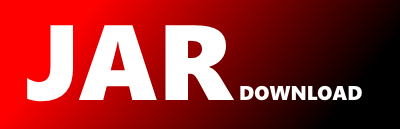
com.webcohesion.ofx4j.generated.SignupMessageSetV1 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ofx4j Show documentation
Show all versions of ofx4j Show documentation
OFX4J is a Java implementation of Open Financial Exchange, which defines web service
APIs for interfacing with financial institutions.
The newest version!
//
// This file was generated by the Eclipse Implementation of JAXB, v3.0.0
// See https://eclipse-ee4j.github.io/jaxb-ri
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2024.09.09 at 02:21:09 PM MDT
//
package com.webcohesion.ofx4j.generated;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
/**
*
* The OFX element "SIGNUPMSGSETV1" is of type "SignupMessageSetV1"
*
*
* Java class for SignupMessageSetV1 complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="SignupMessageSetV1">
* <complexContent>
* <extension base="{http://ofx.net/types/2003/04}AbstractMessageSetVersion">
* <sequence>
* <choice>
* <element name="CLIENTENROLL" type="{http://ofx.net/types/2003/04}ClientEnroll"/>
* <element name="WEBENROLL" type="{http://ofx.net/types/2003/04}WebEnroll"/>
* <element name="OTHERENROLL" type="{http://ofx.net/types/2003/04}OtherEnroll"/>
* </choice>
* <element name="CHGUSERINFO" type="{http://ofx.net/types/2003/04}BooleanType"/>
* <element name="AVAILACCTS" type="{http://ofx.net/types/2003/04}BooleanType"/>
* <element name="CLIENTACTREQ" type="{http://ofx.net/types/2003/04}BooleanType"/>
* <element name="USERINFOAVAIL" type="{http://ofx.net/types/2003/04}BooleanType" minOccurs="0"/>
* </sequence>
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "SignupMessageSetV1", propOrder = {
"clientenroll",
"webenroll",
"otherenroll",
"chguserinfo",
"availaccts",
"clientactreq",
"userinfoavail"
})
public class SignupMessageSetV1
extends AbstractMessageSetVersion
{
@XmlElement(name = "CLIENTENROLL")
protected ClientEnroll clientenroll;
@XmlElement(name = "WEBENROLL")
protected WebEnroll webenroll;
@XmlElement(name = "OTHERENROLL")
protected OtherEnroll otherenroll;
@XmlElement(name = "CHGUSERINFO", required = true)
@XmlSchemaType(name = "string")
protected BooleanType chguserinfo;
@XmlElement(name = "AVAILACCTS", required = true)
@XmlSchemaType(name = "string")
protected BooleanType availaccts;
@XmlElement(name = "CLIENTACTREQ", required = true)
@XmlSchemaType(name = "string")
protected BooleanType clientactreq;
@XmlElement(name = "USERINFOAVAIL")
@XmlSchemaType(name = "string")
protected BooleanType userinfoavail;
/**
* Gets the value of the clientenroll property.
*
* @return
* possible object is
* {@link ClientEnroll }
*
*/
public ClientEnroll getCLIENTENROLL() {
return clientenroll;
}
/**
* Sets the value of the clientenroll property.
*
* @param value
* allowed object is
* {@link ClientEnroll }
*
*/
public void setCLIENTENROLL(ClientEnroll value) {
this.clientenroll = value;
}
/**
* Gets the value of the webenroll property.
*
* @return
* possible object is
* {@link WebEnroll }
*
*/
public WebEnroll getWEBENROLL() {
return webenroll;
}
/**
* Sets the value of the webenroll property.
*
* @param value
* allowed object is
* {@link WebEnroll }
*
*/
public void setWEBENROLL(WebEnroll value) {
this.webenroll = value;
}
/**
* Gets the value of the otherenroll property.
*
* @return
* possible object is
* {@link OtherEnroll }
*
*/
public OtherEnroll getOTHERENROLL() {
return otherenroll;
}
/**
* Sets the value of the otherenroll property.
*
* @param value
* allowed object is
* {@link OtherEnroll }
*
*/
public void setOTHERENROLL(OtherEnroll value) {
this.otherenroll = value;
}
/**
* Gets the value of the chguserinfo property.
*
* @return
* possible object is
* {@link BooleanType }
*
*/
public BooleanType getCHGUSERINFO() {
return chguserinfo;
}
/**
* Sets the value of the chguserinfo property.
*
* @param value
* allowed object is
* {@link BooleanType }
*
*/
public void setCHGUSERINFO(BooleanType value) {
this.chguserinfo = value;
}
/**
* Gets the value of the availaccts property.
*
* @return
* possible object is
* {@link BooleanType }
*
*/
public BooleanType getAVAILACCTS() {
return availaccts;
}
/**
* Sets the value of the availaccts property.
*
* @param value
* allowed object is
* {@link BooleanType }
*
*/
public void setAVAILACCTS(BooleanType value) {
this.availaccts = value;
}
/**
* Gets the value of the clientactreq property.
*
* @return
* possible object is
* {@link BooleanType }
*
*/
public BooleanType getCLIENTACTREQ() {
return clientactreq;
}
/**
* Sets the value of the clientactreq property.
*
* @param value
* allowed object is
* {@link BooleanType }
*
*/
public void setCLIENTACTREQ(BooleanType value) {
this.clientactreq = value;
}
/**
* Gets the value of the userinfoavail property.
*
* @return
* possible object is
* {@link BooleanType }
*
*/
public BooleanType getUSERINFOAVAIL() {
return userinfoavail;
}
/**
* Sets the value of the userinfoavail property.
*
* @param value
* allowed object is
* {@link BooleanType }
*
*/
public void setUSERINFOAVAIL(BooleanType value) {
this.userinfoavail = value;
}
}