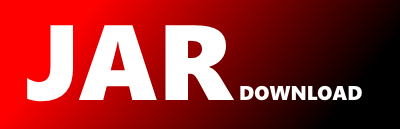
com.webcohesion.ofx4j.generated.ProfileResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ofx4j Show documentation
Show all versions of ofx4j Show documentation
OFX4J is a Java implementation of Open Financial Exchange, which defines web service
APIs for interfacing with financial institutions.
The newest version!
//
// This file was generated by the Eclipse Implementation of JAXB, v3.0.0
// See https://eclipse-ee4j.github.io/jaxb-ri
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2024.09.09 at 02:21:09 PM MDT
//
package com.webcohesion.ofx4j.generated;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
/**
*
* The OFX element "PROFRS" is of type "ProfileResponse"
*
*
* Java class for ProfileResponse complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="ProfileResponse">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="MSGSETLIST" type="{http://ofx.net/types/2003/04}MessageSetList"/>
* <element name="SIGNONINFOLIST" type="{http://ofx.net/types/2003/04}SignonInfoList"/>
* <element name="DTPROFUP" type="{http://ofx.net/types/2003/04}DateTimeType"/>
* <element name="FINAME" type="{http://ofx.net/types/2003/04}GenericNameType"/>
* <sequence>
* <element name="ADDR1" type="{http://ofx.net/types/2003/04}AddressType"/>
* <sequence minOccurs="0">
* <element name="ADDR2" type="{http://ofx.net/types/2003/04}AddressType"/>
* <element name="ADDR3" type="{http://ofx.net/types/2003/04}AddressType" minOccurs="0"/>
* </sequence>
* </sequence>
* <element name="CITY" type="{http://ofx.net/types/2003/04}AddressType"/>
* <element name="STATE" type="{http://ofx.net/types/2003/04}StateType"/>
* <element name="POSTALCODE" type="{http://ofx.net/types/2003/04}ZipType"/>
* <element name="COUNTRY" type="{http://ofx.net/types/2003/04}CountryType"/>
* <element name="CSPHONE" type="{http://ofx.net/types/2003/04}PhoneType" minOccurs="0"/>
* <element name="TSPHONE" type="{http://ofx.net/types/2003/04}PhoneType" minOccurs="0"/>
* <element name="FAXPHONE" type="{http://ofx.net/types/2003/04}PhoneType" minOccurs="0"/>
* <element name="URL" type="{http://ofx.net/types/2003/04}UrlType" minOccurs="0"/>
* <element name="EMAIL" type="{http://ofx.net/types/2003/04}ShortMessageType" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "ProfileResponse", propOrder = {
"msgsetlist",
"signoninfolist",
"dtprofup",
"finame",
"addr1",
"addr2",
"addr3",
"city",
"state",
"postalcode",
"country",
"csphone",
"tsphone",
"faxphone",
"url",
"email"
})
public class ProfileResponse {
@XmlElement(name = "MSGSETLIST", required = true)
protected MessageSetList msgsetlist;
@XmlElement(name = "SIGNONINFOLIST", required = true)
protected SignonInfoList signoninfolist;
@XmlElement(name = "DTPROFUP", required = true)
protected String dtprofup;
@XmlElement(name = "FINAME", required = true)
protected String finame;
@XmlElement(name = "ADDR1", required = true)
protected String addr1;
@XmlElement(name = "ADDR2")
protected String addr2;
@XmlElement(name = "ADDR3")
protected String addr3;
@XmlElement(name = "CITY", required = true)
protected String city;
@XmlElement(name = "STATE", required = true)
protected String state;
@XmlElement(name = "POSTALCODE", required = true)
protected String postalcode;
@XmlElement(name = "COUNTRY", required = true)
protected String country;
@XmlElement(name = "CSPHONE")
protected String csphone;
@XmlElement(name = "TSPHONE")
protected String tsphone;
@XmlElement(name = "FAXPHONE")
protected String faxphone;
@XmlElement(name = "URL")
protected String url;
@XmlElement(name = "EMAIL")
protected String email;
/**
* Gets the value of the msgsetlist property.
*
* @return
* possible object is
* {@link MessageSetList }
*
*/
public MessageSetList getMSGSETLIST() {
return msgsetlist;
}
/**
* Sets the value of the msgsetlist property.
*
* @param value
* allowed object is
* {@link MessageSetList }
*
*/
public void setMSGSETLIST(MessageSetList value) {
this.msgsetlist = value;
}
/**
* Gets the value of the signoninfolist property.
*
* @return
* possible object is
* {@link SignonInfoList }
*
*/
public SignonInfoList getSIGNONINFOLIST() {
return signoninfolist;
}
/**
* Sets the value of the signoninfolist property.
*
* @param value
* allowed object is
* {@link SignonInfoList }
*
*/
public void setSIGNONINFOLIST(SignonInfoList value) {
this.signoninfolist = value;
}
/**
* Gets the value of the dtprofup property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDTPROFUP() {
return dtprofup;
}
/**
* Sets the value of the dtprofup property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDTPROFUP(String value) {
this.dtprofup = value;
}
/**
* Gets the value of the finame property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getFINAME() {
return finame;
}
/**
* Sets the value of the finame property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setFINAME(String value) {
this.finame = value;
}
/**
* Gets the value of the addr1 property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getADDR1() {
return addr1;
}
/**
* Sets the value of the addr1 property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setADDR1(String value) {
this.addr1 = value;
}
/**
* Gets the value of the addr2 property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getADDR2() {
return addr2;
}
/**
* Sets the value of the addr2 property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setADDR2(String value) {
this.addr2 = value;
}
/**
* Gets the value of the addr3 property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getADDR3() {
return addr3;
}
/**
* Sets the value of the addr3 property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setADDR3(String value) {
this.addr3 = value;
}
/**
* Gets the value of the city property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCITY() {
return city;
}
/**
* Sets the value of the city property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCITY(String value) {
this.city = value;
}
/**
* Gets the value of the state property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getSTATE() {
return state;
}
/**
* Sets the value of the state property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setSTATE(String value) {
this.state = value;
}
/**
* Gets the value of the postalcode property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPOSTALCODE() {
return postalcode;
}
/**
* Sets the value of the postalcode property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPOSTALCODE(String value) {
this.postalcode = value;
}
/**
* Gets the value of the country property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCOUNTRY() {
return country;
}
/**
* Sets the value of the country property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCOUNTRY(String value) {
this.country = value;
}
/**
* Gets the value of the csphone property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCSPHONE() {
return csphone;
}
/**
* Sets the value of the csphone property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCSPHONE(String value) {
this.csphone = value;
}
/**
* Gets the value of the tsphone property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTSPHONE() {
return tsphone;
}
/**
* Sets the value of the tsphone property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTSPHONE(String value) {
this.tsphone = value;
}
/**
* Gets the value of the faxphone property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getFAXPHONE() {
return faxphone;
}
/**
* Sets the value of the faxphone property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setFAXPHONE(String value) {
this.faxphone = value;
}
/**
* Gets the value of the url property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getURL() {
return url;
}
/**
* Sets the value of the url property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setURL(String value) {
this.url = value;
}
/**
* Gets the value of the email property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getEMAIL() {
return email;
}
/**
* Sets the value of the email property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setEMAIL(String value) {
this.email = value;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy