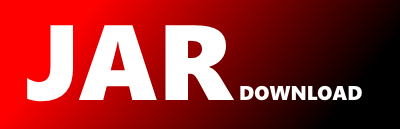
com.webcohesion.ofx4j.generated.CreditCardAccountInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ofx4j Show documentation
Show all versions of ofx4j Show documentation
OFX4J is a Java implementation of Open Financial Exchange, which defines web service
APIs for interfacing with financial institutions.
The newest version!
//
// This file was generated by the Eclipse Implementation of JAXB, v3.0.0
// See https://eclipse-ee4j.github.io/jaxb-ri
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2024.09.09 at 02:21:09 PM MDT
//
package com.webcohesion.ofx4j.generated;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
/**
*
* The OFX element "CCACCTINFO" is of type "CreditCardAccountInfo"
*
*
* Java class for CreditCardAccountInfo complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="CreditCardAccountInfo">
* <complexContent>
* <extension base="{http://ofx.net/types/2003/04}AbstractAccountInfo">
* <sequence>
* <element name="CCACCTFROM" type="{http://ofx.net/types/2003/04}CreditCardAccount"/>
* <element name="OTHERACCTINFO" type="{http://ofx.net/types/2003/04}OtherAccountInfo" minOccurs="0"/>
* <element name="PARENTACCTID" type="{http://ofx.net/types/2003/04}AccountIdType" minOccurs="0"/>
* <element name="SUPTXDL" type="{http://ofx.net/types/2003/04}BooleanType"/>
* <element name="XFERSRC" type="{http://ofx.net/types/2003/04}BooleanType"/>
* <element name="XFERDEST" type="{http://ofx.net/types/2003/04}BooleanType"/>
* <element name="ACCTCLASSIFICATION" type="{http://ofx.net/types/2003/04}AccountClassificationEnum" minOccurs="0"/>
* <element name="SVCSTATUS" type="{http://ofx.net/types/2003/04}ServiceStatusEnum"/>
* </sequence>
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "CreditCardAccountInfo", propOrder = {
"ccacctfrom",
"otheracctinfo",
"parentacctid",
"suptxdl",
"xfersrc",
"xferdest",
"acctclassification",
"svcstatus"
})
public class CreditCardAccountInfo
extends AbstractAccountInfo
{
@XmlElement(name = "CCACCTFROM", required = true)
protected CreditCardAccount ccacctfrom;
@XmlElement(name = "OTHERACCTINFO")
protected OtherAccountInfo otheracctinfo;
@XmlElement(name = "PARENTACCTID")
protected String parentacctid;
@XmlElement(name = "SUPTXDL", required = true)
@XmlSchemaType(name = "string")
protected BooleanType suptxdl;
@XmlElement(name = "XFERSRC", required = true)
@XmlSchemaType(name = "string")
protected BooleanType xfersrc;
@XmlElement(name = "XFERDEST", required = true)
@XmlSchemaType(name = "string")
protected BooleanType xferdest;
@XmlElement(name = "ACCTCLASSIFICATION")
@XmlSchemaType(name = "string")
protected AccountClassificationEnum acctclassification;
@XmlElement(name = "SVCSTATUS", required = true)
@XmlSchemaType(name = "string")
protected ServiceStatusEnum svcstatus;
/**
* Gets the value of the ccacctfrom property.
*
* @return
* possible object is
* {@link CreditCardAccount }
*
*/
public CreditCardAccount getCCACCTFROM() {
return ccacctfrom;
}
/**
* Sets the value of the ccacctfrom property.
*
* @param value
* allowed object is
* {@link CreditCardAccount }
*
*/
public void setCCACCTFROM(CreditCardAccount value) {
this.ccacctfrom = value;
}
/**
* Gets the value of the otheracctinfo property.
*
* @return
* possible object is
* {@link OtherAccountInfo }
*
*/
public OtherAccountInfo getOTHERACCTINFO() {
return otheracctinfo;
}
/**
* Sets the value of the otheracctinfo property.
*
* @param value
* allowed object is
* {@link OtherAccountInfo }
*
*/
public void setOTHERACCTINFO(OtherAccountInfo value) {
this.otheracctinfo = value;
}
/**
* Gets the value of the parentacctid property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPARENTACCTID() {
return parentacctid;
}
/**
* Sets the value of the parentacctid property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPARENTACCTID(String value) {
this.parentacctid = value;
}
/**
* Gets the value of the suptxdl property.
*
* @return
* possible object is
* {@link BooleanType }
*
*/
public BooleanType getSUPTXDL() {
return suptxdl;
}
/**
* Sets the value of the suptxdl property.
*
* @param value
* allowed object is
* {@link BooleanType }
*
*/
public void setSUPTXDL(BooleanType value) {
this.suptxdl = value;
}
/**
* Gets the value of the xfersrc property.
*
* @return
* possible object is
* {@link BooleanType }
*
*/
public BooleanType getXFERSRC() {
return xfersrc;
}
/**
* Sets the value of the xfersrc property.
*
* @param value
* allowed object is
* {@link BooleanType }
*
*/
public void setXFERSRC(BooleanType value) {
this.xfersrc = value;
}
/**
* Gets the value of the xferdest property.
*
* @return
* possible object is
* {@link BooleanType }
*
*/
public BooleanType getXFERDEST() {
return xferdest;
}
/**
* Sets the value of the xferdest property.
*
* @param value
* allowed object is
* {@link BooleanType }
*
*/
public void setXFERDEST(BooleanType value) {
this.xferdest = value;
}
/**
* Gets the value of the acctclassification property.
*
* @return
* possible object is
* {@link AccountClassificationEnum }
*
*/
public AccountClassificationEnum getACCTCLASSIFICATION() {
return acctclassification;
}
/**
* Sets the value of the acctclassification property.
*
* @param value
* allowed object is
* {@link AccountClassificationEnum }
*
*/
public void setACCTCLASSIFICATION(AccountClassificationEnum value) {
this.acctclassification = value;
}
/**
* Gets the value of the svcstatus property.
*
* @return
* possible object is
* {@link ServiceStatusEnum }
*
*/
public ServiceStatusEnum getSVCSTATUS() {
return svcstatus;
}
/**
* Sets the value of the svcstatus property.
*
* @param value
* allowed object is
* {@link ServiceStatusEnum }
*
*/
public void setSVCSTATUS(ServiceStatusEnum value) {
this.svcstatus = value;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy