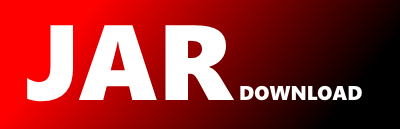
com.welemski.wrench.poi.util.SpreadsheetWriter Maven / Gradle / Ivy
/*
* The MIT License
*
* Copyright 2016 Lemuel Raganas .
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
package com.welemski.wrench.poi.util;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.function.BiConsumer;
import org.apache.commons.io.FilenameUtils;
import org.apache.poi.hssf.usermodel.HSSFWorkbook;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.ss.util.CellReference;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
/**
*
* @author Lemuel Raganas
*/
public class SpreadsheetWriter implements SheetWriter{
private Workbook workbook;
private Sheet currentSheet;
private File file;
public SpreadsheetWriter(File file){
this.workbook = Spreadsheet.getWorkbook(file);
this.file = file;
if(this.workbook == null){
String xt = FilenameUtils.getExtension(file.getName());
if(xt.equalsIgnoreCase("xls")){
this.workbook = new HSSFWorkbook();
}else if(xt.equalsIgnoreCase("xlsx")){
this.workbook = new XSSFWorkbook();
}
}
}
public SpreadsheetWriter(Workbook workbook){
this.workbook = workbook;
}
/**
* Specify the sheet to manipulate and if the sheet does not exists, will auto create.
*
* Always call this method before performing writing to rows
*
* @param sheetName
* @return SpreadsheetWriter
*/
@Override
public SpreadsheetWriter useSheet(String sheetName){
this.currentSheet = this.workbook.getSheet(sheetName);
if(this.currentSheet == null){
this.currentSheet = this.workbook.createSheet(sheetName);
}
return null;
}
@Override
public SpreadsheetWriter setCell(String cellAddress, Object data){
return this.setCell(cellAddress, data, null);
}
@Override
public SpreadsheetWriter setCell(String cellAddress, Object data, BiConsumer
© 2015 - 2025 Weber Informatics LLC | Privacy Policy