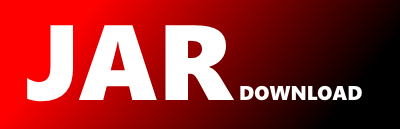
com.wedeploy.api.sdk.ResponseWrapper Maven / Gradle / Ivy
/**
* Copyright (c) 2000-present Liferay, Inc. All rights reserved.
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* 1. Redistributions of source code must retain the above copyright notice,
* this list of conditions and the following disclaimer.
*
* 2. Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* 3. Neither the name of Liferay, Inc. nor the names of its contributors may
* be used to endorse or promote products derived from this software without
* specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*/
package com.wedeploy.api.sdk;
import java.util.List;
import java.util.Map;
/**
* Response wrapper.
*/
public class ResponseWrapper implements Response {
public ResponseWrapper(Response response) {
this.response = response;
}
@Override
public String body() {
return response.body();
}
@Override
public Response body(byte[] body) {
return response.body(body);
}
@Override
public Response body(Object body) {
return response.body(body);
}
@Override
public Response body(String body) {
return response.body(body);
}
@Override
public Response body(String body, ContentType contentType) {
return response.body(body, contentType);
}
@Override
public byte[] bodyBytes() {
return response.bodyBytes();
}
@Override
public List bodyList(Class componentType) {
return response.bodyList(componentType);
}
@Override
public Map bodyMap(Class keyType, Class valueType) {
return response.bodyMap(keyType, valueType);
}
@Override
public Map bodyMap(Class valueType) {
return response.bodyMap(valueType);
}
@Override
public T bodyValue() {
return response.bodyValue();
}
@Override
public T bodyValue(Class type) {
return response.bodyValue(type);
}
@Override
public String contentType() {
return response.contentType();
}
@Override
public Response contentType(ContentType contentType) {
return response.contentType(contentType);
}
@Override
public Context context() {
return response.context();
}
@Override
public Response cookie(Cookie cookie) {
return response.cookie(cookie);
}
@Override
public Cookie cookie(String name) {
return response.cookie(name);
}
@Override
public Map cookies() {
return response.cookies();
}
@Override
public void end() {
response.end();
}
@Override
public void end(Object body) {
response.end(body);
}
@Override
public void end(String body) {
response.end(body);
}
@Override
public void end(String body, ContentType contentType) {
response.end(body, contentType);
}
@Override
public String header(String name) {
return response.header(name);
}
@Override
public Response header(String name, String value) {
return response.header(name, value);
}
@Override
public MultiMap headers() {
return response.headers();
}
@Override
public boolean isCommitted() {
return response.isCommitted();
}
@Override
public boolean isContentType(ContentType contentType) {
return response.isContentType(contentType);
}
@Override
public Response pipe(Response response) {
return this.response.pipe(response);
}
@Override
public void redirect(String url) {
response.redirect(url);
}
@Override
public Request request() {
return response.request();
}
@Override
public Session session() {
return response.session();
}
@Override
public Response status(int statusCode) {
return response.status(statusCode);
}
@Override
public Response status(int statusCode, String statusMessage) {
return response.status(statusCode, statusMessage);
}
@Override
public int statusCode() {
return response.statusCode();
}
@Override
public String statusMessage() {
return response.statusMessage();
}
@Override
public boolean succeeded() {
return response.succeeded();
}
protected final Response response;
}