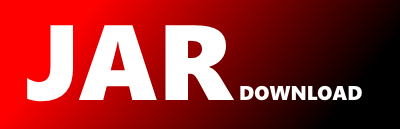
com.welemski.wrench.poi.util.DefaultSpreadsheetQuery Maven / Gradle / Ivy
/*
* The MIT License
*
* Copyright 2016 welemski.
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
package com.welemski.wrench.poi.util;
import com.welemski.wrench.delegate.Delegable;
import java.util.ArrayList;
import java.util.List;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.ss.util.CellReference;
/**
*
* @author Lemuel Raganas
*/
public class DefaultSpreadsheetQuery extends Delegable implements SpreadsheetQuery{
private int limit = 0;
private int atRowIndex = 0;
private final String kCondition = "condition";
private final String kStructure = "structure";
private Workbook workbook;
private String sheetName;
private int sheetIndex = 0;
public DefaultSpreadsheetQuery(){}
public DefaultSpreadsheetQuery(Workbook workbook){
this.workbook = workbook;
}
protected List findAll(Workbook workbook,String sheetName, int sheetIndex, int limit, int atRowIndex ){
List rows = new ArrayList<>();
Sheet sheet = null;
if(workbook == null){
return new ArrayList<>();
}
sheet = getSheet(workbook, sheetName, sheetIndex);
if(sheet == null){
return new ArrayList<>();
}
rows = getAllRows(sheet, limit, atRowIndex);
return rows;
}
protected List getAllRows(Sheet sheet, int limit, int atRowIndex){
List rows = new ArrayList<>();
if( atRowIndex > 0){
if ((atRowIndex + 1 ) > sheet.getPhysicalNumberOfRows()) {
return rows;
}
}
for(int i = atRowIndex ; i 0 && rows.size() >= limit ) {
return rows;
}
Row row = sheet.getRow(i);
SpreadsheetRow sr = new SpreadsheetRow(row);
boolean skip = false;
if(this.hasDelegate(kCondition)){
skip = !((SpreadsheetQueryCondition)this.getDelegate(kCondition)).where(this, sr);
}
if(!skip){
rows.add(sr);
}
}
return rows;
}
@Override
public SpreadsheetQuery where(SpreadsheetQueryCondition condition) {
this.addDelegate(kCondition, condition);
return this;
}
@Override
public SpreadsheetQuery from(String sheetName) {
this.sheetName = sheetName;
return this;
}
@Override
public SpreadsheetQuery from(int sheetIndex) {
this.sheetIndex = sheetIndex;
return this;
}
@Override
public List findAll() {
return this.findAll(workbook, sheetName, sheetIndex,limit,atRowIndex);
}
@Override
public List findAll(int limit) {
this.limit = limit;
return this.findAll(workbook, sheetName, sheetIndex,this.limit,atRowIndex);
}
@Override
public SpreadsheetRow get() {
List list = this.findAll(1);
if(list.isEmpty()){
return null;
}
return list.get(0);
}
@Override
public SpreadsheetRow get(int atIndex) {
this.atRowIndex = atIndex;
Sheet sheet = getSheet(workbook,sheetName,sheetIndex);
if(sheet == null){
return null;
}
if(sheet.getPhysicalNumberOfRows() <= atRowIndex ){
return null;
}
Row row = sheet.getRow(atRowIndex);
SpreadsheetRow sr = new SpreadsheetRow(row);
return sr;
}
@Override
public int count() {
List findAll = this.findAll();
return findAll.size();
}
@Override
public SpreadsheetQuery setLimit(int limit) {
this.limit = limit;
return this;
}
@Override
public SpreadsheetQuery atRow(int rowIndex) {
this.atRowIndex = rowIndex;
return this;
}
@Override
public Workbook getWorkbook() {
return this.workbook;
}
@Override
public SpreadsheetCell getCell(String cellAddress) {
CellReference cellRef = new CellReference(cellAddress);
Sheet sheet = this.getSheet(workbook, sheetName, sheetIndex);
if(sheet == null){
// Incase no sheet was specified and sheet reference exists in the cell address
sheet = this.getSheet(workbook, cellRef.getSheetName(), sheetIndex);
}
if(sheet == null){
return null;
}
Row row = sheet.getRow(cellRef.getRow());
if(row == null){
return null;
}
Cell cell = row.getCell(cellRef.getCol());
if(cell == null){
return null;
}
return new SpreadsheetCell(cell);
}
protected Sheet getSheet(Workbook workbook, String sheetName, int sheetIndex) {
Sheet sheet = null;
if(workbook == null){
return null;
}
if(sheetName != null && !"".equals(sheetName)){
sheet = workbook.getSheet(sheetName);
}
if(sheet == null){
sheet = workbook.getSheetAt(sheetIndex);
}
return sheet;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy