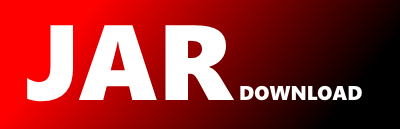
com.webcohesion.ofx4j.generated.PendingTransaction Maven / Gradle / Ivy
Show all versions of ofx4j Show documentation
//
// This file was generated by the Eclipse Implementation of JAXB, v3.0.0
// See https://eclipse-ee4j.github.io/jaxb-ri
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2024.09.09 at 02:21:09 PM MDT
//
package com.webcohesion.ofx4j.generated;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
/**
*
* The OFX element "STMTTRNP" is of type "PendingTransaction"
*
*
* Java class for PendingTransaction complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="PendingTransaction">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="TRNTYPE" type="{http://ofx.net/types/2003/04}TransactionEnum"/>
* <element name="DTTRAN" type="{http://ofx.net/types/2003/04}DateTimeType"/>
* <element name="DTEXPIRE" type="{http://ofx.net/types/2003/04}DateTimeType" minOccurs="0"/>
* <element name="TRNAMT" type="{http://ofx.net/types/2003/04}AmountType"/>
* <element name="REFNUM" type="{http://ofx.net/types/2003/04}ReferenceNumberType" minOccurs="0"/>
* <element name="NAME" type="{http://ofx.net/types/2003/04}GenericNameType"/>
* <element name="EXTDNAME" type="{http://ofx.net/types/2003/04}ExtendedNameType" minOccurs="0"/>
* <element name="MEMO" type="{http://ofx.net/types/2003/04}MessageType" minOccurs="0"/>
* <element name="IMAGEDATA" type="{http://ofx.net/types/2003/04}ImageData" maxOccurs="2" minOccurs="0"/>
* <choice minOccurs="0">
* <element name="CURRENCY" type="{http://ofx.net/types/2003/04}Currency"/>
* <element name="ORIGCURRENCY" type="{http://ofx.net/types/2003/04}Currency"/>
* </choice>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "PendingTransaction", propOrder = {
"trntype",
"dttran",
"dtexpire",
"trnamt",
"refnum",
"name",
"extdname",
"memo",
"imagedata",
"currency",
"origcurrency"
})
public class PendingTransaction {
@XmlElement(name = "TRNTYPE", required = true)
@XmlSchemaType(name = "string")
protected TransactionEnum trntype;
@XmlElement(name = "DTTRAN", required = true)
protected String dttran;
@XmlElement(name = "DTEXPIRE")
protected String dtexpire;
@XmlElement(name = "TRNAMT", required = true)
protected String trnamt;
@XmlElement(name = "REFNUM")
protected String refnum;
@XmlElement(name = "NAME", required = true)
protected String name;
@XmlElement(name = "EXTDNAME")
protected String extdname;
@XmlElement(name = "MEMO")
protected String memo;
@XmlElement(name = "IMAGEDATA")
protected List imagedata;
@XmlElement(name = "CURRENCY")
protected Currency currency;
@XmlElement(name = "ORIGCURRENCY")
protected Currency origcurrency;
/**
* Gets the value of the trntype property.
*
* @return
* possible object is
* {@link TransactionEnum }
*
*/
public TransactionEnum getTRNTYPE() {
return trntype;
}
/**
* Sets the value of the trntype property.
*
* @param value
* allowed object is
* {@link TransactionEnum }
*
*/
public void setTRNTYPE(TransactionEnum value) {
this.trntype = value;
}
/**
* Gets the value of the dttran property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDTTRAN() {
return dttran;
}
/**
* Sets the value of the dttran property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDTTRAN(String value) {
this.dttran = value;
}
/**
* Gets the value of the dtexpire property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDTEXPIRE() {
return dtexpire;
}
/**
* Sets the value of the dtexpire property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDTEXPIRE(String value) {
this.dtexpire = value;
}
/**
* Gets the value of the trnamt property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTRNAMT() {
return trnamt;
}
/**
* Sets the value of the trnamt property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTRNAMT(String value) {
this.trnamt = value;
}
/**
* Gets the value of the refnum property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getREFNUM() {
return refnum;
}
/**
* Sets the value of the refnum property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setREFNUM(String value) {
this.refnum = value;
}
/**
* Gets the value of the name property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getNAME() {
return name;
}
/**
* Sets the value of the name property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setNAME(String value) {
this.name = value;
}
/**
* Gets the value of the extdname property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getEXTDNAME() {
return extdname;
}
/**
* Sets the value of the extdname property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setEXTDNAME(String value) {
this.extdname = value;
}
/**
* Gets the value of the memo property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMEMO() {
return memo;
}
/**
* Sets the value of the memo property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMEMO(String value) {
this.memo = value;
}
/**
* Gets the value of the imagedata property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the imagedata property.
*
*
* For example, to add a new item, do as follows:
*
* getIMAGEDATA().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ImageData }
*
*
*/
public List getIMAGEDATA() {
if (imagedata == null) {
imagedata = new ArrayList();
}
return this.imagedata;
}
/**
* Gets the value of the currency property.
*
* @return
* possible object is
* {@link Currency }
*
*/
public Currency getCURRENCY() {
return currency;
}
/**
* Sets the value of the currency property.
*
* @param value
* allowed object is
* {@link Currency }
*
*/
public void setCURRENCY(Currency value) {
this.currency = value;
}
/**
* Gets the value of the origcurrency property.
*
* @return
* possible object is
* {@link Currency }
*
*/
public Currency getORIGCURRENCY() {
return origcurrency;
}
/**
* Sets the value of the origcurrency property.
*
* @param value
* allowed object is
* {@link Currency }
*
*/
public void setORIGCURRENCY(Currency value) {
this.origcurrency = value;
}
}