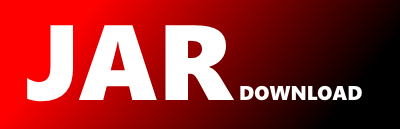
com.wci.umls.server.helpers.FieldedStringTokenizer Maven / Gradle / Ivy
/*
* Copyright 2015 West Coast Informatics, LLC
*/
package com.wci.umls.server.helpers;
import java.util.ArrayList;
import java.util.Enumeration;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
/**
* Breaks character deliminted strings into constituent fields. Unlike the
* {@link java.util.StringTokenizer} it recognizes empty tokens. For example,
* using a pipe character '|' as a delimiter, it would tokenize the string
* "a||b" into three tokens "a", "", "b". Following is an example of how to use
* this class.
*
*
* FieldedStringTokenizer tokenizer = new FieldedStringTokenizer("a|b||c", "|");
* int i = 1;
* while (tokenizer.hasMoreTokens()) {
* Logger.getLogger(getClass()).info(
* "Token " + i + " is: " + tokenizer.nextToken());
* }
*
*
* This class can also be used in a static way to split a line on a particular
* character delmiter and have a String[]
passed back. For example,
*
*
* String[] tokens = FieldedStringTokenizer.split("a|b|c|d", "|");
*
*
* This code will produce the string array {"a","b","c","d"}
.
*
* @author Brian Carlsen ([email protected])
*/
public class FieldedStringTokenizer implements Enumeration
© 2015 - 2025 Weber Informatics LLC | Privacy Policy