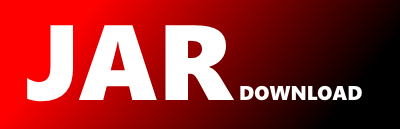
com.wedeploy.api.sdk.ResponseError Maven / Gradle / Ivy
/**
* Copyright (c) 2000-present Liferay, Inc. All rights reserved.
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* 1. Redistributions of source code must retain the above copyright notice,
* this list of conditions and the following disclaimer.
*
* 2. Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* 3. Neither the name of Liferay, Inc. nor the names of its contributors may
* be used to endorse or promote products derived from this software without
* specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*/
package com.wedeploy.api.sdk;
/**
* Main class for building response errors.
*/
public class ResponseError {
/**
* Creates error 400 response.
*/
public static Error400 badRequest() {
return badRequest(null);
}
/**
* Creates error 400 response.
*/
public static Error400 badRequest(String message) {
return new Error400<>(newResponseErrorData(), message);
}
/**
* Creates error 409 response.
*/
public static Error409 conflict() {
return conflict(null);
}
/**
* Creates error 409 response.
*/
public static Error409 conflict(String message) {
return new Error409<>(newResponseErrorData(), message);
}
/**
* Creates error 403 response.
*/
public static Error403 forbidden() {
return forbidden(null);
}
/**
* Creates error 403 response.
*/
public static Error403 forbidden(String message) {
return new Error403<>(newResponseErrorData(), message);
}
/**
* Creates error 500 response.
*/
public static Error500 internalError() {
return internalError(null);
}
/**
* Creates error 500 response.
*/
public static Error500 internalError(String message) {
return new Error500<>(newResponseErrorData(), message);
}
/**
* Creates error 405 response.
*/
public static Error405 methodNotAllowed() {
return methodNotAllowed(null);
}
/**
* Creates error 405 response.
*/
public static Error405 methodNotAllowed(String message) {
return new Error405<>(newResponseErrorData(), message);
}
/**
* Creates error 404 response.
*/
public static Error404 notFound() {
return notFound(null);
}
/**
* Creates error 404 response.
*/
public static Error404 notFound(String message) {
return new Error404<>(newResponseErrorData(), message);
}
/**
* Creates error 408 response.
*/
public static Error408 requestTimeout() {
return requestTimeout(null);
}
/**
* Creates error 408 response.
*/
public static Error408 requestTimeout(String message) {
return new Error408<>(newResponseErrorData(), message);
}
/**
* Created error 503 response.
*/
public static Error503 serviceUnavailable() {
return serviceUnavailable(null);
}
/**
* Created error 503 response.
*/
public static Error503 serviceUnavailable(String message) {
return new Error503<>(newResponseErrorData(), message);
}
/**
* Created error 404 response.
*/
public static Error401 unauthorized() {
return unauthorized(null);
}
/**
* Created error 404 response.
*/
public static Error401 unauthorized(String message) {
return new Error401<>(newResponseErrorData(), message);
}
protected ResponseError() {
}
private static ErrorData newResponseErrorData() {
return new ErrorData() {
@Override
protected Response into(Response response) {
response.status(statusCode(), statusMessage());
headers(response::header);
String errorBody = errorBody();
return response.contentType(ContentType.JSON).body(errorBody);
}
};
}
}