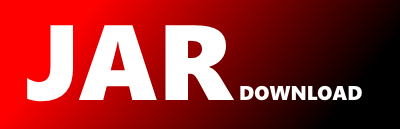
com.wedeploy.api.log.Logger Maven / Gradle / Ivy
/**
* Copyright (c) 2000-present Liferay, Inc. All rights reserved.
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* 1. Redistributions of source code must retain the above copyright notice,
* this list of conditions and the following disclaimer.
*
* 2. Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* 3. Neither the name of Liferay, Inc. nor the names of its contributors may
* be used to endorse or promote products derived from this software without
* specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*/
package com.wedeploy.api.log;
/**
* Pod logger.
*/
public interface Logger {
/**
* Logs a message at DEBUG level.
*/
public default void debug(String message) {
debug(message, NO_ARGS);
}
/**
* Logs a message at DEBUG level.
*/
public void debug(String message, Object... values);
/**
* Logs a message at ERROR level.
*/
public default void error(String message) {
error(message, NO_ARGS);
}
/**
* Logs a message at ERROR level.
*/
public void error(String message, Object... values);
/**
* Logs a message at ERROR level.
*/
public default void error(String message, Throwable throwable) {
error(message, throwable, NO_ARGS);
}
/**
* Logs a message at ERROR level.
*/
public void error(String message, Throwable throwable, Object... values);
/**
* Returns Logger name.
*/
public String getName();
/**
* Logs a message at INFO level.
*/
public default void info(String message) {
info(message, NO_ARGS);
}
/**
* Logs a message at INFO level.
*/
public void info(String message, Object... values);
/**
* Returns true
if DEBUG level is enabled.
*/
public boolean isDebugEnabled();
/**
* Returns true
if certain logging
* level is enabled.
*/
public boolean isEnabled(Level level);
/**
* Returns true
if ERROR level is enabled.
*/
public boolean isErrorEnabled();
/**
* Returns true
if INFO level is enabled.
*/
public boolean isInfoEnabled();
/**
* Returns true
if TRACE level is enabled.
*/
public boolean isTraceEnabled();
/**
* Returns true
if WARN level is enabled.
*/
public boolean isWarnEnabled();
/**
* Logs a message at provided logging level.
*/
public default void log(Level level, String message) {
log(level, message, NO_ARGS);
}
/**
* Logs a message at provided logging level.
*/
public void log(Level level, String message, Object... values);
/**
* Dynamically sets the logger level for given name.
*/
public void setLevel(String name, Level level);
/**
* Logs a message at TRACE level.
*/
public default void trace(String message) {
trace(message, NO_ARGS);
}
/**
* Logs a message at TRACE level.
*/
public void trace(String message, Object... values);
/**
* Logs a message at WARN level.
*/
public default void warn(String message) {
warn(message, NO_ARGS);
}
/**
* Logs a message at WARN level.
*/
public void warn(String message, Object... values);
/**
* Logs a message at WARN level.
*/
public default void warn(String message, Throwable throwable) {
warn(message, throwable, NO_ARGS);
}
/**
* Logs a message at WARN level.
*/
public void warn(String message, Throwable throwable, Object... values);
public Object[] NO_ARGS = new Object[0];
/**
* Logger level.
*/
public enum Level {
TRACE(1), DEBUG(2), INFO(3), WARN(4), ERROR(5);
/**
* Returns true
if this level
* is enabled for given required level.
*/
public boolean isEnabledFor(Level level) {
if (this.value >= level.value) {
return true;
}
return false;
}
private Level(int value) {
this.value = value;
}
private final int value;
}
}