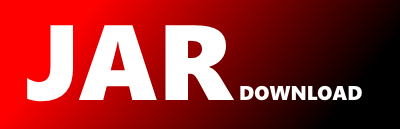
com.whaleal.icefrog.poi.excel.reader.MapSheetReader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of icefrog-poi Show documentation
Show all versions of icefrog-poi Show documentation
icefrog POI工具类(对MS Office操作)
The newest version!
package com.whaleal.icefrog.poi.excel.reader;
import com.whaleal.icefrog.core.collection.CollUtil;
import com.whaleal.icefrog.core.collection.IterUtil;
import com.whaleal.icefrog.core.collection.ListUtil;
import com.whaleal.icefrog.core.util.StrUtil;
import org.apache.poi.ss.usermodel.Sheet;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
/**
* 读取{@link Sheet}为Map的List列表形式
*
* @author Looly
* @author wh
* @since 1.0.0
*/
public class MapSheetReader extends AbstractSheetReader>> {
private final int headerRowIndex;
/**
* 构造
*
* @param headerRowIndex 标题所在行,如果标题行在读取的内容行中间,这行做为数据将忽略
* @param startRowIndex 起始行(包含,从0开始计数)
* @param endRowIndex 结束行(包含,从0开始计数)
*/
public MapSheetReader( int headerRowIndex, int startRowIndex, int endRowIndex ) {
super(startRowIndex, endRowIndex);
this.headerRowIndex = headerRowIndex;
}
@Override
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy