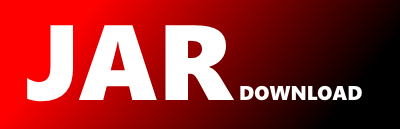
com.wedeploy.api.sdk.MultiMap Maven / Gradle / Ivy
/**
* Copyright (c) 2000-present Liferay, Inc. All rights reserved.
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* 1. Redistributions of source code must retain the above copyright notice,
* this list of conditions and the following disclaimer.
*
* 2. Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* 3. Neither the name of Liferay, Inc. nor the names of its contributors may
* be used to endorse or promote products derived from this software without
* specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*/
package com.wedeploy.api.sdk;
import java.util.List;
import java.util.Map;
import java.util.Set;
/**
* Multi-map.
*/
public interface MultiMap extends Iterable> {
/**
* Creates new case-sensitive implementation of the multi-map.
*/
public static MultiMap newCaseSensitiveMultiMap() {
return MultiMapFactory.Default.factory.createMultiMap(true);
}
/**
* Creates new default implementation of the multi-map.
*/
public static MultiMap newMultiMap() {
return MultiMapFactory.Default.factory.createMultiMap(false);
}
/**
* Adds a new value with the specified name and value.
*
* @param name The name
* @param value The value being added
* @return a reference to this, so the API can be used fluently
*/
public MultiMap add(String name, V value);
/**
* Adds all values from the map.
*
* @param map source map
* @return a reference to this, so the API can be used fluently
*/
public MultiMap addAll(Map map);
/**
* Adds new values under the specified name.
*
* @param name The name being set
* @param values The values
* @return a reference to this, so the API can be used fluently
*/
public MultiMap addAll(String name, Iterable values);
/**
* Removes all values.
*
* @return a reference to this, so the API can be used fluently
*/
public MultiMap clear();
/**
* Checks to see if there is a value with the specified name.
*
* @param name The name to search for
* @return true if at least one entry is found
*/
public boolean contains(String name);
/**
* Returns all entries in the multi-map.
*
* @return A immutable {@link List} of the name-value entries, which will be
* empty if no pairs are found
*/
public List> entries();
/**
* Get the String value associated with a key.
*/
public V get(String name);
/**
* Returns the values with the specified name.
*
* @param name The name to search
* @return A immutable {@link List} of values which will be empty
* if no values are found
*/
public List getAll(String name);
/**
* Returns true
if this map is case sensitive.
*/
public boolean isCaseSensitive();
/**
* Returns true
if empty.
*/
public boolean isEmpty();
/**
* Gets a immutable {@link Set} of all names
*
* @return A {@link Set} of all names
*/
public Set names();
/**
* Removes the value with the given name
*
* @param name The name of the value to remove
* @return a reference to this, so the API can be used fluently
*/
public MultiMap remove(String name);
/**
* Sets a value under the specified name.
*
* If there is an existing key with the same name, it is removed.
*
* @param name The name
* @param value The value
* @return a reference to this, so the API can be used fluently
*/
public MultiMap set(String name, V value);
/**
* Sets values from given map.
*
* @param map The source map
* @return a reference to this, so the API can be used fluently
*/
public MultiMap setAll(Map map);
/**
* Sets values for the specified name.
*
* @param name The name being set
* @param values The values being set
* @return a reference to this, so the API can be used fluently
*/
public MultiMap setAll(String name, Iterable values);
/**
* Return the number of keys.
*/
public int size();
}