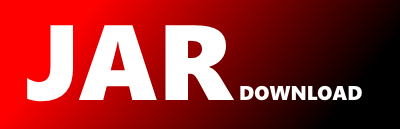
com.wesleyhome.dao.processor.EntityInfoProcessor Maven / Gradle / Ivy
package com.wesleyhome.dao.processor;
import java.util.List;
import javax.lang.model.element.Element;
import javax.lang.model.element.TypeElement;
import javax.persistence.EmbeddedId;
import javax.persistence.Id;
import com.wesleyhome.dao.annotations.Scope;
import com.wesleyhome.dao.annotations.ScopeType;
import com.wesleyhome.dao.annotations.SubPackage;
import com.wesleyhome.dao.processor.model.CustomQueryInfo;
import com.wesleyhome.dao.processor.model.EntityInfo;
import com.wesleyhome.dao.processor.model.NamedQueryInformation;
import com.wesleyhome.dao.processor.model.QueryParameterInfo;
public class EntityInfoProcessor {
protected AnnotationHelper annotationHelper;
public EntityInfoProcessor() {
annotationHelper = new AnnotationHelperImpl();
}
public EntityInfo getEntityInfo(final TypeElement entityElement) {
String fullClassName = getFullName(entityElement);
String entityName = getEntityName(entityElement);
ScopeType scopeType = getScope(entityElement);
List customQueryInfoList = getCustomQueryInfo(entityElement);
List queryParameterInfoList = getQueryParameterInfo(entityElement);
String subPackageName = getSubPackageName(entityElement);
List idElements = getIdElements(entityElement);
List namedQueryInfoList = getNamedQueryInfoList(entityElement);
return new EntityInfo(entityElement, subPackageName, fullClassName, entityName, scopeType, customQueryInfoList,
queryParameterInfoList, idElements, namedQueryInfoList);
}
private List getNamedQueryInfoList(final TypeElement entityElement) {
return new NamedQueryInfoListProcessor().process(entityElement, annotationHelper);
}
/**
* @param entityElement
* @return
*/
private List getIdElements(final TypeElement entityElement) {
List annotatedElements = annotationHelper.getAnnotatedElements(entityElement, Id.class);
if (annotatedElements.isEmpty()) {
List embeddedElementList = annotationHelper.getAnnotatedElements(entityElement, EmbeddedId.class);
if (!embeddedElementList.isEmpty()) {
annotatedElements.add(embeddedElementList.get(0));
}
}
return annotatedElements;
}
/**
* @param entityElement
* @return
*/
private ScopeType getScope(final TypeElement entityElement) {
Object scopeType = annotationHelper.getAnnotationValue(entityElement, Scope.class, "value");
return scopeType == null ? ScopeType.NONE : ScopeType.valueOf(scopeType.toString());
}
/**
* @param entityElement
* @return
*/
private String getFullName(final TypeElement entityElement) {
return entityElement.getQualifiedName().toString();
}
/**
* @param entityElement
* @return
*/
private String getEntityName(final TypeElement entityElement) {
return entityElement.getSimpleName().toString();
}
/**
* @param entityElement
* @return
*/
private String getSubPackageName(final TypeElement entityElement) {
Object subPackageValue = annotationHelper.getAnnotationValue(entityElement, SubPackage.class, "value");
return subPackageValue == null ? "" : "." + subPackageValue.toString();
}
/**
* @param entityElement
* @return
*/
private List getCustomQueryInfo(final TypeElement entityElement) {
return new CustomQueryInfoListProcessor().process(entityElement, annotationHelper);
}
/**
* @param entityElement
* @return
*/
private List getQueryParameterInfo(final TypeElement entityElement) {
return new QueryParameterInfoListProcessor().process(entityElement, annotationHelper);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy