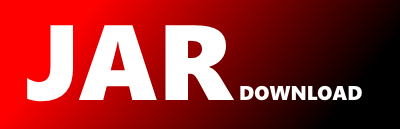
com.windpicker.commons.web.utils.Rslt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of commons Show documentation
Show all versions of commons Show documentation
This project is intended to provide common classes and utils to develop java apps
package com.windpicker.commons.web.utils;
import com.windpicker.commons.web.Result;
/**
* Helper class for {@link com.windpicker.commons.web.Result Result}
* @author Angus Xiao
* @version
*
* - 1.0 (Nov 26, 2015 12:21:04 PM)
* - initiate the class: add getResult functions of SUCCESS, ERROR, EXCEPTION, LOGIN. add isSuccess function
*
*/
public class Rslt {
public static final int SUCCESS = 0;
public static final int ERROR = 1;
public static final int LOGIN = 2;
public static final int EXCEPTION = 3;
/**
* Generate a success result entity with the given resposne object
* @param response object to return (T will have its type)
* @return a success result entity with the given resposne object as the r property
*/
public static Result getSuccessResult(T response){
Result result = new Result();
result.setC(SUCCESS);
result.setR(response);
return result;
}
/**
* Generate an error result entity with the given information String
* @param information error message
* @return an error result entity with the given information String as the i property
*/
public static Result getErrorResult(String information){
Result result = new Result();
result.setC(ERROR);
result.setI(information);
return result;
}
/**
* Generate an exception result entity with the given exception message String
* @param exceptionMessage exception message (nullable)
* @return an exception result entity with the given exception message String as the i property
*/
public static Result getExceptionResult(String exceptionMessage){
Result result = new Result();
result.setC(EXCEPTION);
result.setI(exceptionMessage);
return result;
}
/**
* Generate an login result entity
* @return an login result entity with "LOGIN_NEEDED" as the i property
*/
public static Result getLoginResult(){
Result result = new Result();
result.setC(LOGIN);
result.setI("LOGIN_NEEDED");
return result;
}
/**
* Judge whether the given result is a success entity
* @param result the given result entity
* @return true only if result is not null and its code is SUCCESS
*/
public static boolean isSuccess(Result> result){
return result == null ? false : result.getC()==SUCCESS;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy