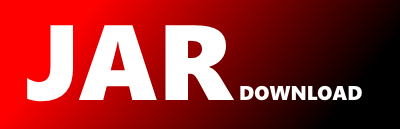
com.alee.utils.ExceptionUtils Maven / Gradle / Ivy
package com.alee.utils;
import com.alee.api.annotations.NotNull;
import java.io.PrintWriter;
import java.io.StringWriter;
/**
* This class provides a set of utilities to work with exceptions.
* Some methods in this class are based on Apache Commons library sources.
*
* @author Mikle Garin
*/
public final class ExceptionUtils
{
/**
* Private constructor to avoid instantiation.
*/
private ExceptionUtils ()
{
throw new UtilityException ( "Utility classes are not meant to be instantiated" );
}
/**
* Returns the stack trace from a Throwable as a String.
*
* The result of this method vary by JDK version as this method uses {@link Throwable#printStackTrace(java.io.PrintWriter)}.
* On JDK1.3 and earlier, the cause exception will not be shown unless the specified throwable alters printStackTrace.
*
* @param throwable the {@code Throwable} to be examined
* @return the stack trace as generated by the exception's {@code printStackTrace(PrintWriter)} method
*/
@NotNull
public static String getStackTrace ( @NotNull final Throwable throwable )
{
final StringWriter sw = new StringWriter ();
final PrintWriter pw = new PrintWriter ( sw, true );
throwable.printStackTrace ( pw );
return sw.getBuffer ().toString ();
}
/**
* Returns String representation of the specified stack trace.
*
* @param trace stack trace
* @return String representation of the specified stack trace
*/
@NotNull
public static String getStackTrace ( @NotNull final StackTraceElement[] trace )
{
final StringWriter sw = new StringWriter ();
final PrintWriter s = new PrintWriter ( sw, true );
for ( final StackTraceElement element : trace )
{
s.println ( "\tat " + element );
}
return sw.getBuffer ().toString ();
}
}