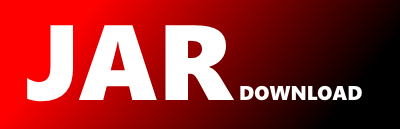
com.wepay.riff.util.RepeatingTask Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of riff-networking Show documentation
Show all versions of riff-networking Show documentation
Riff is a networking library built based on Netty.
package com.wepay.riff.util;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.atomic.AtomicReference;
public abstract class RepeatingTask {
private enum TaskState {
NEW, RUNNING, STOPPED
}
private AtomicReference state = new AtomicReference<>(TaskState.NEW);
private final Thread thread;
private final CompletableFuture shutdownFuture = new CompletableFuture<>();
protected RepeatingTask(String taskName) {
this.thread = new Thread(() -> {
if (isRunning()) {
try {
init();
while (isRunning()) {
try {
task();
} catch (InterruptedException ex) {
interrupted(ex);
} catch (Throwable ex) {
exceptionCaught(ex);
}
}
} catch (Throwable ex) {
stop();
exceptionCaught(ex);
}
}
shutdownFuture.complete(true);
});
this.thread.setName(this.thread.getName() + "-" + taskName);
this.thread.setDaemon(true);
}
public void start() {
if (state.compareAndSet(TaskState.NEW, TaskState.RUNNING)) {
thread.start();
} else {
throw new IllegalStateException("task already started");
}
}
public CompletableFuture stop() {
if (state.compareAndSet(TaskState.NEW, TaskState.STOPPED) || state.compareAndSet(TaskState.RUNNING, TaskState.STOPPED)) {
shutdownFuture.complete(true);
}
return shutdownFuture;
}
public boolean isRunning() {
return state.get() == TaskState.RUNNING;
}
protected void init() throws Exception {
}
protected abstract void task() throws Exception;
protected void interrupted(InterruptedException ex) {
Thread.interrupted();
}
protected abstract void exceptionCaught(Throwable ex);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy