flatMap(_.as[_root_.scala.Option[com.wavesplatform.protobuf.transaction.InvokeScriptResult]])
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = com.wavesplatform.protobuf.transaction.InvokeScriptResult.javaDescriptor.getNestedTypes().get(9)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = com.wavesplatform.protobuf.transaction.InvokeScriptResult.scalaDescriptor.nestedMessages(9)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = {
var __out: _root_.scalapb.GeneratedMessageCompanion[_] = null
(__number: @_root_.scala.unchecked) match {
case 2 => __out = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call
case 3 => __out = com.wavesplatform.protobuf.Amount
case 4 => __out = com.wavesplatform.protobuf.transaction.InvokeScriptResult
}
__out
}
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] = Seq.empty
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = com.wavesplatform.protobuf.transaction.InvokeScriptResult.Invocation(
dApp = _root_.com.google.protobuf.ByteString.EMPTY,
call = _root_.scala.None,
payments = _root_.scala.Seq.empty,
stateChanges = _root_.scala.None
)
implicit class InvocationLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, com.wavesplatform.protobuf.transaction.InvokeScriptResult.Invocation]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, com.wavesplatform.protobuf.transaction.InvokeScriptResult.Invocation](_l) {
def dApp: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.dApp)((c_, f_) => c_.copy(dApp = f_))
def call: _root_.scalapb.lenses.Lens[UpperPB, com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call] = field(_.getCall)((c_, f_) => c_.copy(call = _root_.scala.Option(f_)))
def optionalCall: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call]] = field(_.call)((c_, f_) => c_.copy(call = f_))
def payments: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[com.wavesplatform.protobuf.Amount]] = field(_.payments)((c_, f_) => c_.copy(payments = f_))
def stateChanges: _root_.scalapb.lenses.Lens[UpperPB, com.wavesplatform.protobuf.transaction.InvokeScriptResult] = field(_.getStateChanges)((c_, f_) => c_.copy(stateChanges = _root_.scala.Option(f_)))
def optionalStateChanges: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[com.wavesplatform.protobuf.transaction.InvokeScriptResult]] = field(_.stateChanges)((c_, f_) => c_.copy(stateChanges = f_))
}
final val DAPP_FIELD_NUMBER = 1
final val CALL_FIELD_NUMBER = 2
final val PAYMENTS_FIELD_NUMBER = 3
final val STATECHANGES_FIELD_NUMBER = 4
def of(
dApp: _root_.com.google.protobuf.ByteString,
call: _root_.scala.Option[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Call],
payments: _root_.scala.Seq[com.wavesplatform.protobuf.Amount],
stateChanges: _root_.scala.Option[com.wavesplatform.protobuf.transaction.InvokeScriptResult]
): _root_.com.wavesplatform.protobuf.transaction.InvokeScriptResult.Invocation = _root_.com.wavesplatform.protobuf.transaction.InvokeScriptResult.Invocation(
dApp,
call,
payments,
stateChanges
)
// @@protoc_insertion_point(GeneratedMessageCompanion[waves.InvokeScriptResult.Invocation])
}
implicit class InvokeScriptResultLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, com.wavesplatform.protobuf.transaction.InvokeScriptResult]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, com.wavesplatform.protobuf.transaction.InvokeScriptResult](_l) {
def data: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[com.wavesplatform.protobuf.transaction.DataEntry]] = field(_.data)((c_, f_) => c_.copy(data = f_))
def transfers: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Payment]] = field(_.transfers)((c_, f_) => c_.copy(transfers = f_))
def issues: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Issue]] = field(_.issues)((c_, f_) => c_.copy(issues = f_))
def reissues: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Reissue]] = field(_.reissues)((c_, f_) => c_.copy(reissues = f_))
def burns: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Burn]] = field(_.burns)((c_, f_) => c_.copy(burns = f_))
def errorMessage: _root_.scalapb.lenses.Lens[UpperPB, com.wavesplatform.protobuf.transaction.InvokeScriptResult.ErrorMessage] = field(_.getErrorMessage)((c_, f_) => c_.copy(errorMessage = _root_.scala.Option(f_)))
def optionalErrorMessage: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[com.wavesplatform.protobuf.transaction.InvokeScriptResult.ErrorMessage]] = field(_.errorMessage)((c_, f_) => c_.copy(errorMessage = f_))
def sponsorFees: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.SponsorFee]] = field(_.sponsorFees)((c_, f_) => c_.copy(sponsorFees = f_))
def leases: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Lease]] = field(_.leases)((c_, f_) => c_.copy(leases = f_))
def leaseCancels: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.LeaseCancel]] = field(_.leaseCancels)((c_, f_) => c_.copy(leaseCancels = f_))
def invokes: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Invocation]] = field(_.invokes)((c_, f_) => c_.copy(invokes = f_))
}
final val DATA_FIELD_NUMBER = 1
final val TRANSFERS_FIELD_NUMBER = 2
final val ISSUES_FIELD_NUMBER = 3
final val REISSUES_FIELD_NUMBER = 4
final val BURNS_FIELD_NUMBER = 5
final val ERROR_MESSAGE_FIELD_NUMBER = 6
final val SPONSOR_FEES_FIELD_NUMBER = 7
final val LEASES_FIELD_NUMBER = 8
final val LEASE_CANCELS_FIELD_NUMBER = 9
final val INVOKES_FIELD_NUMBER = 10
def of(
data: _root_.scala.Seq[com.wavesplatform.protobuf.transaction.DataEntry],
transfers: _root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Payment],
issues: _root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Issue],
reissues: _root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Reissue],
burns: _root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Burn],
errorMessage: _root_.scala.Option[com.wavesplatform.protobuf.transaction.InvokeScriptResult.ErrorMessage],
sponsorFees: _root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.SponsorFee],
leases: _root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Lease],
leaseCancels: _root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.LeaseCancel],
invokes: _root_.scala.Seq[com.wavesplatform.protobuf.transaction.InvokeScriptResult.Invocation]
): _root_.com.wavesplatform.protobuf.transaction.InvokeScriptResult = _root_.com.wavesplatform.protobuf.transaction.InvokeScriptResult(
data,
transfers,
issues,
reissues,
burns,
errorMessage,
sponsorFees,
leases,
leaseCancels,
invokes
)
// @@protoc_insertion_point(GeneratedMessageCompanion[waves.InvokeScriptResult])
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy