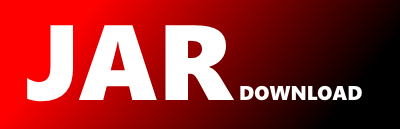
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: waves/transaction_state_snapshot.proto
// Protobuf Java Version: 3.25.1
package com.wavesplatform.protobuf.snapshot;
public final class TransactionStateSnapshotOuterClass {
private TransactionStateSnapshotOuterClass() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
/**
* Protobuf enum {@code waves.TransactionStatus}
*/
public enum TransactionStatus
implements com.google.protobuf.ProtocolMessageEnum {
/**
* SUCCEEDED = 0;
*/
SUCCEEDED(0),
/**
* FAILED = 1;
*/
FAILED(1),
/**
* ELIDED = 2;
*/
ELIDED(2),
UNRECOGNIZED(-1),
;
/**
* SUCCEEDED = 0;
*/
public static final int SUCCEEDED_VALUE = 0;
/**
* FAILED = 1;
*/
public static final int FAILED_VALUE = 1;
/**
* ELIDED = 2;
*/
public static final int ELIDED_VALUE = 2;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static TransactionStatus valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static TransactionStatus forNumber(int value) {
switch (value) {
case 0: return SUCCEEDED;
case 1: return FAILED;
case 2: return ELIDED;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
TransactionStatus> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public TransactionStatus findValueByNumber(int number) {
return TransactionStatus.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.getDescriptor().getEnumTypes().get(0);
}
private static final TransactionStatus[] VALUES = values();
public static TransactionStatus valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private TransactionStatus(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:waves.TransactionStatus)
}
public interface TransactionStateSnapshotOrBuilder extends
// @@protoc_insertion_point(interface_extends:waves.TransactionStateSnapshot)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .waves.TransactionStateSnapshot.Balance balances = 1;
*/
java.util.List
getBalancesList();
/**
* repeated .waves.TransactionStateSnapshot.Balance balances = 1;
*/
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance getBalances(int index);
/**
* repeated .waves.TransactionStateSnapshot.Balance balances = 1;
*/
int getBalancesCount();
/**
* repeated .waves.TransactionStateSnapshot.Balance balances = 1;
*/
java.util.List extends com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.BalanceOrBuilder>
getBalancesOrBuilderList();
/**
* repeated .waves.TransactionStateSnapshot.Balance balances = 1;
*/
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.BalanceOrBuilder getBalancesOrBuilder(
int index);
/**
* repeated .waves.TransactionStateSnapshot.LeaseBalance lease_balances = 2;
*/
java.util.List
getLeaseBalancesList();
/**
* repeated .waves.TransactionStateSnapshot.LeaseBalance lease_balances = 2;
*/
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance getLeaseBalances(int index);
/**
* repeated .waves.TransactionStateSnapshot.LeaseBalance lease_balances = 2;
*/
int getLeaseBalancesCount();
/**
* repeated .waves.TransactionStateSnapshot.LeaseBalance lease_balances = 2;
*/
java.util.List extends com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalanceOrBuilder>
getLeaseBalancesOrBuilderList();
/**
* repeated .waves.TransactionStateSnapshot.LeaseBalance lease_balances = 2;
*/
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalanceOrBuilder getLeaseBalancesOrBuilder(
int index);
/**
* repeated .waves.TransactionStateSnapshot.NewLease new_leases = 3;
*/
java.util.List
getNewLeasesList();
/**
* repeated .waves.TransactionStateSnapshot.NewLease new_leases = 3;
*/
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease getNewLeases(int index);
/**
* repeated .waves.TransactionStateSnapshot.NewLease new_leases = 3;
*/
int getNewLeasesCount();
/**
* repeated .waves.TransactionStateSnapshot.NewLease new_leases = 3;
*/
java.util.List extends com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLeaseOrBuilder>
getNewLeasesOrBuilderList();
/**
* repeated .waves.TransactionStateSnapshot.NewLease new_leases = 3;
*/
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLeaseOrBuilder getNewLeasesOrBuilder(
int index);
/**
* repeated .waves.TransactionStateSnapshot.CancelledLease cancelled_leases = 4;
*/
java.util.List
getCancelledLeasesList();
/**
* repeated .waves.TransactionStateSnapshot.CancelledLease cancelled_leases = 4;
*/
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease getCancelledLeases(int index);
/**
* repeated .waves.TransactionStateSnapshot.CancelledLease cancelled_leases = 4;
*/
int getCancelledLeasesCount();
/**
* repeated .waves.TransactionStateSnapshot.CancelledLease cancelled_leases = 4;
*/
java.util.List extends com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLeaseOrBuilder>
getCancelledLeasesOrBuilderList();
/**
* repeated .waves.TransactionStateSnapshot.CancelledLease cancelled_leases = 4;
*/
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLeaseOrBuilder getCancelledLeasesOrBuilder(
int index);
/**
* repeated .waves.TransactionStateSnapshot.NewAsset asset_statics = 5;
*/
java.util.List
getAssetStaticsList();
/**
* repeated .waves.TransactionStateSnapshot.NewAsset asset_statics = 5;
*/
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset getAssetStatics(int index);
/**
* repeated .waves.TransactionStateSnapshot.NewAsset asset_statics = 5;
*/
int getAssetStaticsCount();
/**
* repeated .waves.TransactionStateSnapshot.NewAsset asset_statics = 5;
*/
java.util.List extends com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAssetOrBuilder>
getAssetStaticsOrBuilderList();
/**
* repeated .waves.TransactionStateSnapshot.NewAsset asset_statics = 5;
*/
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAssetOrBuilder getAssetStaticsOrBuilder(
int index);
/**
* repeated .waves.TransactionStateSnapshot.AssetVolume asset_volumes = 6;
*/
java.util.List
getAssetVolumesList();
/**
* repeated .waves.TransactionStateSnapshot.AssetVolume asset_volumes = 6;
*/
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume getAssetVolumes(int index);
/**
* repeated .waves.TransactionStateSnapshot.AssetVolume asset_volumes = 6;
*/
int getAssetVolumesCount();
/**
* repeated .waves.TransactionStateSnapshot.AssetVolume asset_volumes = 6;
*/
java.util.List extends com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolumeOrBuilder>
getAssetVolumesOrBuilderList();
/**
* repeated .waves.TransactionStateSnapshot.AssetVolume asset_volumes = 6;
*/
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolumeOrBuilder getAssetVolumesOrBuilder(
int index);
/**
* repeated .waves.TransactionStateSnapshot.AssetNameAndDescription asset_names_and_descriptions = 7;
*/
java.util.List
getAssetNamesAndDescriptionsList();
/**
* repeated .waves.TransactionStateSnapshot.AssetNameAndDescription asset_names_and_descriptions = 7;
*/
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription getAssetNamesAndDescriptions(int index);
/**
* repeated .waves.TransactionStateSnapshot.AssetNameAndDescription asset_names_and_descriptions = 7;
*/
int getAssetNamesAndDescriptionsCount();
/**
* repeated .waves.TransactionStateSnapshot.AssetNameAndDescription asset_names_and_descriptions = 7;
*/
java.util.List extends com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescriptionOrBuilder>
getAssetNamesAndDescriptionsOrBuilderList();
/**
* repeated .waves.TransactionStateSnapshot.AssetNameAndDescription asset_names_and_descriptions = 7;
*/
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescriptionOrBuilder getAssetNamesAndDescriptionsOrBuilder(
int index);
/**
* .waves.TransactionStateSnapshot.AssetScript asset_scripts = 8;
* @return Whether the assetScripts field is set.
*/
boolean hasAssetScripts();
/**
* .waves.TransactionStateSnapshot.AssetScript asset_scripts = 8;
* @return The assetScripts.
*/
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript getAssetScripts();
/**
* .waves.TransactionStateSnapshot.AssetScript asset_scripts = 8;
*/
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScriptOrBuilder getAssetScriptsOrBuilder();
/**
* .waves.TransactionStateSnapshot.Alias aliases = 9;
* @return Whether the aliases field is set.
*/
boolean hasAliases();
/**
* .waves.TransactionStateSnapshot.Alias aliases = 9;
* @return The aliases.
*/
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias getAliases();
/**
* .waves.TransactionStateSnapshot.Alias aliases = 9;
*/
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AliasOrBuilder getAliasesOrBuilder();
/**
* repeated .waves.TransactionStateSnapshot.OrderFill order_fills = 10;
*/
java.util.List
getOrderFillsList();
/**
* repeated .waves.TransactionStateSnapshot.OrderFill order_fills = 10;
*/
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill getOrderFills(int index);
/**
* repeated .waves.TransactionStateSnapshot.OrderFill order_fills = 10;
*/
int getOrderFillsCount();
/**
* repeated .waves.TransactionStateSnapshot.OrderFill order_fills = 10;
*/
java.util.List extends com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFillOrBuilder>
getOrderFillsOrBuilderList();
/**
* repeated .waves.TransactionStateSnapshot.OrderFill order_fills = 10;
*/
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFillOrBuilder getOrderFillsOrBuilder(
int index);
/**
* .waves.TransactionStateSnapshot.AccountScript account_scripts = 11;
* @return Whether the accountScripts field is set.
*/
boolean hasAccountScripts();
/**
* .waves.TransactionStateSnapshot.AccountScript account_scripts = 11;
* @return The accountScripts.
*/
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript getAccountScripts();
/**
* .waves.TransactionStateSnapshot.AccountScript account_scripts = 11;
*/
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScriptOrBuilder getAccountScriptsOrBuilder();
/**
* repeated .waves.TransactionStateSnapshot.AccountData account_data = 12;
*/
java.util.List
getAccountDataList();
/**
* repeated .waves.TransactionStateSnapshot.AccountData account_data = 12;
*/
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData getAccountData(int index);
/**
* repeated .waves.TransactionStateSnapshot.AccountData account_data = 12;
*/
int getAccountDataCount();
/**
* repeated .waves.TransactionStateSnapshot.AccountData account_data = 12;
*/
java.util.List extends com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountDataOrBuilder>
getAccountDataOrBuilderList();
/**
* repeated .waves.TransactionStateSnapshot.AccountData account_data = 12;
*/
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountDataOrBuilder getAccountDataOrBuilder(
int index);
/**
* repeated .waves.TransactionStateSnapshot.Sponsorship sponsorships = 13;
*/
java.util.List
getSponsorshipsList();
/**
* repeated .waves.TransactionStateSnapshot.Sponsorship sponsorships = 13;
*/
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship getSponsorships(int index);
/**
* repeated .waves.TransactionStateSnapshot.Sponsorship sponsorships = 13;
*/
int getSponsorshipsCount();
/**
* repeated .waves.TransactionStateSnapshot.Sponsorship sponsorships = 13;
*/
java.util.List extends com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.SponsorshipOrBuilder>
getSponsorshipsOrBuilderList();
/**
* repeated .waves.TransactionStateSnapshot.Sponsorship sponsorships = 13;
*/
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.SponsorshipOrBuilder getSponsorshipsOrBuilder(
int index);
/**
* .waves.TransactionStatus transaction_status = 14;
* @return The enum numeric value on the wire for transactionStatus.
*/
int getTransactionStatusValue();
/**
* .waves.TransactionStatus transaction_status = 14;
* @return The transactionStatus.
*/
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStatus getTransactionStatus();
}
/**
* Protobuf type {@code waves.TransactionStateSnapshot}
*/
public static final class TransactionStateSnapshot extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:waves.TransactionStateSnapshot)
TransactionStateSnapshotOrBuilder {
private static final long serialVersionUID = 0L;
// Use TransactionStateSnapshot.newBuilder() to construct.
private TransactionStateSnapshot(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TransactionStateSnapshot() {
balances_ = java.util.Collections.emptyList();
leaseBalances_ = java.util.Collections.emptyList();
newLeases_ = java.util.Collections.emptyList();
cancelledLeases_ = java.util.Collections.emptyList();
assetStatics_ = java.util.Collections.emptyList();
assetVolumes_ = java.util.Collections.emptyList();
assetNamesAndDescriptions_ = java.util.Collections.emptyList();
orderFills_ = java.util.Collections.emptyList();
accountData_ = java.util.Collections.emptyList();
sponsorships_ = java.util.Collections.emptyList();
transactionStatus_ = 0;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new TransactionStateSnapshot();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.class, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Builder.class);
}
public interface BalanceOrBuilder extends
// @@protoc_insertion_point(interface_extends:waves.TransactionStateSnapshot.Balance)
com.google.protobuf.MessageOrBuilder {
/**
* bytes address = 1;
* @return The address.
*/
com.google.protobuf.ByteString getAddress();
/**
* .waves.Amount amount = 2;
* @return Whether the amount field is set.
*/
boolean hasAmount();
/**
* .waves.Amount amount = 2;
* @return The amount.
*/
com.wavesplatform.protobuf.AmountOuterClass.Amount getAmount();
/**
* .waves.Amount amount = 2;
*/
com.wavesplatform.protobuf.AmountOuterClass.AmountOrBuilder getAmountOrBuilder();
}
/**
* Protobuf type {@code waves.TransactionStateSnapshot.Balance}
*/
public static final class Balance extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:waves.TransactionStateSnapshot.Balance)
BalanceOrBuilder {
private static final long serialVersionUID = 0L;
// Use Balance.newBuilder() to construct.
private Balance(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Balance() {
address_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Balance();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_Balance_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_Balance_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance.class, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance.Builder.class);
}
private int bitField0_;
public static final int ADDRESS_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString address_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes address = 1;
* @return The address.
*/
@java.lang.Override
public com.google.protobuf.ByteString getAddress() {
return address_;
}
public static final int AMOUNT_FIELD_NUMBER = 2;
private com.wavesplatform.protobuf.AmountOuterClass.Amount amount_;
/**
* .waves.Amount amount = 2;
* @return Whether the amount field is set.
*/
@java.lang.Override
public boolean hasAmount() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* .waves.Amount amount = 2;
* @return The amount.
*/
@java.lang.Override
public com.wavesplatform.protobuf.AmountOuterClass.Amount getAmount() {
return amount_ == null ? com.wavesplatform.protobuf.AmountOuterClass.Amount.getDefaultInstance() : amount_;
}
/**
* .waves.Amount amount = 2;
*/
@java.lang.Override
public com.wavesplatform.protobuf.AmountOuterClass.AmountOrBuilder getAmountOrBuilder() {
return amount_ == null ? com.wavesplatform.protobuf.AmountOuterClass.Amount.getDefaultInstance() : amount_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!address_.isEmpty()) {
output.writeBytes(1, address_);
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(2, getAmount());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!address_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, address_);
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getAmount());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance)) {
return super.equals(obj);
}
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance other = (com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance) obj;
if (!getAddress()
.equals(other.getAddress())) return false;
if (hasAmount() != other.hasAmount()) return false;
if (hasAmount()) {
if (!getAmount()
.equals(other.getAmount())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getAddress().hashCode();
if (hasAmount()) {
hash = (37 * hash) + AMOUNT_FIELD_NUMBER;
hash = (53 * hash) + getAmount().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code waves.TransactionStateSnapshot.Balance}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:waves.TransactionStateSnapshot.Balance)
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.BalanceOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_Balance_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_Balance_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance.class, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance.Builder.class);
}
// Construct using com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getAmountFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
address_ = com.google.protobuf.ByteString.EMPTY;
amount_ = null;
if (amountBuilder_ != null) {
amountBuilder_.dispose();
amountBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_Balance_descriptor;
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance getDefaultInstanceForType() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance.getDefaultInstance();
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance build() {
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance buildPartial() {
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance result = new com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.address_ = address_;
}
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000002) != 0)) {
result.amount_ = amountBuilder_ == null
? amount_
: amountBuilder_.build();
to_bitField0_ |= 0x00000001;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance) {
return mergeFrom((com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance other) {
if (other == com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance.getDefaultInstance()) return this;
if (other.getAddress() != com.google.protobuf.ByteString.EMPTY) {
setAddress(other.getAddress());
}
if (other.hasAmount()) {
mergeAmount(other.getAmount());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
address_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
input.readMessage(
getAmountFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000002;
break;
} // case 18
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString address_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes address = 1;
* @return The address.
*/
@java.lang.Override
public com.google.protobuf.ByteString getAddress() {
return address_;
}
/**
* bytes address = 1;
* @param value The address to set.
* @return This builder for chaining.
*/
public Builder setAddress(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
address_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* bytes address = 1;
* @return This builder for chaining.
*/
public Builder clearAddress() {
bitField0_ = (bitField0_ & ~0x00000001);
address_ = getDefaultInstance().getAddress();
onChanged();
return this;
}
private com.wavesplatform.protobuf.AmountOuterClass.Amount amount_;
private com.google.protobuf.SingleFieldBuilderV3<
com.wavesplatform.protobuf.AmountOuterClass.Amount, com.wavesplatform.protobuf.AmountOuterClass.Amount.Builder, com.wavesplatform.protobuf.AmountOuterClass.AmountOrBuilder> amountBuilder_;
/**
* .waves.Amount amount = 2;
* @return Whether the amount field is set.
*/
public boolean hasAmount() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* .waves.Amount amount = 2;
* @return The amount.
*/
public com.wavesplatform.protobuf.AmountOuterClass.Amount getAmount() {
if (amountBuilder_ == null) {
return amount_ == null ? com.wavesplatform.protobuf.AmountOuterClass.Amount.getDefaultInstance() : amount_;
} else {
return amountBuilder_.getMessage();
}
}
/**
* .waves.Amount amount = 2;
*/
public Builder setAmount(com.wavesplatform.protobuf.AmountOuterClass.Amount value) {
if (amountBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
amount_ = value;
} else {
amountBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* .waves.Amount amount = 2;
*/
public Builder setAmount(
com.wavesplatform.protobuf.AmountOuterClass.Amount.Builder builderForValue) {
if (amountBuilder_ == null) {
amount_ = builderForValue.build();
} else {
amountBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* .waves.Amount amount = 2;
*/
public Builder mergeAmount(com.wavesplatform.protobuf.AmountOuterClass.Amount value) {
if (amountBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0) &&
amount_ != null &&
amount_ != com.wavesplatform.protobuf.AmountOuterClass.Amount.getDefaultInstance()) {
getAmountBuilder().mergeFrom(value);
} else {
amount_ = value;
}
} else {
amountBuilder_.mergeFrom(value);
}
if (amount_ != null) {
bitField0_ |= 0x00000002;
onChanged();
}
return this;
}
/**
* .waves.Amount amount = 2;
*/
public Builder clearAmount() {
bitField0_ = (bitField0_ & ~0x00000002);
amount_ = null;
if (amountBuilder_ != null) {
amountBuilder_.dispose();
amountBuilder_ = null;
}
onChanged();
return this;
}
/**
* .waves.Amount amount = 2;
*/
public com.wavesplatform.protobuf.AmountOuterClass.Amount.Builder getAmountBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getAmountFieldBuilder().getBuilder();
}
/**
* .waves.Amount amount = 2;
*/
public com.wavesplatform.protobuf.AmountOuterClass.AmountOrBuilder getAmountOrBuilder() {
if (amountBuilder_ != null) {
return amountBuilder_.getMessageOrBuilder();
} else {
return amount_ == null ?
com.wavesplatform.protobuf.AmountOuterClass.Amount.getDefaultInstance() : amount_;
}
}
/**
* .waves.Amount amount = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.wavesplatform.protobuf.AmountOuterClass.Amount, com.wavesplatform.protobuf.AmountOuterClass.Amount.Builder, com.wavesplatform.protobuf.AmountOuterClass.AmountOrBuilder>
getAmountFieldBuilder() {
if (amountBuilder_ == null) {
amountBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.wavesplatform.protobuf.AmountOuterClass.Amount, com.wavesplatform.protobuf.AmountOuterClass.Amount.Builder, com.wavesplatform.protobuf.AmountOuterClass.AmountOrBuilder>(
getAmount(),
getParentForChildren(),
isClean());
amount_ = null;
}
return amountBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:waves.TransactionStateSnapshot.Balance)
}
// @@protoc_insertion_point(class_scope:waves.TransactionStateSnapshot.Balance)
private static final com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance();
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Balance parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface LeaseBalanceOrBuilder extends
// @@protoc_insertion_point(interface_extends:waves.TransactionStateSnapshot.LeaseBalance)
com.google.protobuf.MessageOrBuilder {
/**
* bytes address = 1;
* @return The address.
*/
com.google.protobuf.ByteString getAddress();
/**
* int64 in = 2;
* @return The in.
*/
long getIn();
/**
* int64 out = 3;
* @return The out.
*/
long getOut();
}
/**
* Protobuf type {@code waves.TransactionStateSnapshot.LeaseBalance}
*/
public static final class LeaseBalance extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:waves.TransactionStateSnapshot.LeaseBalance)
LeaseBalanceOrBuilder {
private static final long serialVersionUID = 0L;
// Use LeaseBalance.newBuilder() to construct.
private LeaseBalance(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private LeaseBalance() {
address_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new LeaseBalance();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_LeaseBalance_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_LeaseBalance_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance.class, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance.Builder.class);
}
public static final int ADDRESS_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString address_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes address = 1;
* @return The address.
*/
@java.lang.Override
public com.google.protobuf.ByteString getAddress() {
return address_;
}
public static final int IN_FIELD_NUMBER = 2;
private long in_ = 0L;
/**
* int64 in = 2;
* @return The in.
*/
@java.lang.Override
public long getIn() {
return in_;
}
public static final int OUT_FIELD_NUMBER = 3;
private long out_ = 0L;
/**
* int64 out = 3;
* @return The out.
*/
@java.lang.Override
public long getOut() {
return out_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!address_.isEmpty()) {
output.writeBytes(1, address_);
}
if (in_ != 0L) {
output.writeInt64(2, in_);
}
if (out_ != 0L) {
output.writeInt64(3, out_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!address_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, address_);
}
if (in_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(2, in_);
}
if (out_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(3, out_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance)) {
return super.equals(obj);
}
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance other = (com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance) obj;
if (!getAddress()
.equals(other.getAddress())) return false;
if (getIn()
!= other.getIn()) return false;
if (getOut()
!= other.getOut()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getAddress().hashCode();
hash = (37 * hash) + IN_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getIn());
hash = (37 * hash) + OUT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getOut());
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code waves.TransactionStateSnapshot.LeaseBalance}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:waves.TransactionStateSnapshot.LeaseBalance)
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalanceOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_LeaseBalance_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_LeaseBalance_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance.class, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance.Builder.class);
}
// Construct using com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
address_ = com.google.protobuf.ByteString.EMPTY;
in_ = 0L;
out_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_LeaseBalance_descriptor;
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance getDefaultInstanceForType() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance.getDefaultInstance();
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance build() {
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance buildPartial() {
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance result = new com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.address_ = address_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.in_ = in_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.out_ = out_;
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance) {
return mergeFrom((com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance other) {
if (other == com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance.getDefaultInstance()) return this;
if (other.getAddress() != com.google.protobuf.ByteString.EMPTY) {
setAddress(other.getAddress());
}
if (other.getIn() != 0L) {
setIn(other.getIn());
}
if (other.getOut() != 0L) {
setOut(other.getOut());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
address_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 10
case 16: {
in_ = input.readInt64();
bitField0_ |= 0x00000002;
break;
} // case 16
case 24: {
out_ = input.readInt64();
bitField0_ |= 0x00000004;
break;
} // case 24
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString address_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes address = 1;
* @return The address.
*/
@java.lang.Override
public com.google.protobuf.ByteString getAddress() {
return address_;
}
/**
* bytes address = 1;
* @param value The address to set.
* @return This builder for chaining.
*/
public Builder setAddress(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
address_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* bytes address = 1;
* @return This builder for chaining.
*/
public Builder clearAddress() {
bitField0_ = (bitField0_ & ~0x00000001);
address_ = getDefaultInstance().getAddress();
onChanged();
return this;
}
private long in_ ;
/**
* int64 in = 2;
* @return The in.
*/
@java.lang.Override
public long getIn() {
return in_;
}
/**
* int64 in = 2;
* @param value The in to set.
* @return This builder for chaining.
*/
public Builder setIn(long value) {
in_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* int64 in = 2;
* @return This builder for chaining.
*/
public Builder clearIn() {
bitField0_ = (bitField0_ & ~0x00000002);
in_ = 0L;
onChanged();
return this;
}
private long out_ ;
/**
* int64 out = 3;
* @return The out.
*/
@java.lang.Override
public long getOut() {
return out_;
}
/**
* int64 out = 3;
* @param value The out to set.
* @return This builder for chaining.
*/
public Builder setOut(long value) {
out_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* int64 out = 3;
* @return This builder for chaining.
*/
public Builder clearOut() {
bitField0_ = (bitField0_ & ~0x00000004);
out_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:waves.TransactionStateSnapshot.LeaseBalance)
}
// @@protoc_insertion_point(class_scope:waves.TransactionStateSnapshot.LeaseBalance)
private static final com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance();
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public LeaseBalance parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface NewLeaseOrBuilder extends
// @@protoc_insertion_point(interface_extends:waves.TransactionStateSnapshot.NewLease)
com.google.protobuf.MessageOrBuilder {
/**
* bytes lease_id = 1;
* @return The leaseId.
*/
com.google.protobuf.ByteString getLeaseId();
/**
* bytes sender_public_key = 2;
* @return The senderPublicKey.
*/
com.google.protobuf.ByteString getSenderPublicKey();
/**
* bytes recipient_address = 3;
* @return The recipientAddress.
*/
com.google.protobuf.ByteString getRecipientAddress();
/**
* int64 amount = 4;
* @return The amount.
*/
long getAmount();
}
/**
* Protobuf type {@code waves.TransactionStateSnapshot.NewLease}
*/
public static final class NewLease extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:waves.TransactionStateSnapshot.NewLease)
NewLeaseOrBuilder {
private static final long serialVersionUID = 0L;
// Use NewLease.newBuilder() to construct.
private NewLease(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private NewLease() {
leaseId_ = com.google.protobuf.ByteString.EMPTY;
senderPublicKey_ = com.google.protobuf.ByteString.EMPTY;
recipientAddress_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new NewLease();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_NewLease_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_NewLease_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease.class, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease.Builder.class);
}
public static final int LEASE_ID_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString leaseId_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes lease_id = 1;
* @return The leaseId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getLeaseId() {
return leaseId_;
}
public static final int SENDER_PUBLIC_KEY_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString senderPublicKey_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes sender_public_key = 2;
* @return The senderPublicKey.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSenderPublicKey() {
return senderPublicKey_;
}
public static final int RECIPIENT_ADDRESS_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString recipientAddress_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes recipient_address = 3;
* @return The recipientAddress.
*/
@java.lang.Override
public com.google.protobuf.ByteString getRecipientAddress() {
return recipientAddress_;
}
public static final int AMOUNT_FIELD_NUMBER = 4;
private long amount_ = 0L;
/**
* int64 amount = 4;
* @return The amount.
*/
@java.lang.Override
public long getAmount() {
return amount_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!leaseId_.isEmpty()) {
output.writeBytes(1, leaseId_);
}
if (!senderPublicKey_.isEmpty()) {
output.writeBytes(2, senderPublicKey_);
}
if (!recipientAddress_.isEmpty()) {
output.writeBytes(3, recipientAddress_);
}
if (amount_ != 0L) {
output.writeInt64(4, amount_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!leaseId_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, leaseId_);
}
if (!senderPublicKey_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, senderPublicKey_);
}
if (!recipientAddress_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, recipientAddress_);
}
if (amount_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(4, amount_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease)) {
return super.equals(obj);
}
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease other = (com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease) obj;
if (!getLeaseId()
.equals(other.getLeaseId())) return false;
if (!getSenderPublicKey()
.equals(other.getSenderPublicKey())) return false;
if (!getRecipientAddress()
.equals(other.getRecipientAddress())) return false;
if (getAmount()
!= other.getAmount()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + LEASE_ID_FIELD_NUMBER;
hash = (53 * hash) + getLeaseId().hashCode();
hash = (37 * hash) + SENDER_PUBLIC_KEY_FIELD_NUMBER;
hash = (53 * hash) + getSenderPublicKey().hashCode();
hash = (37 * hash) + RECIPIENT_ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getRecipientAddress().hashCode();
hash = (37 * hash) + AMOUNT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getAmount());
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code waves.TransactionStateSnapshot.NewLease}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:waves.TransactionStateSnapshot.NewLease)
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLeaseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_NewLease_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_NewLease_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease.class, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease.Builder.class);
}
// Construct using com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
leaseId_ = com.google.protobuf.ByteString.EMPTY;
senderPublicKey_ = com.google.protobuf.ByteString.EMPTY;
recipientAddress_ = com.google.protobuf.ByteString.EMPTY;
amount_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_NewLease_descriptor;
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease getDefaultInstanceForType() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease.getDefaultInstance();
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease build() {
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease buildPartial() {
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease result = new com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.leaseId_ = leaseId_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.senderPublicKey_ = senderPublicKey_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.recipientAddress_ = recipientAddress_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.amount_ = amount_;
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease) {
return mergeFrom((com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease other) {
if (other == com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease.getDefaultInstance()) return this;
if (other.getLeaseId() != com.google.protobuf.ByteString.EMPTY) {
setLeaseId(other.getLeaseId());
}
if (other.getSenderPublicKey() != com.google.protobuf.ByteString.EMPTY) {
setSenderPublicKey(other.getSenderPublicKey());
}
if (other.getRecipientAddress() != com.google.protobuf.ByteString.EMPTY) {
setRecipientAddress(other.getRecipientAddress());
}
if (other.getAmount() != 0L) {
setAmount(other.getAmount());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
leaseId_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
senderPublicKey_ = input.readBytes();
bitField0_ |= 0x00000002;
break;
} // case 18
case 26: {
recipientAddress_ = input.readBytes();
bitField0_ |= 0x00000004;
break;
} // case 26
case 32: {
amount_ = input.readInt64();
bitField0_ |= 0x00000008;
break;
} // case 32
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString leaseId_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes lease_id = 1;
* @return The leaseId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getLeaseId() {
return leaseId_;
}
/**
* bytes lease_id = 1;
* @param value The leaseId to set.
* @return This builder for chaining.
*/
public Builder setLeaseId(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
leaseId_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* bytes lease_id = 1;
* @return This builder for chaining.
*/
public Builder clearLeaseId() {
bitField0_ = (bitField0_ & ~0x00000001);
leaseId_ = getDefaultInstance().getLeaseId();
onChanged();
return this;
}
private com.google.protobuf.ByteString senderPublicKey_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes sender_public_key = 2;
* @return The senderPublicKey.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSenderPublicKey() {
return senderPublicKey_;
}
/**
* bytes sender_public_key = 2;
* @param value The senderPublicKey to set.
* @return This builder for chaining.
*/
public Builder setSenderPublicKey(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
senderPublicKey_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* bytes sender_public_key = 2;
* @return This builder for chaining.
*/
public Builder clearSenderPublicKey() {
bitField0_ = (bitField0_ & ~0x00000002);
senderPublicKey_ = getDefaultInstance().getSenderPublicKey();
onChanged();
return this;
}
private com.google.protobuf.ByteString recipientAddress_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes recipient_address = 3;
* @return The recipientAddress.
*/
@java.lang.Override
public com.google.protobuf.ByteString getRecipientAddress() {
return recipientAddress_;
}
/**
* bytes recipient_address = 3;
* @param value The recipientAddress to set.
* @return This builder for chaining.
*/
public Builder setRecipientAddress(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
recipientAddress_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* bytes recipient_address = 3;
* @return This builder for chaining.
*/
public Builder clearRecipientAddress() {
bitField0_ = (bitField0_ & ~0x00000004);
recipientAddress_ = getDefaultInstance().getRecipientAddress();
onChanged();
return this;
}
private long amount_ ;
/**
* int64 amount = 4;
* @return The amount.
*/
@java.lang.Override
public long getAmount() {
return amount_;
}
/**
* int64 amount = 4;
* @param value The amount to set.
* @return This builder for chaining.
*/
public Builder setAmount(long value) {
amount_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* int64 amount = 4;
* @return This builder for chaining.
*/
public Builder clearAmount() {
bitField0_ = (bitField0_ & ~0x00000008);
amount_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:waves.TransactionStateSnapshot.NewLease)
}
// @@protoc_insertion_point(class_scope:waves.TransactionStateSnapshot.NewLease)
private static final com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease();
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public NewLease parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CancelledLeaseOrBuilder extends
// @@protoc_insertion_point(interface_extends:waves.TransactionStateSnapshot.CancelledLease)
com.google.protobuf.MessageOrBuilder {
/**
* bytes lease_id = 1;
* @return The leaseId.
*/
com.google.protobuf.ByteString getLeaseId();
}
/**
* Protobuf type {@code waves.TransactionStateSnapshot.CancelledLease}
*/
public static final class CancelledLease extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:waves.TransactionStateSnapshot.CancelledLease)
CancelledLeaseOrBuilder {
private static final long serialVersionUID = 0L;
// Use CancelledLease.newBuilder() to construct.
private CancelledLease(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CancelledLease() {
leaseId_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CancelledLease();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_CancelledLease_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_CancelledLease_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease.class, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease.Builder.class);
}
public static final int LEASE_ID_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString leaseId_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes lease_id = 1;
* @return The leaseId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getLeaseId() {
return leaseId_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!leaseId_.isEmpty()) {
output.writeBytes(1, leaseId_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!leaseId_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, leaseId_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease)) {
return super.equals(obj);
}
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease other = (com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease) obj;
if (!getLeaseId()
.equals(other.getLeaseId())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + LEASE_ID_FIELD_NUMBER;
hash = (53 * hash) + getLeaseId().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code waves.TransactionStateSnapshot.CancelledLease}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:waves.TransactionStateSnapshot.CancelledLease)
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLeaseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_CancelledLease_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_CancelledLease_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease.class, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease.Builder.class);
}
// Construct using com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
leaseId_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_CancelledLease_descriptor;
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease getDefaultInstanceForType() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease.getDefaultInstance();
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease build() {
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease buildPartial() {
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease result = new com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.leaseId_ = leaseId_;
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease) {
return mergeFrom((com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease other) {
if (other == com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease.getDefaultInstance()) return this;
if (other.getLeaseId() != com.google.protobuf.ByteString.EMPTY) {
setLeaseId(other.getLeaseId());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
leaseId_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 10
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString leaseId_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes lease_id = 1;
* @return The leaseId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getLeaseId() {
return leaseId_;
}
/**
* bytes lease_id = 1;
* @param value The leaseId to set.
* @return This builder for chaining.
*/
public Builder setLeaseId(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
leaseId_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* bytes lease_id = 1;
* @return This builder for chaining.
*/
public Builder clearLeaseId() {
bitField0_ = (bitField0_ & ~0x00000001);
leaseId_ = getDefaultInstance().getLeaseId();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:waves.TransactionStateSnapshot.CancelledLease)
}
// @@protoc_insertion_point(class_scope:waves.TransactionStateSnapshot.CancelledLease)
private static final com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease();
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CancelledLease parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface NewAssetOrBuilder extends
// @@protoc_insertion_point(interface_extends:waves.TransactionStateSnapshot.NewAsset)
com.google.protobuf.MessageOrBuilder {
/**
* bytes asset_id = 1;
* @return The assetId.
*/
com.google.protobuf.ByteString getAssetId();
/**
* bytes issuer_public_key = 2;
* @return The issuerPublicKey.
*/
com.google.protobuf.ByteString getIssuerPublicKey();
/**
* int32 decimals = 3;
* @return The decimals.
*/
int getDecimals();
/**
* bool nft = 4;
* @return The nft.
*/
boolean getNft();
}
/**
* Protobuf type {@code waves.TransactionStateSnapshot.NewAsset}
*/
public static final class NewAsset extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:waves.TransactionStateSnapshot.NewAsset)
NewAssetOrBuilder {
private static final long serialVersionUID = 0L;
// Use NewAsset.newBuilder() to construct.
private NewAsset(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private NewAsset() {
assetId_ = com.google.protobuf.ByteString.EMPTY;
issuerPublicKey_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new NewAsset();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_NewAsset_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_NewAsset_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset.class, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset.Builder.class);
}
public static final int ASSET_ID_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString assetId_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes asset_id = 1;
* @return The assetId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getAssetId() {
return assetId_;
}
public static final int ISSUER_PUBLIC_KEY_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString issuerPublicKey_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes issuer_public_key = 2;
* @return The issuerPublicKey.
*/
@java.lang.Override
public com.google.protobuf.ByteString getIssuerPublicKey() {
return issuerPublicKey_;
}
public static final int DECIMALS_FIELD_NUMBER = 3;
private int decimals_ = 0;
/**
* int32 decimals = 3;
* @return The decimals.
*/
@java.lang.Override
public int getDecimals() {
return decimals_;
}
public static final int NFT_FIELD_NUMBER = 4;
private boolean nft_ = false;
/**
* bool nft = 4;
* @return The nft.
*/
@java.lang.Override
public boolean getNft() {
return nft_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!assetId_.isEmpty()) {
output.writeBytes(1, assetId_);
}
if (!issuerPublicKey_.isEmpty()) {
output.writeBytes(2, issuerPublicKey_);
}
if (decimals_ != 0) {
output.writeInt32(3, decimals_);
}
if (nft_ != false) {
output.writeBool(4, nft_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!assetId_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, assetId_);
}
if (!issuerPublicKey_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, issuerPublicKey_);
}
if (decimals_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, decimals_);
}
if (nft_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(4, nft_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset)) {
return super.equals(obj);
}
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset other = (com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset) obj;
if (!getAssetId()
.equals(other.getAssetId())) return false;
if (!getIssuerPublicKey()
.equals(other.getIssuerPublicKey())) return false;
if (getDecimals()
!= other.getDecimals()) return false;
if (getNft()
!= other.getNft()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ASSET_ID_FIELD_NUMBER;
hash = (53 * hash) + getAssetId().hashCode();
hash = (37 * hash) + ISSUER_PUBLIC_KEY_FIELD_NUMBER;
hash = (53 * hash) + getIssuerPublicKey().hashCode();
hash = (37 * hash) + DECIMALS_FIELD_NUMBER;
hash = (53 * hash) + getDecimals();
hash = (37 * hash) + NFT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getNft());
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code waves.TransactionStateSnapshot.NewAsset}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:waves.TransactionStateSnapshot.NewAsset)
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAssetOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_NewAsset_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_NewAsset_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset.class, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset.Builder.class);
}
// Construct using com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
assetId_ = com.google.protobuf.ByteString.EMPTY;
issuerPublicKey_ = com.google.protobuf.ByteString.EMPTY;
decimals_ = 0;
nft_ = false;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_NewAsset_descriptor;
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset getDefaultInstanceForType() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset.getDefaultInstance();
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset build() {
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset buildPartial() {
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset result = new com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.assetId_ = assetId_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.issuerPublicKey_ = issuerPublicKey_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.decimals_ = decimals_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.nft_ = nft_;
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset) {
return mergeFrom((com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset other) {
if (other == com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset.getDefaultInstance()) return this;
if (other.getAssetId() != com.google.protobuf.ByteString.EMPTY) {
setAssetId(other.getAssetId());
}
if (other.getIssuerPublicKey() != com.google.protobuf.ByteString.EMPTY) {
setIssuerPublicKey(other.getIssuerPublicKey());
}
if (other.getDecimals() != 0) {
setDecimals(other.getDecimals());
}
if (other.getNft() != false) {
setNft(other.getNft());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
assetId_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
issuerPublicKey_ = input.readBytes();
bitField0_ |= 0x00000002;
break;
} // case 18
case 24: {
decimals_ = input.readInt32();
bitField0_ |= 0x00000004;
break;
} // case 24
case 32: {
nft_ = input.readBool();
bitField0_ |= 0x00000008;
break;
} // case 32
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString assetId_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes asset_id = 1;
* @return The assetId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getAssetId() {
return assetId_;
}
/**
* bytes asset_id = 1;
* @param value The assetId to set.
* @return This builder for chaining.
*/
public Builder setAssetId(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
assetId_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* bytes asset_id = 1;
* @return This builder for chaining.
*/
public Builder clearAssetId() {
bitField0_ = (bitField0_ & ~0x00000001);
assetId_ = getDefaultInstance().getAssetId();
onChanged();
return this;
}
private com.google.protobuf.ByteString issuerPublicKey_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes issuer_public_key = 2;
* @return The issuerPublicKey.
*/
@java.lang.Override
public com.google.protobuf.ByteString getIssuerPublicKey() {
return issuerPublicKey_;
}
/**
* bytes issuer_public_key = 2;
* @param value The issuerPublicKey to set.
* @return This builder for chaining.
*/
public Builder setIssuerPublicKey(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
issuerPublicKey_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* bytes issuer_public_key = 2;
* @return This builder for chaining.
*/
public Builder clearIssuerPublicKey() {
bitField0_ = (bitField0_ & ~0x00000002);
issuerPublicKey_ = getDefaultInstance().getIssuerPublicKey();
onChanged();
return this;
}
private int decimals_ ;
/**
* int32 decimals = 3;
* @return The decimals.
*/
@java.lang.Override
public int getDecimals() {
return decimals_;
}
/**
* int32 decimals = 3;
* @param value The decimals to set.
* @return This builder for chaining.
*/
public Builder setDecimals(int value) {
decimals_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* int32 decimals = 3;
* @return This builder for chaining.
*/
public Builder clearDecimals() {
bitField0_ = (bitField0_ & ~0x00000004);
decimals_ = 0;
onChanged();
return this;
}
private boolean nft_ ;
/**
* bool nft = 4;
* @return The nft.
*/
@java.lang.Override
public boolean getNft() {
return nft_;
}
/**
* bool nft = 4;
* @param value The nft to set.
* @return This builder for chaining.
*/
public Builder setNft(boolean value) {
nft_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* bool nft = 4;
* @return This builder for chaining.
*/
public Builder clearNft() {
bitField0_ = (bitField0_ & ~0x00000008);
nft_ = false;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:waves.TransactionStateSnapshot.NewAsset)
}
// @@protoc_insertion_point(class_scope:waves.TransactionStateSnapshot.NewAsset)
private static final com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset();
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public NewAsset parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface AssetVolumeOrBuilder extends
// @@protoc_insertion_point(interface_extends:waves.TransactionStateSnapshot.AssetVolume)
com.google.protobuf.MessageOrBuilder {
/**
* bytes asset_id = 1;
* @return The assetId.
*/
com.google.protobuf.ByteString getAssetId();
/**
* bool reissuable = 2;
* @return The reissuable.
*/
boolean getReissuable();
/**
* bytes volume = 3;
* @return The volume.
*/
com.google.protobuf.ByteString getVolume();
}
/**
* Protobuf type {@code waves.TransactionStateSnapshot.AssetVolume}
*/
public static final class AssetVolume extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:waves.TransactionStateSnapshot.AssetVolume)
AssetVolumeOrBuilder {
private static final long serialVersionUID = 0L;
// Use AssetVolume.newBuilder() to construct.
private AssetVolume(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private AssetVolume() {
assetId_ = com.google.protobuf.ByteString.EMPTY;
volume_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new AssetVolume();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_AssetVolume_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_AssetVolume_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume.class, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume.Builder.class);
}
public static final int ASSET_ID_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString assetId_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes asset_id = 1;
* @return The assetId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getAssetId() {
return assetId_;
}
public static final int REISSUABLE_FIELD_NUMBER = 2;
private boolean reissuable_ = false;
/**
* bool reissuable = 2;
* @return The reissuable.
*/
@java.lang.Override
public boolean getReissuable() {
return reissuable_;
}
public static final int VOLUME_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString volume_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes volume = 3;
* @return The volume.
*/
@java.lang.Override
public com.google.protobuf.ByteString getVolume() {
return volume_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!assetId_.isEmpty()) {
output.writeBytes(1, assetId_);
}
if (reissuable_ != false) {
output.writeBool(2, reissuable_);
}
if (!volume_.isEmpty()) {
output.writeBytes(3, volume_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!assetId_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, assetId_);
}
if (reissuable_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(2, reissuable_);
}
if (!volume_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, volume_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume)) {
return super.equals(obj);
}
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume other = (com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume) obj;
if (!getAssetId()
.equals(other.getAssetId())) return false;
if (getReissuable()
!= other.getReissuable()) return false;
if (!getVolume()
.equals(other.getVolume())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ASSET_ID_FIELD_NUMBER;
hash = (53 * hash) + getAssetId().hashCode();
hash = (37 * hash) + REISSUABLE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getReissuable());
hash = (37 * hash) + VOLUME_FIELD_NUMBER;
hash = (53 * hash) + getVolume().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code waves.TransactionStateSnapshot.AssetVolume}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:waves.TransactionStateSnapshot.AssetVolume)
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolumeOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_AssetVolume_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_AssetVolume_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume.class, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume.Builder.class);
}
// Construct using com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
assetId_ = com.google.protobuf.ByteString.EMPTY;
reissuable_ = false;
volume_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_AssetVolume_descriptor;
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume getDefaultInstanceForType() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume.getDefaultInstance();
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume build() {
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume buildPartial() {
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume result = new com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.assetId_ = assetId_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.reissuable_ = reissuable_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.volume_ = volume_;
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume) {
return mergeFrom((com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume other) {
if (other == com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume.getDefaultInstance()) return this;
if (other.getAssetId() != com.google.protobuf.ByteString.EMPTY) {
setAssetId(other.getAssetId());
}
if (other.getReissuable() != false) {
setReissuable(other.getReissuable());
}
if (other.getVolume() != com.google.protobuf.ByteString.EMPTY) {
setVolume(other.getVolume());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
assetId_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 10
case 16: {
reissuable_ = input.readBool();
bitField0_ |= 0x00000002;
break;
} // case 16
case 26: {
volume_ = input.readBytes();
bitField0_ |= 0x00000004;
break;
} // case 26
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString assetId_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes asset_id = 1;
* @return The assetId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getAssetId() {
return assetId_;
}
/**
* bytes asset_id = 1;
* @param value The assetId to set.
* @return This builder for chaining.
*/
public Builder setAssetId(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
assetId_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* bytes asset_id = 1;
* @return This builder for chaining.
*/
public Builder clearAssetId() {
bitField0_ = (bitField0_ & ~0x00000001);
assetId_ = getDefaultInstance().getAssetId();
onChanged();
return this;
}
private boolean reissuable_ ;
/**
* bool reissuable = 2;
* @return The reissuable.
*/
@java.lang.Override
public boolean getReissuable() {
return reissuable_;
}
/**
* bool reissuable = 2;
* @param value The reissuable to set.
* @return This builder for chaining.
*/
public Builder setReissuable(boolean value) {
reissuable_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* bool reissuable = 2;
* @return This builder for chaining.
*/
public Builder clearReissuable() {
bitField0_ = (bitField0_ & ~0x00000002);
reissuable_ = false;
onChanged();
return this;
}
private com.google.protobuf.ByteString volume_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes volume = 3;
* @return The volume.
*/
@java.lang.Override
public com.google.protobuf.ByteString getVolume() {
return volume_;
}
/**
* bytes volume = 3;
* @param value The volume to set.
* @return This builder for chaining.
*/
public Builder setVolume(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
volume_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* bytes volume = 3;
* @return This builder for chaining.
*/
public Builder clearVolume() {
bitField0_ = (bitField0_ & ~0x00000004);
volume_ = getDefaultInstance().getVolume();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:waves.TransactionStateSnapshot.AssetVolume)
}
// @@protoc_insertion_point(class_scope:waves.TransactionStateSnapshot.AssetVolume)
private static final com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume();
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public AssetVolume parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface AssetNameAndDescriptionOrBuilder extends
// @@protoc_insertion_point(interface_extends:waves.TransactionStateSnapshot.AssetNameAndDescription)
com.google.protobuf.MessageOrBuilder {
/**
* bytes asset_id = 1;
* @return The assetId.
*/
com.google.protobuf.ByteString getAssetId();
/**
* string name = 2;
* @return The name.
*/
java.lang.String getName();
/**
* string name = 2;
* @return The bytes for name.
*/
com.google.protobuf.ByteString
getNameBytes();
/**
* string description = 3;
* @return The description.
*/
java.lang.String getDescription();
/**
* string description = 3;
* @return The bytes for description.
*/
com.google.protobuf.ByteString
getDescriptionBytes();
}
/**
* Protobuf type {@code waves.TransactionStateSnapshot.AssetNameAndDescription}
*/
public static final class AssetNameAndDescription extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:waves.TransactionStateSnapshot.AssetNameAndDescription)
AssetNameAndDescriptionOrBuilder {
private static final long serialVersionUID = 0L;
// Use AssetNameAndDescription.newBuilder() to construct.
private AssetNameAndDescription(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private AssetNameAndDescription() {
assetId_ = com.google.protobuf.ByteString.EMPTY;
name_ = "";
description_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new AssetNameAndDescription();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_AssetNameAndDescription_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_AssetNameAndDescription_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription.class, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription.Builder.class);
}
public static final int ASSET_ID_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString assetId_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes asset_id = 1;
* @return The assetId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getAssetId() {
return assetId_;
}
public static final int NAME_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object name_ = "";
/**
* string name = 2;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
* string name = 2;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DESCRIPTION_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private volatile java.lang.Object description_ = "";
/**
* string description = 3;
* @return The description.
*/
@java.lang.Override
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
}
}
/**
* string description = 3;
* @return The bytes for description.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!assetId_.isEmpty()) {
output.writeBytes(1, assetId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(name_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, name_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(description_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, description_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!assetId_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, assetId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(name_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, name_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(description_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, description_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription)) {
return super.equals(obj);
}
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription other = (com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription) obj;
if (!getAssetId()
.equals(other.getAssetId())) return false;
if (!getName()
.equals(other.getName())) return false;
if (!getDescription()
.equals(other.getDescription())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ASSET_ID_FIELD_NUMBER;
hash = (53 * hash) + getAssetId().hashCode();
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
hash = (37 * hash) + DESCRIPTION_FIELD_NUMBER;
hash = (53 * hash) + getDescription().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code waves.TransactionStateSnapshot.AssetNameAndDescription}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:waves.TransactionStateSnapshot.AssetNameAndDescription)
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescriptionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_AssetNameAndDescription_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_AssetNameAndDescription_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription.class, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription.Builder.class);
}
// Construct using com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
assetId_ = com.google.protobuf.ByteString.EMPTY;
name_ = "";
description_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_AssetNameAndDescription_descriptor;
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription getDefaultInstanceForType() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription.getDefaultInstance();
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription build() {
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription buildPartial() {
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription result = new com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.assetId_ = assetId_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.name_ = name_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.description_ = description_;
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription) {
return mergeFrom((com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription other) {
if (other == com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription.getDefaultInstance()) return this;
if (other.getAssetId() != com.google.protobuf.ByteString.EMPTY) {
setAssetId(other.getAssetId());
}
if (!other.getName().isEmpty()) {
name_ = other.name_;
bitField0_ |= 0x00000002;
onChanged();
}
if (!other.getDescription().isEmpty()) {
description_ = other.description_;
bitField0_ |= 0x00000004;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
assetId_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
name_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 18
case 26: {
description_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000004;
break;
} // case 26
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString assetId_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes asset_id = 1;
* @return The assetId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getAssetId() {
return assetId_;
}
/**
* bytes asset_id = 1;
* @param value The assetId to set.
* @return This builder for chaining.
*/
public Builder setAssetId(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
assetId_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* bytes asset_id = 1;
* @return This builder for chaining.
*/
public Builder clearAssetId() {
bitField0_ = (bitField0_ & ~0x00000001);
assetId_ = getDefaultInstance().getAssetId();
onChanged();
return this;
}
private java.lang.Object name_ = "";
/**
* string name = 2;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string name = 2;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string name = 2;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
name_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* string name = 2;
* @return This builder for chaining.
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
* string name = 2;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
name_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private java.lang.Object description_ = "";
/**
* string description = 3;
* @return The description.
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string description = 3;
* @return The bytes for description.
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string description = 3;
* @param value The description to set.
* @return This builder for chaining.
*/
public Builder setDescription(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
description_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* string description = 3;
* @return This builder for chaining.
*/
public Builder clearDescription() {
description_ = getDefaultInstance().getDescription();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
* string description = 3;
* @param value The bytes for description to set.
* @return This builder for chaining.
*/
public Builder setDescriptionBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
description_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:waves.TransactionStateSnapshot.AssetNameAndDescription)
}
// @@protoc_insertion_point(class_scope:waves.TransactionStateSnapshot.AssetNameAndDescription)
private static final com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription();
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public AssetNameAndDescription parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface AssetScriptOrBuilder extends
// @@protoc_insertion_point(interface_extends:waves.TransactionStateSnapshot.AssetScript)
com.google.protobuf.MessageOrBuilder {
/**
* bytes asset_id = 1;
* @return The assetId.
*/
com.google.protobuf.ByteString getAssetId();
/**
* bytes script = 2;
* @return The script.
*/
com.google.protobuf.ByteString getScript();
}
/**
* Protobuf type {@code waves.TransactionStateSnapshot.AssetScript}
*/
public static final class AssetScript extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:waves.TransactionStateSnapshot.AssetScript)
AssetScriptOrBuilder {
private static final long serialVersionUID = 0L;
// Use AssetScript.newBuilder() to construct.
private AssetScript(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private AssetScript() {
assetId_ = com.google.protobuf.ByteString.EMPTY;
script_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new AssetScript();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_AssetScript_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_AssetScript_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript.class, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript.Builder.class);
}
public static final int ASSET_ID_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString assetId_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes asset_id = 1;
* @return The assetId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getAssetId() {
return assetId_;
}
public static final int SCRIPT_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString script_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes script = 2;
* @return The script.
*/
@java.lang.Override
public com.google.protobuf.ByteString getScript() {
return script_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!assetId_.isEmpty()) {
output.writeBytes(1, assetId_);
}
if (!script_.isEmpty()) {
output.writeBytes(2, script_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!assetId_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, assetId_);
}
if (!script_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, script_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript)) {
return super.equals(obj);
}
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript other = (com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript) obj;
if (!getAssetId()
.equals(other.getAssetId())) return false;
if (!getScript()
.equals(other.getScript())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ASSET_ID_FIELD_NUMBER;
hash = (53 * hash) + getAssetId().hashCode();
hash = (37 * hash) + SCRIPT_FIELD_NUMBER;
hash = (53 * hash) + getScript().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code waves.TransactionStateSnapshot.AssetScript}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:waves.TransactionStateSnapshot.AssetScript)
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScriptOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_AssetScript_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_AssetScript_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript.class, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript.Builder.class);
}
// Construct using com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
assetId_ = com.google.protobuf.ByteString.EMPTY;
script_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_AssetScript_descriptor;
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript getDefaultInstanceForType() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript.getDefaultInstance();
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript build() {
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript buildPartial() {
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript result = new com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.assetId_ = assetId_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.script_ = script_;
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript) {
return mergeFrom((com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript other) {
if (other == com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript.getDefaultInstance()) return this;
if (other.getAssetId() != com.google.protobuf.ByteString.EMPTY) {
setAssetId(other.getAssetId());
}
if (other.getScript() != com.google.protobuf.ByteString.EMPTY) {
setScript(other.getScript());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
assetId_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
script_ = input.readBytes();
bitField0_ |= 0x00000002;
break;
} // case 18
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString assetId_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes asset_id = 1;
* @return The assetId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getAssetId() {
return assetId_;
}
/**
* bytes asset_id = 1;
* @param value The assetId to set.
* @return This builder for chaining.
*/
public Builder setAssetId(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
assetId_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* bytes asset_id = 1;
* @return This builder for chaining.
*/
public Builder clearAssetId() {
bitField0_ = (bitField0_ & ~0x00000001);
assetId_ = getDefaultInstance().getAssetId();
onChanged();
return this;
}
private com.google.protobuf.ByteString script_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes script = 2;
* @return The script.
*/
@java.lang.Override
public com.google.protobuf.ByteString getScript() {
return script_;
}
/**
* bytes script = 2;
* @param value The script to set.
* @return This builder for chaining.
*/
public Builder setScript(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
script_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* bytes script = 2;
* @return This builder for chaining.
*/
public Builder clearScript() {
bitField0_ = (bitField0_ & ~0x00000002);
script_ = getDefaultInstance().getScript();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:waves.TransactionStateSnapshot.AssetScript)
}
// @@protoc_insertion_point(class_scope:waves.TransactionStateSnapshot.AssetScript)
private static final com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript();
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public AssetScript parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface AliasOrBuilder extends
// @@protoc_insertion_point(interface_extends:waves.TransactionStateSnapshot.Alias)
com.google.protobuf.MessageOrBuilder {
/**
* bytes address = 1;
* @return The address.
*/
com.google.protobuf.ByteString getAddress();
/**
* string alias = 2;
* @return The alias.
*/
java.lang.String getAlias();
/**
* string alias = 2;
* @return The bytes for alias.
*/
com.google.protobuf.ByteString
getAliasBytes();
}
/**
* Protobuf type {@code waves.TransactionStateSnapshot.Alias}
*/
public static final class Alias extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:waves.TransactionStateSnapshot.Alias)
AliasOrBuilder {
private static final long serialVersionUID = 0L;
// Use Alias.newBuilder() to construct.
private Alias(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Alias() {
address_ = com.google.protobuf.ByteString.EMPTY;
alias_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Alias();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_Alias_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_Alias_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias.class, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias.Builder.class);
}
public static final int ADDRESS_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString address_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes address = 1;
* @return The address.
*/
@java.lang.Override
public com.google.protobuf.ByteString getAddress() {
return address_;
}
public static final int ALIAS_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object alias_ = "";
/**
* string alias = 2;
* @return The alias.
*/
@java.lang.Override
public java.lang.String getAlias() {
java.lang.Object ref = alias_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
alias_ = s;
return s;
}
}
/**
* string alias = 2;
* @return The bytes for alias.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getAliasBytes() {
java.lang.Object ref = alias_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
alias_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!address_.isEmpty()) {
output.writeBytes(1, address_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(alias_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, alias_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!address_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, address_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(alias_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, alias_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias)) {
return super.equals(obj);
}
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias other = (com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias) obj;
if (!getAddress()
.equals(other.getAddress())) return false;
if (!getAlias()
.equals(other.getAlias())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getAddress().hashCode();
hash = (37 * hash) + ALIAS_FIELD_NUMBER;
hash = (53 * hash) + getAlias().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code waves.TransactionStateSnapshot.Alias}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:waves.TransactionStateSnapshot.Alias)
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AliasOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_Alias_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_Alias_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias.class, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias.Builder.class);
}
// Construct using com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
address_ = com.google.protobuf.ByteString.EMPTY;
alias_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_Alias_descriptor;
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias getDefaultInstanceForType() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias.getDefaultInstance();
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias build() {
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias buildPartial() {
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias result = new com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.address_ = address_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.alias_ = alias_;
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias) {
return mergeFrom((com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias other) {
if (other == com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias.getDefaultInstance()) return this;
if (other.getAddress() != com.google.protobuf.ByteString.EMPTY) {
setAddress(other.getAddress());
}
if (!other.getAlias().isEmpty()) {
alias_ = other.alias_;
bitField0_ |= 0x00000002;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
address_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
alias_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 18
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString address_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes address = 1;
* @return The address.
*/
@java.lang.Override
public com.google.protobuf.ByteString getAddress() {
return address_;
}
/**
* bytes address = 1;
* @param value The address to set.
* @return This builder for chaining.
*/
public Builder setAddress(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
address_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* bytes address = 1;
* @return This builder for chaining.
*/
public Builder clearAddress() {
bitField0_ = (bitField0_ & ~0x00000001);
address_ = getDefaultInstance().getAddress();
onChanged();
return this;
}
private java.lang.Object alias_ = "";
/**
* string alias = 2;
* @return The alias.
*/
public java.lang.String getAlias() {
java.lang.Object ref = alias_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
alias_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string alias = 2;
* @return The bytes for alias.
*/
public com.google.protobuf.ByteString
getAliasBytes() {
java.lang.Object ref = alias_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
alias_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string alias = 2;
* @param value The alias to set.
* @return This builder for chaining.
*/
public Builder setAlias(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
alias_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* string alias = 2;
* @return This builder for chaining.
*/
public Builder clearAlias() {
alias_ = getDefaultInstance().getAlias();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
* string alias = 2;
* @param value The bytes for alias to set.
* @return This builder for chaining.
*/
public Builder setAliasBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
alias_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:waves.TransactionStateSnapshot.Alias)
}
// @@protoc_insertion_point(class_scope:waves.TransactionStateSnapshot.Alias)
private static final com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias();
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Alias parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface OrderFillOrBuilder extends
// @@protoc_insertion_point(interface_extends:waves.TransactionStateSnapshot.OrderFill)
com.google.protobuf.MessageOrBuilder {
/**
* bytes order_id = 1;
* @return The orderId.
*/
com.google.protobuf.ByteString getOrderId();
/**
* int64 volume = 2;
* @return The volume.
*/
long getVolume();
/**
* int64 fee = 3;
* @return The fee.
*/
long getFee();
}
/**
* Protobuf type {@code waves.TransactionStateSnapshot.OrderFill}
*/
public static final class OrderFill extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:waves.TransactionStateSnapshot.OrderFill)
OrderFillOrBuilder {
private static final long serialVersionUID = 0L;
// Use OrderFill.newBuilder() to construct.
private OrderFill(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private OrderFill() {
orderId_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new OrderFill();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_OrderFill_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_OrderFill_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill.class, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill.Builder.class);
}
public static final int ORDER_ID_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString orderId_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes order_id = 1;
* @return The orderId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getOrderId() {
return orderId_;
}
public static final int VOLUME_FIELD_NUMBER = 2;
private long volume_ = 0L;
/**
* int64 volume = 2;
* @return The volume.
*/
@java.lang.Override
public long getVolume() {
return volume_;
}
public static final int FEE_FIELD_NUMBER = 3;
private long fee_ = 0L;
/**
* int64 fee = 3;
* @return The fee.
*/
@java.lang.Override
public long getFee() {
return fee_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!orderId_.isEmpty()) {
output.writeBytes(1, orderId_);
}
if (volume_ != 0L) {
output.writeInt64(2, volume_);
}
if (fee_ != 0L) {
output.writeInt64(3, fee_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!orderId_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, orderId_);
}
if (volume_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(2, volume_);
}
if (fee_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(3, fee_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill)) {
return super.equals(obj);
}
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill other = (com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill) obj;
if (!getOrderId()
.equals(other.getOrderId())) return false;
if (getVolume()
!= other.getVolume()) return false;
if (getFee()
!= other.getFee()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ORDER_ID_FIELD_NUMBER;
hash = (53 * hash) + getOrderId().hashCode();
hash = (37 * hash) + VOLUME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getVolume());
hash = (37 * hash) + FEE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getFee());
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code waves.TransactionStateSnapshot.OrderFill}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:waves.TransactionStateSnapshot.OrderFill)
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFillOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_OrderFill_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_OrderFill_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill.class, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill.Builder.class);
}
// Construct using com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
orderId_ = com.google.protobuf.ByteString.EMPTY;
volume_ = 0L;
fee_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_OrderFill_descriptor;
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill getDefaultInstanceForType() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill.getDefaultInstance();
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill build() {
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill buildPartial() {
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill result = new com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.orderId_ = orderId_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.volume_ = volume_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.fee_ = fee_;
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill) {
return mergeFrom((com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill other) {
if (other == com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill.getDefaultInstance()) return this;
if (other.getOrderId() != com.google.protobuf.ByteString.EMPTY) {
setOrderId(other.getOrderId());
}
if (other.getVolume() != 0L) {
setVolume(other.getVolume());
}
if (other.getFee() != 0L) {
setFee(other.getFee());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
orderId_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 10
case 16: {
volume_ = input.readInt64();
bitField0_ |= 0x00000002;
break;
} // case 16
case 24: {
fee_ = input.readInt64();
bitField0_ |= 0x00000004;
break;
} // case 24
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString orderId_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes order_id = 1;
* @return The orderId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getOrderId() {
return orderId_;
}
/**
* bytes order_id = 1;
* @param value The orderId to set.
* @return This builder for chaining.
*/
public Builder setOrderId(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
orderId_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* bytes order_id = 1;
* @return This builder for chaining.
*/
public Builder clearOrderId() {
bitField0_ = (bitField0_ & ~0x00000001);
orderId_ = getDefaultInstance().getOrderId();
onChanged();
return this;
}
private long volume_ ;
/**
* int64 volume = 2;
* @return The volume.
*/
@java.lang.Override
public long getVolume() {
return volume_;
}
/**
* int64 volume = 2;
* @param value The volume to set.
* @return This builder for chaining.
*/
public Builder setVolume(long value) {
volume_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* int64 volume = 2;
* @return This builder for chaining.
*/
public Builder clearVolume() {
bitField0_ = (bitField0_ & ~0x00000002);
volume_ = 0L;
onChanged();
return this;
}
private long fee_ ;
/**
* int64 fee = 3;
* @return The fee.
*/
@java.lang.Override
public long getFee() {
return fee_;
}
/**
* int64 fee = 3;
* @param value The fee to set.
* @return This builder for chaining.
*/
public Builder setFee(long value) {
fee_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* int64 fee = 3;
* @return This builder for chaining.
*/
public Builder clearFee() {
bitField0_ = (bitField0_ & ~0x00000004);
fee_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:waves.TransactionStateSnapshot.OrderFill)
}
// @@protoc_insertion_point(class_scope:waves.TransactionStateSnapshot.OrderFill)
private static final com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill();
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public OrderFill parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface AccountScriptOrBuilder extends
// @@protoc_insertion_point(interface_extends:waves.TransactionStateSnapshot.AccountScript)
com.google.protobuf.MessageOrBuilder {
/**
* bytes sender_public_key = 1;
* @return The senderPublicKey.
*/
com.google.protobuf.ByteString getSenderPublicKey();
/**
* bytes script = 2;
* @return The script.
*/
com.google.protobuf.ByteString getScript();
/**
* int64 verifier_complexity = 3;
* @return The verifierComplexity.
*/
long getVerifierComplexity();
}
/**
* Protobuf type {@code waves.TransactionStateSnapshot.AccountScript}
*/
public static final class AccountScript extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:waves.TransactionStateSnapshot.AccountScript)
AccountScriptOrBuilder {
private static final long serialVersionUID = 0L;
// Use AccountScript.newBuilder() to construct.
private AccountScript(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private AccountScript() {
senderPublicKey_ = com.google.protobuf.ByteString.EMPTY;
script_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new AccountScript();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_AccountScript_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_AccountScript_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript.class, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript.Builder.class);
}
public static final int SENDER_PUBLIC_KEY_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString senderPublicKey_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes sender_public_key = 1;
* @return The senderPublicKey.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSenderPublicKey() {
return senderPublicKey_;
}
public static final int SCRIPT_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString script_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes script = 2;
* @return The script.
*/
@java.lang.Override
public com.google.protobuf.ByteString getScript() {
return script_;
}
public static final int VERIFIER_COMPLEXITY_FIELD_NUMBER = 3;
private long verifierComplexity_ = 0L;
/**
* int64 verifier_complexity = 3;
* @return The verifierComplexity.
*/
@java.lang.Override
public long getVerifierComplexity() {
return verifierComplexity_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!senderPublicKey_.isEmpty()) {
output.writeBytes(1, senderPublicKey_);
}
if (!script_.isEmpty()) {
output.writeBytes(2, script_);
}
if (verifierComplexity_ != 0L) {
output.writeInt64(3, verifierComplexity_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!senderPublicKey_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, senderPublicKey_);
}
if (!script_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, script_);
}
if (verifierComplexity_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(3, verifierComplexity_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript)) {
return super.equals(obj);
}
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript other = (com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript) obj;
if (!getSenderPublicKey()
.equals(other.getSenderPublicKey())) return false;
if (!getScript()
.equals(other.getScript())) return false;
if (getVerifierComplexity()
!= other.getVerifierComplexity()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + SENDER_PUBLIC_KEY_FIELD_NUMBER;
hash = (53 * hash) + getSenderPublicKey().hashCode();
hash = (37 * hash) + SCRIPT_FIELD_NUMBER;
hash = (53 * hash) + getScript().hashCode();
hash = (37 * hash) + VERIFIER_COMPLEXITY_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getVerifierComplexity());
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code waves.TransactionStateSnapshot.AccountScript}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:waves.TransactionStateSnapshot.AccountScript)
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScriptOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_AccountScript_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_AccountScript_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript.class, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript.Builder.class);
}
// Construct using com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
senderPublicKey_ = com.google.protobuf.ByteString.EMPTY;
script_ = com.google.protobuf.ByteString.EMPTY;
verifierComplexity_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_AccountScript_descriptor;
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript getDefaultInstanceForType() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript.getDefaultInstance();
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript build() {
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript buildPartial() {
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript result = new com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.senderPublicKey_ = senderPublicKey_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.script_ = script_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.verifierComplexity_ = verifierComplexity_;
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript) {
return mergeFrom((com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript other) {
if (other == com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript.getDefaultInstance()) return this;
if (other.getSenderPublicKey() != com.google.protobuf.ByteString.EMPTY) {
setSenderPublicKey(other.getSenderPublicKey());
}
if (other.getScript() != com.google.protobuf.ByteString.EMPTY) {
setScript(other.getScript());
}
if (other.getVerifierComplexity() != 0L) {
setVerifierComplexity(other.getVerifierComplexity());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
senderPublicKey_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
script_ = input.readBytes();
bitField0_ |= 0x00000002;
break;
} // case 18
case 24: {
verifierComplexity_ = input.readInt64();
bitField0_ |= 0x00000004;
break;
} // case 24
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString senderPublicKey_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes sender_public_key = 1;
* @return The senderPublicKey.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSenderPublicKey() {
return senderPublicKey_;
}
/**
* bytes sender_public_key = 1;
* @param value The senderPublicKey to set.
* @return This builder for chaining.
*/
public Builder setSenderPublicKey(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
senderPublicKey_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* bytes sender_public_key = 1;
* @return This builder for chaining.
*/
public Builder clearSenderPublicKey() {
bitField0_ = (bitField0_ & ~0x00000001);
senderPublicKey_ = getDefaultInstance().getSenderPublicKey();
onChanged();
return this;
}
private com.google.protobuf.ByteString script_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes script = 2;
* @return The script.
*/
@java.lang.Override
public com.google.protobuf.ByteString getScript() {
return script_;
}
/**
* bytes script = 2;
* @param value The script to set.
* @return This builder for chaining.
*/
public Builder setScript(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
script_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* bytes script = 2;
* @return This builder for chaining.
*/
public Builder clearScript() {
bitField0_ = (bitField0_ & ~0x00000002);
script_ = getDefaultInstance().getScript();
onChanged();
return this;
}
private long verifierComplexity_ ;
/**
* int64 verifier_complexity = 3;
* @return The verifierComplexity.
*/
@java.lang.Override
public long getVerifierComplexity() {
return verifierComplexity_;
}
/**
* int64 verifier_complexity = 3;
* @param value The verifierComplexity to set.
* @return This builder for chaining.
*/
public Builder setVerifierComplexity(long value) {
verifierComplexity_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* int64 verifier_complexity = 3;
* @return This builder for chaining.
*/
public Builder clearVerifierComplexity() {
bitField0_ = (bitField0_ & ~0x00000004);
verifierComplexity_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:waves.TransactionStateSnapshot.AccountScript)
}
// @@protoc_insertion_point(class_scope:waves.TransactionStateSnapshot.AccountScript)
private static final com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript();
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public AccountScript parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface AccountDataOrBuilder extends
// @@protoc_insertion_point(interface_extends:waves.TransactionStateSnapshot.AccountData)
com.google.protobuf.MessageOrBuilder {
/**
* bytes address = 1;
* @return The address.
*/
com.google.protobuf.ByteString getAddress();
/**
* repeated .waves.DataEntry entries = 2;
*/
java.util.List
getEntriesList();
/**
* repeated .waves.DataEntry entries = 2;
*/
com.wavesplatform.protobuf.transaction.TransactionOuterClass.DataEntry getEntries(int index);
/**
* repeated .waves.DataEntry entries = 2;
*/
int getEntriesCount();
/**
* repeated .waves.DataEntry entries = 2;
*/
java.util.List extends com.wavesplatform.protobuf.transaction.TransactionOuterClass.DataEntryOrBuilder>
getEntriesOrBuilderList();
/**
* repeated .waves.DataEntry entries = 2;
*/
com.wavesplatform.protobuf.transaction.TransactionOuterClass.DataEntryOrBuilder getEntriesOrBuilder(
int index);
}
/**
* Protobuf type {@code waves.TransactionStateSnapshot.AccountData}
*/
public static final class AccountData extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:waves.TransactionStateSnapshot.AccountData)
AccountDataOrBuilder {
private static final long serialVersionUID = 0L;
// Use AccountData.newBuilder() to construct.
private AccountData(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private AccountData() {
address_ = com.google.protobuf.ByteString.EMPTY;
entries_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new AccountData();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_AccountData_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_AccountData_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData.class, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData.Builder.class);
}
public static final int ADDRESS_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString address_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes address = 1;
* @return The address.
*/
@java.lang.Override
public com.google.protobuf.ByteString getAddress() {
return address_;
}
public static final int ENTRIES_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private java.util.List entries_;
/**
* repeated .waves.DataEntry entries = 2;
*/
@java.lang.Override
public java.util.List getEntriesList() {
return entries_;
}
/**
* repeated .waves.DataEntry entries = 2;
*/
@java.lang.Override
public java.util.List extends com.wavesplatform.protobuf.transaction.TransactionOuterClass.DataEntryOrBuilder>
getEntriesOrBuilderList() {
return entries_;
}
/**
* repeated .waves.DataEntry entries = 2;
*/
@java.lang.Override
public int getEntriesCount() {
return entries_.size();
}
/**
* repeated .waves.DataEntry entries = 2;
*/
@java.lang.Override
public com.wavesplatform.protobuf.transaction.TransactionOuterClass.DataEntry getEntries(int index) {
return entries_.get(index);
}
/**
* repeated .waves.DataEntry entries = 2;
*/
@java.lang.Override
public com.wavesplatform.protobuf.transaction.TransactionOuterClass.DataEntryOrBuilder getEntriesOrBuilder(
int index) {
return entries_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!address_.isEmpty()) {
output.writeBytes(1, address_);
}
for (int i = 0; i < entries_.size(); i++) {
output.writeMessage(2, entries_.get(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!address_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, address_);
}
for (int i = 0; i < entries_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, entries_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData)) {
return super.equals(obj);
}
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData other = (com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData) obj;
if (!getAddress()
.equals(other.getAddress())) return false;
if (!getEntriesList()
.equals(other.getEntriesList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getAddress().hashCode();
if (getEntriesCount() > 0) {
hash = (37 * hash) + ENTRIES_FIELD_NUMBER;
hash = (53 * hash) + getEntriesList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code waves.TransactionStateSnapshot.AccountData}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:waves.TransactionStateSnapshot.AccountData)
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountDataOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_AccountData_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_AccountData_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData.class, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData.Builder.class);
}
// Construct using com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
address_ = com.google.protobuf.ByteString.EMPTY;
if (entriesBuilder_ == null) {
entries_ = java.util.Collections.emptyList();
} else {
entries_ = null;
entriesBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_AccountData_descriptor;
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData getDefaultInstanceForType() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData.getDefaultInstance();
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData build() {
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData buildPartial() {
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData result = new com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData result) {
if (entriesBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0)) {
entries_ = java.util.Collections.unmodifiableList(entries_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.entries_ = entries_;
} else {
result.entries_ = entriesBuilder_.build();
}
}
private void buildPartial0(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.address_ = address_;
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData) {
return mergeFrom((com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData other) {
if (other == com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData.getDefaultInstance()) return this;
if (other.getAddress() != com.google.protobuf.ByteString.EMPTY) {
setAddress(other.getAddress());
}
if (entriesBuilder_ == null) {
if (!other.entries_.isEmpty()) {
if (entries_.isEmpty()) {
entries_ = other.entries_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureEntriesIsMutable();
entries_.addAll(other.entries_);
}
onChanged();
}
} else {
if (!other.entries_.isEmpty()) {
if (entriesBuilder_.isEmpty()) {
entriesBuilder_.dispose();
entriesBuilder_ = null;
entries_ = other.entries_;
bitField0_ = (bitField0_ & ~0x00000002);
entriesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getEntriesFieldBuilder() : null;
} else {
entriesBuilder_.addAllMessages(other.entries_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
address_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
com.wavesplatform.protobuf.transaction.TransactionOuterClass.DataEntry m =
input.readMessage(
com.wavesplatform.protobuf.transaction.TransactionOuterClass.DataEntry.parser(),
extensionRegistry);
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
entries_.add(m);
} else {
entriesBuilder_.addMessage(m);
}
break;
} // case 18
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString address_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes address = 1;
* @return The address.
*/
@java.lang.Override
public com.google.protobuf.ByteString getAddress() {
return address_;
}
/**
* bytes address = 1;
* @param value The address to set.
* @return This builder for chaining.
*/
public Builder setAddress(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
address_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* bytes address = 1;
* @return This builder for chaining.
*/
public Builder clearAddress() {
bitField0_ = (bitField0_ & ~0x00000001);
address_ = getDefaultInstance().getAddress();
onChanged();
return this;
}
private java.util.List entries_ =
java.util.Collections.emptyList();
private void ensureEntriesIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
entries_ = new java.util.ArrayList(entries_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.wavesplatform.protobuf.transaction.TransactionOuterClass.DataEntry, com.wavesplatform.protobuf.transaction.TransactionOuterClass.DataEntry.Builder, com.wavesplatform.protobuf.transaction.TransactionOuterClass.DataEntryOrBuilder> entriesBuilder_;
/**
* repeated .waves.DataEntry entries = 2;
*/
public java.util.List getEntriesList() {
if (entriesBuilder_ == null) {
return java.util.Collections.unmodifiableList(entries_);
} else {
return entriesBuilder_.getMessageList();
}
}
/**
* repeated .waves.DataEntry entries = 2;
*/
public int getEntriesCount() {
if (entriesBuilder_ == null) {
return entries_.size();
} else {
return entriesBuilder_.getCount();
}
}
/**
* repeated .waves.DataEntry entries = 2;
*/
public com.wavesplatform.protobuf.transaction.TransactionOuterClass.DataEntry getEntries(int index) {
if (entriesBuilder_ == null) {
return entries_.get(index);
} else {
return entriesBuilder_.getMessage(index);
}
}
/**
* repeated .waves.DataEntry entries = 2;
*/
public Builder setEntries(
int index, com.wavesplatform.protobuf.transaction.TransactionOuterClass.DataEntry value) {
if (entriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEntriesIsMutable();
entries_.set(index, value);
onChanged();
} else {
entriesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .waves.DataEntry entries = 2;
*/
public Builder setEntries(
int index, com.wavesplatform.protobuf.transaction.TransactionOuterClass.DataEntry.Builder builderForValue) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
entries_.set(index, builderForValue.build());
onChanged();
} else {
entriesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .waves.DataEntry entries = 2;
*/
public Builder addEntries(com.wavesplatform.protobuf.transaction.TransactionOuterClass.DataEntry value) {
if (entriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEntriesIsMutable();
entries_.add(value);
onChanged();
} else {
entriesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .waves.DataEntry entries = 2;
*/
public Builder addEntries(
int index, com.wavesplatform.protobuf.transaction.TransactionOuterClass.DataEntry value) {
if (entriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEntriesIsMutable();
entries_.add(index, value);
onChanged();
} else {
entriesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .waves.DataEntry entries = 2;
*/
public Builder addEntries(
com.wavesplatform.protobuf.transaction.TransactionOuterClass.DataEntry.Builder builderForValue) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
entries_.add(builderForValue.build());
onChanged();
} else {
entriesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .waves.DataEntry entries = 2;
*/
public Builder addEntries(
int index, com.wavesplatform.protobuf.transaction.TransactionOuterClass.DataEntry.Builder builderForValue) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
entries_.add(index, builderForValue.build());
onChanged();
} else {
entriesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .waves.DataEntry entries = 2;
*/
public Builder addAllEntries(
java.lang.Iterable extends com.wavesplatform.protobuf.transaction.TransactionOuterClass.DataEntry> values) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, entries_);
onChanged();
} else {
entriesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .waves.DataEntry entries = 2;
*/
public Builder clearEntries() {
if (entriesBuilder_ == null) {
entries_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
entriesBuilder_.clear();
}
return this;
}
/**
* repeated .waves.DataEntry entries = 2;
*/
public Builder removeEntries(int index) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
entries_.remove(index);
onChanged();
} else {
entriesBuilder_.remove(index);
}
return this;
}
/**
* repeated .waves.DataEntry entries = 2;
*/
public com.wavesplatform.protobuf.transaction.TransactionOuterClass.DataEntry.Builder getEntriesBuilder(
int index) {
return getEntriesFieldBuilder().getBuilder(index);
}
/**
* repeated .waves.DataEntry entries = 2;
*/
public com.wavesplatform.protobuf.transaction.TransactionOuterClass.DataEntryOrBuilder getEntriesOrBuilder(
int index) {
if (entriesBuilder_ == null) {
return entries_.get(index); } else {
return entriesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .waves.DataEntry entries = 2;
*/
public java.util.List extends com.wavesplatform.protobuf.transaction.TransactionOuterClass.DataEntryOrBuilder>
getEntriesOrBuilderList() {
if (entriesBuilder_ != null) {
return entriesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(entries_);
}
}
/**
* repeated .waves.DataEntry entries = 2;
*/
public com.wavesplatform.protobuf.transaction.TransactionOuterClass.DataEntry.Builder addEntriesBuilder() {
return getEntriesFieldBuilder().addBuilder(
com.wavesplatform.protobuf.transaction.TransactionOuterClass.DataEntry.getDefaultInstance());
}
/**
* repeated .waves.DataEntry entries = 2;
*/
public com.wavesplatform.protobuf.transaction.TransactionOuterClass.DataEntry.Builder addEntriesBuilder(
int index) {
return getEntriesFieldBuilder().addBuilder(
index, com.wavesplatform.protobuf.transaction.TransactionOuterClass.DataEntry.getDefaultInstance());
}
/**
* repeated .waves.DataEntry entries = 2;
*/
public java.util.List
getEntriesBuilderList() {
return getEntriesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.wavesplatform.protobuf.transaction.TransactionOuterClass.DataEntry, com.wavesplatform.protobuf.transaction.TransactionOuterClass.DataEntry.Builder, com.wavesplatform.protobuf.transaction.TransactionOuterClass.DataEntryOrBuilder>
getEntriesFieldBuilder() {
if (entriesBuilder_ == null) {
entriesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.wavesplatform.protobuf.transaction.TransactionOuterClass.DataEntry, com.wavesplatform.protobuf.transaction.TransactionOuterClass.DataEntry.Builder, com.wavesplatform.protobuf.transaction.TransactionOuterClass.DataEntryOrBuilder>(
entries_,
((bitField0_ & 0x00000002) != 0),
getParentForChildren(),
isClean());
entries_ = null;
}
return entriesBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:waves.TransactionStateSnapshot.AccountData)
}
// @@protoc_insertion_point(class_scope:waves.TransactionStateSnapshot.AccountData)
private static final com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData();
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public AccountData parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface SponsorshipOrBuilder extends
// @@protoc_insertion_point(interface_extends:waves.TransactionStateSnapshot.Sponsorship)
com.google.protobuf.MessageOrBuilder {
/**
* bytes asset_id = 1;
* @return The assetId.
*/
com.google.protobuf.ByteString getAssetId();
/**
* int64 min_fee = 2;
* @return The minFee.
*/
long getMinFee();
}
/**
* Protobuf type {@code waves.TransactionStateSnapshot.Sponsorship}
*/
public static final class Sponsorship extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:waves.TransactionStateSnapshot.Sponsorship)
SponsorshipOrBuilder {
private static final long serialVersionUID = 0L;
// Use Sponsorship.newBuilder() to construct.
private Sponsorship(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Sponsorship() {
assetId_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Sponsorship();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_Sponsorship_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_Sponsorship_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship.class, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship.Builder.class);
}
public static final int ASSET_ID_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString assetId_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes asset_id = 1;
* @return The assetId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getAssetId() {
return assetId_;
}
public static final int MIN_FEE_FIELD_NUMBER = 2;
private long minFee_ = 0L;
/**
* int64 min_fee = 2;
* @return The minFee.
*/
@java.lang.Override
public long getMinFee() {
return minFee_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!assetId_.isEmpty()) {
output.writeBytes(1, assetId_);
}
if (minFee_ != 0L) {
output.writeInt64(2, minFee_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!assetId_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, assetId_);
}
if (minFee_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(2, minFee_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship)) {
return super.equals(obj);
}
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship other = (com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship) obj;
if (!getAssetId()
.equals(other.getAssetId())) return false;
if (getMinFee()
!= other.getMinFee()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ASSET_ID_FIELD_NUMBER;
hash = (53 * hash) + getAssetId().hashCode();
hash = (37 * hash) + MIN_FEE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getMinFee());
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code waves.TransactionStateSnapshot.Sponsorship}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:waves.TransactionStateSnapshot.Sponsorship)
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.SponsorshipOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_Sponsorship_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_Sponsorship_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship.class, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship.Builder.class);
}
// Construct using com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
assetId_ = com.google.protobuf.ByteString.EMPTY;
minFee_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_Sponsorship_descriptor;
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship getDefaultInstanceForType() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship.getDefaultInstance();
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship build() {
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship buildPartial() {
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship result = new com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.assetId_ = assetId_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.minFee_ = minFee_;
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship) {
return mergeFrom((com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship other) {
if (other == com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship.getDefaultInstance()) return this;
if (other.getAssetId() != com.google.protobuf.ByteString.EMPTY) {
setAssetId(other.getAssetId());
}
if (other.getMinFee() != 0L) {
setMinFee(other.getMinFee());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
assetId_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 10
case 16: {
minFee_ = input.readInt64();
bitField0_ |= 0x00000002;
break;
} // case 16
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString assetId_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes asset_id = 1;
* @return The assetId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getAssetId() {
return assetId_;
}
/**
* bytes asset_id = 1;
* @param value The assetId to set.
* @return This builder for chaining.
*/
public Builder setAssetId(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
assetId_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* bytes asset_id = 1;
* @return This builder for chaining.
*/
public Builder clearAssetId() {
bitField0_ = (bitField0_ & ~0x00000001);
assetId_ = getDefaultInstance().getAssetId();
onChanged();
return this;
}
private long minFee_ ;
/**
* int64 min_fee = 2;
* @return The minFee.
*/
@java.lang.Override
public long getMinFee() {
return minFee_;
}
/**
* int64 min_fee = 2;
* @param value The minFee to set.
* @return This builder for chaining.
*/
public Builder setMinFee(long value) {
minFee_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* int64 min_fee = 2;
* @return This builder for chaining.
*/
public Builder clearMinFee() {
bitField0_ = (bitField0_ & ~0x00000002);
minFee_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:waves.TransactionStateSnapshot.Sponsorship)
}
// @@protoc_insertion_point(class_scope:waves.TransactionStateSnapshot.Sponsorship)
private static final com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship();
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Sponsorship parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
public static final int BALANCES_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private java.util.List balances_;
/**
* repeated .waves.TransactionStateSnapshot.Balance balances = 1;
*/
@java.lang.Override
public java.util.List getBalancesList() {
return balances_;
}
/**
* repeated .waves.TransactionStateSnapshot.Balance balances = 1;
*/
@java.lang.Override
public java.util.List extends com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.BalanceOrBuilder>
getBalancesOrBuilderList() {
return balances_;
}
/**
* repeated .waves.TransactionStateSnapshot.Balance balances = 1;
*/
@java.lang.Override
public int getBalancesCount() {
return balances_.size();
}
/**
* repeated .waves.TransactionStateSnapshot.Balance balances = 1;
*/
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance getBalances(int index) {
return balances_.get(index);
}
/**
* repeated .waves.TransactionStateSnapshot.Balance balances = 1;
*/
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.BalanceOrBuilder getBalancesOrBuilder(
int index) {
return balances_.get(index);
}
public static final int LEASE_BALANCES_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private java.util.List leaseBalances_;
/**
* repeated .waves.TransactionStateSnapshot.LeaseBalance lease_balances = 2;
*/
@java.lang.Override
public java.util.List getLeaseBalancesList() {
return leaseBalances_;
}
/**
* repeated .waves.TransactionStateSnapshot.LeaseBalance lease_balances = 2;
*/
@java.lang.Override
public java.util.List extends com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalanceOrBuilder>
getLeaseBalancesOrBuilderList() {
return leaseBalances_;
}
/**
* repeated .waves.TransactionStateSnapshot.LeaseBalance lease_balances = 2;
*/
@java.lang.Override
public int getLeaseBalancesCount() {
return leaseBalances_.size();
}
/**
* repeated .waves.TransactionStateSnapshot.LeaseBalance lease_balances = 2;
*/
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance getLeaseBalances(int index) {
return leaseBalances_.get(index);
}
/**
* repeated .waves.TransactionStateSnapshot.LeaseBalance lease_balances = 2;
*/
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalanceOrBuilder getLeaseBalancesOrBuilder(
int index) {
return leaseBalances_.get(index);
}
public static final int NEW_LEASES_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private java.util.List newLeases_;
/**
* repeated .waves.TransactionStateSnapshot.NewLease new_leases = 3;
*/
@java.lang.Override
public java.util.List getNewLeasesList() {
return newLeases_;
}
/**
* repeated .waves.TransactionStateSnapshot.NewLease new_leases = 3;
*/
@java.lang.Override
public java.util.List extends com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLeaseOrBuilder>
getNewLeasesOrBuilderList() {
return newLeases_;
}
/**
* repeated .waves.TransactionStateSnapshot.NewLease new_leases = 3;
*/
@java.lang.Override
public int getNewLeasesCount() {
return newLeases_.size();
}
/**
* repeated .waves.TransactionStateSnapshot.NewLease new_leases = 3;
*/
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease getNewLeases(int index) {
return newLeases_.get(index);
}
/**
* repeated .waves.TransactionStateSnapshot.NewLease new_leases = 3;
*/
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLeaseOrBuilder getNewLeasesOrBuilder(
int index) {
return newLeases_.get(index);
}
public static final int CANCELLED_LEASES_FIELD_NUMBER = 4;
@SuppressWarnings("serial")
private java.util.List cancelledLeases_;
/**
* repeated .waves.TransactionStateSnapshot.CancelledLease cancelled_leases = 4;
*/
@java.lang.Override
public java.util.List getCancelledLeasesList() {
return cancelledLeases_;
}
/**
* repeated .waves.TransactionStateSnapshot.CancelledLease cancelled_leases = 4;
*/
@java.lang.Override
public java.util.List extends com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLeaseOrBuilder>
getCancelledLeasesOrBuilderList() {
return cancelledLeases_;
}
/**
* repeated .waves.TransactionStateSnapshot.CancelledLease cancelled_leases = 4;
*/
@java.lang.Override
public int getCancelledLeasesCount() {
return cancelledLeases_.size();
}
/**
* repeated .waves.TransactionStateSnapshot.CancelledLease cancelled_leases = 4;
*/
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease getCancelledLeases(int index) {
return cancelledLeases_.get(index);
}
/**
* repeated .waves.TransactionStateSnapshot.CancelledLease cancelled_leases = 4;
*/
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLeaseOrBuilder getCancelledLeasesOrBuilder(
int index) {
return cancelledLeases_.get(index);
}
public static final int ASSET_STATICS_FIELD_NUMBER = 5;
@SuppressWarnings("serial")
private java.util.List assetStatics_;
/**
* repeated .waves.TransactionStateSnapshot.NewAsset asset_statics = 5;
*/
@java.lang.Override
public java.util.List getAssetStaticsList() {
return assetStatics_;
}
/**
* repeated .waves.TransactionStateSnapshot.NewAsset asset_statics = 5;
*/
@java.lang.Override
public java.util.List extends com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAssetOrBuilder>
getAssetStaticsOrBuilderList() {
return assetStatics_;
}
/**
* repeated .waves.TransactionStateSnapshot.NewAsset asset_statics = 5;
*/
@java.lang.Override
public int getAssetStaticsCount() {
return assetStatics_.size();
}
/**
* repeated .waves.TransactionStateSnapshot.NewAsset asset_statics = 5;
*/
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset getAssetStatics(int index) {
return assetStatics_.get(index);
}
/**
* repeated .waves.TransactionStateSnapshot.NewAsset asset_statics = 5;
*/
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAssetOrBuilder getAssetStaticsOrBuilder(
int index) {
return assetStatics_.get(index);
}
public static final int ASSET_VOLUMES_FIELD_NUMBER = 6;
@SuppressWarnings("serial")
private java.util.List assetVolumes_;
/**
* repeated .waves.TransactionStateSnapshot.AssetVolume asset_volumes = 6;
*/
@java.lang.Override
public java.util.List getAssetVolumesList() {
return assetVolumes_;
}
/**
* repeated .waves.TransactionStateSnapshot.AssetVolume asset_volumes = 6;
*/
@java.lang.Override
public java.util.List extends com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolumeOrBuilder>
getAssetVolumesOrBuilderList() {
return assetVolumes_;
}
/**
* repeated .waves.TransactionStateSnapshot.AssetVolume asset_volumes = 6;
*/
@java.lang.Override
public int getAssetVolumesCount() {
return assetVolumes_.size();
}
/**
* repeated .waves.TransactionStateSnapshot.AssetVolume asset_volumes = 6;
*/
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume getAssetVolumes(int index) {
return assetVolumes_.get(index);
}
/**
* repeated .waves.TransactionStateSnapshot.AssetVolume asset_volumes = 6;
*/
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolumeOrBuilder getAssetVolumesOrBuilder(
int index) {
return assetVolumes_.get(index);
}
public static final int ASSET_NAMES_AND_DESCRIPTIONS_FIELD_NUMBER = 7;
@SuppressWarnings("serial")
private java.util.List assetNamesAndDescriptions_;
/**
* repeated .waves.TransactionStateSnapshot.AssetNameAndDescription asset_names_and_descriptions = 7;
*/
@java.lang.Override
public java.util.List getAssetNamesAndDescriptionsList() {
return assetNamesAndDescriptions_;
}
/**
* repeated .waves.TransactionStateSnapshot.AssetNameAndDescription asset_names_and_descriptions = 7;
*/
@java.lang.Override
public java.util.List extends com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescriptionOrBuilder>
getAssetNamesAndDescriptionsOrBuilderList() {
return assetNamesAndDescriptions_;
}
/**
* repeated .waves.TransactionStateSnapshot.AssetNameAndDescription asset_names_and_descriptions = 7;
*/
@java.lang.Override
public int getAssetNamesAndDescriptionsCount() {
return assetNamesAndDescriptions_.size();
}
/**
* repeated .waves.TransactionStateSnapshot.AssetNameAndDescription asset_names_and_descriptions = 7;
*/
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription getAssetNamesAndDescriptions(int index) {
return assetNamesAndDescriptions_.get(index);
}
/**
* repeated .waves.TransactionStateSnapshot.AssetNameAndDescription asset_names_and_descriptions = 7;
*/
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescriptionOrBuilder getAssetNamesAndDescriptionsOrBuilder(
int index) {
return assetNamesAndDescriptions_.get(index);
}
public static final int ASSET_SCRIPTS_FIELD_NUMBER = 8;
private com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript assetScripts_;
/**
* .waves.TransactionStateSnapshot.AssetScript asset_scripts = 8;
* @return Whether the assetScripts field is set.
*/
@java.lang.Override
public boolean hasAssetScripts() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* .waves.TransactionStateSnapshot.AssetScript asset_scripts = 8;
* @return The assetScripts.
*/
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript getAssetScripts() {
return assetScripts_ == null ? com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript.getDefaultInstance() : assetScripts_;
}
/**
* .waves.TransactionStateSnapshot.AssetScript asset_scripts = 8;
*/
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScriptOrBuilder getAssetScriptsOrBuilder() {
return assetScripts_ == null ? com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript.getDefaultInstance() : assetScripts_;
}
public static final int ALIASES_FIELD_NUMBER = 9;
private com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias aliases_;
/**
* .waves.TransactionStateSnapshot.Alias aliases = 9;
* @return Whether the aliases field is set.
*/
@java.lang.Override
public boolean hasAliases() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* .waves.TransactionStateSnapshot.Alias aliases = 9;
* @return The aliases.
*/
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias getAliases() {
return aliases_ == null ? com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias.getDefaultInstance() : aliases_;
}
/**
* .waves.TransactionStateSnapshot.Alias aliases = 9;
*/
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AliasOrBuilder getAliasesOrBuilder() {
return aliases_ == null ? com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias.getDefaultInstance() : aliases_;
}
public static final int ORDER_FILLS_FIELD_NUMBER = 10;
@SuppressWarnings("serial")
private java.util.List orderFills_;
/**
* repeated .waves.TransactionStateSnapshot.OrderFill order_fills = 10;
*/
@java.lang.Override
public java.util.List getOrderFillsList() {
return orderFills_;
}
/**
* repeated .waves.TransactionStateSnapshot.OrderFill order_fills = 10;
*/
@java.lang.Override
public java.util.List extends com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFillOrBuilder>
getOrderFillsOrBuilderList() {
return orderFills_;
}
/**
* repeated .waves.TransactionStateSnapshot.OrderFill order_fills = 10;
*/
@java.lang.Override
public int getOrderFillsCount() {
return orderFills_.size();
}
/**
* repeated .waves.TransactionStateSnapshot.OrderFill order_fills = 10;
*/
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill getOrderFills(int index) {
return orderFills_.get(index);
}
/**
* repeated .waves.TransactionStateSnapshot.OrderFill order_fills = 10;
*/
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFillOrBuilder getOrderFillsOrBuilder(
int index) {
return orderFills_.get(index);
}
public static final int ACCOUNT_SCRIPTS_FIELD_NUMBER = 11;
private com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript accountScripts_;
/**
* .waves.TransactionStateSnapshot.AccountScript account_scripts = 11;
* @return Whether the accountScripts field is set.
*/
@java.lang.Override
public boolean hasAccountScripts() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* .waves.TransactionStateSnapshot.AccountScript account_scripts = 11;
* @return The accountScripts.
*/
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript getAccountScripts() {
return accountScripts_ == null ? com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript.getDefaultInstance() : accountScripts_;
}
/**
* .waves.TransactionStateSnapshot.AccountScript account_scripts = 11;
*/
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScriptOrBuilder getAccountScriptsOrBuilder() {
return accountScripts_ == null ? com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript.getDefaultInstance() : accountScripts_;
}
public static final int ACCOUNT_DATA_FIELD_NUMBER = 12;
@SuppressWarnings("serial")
private java.util.List accountData_;
/**
* repeated .waves.TransactionStateSnapshot.AccountData account_data = 12;
*/
@java.lang.Override
public java.util.List getAccountDataList() {
return accountData_;
}
/**
* repeated .waves.TransactionStateSnapshot.AccountData account_data = 12;
*/
@java.lang.Override
public java.util.List extends com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountDataOrBuilder>
getAccountDataOrBuilderList() {
return accountData_;
}
/**
* repeated .waves.TransactionStateSnapshot.AccountData account_data = 12;
*/
@java.lang.Override
public int getAccountDataCount() {
return accountData_.size();
}
/**
* repeated .waves.TransactionStateSnapshot.AccountData account_data = 12;
*/
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData getAccountData(int index) {
return accountData_.get(index);
}
/**
* repeated .waves.TransactionStateSnapshot.AccountData account_data = 12;
*/
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountDataOrBuilder getAccountDataOrBuilder(
int index) {
return accountData_.get(index);
}
public static final int SPONSORSHIPS_FIELD_NUMBER = 13;
@SuppressWarnings("serial")
private java.util.List sponsorships_;
/**
* repeated .waves.TransactionStateSnapshot.Sponsorship sponsorships = 13;
*/
@java.lang.Override
public java.util.List getSponsorshipsList() {
return sponsorships_;
}
/**
* repeated .waves.TransactionStateSnapshot.Sponsorship sponsorships = 13;
*/
@java.lang.Override
public java.util.List extends com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.SponsorshipOrBuilder>
getSponsorshipsOrBuilderList() {
return sponsorships_;
}
/**
* repeated .waves.TransactionStateSnapshot.Sponsorship sponsorships = 13;
*/
@java.lang.Override
public int getSponsorshipsCount() {
return sponsorships_.size();
}
/**
* repeated .waves.TransactionStateSnapshot.Sponsorship sponsorships = 13;
*/
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship getSponsorships(int index) {
return sponsorships_.get(index);
}
/**
* repeated .waves.TransactionStateSnapshot.Sponsorship sponsorships = 13;
*/
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.SponsorshipOrBuilder getSponsorshipsOrBuilder(
int index) {
return sponsorships_.get(index);
}
public static final int TRANSACTION_STATUS_FIELD_NUMBER = 14;
private int transactionStatus_ = 0;
/**
* .waves.TransactionStatus transaction_status = 14;
* @return The enum numeric value on the wire for transactionStatus.
*/
@java.lang.Override public int getTransactionStatusValue() {
return transactionStatus_;
}
/**
* .waves.TransactionStatus transaction_status = 14;
* @return The transactionStatus.
*/
@java.lang.Override public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStatus getTransactionStatus() {
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStatus result = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStatus.forNumber(transactionStatus_);
return result == null ? com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStatus.UNRECOGNIZED : result;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < balances_.size(); i++) {
output.writeMessage(1, balances_.get(i));
}
for (int i = 0; i < leaseBalances_.size(); i++) {
output.writeMessage(2, leaseBalances_.get(i));
}
for (int i = 0; i < newLeases_.size(); i++) {
output.writeMessage(3, newLeases_.get(i));
}
for (int i = 0; i < cancelledLeases_.size(); i++) {
output.writeMessage(4, cancelledLeases_.get(i));
}
for (int i = 0; i < assetStatics_.size(); i++) {
output.writeMessage(5, assetStatics_.get(i));
}
for (int i = 0; i < assetVolumes_.size(); i++) {
output.writeMessage(6, assetVolumes_.get(i));
}
for (int i = 0; i < assetNamesAndDescriptions_.size(); i++) {
output.writeMessage(7, assetNamesAndDescriptions_.get(i));
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(8, getAssetScripts());
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(9, getAliases());
}
for (int i = 0; i < orderFills_.size(); i++) {
output.writeMessage(10, orderFills_.get(i));
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeMessage(11, getAccountScripts());
}
for (int i = 0; i < accountData_.size(); i++) {
output.writeMessage(12, accountData_.get(i));
}
for (int i = 0; i < sponsorships_.size(); i++) {
output.writeMessage(13, sponsorships_.get(i));
}
if (transactionStatus_ != com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStatus.SUCCEEDED.getNumber()) {
output.writeEnum(14, transactionStatus_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < balances_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, balances_.get(i));
}
for (int i = 0; i < leaseBalances_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, leaseBalances_.get(i));
}
for (int i = 0; i < newLeases_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, newLeases_.get(i));
}
for (int i = 0; i < cancelledLeases_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, cancelledLeases_.get(i));
}
for (int i = 0; i < assetStatics_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, assetStatics_.get(i));
}
for (int i = 0; i < assetVolumes_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, assetVolumes_.get(i));
}
for (int i = 0; i < assetNamesAndDescriptions_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, assetNamesAndDescriptions_.get(i));
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, getAssetScripts());
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, getAliases());
}
for (int i = 0; i < orderFills_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, orderFills_.get(i));
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(11, getAccountScripts());
}
for (int i = 0; i < accountData_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(12, accountData_.get(i));
}
for (int i = 0; i < sponsorships_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(13, sponsorships_.get(i));
}
if (transactionStatus_ != com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStatus.SUCCEEDED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(14, transactionStatus_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot)) {
return super.equals(obj);
}
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot other = (com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot) obj;
if (!getBalancesList()
.equals(other.getBalancesList())) return false;
if (!getLeaseBalancesList()
.equals(other.getLeaseBalancesList())) return false;
if (!getNewLeasesList()
.equals(other.getNewLeasesList())) return false;
if (!getCancelledLeasesList()
.equals(other.getCancelledLeasesList())) return false;
if (!getAssetStaticsList()
.equals(other.getAssetStaticsList())) return false;
if (!getAssetVolumesList()
.equals(other.getAssetVolumesList())) return false;
if (!getAssetNamesAndDescriptionsList()
.equals(other.getAssetNamesAndDescriptionsList())) return false;
if (hasAssetScripts() != other.hasAssetScripts()) return false;
if (hasAssetScripts()) {
if (!getAssetScripts()
.equals(other.getAssetScripts())) return false;
}
if (hasAliases() != other.hasAliases()) return false;
if (hasAliases()) {
if (!getAliases()
.equals(other.getAliases())) return false;
}
if (!getOrderFillsList()
.equals(other.getOrderFillsList())) return false;
if (hasAccountScripts() != other.hasAccountScripts()) return false;
if (hasAccountScripts()) {
if (!getAccountScripts()
.equals(other.getAccountScripts())) return false;
}
if (!getAccountDataList()
.equals(other.getAccountDataList())) return false;
if (!getSponsorshipsList()
.equals(other.getSponsorshipsList())) return false;
if (transactionStatus_ != other.transactionStatus_) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getBalancesCount() > 0) {
hash = (37 * hash) + BALANCES_FIELD_NUMBER;
hash = (53 * hash) + getBalancesList().hashCode();
}
if (getLeaseBalancesCount() > 0) {
hash = (37 * hash) + LEASE_BALANCES_FIELD_NUMBER;
hash = (53 * hash) + getLeaseBalancesList().hashCode();
}
if (getNewLeasesCount() > 0) {
hash = (37 * hash) + NEW_LEASES_FIELD_NUMBER;
hash = (53 * hash) + getNewLeasesList().hashCode();
}
if (getCancelledLeasesCount() > 0) {
hash = (37 * hash) + CANCELLED_LEASES_FIELD_NUMBER;
hash = (53 * hash) + getCancelledLeasesList().hashCode();
}
if (getAssetStaticsCount() > 0) {
hash = (37 * hash) + ASSET_STATICS_FIELD_NUMBER;
hash = (53 * hash) + getAssetStaticsList().hashCode();
}
if (getAssetVolumesCount() > 0) {
hash = (37 * hash) + ASSET_VOLUMES_FIELD_NUMBER;
hash = (53 * hash) + getAssetVolumesList().hashCode();
}
if (getAssetNamesAndDescriptionsCount() > 0) {
hash = (37 * hash) + ASSET_NAMES_AND_DESCRIPTIONS_FIELD_NUMBER;
hash = (53 * hash) + getAssetNamesAndDescriptionsList().hashCode();
}
if (hasAssetScripts()) {
hash = (37 * hash) + ASSET_SCRIPTS_FIELD_NUMBER;
hash = (53 * hash) + getAssetScripts().hashCode();
}
if (hasAliases()) {
hash = (37 * hash) + ALIASES_FIELD_NUMBER;
hash = (53 * hash) + getAliases().hashCode();
}
if (getOrderFillsCount() > 0) {
hash = (37 * hash) + ORDER_FILLS_FIELD_NUMBER;
hash = (53 * hash) + getOrderFillsList().hashCode();
}
if (hasAccountScripts()) {
hash = (37 * hash) + ACCOUNT_SCRIPTS_FIELD_NUMBER;
hash = (53 * hash) + getAccountScripts().hashCode();
}
if (getAccountDataCount() > 0) {
hash = (37 * hash) + ACCOUNT_DATA_FIELD_NUMBER;
hash = (53 * hash) + getAccountDataList().hashCode();
}
if (getSponsorshipsCount() > 0) {
hash = (37 * hash) + SPONSORSHIPS_FIELD_NUMBER;
hash = (53 * hash) + getSponsorshipsList().hashCode();
}
hash = (37 * hash) + TRANSACTION_STATUS_FIELD_NUMBER;
hash = (53 * hash) + transactionStatus_;
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code waves.TransactionStateSnapshot}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:waves.TransactionStateSnapshot)
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshotOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.class, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Builder.class);
}
// Construct using com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getBalancesFieldBuilder();
getLeaseBalancesFieldBuilder();
getNewLeasesFieldBuilder();
getCancelledLeasesFieldBuilder();
getAssetStaticsFieldBuilder();
getAssetVolumesFieldBuilder();
getAssetNamesAndDescriptionsFieldBuilder();
getAssetScriptsFieldBuilder();
getAliasesFieldBuilder();
getOrderFillsFieldBuilder();
getAccountScriptsFieldBuilder();
getAccountDataFieldBuilder();
getSponsorshipsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
if (balancesBuilder_ == null) {
balances_ = java.util.Collections.emptyList();
} else {
balances_ = null;
balancesBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
if (leaseBalancesBuilder_ == null) {
leaseBalances_ = java.util.Collections.emptyList();
} else {
leaseBalances_ = null;
leaseBalancesBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
if (newLeasesBuilder_ == null) {
newLeases_ = java.util.Collections.emptyList();
} else {
newLeases_ = null;
newLeasesBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
if (cancelledLeasesBuilder_ == null) {
cancelledLeases_ = java.util.Collections.emptyList();
} else {
cancelledLeases_ = null;
cancelledLeasesBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000008);
if (assetStaticsBuilder_ == null) {
assetStatics_ = java.util.Collections.emptyList();
} else {
assetStatics_ = null;
assetStaticsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000010);
if (assetVolumesBuilder_ == null) {
assetVolumes_ = java.util.Collections.emptyList();
} else {
assetVolumes_ = null;
assetVolumesBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000020);
if (assetNamesAndDescriptionsBuilder_ == null) {
assetNamesAndDescriptions_ = java.util.Collections.emptyList();
} else {
assetNamesAndDescriptions_ = null;
assetNamesAndDescriptionsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000040);
assetScripts_ = null;
if (assetScriptsBuilder_ != null) {
assetScriptsBuilder_.dispose();
assetScriptsBuilder_ = null;
}
aliases_ = null;
if (aliasesBuilder_ != null) {
aliasesBuilder_.dispose();
aliasesBuilder_ = null;
}
if (orderFillsBuilder_ == null) {
orderFills_ = java.util.Collections.emptyList();
} else {
orderFills_ = null;
orderFillsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000200);
accountScripts_ = null;
if (accountScriptsBuilder_ != null) {
accountScriptsBuilder_.dispose();
accountScriptsBuilder_ = null;
}
if (accountDataBuilder_ == null) {
accountData_ = java.util.Collections.emptyList();
} else {
accountData_ = null;
accountDataBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000800);
if (sponsorshipsBuilder_ == null) {
sponsorships_ = java.util.Collections.emptyList();
} else {
sponsorships_ = null;
sponsorshipsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00001000);
transactionStatus_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.internal_static_waves_TransactionStateSnapshot_descriptor;
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot getDefaultInstanceForType() {
return com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.getDefaultInstance();
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot build() {
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot buildPartial() {
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot result = new com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot result) {
if (balancesBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
balances_ = java.util.Collections.unmodifiableList(balances_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.balances_ = balances_;
} else {
result.balances_ = balancesBuilder_.build();
}
if (leaseBalancesBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0)) {
leaseBalances_ = java.util.Collections.unmodifiableList(leaseBalances_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.leaseBalances_ = leaseBalances_;
} else {
result.leaseBalances_ = leaseBalancesBuilder_.build();
}
if (newLeasesBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0)) {
newLeases_ = java.util.Collections.unmodifiableList(newLeases_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.newLeases_ = newLeases_;
} else {
result.newLeases_ = newLeasesBuilder_.build();
}
if (cancelledLeasesBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0)) {
cancelledLeases_ = java.util.Collections.unmodifiableList(cancelledLeases_);
bitField0_ = (bitField0_ & ~0x00000008);
}
result.cancelledLeases_ = cancelledLeases_;
} else {
result.cancelledLeases_ = cancelledLeasesBuilder_.build();
}
if (assetStaticsBuilder_ == null) {
if (((bitField0_ & 0x00000010) != 0)) {
assetStatics_ = java.util.Collections.unmodifiableList(assetStatics_);
bitField0_ = (bitField0_ & ~0x00000010);
}
result.assetStatics_ = assetStatics_;
} else {
result.assetStatics_ = assetStaticsBuilder_.build();
}
if (assetVolumesBuilder_ == null) {
if (((bitField0_ & 0x00000020) != 0)) {
assetVolumes_ = java.util.Collections.unmodifiableList(assetVolumes_);
bitField0_ = (bitField0_ & ~0x00000020);
}
result.assetVolumes_ = assetVolumes_;
} else {
result.assetVolumes_ = assetVolumesBuilder_.build();
}
if (assetNamesAndDescriptionsBuilder_ == null) {
if (((bitField0_ & 0x00000040) != 0)) {
assetNamesAndDescriptions_ = java.util.Collections.unmodifiableList(assetNamesAndDescriptions_);
bitField0_ = (bitField0_ & ~0x00000040);
}
result.assetNamesAndDescriptions_ = assetNamesAndDescriptions_;
} else {
result.assetNamesAndDescriptions_ = assetNamesAndDescriptionsBuilder_.build();
}
if (orderFillsBuilder_ == null) {
if (((bitField0_ & 0x00000200) != 0)) {
orderFills_ = java.util.Collections.unmodifiableList(orderFills_);
bitField0_ = (bitField0_ & ~0x00000200);
}
result.orderFills_ = orderFills_;
} else {
result.orderFills_ = orderFillsBuilder_.build();
}
if (accountDataBuilder_ == null) {
if (((bitField0_ & 0x00000800) != 0)) {
accountData_ = java.util.Collections.unmodifiableList(accountData_);
bitField0_ = (bitField0_ & ~0x00000800);
}
result.accountData_ = accountData_;
} else {
result.accountData_ = accountDataBuilder_.build();
}
if (sponsorshipsBuilder_ == null) {
if (((bitField0_ & 0x00001000) != 0)) {
sponsorships_ = java.util.Collections.unmodifiableList(sponsorships_);
bitField0_ = (bitField0_ & ~0x00001000);
}
result.sponsorships_ = sponsorships_;
} else {
result.sponsorships_ = sponsorshipsBuilder_.build();
}
}
private void buildPartial0(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000080) != 0)) {
result.assetScripts_ = assetScriptsBuilder_ == null
? assetScripts_
: assetScriptsBuilder_.build();
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000100) != 0)) {
result.aliases_ = aliasesBuilder_ == null
? aliases_
: aliasesBuilder_.build();
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000400) != 0)) {
result.accountScripts_ = accountScriptsBuilder_ == null
? accountScripts_
: accountScriptsBuilder_.build();
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00002000) != 0)) {
result.transactionStatus_ = transactionStatus_;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot) {
return mergeFrom((com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot other) {
if (other == com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.getDefaultInstance()) return this;
if (balancesBuilder_ == null) {
if (!other.balances_.isEmpty()) {
if (balances_.isEmpty()) {
balances_ = other.balances_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureBalancesIsMutable();
balances_.addAll(other.balances_);
}
onChanged();
}
} else {
if (!other.balances_.isEmpty()) {
if (balancesBuilder_.isEmpty()) {
balancesBuilder_.dispose();
balancesBuilder_ = null;
balances_ = other.balances_;
bitField0_ = (bitField0_ & ~0x00000001);
balancesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getBalancesFieldBuilder() : null;
} else {
balancesBuilder_.addAllMessages(other.balances_);
}
}
}
if (leaseBalancesBuilder_ == null) {
if (!other.leaseBalances_.isEmpty()) {
if (leaseBalances_.isEmpty()) {
leaseBalances_ = other.leaseBalances_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureLeaseBalancesIsMutable();
leaseBalances_.addAll(other.leaseBalances_);
}
onChanged();
}
} else {
if (!other.leaseBalances_.isEmpty()) {
if (leaseBalancesBuilder_.isEmpty()) {
leaseBalancesBuilder_.dispose();
leaseBalancesBuilder_ = null;
leaseBalances_ = other.leaseBalances_;
bitField0_ = (bitField0_ & ~0x00000002);
leaseBalancesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getLeaseBalancesFieldBuilder() : null;
} else {
leaseBalancesBuilder_.addAllMessages(other.leaseBalances_);
}
}
}
if (newLeasesBuilder_ == null) {
if (!other.newLeases_.isEmpty()) {
if (newLeases_.isEmpty()) {
newLeases_ = other.newLeases_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureNewLeasesIsMutable();
newLeases_.addAll(other.newLeases_);
}
onChanged();
}
} else {
if (!other.newLeases_.isEmpty()) {
if (newLeasesBuilder_.isEmpty()) {
newLeasesBuilder_.dispose();
newLeasesBuilder_ = null;
newLeases_ = other.newLeases_;
bitField0_ = (bitField0_ & ~0x00000004);
newLeasesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getNewLeasesFieldBuilder() : null;
} else {
newLeasesBuilder_.addAllMessages(other.newLeases_);
}
}
}
if (cancelledLeasesBuilder_ == null) {
if (!other.cancelledLeases_.isEmpty()) {
if (cancelledLeases_.isEmpty()) {
cancelledLeases_ = other.cancelledLeases_;
bitField0_ = (bitField0_ & ~0x00000008);
} else {
ensureCancelledLeasesIsMutable();
cancelledLeases_.addAll(other.cancelledLeases_);
}
onChanged();
}
} else {
if (!other.cancelledLeases_.isEmpty()) {
if (cancelledLeasesBuilder_.isEmpty()) {
cancelledLeasesBuilder_.dispose();
cancelledLeasesBuilder_ = null;
cancelledLeases_ = other.cancelledLeases_;
bitField0_ = (bitField0_ & ~0x00000008);
cancelledLeasesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getCancelledLeasesFieldBuilder() : null;
} else {
cancelledLeasesBuilder_.addAllMessages(other.cancelledLeases_);
}
}
}
if (assetStaticsBuilder_ == null) {
if (!other.assetStatics_.isEmpty()) {
if (assetStatics_.isEmpty()) {
assetStatics_ = other.assetStatics_;
bitField0_ = (bitField0_ & ~0x00000010);
} else {
ensureAssetStaticsIsMutable();
assetStatics_.addAll(other.assetStatics_);
}
onChanged();
}
} else {
if (!other.assetStatics_.isEmpty()) {
if (assetStaticsBuilder_.isEmpty()) {
assetStaticsBuilder_.dispose();
assetStaticsBuilder_ = null;
assetStatics_ = other.assetStatics_;
bitField0_ = (bitField0_ & ~0x00000010);
assetStaticsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getAssetStaticsFieldBuilder() : null;
} else {
assetStaticsBuilder_.addAllMessages(other.assetStatics_);
}
}
}
if (assetVolumesBuilder_ == null) {
if (!other.assetVolumes_.isEmpty()) {
if (assetVolumes_.isEmpty()) {
assetVolumes_ = other.assetVolumes_;
bitField0_ = (bitField0_ & ~0x00000020);
} else {
ensureAssetVolumesIsMutable();
assetVolumes_.addAll(other.assetVolumes_);
}
onChanged();
}
} else {
if (!other.assetVolumes_.isEmpty()) {
if (assetVolumesBuilder_.isEmpty()) {
assetVolumesBuilder_.dispose();
assetVolumesBuilder_ = null;
assetVolumes_ = other.assetVolumes_;
bitField0_ = (bitField0_ & ~0x00000020);
assetVolumesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getAssetVolumesFieldBuilder() : null;
} else {
assetVolumesBuilder_.addAllMessages(other.assetVolumes_);
}
}
}
if (assetNamesAndDescriptionsBuilder_ == null) {
if (!other.assetNamesAndDescriptions_.isEmpty()) {
if (assetNamesAndDescriptions_.isEmpty()) {
assetNamesAndDescriptions_ = other.assetNamesAndDescriptions_;
bitField0_ = (bitField0_ & ~0x00000040);
} else {
ensureAssetNamesAndDescriptionsIsMutable();
assetNamesAndDescriptions_.addAll(other.assetNamesAndDescriptions_);
}
onChanged();
}
} else {
if (!other.assetNamesAndDescriptions_.isEmpty()) {
if (assetNamesAndDescriptionsBuilder_.isEmpty()) {
assetNamesAndDescriptionsBuilder_.dispose();
assetNamesAndDescriptionsBuilder_ = null;
assetNamesAndDescriptions_ = other.assetNamesAndDescriptions_;
bitField0_ = (bitField0_ & ~0x00000040);
assetNamesAndDescriptionsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getAssetNamesAndDescriptionsFieldBuilder() : null;
} else {
assetNamesAndDescriptionsBuilder_.addAllMessages(other.assetNamesAndDescriptions_);
}
}
}
if (other.hasAssetScripts()) {
mergeAssetScripts(other.getAssetScripts());
}
if (other.hasAliases()) {
mergeAliases(other.getAliases());
}
if (orderFillsBuilder_ == null) {
if (!other.orderFills_.isEmpty()) {
if (orderFills_.isEmpty()) {
orderFills_ = other.orderFills_;
bitField0_ = (bitField0_ & ~0x00000200);
} else {
ensureOrderFillsIsMutable();
orderFills_.addAll(other.orderFills_);
}
onChanged();
}
} else {
if (!other.orderFills_.isEmpty()) {
if (orderFillsBuilder_.isEmpty()) {
orderFillsBuilder_.dispose();
orderFillsBuilder_ = null;
orderFills_ = other.orderFills_;
bitField0_ = (bitField0_ & ~0x00000200);
orderFillsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getOrderFillsFieldBuilder() : null;
} else {
orderFillsBuilder_.addAllMessages(other.orderFills_);
}
}
}
if (other.hasAccountScripts()) {
mergeAccountScripts(other.getAccountScripts());
}
if (accountDataBuilder_ == null) {
if (!other.accountData_.isEmpty()) {
if (accountData_.isEmpty()) {
accountData_ = other.accountData_;
bitField0_ = (bitField0_ & ~0x00000800);
} else {
ensureAccountDataIsMutable();
accountData_.addAll(other.accountData_);
}
onChanged();
}
} else {
if (!other.accountData_.isEmpty()) {
if (accountDataBuilder_.isEmpty()) {
accountDataBuilder_.dispose();
accountDataBuilder_ = null;
accountData_ = other.accountData_;
bitField0_ = (bitField0_ & ~0x00000800);
accountDataBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getAccountDataFieldBuilder() : null;
} else {
accountDataBuilder_.addAllMessages(other.accountData_);
}
}
}
if (sponsorshipsBuilder_ == null) {
if (!other.sponsorships_.isEmpty()) {
if (sponsorships_.isEmpty()) {
sponsorships_ = other.sponsorships_;
bitField0_ = (bitField0_ & ~0x00001000);
} else {
ensureSponsorshipsIsMutable();
sponsorships_.addAll(other.sponsorships_);
}
onChanged();
}
} else {
if (!other.sponsorships_.isEmpty()) {
if (sponsorshipsBuilder_.isEmpty()) {
sponsorshipsBuilder_.dispose();
sponsorshipsBuilder_ = null;
sponsorships_ = other.sponsorships_;
bitField0_ = (bitField0_ & ~0x00001000);
sponsorshipsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getSponsorshipsFieldBuilder() : null;
} else {
sponsorshipsBuilder_.addAllMessages(other.sponsorships_);
}
}
}
if (other.transactionStatus_ != 0) {
setTransactionStatusValue(other.getTransactionStatusValue());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance m =
input.readMessage(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance.parser(),
extensionRegistry);
if (balancesBuilder_ == null) {
ensureBalancesIsMutable();
balances_.add(m);
} else {
balancesBuilder_.addMessage(m);
}
break;
} // case 10
case 18: {
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance m =
input.readMessage(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance.parser(),
extensionRegistry);
if (leaseBalancesBuilder_ == null) {
ensureLeaseBalancesIsMutable();
leaseBalances_.add(m);
} else {
leaseBalancesBuilder_.addMessage(m);
}
break;
} // case 18
case 26: {
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease m =
input.readMessage(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease.parser(),
extensionRegistry);
if (newLeasesBuilder_ == null) {
ensureNewLeasesIsMutable();
newLeases_.add(m);
} else {
newLeasesBuilder_.addMessage(m);
}
break;
} // case 26
case 34: {
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease m =
input.readMessage(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease.parser(),
extensionRegistry);
if (cancelledLeasesBuilder_ == null) {
ensureCancelledLeasesIsMutable();
cancelledLeases_.add(m);
} else {
cancelledLeasesBuilder_.addMessage(m);
}
break;
} // case 34
case 42: {
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset m =
input.readMessage(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset.parser(),
extensionRegistry);
if (assetStaticsBuilder_ == null) {
ensureAssetStaticsIsMutable();
assetStatics_.add(m);
} else {
assetStaticsBuilder_.addMessage(m);
}
break;
} // case 42
case 50: {
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume m =
input.readMessage(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume.parser(),
extensionRegistry);
if (assetVolumesBuilder_ == null) {
ensureAssetVolumesIsMutable();
assetVolumes_.add(m);
} else {
assetVolumesBuilder_.addMessage(m);
}
break;
} // case 50
case 58: {
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription m =
input.readMessage(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription.parser(),
extensionRegistry);
if (assetNamesAndDescriptionsBuilder_ == null) {
ensureAssetNamesAndDescriptionsIsMutable();
assetNamesAndDescriptions_.add(m);
} else {
assetNamesAndDescriptionsBuilder_.addMessage(m);
}
break;
} // case 58
case 66: {
input.readMessage(
getAssetScriptsFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000080;
break;
} // case 66
case 74: {
input.readMessage(
getAliasesFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000100;
break;
} // case 74
case 82: {
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill m =
input.readMessage(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill.parser(),
extensionRegistry);
if (orderFillsBuilder_ == null) {
ensureOrderFillsIsMutable();
orderFills_.add(m);
} else {
orderFillsBuilder_.addMessage(m);
}
break;
} // case 82
case 90: {
input.readMessage(
getAccountScriptsFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000400;
break;
} // case 90
case 98: {
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData m =
input.readMessage(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData.parser(),
extensionRegistry);
if (accountDataBuilder_ == null) {
ensureAccountDataIsMutable();
accountData_.add(m);
} else {
accountDataBuilder_.addMessage(m);
}
break;
} // case 98
case 106: {
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship m =
input.readMessage(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship.parser(),
extensionRegistry);
if (sponsorshipsBuilder_ == null) {
ensureSponsorshipsIsMutable();
sponsorships_.add(m);
} else {
sponsorshipsBuilder_.addMessage(m);
}
break;
} // case 106
case 112: {
transactionStatus_ = input.readEnum();
bitField0_ |= 0x00002000;
break;
} // case 112
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.util.List balances_ =
java.util.Collections.emptyList();
private void ensureBalancesIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
balances_ = new java.util.ArrayList(balances_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance.Builder, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.BalanceOrBuilder> balancesBuilder_;
/**
* repeated .waves.TransactionStateSnapshot.Balance balances = 1;
*/
public java.util.List getBalancesList() {
if (balancesBuilder_ == null) {
return java.util.Collections.unmodifiableList(balances_);
} else {
return balancesBuilder_.getMessageList();
}
}
/**
* repeated .waves.TransactionStateSnapshot.Balance balances = 1;
*/
public int getBalancesCount() {
if (balancesBuilder_ == null) {
return balances_.size();
} else {
return balancesBuilder_.getCount();
}
}
/**
* repeated .waves.TransactionStateSnapshot.Balance balances = 1;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance getBalances(int index) {
if (balancesBuilder_ == null) {
return balances_.get(index);
} else {
return balancesBuilder_.getMessage(index);
}
}
/**
* repeated .waves.TransactionStateSnapshot.Balance balances = 1;
*/
public Builder setBalances(
int index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance value) {
if (balancesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureBalancesIsMutable();
balances_.set(index, value);
onChanged();
} else {
balancesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.Balance balances = 1;
*/
public Builder setBalances(
int index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance.Builder builderForValue) {
if (balancesBuilder_ == null) {
ensureBalancesIsMutable();
balances_.set(index, builderForValue.build());
onChanged();
} else {
balancesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.Balance balances = 1;
*/
public Builder addBalances(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance value) {
if (balancesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureBalancesIsMutable();
balances_.add(value);
onChanged();
} else {
balancesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.Balance balances = 1;
*/
public Builder addBalances(
int index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance value) {
if (balancesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureBalancesIsMutable();
balances_.add(index, value);
onChanged();
} else {
balancesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.Balance balances = 1;
*/
public Builder addBalances(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance.Builder builderForValue) {
if (balancesBuilder_ == null) {
ensureBalancesIsMutable();
balances_.add(builderForValue.build());
onChanged();
} else {
balancesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.Balance balances = 1;
*/
public Builder addBalances(
int index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance.Builder builderForValue) {
if (balancesBuilder_ == null) {
ensureBalancesIsMutable();
balances_.add(index, builderForValue.build());
onChanged();
} else {
balancesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.Balance balances = 1;
*/
public Builder addAllBalances(
java.lang.Iterable extends com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance> values) {
if (balancesBuilder_ == null) {
ensureBalancesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, balances_);
onChanged();
} else {
balancesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.Balance balances = 1;
*/
public Builder clearBalances() {
if (balancesBuilder_ == null) {
balances_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
balancesBuilder_.clear();
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.Balance balances = 1;
*/
public Builder removeBalances(int index) {
if (balancesBuilder_ == null) {
ensureBalancesIsMutable();
balances_.remove(index);
onChanged();
} else {
balancesBuilder_.remove(index);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.Balance balances = 1;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance.Builder getBalancesBuilder(
int index) {
return getBalancesFieldBuilder().getBuilder(index);
}
/**
* repeated .waves.TransactionStateSnapshot.Balance balances = 1;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.BalanceOrBuilder getBalancesOrBuilder(
int index) {
if (balancesBuilder_ == null) {
return balances_.get(index); } else {
return balancesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .waves.TransactionStateSnapshot.Balance balances = 1;
*/
public java.util.List extends com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.BalanceOrBuilder>
getBalancesOrBuilderList() {
if (balancesBuilder_ != null) {
return balancesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(balances_);
}
}
/**
* repeated .waves.TransactionStateSnapshot.Balance balances = 1;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance.Builder addBalancesBuilder() {
return getBalancesFieldBuilder().addBuilder(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance.getDefaultInstance());
}
/**
* repeated .waves.TransactionStateSnapshot.Balance balances = 1;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance.Builder addBalancesBuilder(
int index) {
return getBalancesFieldBuilder().addBuilder(
index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance.getDefaultInstance());
}
/**
* repeated .waves.TransactionStateSnapshot.Balance balances = 1;
*/
public java.util.List
getBalancesBuilderList() {
return getBalancesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance.Builder, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.BalanceOrBuilder>
getBalancesFieldBuilder() {
if (balancesBuilder_ == null) {
balancesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Balance.Builder, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.BalanceOrBuilder>(
balances_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
balances_ = null;
}
return balancesBuilder_;
}
private java.util.List leaseBalances_ =
java.util.Collections.emptyList();
private void ensureLeaseBalancesIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
leaseBalances_ = new java.util.ArrayList(leaseBalances_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance.Builder, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalanceOrBuilder> leaseBalancesBuilder_;
/**
* repeated .waves.TransactionStateSnapshot.LeaseBalance lease_balances = 2;
*/
public java.util.List getLeaseBalancesList() {
if (leaseBalancesBuilder_ == null) {
return java.util.Collections.unmodifiableList(leaseBalances_);
} else {
return leaseBalancesBuilder_.getMessageList();
}
}
/**
* repeated .waves.TransactionStateSnapshot.LeaseBalance lease_balances = 2;
*/
public int getLeaseBalancesCount() {
if (leaseBalancesBuilder_ == null) {
return leaseBalances_.size();
} else {
return leaseBalancesBuilder_.getCount();
}
}
/**
* repeated .waves.TransactionStateSnapshot.LeaseBalance lease_balances = 2;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance getLeaseBalances(int index) {
if (leaseBalancesBuilder_ == null) {
return leaseBalances_.get(index);
} else {
return leaseBalancesBuilder_.getMessage(index);
}
}
/**
* repeated .waves.TransactionStateSnapshot.LeaseBalance lease_balances = 2;
*/
public Builder setLeaseBalances(
int index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance value) {
if (leaseBalancesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLeaseBalancesIsMutable();
leaseBalances_.set(index, value);
onChanged();
} else {
leaseBalancesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.LeaseBalance lease_balances = 2;
*/
public Builder setLeaseBalances(
int index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance.Builder builderForValue) {
if (leaseBalancesBuilder_ == null) {
ensureLeaseBalancesIsMutable();
leaseBalances_.set(index, builderForValue.build());
onChanged();
} else {
leaseBalancesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.LeaseBalance lease_balances = 2;
*/
public Builder addLeaseBalances(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance value) {
if (leaseBalancesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLeaseBalancesIsMutable();
leaseBalances_.add(value);
onChanged();
} else {
leaseBalancesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.LeaseBalance lease_balances = 2;
*/
public Builder addLeaseBalances(
int index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance value) {
if (leaseBalancesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLeaseBalancesIsMutable();
leaseBalances_.add(index, value);
onChanged();
} else {
leaseBalancesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.LeaseBalance lease_balances = 2;
*/
public Builder addLeaseBalances(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance.Builder builderForValue) {
if (leaseBalancesBuilder_ == null) {
ensureLeaseBalancesIsMutable();
leaseBalances_.add(builderForValue.build());
onChanged();
} else {
leaseBalancesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.LeaseBalance lease_balances = 2;
*/
public Builder addLeaseBalances(
int index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance.Builder builderForValue) {
if (leaseBalancesBuilder_ == null) {
ensureLeaseBalancesIsMutable();
leaseBalances_.add(index, builderForValue.build());
onChanged();
} else {
leaseBalancesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.LeaseBalance lease_balances = 2;
*/
public Builder addAllLeaseBalances(
java.lang.Iterable extends com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance> values) {
if (leaseBalancesBuilder_ == null) {
ensureLeaseBalancesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, leaseBalances_);
onChanged();
} else {
leaseBalancesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.LeaseBalance lease_balances = 2;
*/
public Builder clearLeaseBalances() {
if (leaseBalancesBuilder_ == null) {
leaseBalances_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
leaseBalancesBuilder_.clear();
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.LeaseBalance lease_balances = 2;
*/
public Builder removeLeaseBalances(int index) {
if (leaseBalancesBuilder_ == null) {
ensureLeaseBalancesIsMutable();
leaseBalances_.remove(index);
onChanged();
} else {
leaseBalancesBuilder_.remove(index);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.LeaseBalance lease_balances = 2;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance.Builder getLeaseBalancesBuilder(
int index) {
return getLeaseBalancesFieldBuilder().getBuilder(index);
}
/**
* repeated .waves.TransactionStateSnapshot.LeaseBalance lease_balances = 2;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalanceOrBuilder getLeaseBalancesOrBuilder(
int index) {
if (leaseBalancesBuilder_ == null) {
return leaseBalances_.get(index); } else {
return leaseBalancesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .waves.TransactionStateSnapshot.LeaseBalance lease_balances = 2;
*/
public java.util.List extends com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalanceOrBuilder>
getLeaseBalancesOrBuilderList() {
if (leaseBalancesBuilder_ != null) {
return leaseBalancesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(leaseBalances_);
}
}
/**
* repeated .waves.TransactionStateSnapshot.LeaseBalance lease_balances = 2;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance.Builder addLeaseBalancesBuilder() {
return getLeaseBalancesFieldBuilder().addBuilder(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance.getDefaultInstance());
}
/**
* repeated .waves.TransactionStateSnapshot.LeaseBalance lease_balances = 2;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance.Builder addLeaseBalancesBuilder(
int index) {
return getLeaseBalancesFieldBuilder().addBuilder(
index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance.getDefaultInstance());
}
/**
* repeated .waves.TransactionStateSnapshot.LeaseBalance lease_balances = 2;
*/
public java.util.List
getLeaseBalancesBuilderList() {
return getLeaseBalancesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance.Builder, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalanceOrBuilder>
getLeaseBalancesFieldBuilder() {
if (leaseBalancesBuilder_ == null) {
leaseBalancesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalance.Builder, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.LeaseBalanceOrBuilder>(
leaseBalances_,
((bitField0_ & 0x00000002) != 0),
getParentForChildren(),
isClean());
leaseBalances_ = null;
}
return leaseBalancesBuilder_;
}
private java.util.List newLeases_ =
java.util.Collections.emptyList();
private void ensureNewLeasesIsMutable() {
if (!((bitField0_ & 0x00000004) != 0)) {
newLeases_ = new java.util.ArrayList(newLeases_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease.Builder, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLeaseOrBuilder> newLeasesBuilder_;
/**
* repeated .waves.TransactionStateSnapshot.NewLease new_leases = 3;
*/
public java.util.List getNewLeasesList() {
if (newLeasesBuilder_ == null) {
return java.util.Collections.unmodifiableList(newLeases_);
} else {
return newLeasesBuilder_.getMessageList();
}
}
/**
* repeated .waves.TransactionStateSnapshot.NewLease new_leases = 3;
*/
public int getNewLeasesCount() {
if (newLeasesBuilder_ == null) {
return newLeases_.size();
} else {
return newLeasesBuilder_.getCount();
}
}
/**
* repeated .waves.TransactionStateSnapshot.NewLease new_leases = 3;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease getNewLeases(int index) {
if (newLeasesBuilder_ == null) {
return newLeases_.get(index);
} else {
return newLeasesBuilder_.getMessage(index);
}
}
/**
* repeated .waves.TransactionStateSnapshot.NewLease new_leases = 3;
*/
public Builder setNewLeases(
int index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease value) {
if (newLeasesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureNewLeasesIsMutable();
newLeases_.set(index, value);
onChanged();
} else {
newLeasesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.NewLease new_leases = 3;
*/
public Builder setNewLeases(
int index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease.Builder builderForValue) {
if (newLeasesBuilder_ == null) {
ensureNewLeasesIsMutable();
newLeases_.set(index, builderForValue.build());
onChanged();
} else {
newLeasesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.NewLease new_leases = 3;
*/
public Builder addNewLeases(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease value) {
if (newLeasesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureNewLeasesIsMutable();
newLeases_.add(value);
onChanged();
} else {
newLeasesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.NewLease new_leases = 3;
*/
public Builder addNewLeases(
int index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease value) {
if (newLeasesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureNewLeasesIsMutable();
newLeases_.add(index, value);
onChanged();
} else {
newLeasesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.NewLease new_leases = 3;
*/
public Builder addNewLeases(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease.Builder builderForValue) {
if (newLeasesBuilder_ == null) {
ensureNewLeasesIsMutable();
newLeases_.add(builderForValue.build());
onChanged();
} else {
newLeasesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.NewLease new_leases = 3;
*/
public Builder addNewLeases(
int index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease.Builder builderForValue) {
if (newLeasesBuilder_ == null) {
ensureNewLeasesIsMutable();
newLeases_.add(index, builderForValue.build());
onChanged();
} else {
newLeasesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.NewLease new_leases = 3;
*/
public Builder addAllNewLeases(
java.lang.Iterable extends com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease> values) {
if (newLeasesBuilder_ == null) {
ensureNewLeasesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, newLeases_);
onChanged();
} else {
newLeasesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.NewLease new_leases = 3;
*/
public Builder clearNewLeases() {
if (newLeasesBuilder_ == null) {
newLeases_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
newLeasesBuilder_.clear();
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.NewLease new_leases = 3;
*/
public Builder removeNewLeases(int index) {
if (newLeasesBuilder_ == null) {
ensureNewLeasesIsMutable();
newLeases_.remove(index);
onChanged();
} else {
newLeasesBuilder_.remove(index);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.NewLease new_leases = 3;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease.Builder getNewLeasesBuilder(
int index) {
return getNewLeasesFieldBuilder().getBuilder(index);
}
/**
* repeated .waves.TransactionStateSnapshot.NewLease new_leases = 3;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLeaseOrBuilder getNewLeasesOrBuilder(
int index) {
if (newLeasesBuilder_ == null) {
return newLeases_.get(index); } else {
return newLeasesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .waves.TransactionStateSnapshot.NewLease new_leases = 3;
*/
public java.util.List extends com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLeaseOrBuilder>
getNewLeasesOrBuilderList() {
if (newLeasesBuilder_ != null) {
return newLeasesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(newLeases_);
}
}
/**
* repeated .waves.TransactionStateSnapshot.NewLease new_leases = 3;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease.Builder addNewLeasesBuilder() {
return getNewLeasesFieldBuilder().addBuilder(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease.getDefaultInstance());
}
/**
* repeated .waves.TransactionStateSnapshot.NewLease new_leases = 3;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease.Builder addNewLeasesBuilder(
int index) {
return getNewLeasesFieldBuilder().addBuilder(
index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease.getDefaultInstance());
}
/**
* repeated .waves.TransactionStateSnapshot.NewLease new_leases = 3;
*/
public java.util.List
getNewLeasesBuilderList() {
return getNewLeasesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease.Builder, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLeaseOrBuilder>
getNewLeasesFieldBuilder() {
if (newLeasesBuilder_ == null) {
newLeasesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLease.Builder, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewLeaseOrBuilder>(
newLeases_,
((bitField0_ & 0x00000004) != 0),
getParentForChildren(),
isClean());
newLeases_ = null;
}
return newLeasesBuilder_;
}
private java.util.List cancelledLeases_ =
java.util.Collections.emptyList();
private void ensureCancelledLeasesIsMutable() {
if (!((bitField0_ & 0x00000008) != 0)) {
cancelledLeases_ = new java.util.ArrayList(cancelledLeases_);
bitField0_ |= 0x00000008;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease.Builder, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLeaseOrBuilder> cancelledLeasesBuilder_;
/**
* repeated .waves.TransactionStateSnapshot.CancelledLease cancelled_leases = 4;
*/
public java.util.List getCancelledLeasesList() {
if (cancelledLeasesBuilder_ == null) {
return java.util.Collections.unmodifiableList(cancelledLeases_);
} else {
return cancelledLeasesBuilder_.getMessageList();
}
}
/**
* repeated .waves.TransactionStateSnapshot.CancelledLease cancelled_leases = 4;
*/
public int getCancelledLeasesCount() {
if (cancelledLeasesBuilder_ == null) {
return cancelledLeases_.size();
} else {
return cancelledLeasesBuilder_.getCount();
}
}
/**
* repeated .waves.TransactionStateSnapshot.CancelledLease cancelled_leases = 4;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease getCancelledLeases(int index) {
if (cancelledLeasesBuilder_ == null) {
return cancelledLeases_.get(index);
} else {
return cancelledLeasesBuilder_.getMessage(index);
}
}
/**
* repeated .waves.TransactionStateSnapshot.CancelledLease cancelled_leases = 4;
*/
public Builder setCancelledLeases(
int index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease value) {
if (cancelledLeasesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCancelledLeasesIsMutable();
cancelledLeases_.set(index, value);
onChanged();
} else {
cancelledLeasesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.CancelledLease cancelled_leases = 4;
*/
public Builder setCancelledLeases(
int index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease.Builder builderForValue) {
if (cancelledLeasesBuilder_ == null) {
ensureCancelledLeasesIsMutable();
cancelledLeases_.set(index, builderForValue.build());
onChanged();
} else {
cancelledLeasesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.CancelledLease cancelled_leases = 4;
*/
public Builder addCancelledLeases(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease value) {
if (cancelledLeasesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCancelledLeasesIsMutable();
cancelledLeases_.add(value);
onChanged();
} else {
cancelledLeasesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.CancelledLease cancelled_leases = 4;
*/
public Builder addCancelledLeases(
int index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease value) {
if (cancelledLeasesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCancelledLeasesIsMutable();
cancelledLeases_.add(index, value);
onChanged();
} else {
cancelledLeasesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.CancelledLease cancelled_leases = 4;
*/
public Builder addCancelledLeases(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease.Builder builderForValue) {
if (cancelledLeasesBuilder_ == null) {
ensureCancelledLeasesIsMutable();
cancelledLeases_.add(builderForValue.build());
onChanged();
} else {
cancelledLeasesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.CancelledLease cancelled_leases = 4;
*/
public Builder addCancelledLeases(
int index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease.Builder builderForValue) {
if (cancelledLeasesBuilder_ == null) {
ensureCancelledLeasesIsMutable();
cancelledLeases_.add(index, builderForValue.build());
onChanged();
} else {
cancelledLeasesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.CancelledLease cancelled_leases = 4;
*/
public Builder addAllCancelledLeases(
java.lang.Iterable extends com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease> values) {
if (cancelledLeasesBuilder_ == null) {
ensureCancelledLeasesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, cancelledLeases_);
onChanged();
} else {
cancelledLeasesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.CancelledLease cancelled_leases = 4;
*/
public Builder clearCancelledLeases() {
if (cancelledLeasesBuilder_ == null) {
cancelledLeases_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
} else {
cancelledLeasesBuilder_.clear();
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.CancelledLease cancelled_leases = 4;
*/
public Builder removeCancelledLeases(int index) {
if (cancelledLeasesBuilder_ == null) {
ensureCancelledLeasesIsMutable();
cancelledLeases_.remove(index);
onChanged();
} else {
cancelledLeasesBuilder_.remove(index);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.CancelledLease cancelled_leases = 4;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease.Builder getCancelledLeasesBuilder(
int index) {
return getCancelledLeasesFieldBuilder().getBuilder(index);
}
/**
* repeated .waves.TransactionStateSnapshot.CancelledLease cancelled_leases = 4;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLeaseOrBuilder getCancelledLeasesOrBuilder(
int index) {
if (cancelledLeasesBuilder_ == null) {
return cancelledLeases_.get(index); } else {
return cancelledLeasesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .waves.TransactionStateSnapshot.CancelledLease cancelled_leases = 4;
*/
public java.util.List extends com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLeaseOrBuilder>
getCancelledLeasesOrBuilderList() {
if (cancelledLeasesBuilder_ != null) {
return cancelledLeasesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(cancelledLeases_);
}
}
/**
* repeated .waves.TransactionStateSnapshot.CancelledLease cancelled_leases = 4;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease.Builder addCancelledLeasesBuilder() {
return getCancelledLeasesFieldBuilder().addBuilder(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease.getDefaultInstance());
}
/**
* repeated .waves.TransactionStateSnapshot.CancelledLease cancelled_leases = 4;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease.Builder addCancelledLeasesBuilder(
int index) {
return getCancelledLeasesFieldBuilder().addBuilder(
index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease.getDefaultInstance());
}
/**
* repeated .waves.TransactionStateSnapshot.CancelledLease cancelled_leases = 4;
*/
public java.util.List
getCancelledLeasesBuilderList() {
return getCancelledLeasesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease.Builder, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLeaseOrBuilder>
getCancelledLeasesFieldBuilder() {
if (cancelledLeasesBuilder_ == null) {
cancelledLeasesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLease.Builder, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.CancelledLeaseOrBuilder>(
cancelledLeases_,
((bitField0_ & 0x00000008) != 0),
getParentForChildren(),
isClean());
cancelledLeases_ = null;
}
return cancelledLeasesBuilder_;
}
private java.util.List assetStatics_ =
java.util.Collections.emptyList();
private void ensureAssetStaticsIsMutable() {
if (!((bitField0_ & 0x00000010) != 0)) {
assetStatics_ = new java.util.ArrayList(assetStatics_);
bitField0_ |= 0x00000010;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset.Builder, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAssetOrBuilder> assetStaticsBuilder_;
/**
* repeated .waves.TransactionStateSnapshot.NewAsset asset_statics = 5;
*/
public java.util.List getAssetStaticsList() {
if (assetStaticsBuilder_ == null) {
return java.util.Collections.unmodifiableList(assetStatics_);
} else {
return assetStaticsBuilder_.getMessageList();
}
}
/**
* repeated .waves.TransactionStateSnapshot.NewAsset asset_statics = 5;
*/
public int getAssetStaticsCount() {
if (assetStaticsBuilder_ == null) {
return assetStatics_.size();
} else {
return assetStaticsBuilder_.getCount();
}
}
/**
* repeated .waves.TransactionStateSnapshot.NewAsset asset_statics = 5;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset getAssetStatics(int index) {
if (assetStaticsBuilder_ == null) {
return assetStatics_.get(index);
} else {
return assetStaticsBuilder_.getMessage(index);
}
}
/**
* repeated .waves.TransactionStateSnapshot.NewAsset asset_statics = 5;
*/
public Builder setAssetStatics(
int index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset value) {
if (assetStaticsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAssetStaticsIsMutable();
assetStatics_.set(index, value);
onChanged();
} else {
assetStaticsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.NewAsset asset_statics = 5;
*/
public Builder setAssetStatics(
int index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset.Builder builderForValue) {
if (assetStaticsBuilder_ == null) {
ensureAssetStaticsIsMutable();
assetStatics_.set(index, builderForValue.build());
onChanged();
} else {
assetStaticsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.NewAsset asset_statics = 5;
*/
public Builder addAssetStatics(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset value) {
if (assetStaticsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAssetStaticsIsMutable();
assetStatics_.add(value);
onChanged();
} else {
assetStaticsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.NewAsset asset_statics = 5;
*/
public Builder addAssetStatics(
int index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset value) {
if (assetStaticsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAssetStaticsIsMutable();
assetStatics_.add(index, value);
onChanged();
} else {
assetStaticsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.NewAsset asset_statics = 5;
*/
public Builder addAssetStatics(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset.Builder builderForValue) {
if (assetStaticsBuilder_ == null) {
ensureAssetStaticsIsMutable();
assetStatics_.add(builderForValue.build());
onChanged();
} else {
assetStaticsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.NewAsset asset_statics = 5;
*/
public Builder addAssetStatics(
int index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset.Builder builderForValue) {
if (assetStaticsBuilder_ == null) {
ensureAssetStaticsIsMutable();
assetStatics_.add(index, builderForValue.build());
onChanged();
} else {
assetStaticsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.NewAsset asset_statics = 5;
*/
public Builder addAllAssetStatics(
java.lang.Iterable extends com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset> values) {
if (assetStaticsBuilder_ == null) {
ensureAssetStaticsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, assetStatics_);
onChanged();
} else {
assetStaticsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.NewAsset asset_statics = 5;
*/
public Builder clearAssetStatics() {
if (assetStaticsBuilder_ == null) {
assetStatics_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
} else {
assetStaticsBuilder_.clear();
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.NewAsset asset_statics = 5;
*/
public Builder removeAssetStatics(int index) {
if (assetStaticsBuilder_ == null) {
ensureAssetStaticsIsMutable();
assetStatics_.remove(index);
onChanged();
} else {
assetStaticsBuilder_.remove(index);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.NewAsset asset_statics = 5;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset.Builder getAssetStaticsBuilder(
int index) {
return getAssetStaticsFieldBuilder().getBuilder(index);
}
/**
* repeated .waves.TransactionStateSnapshot.NewAsset asset_statics = 5;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAssetOrBuilder getAssetStaticsOrBuilder(
int index) {
if (assetStaticsBuilder_ == null) {
return assetStatics_.get(index); } else {
return assetStaticsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .waves.TransactionStateSnapshot.NewAsset asset_statics = 5;
*/
public java.util.List extends com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAssetOrBuilder>
getAssetStaticsOrBuilderList() {
if (assetStaticsBuilder_ != null) {
return assetStaticsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(assetStatics_);
}
}
/**
* repeated .waves.TransactionStateSnapshot.NewAsset asset_statics = 5;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset.Builder addAssetStaticsBuilder() {
return getAssetStaticsFieldBuilder().addBuilder(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset.getDefaultInstance());
}
/**
* repeated .waves.TransactionStateSnapshot.NewAsset asset_statics = 5;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset.Builder addAssetStaticsBuilder(
int index) {
return getAssetStaticsFieldBuilder().addBuilder(
index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset.getDefaultInstance());
}
/**
* repeated .waves.TransactionStateSnapshot.NewAsset asset_statics = 5;
*/
public java.util.List
getAssetStaticsBuilderList() {
return getAssetStaticsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset.Builder, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAssetOrBuilder>
getAssetStaticsFieldBuilder() {
if (assetStaticsBuilder_ == null) {
assetStaticsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAsset.Builder, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.NewAssetOrBuilder>(
assetStatics_,
((bitField0_ & 0x00000010) != 0),
getParentForChildren(),
isClean());
assetStatics_ = null;
}
return assetStaticsBuilder_;
}
private java.util.List assetVolumes_ =
java.util.Collections.emptyList();
private void ensureAssetVolumesIsMutable() {
if (!((bitField0_ & 0x00000020) != 0)) {
assetVolumes_ = new java.util.ArrayList(assetVolumes_);
bitField0_ |= 0x00000020;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume.Builder, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolumeOrBuilder> assetVolumesBuilder_;
/**
* repeated .waves.TransactionStateSnapshot.AssetVolume asset_volumes = 6;
*/
public java.util.List getAssetVolumesList() {
if (assetVolumesBuilder_ == null) {
return java.util.Collections.unmodifiableList(assetVolumes_);
} else {
return assetVolumesBuilder_.getMessageList();
}
}
/**
* repeated .waves.TransactionStateSnapshot.AssetVolume asset_volumes = 6;
*/
public int getAssetVolumesCount() {
if (assetVolumesBuilder_ == null) {
return assetVolumes_.size();
} else {
return assetVolumesBuilder_.getCount();
}
}
/**
* repeated .waves.TransactionStateSnapshot.AssetVolume asset_volumes = 6;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume getAssetVolumes(int index) {
if (assetVolumesBuilder_ == null) {
return assetVolumes_.get(index);
} else {
return assetVolumesBuilder_.getMessage(index);
}
}
/**
* repeated .waves.TransactionStateSnapshot.AssetVolume asset_volumes = 6;
*/
public Builder setAssetVolumes(
int index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume value) {
if (assetVolumesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAssetVolumesIsMutable();
assetVolumes_.set(index, value);
onChanged();
} else {
assetVolumesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.AssetVolume asset_volumes = 6;
*/
public Builder setAssetVolumes(
int index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume.Builder builderForValue) {
if (assetVolumesBuilder_ == null) {
ensureAssetVolumesIsMutable();
assetVolumes_.set(index, builderForValue.build());
onChanged();
} else {
assetVolumesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.AssetVolume asset_volumes = 6;
*/
public Builder addAssetVolumes(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume value) {
if (assetVolumesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAssetVolumesIsMutable();
assetVolumes_.add(value);
onChanged();
} else {
assetVolumesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.AssetVolume asset_volumes = 6;
*/
public Builder addAssetVolumes(
int index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume value) {
if (assetVolumesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAssetVolumesIsMutable();
assetVolumes_.add(index, value);
onChanged();
} else {
assetVolumesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.AssetVolume asset_volumes = 6;
*/
public Builder addAssetVolumes(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume.Builder builderForValue) {
if (assetVolumesBuilder_ == null) {
ensureAssetVolumesIsMutable();
assetVolumes_.add(builderForValue.build());
onChanged();
} else {
assetVolumesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.AssetVolume asset_volumes = 6;
*/
public Builder addAssetVolumes(
int index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume.Builder builderForValue) {
if (assetVolumesBuilder_ == null) {
ensureAssetVolumesIsMutable();
assetVolumes_.add(index, builderForValue.build());
onChanged();
} else {
assetVolumesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.AssetVolume asset_volumes = 6;
*/
public Builder addAllAssetVolumes(
java.lang.Iterable extends com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume> values) {
if (assetVolumesBuilder_ == null) {
ensureAssetVolumesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, assetVolumes_);
onChanged();
} else {
assetVolumesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.AssetVolume asset_volumes = 6;
*/
public Builder clearAssetVolumes() {
if (assetVolumesBuilder_ == null) {
assetVolumes_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
} else {
assetVolumesBuilder_.clear();
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.AssetVolume asset_volumes = 6;
*/
public Builder removeAssetVolumes(int index) {
if (assetVolumesBuilder_ == null) {
ensureAssetVolumesIsMutable();
assetVolumes_.remove(index);
onChanged();
} else {
assetVolumesBuilder_.remove(index);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.AssetVolume asset_volumes = 6;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume.Builder getAssetVolumesBuilder(
int index) {
return getAssetVolumesFieldBuilder().getBuilder(index);
}
/**
* repeated .waves.TransactionStateSnapshot.AssetVolume asset_volumes = 6;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolumeOrBuilder getAssetVolumesOrBuilder(
int index) {
if (assetVolumesBuilder_ == null) {
return assetVolumes_.get(index); } else {
return assetVolumesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .waves.TransactionStateSnapshot.AssetVolume asset_volumes = 6;
*/
public java.util.List extends com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolumeOrBuilder>
getAssetVolumesOrBuilderList() {
if (assetVolumesBuilder_ != null) {
return assetVolumesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(assetVolumes_);
}
}
/**
* repeated .waves.TransactionStateSnapshot.AssetVolume asset_volumes = 6;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume.Builder addAssetVolumesBuilder() {
return getAssetVolumesFieldBuilder().addBuilder(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume.getDefaultInstance());
}
/**
* repeated .waves.TransactionStateSnapshot.AssetVolume asset_volumes = 6;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume.Builder addAssetVolumesBuilder(
int index) {
return getAssetVolumesFieldBuilder().addBuilder(
index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume.getDefaultInstance());
}
/**
* repeated .waves.TransactionStateSnapshot.AssetVolume asset_volumes = 6;
*/
public java.util.List
getAssetVolumesBuilderList() {
return getAssetVolumesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume.Builder, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolumeOrBuilder>
getAssetVolumesFieldBuilder() {
if (assetVolumesBuilder_ == null) {
assetVolumesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolume.Builder, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetVolumeOrBuilder>(
assetVolumes_,
((bitField0_ & 0x00000020) != 0),
getParentForChildren(),
isClean());
assetVolumes_ = null;
}
return assetVolumesBuilder_;
}
private java.util.List assetNamesAndDescriptions_ =
java.util.Collections.emptyList();
private void ensureAssetNamesAndDescriptionsIsMutable() {
if (!((bitField0_ & 0x00000040) != 0)) {
assetNamesAndDescriptions_ = new java.util.ArrayList(assetNamesAndDescriptions_);
bitField0_ |= 0x00000040;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription.Builder, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescriptionOrBuilder> assetNamesAndDescriptionsBuilder_;
/**
* repeated .waves.TransactionStateSnapshot.AssetNameAndDescription asset_names_and_descriptions = 7;
*/
public java.util.List getAssetNamesAndDescriptionsList() {
if (assetNamesAndDescriptionsBuilder_ == null) {
return java.util.Collections.unmodifiableList(assetNamesAndDescriptions_);
} else {
return assetNamesAndDescriptionsBuilder_.getMessageList();
}
}
/**
* repeated .waves.TransactionStateSnapshot.AssetNameAndDescription asset_names_and_descriptions = 7;
*/
public int getAssetNamesAndDescriptionsCount() {
if (assetNamesAndDescriptionsBuilder_ == null) {
return assetNamesAndDescriptions_.size();
} else {
return assetNamesAndDescriptionsBuilder_.getCount();
}
}
/**
* repeated .waves.TransactionStateSnapshot.AssetNameAndDescription asset_names_and_descriptions = 7;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription getAssetNamesAndDescriptions(int index) {
if (assetNamesAndDescriptionsBuilder_ == null) {
return assetNamesAndDescriptions_.get(index);
} else {
return assetNamesAndDescriptionsBuilder_.getMessage(index);
}
}
/**
* repeated .waves.TransactionStateSnapshot.AssetNameAndDescription asset_names_and_descriptions = 7;
*/
public Builder setAssetNamesAndDescriptions(
int index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription value) {
if (assetNamesAndDescriptionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAssetNamesAndDescriptionsIsMutable();
assetNamesAndDescriptions_.set(index, value);
onChanged();
} else {
assetNamesAndDescriptionsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.AssetNameAndDescription asset_names_and_descriptions = 7;
*/
public Builder setAssetNamesAndDescriptions(
int index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription.Builder builderForValue) {
if (assetNamesAndDescriptionsBuilder_ == null) {
ensureAssetNamesAndDescriptionsIsMutable();
assetNamesAndDescriptions_.set(index, builderForValue.build());
onChanged();
} else {
assetNamesAndDescriptionsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.AssetNameAndDescription asset_names_and_descriptions = 7;
*/
public Builder addAssetNamesAndDescriptions(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription value) {
if (assetNamesAndDescriptionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAssetNamesAndDescriptionsIsMutable();
assetNamesAndDescriptions_.add(value);
onChanged();
} else {
assetNamesAndDescriptionsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.AssetNameAndDescription asset_names_and_descriptions = 7;
*/
public Builder addAssetNamesAndDescriptions(
int index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription value) {
if (assetNamesAndDescriptionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAssetNamesAndDescriptionsIsMutable();
assetNamesAndDescriptions_.add(index, value);
onChanged();
} else {
assetNamesAndDescriptionsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.AssetNameAndDescription asset_names_and_descriptions = 7;
*/
public Builder addAssetNamesAndDescriptions(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription.Builder builderForValue) {
if (assetNamesAndDescriptionsBuilder_ == null) {
ensureAssetNamesAndDescriptionsIsMutable();
assetNamesAndDescriptions_.add(builderForValue.build());
onChanged();
} else {
assetNamesAndDescriptionsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.AssetNameAndDescription asset_names_and_descriptions = 7;
*/
public Builder addAssetNamesAndDescriptions(
int index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription.Builder builderForValue) {
if (assetNamesAndDescriptionsBuilder_ == null) {
ensureAssetNamesAndDescriptionsIsMutable();
assetNamesAndDescriptions_.add(index, builderForValue.build());
onChanged();
} else {
assetNamesAndDescriptionsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.AssetNameAndDescription asset_names_and_descriptions = 7;
*/
public Builder addAllAssetNamesAndDescriptions(
java.lang.Iterable extends com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription> values) {
if (assetNamesAndDescriptionsBuilder_ == null) {
ensureAssetNamesAndDescriptionsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, assetNamesAndDescriptions_);
onChanged();
} else {
assetNamesAndDescriptionsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.AssetNameAndDescription asset_names_and_descriptions = 7;
*/
public Builder clearAssetNamesAndDescriptions() {
if (assetNamesAndDescriptionsBuilder_ == null) {
assetNamesAndDescriptions_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
} else {
assetNamesAndDescriptionsBuilder_.clear();
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.AssetNameAndDescription asset_names_and_descriptions = 7;
*/
public Builder removeAssetNamesAndDescriptions(int index) {
if (assetNamesAndDescriptionsBuilder_ == null) {
ensureAssetNamesAndDescriptionsIsMutable();
assetNamesAndDescriptions_.remove(index);
onChanged();
} else {
assetNamesAndDescriptionsBuilder_.remove(index);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.AssetNameAndDescription asset_names_and_descriptions = 7;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription.Builder getAssetNamesAndDescriptionsBuilder(
int index) {
return getAssetNamesAndDescriptionsFieldBuilder().getBuilder(index);
}
/**
* repeated .waves.TransactionStateSnapshot.AssetNameAndDescription asset_names_and_descriptions = 7;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescriptionOrBuilder getAssetNamesAndDescriptionsOrBuilder(
int index) {
if (assetNamesAndDescriptionsBuilder_ == null) {
return assetNamesAndDescriptions_.get(index); } else {
return assetNamesAndDescriptionsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .waves.TransactionStateSnapshot.AssetNameAndDescription asset_names_and_descriptions = 7;
*/
public java.util.List extends com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescriptionOrBuilder>
getAssetNamesAndDescriptionsOrBuilderList() {
if (assetNamesAndDescriptionsBuilder_ != null) {
return assetNamesAndDescriptionsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(assetNamesAndDescriptions_);
}
}
/**
* repeated .waves.TransactionStateSnapshot.AssetNameAndDescription asset_names_and_descriptions = 7;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription.Builder addAssetNamesAndDescriptionsBuilder() {
return getAssetNamesAndDescriptionsFieldBuilder().addBuilder(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription.getDefaultInstance());
}
/**
* repeated .waves.TransactionStateSnapshot.AssetNameAndDescription asset_names_and_descriptions = 7;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription.Builder addAssetNamesAndDescriptionsBuilder(
int index) {
return getAssetNamesAndDescriptionsFieldBuilder().addBuilder(
index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription.getDefaultInstance());
}
/**
* repeated .waves.TransactionStateSnapshot.AssetNameAndDescription asset_names_and_descriptions = 7;
*/
public java.util.List
getAssetNamesAndDescriptionsBuilderList() {
return getAssetNamesAndDescriptionsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription.Builder, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescriptionOrBuilder>
getAssetNamesAndDescriptionsFieldBuilder() {
if (assetNamesAndDescriptionsBuilder_ == null) {
assetNamesAndDescriptionsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescription.Builder, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetNameAndDescriptionOrBuilder>(
assetNamesAndDescriptions_,
((bitField0_ & 0x00000040) != 0),
getParentForChildren(),
isClean());
assetNamesAndDescriptions_ = null;
}
return assetNamesAndDescriptionsBuilder_;
}
private com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript assetScripts_;
private com.google.protobuf.SingleFieldBuilderV3<
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript.Builder, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScriptOrBuilder> assetScriptsBuilder_;
/**
* .waves.TransactionStateSnapshot.AssetScript asset_scripts = 8;
* @return Whether the assetScripts field is set.
*/
public boolean hasAssetScripts() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
* .waves.TransactionStateSnapshot.AssetScript asset_scripts = 8;
* @return The assetScripts.
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript getAssetScripts() {
if (assetScriptsBuilder_ == null) {
return assetScripts_ == null ? com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript.getDefaultInstance() : assetScripts_;
} else {
return assetScriptsBuilder_.getMessage();
}
}
/**
* .waves.TransactionStateSnapshot.AssetScript asset_scripts = 8;
*/
public Builder setAssetScripts(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript value) {
if (assetScriptsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
assetScripts_ = value;
} else {
assetScriptsBuilder_.setMessage(value);
}
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
* .waves.TransactionStateSnapshot.AssetScript asset_scripts = 8;
*/
public Builder setAssetScripts(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript.Builder builderForValue) {
if (assetScriptsBuilder_ == null) {
assetScripts_ = builderForValue.build();
} else {
assetScriptsBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
* .waves.TransactionStateSnapshot.AssetScript asset_scripts = 8;
*/
public Builder mergeAssetScripts(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript value) {
if (assetScriptsBuilder_ == null) {
if (((bitField0_ & 0x00000080) != 0) &&
assetScripts_ != null &&
assetScripts_ != com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript.getDefaultInstance()) {
getAssetScriptsBuilder().mergeFrom(value);
} else {
assetScripts_ = value;
}
} else {
assetScriptsBuilder_.mergeFrom(value);
}
if (assetScripts_ != null) {
bitField0_ |= 0x00000080;
onChanged();
}
return this;
}
/**
* .waves.TransactionStateSnapshot.AssetScript asset_scripts = 8;
*/
public Builder clearAssetScripts() {
bitField0_ = (bitField0_ & ~0x00000080);
assetScripts_ = null;
if (assetScriptsBuilder_ != null) {
assetScriptsBuilder_.dispose();
assetScriptsBuilder_ = null;
}
onChanged();
return this;
}
/**
* .waves.TransactionStateSnapshot.AssetScript asset_scripts = 8;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript.Builder getAssetScriptsBuilder() {
bitField0_ |= 0x00000080;
onChanged();
return getAssetScriptsFieldBuilder().getBuilder();
}
/**
* .waves.TransactionStateSnapshot.AssetScript asset_scripts = 8;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScriptOrBuilder getAssetScriptsOrBuilder() {
if (assetScriptsBuilder_ != null) {
return assetScriptsBuilder_.getMessageOrBuilder();
} else {
return assetScripts_ == null ?
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript.getDefaultInstance() : assetScripts_;
}
}
/**
* .waves.TransactionStateSnapshot.AssetScript asset_scripts = 8;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript.Builder, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScriptOrBuilder>
getAssetScriptsFieldBuilder() {
if (assetScriptsBuilder_ == null) {
assetScriptsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScript.Builder, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AssetScriptOrBuilder>(
getAssetScripts(),
getParentForChildren(),
isClean());
assetScripts_ = null;
}
return assetScriptsBuilder_;
}
private com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias aliases_;
private com.google.protobuf.SingleFieldBuilderV3<
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias.Builder, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AliasOrBuilder> aliasesBuilder_;
/**
* .waves.TransactionStateSnapshot.Alias aliases = 9;
* @return Whether the aliases field is set.
*/
public boolean hasAliases() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
* .waves.TransactionStateSnapshot.Alias aliases = 9;
* @return The aliases.
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias getAliases() {
if (aliasesBuilder_ == null) {
return aliases_ == null ? com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias.getDefaultInstance() : aliases_;
} else {
return aliasesBuilder_.getMessage();
}
}
/**
* .waves.TransactionStateSnapshot.Alias aliases = 9;
*/
public Builder setAliases(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias value) {
if (aliasesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
aliases_ = value;
} else {
aliasesBuilder_.setMessage(value);
}
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
* .waves.TransactionStateSnapshot.Alias aliases = 9;
*/
public Builder setAliases(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias.Builder builderForValue) {
if (aliasesBuilder_ == null) {
aliases_ = builderForValue.build();
} else {
aliasesBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
* .waves.TransactionStateSnapshot.Alias aliases = 9;
*/
public Builder mergeAliases(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias value) {
if (aliasesBuilder_ == null) {
if (((bitField0_ & 0x00000100) != 0) &&
aliases_ != null &&
aliases_ != com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias.getDefaultInstance()) {
getAliasesBuilder().mergeFrom(value);
} else {
aliases_ = value;
}
} else {
aliasesBuilder_.mergeFrom(value);
}
if (aliases_ != null) {
bitField0_ |= 0x00000100;
onChanged();
}
return this;
}
/**
* .waves.TransactionStateSnapshot.Alias aliases = 9;
*/
public Builder clearAliases() {
bitField0_ = (bitField0_ & ~0x00000100);
aliases_ = null;
if (aliasesBuilder_ != null) {
aliasesBuilder_.dispose();
aliasesBuilder_ = null;
}
onChanged();
return this;
}
/**
* .waves.TransactionStateSnapshot.Alias aliases = 9;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias.Builder getAliasesBuilder() {
bitField0_ |= 0x00000100;
onChanged();
return getAliasesFieldBuilder().getBuilder();
}
/**
* .waves.TransactionStateSnapshot.Alias aliases = 9;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AliasOrBuilder getAliasesOrBuilder() {
if (aliasesBuilder_ != null) {
return aliasesBuilder_.getMessageOrBuilder();
} else {
return aliases_ == null ?
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias.getDefaultInstance() : aliases_;
}
}
/**
* .waves.TransactionStateSnapshot.Alias aliases = 9;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias.Builder, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AliasOrBuilder>
getAliasesFieldBuilder() {
if (aliasesBuilder_ == null) {
aliasesBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Alias.Builder, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AliasOrBuilder>(
getAliases(),
getParentForChildren(),
isClean());
aliases_ = null;
}
return aliasesBuilder_;
}
private java.util.List orderFills_ =
java.util.Collections.emptyList();
private void ensureOrderFillsIsMutable() {
if (!((bitField0_ & 0x00000200) != 0)) {
orderFills_ = new java.util.ArrayList(orderFills_);
bitField0_ |= 0x00000200;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill.Builder, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFillOrBuilder> orderFillsBuilder_;
/**
* repeated .waves.TransactionStateSnapshot.OrderFill order_fills = 10;
*/
public java.util.List getOrderFillsList() {
if (orderFillsBuilder_ == null) {
return java.util.Collections.unmodifiableList(orderFills_);
} else {
return orderFillsBuilder_.getMessageList();
}
}
/**
* repeated .waves.TransactionStateSnapshot.OrderFill order_fills = 10;
*/
public int getOrderFillsCount() {
if (orderFillsBuilder_ == null) {
return orderFills_.size();
} else {
return orderFillsBuilder_.getCount();
}
}
/**
* repeated .waves.TransactionStateSnapshot.OrderFill order_fills = 10;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill getOrderFills(int index) {
if (orderFillsBuilder_ == null) {
return orderFills_.get(index);
} else {
return orderFillsBuilder_.getMessage(index);
}
}
/**
* repeated .waves.TransactionStateSnapshot.OrderFill order_fills = 10;
*/
public Builder setOrderFills(
int index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill value) {
if (orderFillsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOrderFillsIsMutable();
orderFills_.set(index, value);
onChanged();
} else {
orderFillsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.OrderFill order_fills = 10;
*/
public Builder setOrderFills(
int index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill.Builder builderForValue) {
if (orderFillsBuilder_ == null) {
ensureOrderFillsIsMutable();
orderFills_.set(index, builderForValue.build());
onChanged();
} else {
orderFillsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.OrderFill order_fills = 10;
*/
public Builder addOrderFills(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill value) {
if (orderFillsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOrderFillsIsMutable();
orderFills_.add(value);
onChanged();
} else {
orderFillsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.OrderFill order_fills = 10;
*/
public Builder addOrderFills(
int index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill value) {
if (orderFillsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOrderFillsIsMutable();
orderFills_.add(index, value);
onChanged();
} else {
orderFillsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.OrderFill order_fills = 10;
*/
public Builder addOrderFills(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill.Builder builderForValue) {
if (orderFillsBuilder_ == null) {
ensureOrderFillsIsMutable();
orderFills_.add(builderForValue.build());
onChanged();
} else {
orderFillsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.OrderFill order_fills = 10;
*/
public Builder addOrderFills(
int index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill.Builder builderForValue) {
if (orderFillsBuilder_ == null) {
ensureOrderFillsIsMutable();
orderFills_.add(index, builderForValue.build());
onChanged();
} else {
orderFillsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.OrderFill order_fills = 10;
*/
public Builder addAllOrderFills(
java.lang.Iterable extends com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill> values) {
if (orderFillsBuilder_ == null) {
ensureOrderFillsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, orderFills_);
onChanged();
} else {
orderFillsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.OrderFill order_fills = 10;
*/
public Builder clearOrderFills() {
if (orderFillsBuilder_ == null) {
orderFills_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000200);
onChanged();
} else {
orderFillsBuilder_.clear();
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.OrderFill order_fills = 10;
*/
public Builder removeOrderFills(int index) {
if (orderFillsBuilder_ == null) {
ensureOrderFillsIsMutable();
orderFills_.remove(index);
onChanged();
} else {
orderFillsBuilder_.remove(index);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.OrderFill order_fills = 10;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill.Builder getOrderFillsBuilder(
int index) {
return getOrderFillsFieldBuilder().getBuilder(index);
}
/**
* repeated .waves.TransactionStateSnapshot.OrderFill order_fills = 10;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFillOrBuilder getOrderFillsOrBuilder(
int index) {
if (orderFillsBuilder_ == null) {
return orderFills_.get(index); } else {
return orderFillsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .waves.TransactionStateSnapshot.OrderFill order_fills = 10;
*/
public java.util.List extends com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFillOrBuilder>
getOrderFillsOrBuilderList() {
if (orderFillsBuilder_ != null) {
return orderFillsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(orderFills_);
}
}
/**
* repeated .waves.TransactionStateSnapshot.OrderFill order_fills = 10;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill.Builder addOrderFillsBuilder() {
return getOrderFillsFieldBuilder().addBuilder(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill.getDefaultInstance());
}
/**
* repeated .waves.TransactionStateSnapshot.OrderFill order_fills = 10;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill.Builder addOrderFillsBuilder(
int index) {
return getOrderFillsFieldBuilder().addBuilder(
index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill.getDefaultInstance());
}
/**
* repeated .waves.TransactionStateSnapshot.OrderFill order_fills = 10;
*/
public java.util.List
getOrderFillsBuilderList() {
return getOrderFillsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill.Builder, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFillOrBuilder>
getOrderFillsFieldBuilder() {
if (orderFillsBuilder_ == null) {
orderFillsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFill.Builder, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.OrderFillOrBuilder>(
orderFills_,
((bitField0_ & 0x00000200) != 0),
getParentForChildren(),
isClean());
orderFills_ = null;
}
return orderFillsBuilder_;
}
private com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript accountScripts_;
private com.google.protobuf.SingleFieldBuilderV3<
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript.Builder, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScriptOrBuilder> accountScriptsBuilder_;
/**
* .waves.TransactionStateSnapshot.AccountScript account_scripts = 11;
* @return Whether the accountScripts field is set.
*/
public boolean hasAccountScripts() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
* .waves.TransactionStateSnapshot.AccountScript account_scripts = 11;
* @return The accountScripts.
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript getAccountScripts() {
if (accountScriptsBuilder_ == null) {
return accountScripts_ == null ? com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript.getDefaultInstance() : accountScripts_;
} else {
return accountScriptsBuilder_.getMessage();
}
}
/**
* .waves.TransactionStateSnapshot.AccountScript account_scripts = 11;
*/
public Builder setAccountScripts(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript value) {
if (accountScriptsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
accountScripts_ = value;
} else {
accountScriptsBuilder_.setMessage(value);
}
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
* .waves.TransactionStateSnapshot.AccountScript account_scripts = 11;
*/
public Builder setAccountScripts(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript.Builder builderForValue) {
if (accountScriptsBuilder_ == null) {
accountScripts_ = builderForValue.build();
} else {
accountScriptsBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
* .waves.TransactionStateSnapshot.AccountScript account_scripts = 11;
*/
public Builder mergeAccountScripts(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript value) {
if (accountScriptsBuilder_ == null) {
if (((bitField0_ & 0x00000400) != 0) &&
accountScripts_ != null &&
accountScripts_ != com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript.getDefaultInstance()) {
getAccountScriptsBuilder().mergeFrom(value);
} else {
accountScripts_ = value;
}
} else {
accountScriptsBuilder_.mergeFrom(value);
}
if (accountScripts_ != null) {
bitField0_ |= 0x00000400;
onChanged();
}
return this;
}
/**
* .waves.TransactionStateSnapshot.AccountScript account_scripts = 11;
*/
public Builder clearAccountScripts() {
bitField0_ = (bitField0_ & ~0x00000400);
accountScripts_ = null;
if (accountScriptsBuilder_ != null) {
accountScriptsBuilder_.dispose();
accountScriptsBuilder_ = null;
}
onChanged();
return this;
}
/**
* .waves.TransactionStateSnapshot.AccountScript account_scripts = 11;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript.Builder getAccountScriptsBuilder() {
bitField0_ |= 0x00000400;
onChanged();
return getAccountScriptsFieldBuilder().getBuilder();
}
/**
* .waves.TransactionStateSnapshot.AccountScript account_scripts = 11;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScriptOrBuilder getAccountScriptsOrBuilder() {
if (accountScriptsBuilder_ != null) {
return accountScriptsBuilder_.getMessageOrBuilder();
} else {
return accountScripts_ == null ?
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript.getDefaultInstance() : accountScripts_;
}
}
/**
* .waves.TransactionStateSnapshot.AccountScript account_scripts = 11;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript.Builder, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScriptOrBuilder>
getAccountScriptsFieldBuilder() {
if (accountScriptsBuilder_ == null) {
accountScriptsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScript.Builder, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountScriptOrBuilder>(
getAccountScripts(),
getParentForChildren(),
isClean());
accountScripts_ = null;
}
return accountScriptsBuilder_;
}
private java.util.List accountData_ =
java.util.Collections.emptyList();
private void ensureAccountDataIsMutable() {
if (!((bitField0_ & 0x00000800) != 0)) {
accountData_ = new java.util.ArrayList(accountData_);
bitField0_ |= 0x00000800;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData.Builder, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountDataOrBuilder> accountDataBuilder_;
/**
* repeated .waves.TransactionStateSnapshot.AccountData account_data = 12;
*/
public java.util.List getAccountDataList() {
if (accountDataBuilder_ == null) {
return java.util.Collections.unmodifiableList(accountData_);
} else {
return accountDataBuilder_.getMessageList();
}
}
/**
* repeated .waves.TransactionStateSnapshot.AccountData account_data = 12;
*/
public int getAccountDataCount() {
if (accountDataBuilder_ == null) {
return accountData_.size();
} else {
return accountDataBuilder_.getCount();
}
}
/**
* repeated .waves.TransactionStateSnapshot.AccountData account_data = 12;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData getAccountData(int index) {
if (accountDataBuilder_ == null) {
return accountData_.get(index);
} else {
return accountDataBuilder_.getMessage(index);
}
}
/**
* repeated .waves.TransactionStateSnapshot.AccountData account_data = 12;
*/
public Builder setAccountData(
int index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData value) {
if (accountDataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAccountDataIsMutable();
accountData_.set(index, value);
onChanged();
} else {
accountDataBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.AccountData account_data = 12;
*/
public Builder setAccountData(
int index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData.Builder builderForValue) {
if (accountDataBuilder_ == null) {
ensureAccountDataIsMutable();
accountData_.set(index, builderForValue.build());
onChanged();
} else {
accountDataBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.AccountData account_data = 12;
*/
public Builder addAccountData(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData value) {
if (accountDataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAccountDataIsMutable();
accountData_.add(value);
onChanged();
} else {
accountDataBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.AccountData account_data = 12;
*/
public Builder addAccountData(
int index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData value) {
if (accountDataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAccountDataIsMutable();
accountData_.add(index, value);
onChanged();
} else {
accountDataBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.AccountData account_data = 12;
*/
public Builder addAccountData(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData.Builder builderForValue) {
if (accountDataBuilder_ == null) {
ensureAccountDataIsMutable();
accountData_.add(builderForValue.build());
onChanged();
} else {
accountDataBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.AccountData account_data = 12;
*/
public Builder addAccountData(
int index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData.Builder builderForValue) {
if (accountDataBuilder_ == null) {
ensureAccountDataIsMutable();
accountData_.add(index, builderForValue.build());
onChanged();
} else {
accountDataBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.AccountData account_data = 12;
*/
public Builder addAllAccountData(
java.lang.Iterable extends com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData> values) {
if (accountDataBuilder_ == null) {
ensureAccountDataIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, accountData_);
onChanged();
} else {
accountDataBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.AccountData account_data = 12;
*/
public Builder clearAccountData() {
if (accountDataBuilder_ == null) {
accountData_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000800);
onChanged();
} else {
accountDataBuilder_.clear();
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.AccountData account_data = 12;
*/
public Builder removeAccountData(int index) {
if (accountDataBuilder_ == null) {
ensureAccountDataIsMutable();
accountData_.remove(index);
onChanged();
} else {
accountDataBuilder_.remove(index);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.AccountData account_data = 12;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData.Builder getAccountDataBuilder(
int index) {
return getAccountDataFieldBuilder().getBuilder(index);
}
/**
* repeated .waves.TransactionStateSnapshot.AccountData account_data = 12;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountDataOrBuilder getAccountDataOrBuilder(
int index) {
if (accountDataBuilder_ == null) {
return accountData_.get(index); } else {
return accountDataBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .waves.TransactionStateSnapshot.AccountData account_data = 12;
*/
public java.util.List extends com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountDataOrBuilder>
getAccountDataOrBuilderList() {
if (accountDataBuilder_ != null) {
return accountDataBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(accountData_);
}
}
/**
* repeated .waves.TransactionStateSnapshot.AccountData account_data = 12;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData.Builder addAccountDataBuilder() {
return getAccountDataFieldBuilder().addBuilder(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData.getDefaultInstance());
}
/**
* repeated .waves.TransactionStateSnapshot.AccountData account_data = 12;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData.Builder addAccountDataBuilder(
int index) {
return getAccountDataFieldBuilder().addBuilder(
index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData.getDefaultInstance());
}
/**
* repeated .waves.TransactionStateSnapshot.AccountData account_data = 12;
*/
public java.util.List
getAccountDataBuilderList() {
return getAccountDataFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData.Builder, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountDataOrBuilder>
getAccountDataFieldBuilder() {
if (accountDataBuilder_ == null) {
accountDataBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountData.Builder, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.AccountDataOrBuilder>(
accountData_,
((bitField0_ & 0x00000800) != 0),
getParentForChildren(),
isClean());
accountData_ = null;
}
return accountDataBuilder_;
}
private java.util.List sponsorships_ =
java.util.Collections.emptyList();
private void ensureSponsorshipsIsMutable() {
if (!((bitField0_ & 0x00001000) != 0)) {
sponsorships_ = new java.util.ArrayList(sponsorships_);
bitField0_ |= 0x00001000;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship.Builder, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.SponsorshipOrBuilder> sponsorshipsBuilder_;
/**
* repeated .waves.TransactionStateSnapshot.Sponsorship sponsorships = 13;
*/
public java.util.List getSponsorshipsList() {
if (sponsorshipsBuilder_ == null) {
return java.util.Collections.unmodifiableList(sponsorships_);
} else {
return sponsorshipsBuilder_.getMessageList();
}
}
/**
* repeated .waves.TransactionStateSnapshot.Sponsorship sponsorships = 13;
*/
public int getSponsorshipsCount() {
if (sponsorshipsBuilder_ == null) {
return sponsorships_.size();
} else {
return sponsorshipsBuilder_.getCount();
}
}
/**
* repeated .waves.TransactionStateSnapshot.Sponsorship sponsorships = 13;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship getSponsorships(int index) {
if (sponsorshipsBuilder_ == null) {
return sponsorships_.get(index);
} else {
return sponsorshipsBuilder_.getMessage(index);
}
}
/**
* repeated .waves.TransactionStateSnapshot.Sponsorship sponsorships = 13;
*/
public Builder setSponsorships(
int index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship value) {
if (sponsorshipsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSponsorshipsIsMutable();
sponsorships_.set(index, value);
onChanged();
} else {
sponsorshipsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.Sponsorship sponsorships = 13;
*/
public Builder setSponsorships(
int index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship.Builder builderForValue) {
if (sponsorshipsBuilder_ == null) {
ensureSponsorshipsIsMutable();
sponsorships_.set(index, builderForValue.build());
onChanged();
} else {
sponsorshipsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.Sponsorship sponsorships = 13;
*/
public Builder addSponsorships(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship value) {
if (sponsorshipsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSponsorshipsIsMutable();
sponsorships_.add(value);
onChanged();
} else {
sponsorshipsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.Sponsorship sponsorships = 13;
*/
public Builder addSponsorships(
int index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship value) {
if (sponsorshipsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSponsorshipsIsMutable();
sponsorships_.add(index, value);
onChanged();
} else {
sponsorshipsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.Sponsorship sponsorships = 13;
*/
public Builder addSponsorships(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship.Builder builderForValue) {
if (sponsorshipsBuilder_ == null) {
ensureSponsorshipsIsMutable();
sponsorships_.add(builderForValue.build());
onChanged();
} else {
sponsorshipsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.Sponsorship sponsorships = 13;
*/
public Builder addSponsorships(
int index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship.Builder builderForValue) {
if (sponsorshipsBuilder_ == null) {
ensureSponsorshipsIsMutable();
sponsorships_.add(index, builderForValue.build());
onChanged();
} else {
sponsorshipsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.Sponsorship sponsorships = 13;
*/
public Builder addAllSponsorships(
java.lang.Iterable extends com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship> values) {
if (sponsorshipsBuilder_ == null) {
ensureSponsorshipsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, sponsorships_);
onChanged();
} else {
sponsorshipsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.Sponsorship sponsorships = 13;
*/
public Builder clearSponsorships() {
if (sponsorshipsBuilder_ == null) {
sponsorships_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00001000);
onChanged();
} else {
sponsorshipsBuilder_.clear();
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.Sponsorship sponsorships = 13;
*/
public Builder removeSponsorships(int index) {
if (sponsorshipsBuilder_ == null) {
ensureSponsorshipsIsMutable();
sponsorships_.remove(index);
onChanged();
} else {
sponsorshipsBuilder_.remove(index);
}
return this;
}
/**
* repeated .waves.TransactionStateSnapshot.Sponsorship sponsorships = 13;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship.Builder getSponsorshipsBuilder(
int index) {
return getSponsorshipsFieldBuilder().getBuilder(index);
}
/**
* repeated .waves.TransactionStateSnapshot.Sponsorship sponsorships = 13;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.SponsorshipOrBuilder getSponsorshipsOrBuilder(
int index) {
if (sponsorshipsBuilder_ == null) {
return sponsorships_.get(index); } else {
return sponsorshipsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .waves.TransactionStateSnapshot.Sponsorship sponsorships = 13;
*/
public java.util.List extends com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.SponsorshipOrBuilder>
getSponsorshipsOrBuilderList() {
if (sponsorshipsBuilder_ != null) {
return sponsorshipsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(sponsorships_);
}
}
/**
* repeated .waves.TransactionStateSnapshot.Sponsorship sponsorships = 13;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship.Builder addSponsorshipsBuilder() {
return getSponsorshipsFieldBuilder().addBuilder(
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship.getDefaultInstance());
}
/**
* repeated .waves.TransactionStateSnapshot.Sponsorship sponsorships = 13;
*/
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship.Builder addSponsorshipsBuilder(
int index) {
return getSponsorshipsFieldBuilder().addBuilder(
index, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship.getDefaultInstance());
}
/**
* repeated .waves.TransactionStateSnapshot.Sponsorship sponsorships = 13;
*/
public java.util.List
getSponsorshipsBuilderList() {
return getSponsorshipsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship.Builder, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.SponsorshipOrBuilder>
getSponsorshipsFieldBuilder() {
if (sponsorshipsBuilder_ == null) {
sponsorshipsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.Sponsorship.Builder, com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot.SponsorshipOrBuilder>(
sponsorships_,
((bitField0_ & 0x00001000) != 0),
getParentForChildren(),
isClean());
sponsorships_ = null;
}
return sponsorshipsBuilder_;
}
private int transactionStatus_ = 0;
/**
* .waves.TransactionStatus transaction_status = 14;
* @return The enum numeric value on the wire for transactionStatus.
*/
@java.lang.Override public int getTransactionStatusValue() {
return transactionStatus_;
}
/**
* .waves.TransactionStatus transaction_status = 14;
* @param value The enum numeric value on the wire for transactionStatus to set.
* @return This builder for chaining.
*/
public Builder setTransactionStatusValue(int value) {
transactionStatus_ = value;
bitField0_ |= 0x00002000;
onChanged();
return this;
}
/**
* .waves.TransactionStatus transaction_status = 14;
* @return The transactionStatus.
*/
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStatus getTransactionStatus() {
com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStatus result = com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStatus.forNumber(transactionStatus_);
return result == null ? com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStatus.UNRECOGNIZED : result;
}
/**
* .waves.TransactionStatus transaction_status = 14;
* @param value The transactionStatus to set.
* @return This builder for chaining.
*/
public Builder setTransactionStatus(com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStatus value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00002000;
transactionStatus_ = value.getNumber();
onChanged();
return this;
}
/**
* .waves.TransactionStatus transaction_status = 14;
* @return This builder for chaining.
*/
public Builder clearTransactionStatus() {
bitField0_ = (bitField0_ & ~0x00002000);
transactionStatus_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:waves.TransactionStateSnapshot)
}
// @@protoc_insertion_point(class_scope:waves.TransactionStateSnapshot)
private static final com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot();
}
public static com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public TransactionStateSnapshot parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.wavesplatform.protobuf.snapshot.TransactionStateSnapshotOuterClass.TransactionStateSnapshot getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_waves_TransactionStateSnapshot_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_waves_TransactionStateSnapshot_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_waves_TransactionStateSnapshot_Balance_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_waves_TransactionStateSnapshot_Balance_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_waves_TransactionStateSnapshot_LeaseBalance_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_waves_TransactionStateSnapshot_LeaseBalance_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_waves_TransactionStateSnapshot_NewLease_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_waves_TransactionStateSnapshot_NewLease_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_waves_TransactionStateSnapshot_CancelledLease_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_waves_TransactionStateSnapshot_CancelledLease_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_waves_TransactionStateSnapshot_NewAsset_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_waves_TransactionStateSnapshot_NewAsset_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_waves_TransactionStateSnapshot_AssetVolume_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_waves_TransactionStateSnapshot_AssetVolume_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_waves_TransactionStateSnapshot_AssetNameAndDescription_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_waves_TransactionStateSnapshot_AssetNameAndDescription_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_waves_TransactionStateSnapshot_AssetScript_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_waves_TransactionStateSnapshot_AssetScript_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_waves_TransactionStateSnapshot_Alias_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_waves_TransactionStateSnapshot_Alias_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_waves_TransactionStateSnapshot_OrderFill_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_waves_TransactionStateSnapshot_OrderFill_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_waves_TransactionStateSnapshot_AccountScript_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_waves_TransactionStateSnapshot_AccountScript_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_waves_TransactionStateSnapshot_AccountData_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_waves_TransactionStateSnapshot_AccountData_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_waves_TransactionStateSnapshot_Sponsorship_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_waves_TransactionStateSnapshot_Sponsorship_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n&waves/transaction_state_snapshot.proto" +
"\022\005waves\032\022waves/amount.proto\032\027waves/trans" +
"action.proto\"\225\016\n\030TransactionStateSnapsho" +
"t\0229\n\010balances\030\001 \003(\0132\'.waves.TransactionS" +
"tateSnapshot.Balance\022D\n\016lease_balances\030\002" +
" \003(\0132,.waves.TransactionStateSnapshot.Le" +
"aseBalance\022<\n\nnew_leases\030\003 \003(\0132(.waves.T" +
"ransactionStateSnapshot.NewLease\022H\n\020canc" +
"elled_leases\030\004 \003(\0132..waves.TransactionSt" +
"ateSnapshot.CancelledLease\022?\n\rasset_stat" +
"ics\030\005 \003(\0132(.waves.TransactionStateSnapsh" +
"ot.NewAsset\022B\n\rasset_volumes\030\006 \003(\0132+.wav" +
"es.TransactionStateSnapshot.AssetVolume\022" +
"]\n\034asset_names_and_descriptions\030\007 \003(\01327." +
"waves.TransactionStateSnapshot.AssetName" +
"AndDescription\022B\n\rasset_scripts\030\010 \001(\0132+." +
"waves.TransactionStateSnapshot.AssetScri" +
"pt\0226\n\007aliases\030\t \001(\0132%.waves.TransactionS" +
"tateSnapshot.Alias\022>\n\013order_fills\030\n \003(\0132" +
").waves.TransactionStateSnapshot.OrderFi" +
"ll\022F\n\017account_scripts\030\013 \001(\0132-.waves.Tran" +
"sactionStateSnapshot.AccountScript\022A\n\014ac" +
"count_data\030\014 \003(\0132+.waves.TransactionStat" +
"eSnapshot.AccountData\022A\n\014sponsorships\030\r " +
"\003(\0132+.waves.TransactionStateSnapshot.Spo" +
"nsorship\0224\n\022transaction_status\030\016 \001(\0162\030.w" +
"aves.TransactionStatus\0329\n\007Balance\022\017\n\007add" +
"ress\030\001 \001(\014\022\035\n\006amount\030\002 \001(\0132\r.waves.Amoun" +
"t\0328\n\014LeaseBalance\022\017\n\007address\030\001 \001(\014\022\n\n\002in" +
"\030\002 \001(\003\022\013\n\003out\030\003 \001(\003\032b\n\010NewLease\022\020\n\010lease" +
"_id\030\001 \001(\014\022\031\n\021sender_public_key\030\002 \001(\014\022\031\n\021" +
"recipient_address\030\003 \001(\014\022\016\n\006amount\030\004 \001(\003\032" +
"\"\n\016CancelledLease\022\020\n\010lease_id\030\001 \001(\014\032V\n\010N" +
"ewAsset\022\020\n\010asset_id\030\001 \001(\014\022\031\n\021issuer_publ" +
"ic_key\030\002 \001(\014\022\020\n\010decimals\030\003 \001(\005\022\013\n\003nft\030\004 " +
"\001(\010\032C\n\013AssetVolume\022\020\n\010asset_id\030\001 \001(\014\022\022\n\n" +
"reissuable\030\002 \001(\010\022\016\n\006volume\030\003 \001(\014\032N\n\027Asse" +
"tNameAndDescription\022\020\n\010asset_id\030\001 \001(\014\022\014\n" +
"\004name\030\002 \001(\t\022\023\n\013description\030\003 \001(\t\032/\n\013Asse" +
"tScript\022\020\n\010asset_id\030\001 \001(\014\022\016\n\006script\030\002 \001(" +
"\014\032\'\n\005Alias\022\017\n\007address\030\001 \001(\014\022\r\n\005alias\030\002 \001" +
"(\t\032:\n\tOrderFill\022\020\n\010order_id\030\001 \001(\014\022\016\n\006vol" +
"ume\030\002 \001(\003\022\013\n\003fee\030\003 \001(\003\032W\n\rAccountScript\022" +
"\031\n\021sender_public_key\030\001 \001(\014\022\016\n\006script\030\002 \001" +
"(\014\022\033\n\023verifier_complexity\030\003 \001(\003\032A\n\013Accou" +
"ntData\022\017\n\007address\030\001 \001(\014\022!\n\007entries\030\002 \003(\013" +
"2\020.waves.DataEntry\0320\n\013Sponsorship\022\020\n\010ass" +
"et_id\030\001 \001(\014\022\017\n\007min_fee\030\002 \001(\003*:\n\021Transact" +
"ionStatus\022\r\n\tSUCCEEDED\020\000\022\n\n\006FAILED\020\001\022\n\n\006" +
"ELIDED\020\002Bh\n#com.wavesplatform.protobuf.s" +
"napshotZ9github.com/wavesplatform/gowave" +
"s/pkg/grpc/generated/waves\252\002\005Wavesb\006prot" +
"o3"
};
descriptor = com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
com.wavesplatform.protobuf.AmountOuterClass.getDescriptor(),
com.wavesplatform.protobuf.transaction.TransactionOuterClass.getDescriptor(),
});
internal_static_waves_TransactionStateSnapshot_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_waves_TransactionStateSnapshot_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_waves_TransactionStateSnapshot_descriptor,
new java.lang.String[] { "Balances", "LeaseBalances", "NewLeases", "CancelledLeases", "AssetStatics", "AssetVolumes", "AssetNamesAndDescriptions", "AssetScripts", "Aliases", "OrderFills", "AccountScripts", "AccountData", "Sponsorships", "TransactionStatus", });
internal_static_waves_TransactionStateSnapshot_Balance_descriptor =
internal_static_waves_TransactionStateSnapshot_descriptor.getNestedTypes().get(0);
internal_static_waves_TransactionStateSnapshot_Balance_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_waves_TransactionStateSnapshot_Balance_descriptor,
new java.lang.String[] { "Address", "Amount", });
internal_static_waves_TransactionStateSnapshot_LeaseBalance_descriptor =
internal_static_waves_TransactionStateSnapshot_descriptor.getNestedTypes().get(1);
internal_static_waves_TransactionStateSnapshot_LeaseBalance_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_waves_TransactionStateSnapshot_LeaseBalance_descriptor,
new java.lang.String[] { "Address", "In", "Out", });
internal_static_waves_TransactionStateSnapshot_NewLease_descriptor =
internal_static_waves_TransactionStateSnapshot_descriptor.getNestedTypes().get(2);
internal_static_waves_TransactionStateSnapshot_NewLease_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_waves_TransactionStateSnapshot_NewLease_descriptor,
new java.lang.String[] { "LeaseId", "SenderPublicKey", "RecipientAddress", "Amount", });
internal_static_waves_TransactionStateSnapshot_CancelledLease_descriptor =
internal_static_waves_TransactionStateSnapshot_descriptor.getNestedTypes().get(3);
internal_static_waves_TransactionStateSnapshot_CancelledLease_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_waves_TransactionStateSnapshot_CancelledLease_descriptor,
new java.lang.String[] { "LeaseId", });
internal_static_waves_TransactionStateSnapshot_NewAsset_descriptor =
internal_static_waves_TransactionStateSnapshot_descriptor.getNestedTypes().get(4);
internal_static_waves_TransactionStateSnapshot_NewAsset_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_waves_TransactionStateSnapshot_NewAsset_descriptor,
new java.lang.String[] { "AssetId", "IssuerPublicKey", "Decimals", "Nft", });
internal_static_waves_TransactionStateSnapshot_AssetVolume_descriptor =
internal_static_waves_TransactionStateSnapshot_descriptor.getNestedTypes().get(5);
internal_static_waves_TransactionStateSnapshot_AssetVolume_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_waves_TransactionStateSnapshot_AssetVolume_descriptor,
new java.lang.String[] { "AssetId", "Reissuable", "Volume", });
internal_static_waves_TransactionStateSnapshot_AssetNameAndDescription_descriptor =
internal_static_waves_TransactionStateSnapshot_descriptor.getNestedTypes().get(6);
internal_static_waves_TransactionStateSnapshot_AssetNameAndDescription_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_waves_TransactionStateSnapshot_AssetNameAndDescription_descriptor,
new java.lang.String[] { "AssetId", "Name", "Description", });
internal_static_waves_TransactionStateSnapshot_AssetScript_descriptor =
internal_static_waves_TransactionStateSnapshot_descriptor.getNestedTypes().get(7);
internal_static_waves_TransactionStateSnapshot_AssetScript_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_waves_TransactionStateSnapshot_AssetScript_descriptor,
new java.lang.String[] { "AssetId", "Script", });
internal_static_waves_TransactionStateSnapshot_Alias_descriptor =
internal_static_waves_TransactionStateSnapshot_descriptor.getNestedTypes().get(8);
internal_static_waves_TransactionStateSnapshot_Alias_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_waves_TransactionStateSnapshot_Alias_descriptor,
new java.lang.String[] { "Address", "Alias", });
internal_static_waves_TransactionStateSnapshot_OrderFill_descriptor =
internal_static_waves_TransactionStateSnapshot_descriptor.getNestedTypes().get(9);
internal_static_waves_TransactionStateSnapshot_OrderFill_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_waves_TransactionStateSnapshot_OrderFill_descriptor,
new java.lang.String[] { "OrderId", "Volume", "Fee", });
internal_static_waves_TransactionStateSnapshot_AccountScript_descriptor =
internal_static_waves_TransactionStateSnapshot_descriptor.getNestedTypes().get(10);
internal_static_waves_TransactionStateSnapshot_AccountScript_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_waves_TransactionStateSnapshot_AccountScript_descriptor,
new java.lang.String[] { "SenderPublicKey", "Script", "VerifierComplexity", });
internal_static_waves_TransactionStateSnapshot_AccountData_descriptor =
internal_static_waves_TransactionStateSnapshot_descriptor.getNestedTypes().get(11);
internal_static_waves_TransactionStateSnapshot_AccountData_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_waves_TransactionStateSnapshot_AccountData_descriptor,
new java.lang.String[] { "Address", "Entries", });
internal_static_waves_TransactionStateSnapshot_Sponsorship_descriptor =
internal_static_waves_TransactionStateSnapshot_descriptor.getNestedTypes().get(12);
internal_static_waves_TransactionStateSnapshot_Sponsorship_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_waves_TransactionStateSnapshot_Sponsorship_descriptor,
new java.lang.String[] { "AssetId", "MinFee", });
com.wavesplatform.protobuf.AmountOuterClass.getDescriptor();
com.wavesplatform.protobuf.transaction.TransactionOuterClass.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy