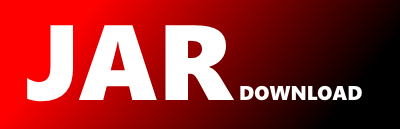
com.wichell.framework.interceptor.KafKaSendAdvice Maven / Gradle / Ivy
package com.wichell.framework.interceptor;
import static com.wichell.framework.ecache.EcacheUtil.cache;
import static com.wichell.framework.ecache.EcacheUtil.del;
import static com.wichell.framework.ecache.EcacheUtil.get;
import java.util.List;
import java.util.Map;
import java.util.Properties;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.CopyOnWriteArrayList;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.LinkedBlockingQueue;
import java.util.concurrent.ThreadPoolExecutor;
import java.util.concurrent.TimeUnit;
import org.apache.log4j.LogManager;
import org.apache.log4j.Logger;
import com.wichell.framework.bean.MsgBean;
import com.wichell.framework.util.JacksonUtil;
import kafka.javaapi.producer.Producer;
import kafka.producer.KeyedMessage;
import kafka.producer.ProducerConfig;
public class KafKaSendAdvice implements SendAdvice {
private Properties props;
private Producer producer;
private final static String CACHE_KEY = "kafkalog";
private final static String CACHE_NAME = "kafkaCache";
private ThreadPoolExecutor pool = new ThreadPoolExecutor(1, 1, 60L, TimeUnit.SECONDS,
new LinkedBlockingQueue());
private Map count = new ConcurrentHashMap<>();
private ExecutorService cachedThreadPool = Executors.newCachedThreadPool();
private static Logger logger = LogManager.getLogger(KafKaSendAdvice.class);
public void setProps(Properties props) {
this.props = props;
}
public void init() {
if (producer == null) {
producer = new Producer(new ProducerConfig(props));
}
if (pool.getPoolSize() == 0) {
pool.execute(new kafkaRunable());
}
}
@SuppressWarnings({ "rawtypes" })
public synchronized void addMessage(MsgBean kafka) {
KeyedMessage msg = null;
try {
msg = new KeyedMessage(kafka.getTopic(), JacksonUtil.obj2Json(kafka));
cachedThreadPool.execute(new kafkaSendRunable(msg));
} catch (Exception e) {
e.printStackTrace();
}
}
@SuppressWarnings("unchecked")
public synchronized void send() {
Object obj = get(CACHE_NAME, CACHE_KEY);
if (obj != null) {
List> list = (List>) obj;
producer.send(list);
del(CACHE_NAME, CACHE_KEY);
}
}
class kafkaRunable extends Thread {
@SuppressWarnings("static-access")
@Override
public void run() {
long heartMs = Long.parseLong((String) props.get("heart.ms"));
while (true) {
try {
send();
this.sleep(heartMs);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
@SuppressWarnings("unchecked")
class kafkaSendRunable extends Thread {
@SuppressWarnings("rawtypes")
private KeyedMessage msg;
@SuppressWarnings("rawtypes")
public kafkaSendRunable(KeyedMessage msg) {
super();
this.msg = msg;
}
@Override
public void run() {
try {
producer.send(msg);
} catch (Exception e) {
logger.error("=============================message not operated" + msg);
e.printStackTrace();
List> list = null;
Object obj = get(CACHE_NAME, CACHE_KEY);
if (obj == null) {
list = new CopyOnWriteArrayList>();
} else {
list = (List>) obj;
}
if (count.containsKey(msg.hashCode())) {
Integer maxCount = count.get(msg.hashCode());
if (maxCount >= 3) {
count.remove(msg.hashCode());
list.remove(msg);
cache(CACHE_NAME, CACHE_KEY, list);
} else {
count.put(msg.hashCode(), maxCount + 1);
}
} else {
count.put(msg.hashCode(), 1);
list.add(msg);
cache(CACHE_NAME, CACHE_KEY, list);
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy