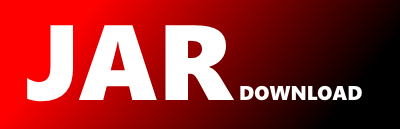
com.welemski.gobblerparty.PoolPartyConcept Maven / Gradle / Ivy
/*
* The MIT License
*
* Copyright 2016 welemski.
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
package com.welemski.gobblerparty;
import com.welemski.gobblerparty.transformer.DefaultSPARQLFieldFilter;
import com.welemski.gobblerparty.transformer.SPARQLXMLConceptFilter;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
*
* @author welemski
*/
public class PoolPartyConcept {
private String uri;
private String prefLabel;
private String broader;
private List narrowers = new ArrayList();
private List conceptSchemes = new ArrayList();
private List inSchemes = new ArrayList();
private List topConceptOf = new ArrayList();
/**
*
* The properties returned from PoolParty JSON object using PoolParty's standard API is stored as a key-value pair.
*
*
* All other properties returned from using SPARQL end points are stored inside a key named "attributes". See {@link DefaultSPARQLFieldFilter DefaultSPARQLFieldFilter} class.
*
*
* To control what fields to store into what key, use {@link SPARQLXMLConceptFilter SPARQLXMLConceptFilter} class and fine tune the field to filter.
*
*/
private HashMap properties = new HashMap();
private List children = new ArrayList();
public void setProperty(String name, Object value){
this.properties.put(name, value);
}
public Object getProperty(String key){
return this.properties.get(key);
}
public String getPropertyString(String key){
Object obj = this.properties.get(key);
if(obj instanceof String){
return (String)obj;
}
return null;
}
public String getPropertyString(String key, String defaultValue){
Object obj = this.properties.get(key);
if(obj instanceof String){
return (String)obj;
}
return defaultValue;
}
public List getPropertyList(String key){
Object obj = this.properties.get(key);
if(obj instanceof List){
return (List)obj;
}
return new ArrayList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy