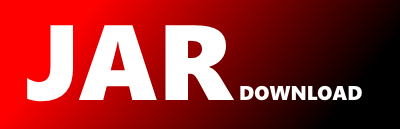
com.wesleyhome.test.jupiter.ReflectionHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of junit-jupiter-params-generated Show documentation
Show all versions of junit-jupiter-params-generated Show documentation
Library to help generate test parameter permutations for parameterized tests in JUnit.
This version is an initial attempt to convert to building with Gradle.
package com.wesleyhome.test.jupiter;
import java.lang.reflect.AccessibleObject;
import java.lang.reflect.Constructor;
import java.lang.reflect.InvocationTargetException;
import java.util.Arrays;
import java.util.function.Function;
import java.util.stream.Stream;
@SuppressWarnings("unchecked")
public class ReflectionHelper {
public static Stream> getConstructorStream(Class> providerClass) {
return getAOStream(providerClass, Class::getDeclaredConstructors, Class::getConstructors);
}
private static Stream getAOStream(
Class> cls, Function, T[]> function1,
Function, T[]>... otherFunctions
) {
return StreamHelper.stream(function1, otherFunctions)
.map(f -> f.apply(cls))
.map(Stream::of)
.reduce(Stream::concat)
.orElseGet(Stream::empty);
}
private static R _invoke(T accessibleObject, Function function) {
boolean accessible = accessibleObject.isAccessible();
if (!accessible) {
accessibleObject.setAccessible(true);
}
try {
return function.apply(accessibleObject);
} finally {
accessibleObject.setAccessible(accessible);
}
}
public static T invokeConstructor(Class cls, Object... parameter) {
try {
Class>[] parameterTypes = Stream.of(parameter)
.map(Object::getClass)
.toArray(Class>[]::new);
Constructor constructor = (Constructor) getConstructorStream(cls)
.filter(c -> Arrays.equals(parameterTypes, c.getParameterTypes()))
.findFirst()
.orElseThrow(() -> new NoSuchMethodException("Unable to find matching constructor"));
return _invoke(constructor, c1 -> {
try {
return c1.newInstance(parameter);
} catch (InstantiationException | IllegalAccessException | InvocationTargetException e) {
throw new RuntimeException(e);
}
});
} catch (NoSuchMethodException e) {
throw new RuntimeException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy