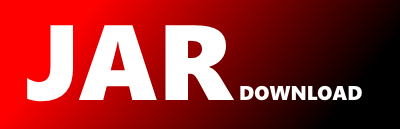
com.wedeploy.api.sdk.Request Maven / Gradle / Ivy
/**
* Copyright (c) 2000-present Liferay, Inc. All rights reserved.
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* 1. Redistributions of source code must retain the above copyright notice,
* this list of conditions and the following disclaimer.
*
* 2. Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* 3. Neither the name of Liferay, Inc. nor the names of its contributors may
* be used to endorse or promote products derived from this software without
* specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*/
package com.wedeploy.api.sdk;
import java.util.List;
import java.util.Map;
/**
* HTTP request.
*/
public interface Request {
/**
* Returns the auth.
*/
public Auth auth();
/**
* Returns the base URL corresponding to the the HTTP request.
* If null
the request represents a 'local' call,
* with only a {@link #path()} existing.
*/
public String baseUrl();
/**
* Returns the body content as a string.
*/
public String body();
/**
* Sets the raw body content.
*/
public Request body(byte[] body);
/**
* Sets the body content, assuming JSON content type.
*/
public Request body(Object body);
/**
* Sets the body content by passing the string value.
*/
public Request body(String body);
/**
* Sets the raw body content and the {@link ContentType content-type}.
* This is just a shortcut method.
*/
public Request body(String body, ContentType contentType);
/**
* Returns the raw body content.
*/
public byte[] bodyBytes();
/**
* Returns parsed {@link #body() body content} as a List.
*/
public List bodyList(Class componentType);
/**
* Returns parsed {@link #body() body content}.
* If body is not set, returns {@code null}.
*/
public Map bodyMap(Class keyType, Class valueType);
/**
* REturns parsed {@link #body() body content}.
* If body is not set, returns {@code null}.
*/
public default Map bodyMap(Class valueType) {
return bodyMap(String.class, valueType);
}
/**
* Parses the body depending on content-type. If content-type is NOT set,
* it will use assume the "plain/text" content type.
* Returns parsed {@link #body() body content}.
* If body is not set, returns null
.
* If body can not be parsed, throws an Exception.
*/
public T bodyValue();
/**
* Parses the body depending on content-type into the target type.
*
* @see #bodyValue()
*/
public T bodyValue(Class type);
/**
* Gets the content type header.
*/
public String contentType();
/**
* Sets the content type header.
*/
public Request contentType(ContentType contentType);
/**
* Returns the context that this request belongs to.
*/
public Context context();
/**
* @return A map of all the cookies.
*/
Map cookies();
/**
* Adds a cookie to the response.
*/
public Request cookie(Cookie cookie);
/**
* Gets cookie value by name.
*/
public Cookie cookie(String name);
/**
* Returns array of uploaded files. Returns null
if nothing
* was uploaded.
*/
public FileUpload[] fileUploads();
/**
* Returns the form parameter.
*/
public Object form(String name);
/**
* Sets the form parameter.
*/
public Request form(String name, Object value);
/**
* Returns form parameters.
*/
public MultiMap