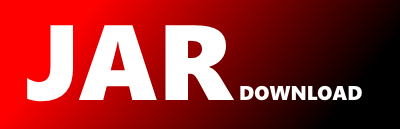
com.wichell.framework.proxy.mybatis.MybatisProxyFactory Maven / Gradle / Ivy
package com.wichell.framework.proxy.mybatis;
import java.util.Collection;
import java.util.List;
import org.apache.ibatis.reflection.ReflectionException;
import org.apache.ibatis.reflection.factory.DefaultObjectFactory;
import com.wichell.framework.proxy.ProxyFactory;
@SuppressWarnings("serial")
public abstract class MybatisProxyFactory extends DefaultObjectFactory implements IMybatisProxyFactory {
private static ProxyFactory proxyFactory;
@Override
public T create(Class type) {
return create(type, null, null);
}
@SuppressWarnings("static-access")
public MybatisProxyFactory() {
this.proxyFactory = new ProxyFactory(init());
}
@Override
public T create(Class type, List> constructorArgTypes, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy